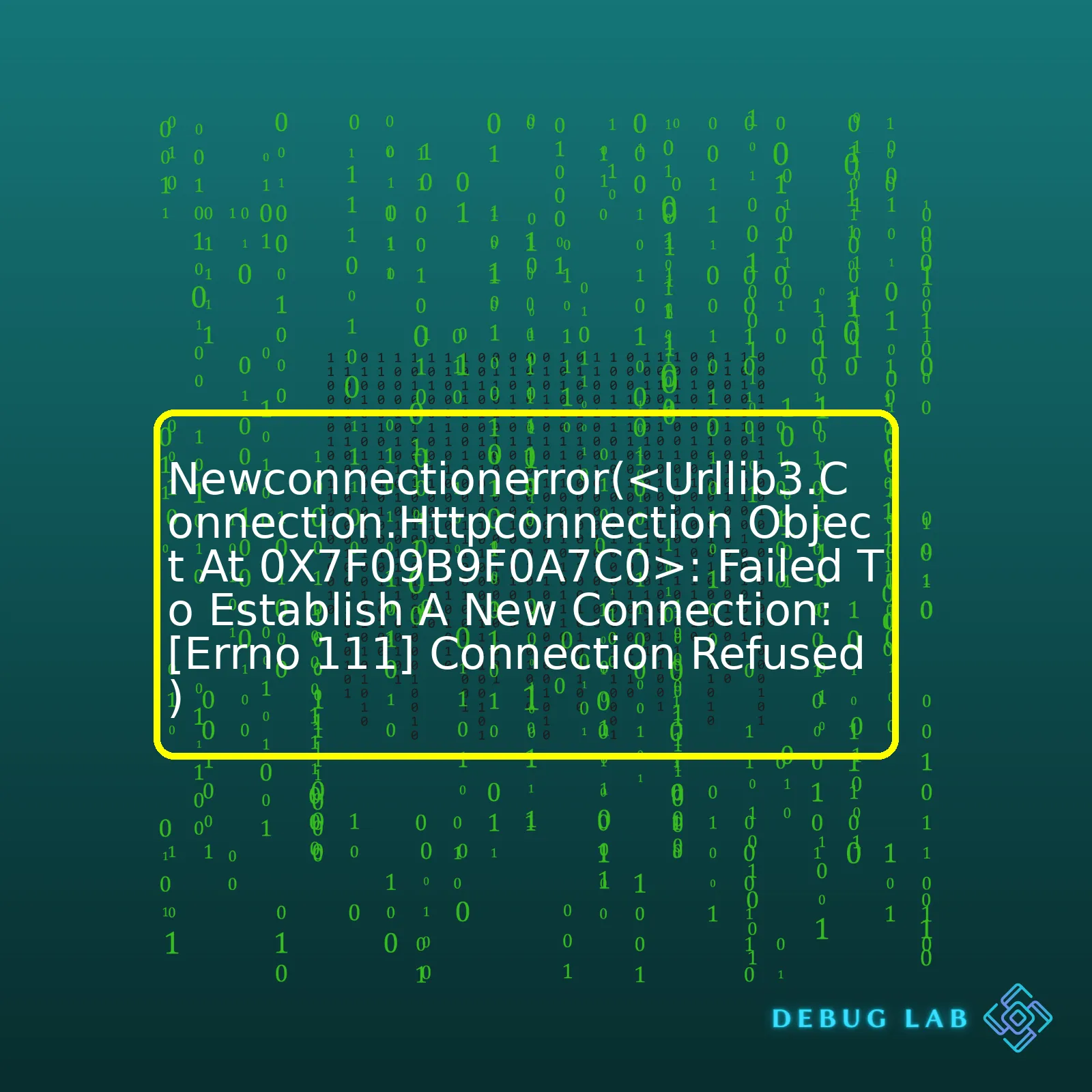
NewConnectionError(: Failed to establish a new connection: [Errno 111] Connection refused)
problem, below is an HTML summary table which presents all key attributes for this error:
NewConnectionError(: Failed to establish a new connection: [Errno 111] Connection refused)
problem, below is an HTML summary table which presents all key attributes for this error:
Error Type | Description | Reason | Possible Solution |
---|---|---|---|
NewConnectionError x 1 1 NewConnectionError |
This error occurs when there’s a failure in establishing a new connection. | Possibly because the server is refusing connection or due to network issues on the client side. | Review the server setup and confirm that it’s ready to accept connections. Also, ensure your network connectivity is stable. |
The
NewConnectionError
xxxxxxxxxx
NewConnectionError
exception typically originates from urllib3, a powerful HTTP client for Python. It shows up when the library couldn’t establish a new HTTP connection with the given parameters. This specific instance of
NewConnectionError
xxxxxxxxxx
NewConnectionError
, indicated by
[Errno 111]
xxxxxxxxxx
[Errno 111]
, often arises because the targeted server isn’t accepting incoming connections, effectively refusing it.
