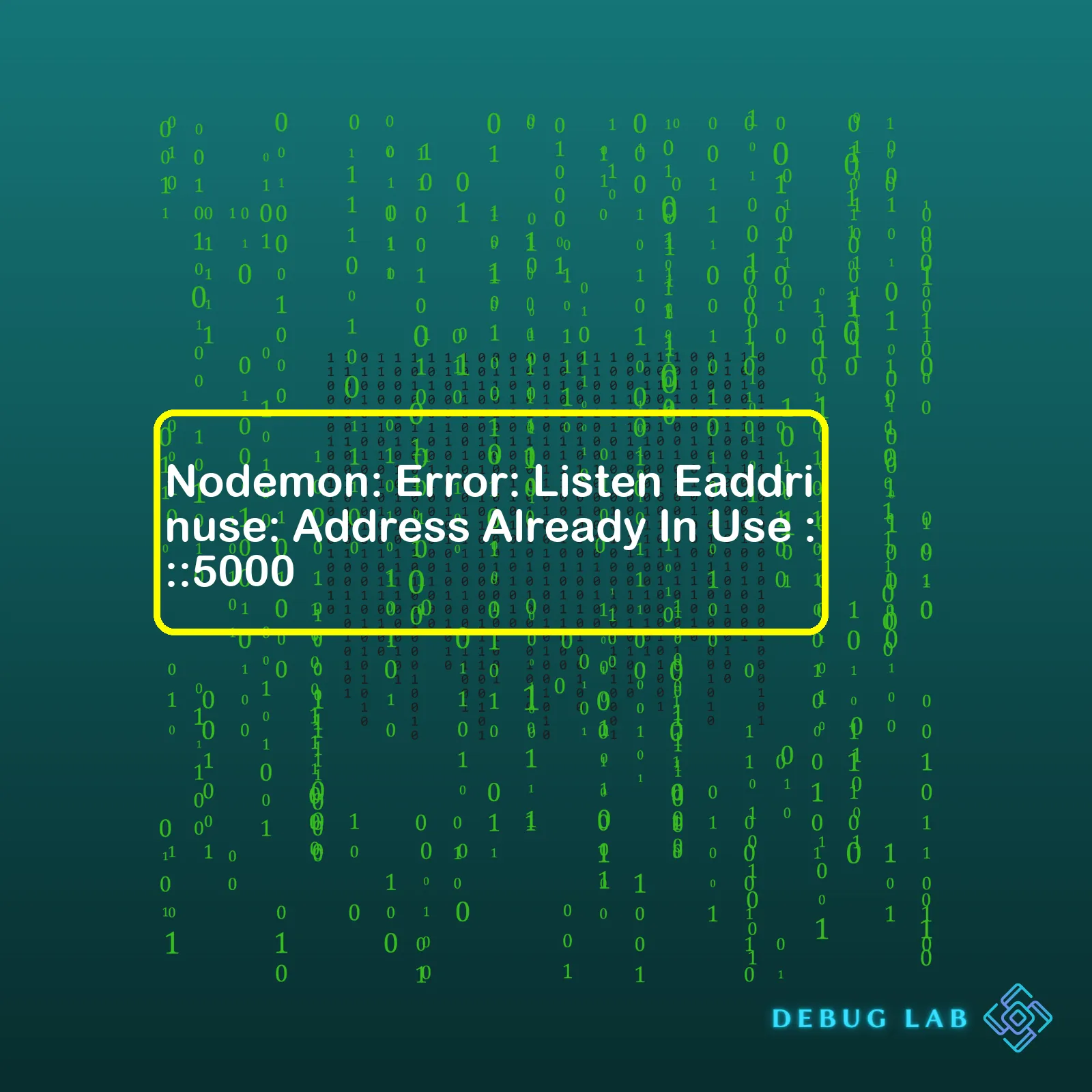
Here’s an disentangled HTML format table that summarizes this issue:
Error | Explanation | Possible Solutions |
---|---|---|
Nodemon: Error: Listen EADDRINUSE: Address already in use :::5000 | This error occurs when an application tries to listen on a port, like 5000 in this case, which is already occupied by another running process |
|
When you’re trying to run a Node.js application using Nodemon, the utility continually listens for file changes in the directory and automatically restarts the server, providing for efficient development. When there comes an attempt to have your application listen at port 5000, and if there’s another active process occupying this port, it results in the error “Nodemon: Error: Listen EADDRINUSE: Address Already in Use :::5000”.
