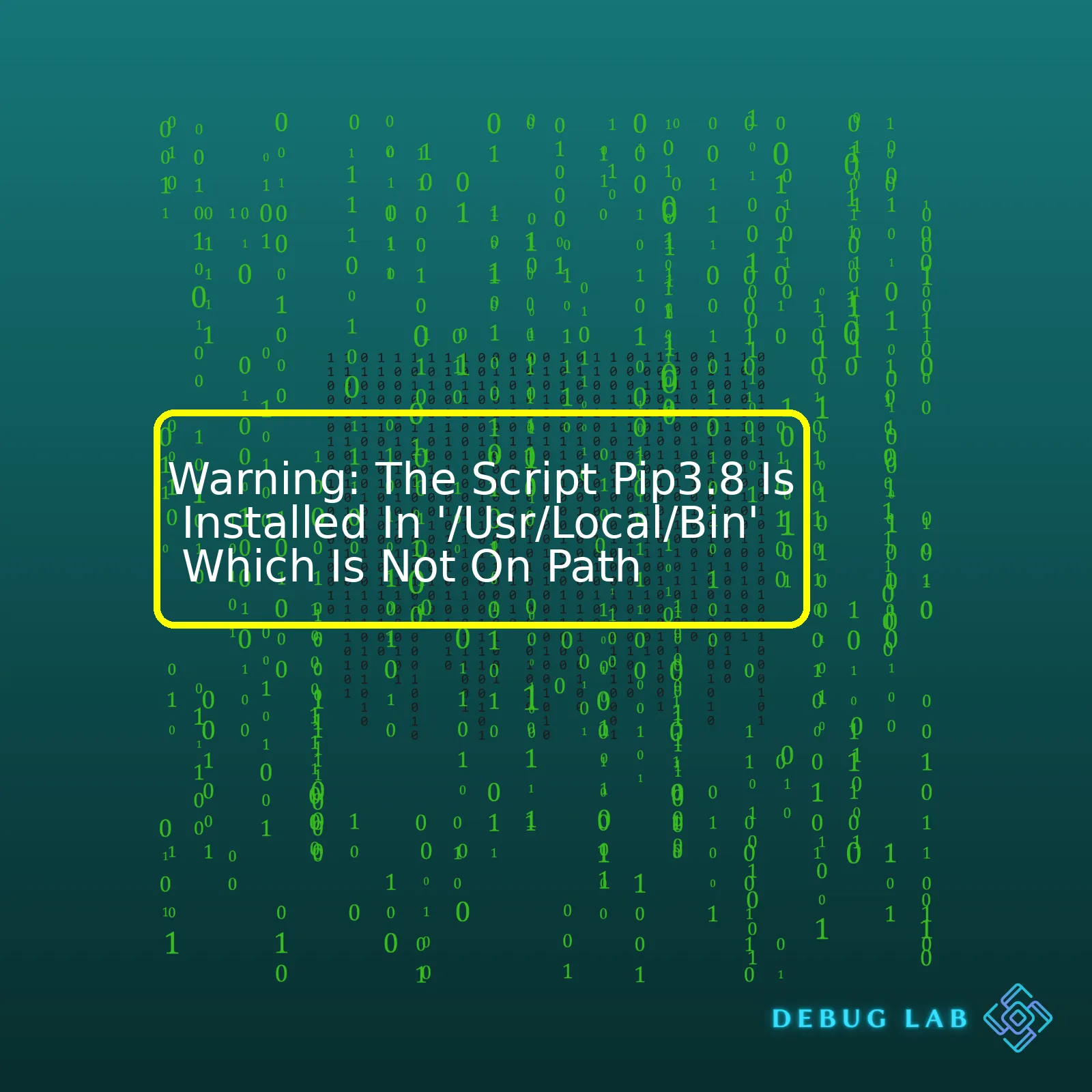
<table>
<tr>
<th>Warning Type</th>
<th>Trouble Area</th>
<th>Possible Solution</th>
</tr>
<tr>
<td>Environment Path Issue</td>
<td>pip3.8 installed in /usr/local/bin but not on PATH</td>
<td>Add '/usr/local/bin' to your system's PATH environment variable</td>
</tr>
</table>
This HTML generates a table which provides a possible solution to the stated problem area of the environment path issue we received as a warning.