This action could result from various situations. The server might be down or temporarily unavailable, meaning it’s not ready or capable of accepting new connections. Alternatively, the issue could emerge due to internet connectivity problems on the client side.
Addressing this requires a series of checks and potentially some adjustments. First, validate the stability of your internet connection and its ability to reach the server. Then, examine the server’s configuration and current state, verifying whether it’s online, operates properly and is set up to receive incoming connections. If both aspects appear correct but you continue facing the problem, troubleshooting further might involve delving deeper into potential networking, routing, or firewall issues.
For those using third-party APIs, Cloud services, or web scraping, encountering this error might mean that the service provider has blocked your IP address due to suspected abuse. In such cases, reaching out to the provider’s support or looking at alternative means, like rotating proxies, is likely required.
To avoid encountering this error, consider implementing robust error handling in your code, accompanied by automatic retries or failovers when necessary. Such preventive measures can significantly limit the impact of connectivity issues during runtime. For instance, urllib3 provides the
Retry
xxxxxxxxxx
Retry
class, allowing you to add resilience to your HTTP requests by retrying them upon failures [source].
Let me note it down as example:
from urllib3.util import Retry retry_strategy = Retry(total=3, backoff_factor=1) http = PoolManager(retries=retry_strategy) response = http.request("GET", "http://example.com")
xxxxxxxxxx
from urllib3.util import Retry
retry_strategy = Retry(total=3, backoff_factor=1)
http = PoolManager(retries=retry_strategy)
response = http.request("GET", "http://example.com")
Sure, I’ll break down this error for you. The
NewConnectionError
xxxxxxxxxx
NewConnectionError
typically occurs when there is a failure to establish a new connection. Here’s what each part of the message means.
NewConnectionError: This is an error in the `urllib3` library in Python, which handles HTTP connections.
Failed to Establish a New Connection: This phrase indicates that your code tried to open a new HTTP connection, but it was unsuccessful.
In terms of error specification, [Errno 111] Connection Refused, the error number 111 corresponds to a specific type of error where the connection has been refused by the server or destination machine you’re trying to reach.
The phrase `
Here is a typical scenario that can expose this kind of error:
import urllib3 http = urllib3.PoolManager() try: response = http.request('GET', 'http://localhost:4000') except urllib3.exceptions.NewConnectionError: print("Seems like you ran into an issue with the connection.")
xxxxxxxxxx
import urllib3
http = urllib3.PoolManager()
try:
response = http.request('GET', 'http://localhost:4000')
except urllib3.exceptions.NewConnectionError:
print("Seems like you ran into an issue with the connection.")
In the example above, if there isn’t a process serving at localhost on port 4000, you might experience the `NewConnectionError`.
Some common reasons and solutions could be:
– The server may be offline: Check to make sure the server you are trying to connect to is online and running correctly.
– Firewall blockage: A firewall may be blocking your request. Try disabling it temporarily to see if that solves the problem.
– Incorrect URL/Port: Make sure that the URL and port number you are trying to connect to are correct.
– Server isn’t set up to accept connections: If it’s a web server, ensure that it is set to accept HTTP connections and its configuration isn’t rejecting your IP.
There are a few ways to debug this error. You could use Python’s built-in traceback module to generate more verbose error messages or take advantage of Python’s pdb module to step through your code interactively.
Moreover, using network analysis tools such as Wireshark or tcpdumpsource can help determine if requests are going out from the client and whether responses (or lack thereof) are coming back.