Understanding why this conundrum arises primarily roots to how networking ports function. Consider them as doorways into your computer where only one application or service can use a specific port at any given time. So, if another process, maybe another Node.js app or a completely different service, is already listening on port 5000, then your current app won’t be able to occupy that same port, causing the aforementioned distressing error message.
There are essentially two straightforward fixes to this:
-
x11
Terminate the process
that’s currently occupying the port. This can be done through command-line instructions such as
xxxxxxxxxx
111lsof -i :5000
to find out the PID (Process ID) of the service holding the port followed by
xxxxxxxxxx
111kill -9 PID
to terminate the service, where PID represents the ID of the process.
-
xxxxxxxxxx
111Utilize a different port
, clearly not occupied by another service, for your application. You can easily do so by changing the listening port in your server setup code; for instance, if using Express, the line might read
xxxxxxxxxx
111app.listen(5001)
instead of previously trying to listen to 5000.
Note that these solutions rely on either freeing up the desired port from its existing obliger or graciously settling for a different port entirely.Understanding the Nodemon error: “Eaddrinuse” requires a comprehension of what this specific error means and why it occurs in our development environment. The error Eaddrinuse, means that the address, typically involving the combination of IP address and port number like
xxxxxxxxxx
:::5000
, is already in use.
A typical scenario where you may encounter this error specifically with Nodemon (a utility that will monitor for any changes in your source files and automatically restart your server) is when you have an application instance running on the specified port, then you attempt to start another instance without first closing the active one.
To put it into context, here’s an illustration. You designate port 5000 for your local development server.
So to start your server, you’d indicate something similar to:
xxxxxxxxxx
app.listen(5000, function(){
console.log('Listening on port 5000...');
});
Then you started Nodemon for it to monitor file changes in your app folder so it can conveniently restart the server whenever it identifies modifications. This is when issues arise because Nodemon initiates another server that attempts to bind to port 5000, which is currently occupied.
Addressing the “EADDRINUSE” error generally entails doing either of two things:
1. Firstly, close the existing application instance running on that specified port:
You can manually find the process occupying port 5000 by typing in the terminal:
xxxxxxxxxx
lsof -i :5000
After identifying the process, terminate it using:
xxxxxxxxxx
kill -9 {PID}
Where {PID} represents the Process ID you distinguished from the command line before.
2. Secondly, utilize different ports for each running instance of your application. Always ensure that no other services are utilizing your designated port before running your server.
Remember, like all tools, Nodemon is programmed to help make life easier for developers. Should it turn out to be problematic or contribute to confusion, there are alternatives to use, such as Node.js built-in file system (fs) module to watch files, and others like Chokidar, node-supervisor, and pm2.
Knowing how to resolve this particular error will lead to an enhanced coding experience, minimizing downtimes, frustrations, and above all making your development journey smoother and enjoyable. Avoiding errors and knowing how to fix them when they occur is just as significant as producing ‘clean’ code and creating fantastic applications.
You can read more about understanding common nodejs errors [here](https://www.joyent.com/node-js/production/design/errors).
As a professional coder, it becomes second nature to debug these common environment setup issues, reducing time spent on setup and focusing more on actual code implementation.When working as a professional coder, we’ve all run into the ‘Address Already in Use: :::5000’ error at one point or the other. This particular message indicates that you’re trying to start your server while something else – possibly an instance of your application – is already running on the same port (in this case, port 5000). More specifically, this issue tends to crop up when using Nodemon, especially during rapid development, where server restarts can be frequent.