The warning “The Script Pip3.8 Is Installed In ‘/Usr/Local/Bin’ Which Is Not On Path” typically occurs when one tries to use the pip3 Python package manager after installation. This message simply means that the operating system cannot find the executable ‘pip3.8’ when you want to perform tasks such as installing, updating or deleting python packages because the directory ‘/usr/local/bin’ is not included in the system’s PATH environment variable.
The PATH is an environment variable. It is a list of directories separated by a colon, which maintains a list of paths to locations where executables are stored. When you type a command, the system looks for it in the directories specified in PATH.
So, “/usr/local/bin” must be included in the PATH so that commands can be easily found. To solve this, you can append “/usr/local/bin” to the PATH environment variable. By modifying the .bashrc file (assuming you’re using a bash shell), you can add the following line at the end:
xxxxxxxxxx
export PATH=$PATH:/usr/local/bin
This modification will take effect after a system reboot or by sourcing the .bashrc file with the command “source ~/.bashrc”. From then on, the system will be able to find the ‘pip3.8’ script and the warning will no longer appear.
Sources:
– Stackoverflow: /usr/local/bin is not on the PATH
– How to Add Directory to PATH in LinuxWhen working with Python, it is crucial to understand the system’s PATH and how packages like pip3.8 are installed and accessed. If you encounter a warning message like “The script pip3.8 is installed in ‘/usr/local/bin’ which is not on PATH”, it means your system doesn’t know where to find the version of pip3.8 you have installed.
Here’s why this scenario happens:
– You’ve installed pip3.8 (or any other package), possibly as part of a Python3.8 installation.
– But, when you’re trying to use pip3.8 from your terminal by typing
xxxxxxxxxx
pip3.8
, the system cannot locate it because it isn’t in the system’s PATH.
What is PATH, you ask? PATH is an environment variable in Unix-like operating systems, DOS, OS/2, and Microsoft Windows, specifying a set of directories where executable programs are located. Essentially, when you enter a command in your terminal, the system checks these directories to find the corresponding executable file.
You usually install pip in one directory (for instance ‘/usr/local/bin’), but your operating system also searches in other directories specified in the PATH variable when you type commands into the terminal.
Briefly diving into the filesystem of Linux-based systems for understanding:
– ‘/usr’ is designed to hold shared, read-only data, including files that are used by several different applications. This makes it a fitting place for Python, which can be used by multiple users and across various applications.
– ‘/usr/local’ contains users’ programs that you install from source. It has its bin directory for executables, hence ‘/usr/local/bin’.
Essentially, ‘/usr/local/bin’ serves as a local repository for machine-local binaries.
When you install pip (Python Package Installer), pip3.8 in this case, via Python’s installation, it gets placed in this ‘/usr/local/bin’ directory.
But let’s return to the initial warning – you can’t access pip3.8 despite it being installed successfully. The solution is relatively straightforward; all you need to do is add ‘/usr/local/bin’ to PATH.
Here’s how you’d do it if you’re using bash shell:
xxxxxxxxxx
export PATH=$PATH:/usr/local/bin
This command appends ‘/usr/local/bin’ to your existing PATH. To make this change permanent across sessions, you should add this line to your bashrc file located at ‘~/.bashrc’.
And voila! You can now use pip3.8 straight from your terminal without encountering the earlier warning.Sure, let’s talk about how to resolve the warning: “The script pip3.8 is installed in ‘/usr/local/bin’ which is not on PATH”. To start, this typically implies that the directory where the pip3.8 executable is stored does not exist within your system’s PATH.
