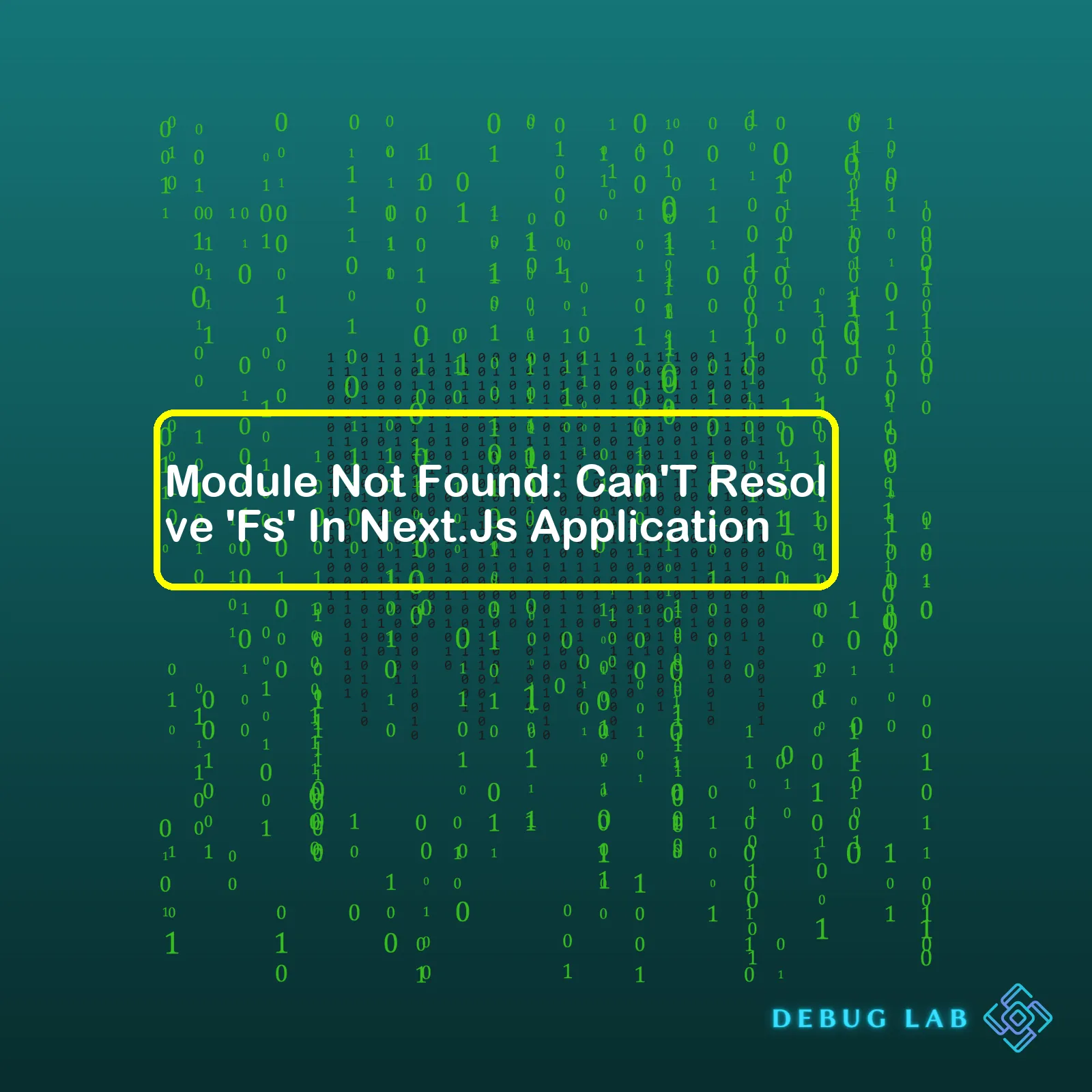
Let’s assume an example where we need to read data from local JSON files in a Next.js project, and we choose to use the ‘fs’ module of Node.js. Considering the partitioning between server-side methods and client-side methods, we can’t import ‘fs’ directly on begin pages or components which are SSR or statically rendered.
