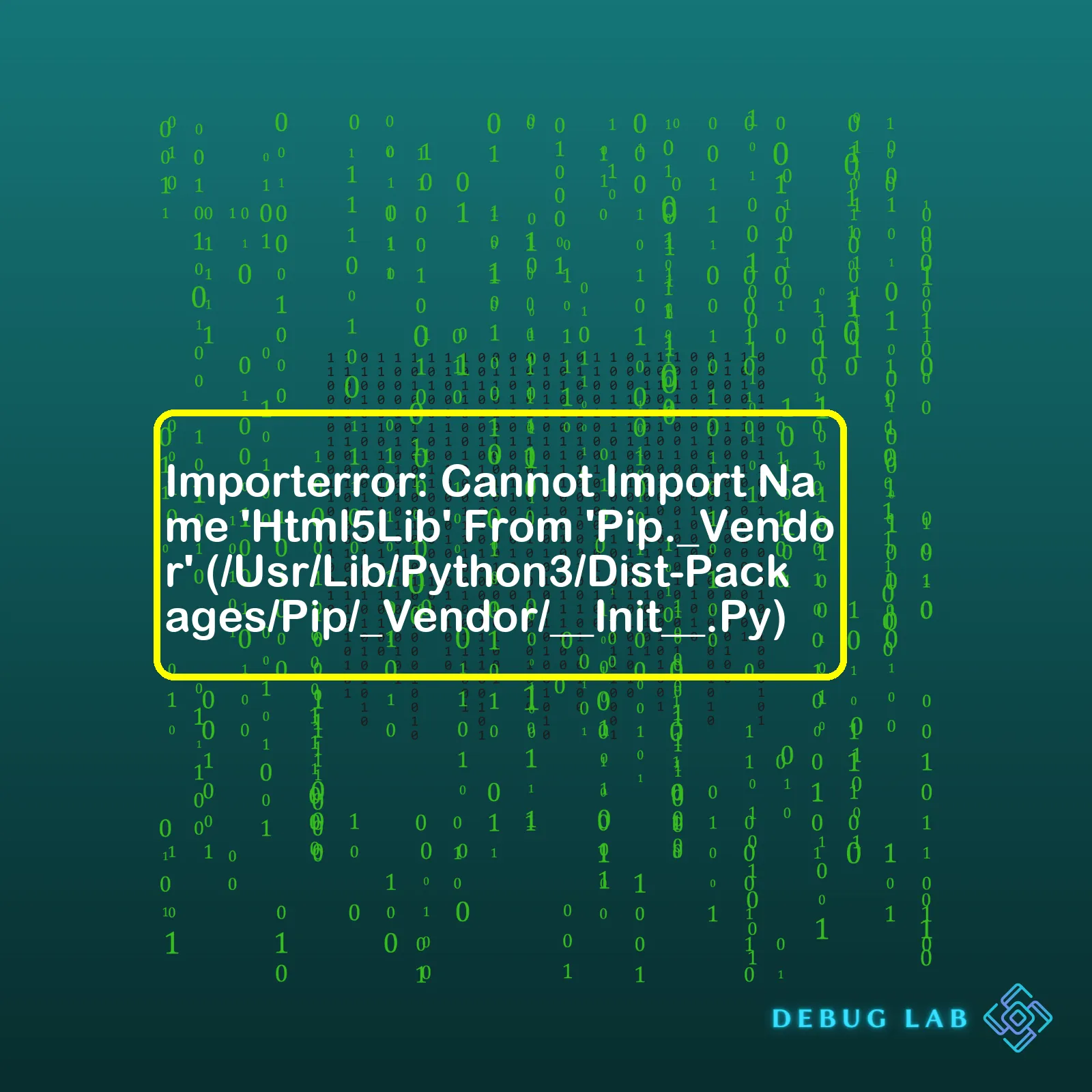
Error | Cause | Solutions |
---|---|---|
ImportError: Cannot Import Name ‘Html5Lib’ | The html5lib library isn’t correctly installed or there’s an environment path issue | Reinstall html5lib or correct Python Environment Path |
This table describes a common Python error – `ImportError: Cannot Import Name ‘Html5Lib’`. This shows up when you’re trying to import the Html5Lib module from pip._vendor package in your Python code, but it does not find it. This can generally arise from one of two scenarios, or both:
1. The Html5lib library is not correctly installed. This is a Python library that provides you with a pure-python library for parsing HTML. It is designed to conform to the WHATWG HTML specification, as closely as possible.
