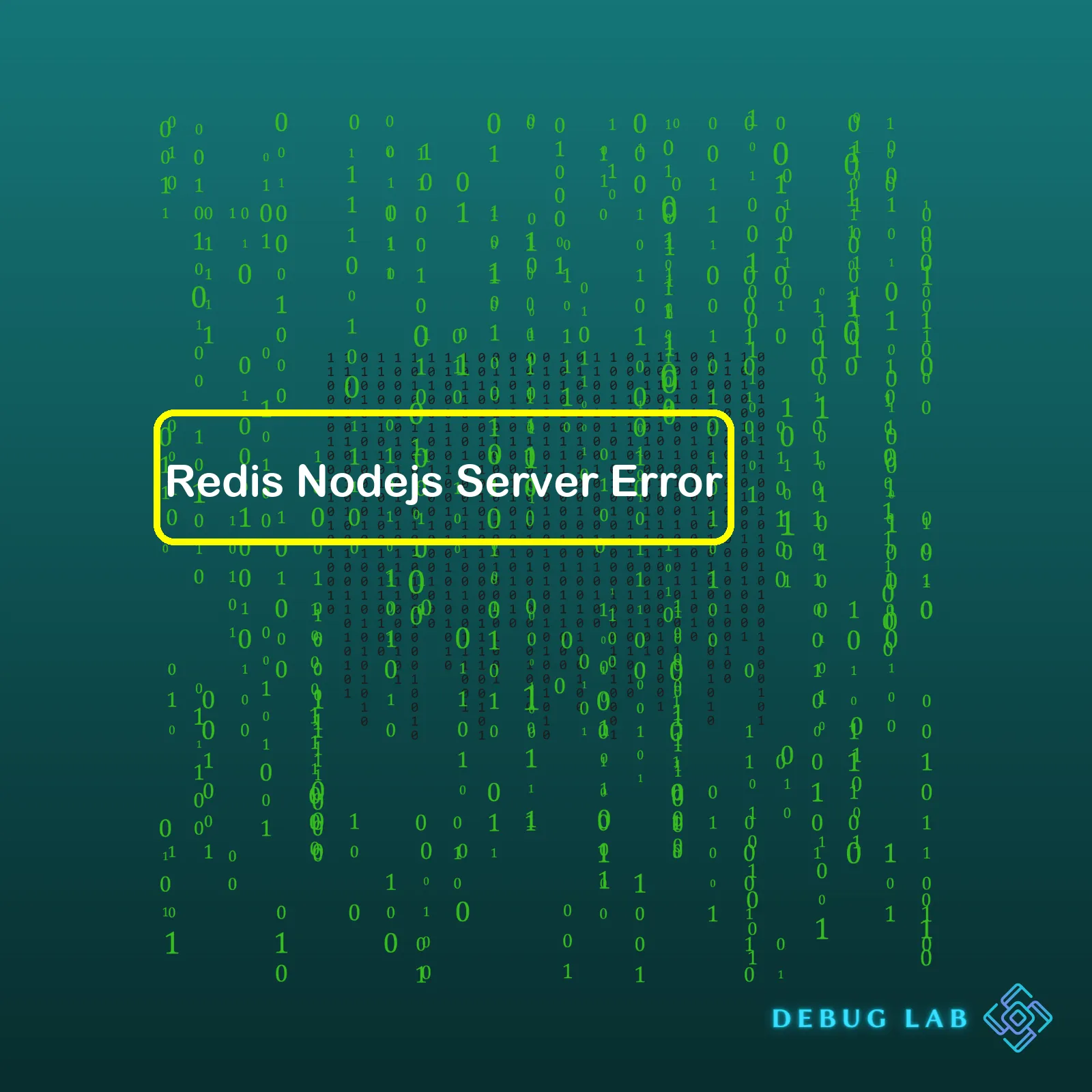
Error Type | Description |
---|---|
Connection Error | Occurs when the client is unable to establish a connection to the Redis server. This could be due to network problems or incorrect configuration settings such as host or port. |
Timeout Error | A timeout error occurs when the server does not respond within a specified period of time. This could indicate an excessively long operation on the Redis server, or network latency issues. |
Authentication Error | Fires when authentication to the Redis server fails due to incorrect credentials. It may also occur if authentication is required by the server, but not provided by the client. |
Invalid Data Error | This error happens when sending invalid data to the Redis server. For example, trying to increment the value of a key that holds a non-numeric string would yield this error. |
const redis = require('redis');
const client = redis.createClient();
client.on('error', function(err) {
console.log('Error: ', err);
});
In the context of web development with Node.js and Redis, these are common server errors that you might encounter. The first type, Connection Error, indicates that a successful connection couldn’t be established between the client (your Node.js application) and the Redis server. This can be due to various reasons like invalid hostname or port, or network-related issues.