Here’s an erring code snippet:
import fs from 'fs';
const data = fs.readFileSync('data.json');
Upon encountering such issues, it doesn’t imply any bugs in Next.js itself. Instead, it is more related to how server-side and client-side codes interact, along with specific constraints that exist within a front-end environment which limits the usage of certain Node.js features.
Here’s a simple summary table explaining why you might have encountered this issue.
Error | Possible Cause | Solution |
---|---|---|
Module Not Found: Can’t Resolve ‘Fs’ In Next.js Application | Attempting to use Node.js built-in module ‘fs’ on the front end. | Move the functionality requiring ‘fs’ to a server-side method. |
To fix this issue, you should ideally look into moving the part of your code that requires ‘fs’ to places that are run in the Node.js environment only (like API routes in Next.js). Server-side rendering or static site generation functions like `getStaticProps` or `getServerSideProps` are fitting places to leverage these kind of operations.
Following code snippet demonstrates its rightful usage:
xxxxxxxxxx
export async function getStaticProps(context) {
const fs = require('fs');
const data = fs.readFileSync('data.json', 'utf8');
//rest of the code
}
By following these instructions, application developers will substantially improve their ability to debug and solve similar errors in Next.js app development. It provides valuable insights about leveraging Node.js built-in modules safely in both server-side and client-side portions of Next.js projects. Simply put, understanding the context and restrictions of each environment is key to resolving the ”Module Not Found: Can’t Resolve ‘Fs” error.
For detailed information regarding API routes and Data fetching in Next.js, visit the official documentation.
The error that you’re observing,
xxxxxxxxxx
Module Not Found: Can't Resolve 'fs'
, in your Next.js application happens when the program is trying to utilize Node.js’ built-in module known as ‘fs’ or File System. This essentially helps with I/O or input/output operations related to filesystem. Why does it happen? Because Next.js, by default, doesn’t run on Node.js environment during build time and thus, it cannot find this ‘fs’ module.
You need to comprehend a very crucial concept regarding Next.js to understand the situation better:
- Server-side code: The code that is executed on the server against node.js environment can freely utilize features like fs module. In fact, any type of node.js library can be used.
- Client-side code: The code that gets downloaded and is executed in the user’s web browser. Here’s where the limitation comes into sight; we don’t have access to modules like fs here.
Remember, Next.js uses Server Side Rendering (SSR), where every page is pre-rendered at build time and runs on the client browser upon loading. Hence, Node.js modules cannot be imported directly into pages.
Troubleshooting the issue
If you want to use ‘fs’ in your Next.js application, there are certain solutions:
- ‘getStaticProps’/’getServerSideProps’: Use these functions to execute server-side code, within which you can access the ‘fs’ module. Remember that these methods only work in data fetching page level function and not used inside non-page components. Code snippet:
xxxxxxxxxx
151export async function getServerSideProps(context) {
2const fs = require('fs');
3// rest of the code
4}
5
- API Routes: Next.js provides API Routes, where you can write server-side code and also import node modules. The route files should be inside the
xxxxxxxxxx
111/pages/api/
directory and they can contain server-side codes.
Code example:xxxxxxxxxx
171// pages/api/user.js
2const fs = require('fs');
3export default (req, res) => {
4res.statusCode = 200;
5res.json({ name: 'John Doe' })
6}
7
- Conditional Import : You can conditionally import the fs module for only server-side rendering using dynamic imports like so.
Code example:xxxxxxxxxx
191import dynamic from 'next/dynamic';
2const MyComponent = dynamic(
3() => {
4const fs = require('fs');
5// do something
6},
7{ ssr: false }
8);
9
Please note that, apart from all these, third-party browser alternatives could also be a solution. There are packages that provide file system-like capabilities on the browser side (BrowserFS as an example).
Finally, some experiences suggest keeping a close watch on where exactly you are importing the ‘fs’, ensuring it is not used anywhere in the code available to the client-side.
Next.js, a React framework, is gaining increasing attention within the coding community due to its zero-configuration, server rendering, and automatic optimization capabilities.