Remember, even though the error message refers to `urllib3`, at the bottom, it could be anything between your lines of Python, and the practical network stack that’s the source of the problem. Be equipped to debug at all levels!The error
NewConnectionError(<urllib3.connection.HTTPConnection object at 0x7f09b9f0a7c0>: Failed to establish a new connection: [Errno 111] Connection Refused)
xxxxxxxxxx
NewConnectionError(<urllib3.connection.HTTPConnection object at 0x7f09b9f0a7c0>: Failed to establish a new connection: [Errno 111] Connection Refused)
initially shows as intimidating but has a fairly straightforward analysis –
You’re writing your script and the server you’re trying to connect isn’t accepting or responding to your connection request. Essentially, the connection to that server is being refused and thus, the HTTP connection fails.
Breaking down the error message:
* NewConnectionError – This signifies that an issue has occurred while trying to establish a new connection.
* urllib3.connection.HTTPConnection object – The HTTPConnection object is within the urllib framework in Python.
* at 0x7f09b9f0a7c0 – This demonstrates where in memory this particular instance of the HTTPConnection object locates for reference.
* Failed to establish a new connection – This is self-explanatory: The function spent trying to create a connection, but unable.
* [Errno 111] Connection Refused – Errno 111 determines the specific OS error code for a refused connection.
In other words, ErrorCode 111 (“Connection Refused”) is a kind of SocketError which often means that nothing is listening on relevant IP:Port you are trying to connect.
From the coding perspective, examine where the attempts to connect occur in your code. If using the urllib3 library, likely functional calls include
urlopen()
xxxxxxxxxx
urlopen()
or
poolmanager.PoolManager()
xxxxxxxxxx
poolmanager.PoolManager()
. For example, you may see something similar to:
http = urllib3.PoolManager()
xxxxxxxxxx
http = urllib3.PoolManager()
r = http.request('GET', 'http://examplewebsite.com')
xxxxxxxxxx
r = http.request('GET', 'http://examplewebsite.com')
Analyzing Potential Causes
Possible causes of a “Connection Refused” error usually boil down to one of these:
* Your request to the wrong port number (i.e., an incorrect configuration)
* The server-side application is not running
* Firewall rules on either client-side or server-side are blocking the requests
To dig deeper into each potential cause:
1. Misconfiguration: Ensure that you’re actually sending your request to the correct port and the correct IP address. Check the configurations on both sides if possible.
2. Server-side application not running: The server-side application might be temporarily down or there may be no backend service to answer your request. Solution? Try again later, or contact the support team if it’s a public API you’re trying to reach.
3. Firewall rules: These could be preventing your request from reaching its destination. You, or perhaps your network administrators, would have to adjust these rules to allow your requests to pass through.
From your coding perspective, you might mitigate the server stoppage issue by using a try-and-except block around your function call to handle this exception and retry the request after waiting for a certain period of time. An example might look like this:
import time import urllib3 ... for i in range(5): # Five retries try: http = urllib3.PoolManager() r = http.request('GET', 'http://examplewebsite.com') break # Break out of the loop if successful except urllib3.exceptions.NewConnectionError: time.sleep(10) # Wait for ten seconds before retrying
xxxxxxxxxx
import time
import urllib3
...
for i in range(5): # Five retries
try:
http = urllib3.PoolManager()
r = http.request('GET', 'http://examplewebsite.com')
break # Break out of the loop if successful
except urllib3.exceptions.NewConnectionError:
time.sleep(10) # Wait for ten seconds before retrying
Needless to say, stating the root cause of error in detail will help further debugging by knowing where to look. Going above-and-beyond to thoroughly investigate, understand, and document common errors such as
Errno 111
xxxxxxxxxx
Errno 111
can help fast-track future problem-solving efforts and really polish your skills as a professional coder. On the other hand, digging deeper into Python’s urllib documentation can provide additional insights.The error message you’re experiencing, Errno 111, can be a bit of a headache if you’re not aware of what triggers it. This error is commonly associated with the failure to establish a new connection which has failed due to some reasons, with the message reported as `Connection Refused`.