Timeout Error is another type, which signifies that a certain operation exceeded the maximum allowed execution time. This usually signals either network latency or some intensive compute task that’s blocking the server.
Then we have Authentication Error. As its name suggests, it arises from failed authentication attempts. Your Node.js app might be using incorrect or missing credentials, or perhaps the server doesn’t allow unauthenticated access at all.
Lastly we consider Invalid Data Error, this usually happens upon supplying incompatible data types for specific operations, like trying to perform mathematical operations on non-numeric data.
To handle these errors, you would typically listen for an ‘error’ event on the Redis client object, as illustrated in the provided code snippet. By utilizing Node.js’s built-in error handling mechanisms, your app becomes robust against unexpected situations and more manageable in the face of encountered problems.The Redis Node.js server error is typically an indication of connectivity issues between your application’s back-end or networking configuration and the Redis database. There are several reasons why you may encounter such an error, including invalid connections strings, overcrowded memory, network connection failures, an incorrectly configured Redis instance, or in larger organizations, firewall settings may restrict certain outbound connections.
Let’s take a silent walk through each one in an elaborate manner:
Invalid Connection String:
The connection string or Uniform Resource Identifier (URI) identifies the host and the port number to which Redis server listens, and it authenticates clients that connect to them. If there’s syntactical or referential difference, as per committed in the code snippet below, it could lead to a Redis server error:
xxxxxxxxxx
client.on("error", function(error) {
console.error(`Redis client not connected: ${error}`);
});
Overcrowded Memory:
When Redis hits its memory limit, either because of the volume of data stored or a memory leak within Redis, it will start giving errors. Your memory usage can be tracked using INFO MEMORY command. By adjusting the ‘maxmemory’ configuration and applying suitable eviction policies, these problems can be mitigated.
Network Connection Failures:
Another cause for the Redis server error is a failure in connecting the app with Redis server. This can be at the network level itself due to Internet Service Provider (ISP) issues, server downtime, packet drops, or even construction near your premises disrupting signal strength.
Incorrectly Configured Redis Instance:
A misconfigured Redis instance may also result in server errors. Critical settings like dbfilename, dir, requirepass should be accurately specified. Failure to do so can render the Redis server unresponsive or not accessible.
xxxxxxxxxx
// Create Redis Client
let client = redis.createClient();
client.auth('password', function(err){
if (err) throw err;
});
client.on('connect', function(){
console.log('Connected to Redis...');
});
Firewall Settings:
In larger organizations or more complex network environments, outbound connections to certain IP addresses or ports might be restricted by a firewall. If Redis is located on such an address or port, this can be another reason for server-side errors.
By identifying the specific cause behind the Redis Node.js server error and applying appropriate corrective actions, software professionals can ensure their applications interact smoothly with Redis databases. For further understanding, tutorials you find here and official Redis documentation are recommended.
Reading and interpreting error messages from the combination of Redis and Node.js can be quite a task, more so when you’re trying to keep your server error-free. However, understanding common error types, why they occur, and knowing how to resolve them goes a long way in maintaining an optimized Redis Node.js Server.
One very common type of error encountered is “Redis connection to localhost:6379 failed”. This error message essentially signifies that Redis was not able to establish a connection with your local host at the mentioned port number (6379 here).