When an application or script executes through the command line, the operating system looks in all directories listed in the PATH environment variable to find it. If ‘/usr/local/bin’ isn’t in PATH, your OS can’t locate pip3.8, thus triggering the aforementioned warning. To solve this issue, we need to add ‘/usr/local/bin’ to the PATH:
On Unix-like systems (including Linux and MacOS), Python uses
xxxxxxxxxx
bash
shells. So, the code block will differ based on your shell. However, if you are running a Windows machine with Cygwin installed, the following guide will help you set up your environment properly.
If you’re using Bash Shell, execute the following command:
xxxxxxxxxx
echo 'export PATH=/usr/local/bin:$PATH' >> ~/.bash_profile
For macOS users, update your .bash\_profile or .zshrc file by performing the below command:
xxxxxxxxxx
echo 'export PATH=/usr/local/bin:$PATH' >> ~/.zshrc
Logically, these commands append the ‘/usr/local/bin’ directory into your PATH. The double ‘greater than’ symbols ensure output from the echo statement gets appended at the end of the mentioned files. The colon acts as a separator between multiple path values.
However, modifying shell configuration files alone is insufficient and will not solve our problem immediately. The changes won’t be applied until you restart your terminal or run below command.
xxxxxxxxxx
source ~/.bash_profile
or on macOS
xxxxxxxxxx
source ~/.zshrc
You can now verify whether pip3.8 is reachable through your command line by executing the following:
xxxxxxxxxx
pip3.8 --version
If ‘/usr/local/bin’ is added correctly, this command should return pip’s current version without issuing any warnings.
Please note there may be cases wherein multiple versions of Python are installed on your computer, each with its own version of pip. In such cases, if pip3.8 isn’t working as expected, confirm whether you’re using the correct version of pip. Use
xxxxxxxxxx
which pip
or
xxxxxxxxxx
which pip3.8
to display the exact path of pip being accessed.
All in all, the shell looks for executables in specific places. The key to solving this warning lies in adjusting your PATH variable so it points towards the appropriate executables.
What I’ve covered in this discussion reflects just a tiny fraction of coding intricacies; there’s an officially provided online documentation(Python Documentation) that covers Python in detail. Complete posts like this become the stepping stones helping coders decode the plethora of challenges they might come across.When it comes to troubleshooting PATH discrepancies, especially concerning something like the warning message: “The script pip3.8 is installed in ‘/usr/local/bin’ which is not on path”, it entails a multi-pronged approach that has technical rigour while being attuned to the exact nature of the problem at hand.
If you ever find yourself experiencing this issue, it indicates that the directory where ‘pip3.8’ is installed – in this case, ‘/usr/local/bin’, – is not included in the PATH environment variable. This is essentially meaning the system doesn’t know to look in ‘/usr/local/bin’ when executing commands.
For troubleshooting, steps include
– Checking Your PATH
– Adding Directory to PATH
– Making the Change Permanent
To begin with, always Check Your PATH. Execute the following command in the terminal:
xxxxxxxxxx
echo $PATH
This command will output the directories currently included in PATH, if ‘/usr/local/bin’ is not present among them, that’s the cause of the discrepancy. You’ll have to proceed with adding the directory to PATH.
Next step would be Adding Directory to PATH. To append ‘/usr/local/bin’ to your PATH use the export command:
xxxxxxxxxx
export PATH=/usr/local/bin:$PATH
The above command only impacts the current terminal session. If you open a new terminal window or reboot your computer, the changes to PATH will be lost. Therefore, to make the change persistent across all sessions, you need to apply another method.