2. There’s a problem concerning the Python environment path. When Python imports a module, it first checks the module registry (sys.modules) to see if the module is already imported. If that’s the case, Python uses that existing object from sys.modules. If not, then it proceeds to import the module; loads the module and run the top-level code in it (usually the definitions of functions and class). It may be the case that the Html5lib module is located on a path that’s currently not in the Python’s search path list, leading to our ImportError.
Suggested solutions to this issue include:
• Reinstalling Html5lib, making sure all its dependencies are fulfilled and it’s properly configured with Python. You can do this using
pip install --upgrade --force-reinstall html5lib
.
• Checking and correcting the Python Environment Path, assuring it includes the directory where Html5lib is installed. You can verify the current Python path during runtime by importing the sys module and printing out the content of sys.path variable i.e.
xxxxxxxxxx
import sys; print(sys.path)
. If the path of Html5lib is not among them, update Python’s path(s) accordingly.
Keep in mind that Python, its packages, and third-party modules should all be properly configured and compatible to ensure smooth functioning. For example, the version of Html5lib should be compatible with the version of Python you’re using. Always refer to the official [documentation](https://html5lib.readthedocs.io/en/latest/) or trusted sources when addressing such issues to avoid cascade errors or malfunctions.The error message ‘
xxxxxxxxxx
Importerror: Cannot Import Name 'Html5Lib' From 'Pip._Vendor'
‘ appears when Python cannot find the specified module in its import paths. This is a common Python error and can be caused by several factors such as:
– The module is not installed.
– The module is installed but not in a directory Python checks for modules.
– The module’s name has been misspelled.
Understanding this specific error requires knowledge of how Python manages imports and packages.
When we attempt to import a module, Python first searches a list of directories defined in sys.path, which is initialized from these locations in the following order:
– The directory containing the input script (or the current directory when no file is specified).
– PYTHONPATH (a list of directory names, with the same syntax as the shell variable PATH).
– The installation-dependent default directory.
Analyzing the Issue for
xxxxxxxxxx
'Html5Lib'
:
The pip._vendor package is used by pip, the Python package installer, to bundle its dependencies and reduce conflicts between different versions or installation methods. Pip embeds its dependencies such that they are isolated from other packages installed in the environment. Hence, modules inside pip._vendor are not meant to be available for import.
For
xxxxxxxxxx
'import error: Cannot import Name 'Html5Lib' From 'Pip._Vendor'
, it is likely that another Python package or script tried to directly import the html5lib from pip’s vendored packages, resulting in this error. This is incorrect because those vendored packages do not form part of the public API.
Here’s how an import should look:
xxxxxxxxxx
from html5lib import HTMLParser
To resolve this error, you will need to install the required package in your project’s environment, rather than trying to access it through pip’s bundled dependencies. In your case, that’s the package
xxxxxxxxxx
'HTML5lib'
.
You can install
xxxxxxxxxx
'HTML5Lib'
using pip:
xxxxxxxxxx
pip install html5lib
For pip install to work, you require internet access and also pip must be correctly installed on your Python platform. Once the package is installed, Python should ideally be able to find and load the needed module without throwing any import errors.
Takeaways:
Remember, when encountering import errors:
– Verify that the package is installed.
– Make sure the correct name is being used when attempting to import the module.
– Avoid importing packages from
xxxxxxxxxx
pip._vendor
directly.
Always approach any ImportError by understanding what exactly Python is attempting to import, ensuring it’s correctly installed in your usable path, and referred to by the right name.When you experience the error
xxxxxxxxxx
Importerror: cannot import name 'html5lib' from 'pip._vendor'
, it is due to Python’s inability to locate the specified module. The code has attempted to import a module named ‘html5lib’ located in pip._vendor, but Python couldn’t find this module – hence yielding the ImportError.
