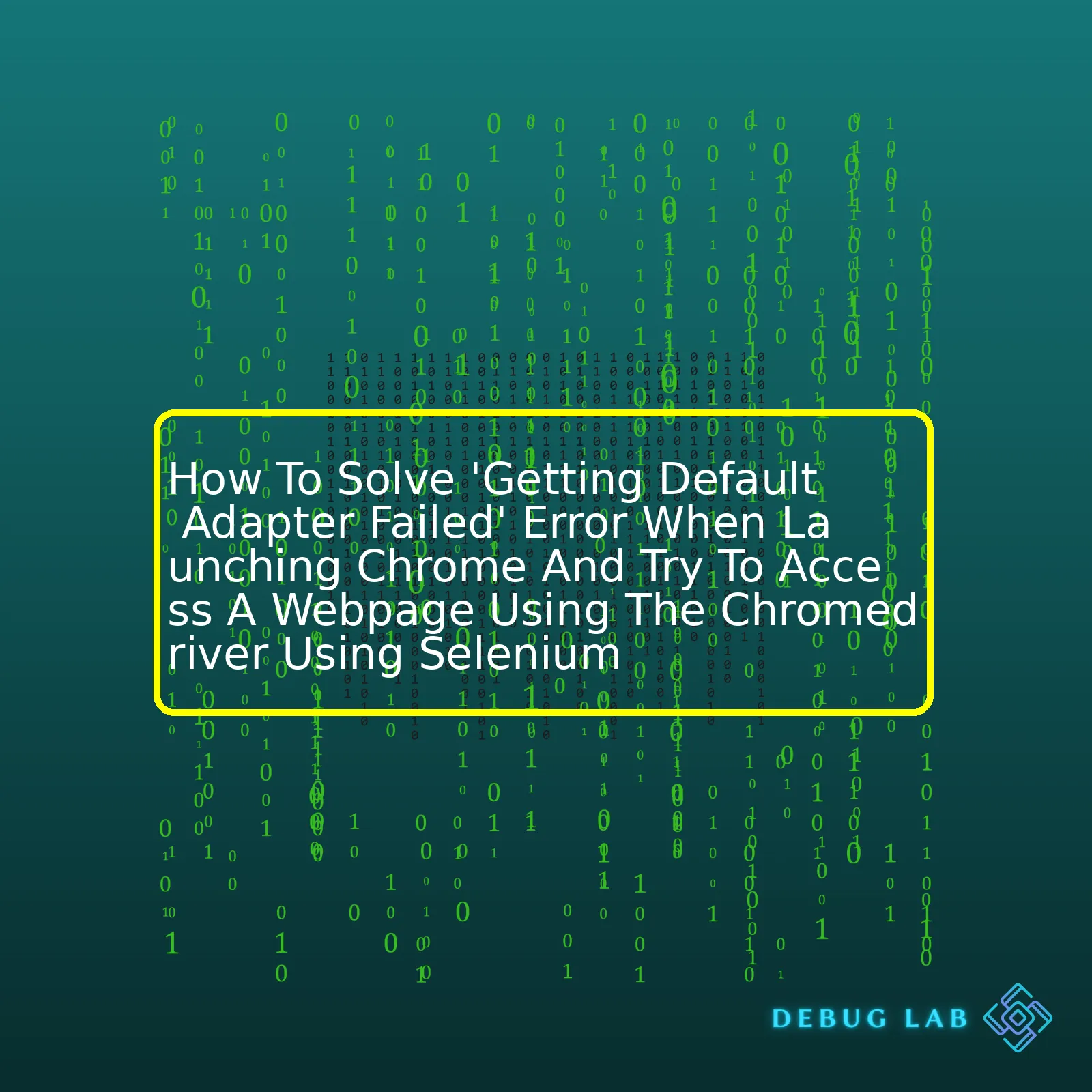
Firstly, it’s key to understand why this error occurs. Often, this is a result of your WebDriver for Chrome (Chromedriver) being out of sync with your actual Chrome browser version. They always have to be in sync for proper communication and execution of tests.