To Make the Change Permanent, modify the ‘.bash_profile’ or ‘.bashrc’ file (depending on your system setup). Append the previously used export command to the end of the file. Here is how you can do this:
xxxxxxxxxx
echo 'export PATH=/usr/local/bin:$PATH' >> ~/.bashrc
After running the above code snippet, any new terminal session will have ‘/usr/local/bin’ included in PATH, and should function correctly. Make sure to replace .bashrc with .bash_profile or any other appropriate file used by your system’s shell if necessary.
In detail, the PATH is an environment variable on Unix-like operating systems, DOS, OS/2, and Microsoft Windows, specifying a set of directories where executable programs are located. More about PATH varibale may be found in online resources such as Wikipedia.
And if troubleshooting PATH discrepancies does not resolve your issue, you may consider reinstalling python3.8 and pip3.8. For reference, here’s how to install Python 3.8:
xxxxxxxxxx
brew install python@3.8
Subsequently install pip for Python 3.8:
xxxxxxxxxx
sudo easy_install pip
Always remember, before making any modifications to environment variables or system files, it’s good practice to create a backup. Careful modification of these settings can resolve issues, but incorrect configurations can sometimes lead to more problems.
Reference:
StackoverflowSure, let’s dive into Python’s Pip Version 3.8 and its relevance to the warning message ‘The Script Pip3.8 Is Installed In ‘/Usr/Local/Bin’ Which Is Not On Path’.
Python’s package manager, pip version 3.8, handles installing and managing software packages found in the Python Package Index (PyPI) and other indexes. It’s analogous to npm for Node.js or gem for Ruby. This means it links and regulates over a thousand reusable Python modules from web scraping to natural language processing, making a vast array of features easily accessible.
For instance, some specific features of Python’s pip include:
– Installing Python packages:. By using a simple command line interface, you can install Python packages listed in PyPI and other repositories. A package installation can be as simple as typing
xxxxxxxxxx
>pip3.8 install package_name
.
– Uninstalling Python packages: Using an identical CLI, packages can be efficiently uninstalled when they’re no longer needed. Again, this procedure is straightforward
xxxxxxxxxx
pip3.8 uninstall package_name
.
– Managing package versions: Pip can manage package versions to ensure compatibility with your Python environment. Dependence on a specific version of a Python package can be specified by
xxxxxxxxxx
pip3.8 install 'package_name==2.0.0'
– Listing installed packages: You can quickly identify what packages are already installed in your environment using pip’s listing feature.
xxxxxxxxxx
pip3.8 list
Now, the warning ‘The Script Pip3.8 Is Installed In ‘/Usr/Local/Bin’ Which Is Not On Path’ typically occurs when the location where pip3.8 has been installed isn’t included in your PATH environment variable. So what does this mean?
Your operating system uses directories in the PATH environment variable to search for executable files. If the directory containing the pip3.8 script isn’t part of PATH, the system wouldn’t be able to locate the script when you type pip3.8 at the console. The ‘/usr/local/bin’ directory is commonly used for scripts for locally installed packages, so pip3.8 gets installed there often.
To fix this issue, you can add the directory containing pip3.8 to your PATH variable. In UNIX-like systems like Linux, OS X, or Cygwin, you can achieve this by adding export PATH=$PATH:/usr/local/bin to your shell startup file (~/.bashrc or ~/.bash_profile for Bash). Here’s an example:
xxxxxxxxxx
export PATH=$PATH:/usr/local/bin
Finally, source your shell startup file or restart your console for these changes to take effect:
xxxxxxxxxx
source ~/.bashrc
or
source ~/.bash_profile
In sum, Python’s Pip provides a wealth of package management functionality that enables developers to integrate useful libraries into their projects effortlessly. However, to use pip effectively, you need to ensure your PATH variable reflects the actual path to the installed binary. Doing so eliminates the described warning and ensures smooth execution of pip commands on your console. For more information about managing PATH on different operating systems, consider visiting Super User PATH guidance.
Let us break it down and analyze why we are getting this warning when trying to use pip3.8 in a Linux system.
