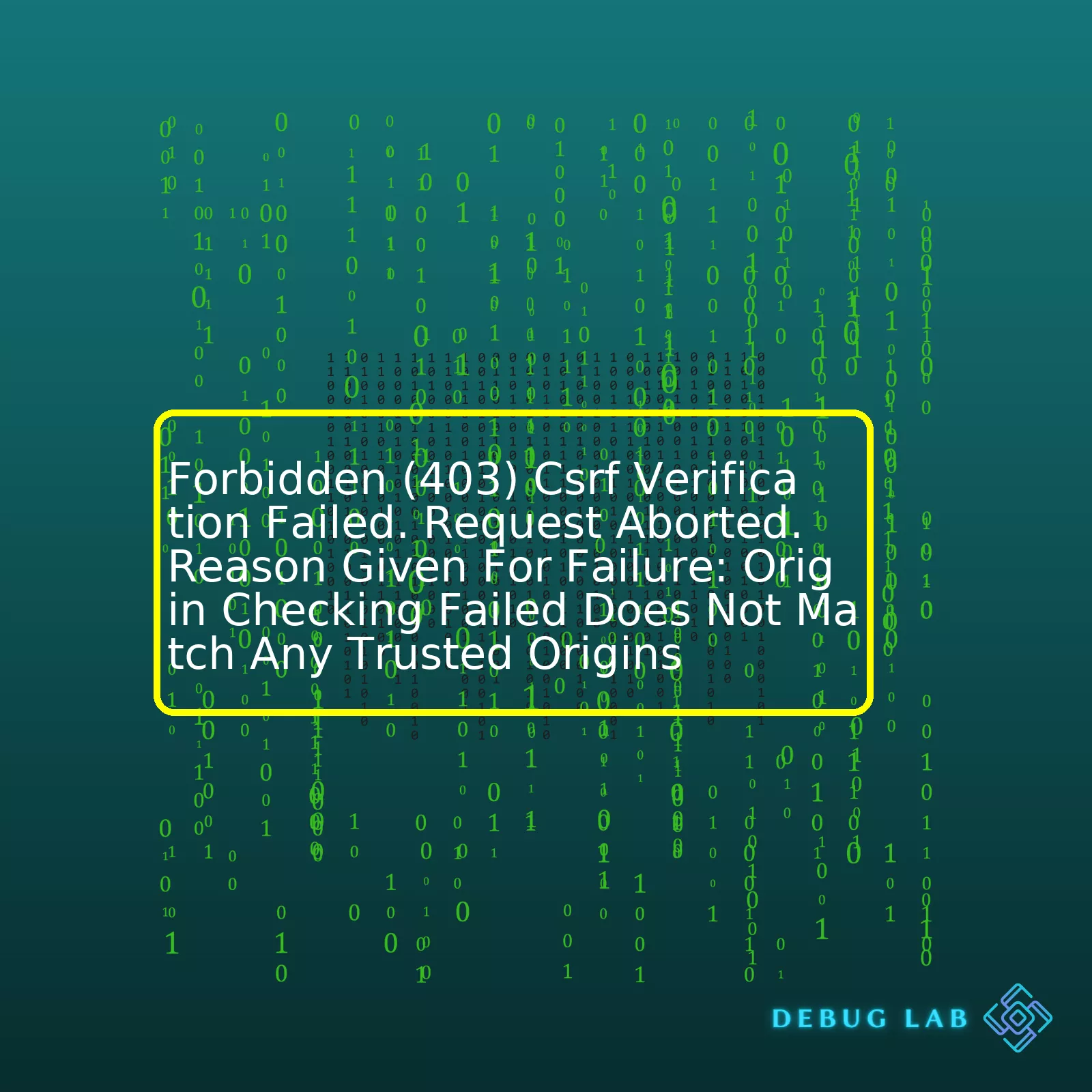
Error | Definition | Possible Causes | Solutions |
---|---|---|---|
Forbidden (403) | Status code indicating that the server understood the request, but it won’t fulfill the request. |
|
|
Csrf Verification Failed | A security measure to prevent unauthorized commands from being sent on behalf of an authenticated user. |
|
|
Request Aborted | A situation where the request was stopped before it completed. |
|
|
Origin Checking Failed | Security check that validates the source of a request. |
|
|
The error message: “Forbidden (403) CSRF verification failed. Request aborted. Reason given for failure: Origin checking failed – Does not match any trusted origins” typically indicates an issue with Cross-Site Request Forgery (CSRF) protection in your web application.