Let’s dive a bit deeper and uncover some potential causes that might be leading to the ‘Error: Listen EADDRINUSE: address already in use :::5000’:
● The first and most common culprit has to do with lingering Nodemon or Node.js processes. If your application crashes or exits abruptly, or if a previous instance didn’t shut down correctly, these “zombie” processes could survive and continue to occupy port 5000. The next time you attempt to start your server, the system perceives the port to be taken, resulting in the EADDRINUSE error message.
Some possible solutions for cleaning up these runaway processes include:
– You could manually search for and terminate these processes via command-line utilities like
xxxxxxxxxx
lsof
, or
xxxxxxxxxx
pkill
. Here’s a sample command:
xxxxxxxxxx
kill $(lsof -t -i:5000)
– Another alternative could be to include error-handling logic in your codebase itself to ensure graceful shutdowns even when encountering exceptions or signals like SIGINT or SIGTERM.
● A less apparent reason could be associated with OS-level network configurations or security software (like firewall settings) interfering with your software stack. While less likely, it’s not completely out of the realm of possibility. This would require a more in-depth look at your specific set-up.
Apart from these, there may indeed be other, more subtle causes that are harder to pinpoint without more context or understanding of your exact circumstances.
To keep your coding process smooth and hassle-free, I’d also recommend exploring NPM packages like ‘dotenv’ for managing environment variables independently or perfecting a debugger setup that supports hot-reload. These tools help maintain a rapid testing and development cycle without falling prey to annoying hitches as highlighted above.
In the hopes of keeping our conversations open and fluid, feel free to ask further questions or provide additional details regarding your experiences with the ‘Error: Listen EADDRINUSE: address already in use :::5000’. Remember, behind every error message, there’s a wealth of learning lurking!
Sources:
stackoverflow.com – ‘Node.js / Express: “address in use” error’ |
shapeshed.com – ‘Command-line Utilities with Node.js’ |
npmjs.com – ‘dotenv’ package description |
If you’re dealing with the “Nodemon: Error: Listen Eaddrinuse: Address Already In Use :::5000” issue, it is mostly likely because port 5000 on the network interface that your Node.js application is trying to bind to, is already in use. This might happen when there are multiple requests sent to the same server at a time.
Troubleshooting this error involves:
- Determining the process currently using the port
- Stopping the process using the port
- Resolving the root cause to prevent it from happening again
Determining the Process Using the Port:
Firstly, to find out which process is currently using port 5000 – execute below command:
xxxxxxxxxx
lsof -i :5000
This will return information about the process currently listening on port 5000. Note the PID (Process ID) for the next step.
Stopping the Process:
Secondly, once you’ve identified the offending process, you can stop it by its PID as shown below:
xxxxxxxxxx
kill -9 <PID>
Replace `<PID>` with the process ID you found in the previous step.
Doing so should close the program binding to port 5000 and free up the port.
Preventing Future Errors:
Last, but not least, let’s look into addressing the root cause of this issue. The common scenarios include:
- Sometimes, the nodemon process doesn’t shut down correctly, and so it continues to occupy the network port. To prevent this, ensure graceful shutdown or consider using
xxxxxxxxxx
111--delay
option.
The command will appear something like:
xxxxxxxxxx
nodemon --delay 1.5 server.js
Here, Nodemon will wait 1.5 seconds before restarting in case of file changes, providing enough time for the existing process to shut down gracefully.
- In others scenarios, conflicting services may be running on the chosen port or ports may not have been released correctly. Ensure no conflicting service runs on the same port, or ideally, let the operating system choose a free port dynamically if your app allows it.
Remember that these methods merely offer immediate relief. It is critical to identify why the process didn’t terminate as expected or why the port was occupied, possibly pointing to design flaws in the application being developed – which ultimately requires proper debugging.