First, Pip is a package manager for Python. It allows you to install additional libraries and dependencies not packed with your Python installation. In your case, version 3.8 of pip corresponds to the version of Python you’re using (Python 3.8).
The problem you’re encountering revolves around something known as the PATH environment variable. Environment variables store values that configuration files can utilize while running scripts or services on your Linux machine. The PATH variable specifically holds a list of directories where executables reside.
Kindly note that the PATH is defined in ‘/etc/environment’ file in most Linux systems. When you attempt to run a program without specifying the whole path to its executable, the PATH is parsed for potential locations of that program. It results in a convenient shortcut – enabling us to type ‘python’, ‘pip’, ‘ls’, etc., instead of the full paths.
Now let’s focus on the key issue – the directory ‘/usr/local/bin’ isn’t part of your PATH. Hence, the pip3.8 script within cannot be found, which generates the warning.
Solution – Adding ‘/Usr/Local/Bin’ to PATH:
The primary solution to this particular warning is to add the ‘/usr/local/bin’ directory to your PATH.
Here is how you do this:
Open ‘.bashrc’ or ‘.zshrc’ file in your home directory with any text editor:
xxxxxxxxxx
nano ~/.bashrc
Include the export line at the end:
xxxxxxxxxx
export PATH=$PATH:/usr/local/bin
Save and exit.
Load the update you made into the current shell session by sourcing the file:
xxxxxxxxxx
source ~/.bashrc
This will instantly include ‘/usr/local/bin’ in your PATH, cutting the discrepancy and the warnings with it. The programs installed in this location (like your pip3.8) will now be readily accessible only by calling their names.
Table illustrating each step:
Step | Command |
---|---|
Edit .bashrc |
xxxxxxxxxx 1 1 1 nano ~/.bashrc |
Add new PATH |
xxxxxxxxxx 1 1 1 export PATH=$PATH:/usr/local/bin |
Reload .bashrc |
xxxxxxxxxx 1 1 1 source ~/.bashrc |
It’s important to note this method of adjusting your PATH is temporary (only until the shell session or terminal window is closed). To make it permanent, consider editing your /etc/environment file or place the export command in the profile-based files like ~/.bash_profile or ~/.profile. Please consult your specific Linux distribution guidelines before altering these files.
Finally, to check if ‘/usr/local/bin’ is successfully included in your PATH:
Run:
xxxxxxxxxx
echo $PATH
You should get an output with ‘/usr/local/bin’ in the list.
To confirm you’ve completely got rid of the warning, try running pip3.8 again. Everything should work perfectly fine after doing the above-mentioned steps.
For more comprehensive guides about Linux PATH and its usage in different distributions, refer to IBM Documentation and CyberCiti’s guide.Sure, the warning you encountered relative to
xxxxxxxxxx
pip3.8
being installed in
xxxxxxxxxx
'/usr/local/bin'
, which is not on your PATH, essentially means that your system does not know where to look for the
xxxxxxxxxx
pip3.8
command. It’s not aware that this location is a place it should be searching for executable scripts. Let’s delve into what this means and how you could solve this issue.
xxxxxxxxxx
pip3.8
is the Python 3.8 package installer. It’s a handy tool that lets you install Python packages from PyPI (Python Package Index) and other package indexes. `/usr/local/bin` is an example of an absolute PATH in a Unix-like operating system. Most systems will recognize this directory as the standard location for user-installed binaries (executable files).
PATH is an environmental variable in Unix and Unix-like operating systems that specifies a set of directories where executable programs and scripts are located. Your system always goes through this list of directories whenever you request to run a program or script.
The essence of the warning is that your system can’t find
xxxxxxxxxx
pip3.8
because
xxxxxxxxxx
/usr/local/bin
is not listed in your PATH variable. Hence, to clear this warning you’ll have to manually include this directory path to your system variables.
To resolve this, please refer to the following steps:
– To check the current PATH:
echo $PATH