Where does ‘html5lib’ come from?
Html5lib is a pure-python library for parsing HTML documents. It is designed to conform to the WHATWG specifications and has an interface that is inspired by Beautiful Soup. Html5lib is often used in web scraping or when dealing with HTML content in your Python scripts.[source]
What could be causing this error?
The reasons for encountering this error can range from:
* Your environment doesn’t have the html5lib module installed.
* You might be using a version of pip that doesn’t include html5lib in pip._vendor.
* A conflict between python installations.
* Incorrectly set PYTHONPATH environment variable.
How do we diagnose and solve this problem?
1. Check if html5lib is installed
First make sure if html5lib module is installed in your python environment. If it isn’t, it needs to be installed. You can verify its presence by running:
xxxxxxxxxx
pip show html5lib
In case it’s not installed, you can add it to your environment using:
xxxxxxxxxx
pip install html5lib
You may want to use –user flag if you are not using a virtualenv and want to install the package for your user only:
xxxxxxxxxx
pip install --user html5lib
2. Check pip version
It’s also possible that your version of pip doesn’t include html5lib in pip._vendor. To check your pip version you can run:
xxxxxxxxxx
pip --version
Then you can either look through pip’s documentation or source code on Github to see whether html5lib should be present. In case your pip version doesn’t support it, you can upgrade pip:
xxxxxxxxxx
pip install --upgrade pip
3. Clean up your Python Environment
There may be conflicts between multiple installations of Python or multiple environments on your system leading to the usage of incorrect or unexpected versions of Python packages. There might also be left-over files from previous python installations conflicting with the current one.
One way to clean up your environment is to uninstall Python, delete any remaining files and directories related to Python, then reinstall Python.
Another way is to use a virtual environment which allows you to isolate different Python environments on the same machine, thus avoiding conflicts between packages.
4. Examine PYTHONPATH
PYTHONPATH is an environment variable that tells Python where to look for modules to import. If PYTHONPATH is incorrectly set to a directory that does not contain html5lib, then import will fail.
Try printing out PYTHONPATH to examine if it’s correct:
xxxxxxxxxx
echo $PYTHONPATH
To temporarily amend PYTHONPATH you can use the export command in unix systems, for windows use the set command:
Unix:
xxxxxxxxxx
export PYTHONPATH=/new/path
Windows:
xxxxxxxxxx
set PYTHONPATH = C:\new\path\
Remember that these changes won’t persist after your session ends so you may need to additionally amend your system/enviroment variables for a perment amendement.
Solving import errors can be quite convoluted at times since there are multiple underlying factors involved. But rest assured, with a systematic approach to diagnosing the issue, a solution can definitely be found!HTML5lib is a library for parsing HTML documents, including those with malformed markup. In Python programming, it’s inevitably an integral tool in web scraping and data extraction from websites. PIP (Pip Installs Python) on the other hand, is your go-to tool when you want to install python packages. The pip._vendor directory contains packaged versions of libraries that pip depends on. Therefore, html5lib being a python module can be installed using pip.
The error “ImportError: Cannot import name ‘html5lib’ from ‘pip._vendor'” usually happens in Python when there are conflicts with dependencies or the paths to the specific modules aren’t correctly defined. This means that your Python environment cannot access or find the required html5lib package from its installed location.
To ensure that html5lib is correctly installed, you should use the pip command:
xxxxxxxxxx
pip install html5lib
However, if you’re seeing this error message, it implies that Python is attempting to import html5lib from the pip._vendor directory which isn’t quite right as html5lib is a separate installable package itself not a vendor under pip.
Sometimes, certain Python packages like BeautifulSoup4 requires the installation html5lib for their operation. Here’s how you can import html5lib when using BeautifulSoup4
xxxxxxxxxx
from bs4 import BeautifulSoup
soup = BeautifulSoup(some_markup, 'html5lib')
A key point here is Python’s namespacing mechanism – by importing ‘html5lib’ under ‘pip._vendor’, the interpreter looks for an html5lib module within a larger package. Although pip does contain a _vendor subpackage, html5lib is not part of this package, hence the error.