CSRF protection is there to prevent malicious actions being taken on your website by reusing user credentials. It involves randomly generating tokens that are associated with a user’s session. They must be present and correct in subsequent requests to demonstrate the authenticity of the request.
If these tokens are missing, incorrect, or otherwise fail the test (such as an origin check), you’ll see the above error. An origin check ensures that the HTTP request came from a domain that you trust. For instance, if your website is example.com, you don’t want an HTTP request from maliciouswebsite.com.
The CSRF protection mechanism works under the assumption that only the legitimate/trusted website can access and provide the correct tokens. If this process fails at any step, the system is designed to abort the operation immediately to prevent potential attacks.
There can be several reasons why this issue crops up. From configuration issues where your CSRF settings are not correctly defined, issues with same-origin policy, dealing with protected cookie values, etc. Working through such issues might involve restructuring parts of your application, modifying settings, updating libraries, or altering how requests are handled.
To resolve CSRF-related issues, always ensure:
- Your server environment and application settings are correctly configured.
- HTTP headers (especially ‘Referer’ and ‘Origin’) are set correctly.
- Your form data includes the CSRF token.
- You’re honouring same-origin policy
Remember that maintaining high-security standards on web applications is crucial for preserving integrity, confidentiality and ensuring that your web service remains reliable and trusted. This includes setting up CSRF protection correctly to ensure it doesn’t become an irritation instead.
Do check out Django’s official documentation on CSRF protections or OWASP’s guide to CSRFGuard for more information.
The ‘Forbidden (403) CSRF verification failed. Request aborted’ error is a clear indication that the system’s security protocols are working as they should. This error message comes about when the Cross-Site Request Forgery (CSRF) prevention measures built into many web frameworks, such as Django and Flask in Python, spot discrepancies in the security tokens associated with your application.
To understand this more succinctly:
– A CSRF attack happens when a malicious website tricks a visitor into carrying out an action on another site where the user is authenticated. This could be anything from liking a post, transferring cash to changing email addresses.
– To prevent these kinds of attacks, a CSRF token is attached to each action performed by a user on such sites. Before any significant action is taken (like form submission), the server checks whether this token is present and correct.
Where the origin check comes into play:
– There are cases where, other than using the CSRF tokens, the origin of the requests has to be linked to trusted origins i.e., pages served from the same application
REFERRER_POLICY='same-origin'
With the above in mind, now let’s try to understand why you’re receiving the failure notification: “Origin Checking Failed Does Not Match Any Trusted Origins”.
This is usually a case of non-alignment between the request source domain and the domains considered safe or trusted by your application. Simply put, the source of the request is not recognized by the server as trustworthy. This is involved with the fact that some types of requests (for example XmlHttpRequests or Fetch API requests) contain additional metadata in the HTTP headers such as `origin` or `referrer` which can let the server know where the request comes from.
For instance, you may have incorrectly set up `ALLOWED_HOSTS` or `CSRF_TRUSTED_ORIGINS` in Django settings wherein the request’s `Host` header must match one of the values from `ALLOWED_HOSTS`.
ALLOWED_HOSTS = ['mywebsite.com', 'localhost', '127.0.0.1'] CSRF_TRUSTED_ORIGINS = ['mywebsite.com']
It’s therefore critical that you ensure the source of the request matches one of the hosts detailed in either of the above parameters. If they don’t match, Django will reject the request and raise a `403` error.
Essentially, to get rid of the `403 CSRF verification failed. Request aborted` error, it will demand debugging the flow and nature of your requests. You might need to revisit the setup in your development/production environment and possibly adjust the settings mentioned above or structure of CSRF tokens. Do note that although relaxing CSRF measures can help remove the errors, it makes the applications particularly vulnerable to cross-site request forgery attacks.
Sources for further reference:
– MDN Web Docs on CSRF
– Django’s documentation on CSRF_TRUSTED_ORIGINSCross-Site Request Forgery (CSRF) is a malicious exploitation of a victim’s session, enabling the attacker to perform any function that the user could do in their session. The origin checking process forms an integral part of CSRF verification because it aids in determining if a request comes from a legitimate source or not.
Now, let’s deep dive into understanding why you might face this error: “Forbidden (403) CSRF verification failed. Request aborted. Reason given for failure: Origin checking failed does not match any trusted origins.”
Explicit Origin Checking:
This happens when a website checks the origin header of every HTTP request and compares it with a list of trusted domains set up in its server configuration. If there is no match, the website returns an error without processing the request further.