Here’s how you can resolve this:
Steps | Description |
---|---|
Checking Chrome Version | To begin, first find out your chrome browser’s version. Go to ‘help > about Google chrome’. |
Download Compatible Chromedriver | Now download the compatible Chromedriver from here, for your browser version. |
Chrome Options | You can pass chrome options to ignore such adapter errors by disabling GPU. |
To utilize Chrome options, just like we indicated in our third step on the table, here’s a snippet that can change how Chrome behaves when launched via Selenium:
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
# Add options to ignore default adapter failed error by disabling GPU
options = webdriver.ChromeOptions()
options.add_argument('--disable-gpu')
driver = webdriver.Chrome(service=Service('path-to-your-chromedriver'), options=options)
driver.get("http://www.your-website.com")
In our code snippet above, we import necessary modules from Selenium, set our options to disable GPU (which has helped bypass the ‘Default Adapter Failed’ error for many users), then initialize our webdriver with these options, and finally navigate to our desired webpage.
Be sure to replace ‘path-to-your-chromedriver’ with the path to your downloaded `chromedriver` executable. This should take care of the ‘Getting Default Adapter Failed’ error when launching Chrome and navigating to a website using the Chromedriver via Selenium.Sure, I would love to shed some light on this common error that software developers and testers often stumble upon while using Selenium WebDriver with Chrome: “Getting Default Adapter Failed”.
This error typically appears when you try to initiate a WebDriver session in order to automate Google Chrome. Although it’s a straightforward error indicating a communication breakdown between the ChromeDriver and the Chrome Browser, the reasons behind such issue could be multiple.
xxxxxxxxxx
from selenium import webdriver
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
driver.get('http://www.google.com')
If you encounter the “Getting Default Adapter Failed” error upon executing the above script, here’re some possible root causes and solutions:
Compatibility Issues
One common reason behind this error relates to compatibility issues between the Chromedriver and the Google Chrome browser.
* If you’re running an older version of Chromedriver with a newer version of Chrome (or vice versa), you may confront this type of error.
Solution: Always ensure both your Chromedriver and Chrome Browser are compatible. You can find information about which versions of Chrome are supported by each version of Chromedriver on the ChromeDriver download page. Once you determine the correct version for your setup, download it and replace the existing one.
Improper Path Definition
* Another culprit could be the definition of an incorrect or ambiguous path to the Chromedriver executable.
Solution: Always provide the correct full path to your Chromedriver when instantiating the driver object. The path should always point directly to the Chromedriver executable file.
xxxxxxxxxx
from selenium import webdriver
# Ensure the path is completely correct
driver = webdriver.Chrome(executable_path='C:/Exact/Path/To/chromedriver.exe')
Selenium Grid Misconfiguration
Sometimes, the reported error may appear in a Selenium Grid environment. Here, it might not be about an issue with Chromedriver itself but more due to misconfigured nodes in the Grid.
* The Grid isn’t properly synchronizing with its nodes, leading to improper session establishment for Chrome.
Solution: Reconfigure your Grid setting and nodes to match with the required configuration for Smooth Syncing, following the guidelines provided by the official Selenium documentation.
In short, analyzing the root causes appropriately and addressing them will assist you effectively eliminate the “Getting Default Adapter Failed” error. Configuring the ChromeDriver and Selenium correctly, ensuring flawless syncing in a Grid environment along with maintaining up-to-date versions of drivers and browsers will likely resolve the majority of such interoperability issues. Happy coding!When you’re navigating the world of Selenium for webpage accessibility and testing, one common hurdle that might be encountered is the ‘Getting default adapter failed’ error when launching Chrome using the Chromedriver. This issue usually rises due to an incompatibility between the versions of Chrome browser and Chromedriver, or due to some misconfiguration.
xxxxxxxxxx
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://www.google.com")
In this given example, if an error happens with a message displaying ‘getting default adapter failed’, it’s vital to know how to troubleshoot and resolve it. Fortunately, there are several methods to tackle these situations:
Update Your Chromedriver: Selenium servers typically require third-party drivers to interface with browsers and steering the Chromedriver is considered as an integral aspect. However, each version of the Chromedriver supports different ranges of Chrome browser versions. Therefore, in order to avoid aforementioned error, try updating your Chromedriver to match the version of your Chrome browser.