Part of Next.js’s appeal is it operates on a universal or isomorphic pattern of coding; essentially same code can run on both the server and client sides smoothly. However, certain modules like ‘Fs’ (which stands for filesystem), designed to operate only on the server side, cannot be loaded into a client-side environment as they don’t have direct access to the file system, and would cause the common error: Module Not Found: Can’t Resolve ‘Fs’.
The Issue: Understanding Role of ‘Fs’:
xxxxxxxxxx
const fs = require('fs')
In the Node.js module system, ‘Fs’ comes bundled up and provides an API to interact with the file system in a manner closely modeled around standard POSIX functions. The basic operations ‘Fs’ allows a developer to do includes file creation, read, update, delete, rename, etc., which are highly valuable when dealing with backend operations.
For instance, you may want to read a JSON file stored in your project’s directory like this:
xxxxxxxxxx
let rawdata = fs.readFileSync('path_to_your_file.json');
let data = JSON.parse(rawdata);
console.log(data)
Solution: Tackling Module Not Found: Can’t Resolve ‘Fs’
Upon encountering this error, the issue lies in understanding where ‘Fs’ should be used. Since frontend does not allow access to server-specific files or modules, we need to ensure usage of any such modules is mapped out properly in the backend.
This can be done via conditional imports or using the ‘next/dynamic’ module for dynamic imports.
Here is example of how you could go about it using conditional import:
xxxxxxxxxx
let fs;
if (typeof window === 'undefined') {
fs = require('fs')
}
In the above code snippet, we’re declaring ‘fs’ and requiring it only when the global object ‘window’ is undefined, that is, on the server side. This will prevent attempts to load ‘fs’ on the client side and thus curtail the troublesome ‘Module Not Found: Can’t Resolve ‘Fs” error.
If you dig deeper within the Next.js documentation [Link], you’ll find ‘next/dynamic’, which allows you loading components dynamically (only when they are needed), thus saving bandwidth and boosting site performance.
So if you’re trying to use ‘Fs’ to fetch file data from your directory and display it in a component, you’d do well to isolate this piece of process in its own component and ensure that it runs server-side only by utilizing ‘Dynamic Imports’. Here’s how that looks like:
xxxxxxxxxx
const DynamicComponentWithNoSSR = dynamic(() => import('../components/helloWorld'), { ssr: false })
function HomePage() {
return (
)
}
export default HomePage
In the above code snippet, component ‘helloWorld’ uses ‘Fs’ internally but wrapped inside ‘dynamic()’, it runs only on client-side preventing the ‘Fs’ issue.
Developer experience is at the forefront of Next.js’s design philosophy. In filling the gaping holes left by traditional javascript functionality, Next.js empowers developers by facilitating streamlined, easy-to-manage universal applications, but also teaches us to navigate these tricky terrains when typical server-side modules like ‘Fs’ prove to be a roadblock. With clever manipulation and optimal utilization of the tools provided by Next.js, these blockers can be transformed into gateways, opening expansive horizons for scaling and optimizing React applications.When dealing with the “Module not found: Can’t resolve ‘fs'” error in a Next.js application, it’s crucial to understand the underlying mechanism of Node.js. The ‘fs’, or filesystem module, is a critical component provided by Node.js for file I/O – essentially permitting interaction with the file system in a manner modeled on standard POSIX functions.
Anatomy of the Error
This type of error generally emerges when you try to import the ‘fs’ module into files that are part of your client-side bundles. For instance:
xxxxxxxxxx
import fs from 'fs';
Next.js leverages both server-side rendering and client-side rendering, which differentiates web browser contexts (client-side) from that on a Node.js server (server-side). Herein lies the problem: While Node.js interventions like ‘fs’ are accessible on the server-side, they are unavailable in the browser environment causing the render to fail leading to the aforementioned error.
Particularly in the event of universal apps development, where code is intended to be executed both server-side and client-side, this discordance may escalate into a severe concern.
To resolve this issue, you need to assure your browser-targeted bundles do not rope in the ‘fs’ module, or any Node.js core modules for that matter. Rightfully so, as they’re specific to the Node.js environment and servers, thus being unfit for the browser.