You can also refer to online resources and communities like Stack Overflow where similar queries and their solutions have been discussed extensively. Remember to keep your application updated and follow best coding practices to minimize encountering such errors.
Example Source Code:
xxxxxxxxxx
// RESTful API built with Express.js
const express = require('express');
const app = express();
const PORT = process.env.PORT || 5000;
app.get('/', (req, res) => {
res.send('Hello World!')
})
app.listen(PORT, () => {
console.log(`Server listening on port ${PORT}`);
})
Nodemon is a highly beneficial utility that automatically restarts the node application when file changes occur in the directory. However, it can come up with this specific error message:
xxxxxxxxxx
Error: listen EADDRINUSE: Address already in use :::5000
If you’re faced with this error, it’s telling you that the port your application is trying to use (in this case, port 5000) is already occupied by another process. This conflict leads to an inability for Nodemon to initiate your Node.js application, resulting in the EADDRINUSE error.
Understanding the Cause and Resolution
This error is most common if there are multiple instances of your server running. If you unexpectedly terminate a server or if it fails to stop correctly, it could continue occupying the port. Subsequent attempts to start a server on the same port will then fail due to the address in use issue.
To resolve the problem, follow these steps:
-
Kill all existing node processes.
-
You can also run a more specific command to kill only the process using the specific port (in this case, we are targeting port 5000).
xxxxxxxxxx
121$ lsof -i :5000
2
The result of this command gives you the details of the process currently using the port. Identify the PID (Process ID) and terminate it with a kill command:
xxxxxxxxxx
121$ kill -9 [PID]
2
-
Use different ports for different servers. Ensure each server runs on its unique port. Add the following at the beginning of your script:
xxxxxxxxxx
121process.env.PORT = process.env.PORT || '5001';
2
xxxxxxxxxx
$ killall -9 node
After successfully freeing up the port, you should be able to start your server again without encountering the Nodemon EADDRINUSE error.
Preventing Future Conflicts
Though the steps mentioned above can solve your immediate issue, it is equally important to prevent this conflict from reoccurring in the future. Here are some tips:
- Make sure to correctly shut down your servers every time to ensure they aren’t left running and occupying a port.
- Have error checks within your codebase if the server fails to instantiate correctly, particularly on an EADDRINUSE error. If such an error occurs, let the application log it and shut down gracefully, thereby releasing the port.
- Where possible, randomize the ports used by your Nodemon applications if you’re managing several projects concurrently. This minimizes the chances of port conflicts.
Resolving Nodemon port conflicts requires understanding of how node processes works and what causes these conflicts. With correct error-handling practices and strict adherence to shutting down servers, you will maintain smooth operation of your applications every time you use Nodemon.
For more information on how to use Nodemon effectively, visit the official NPM Documentation Page.
The error “Listen EADDRINUSE: address already in use :::5000” is a common issue that many developers encounter when they are trying to build or maintain web applications. This happens when the application tries to open a server on port 5000, but this is not possible because there’s already an app running on the same port.
Well, you should know that ports on any network can be used only by one application at a time. Now let’s get into deeper analysis and look at common solutions on how to resolve this. We’ll later zoom into Nodemon situations as well.
Step One – Identify the Problem
The thing is, you cannot run multiple apps on the same port concurrently. Every app must have a unique port it’s using. To fix the problem, you need to identify which other process is currently using port 5000.
To do this, we can utilize the terminal command line. If you’re on one of the Unix-based operating systems like Linux or Mac, you could use the
xxxxxxxxxx
lsof
(list of files) command:
xxxxxxxxxx
lsof -i :5000
In the Windows world, you can use the
xxxxxxxxxx
netstat
command:
xxxxxxxxxx
netstat -ano | findstr :5000
These commands return a process ID or PID for the program that’s using the port In question.