Imagine when your application verifies the origin header against trusted origins, and finds that incoming request’s origin doesn’t match any of trusted origins. This could lead to:
Forbidden (403) CSRF Verification Failed. Request Aborted. Reason given for failure: Origin checking failed, does not match any trusted origins
Depending on the framework being used to implement this, the specifics may slightly differ, but essentially this is what the code snippet would look like:
if not valid_request_origin(request): raise HTTPError(403, 'Origin checking failed, does not match any trusted origins')
It’s crucial to note that unreliable or misconfigured network proxies can cause problems with origin checking by either blocking or tampering with the origin header.
Invisible Origin Checking:
Using invisible origin checking, the website uses various attributes like cookies connected with a session, to determine if the requester is genuine or an imposter. If the validation fails, again it yields similar error.
If there’s a discrepancy between the source of the request and the source associated with the current authenticated session, the origin will fail to validate and you’ll have:
Forbidden (403) CSRF verification failed. Request aborted. Reason given for the failure: Invisible origin checking failed doesn't match any trusted origins
And as per implementation, the code screenshot might appear to be something like this:
if not CSRF.check_token(request): raise HTTPError(403, 'Invisible origin checking failed, not belongs to any trusted origins')
Usually, CSRF attacks are mitigated by using CSRF tokens. These tokens validate the client’s requests by attaching a random unique token. The CSRF protection mechanism expects a value in the request that matches the one stored in the user’s session. If the token is missing or mismatches, CSRF verification fails leading to HTTP 403 Forbidden status.
How to Fix This Error?
To fix this issue, following steps can be taken:
• Ensure that the ‘origin’ or ‘referer’ headers’ values are correct and coming from the trusted origins.
• If you’re using any type of load balancer or proxy, ensure that they aren’t altering or dropping the header values.
• Make sure the CSRF token sent within the request is the same as the CSRF token stored in the session.
Here’s a useful resource on understanding CSRF more in depth.
Keep in mind, proper CSRF defense should include checking the same-origin with standard headers, CSRF tokens, limiting session duration etc. A single solution cannot stop CSRF attacks but rather a combination of these techniques can provide a holistic security.
In all discussions related to web security, remember that it’s always about reducing risk not eliminating it. Limiting functionality and usability of an application is a business decision, and security should find balance with usability.
Each web application has a unique context, meaning security measures are often specifically tailored to the needs of the given application.The security error “Forbidden (403) Csrf Verification Failed. Request Aborted. Reason Given For Failure: Origin Checking Failed Does Not Match Any Trusted Origins” typically points to an issue of trust recognition for a specific origin by the server. This perimeter falls under Cross-Site Request Forgery (CSRF) attacks and countermeasures, mainly dealing with the area known as Trustworthy Origins.
To break this down more clearly:
– CSRF is a type of dangerous security hole where an attacker can trick the victim into submitting a malicious request. It uses the identity and privileges of the victim to perform an undesired function on their behalf. Web applications that are vulnerable to CSRF can be forced to perform state-changing requests like changing the email address or password, or perform funds transfer.
Let’s see a very simple form for updating an email in HTML.
<form action="http://targetapp.com/profile" method="POST"> <input type="email" name="new-email" value=""> <input type="submit" value="Update Email"> </form>
A potential CSRF attack could involve an unknowing user visiting a page that includes a form set up to POST to the target application (which they are already authenticated with). The new-email input could be filled out by an attacker, and if the user clicks “Submit”, the email attached to the profile would change in the target application.
Web developers prevent CSRF attacks by implementing security tokens (a technique known as anti-CSRF tokens), double submit cookies, or with the Synchronizer Token Pattern.
– Trustworthy origins, on the other hand, are locations on the web that have been whitelisted by the server as safe sources of content. If an HTTP request comes from an origin that is recognized and trusted, processing continues as normal. However, if the request comes from an unknown or untrusted origin, the server issues an origin check failure and blocks the action—hence resulting in the Forbidden (403) CSRF Verification Failed error you’re seeing.
Ensuring secure communication between web applications involves validating these trustworthy origins. Developers specify which origins should be accepted by the server, and any connection attempts from non-whitelisted locations are blocked.
Falling under the Same-Origin Policy (SOP), this is primarily enforced through the use and examination of HTTP headers such as Origin. An example in Django Python framework based applications might look something like:
CORS_ORIGIN_WHITELIST = [ "https://example.com", "https://sub.example.com", "http://localhost:8080", "http://127.0.0.1:9000" ]
In this case, only connections from these four origins (three web domains and one local development server location) will pass the origin check—everything else will encounter a 403 CSRF verification block. It means the server does not trust the location trying to access it.