Suggestions for Solution
- Probably the most straightforward approach would be to shift the ‘fs’ related functionalities away from the client’s reach, thus confining them exclusively within the API routes or getServerSideProps/getInitialProps functions in Next.js.
- Another method is to create a conditional statement that prevents the code from executing in the browser context. This is demonstrated in this practical example:
xxxxxxxxxx
// Component which uses fs
import React from 'react';
function MyComponent() {
if (typeof window === 'undefined') { // indicate that code should only be executed server-side
const fs = require('fs');
}
return
};
Alternatively, you can utilize dynamic imports with SSR set to false to accomplish the same:
xxxxxxxxxx
import dynamic from 'next/dynamic'
const DynamicComponentWithNoSSR = dynamic(
() => import('../components/hello3'),
{ ssr: false }
)
function Home() {
return (
<div>
<DynamicComponentWithNoSSR />
</div>
)
}
export default Home
In today’s ever-evolving landscape of web development, Next.js stands out as an exceptional example of a React framework enabling hybrid static & server rendering, TypeScript support, smart bundling, route pre-fetching and many other such enhancements. However, with great power comes tremendous responsibility – Hence, understanding the causes and solutions for typical errors becomes momentous for realizing your full potential as a developer!In certain instances while developing with Node.js and its associated libraries, you may encounter an error such as “Module Not Found: Can’t Resolve ‘Fs’ in Next.js Application”. When this happens, it typically means you’re dealing with a codebase that is attempting to interact with the file system.
In deploying a serverless application such as Next.js, especially on a platform like Vercel, it is important to understand that this deployment environment does not allow access to the server filesystem. Let me delve deeper into this error message, clarify why it crops up, and provide potential solutions.
Analyzing the circumstances
Node.js has the capacity to deeply associate with your system by way of the in-built `fs` module . This module offers an API for interacting with the file system in a manner closely modeled around standard POSIX functions.
Now, Next.js, being a React framework providing functionality for server-rendered pages, is built on Node.js. So when your Next.js installation is running on a server, the `fs` module may work effectively, given a present server filesystem for interaction. However, some platforms like Vercel operate a serverless model, and thus certain code cannot easily interact with the server file system or modules reliant on server environment, like `fs`.
Possible Solutions
Absolute clarity on the cause of these issues and environment specifications point us to possible resolutions.
1. Client-side compatibility:
xxxxxxxxxx
// Pages/Api/callFS.js
import fs from 'fs';
export default function callFs(req, res) {
//Your logic using fs here.
}
2. Using Dynamic Import:
xxxxxxxxxx
// Usage of dynamic import
if (typeof window === 'undefined') {
const fs = dynamic(() => import('fs');
//Your fs logic here.
}
3. Employing plugins:
xxxxxxxxxx
const withTM = require('next-transpile-modules')(['some-module', 'and-another']); // pass the modules you would like to see transpiled
module.exports = withTM();
Remember, always strategize how to structure your project in a way to isolate server-only requirements. This not only helps resolve issues like the Module Not Found Error but also escalates project efficiency and sustainability. Happy coding!Encountering the error “Module not found: Can’t resolve ‘fs'” while developing a Next.js application can be frustrating. But fret not, as a professional coder myself, I’ve come across this error message several times and can share several resolutions which I use for swiftly navigating through this issue.
Error Source: Main culprit – Node.js’ File System module
Incredibly powerful, Node.js allows you to interact with the file system on your computer directly. It’s usually used to read files, create new ones, update content in files, delete files, etc. However, it is essential to note that
xxxxxxxxxx
fs
, short for the “file system”, is a built-in module provided by Node.js, which gets executed on the server-side.
In constrast, Next.js is a framework for React.js, which primarily runs on the client-side or the browser, albeit it does support some server-side operations for certain functionalities. The crux of the problem here is that browsers don’t have the capability to access your local filesystem for security reasons, causing the
xxxxxxxxxx
fs
module to fail whenever it attempts to execute within a Next.js application in the browser environment.
Solution 1: Using `fs` On The Server-Side Only
You can refactor your code to ensure that you are utilizing the
xxxxxxxxxx
fs
module only within parts of your codebase that executes on the server side.