Here’s example code:
xxxxxxxxxx
// Import redis
const redis = require('redis');
// Create client
const client = redis.createClient();
client.on('error', err => console.log(`Error ${err}`));
This error could occur due to several reasons:
- Redis server might not be running: Ensure that the Redis server is up and running on your machine.
- Firewalls blocking your connections: Check firewall or network settings for any blockages stopping Redis from connecting with Node.js.
- Wrong hostname or port number specified: Incorrect IP address or port number may have been used in specifying your Redis server.
Another common error is the “ReplyError: ERR wrong number of arguments for ‘set’ command” error. This occurs when the required parameters for a Redis operation aren’t provided.
Example code:
xxxxxxxxxx
// Set key-value without value
client.set('key1', (err, res) => console.log(err));
To solve this, it is necessary to provide both a key and a valid value for the set operations.
Example code:
xxxxxxxxxx
// Correct syntax for SET
client.set('key1', 'value1', (err, res) => console.log(err));
Lastly, a frequent issue is the “AbortError: SET can’t be processed. The connection is already closed.” error.
These errors are mostly related to network issues like unstable internet connections, or attempting to perform an operation after calling the
xxxxxxxxxx
quit()
method on your Redis client.
Example code:
xxxxxxxxxx
// Close connection before operations
client.quit();
client.set('key1', 'value1', (err, res) => console.log(err));
Ensure stable network connections, correctly sequence your operations, and always call the
xxxxxxxxxx
quit()
method at the end of your operations.
No matter the type of error message received, understanding what each statement signifies provides invaluable insight into making necessary adjustments and getting your Redis Node.js server back to optimal functionality.Certainly, while working with Redis and Node.js, you may experience a number of server errors. Several of these can be particularly tricky to troubleshoot due to their common occurrence and similarity in error messages. By delving into the mechanics of these errors and proposing potential solutions, we can further our understanding of the interaction between Redis and Node.js.
Connection Refused Error:
A frequently spotted error, “Error: Redis connection to localhost:6379 failed – connect ECONNREFUSED”, occurs when your application can’t establish a connection with your Redis server. It often arises from:
- Redis Server not running: You can check this by using
xxxxxxxxxx
111redis-cli ping
. If it returns “pong”, then your server is active.
- Incorrect Configuration: Ensure the Redis host and port in your Nodejs application match the actual Redis server’s host and port.
Error: Ready Check Failed
This error implies a failure during the initial Redis connectivity checks as Node.js is attempting to confirm the readiness of the Redis server.
Here are some resolutions:
- Add this to your configuration:
xxxxxxxxxx
111{no_ready_check: true}
- A faulty network could also cause this error —ensure your network configuration is accurate.
Error: Connection Closed
‘Error: Connection closed’ happens when the established connection between Node.js and Redis gets terminated while an operation is in progress. Reasons might include:
- Commands that terminate the Redis server: Be cautious about utilizing commands such as
xxxxxxxxxx
111SHUTDOWN
, which terminate your Redis server.
- Persistent database connections: Errors can occur when connections remain open past request/response lifecycles. Use connection pooling or built-in client disconnection methods to prevent this issue.
Error: Auth Error
‘Redis auth error’ suggests that authentication isn’t happening properly due to invalid or missing credentials. Verify if your password has been set correctly in the Redis config file.
Node.js and Redis indeed offer quite a few advantages for modern application development, but their inherent errors can prove challenging. I hope this dive into common Node.js Redis server errors equips you to handle and resolve them more effectively. Remember, the responses to commands in the application go a long way in indicating the status of operations and the subsequent resolution in case of any errors. Refer to the official Redis documentation and the vast resource pool available on the Node.js official website for more detailed insights.
To illustrate how you’d typically connect to Redis using Node.js, here’s a quick snippet to use as a reference;
xxxxxxxxxx
var redis = require('redis');
var client = redis.createClient();
client.on('connect', function() {
console.log('connected to Redis');
});
client.set('framework', 'AngularJS');
Remember, practicing optimal error handling techniques is paramount to building robust Node.js applications backed by Redis.