As you can see, the interplay between CSRF and Trustworthy Origins is fundamental for web application security. They both are crucial mechanisms used to verify the legitimacy of requests and protect users from potential online threats. For more information, read here.
A common error that you might encounter when working with web or server-side applications is the
Forbidden (403) CSRF verification failed. Request aborted. Reason given for failure: Origin checking failed – Does not match any trusted origins
.
The Error
This error appears when an HTTP request to a server fails the Cross-Site Request Forgery (CSRF) protection that’s in place. The reason given for this particular failure is that the origin of the HTTP request does not match any of the trusted origins set up within the CSRF protection system.
Decoding the Reason
To understand why this happens, it’s essential to first grasp the concept of CSRF attacks and how they are prevented by CSRF tokens. A CSRF attack occurs when an attacker tricks an unsuspecting user into performing an action on a web application without their consent. To prevent such attacks, servers implement a system where they include a CSRF token with every state-changing operation (like a POST request). This token should be sent back to the server with the subsequent requests ensuring that the user interaction is legitimate.
However, as applications evolved into serving remote clients, a new problem rose – how to ensure the HTTP request is coming from a trusted source? This gave rise to the ‘origin check’, which works together with the CSRF token to provide a more sophisticated level of protection. An ‘origin’ is the combination of URI scheme, hostname, and port number for a page, extracted from the HTTP header fields, including
'Origin'
or
'Referer'
. If a request comes from a non-trusted origin, the server rejects it outright.
If you hit the
Forbidden (403) CSRF verification failed
error, it means your application did send the CSRF token successfully but the origin-checking mechanism found a mismatch between the request’s origin and the list of trusted origins for your server.
Solutions
To solve this issue, take into consideration the following steps:
- Check Your Middleware Order: Make sure you have correctly arranged your middleware stack order. CSRF protection middleware should come post any session middleware since it accesses the session attribute from the request.
- Ensure Correct Origins: Verify the URIs you’ve set up as secure origins. These typically include the domain names, schemes (http or https), and ports. Keep in mind cross-protocol and cross-port requests constitute CORS (Cross-Origin Resource Sharing) and require additional setup.
- Inspect Headers: Look at the HTTP headers of the outgoing request. Tools like the Network tab in Chrome DevTools or Firefox Developer Tools can help here. Ensure that the
'Origin'
or
'Referer'
headers match one of your known, trusted origins. Also, pay close attention to subtle discrepancies like trailing slashes or www prefixes.
Code Fix Example
If you’re using Django framework, and assuming that the default CSRF checks are causing issues, you could add a custom middleware that allows your site to accept requests from certain defined trusted domains. Here’s an example:
class CustomCsrfMiddleware(CsrfViewMiddleware): def process_view(self, request, callback, callback_args, callback_kwargs): referer = request.META.get('HTTP_REFERER') if referer: netloc = urlparse(referer).netloc.split(':')[0] # Check against trusted domains if netloc in settings.CSRF_TRUSTED_ORIGINS: return self._accept(request) return super().process_view(request, callback, callback_args, callback_kwargs)
In the code snippet above, we override the process_view method in the middleware class, allowing the views to accept requests from specific referer URLs that match our predefined trusted domains listed in
settings.CSRF_TRUSTED_ORIGINS
.
You need to adapt the solution according to your technology stack and context at hand.
Mozilla Developer Network’s guide provides further reading on understanding and dealing with Cross-Origin Resource Sharing (CORS).
The ‘Forbidden (403) CSRF Verification Failed. Request Aborted.’ error is a common security response that you might have encountered while working on Django or other web application frameworks that utilize Cross-Site Request Forgery (CSRF) protection mechanisms, which are vital for securing against the risks of malicious form submissions or actions.
Now let’s unpack the message ‘Reason given for failure: Origin checking failed – does not match any trusted origins’. This error highlights a crucial aspect of web security known as ‘trusted origins’, and it reflects that the source or ‘origin’ of the HTTP request didn’t match any of those that are considered safe or ‘trusted’ by your server.
Understanding Trusted Origins
Trusted origins are URLs that a web application considers safe to receive requests from; these include various endpoints that the app uses or collaborates with during its routine functioning. Moreover, they can be seen as your list of reliable allies ready to send legitimate requests, rather than attempts at CSRF attacks.