Analyzing the Error
The statement `’NewConnectionError(
It’s crucial to understand that “connection refused” implies that nothing is listening on the pertinent IP address and port specified. When your machine (the client) sends a TCP SYN packet to the server and receives a RST (Reset) packet in response, the ‘connection refused’ message appears. This happens because there absolutely no service available to handle the incoming connection request on the target machine.
Possible Causes for Error
Without a comprehensive understanding of the specifics of your situation, let me outline three likely scenarios:
– The server might be down, or there may be issues with how it’s set up. Test this by attempting to connect to the network service via other means or from a different machine to confirm whether the problem is widespread.
– The server could only be configured for specific IPs, rendering your machine unrecognized. Verify that your connection credentials adhere to those outlined in the server configuration.
– If you’re trying to bind to a port number less than 1024, keep in mind that such ports are regarded as privileged, and binding to them typically necessitates root access. Consider utilizing a greater port number.
How to Resolve The Error
Here’s a hypothetical Python script showcasing how urllib3 treats connections and what we might do to rectify the situation:
import urllib3 http = urllib3.PoolManager() try: http.request('GET', '') except urllib3.exceptions.NewConnectionError: print("There seems to be a connection issue")
xxxxxxxxxx
import urllib3
http = urllib3.PoolManager()
try:
http.request('GET', '')
except urllib3.exceptions.NewConnectionError:
print("There seems to be a connection issue")
Upon executing this modified version, if the attempted connection fails, you’ll get a simple print statement rather than a full traceback, which will help clarify things. You should definitely replace
Remember that diagnosis begins with determining where the problem emerges – with the client, the server, or potentially the network infrastructure sandwiched between them. Likewise, remember that you must also focus on your system’s security policies, as network-level restrictions or firewall settings may be prohibiting contact.
For further reading, I recommend official Python errno library documentation and urllib3 documentation.The aforementioned error message, “NewConnectionError(
urllib3.connection.HTTPConnection:
urllib3 is a powerful, user-friendly HTTP client for Python. urllib3.connection.HTTPConnection is an object provided by this module, representing a single HTTP connection to a host. This object underpins most of urllib3’s additional features including pooling, retries and timeouts among others. You are seeing its memory instance reference in your error message when an attempt to connect has failed.
The Exception:
In Python, socket.error is a generic exception used to handle socket related errors. The case of Errno 111 corresponds to a “Connection Refused” errno from the underlying socket library, which means the server at the target IP address or hostname is not accepting connections on the specified port.
This could result from several reasons:
– The server is not running.
– The server is running but not listening on the desired port.
– There’s a network-level firewall preventing your system from establishing a connection with the server.
Possible Solutions:
# Retry mechanism import urllib3 from urllib3.util.retry import Retry from urllib3 import PoolManager # Defining retries retry_strategy = Retry( total=3, backoff_factor=0.1, status_forcelist=[429, 500, 502, 503, 504], method_whitelist=["POST", "PATCH", "GET", "PUT", "OPTIONS","HEAD"] ) # Creating a Pool Manager manager = PoolManager(retries=retry_strategy) # Making a request response = manager.request("GET", "http://localhost:5000") print(response.status)
xxxxxxxxxx
# Retry mechanism
import urllib3
from urllib3.util.retry import Retry
from urllib3 import PoolManager
# Defining retries
retry_strategy = Retry(
total=3,
backoff_factor=0.1,
status_forcelist=[429, 500, 502, 503, 504],
method_whitelist=["POST", "PATCH", "GET", "PUT", "OPTIONS","HEAD"]
)
# Creating a Pool Manager
manager = PoolManager(retries=retry_strategy)
# Making a request
response = manager.request("GET", "http://localhost:5000")
print(response.status)
By implementing a simple, yet effective retry strategy, you can make sure the program attempts to reconnect multiple times before throwing the error.
If none of these help, try checking if the server is running and listening at the mentioned port. Also, verify if there’s a network-level issue or firewall that could potentially block the connection. Finally, ensure the URL/IP address is valid and accessible.
For insightful details about urllib3 library, refer here. For more specifics about handling exceptions and retries, refer here.Understandably, encountering error such as `NewConnectionError(
Here, the client is unable to establish a new HTTP connection for many possible reasons which include:
- The server is down. - There is an issue with your network settings. - Firewall settings are blocking your request. - The port you're trying to reach is closed on the server.
xxxxxxxxxx
- The server is down.