In summary;
– HTML5lib is a pure-Python library that’s used to parse HTML documents which can be installed using pip.
– pip._vendor is specifically for packaged versions of libraries only pip depends on.
– You encounter the ImportError when there is a conflict in namespacing, resulting in Python trying to import html5lib from within pip._vendor where it doesn’t exist rather than as a standalone package.
To overcome such challenges with import errors, always check and validate:
– The package names for typing errors.
– If the package has been correctly installed via pip.
– If need be, provide absolute references while importing to avoid any ambiguity. Use sys.path to ensure modules are being imported from the correct locations.
It’s always beneficial to familiarize yourself with Python’s namespacing rules and the functionality of key tools like pip and Imported Modules. To get more insights into the import system of Python, please see Python’s official documentation page.
The ‘ImportError: Cannot import name ‘html5lib’ from ‘pip._vendor” can take place due to a host of reasons. In order to better explain the causes and ways to circumvent them, it is vital to have a fundamental understanding of pip.
Pip is a Python-based package manager that allows developers to install community-developed packages for their Python programs (official documentation). The pip._vendor directory contains packages that pip depends on for its functionality.
Despite being critical for the smooth operation of Python processes, certain situations lead to import errors from pip._vendor.
– One of the chief scenarios leading to this error involves pip itself—specifically, if pip is incorrectly or partially installed. This could cause pip’s dependencies to be misconfigured, causing problems importing specific modules. To rectify this, reinstallation or system-wide upgrade of pip may prove beneficial using this command:
xxxxxxxxxx
python -m pip install --upgrade pip
– Another predominant scenario includes having multiple Python versions installed on your system. Dependencies for different Python projects may differ based on the project’s Python version. If an incorrect Python version has been referenced during an installation, it might lead to import errors. You can check your Python version with this command:
xxxxxxxxxx
python --version
– The last reason pertains to the python environment itself—it might be contaminated due to the installation of incompatible packages or deprecated methods. This can cause the import error, as the Python interpreter cannot locate the required packages or libraries. Here, creating isolated Python environments using virtualenv (pip official documentation) can serve as a quick fix.
The ‘cannot import name html5lib from pip._vendor’ error specifically arises when the Python library html5lib is missing from the pip_vendor directory or is inaccessible. The latter may occur if conflicting Python environments are preventing proper references.
In order to resolve these conflicts and avoid import errors:
– Ensure you work within a single, isolated Python environment.
– Make sure you’ve correctly installed all necessary libraries, including html5lib, by using pip:
xxxxxxxxxx
pip install html5lib
– Investigate your code base for any instances where incompatible packages might be clogging up the Python environment. If found, uninstall these conflicting packages using pip:
xxxxxxxxxx
pip uninstall [package-name]
These steps properly outline the problem arised and provides a comprehensive method in resolving the import error not only involving html5lib but the general ImportError from pip._vendor. Always remember, understanding the underlying issue will guide you through debugging, and allow you to proceed in the most efficient way possible.Ah, the dreaded import error. In coding, there’s nothing more frustrating than encountering an ImportError that grinds your progress to a halt. Let’s dive into this particular one – `ImportError: Cannot import name ‘html5lib’ from ‘pip._vendor'(/usr/lib/python3/dist-packages/pip/_vendor/__init__.py)`. It’s not as bad as it looks!
Firstly, this problem generally arises when you attempt to import the `html5lib` module which isn’t correctly installed in your Python environment. The error indicates that Python can’t find this package in the place it’s looking (namely, pip’s _vendor directory). And here’s how you can resolve it.
1. Reinstall html5lib:
The issue likely lies with an unsuccessful or incomplete installation of the `html5lib` package. A straightforward solution is to reinstall it.
You can start by uninstalling any previous instances using pip:
xxxxxxxxxx
pip uninstall html5lib
Once this process has finished, you can reinstall `html5lib`.
xxxxxxxxxx
pip install html5lib
If you’re working in a virtual environment, don’t forget to activate it before running these commands!