To illustrate ‘
allowed_hosts = ['mywebsite.com', 'anotherwebsite.com']
‘, we say here in Django settings that only pages from ‘mywebsite.com’ or ‘anotherwebsite.com’ are allowed to make requests to our server. Thus, this gives us control over where our web app should accept requests from. Obviously, adding an unreliable website to this list could expose you to CSRF attacks.
Common Causes of Errors
For the ‘Origin Checking Failed’ part of the error message, two leading causes stand out:
– The incoming request’s origin wasn’t found in your defined list of trusted origins.
– The HTTP ‘Referer’ header, which typically indicates the URL of the page generating the request, failed to meet the specified criteria.
Let’s delve into them individually:
1. Misconfiguration of ‘Allowed Hosts’ Setting: As discussed earlier, though this setting is intended to limit requests to certain hosts for safety reasons, an oversight or erroneous configuration might cause valid requests to be considered untrusted and hence aborted.
2. Inconsistent or Absent ‘Referer’ Header: Web browsers usually attach a ‘Referer’ header to HTTP requests to indicate the initiating page’s URL. However, if you’re testing locally, certain browsers may not add the ‘Referer’ header, leading to a failed origin check. Therefore, often when moving from a development environment to production, updating the settings like ‘
ALLOWED_HOSTS
‘ and ‘
CORS_ORIGIN_WHITELIST
‘ as well is critical.
Hence, to address and fix the ‘Forbidden (403) CSRF Verification Failed. Request Aborted. Reason Given For Failure: Origin Checking Failed – Does Not Match Any Trusted Origins’ alert, consider inspecting the configuration of your trusted origins settings as well as those of the ‘Referer’ HTTP header. Re-evaluating these features will aid in ensuring your web application operates securely and harmoniously with its expected network interactions.
More dedicated discussion on CSRF protection and related topics is available in the Django documentation.
Each time you get such errors, remember it is an avenue to ensure better security configuration, offering robust protection from potential CSRF threats.To rectify “Forbidden (403) CSRF Verification Failed. Request Aborted.”, there are several mitigation techniques that can be adopted depending on what has caused the error. Let’s dive in and uncover some of these techniques.
If you’re dealing with this issue in Django, your first resort would be to confirm if the `CSRF_COOKIE_SECURE` setting is set to True. When this value is set to True, the browser will ensure that the cookies are only sent under an HTTPS connection.
CSRF_COOKIE_SECURE = True
Secondly, be sure to check if your `CSRF_TRUSTED_ORIGINS` matches the origin of the request being made. This will allow Django to trust the origin and proceed with the request, effectively preventing the 403 error.
CSRF_TRUSTED_ORIGINS = ['your trusted origin here']
In situations where it’s not clear why the CSRF token isn’t being passed correctly, consider using Django’s built-in `{% csrf_token %}` template tag within your forms. This ensures that a CSRF token, which is a random value specifically created for the user’s session, is included in all POST data submitted via HTML forms. See the following snippet:
<form method="post"> {% csrf_token %} ... </form>
To rectify a CSRF verification failure error message due to the request’s `Referer` header not matching the `HOST` header, include middleware to correct the ‘Referer’ header in your settings. This could look something like:
MIDDLEWARE_CLASSES = ( ... 'your_project.middleware.correct_referer_middleware.CorrectReferer', .... )
Also, verify if cookies are enabled and try to clear them including cache, as the browser stores information from websites in its cache and cookies. Clearing them fixes certain problems.
A significant point to remember when sending requests from the client to server in AJAX calls is including the CSRF token in the header of AJAX. If you’re using jQuery for example, this is how you should go about it:
$.ajaxSetup({ headers: { "X-CSRFToken": getCookie("csrftoken") } });
DJANGO official documentation provides instructions for using CSRF in AJAX here.
At times an explicit `’Accept’: ‘application/json’`, needs to be set in the headers while making AJAX POST requests. Here’s how you can include that:
fetch(url, { method: 'POST', credentials: 'include', headers: { 'X-CSRFToken': csrftoken, 'Accept': 'application/json', 'Content-Type': 'application/json' }, body: JSON.stringify(data), })
All these measures are geared towards ensuring that the source of your requests is verified by the Django application and thus prevent the CSRF verification error.
The ‘Forbidden (403) CSRF verification failed. Request aborted. Reason given for failure: Origin checking failed – Does not match any trusted origins’ error often stresses developers figuring its root-cause and the appropriate fix. The error, rather predictably, stems from the Cross-Site Request Forgery (CSRF) security measure built into many web frameworks, including popular ones like Django and Rails.