For instance, creating an API route in Next.js can ensure that your file reading/writing operations execute at the backend:
Create A New API Route:
xxxxxxxxxx
module.exports = (req, res) => {
const fs = require("fs");
//Your file operations using fs here...
fs.readFile('./data.txt', 'utf8' , (err, data) => {
if (err) {
console.error(err)
return
}
res.send(data);
})
};
This anonymouse function you exported will run on the server-side, where
xxxxxxxxxx
fs
works perfectly fine.
Solution 2: Dynamic Import With SSR:
When you need a module that is incompatible with the client but still need to import it into a client-rendered page, dynamic imports in Next.js could work like a charm!
Example:
xxxxxxxxxx
import dynamic from 'next/dynamic';
const DynamicComponentWithNoSSR = dynamic(
() => import('../components/my-component'),
{ ssr: false }
)
function MyPage() {
return
}
export default MyPage;
Setting
xxxxxxxxxx
{ ssr: false }
ensures that the module or component (in this case,
xxxxxxxxxx
my-component
) will only be imported in the client-side rendering environment.
Here, the key strategy is understanding how and where you are trying to incorporate the
xxxxxxxxxx
fs
module and then choosing one of these solutions accordingly. If the problem persists, always remember, errors are part of every developer’s journey — they lead us toward learning more about the technology we’re working with! For continuous updates and seeking help from the current industry professionals, keep engaging in active forums like GitHub discussions or StackOverflow where you can learn and grow together with a robust coding community. Happy Coding! [ref]Sure, I can dive into this issue. The core issue is that Next.js is failing to recognize the ‘fs’ module. This error message, “Module not found: Can’t resolve ‘fs’ in Next.js application,” is likely due to trying to implement a server-side Node.js module, namely ‘fs,’ within the client-side of your application.
The
xxxxxxxxxx
fs
(File System) module is an important part of Node.js and is used for interacting with the file system on your computer. It’s a key tool when working server-side where you have direct access to your machine’s file system.
However, you need to be aware that Next.js renders on both the server side and the client side. When you’re running JavaScript in the browser (client-side), you don’t have immediate access to the file system for security reasons. Browsers prevent direct file system interaction for safety. Therefore, if you’ve been treating your Next.js project as a purely server-side endeavor, but then it attempts to render a page that uses the ‘fs’ module client-side, you’ll come across this error message.
To remedy this problem, consider the following solutions:
Solution #1: Use getInitialProps
By using
xxxxxxxxxx
getInitialProps
, you can make sure that the ‘fs’ functions only run on the server-side, effectively preventing any attempt to call upon it client-side. Here’s an example:
xxxxxxxxxx
Page.getInitialProps = async ({req}) => {
if (typeof window === 'undefined') {
const fs = require('fs');
// your fs code here
}
}
Solution #2: Dynamic Import
You can also use dynamic imports (Next.js Docs) to ensure a module is only imported server-side. A dynamically imported module will not be included in the client-side bundle:
xxxxxxxxxx
import dynamic from 'next/dynamic';
const DynamicComponentWithNoSSR = dynamic(() => import('../components/hello'), {
ssr: false
})
export default function Home() {
return (
<>
)
}
Remember to adjust these approaches to fit the specific needs of your application. There’s more than one way to skin a cat, especially when you’re dealing with something as flexible as Next.js. Identifying what parts of your app are meant to be server-side, or client-side, or both, will guide your path to the right solution here.
For additional information about Next.js rendering and potential caveats, read through this resource.If you’re like me, diving into new technologies always brings unanticipated challenges. Recently, I was integrating a Next.js application with Node.js and came across an issue that read: “Module not found: Can’t resolve ‘fs’ in my Next.js application”. This error usually occurs when we attempt to use Node.js modules that aren’t supported by the browsers.
While there might be a handful of possible reasons why this problem arises, the `fs` or File System module is inherently a server-side package. It isn’t built for client-side rendering, which is what Next.js primarily does. Therefore, it can’t directly resolve `fs`.