However, if reinstalling `html5lib` doesn’t do the trick, don’t worry! There’s another solution on the horizon.
2. Install an older version of pip:
One possible reason for the error might be a conflict between newer versions of pip and certain libraries. Downgrading pip to an earlier version often remedies this issue.
Now, let’s see this in action!
xxxxxxxxxx
pip install pip==20.2.4
This command will downgrade your current pip version to 20.2.4. Then, try to import `html5lib` again.
Remember, the key is to respond to the import error by examining the issue through various angles: trying to reinstall `html5lib`, downgrading pip, starting afresh in a new virtual environment, and so on. Don’t let a little `ImportError` throw a wrench in your coding path!
3. Isolate Your Environment With Virtualenv:
Another way to handle dependencies and avoid such errors is via isolating your Python environment. This can be achieved with tools like `virtualenv`.
xxxxxxxxxx
pip install virtualenv
Then, create a new virtual environment:
xxxxxxxxxx
virtualenv venv
And then activate it:
xxxxxxxxxx
source venv/bin/activate
While the virtual environment is activated, you can now install html5lib:
xxxxxxxxxx
pip install html5lib
By creating isolated environments, each project can have its own dependencies, regardless of what dependencies every other project might need. These kinds of practices are known as good DevOps hygiene.
These approaches will comfortably iron out issues relating to `ImportError: Cannot import name ‘html5lib’`.
I must remind you, though, that despite these headaches, dependency management is crucial within the world of programming. But hey, no one said it would be easy, but that’s part of why we love it, right? Good luck, fellow coder!This error arises due to a conflict between Python’s system packages and pip. It typically occurs when you’re using Ubuntu or other Linux distributions that use python3-pip in global directories.
When ‘Html5Lib’ cannot be imported from ‘Pip._Vendor’, it’s usually because this library isn’t installed correctly, it’s not available in the Python path, or there are multiple copies of pip installed causing confusion. Here’s how I would approach fixing this issue.
Strategy One: Uninstalling and Reinstalling Pip
Python’s pip manages dependencies for your package installation. If any discrepancies or incompatibilities arise, uninstalling and reinstalling pip could resolve the issues.
xxxxxxxxxx
sudo apt-get remove python3-pip
sudo apt-get install python3-pip
Strategy Two: Using Virtual Environments
Virtual environments can help to create isolated Python environments, effectively eliminating potential conflicts between system packages and local project dependencies. Here’s an example of creating a virtual environment using the venv module:
xxxxxxxxxx
python3 -m venv env
source env/bin/activate
Strategy Three: Use Pip’s User Install Option
Installing Python packages locally (instead of globally) can help avoid conflicts with system-level packages. Pip provides a user install option for this purpose.
xxxxxxxxxx
pip3 install --user html5lib
Before moving onto the next solutions, verify if the ‘Importerror: Cannot Import Name ‘Html5Lib’ From ‘Pip._Vendor’ error is resolved. If it continues, consider these advanced solutions.
Advanced Solutions
Strategy Four: Modify Python’s Path
Mostly, ‘html5lib’ is present in some directory, but Python’s PATH doesn’t include that directory. In such cases, update the sys.path variable
xxxxxxxxxx
import sys
sys.path.append('')
Lastly, remember, it’s always a good idea to periodically update your Python and pip versions, as most issues get fixed in newer updates.
Bear in mind that messing with the system Python and its packages can lead your system to an unstable state. So, always take a note of the changes you’re doing to revert them back if required.
Here are references that might help:
An `ImportError` usually means that a module can’t be found. In your specific case, the Exception is complaining about not being able to import the name ‘html5lib’. Html5lib is a standalone pure Python HTML5 parser, which is required by pip for several operations.