Step Two – Kill the Offending Process
Once you have identified the PID, you can stop the process with a simple command:
xxxxxxxxxx
kill -9 [PID]
In Windows, that would look like:
xxxxxxxxxx
taskkill /PID [PID] /F
Replace ‘[PID]’ with the actual process ID number returned from the first command.
Error Specifics to Nodemon
The error might keep recurring if you’re using a tool like nodemon. This is mainly because nodemon is designed to supervise your Node.js app, watch for any file changes in your directory, and whenever a change is detected, it automatically restarts your application.
However, sometimes, especially after crashes or ungraceful shutdowns, nodemon or the app itself cannot clean up properly and will leave an instance running in the background. Therefore, anytime nodemon attempts to restart a crashed app or if you’re trying to run your app again before nodemon finishes shutting down the previous one, you end up trying to create an app that “listens” on the same port as the still-running old instance.
To handle this scenario, ensure that in your app code, you handle exceptions properly to allow a graceful shutdown. This way, you give nodemon enough time to restart your app.
Also, try increasing nodemon delay restart time by setting delay option:
xxxxxxxxxx
nodemon --delay 1.5 [file]
This gives nodemon about 1.5 seconds to complete shut down/restart operations.
All these mentioned actions put together should entirely help resolve the “Listen EADDRINUSE: address already in use :::5000”.
To learn more, follow the hyperlink “StackOverflow thread on EADDRINUSE“Many developers encounter the
xxxxxxxxxx
EADDRINUSE
error while using Nodemon, a convenient utility that auto-restarts your Node.js server every time code changes are detected. This typically occurs when a previous instance of the Node.js server is still running on the same port you’re trying to open for a new instance.
The error listens as:
xxxxxxxxxx
Error: listen EADDRINUSE: address already in use
To walk you through this common issue, I’ll outline some functional remedies which can prove useful for resolving this error:
Kill The Process Manually
Firstly, we can identify the process still running on port 5000 and manually kill it. We’ll need the PID (Process ID) to do so. On Unix-based systems like macOS or Linux, the following command will reveal the needed PID:
xxxxxxxxxx
lsof -i :5000
This command lists all processes running on port 5000. To kill a process running on this port, we’d use the following command:
xxxxxxxxxx
kill -9 [PID]
If the issue persists, consider building an automated solution by creating a script that combines above commands.
Create a Script to Kill The Process Automatically
A more automated approach requires generating a script that automatically kills any process running on a specific port before starting your application.
xxxxxxxxxx
"scripts": {
"start": "fuser -k 5000/tcp && node server.js"
}
Note: the ‘fuser’ command may need installation on some Unix-based systems.
Using Different Ports with Environment Variables
Lastly, utilizing environment variables to run servers on different ports can prevent conflicts. In development, we often switch between multiple applications/services; assigning each one a unique port helps avoid
xxxxxxxxxx
EADDRINUSE
.
In your main server file:
xxxxxxxxxx
var server = app.listen(process.env.PORT || 5000)
When launching your server:
xxxxxxxxxx
PORT=3000 nodemon server.js
With these steps, if one service exclusively occupies port 5000, other services will fallback to an available port such as 3000.
Remember: Always repost your updates within your source control management system, whether it’s GitHub, Bitbucket or something else to keep everyone in the loop on these changes. For an in-depth reading on managing node.js processes, check out this post on Dev Community.Nodemon, developed by Remy Sharp, is an essential tool for Node.js application developers. It significantly enhances the development process by automatically restarting the application whenever file changes are detected within the directory it is being run from. Its usage generally standardizes the approach towards server restarts, leading to the continuous improvement of the coding strategy.
Understanding Nodemon’s Functionality
The traditional approach while working on a Node.js application involves manually stopping and starting the server each time a change has been made. This can quickly become tedious and time-consuming. Nodemon simplifies this approach by automating the server restarts. To use it, follow these steps:
• Firstly, install Nodemon globally using npm (Node Package Manager) with the command
xxxxxxxxxx
npm install -g nodemon
.