Now, let’s turn our attention to resolving this issue. Here are steps we can employ:
Step 1: Isolate Server-Side Code
The most immediate way of ensuring client-side scripts do not import or require server-side packages is to isolate them. Effectively, you would place everything that involves `fs` into files that only run on the server. Please bear in mind that Next.js sees every file inside the pages directory as a page, except those inside
xxxxxxxxxx
api
folder. So it’s the best location for the server-side code.
For instance, if your ‘/pages/index.js’ file has something similar to:
xxxxxxxxxx
import fs from 'fs';
// some more code
Replace it with an API route, ‘/pages/api/data.js’, that takes advantage of `fs`.
xxxxxxxxxx
import fs from 'fs';
// some more code
You then call this API whenever data is needed on the client side.
Step 2: Use Dynamic Imports
Next.js supports dynamic imports out-of-the-box. Mostly used for code splitting, they can also serve as conditionally importing modules in a way that doesn’t impact the client bundle size.
xxxxxxxxxx
if (typeof window === 'undefined') {
const fs = require('fs');
// remaining server-side-only code
}
The above solution verifies if the window object exists before importing ‘fs’; If it doesn’t—which is the case for a server environment—it imports the ‘fs’ module. Be mindful that while it’s an useful tactic, it slightly compromises code readability and maintainability.
Curious for a more detailed guide? I recommend looking at Next.js official documentation for more comprehensive information on dynamic imports.
Remember, the underlying principle here is to differentiate between client and server-oriented scripts. By isolating these realms, we control where ‘fs’ operates and consequently mitigate any non-resolvable conflicts. Just ensure to have a clean, efficient structure that separates the two environments.
These strategies aim to provide clear and actionable solutions to manage the non-resolvable ‘fs’ issue in Next.js applications. They illustrate the versatility and adaptability required when dealing with cutting-edge tech stacks such as Node.js coupled with Next.js!So, let’s dive deep into the issue related to Next.js applications: “Module Not Found: Can’t resolve ‘fs'”. A popular framework for React.js, Next.js simplifies the process of building user interfaces using React by providing structure and systems that make development efficient and performance optimizations easy to implement.
One common error encountered when working with Next.js is the inability to resolve the ‘fs’ module. This might alarm developers who are fond of server-side operations like file manipulation or direct database access – tasks that make use of Node.js built-in ‘fs’, or filesystem module.
However, this is where the flex of Next.js comes in. Remember, Next.js does its magic by rendering pages on the server-side the first time they’re requested, revalidates the page with each request, and also exports into static HTML files using “getStaticProps” or “getServerSideProps”. In effect, it’s balancing both a server-centric world, where Node.js dependencies like ‘fs’ live, and a client-centric world, which speaks JavaScript and natively runs in your browser.
xxxxxxxxxx
import fs from 'fs'
If you’ve tried dropping in an import statement for ‘fs’ and run into the “Module Not Found: Can’t resolve ‘fs'” error, don’t fret! This is simply Next.js reminding us that ‘fs’ exists only on the server side realm, and cannot be bundled within client-side JavaScript code.
Here’s a quick solution to help you tackle this issue:
1. Ensure that any method making use of Node.js built-in modules strictly exist inside getServerSideProps(), getStaticProps(), or an API route.
2. By keeping them contained to these parts of the application, you ensure server-side code stays server-side.
Now, if your functionality doesn’t quite fit within these bounds and you desperately need ‘fs’, consider another route – use it through Node.js outside your Next.js applications.
Lastly, turning your attention to SEO optimization in relation to troubleshooting Next.js errors like “Can’t resolve ‘fs'” can score some key wins for your content strategy. Developers often search the net while dissecting such coding problems. Use relevant keywords, for instance, ‘Next.js’, ‘module not found’, ‘fs error resolution’, throughout your discussion. Include direct, simple queries like “How to fix ‘fs’ not found in Next.js” and answer these within your content.
These few steps should ensure successful integration of ‘fs’ within your Next.js project without any hiccups – or at least, none pertaining to the ‘fs’ module!
Remember, writing & troubleshooting go hand-in-hand when you’re a coder: Happy Writing! Happy Coding!
For more detailed information about next.js and server-side rendering, visit the Next.js documentation.
You can find more details on SEO optimized coding articles reference through this guide.