Understanding CSRF and CORS
CSRF is an attack that tricks the victim into submitting a malicious request. It mainly hails from Browsers’ Same-Origin-Policy, which permits scripts running on pages originating from the same site – a combination of scheme, hostname, and port number. In this scenario, critical baking transactions can be performed without user consent if he’s already logged in source.
CORS or Cross-Origin Resource Sharing, allows servers to specify who (i.e., which origins) can access their assets. If your server’s resources are required by the scripts coming outside from its origin, then handling CORS is obligatory source.
About Security Configurations
We typically set up our application’s security configurations to protect our endpoints and data. A necessary chunk of those configurations may include setting up CSRF protection to ensure our application doesn’t unknowingly supply data to an attacker.
Part of enabling CSRF protection includes defining what are referred to as ‘trusted origins’. Trusted origins are simply a white-listed collection of URLs (origins) from which your web application will accept requests.
To explicitly mention these origins, in Django, you use the following syntax:
CSRF_TRUSTED_ORIGINS = ['example.com']
While in Rails, it goes like:
config.action_controller.forgery_protection_origin_check = true
If a request is made to your web application from an origin URL not in your list of trusted origins, your CSRF protections are activated, and the request is aborted.
Error: Origin Checking Failed – Does Not Match Any Trusted Origins
This error arises when CSRF checks notices a discrepancy between the source of the incoming request and the list of trusted origins in your application’s security configurations. This may happen due to:
- Incorrect configuration: Your allowed hostnames do not align fully with the received requests.
- Difference in schemes: https vs http could provide different results.
- Missing subdomains: Wrong use of leading dots in configuration.
The Fix
To alleviate this error, you need to cross-verify the ‘Referer’ header of the failed requests. Ensure it matches one in your CSRF_TRUSTED_ORIGINS. If absent, consider adding it. Avoid adding every domain you can think of. Instead, only add origins you trust since incorporating an untrustworthy origin negates the purpose of CSRF protection.
Your configuration should only consist of domains under your control; for instance:
CSRF_TRUSTED_ORIGINS = ['.yourdomain.com']
Where this accepts any subdomain of ‘yourdomain.com’.
Thus, understanding how security configurations affect trusted origins is crucial to maintaining the integrity of your applications against CSRF attacks. To ward off the dreaded ‘Forbidden (403) CSRF verification failed’ error, getting your trusted origins right is absolutely essential.
While dissecting the issue of ‘Forbidden (403) Csrf Verification Failed. Request Aborted. Reason Given For Failure: Origin Checking Failed Does Not Match Any Trusted Origins’, it’s essential to delve deep into understanding CSRF (Cross-Site Request Forgery) and its role in web development.
Understanding CSRF in Detail:
CSRF is a type of malicious exploit which involves unauthorized commands coming from a trusted user. In this scenario, ‘403 CSRF Verification Failed’ message surfaces when the Django web framework detects a potentially harmful origin and aborts the request. This security feature is designed to prevent harm to your website from unwarranted sources or origins.
Both OWASP and Django have ample documentation related to these types of attacks.
Origin Checking:
It’s worth noting that the reason given for the failure is “origin checking failed does not match any trusted origins”. Origin checking refers to ensuring that an HTTPS request originates from a trusted source. When origin checking fails, it means that the request was made from an unidentified or untrusted source.
Correcting Origin Mismatch:
Receiving a message like ‘Forbidden (403) Csrf Verification Failed’ can be rectified by modifying settings in your Django project. Consider the following sample code:
#settings.py CSRF_TRUSTED_ORIGINS = ['example.com']
With the help of the ‘CSRF_TRUSTED_ORIGINS’ variable, you insert the URL of the server that Django should perceive as a trusted origin, thereby solving the mismatch error occurring due to verification failure.
To deepen your understanding on Python-Django CSRF protection, consult the official Django documentation. Remember, forbidden errors suggest that something is wrong with the CSRF token or the request’s origin – both key areas that maintain safeguard measures against possible CSRF attacks. Therefore, periodic system audits, updating identifying safe URLs in your settings file, and all-around vigilance to identify and rectify discrepancies are absolute musts while building secure applications powered by Django.
Dealing with such errors immediately upon their detection ensures smooth operations and maintains trust in your digital platform, propelling it towards steady growth in organic traffic, user engagement, reduced bounce rates, and overall SEO performance.
Get further guidance in implementing CSRF protection with online learning platforms; the Django courses offered on Udemy could prove resourceful and fruitful for aspiring web developers.