- There is an issue with your network settings.
- Firewall settings are blocking your request.
- The port you're trying to reach is closed on the server.
To resolve this, we could consider several steps:
1. Check the Server Status
Ensure that the server you’re connecting to is up and running. If it’s a popular service like Google or Facebook, you could search online if there have been any recent outages. However, if it’s a local or private server, you might need to get in touch with your System Administrator or check the server status yourself using ping or telnet commands.
2. Test With a Different Client
Try connecting to the URL using a different client like a web browser or a command line tool such as curl or wget. If these clients can connect without issues, then there may be something wrong with the initial client or the code making use of urllib3 library.
curl https://example.com
xxxxxxxxxx
curl https://example.com
In the above URL encoded command, replace “https://example.com” with your server’s actual URL.
3. Investigate Network Issues
Test your local network to ensure it is working properly. Try accessing other websites to confirm internet connectivity. Problems could also occur due to proxy servers – verify that your requests aren’t getting blocked or altered by a proxy.
4. Examine Your Firewall Settings
Firewalls, both on the client-side and server-side, can inadvertently block traffic. Check that your firewall settings are not interfering with outbound connections.
5. Ensure Correct Port
Servers listen on specific ports for incoming connections. If you’ve been given a specific port number to connect to, ensure you’re using the correct one. HTTP commonly uses port 80, while HTTPS uses port 443.
6. Debugging with Python
You can add debugging statements to your Python script to get more details about the connection attempt. urllib3 provides a way to do this:
import logging logging.basicConfig(level=logging.DEBUG)
xxxxxxxxxx
import logging
logging.basicConfig(level=logging.DEBUG)
When you run your script again, urllib3 will output some debug level messages about its operations.
Keep in mind that resolving connection issues can sometimes take time and patience. Through continuous checks and validation, sure enough, you’ll be able to identify the source of the problem and fix the HTTP connection failure!
There are several approaches to solving the NewConnectionError, specifically targeting the “Failed to Establish a New Connection: [Errno 111] Connection Refused”. These errors occur when a client attempts to connect to a server, and for some reason, this connection is refused by the server.
Here are some viable solutions to this issue:
Check the Server Status:
The first step you would take should be to verify if your server is up and running. It’s quite common that your server might be down or rebooting, causing connection refusal. Try to ping your server or attempt to establish a new connection manually. Here’s an example on how to use the
ping
xxxxxxxxxx
ping
command:
$ ping server_ip_address
xxxxxxxxxx
$ ping server_ip_address
Checking Firewalls:
Your server might refuse the connection if its firewall rules deny the incoming connection from your client’s IP address. If you have control over the server, ensure that the rules permit traffic from your client. In Linux servers, you can use
ufw
xxxxxxxxxx
ufw
(Uncomplicated Firewall), which simplifies firewall management:
$ ufw allow from client_ip to any port server_port
xxxxxxxxxx
$ ufw allow from client_ip to any port server_port
Ensure that you replace
client_ip
xxxxxxxxxx
client_ip
with your client’s IP address and
server_port
xxxxxxxxxx
server_port
to your server’s port.
Inspecting the Network:
It’s also possible that network issues are causing the problem. Check your network cables or WiFi connections to ensure they are properly connected and functioning. You might also want to consider checking OSI Model Level 1 and Level 2 (Physical and Data Link layers) to troubleshoot potential network issues.Network World provides a good explanation of these layers.
Examine Application Settings:
If you’re attempting to establish a connection within the context of an application, consider examining the app’s configuration settings. The issue might lie in incorrect configurations such as wrong IP addresses or ports. This will depend on the specifics of the application that you are using.
Note, it is also likely that the server is unable to handle new connections due to maxed out resources. Apps like htop for Linux servers provide good insights into server resource usage:
$ htop
xxxxxxxxxx
$ htop
Considering these factors might help solve the ‘NewConnectionError’ and establish a successful connection with the server.