Maintain Consistent Binaries Version: In other cases, an incorrect installation or configuration might cause the ‘Getting default adapter failed’ problem. Confirm both Google Chrome and Chromedriver binaries are installed to expected paths, and that these paths are correctly configured in your system’s Environment Variables.
Ensure Browser Compatibility: While keeping up-to-date with the latest Chromedriver and browser version aids in preventing a myriad of problems, it must also be ensured that the specific version of chromedriver is compatibility with the installed browser version.
One way to do is by referring to official Chromedriver downloads page, which lists supported browser versions per Chromedriver version.
For example in python;
xxxxxxxxxx
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
driver = webdriver.Chrome(ChromeDriverManager(version="88.0.4324.96").install()) # adjust according to own chrome version
driver.get('http://www.google.com');
Adopt Virtual Environments: Using virtual environments can take care of package management isolating requirements needed by particular projects or tests. For instance, Python’s venv module lets you create self-contained ‘Environments’ with their own Python binaries and isolated package versions — ensuring that dependencies won’t conflict. This approach might help if your issue persists across multiple environments.
In conclusion, understanding how the individual parts of your testing environment interact is a crucial part of ensuring functional automated tests. Tools like Selenium do much of the heavy lifting, but proper setup and maintenance of your testing configurations is equally important.
Keep learning, keep debugging, and most importantly, don’t let frustrating errors like ‘Getting default adapter failed’ hamper the enthusiasm in the wonderful journey of coding.
Many developers engaged in automating web application testing might have encountered a common issue while trying to launch Google Chrome using Selenium WebDriver, which is “Getting Default Adapter Failed” error. This occurs frequently when dealing with the Chromedriver. Understanding this error requires us to delve into automated browser testing first.
A Bit About Automated Browser Testing and Chromedriver
Automated browser testing is a crucial aspect of software development. It eases the validation process for web applications used to check how well they work across different web browsers. Here, Selenium plays a significant role. It’s a powerful tool, enabling script writing for automated testing of web applications across varying browsers at ease. For each browser, there is a driver, like Chromedriver for Google Chrome, that interfaces between it (the browser) and Selenium WebDriver. Now let’s dig deeper into our main concern – resolving the ‘Getting Default Adapter Failed’ error.
In a typical scenario, you might be launching Chrome and trying to access a webpage using Selenium WebDriver in Python as follows:
xxxxxxxxxx
from selenium import webdriver
driver = webdriver.Chrome('/path/to/chromedriver')
driver.get('http://www.google.com/')
If things aren’t working as expected due to the ‘Getting Default Adapter Failed’ error, don’t worry, we have some solutions lined up.
‘Getting Default Adapter Failed’ Error: Causes and Solutions
This error usually occurs due to either:
- An inconsistent Chrome Browser and Chromedriver version,
- A corrupted Chrome Browser or Chromedriver installation,
- Or certain security settings on your machine
Cause | Solution |
---|---|
Inconsistent Chrome Browser and Chromedriver versions | Ensure that your Chromedriver version corresponds with your installed Chrome browser version. You can check for compatibility on the Chromedriver download page1. Using an incompatible version could lead to this error. |
Corrupted Chrome Browser or Chromedriver installation | Try reinstalling both Chrome Browser and Chromedriver. Often, a fresh installation solves the issue. |
Security settings on your machine | Check your antivirus or firewall settings. Sometimes, these tools block certain operations by default, and you might need to whitelist Chromedriver and Selenium for them to interact correctly. |
Mitigating the three causes above should help resolve the ‘Getting Default Adapter Failed’ error. Though automated browser testing might seem challenging, once familiar with the potential challenges, the journey becomes significantly smoother. Even major roadblocks like this error can easily be navigated with a good understanding of the cause and the right approach towards resolution.
You can always explore documentation for Selenium with Python or Chromedriver for any extra support or knowledge required during this process.