Here are several alternative ways you could resolve this ImportError:
1. Install or Update html5lib
This issue might arise if you don’t have html5lib installed in your environment. Install it using PIP:
xxxxxxxxxx
pip install html5lib
Or update using:
xxxxxxxxxx
pip install --upgrade html5lib
2. Verify Your Installation
You might want to verify whether or not ‘html5lib’ is already installed in your system. If it’s installed, but in a different location than expected by ‘pip._vendor.__init__.py’, you’ll need to add the install location to your python PATH. You can print paths from the PYTHONPATH as follows:
xxxxxxxxxx
import sys
print(sys.path)
3. Use Virtual Environment:
A virtual environment can help provide a separate context for your project, thus preventing it from affecting the entire system and vice versa. Thus, this is a neat solution to avoiding dependency problems specially when dealing with different versions of packages.
To create a new virtual environment, use:
xxxxxxxxxx
python3 -m venv myvenv
Next, activate the virtual environment using:
On UNIX or MacOS:
xxxxxxxxxx
source myvenv/bin/activate
Or On Windows:
xxxxxxxxxx
.\\myvenv\\Scripts\\activate
Then try reinstalling ‘html5lib’ inside this virtual environment.
4. Use an Earlier Version of html5lib:
If updating html5lib does not work, perhaps your version of pip is incompatible with the latest version of html5lib. Downgrade your html5lib installation to a previous known working version, e.g.,
xxxxxxxxxx
pip uninstall html5lib
xxxxxxxxxx
pip install html5lib==0.9999999
5. Check Dependencies:
Check if another package called ‘six’ exists. If ‘six’ is missing, ‘html5lib’ won’t work. Try installing it:
xxxxxxxxxx
pip install six
6. Check Python Interpreter:
Ensure you’re running the correct version of python where ‘html5lib’ is expected to be available. Use ‘which python’ or ‘python –version’ to confirm. Sometimes, we tend to use different interpreters implicitly and it may cause such unknown errors.
Remember, all packages should reside in ‘Lib/site-packages/’ under Python directory in your virtual environment.
It’s also worth noting that these actions would require administrative privileges on some systems.
When working with modern Python development workflows, troubleshooting ImportErrors is quite often a matter of understanding your Python environment, particularly when working across different projects and dependencies.
For future reference, you might find the official Python documentation on the import system useful.The error message “ImportError: Cannot import name ‘html5lib’ from ‘pip._vendor'” is a relatively common issue that can be a stumbling block for users navigating the Python ecosystem. The crux of this problem lies in your Python environment’s inability to locate or import the ‘html5lib’ module specifically from the ‘pip._vendor’ package.
To dissect this a bit more, ‘html5lib’ is a pure-Python library designed to parse HTML documents, including ones with malformed or incorrect markup. It forms an integral part of web scraping and other data parsing tasks in Python.
On the other hand, ‘pip._vendor’ is a package that includes libraries bundled with ‘pip’. In order to avoid causing a conflict if you already have these libraries installed in your system, when pip needs one of these libraries, it uses the one in ‘_vendor’. The import error message is implying that Python isn’t able to find ‘html5lib’ in this particular location.
One potential solution for this complexity is to install ‘html5lib’ directly. As depicted below,
xxxxxxxxxx
pip install html5lib
Alternatively, you might require updating or reinstalling ‘pip’ to ensure ‘html5lib’ resides within its vendor dependencies. Here’s how you’d do it,
xxxxxxxxxx
python -m pip install --upgrade pip
Sometimes, discrepancies between different versions of Python can cause such issues. If you’re using multiple Python environments, consider explicitly running pip pertaining to Python3.
xxxxxxxxxx
pip3 install html5lib
Realize that Python environments can often be intricate to juggle with. Enforcing good practices like isolating your project into a virtual environment could potentially save you from such errors. Tools like virtualenv and venv make this process smooth and seamless. Get started with Python virtual environments here.
It is extremely essential to remember that resolving import errors often involves understanding why they occur and the architecture of the package, tool, or library you are trying to use (in this case, pip and ’html5lib’). A deeper dive into both pip’s architecture can be found here, as well as html5lib’s documentation here .
This error indeed encapsulates the complexity of managing packages and dependencies in Python, but armed with the best practices highlighted in this discussion, you should be equipped to navigate these quandaries like a pro coder. Feel free to reach out in case the uncertainties persist.