Errors relating to Redis and Node.js can often bring a server to a standstill. Knowing how to diagnose and resolve these issues is crucial for ensuring your platform runs as smoothly and efficiently as possible. For a Redis Node.js Server Error, we will look at some of the most common causes, followed by effective solutions.
1)Connection Errors or Failures
In many cases, an error could be caused simply because Node.js is having trouble establishing a connection to Redis. This might occur if your Redis server is down, or if your Node.js application failed to locate Redis due to incorrect configuration settings.
xxxxxxxxxx
const redis = require('redis');
const client = redis.createClient({
host: 'localhost', // Ensure that the host address is correct
port: 6379 // Also, check that the Redis port number matches
});
2)Running Out of Memory
Redis is an in-memory database, meaning it stores all its data within RAM for quick access. While this allows for rapid read-write processes, it may lead to memory issues if the amount of data stored exceeds the available physical memory.
To troubleshoot, you can monitor your Redis memory usage regularly using the
xxxxxxxxxx
INFO MEMORY
and
xxxxxxxxxx
MEMORY DOCTOR
Redis command line interface (CLI) commands.
3)Operating System Limitations
Each operating system has a limit to how many file descriptors it can have open at once. If this limit is reached, new connections can’t be established, potentially leading to a Redis Node.js server error.
To diagnose, use the
xxxxxxxxxx
INFO STATS
CLI command to view the maximum number of clients allowed and compare it against active connections.
4)Redis Blocking Operations
If you are using blocking operations from Redis such as
xxxxxxxxxx
BLPOP
,
xxxxxxxxxx
BRPOP
, or
xxxxxxxxxx
BRPOPLPUSH
, they could keep your Node.js instance running even when there aren’t any other tasks to process.
xxxxxxxxxx
// Creating a list
client.lpush("info", "test");
// Blocking operation
client.blpop("info", 0, function(err, reply) {
// Process the data here
});
To resolve this issue, consider restructuring your code in a non-blocking manner or implement checks such that blocking operations do not leave Node.js hanging indefinitely.
5)Outdated Modules/Dependencies
Working with outdated versions of Node.js or Redis modules can create compatibility issues, therefore it’s vital to keep them up-to-date. Always ensure to check and update your modules as part of your general maintenance routine.
xxxxxxxxxx
npm update // Updates all packages in your project
npm update [package_name] // Updates specific package
Bonus Tip
A quality of life improvement is to use promise-based or async-based interactions with Redis instead of traditional callback methods. This makes error handling more streamlined and allows your Node.js code to maintain a consistent structure throughout.
xxxxxxxxxx
(async () => {
const value = await getAsync('key-to-get'); // Using async/await instead of callbacks
})();
For more information on handling errors with these technologies, take a look at the official Node.js documentation and the extensive community resources available regarding Redis. Constant learning and practice make troubleshooting these kind of issues much easier and quicker over time.Sure! Even when you are developing on Redis Node.js server, it’s crucial to follow efficient coding practices to avoid imposing potential errors. This not only aids in the production of higher-quality work but also saves time and effort in the future.
One popular Redis Node.js related error is
xxxxxxxxxx
"Redis server went away"
. It often arises from a poor connection management strategy. To avoid this error and many more, here are some preventive measures:
+ Improve Connection Management
You need to ensure proper interaction with the Redis server. Implement reconnect logic for lost connections to keep your application running smoothly. An example solution can be implemented using the
xxxxxxxxxx
retry_strategy
option as follows:
html
xxxxxxxxxx
var redis = require('redis');
var client = redis.createClient({
retry_strategy: function(options) {
if (options.error && options.error.code === 'ECONNREFUSED') {
return new Error('The server refused the connection');
}
if (options.total_retry_time > 1000 * 60 * 60) {
return new Error('Retry time exhausted');
}
if (options.attempt > 10) {
return undefined;
}
return Math.min(options.attempt * 100, 3000);
}
});
client.on("error", function (err) {
console.log("Error " + err);
});