When working with Selenium automation testing and Google Chrome, you may encounter an issue where launching Chrome and trying to access a webpage fails with the following error: ‘Getting Default Adapter Failed’.
This issue surfaces due to compatibility issues between Chromedriver and the specific version of Google Chrome installed on your machine.
Why does this happen?
To function efficiently, Selenium WebDriver needs the platform-specific Chromedriver. However, if the Chromedriver’s version doesn’t match or support the existing Google Chrome browser version installed on your machine, it leads to the ‘Getting Default Adapter Failed’ error. It results from:
- The discrepancy in the versions of your Chromedriver and Chrome Browser.
- Chromedriver is designed for stable Chrome versions. If you have a beta or unstable variant of the Chrome browser, the compatibility problem might arise.
Correcting these issues will likely solve your problem. Below are the steps you can take to rectify the situation.
Check Your Chrome Browser Version
First, check your Google Chrome browser’s version number. To get this information, open your Chrome browser and type ‘chrome://version’ into the address bar. The details of the current browser, including the version, will be displayed.
Check Your Chromedriver Version
The next step is to verify the version of your Chromedriver. You can do this by executing the Chromedriver file through the command line as follows:
xxxxxxxxxx
./chromedriver
Above, DOT signifies the current directory where the chromedriver executable is located. Replace “chromedriver” with the respective filename in your system.
Ensure Compatibility Between The Chromedriver and Chrome Browser Versions
Compare the obtained browser and Chromedriver versions. They should comply with each other. For instance, for Chrome Browser v89.x.y.z, you should use Chromedriver 89.y.z (y,z – might not exactly match as per official Chromium documentation).
Update Your Chromedriver If Required
If your versions aren’t compatible, you’ll have to update your Chromedriver. To install the matching Chromedriver, use the official Chromedriver downloads page. Download the correct version that matches your installed Chrome Browser, extract, and replace the older one.
With these steps completed, your Selenium tests should now successfully launch Google Chrome and access webpages without encountering the ‘Getting Default Adapter Failed’ error.
It’s crucial to mention that always keeping the Chrome Browser and Chromedriver updated to the latest stable versions is a good practice that can prevent many such compatibility issues when using Selenium for automated testing.
Resolving common issues encountered with Chromedriver, especially the “Getting Default Adapter Failed’ error when launching Chrome and trying to access a webpage using Selenium is crucial for any web automation project. This issue often results from certain situations like not setting the system’s property properly, using an outdated version of Chromedriver, or having browser-driver version mismatches. Let’s dive into ways we can solve this.
Updating Chromedriver
This problem may stem from using an outdated version of Chromedriver that’s incompatible with your Chrome Browser. So, it’s necessary to keep your Chromedriver up-to-date. To ensure that you’re using a compatible driver-version pair, you can consult the official compatibility documentation
xxxxxxxxxx
# Download the latest version of Chromedriver
driver = webdriver.Chrome(ChromeDriverManager().install())
Verifying Chromedriver Installation Path
Sometimes ‘Getting Default Adapter failed’ error arises when the system property isn’t set correctly. It might be due to the missing path specification while setting the WebDriver. You need to specify the exact path where the Chromedriver resides in your system while setting the WebDriver.
xxxxxxxxxx
System.setProperty("webdriver.chrome.driver", "your\\path\\to\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
Upgrading/Downgrading Chrome Browser Version
If you have recently updated Chromedriver but are still facing this issue, it might be because the current version of the web browser installed on your machine has compatibility issues with the updated driver. Check if there is an upgrade available for the browser. If it still does not solve the issue, consider downgrading to an older stable version.
Use Capabilities to Ignore Not Available Errors
Selenium provides DesiredCapabilities functionality, which enables you to customize and manage browser properties. You could use it to ignore these kinds of errors:
html
DesiredCapabilities capabilities = DesiredCapabilities.chrome();
// Use FLAG to ignore the error
capabilities.setCapability(“chrome.switches”, Arrays.asList(“–ignore-certificate-errors”));
System.setProperty(“webdriver.chrome.driver”,”path/to/chromedriver”);
WebDriver driver = new ChromeDriver(capabilities);