• When developing your application, rather than running it with
xxxxxxxxxx
node server.js
, use
xxxxxxxxxx
nodemon server.js
instead.
This allows Nodemon to watch all the files in your directory for any changes. File events such as adding, updating or deleting a file prompt Nodemon into action, triggering a server restart.
Error: Listen EADDRINUSE: Address Already In Use :::5000
One prominent issue encountered when using Nodemon is the “Error: Listen EADDRINUSE: Address already in use :::5000”. This error pops up when another process is already running on port 5000. To resolve this issue, there are a few possible solutions:
1. Rerun Nodemon:
Sometimes, simply rerunning Nodemon may clear up pending processes and make port 5000 free for use again. Make sure to stop Nodemon completely using ‘ctrl+c’ before restarting it.
2. Kill the Process Using the Port Individually:
You can find and kill the process occupying the port directly. Use
xxxxxxxxxx
lsof -i :5000
to list all PID’s using the port and then
xxxxxxxxxx
kill -9 PID
to terminate them individually.
3. Change the Port:
If other options don’t work, consider changing the port that your app uses. If 5000 isn’t available, switch to 5001, 5002 or any other valid port number not currently in use.
Preventing Port Blocking Issues
While dealing with port-related issues such as the EADDRINUSE error, it can be beneficial to set up a mechanism to prevent overlooked server shutdowns. One way to achieve this is by attaching a listener to the ‘SIGINT’ signal, ensuring the server stops when Nodemon restarts it or you manually stop it. Here’s a piece of code showing how to do this:
xxxxxxxxxx
process.on('SIGINT', ()=>{
server.close(()=>{
console.log('Process terminated')
process.exit(0)
})
})
In essence, Nodemon plays an integral part in enriching the experience of developing Node.js applications. It simplifies testing, debugging and implementation activities by monitoring changes and triggering automatic server restarts. However, care must be taken to effectively manage resources like ports to avoid stumbling blocks during the development journey. Working with Nodemon and understanding its functionality along with associated errors contributes positively to the growth trajectory of every developer.source.Digging deep into the node.js world, we encounter a common issue that most developers face – the
xxxxxxxxxx
Nodemon: Error: Listen Eaddrinuse: Address Already In Use :::5000
error. This error is a throwback from your system telling you that the port 5000 is already used by another process.
Understanding and resolving such errors is essential for all Node.js developers.
xxxxxxxxxx
server.listen(port, () => {
console.log(`Server running on port ${port}`)
})
All looks good until that dreadful error pops up. You might wonder, “Why this error?”, especially if you just started your Express server without any issue previously. Well, this is because each port on a system can only be used by one application at a time. So when two applications try using the same port (in this case, port 5000), the conflict arises leading to the ‘EADDRINUSE’ error.
There are several approaches on how to get around the error:
• The simplest way is to reboot your system. This will automatically kill all running processes including the blocking one. But, as code ninjas, we know it’s not the most efficient solution.
• Another approach is finding out the process that’s using the port using commands like
xxxxxxxxxx
lsof -i :5000
or
xxxxxxxxxx
fuser -k 5000/tcp
for Unix-based systems, and then killing that process.
• My personal favorite approach, which I believe also develops better coding habits, is ensuring to properly close your servers after use. It’s a cleaner way of preventing future port conflicts. This can be incorporated in your codes using triggers than can automatically stop the server once you’re done with it.
Great tools like [pm2](https://pm2.keymetrics.io/) can help manage your node apps in a production environment and avoid errors like these.[1].
Nonetheless, experiencing such errors doesn’t reflect poorly on your development skills. On the contrary, they provide a chance to dive deeper into the functionalities and grow as a professional in the Node.js realm.
Remember, code fails != coder fails. Every problem encountered is an opportunity in disguise paving way for us to become better coders. Happy Coding!
Sources
1. PM2 | Runtime Application for AI/Machine Learning. PM2. https://pm2.keymetrics.io/