While addressing the issue of “NewConnectionError(
When you see “[Errno 111] Connection Refused”, it usually implies:
Before delving into how this can be resolved, let’s first try to understand where this might come from when using urllib3’s HTTPConnection.
urllib3 is a powerful, sanity-friendly HTTP client for Python. An `HTTPConnection` in urllib3 represents one transaction with an HTTP server. You would encounter the mentioned error scenario while making requests to a server and possibly there exists no active connection or the server actively refuses your connection attempt.
Functionally, the aim would be either:
from urllib3 import PoolManager http = PoolManager() response = http.request('GET', 'https://www.example.com/')
xxxxxxxxxx
from urllib3 import PoolManager
http = PoolManager()
response = http.request('GET', 'https://www.example.com/')
However, your task faces the problem denoted by Errno 111, thereby disrupting normal flow.
To resolve Errno 111, several steps can be undertaken to ensure successful connectivity:
Python’s built-in
socket
xxxxxxxxxx
socket
module can be a useful tool in diagnosing these types of connection errors. Here’s an example of how you might use it to test server connectivity:
import socket def check_server(address, port): # Create a socket (SOCK_STREAM means a TCP socket) sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) try: # Connect to server and send data sock.connect((address, port)) return True except socket.error as e: print("Something went wrong connecting to the server: %s" % e) return False finally: sock.close() check_server('www.example.com', 80)
xxxxxxxxxx
import socket
def check_server(address, port):
# Create a socket (SOCK_STREAM means a TCP socket)
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
try:
# Connect to server and send data
sock.connect((address, port))
return True
except socket.error as e:
print("Something went wrong connecting to the server: %s" % e)
return False
finally:
sock.close()
check_server('www.example.com', 80)
If our function returns True, that signifies there isn’t an issue with the server or the port. If it returns False, then there may be a server-side problem causing Errno 111.
Remember, the symbiotic relationship between HTTPConnection and server lies at the heart of this issue. Understanding this congruence allows us to troubleshoot more efficiently.
For further insights refer to socket — Low-level networking interface – Python documentation.Diving deeper into the issue, the
NewConnectionError
xxxxxxxxxx
NewConnectionError
associated with the
urllib3.Connection.HTTPConnection object
xxxxxxxxxx
urllib3.Connection.HTTPConnection object
is a common Python exception that arises due to issues in establishing a new connection. More specifically, when the backend server or the recipient of the requests refuses the connection initiation, you may come across this error. The [Errno 111] tag appends itself to signify that the connection has indeed been refused.
This refusal can stem from multiple reasons:
To better illustrate the problem and possible solutions, let’s take a look at an example where we are trying to use urllib3 to send a HTTP request:
import urllib3 http = urllib3.PoolManager() try: r = http.request('GET', 'http://localhost:8000') except urllib3.exceptions.NewConnectionError as e: print("OOPS!! Connection Error. Make sure you are connected to Internet. Technical Details given below.\n") print(str(e)) except urllib3.exceptions.MaxRetryError: print("OOPS!! MaxRetry Error!")
xxxxxxxxxx
import urllib3
http = urllib3.PoolManager()
try:
r = http.request('GET', 'http://localhost:8000')
except urllib3.exceptions.NewConnectionError as e:
print("OOPS!! Connection Error. Make sure you are connected to Internet. Technical Details given below.\n")
print(str(e))
except urllib3.exceptions.MaxRetryError:
print("OOPS!! MaxRetry Error!")
In the above code snippet, we’re making a GET request to the local server at ‘http://localhost:8000’.
Should there occur a
NewConnectionError
xxxxxxxxxx
NewConnectionError
, the error handling mechanism nestled inside the
except
xxxxxxxxxx
except
clause will prompt, allowing us to comprehend whether the connection was denied due to server issues, incorrect endpoints, or network problems.
Patching these errors involves troubleshooting based on the specific cause. You can try restarting your server, ensuring the correctness of your endpoint, checking your network and firewall settings and ensure sufficient spacing between consecutive requests to prevent overwhelming the server.
Referencing an external resource like StackOverflow discussions may also seed insights and popularly accepted solutions. This familiarity with various scenarios and solutions associated with the
NewConnectionError
xxxxxxxxxx
NewConnectionError
catalyzes efficient debugging and enhanced coding skills.