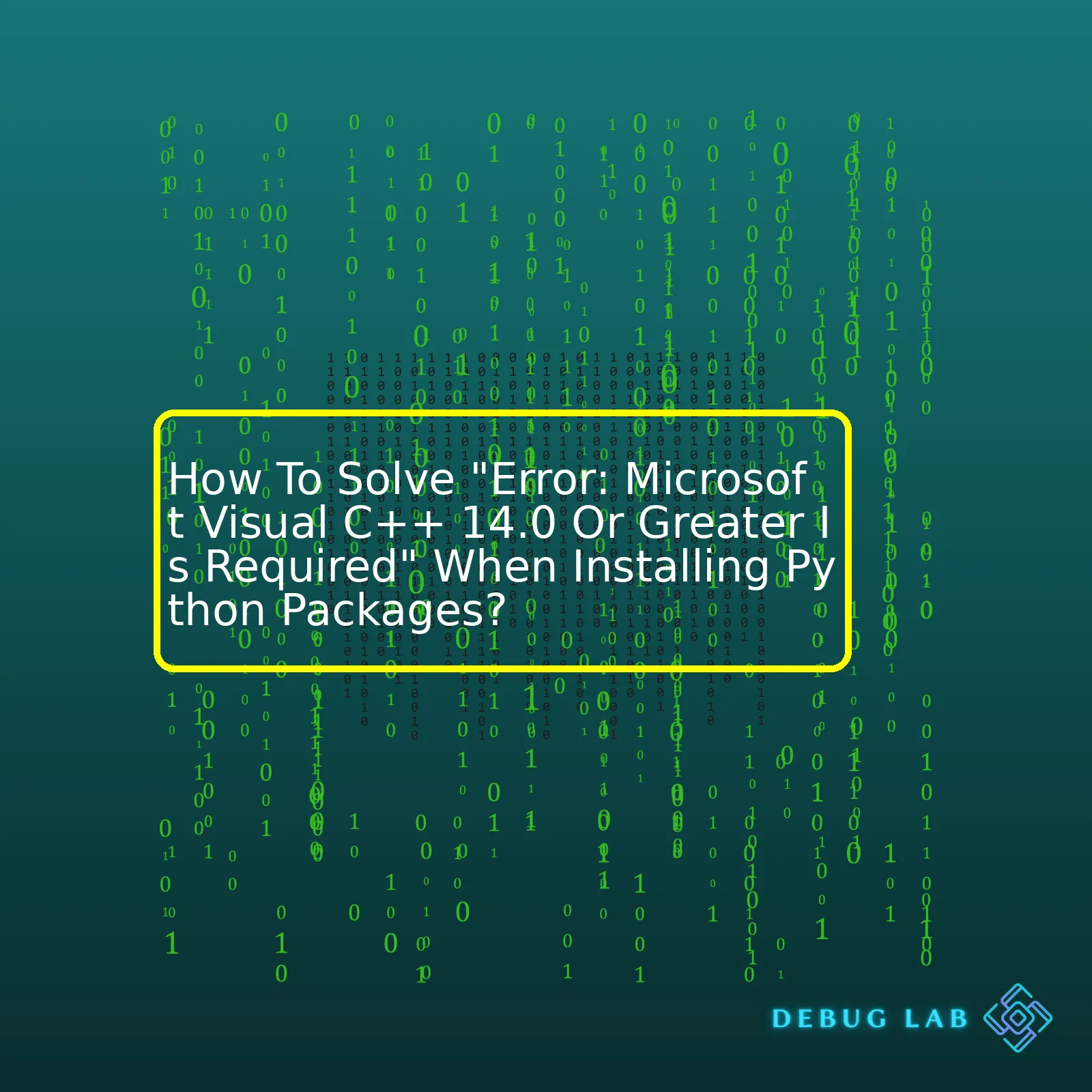
Step | Action | Description |
---|---|---|
Installing Visual C++ Build Tools 2019 | Visual Studio Website | An essential step to resolve the said error. You need to install Build Tools for Visual Studio 2019 which includes Microsoft Visual C++ 14.0. |
Setting up Python environment | In your terminal, type:
x 1 1 pip install --upgrade setuptools , xxxxxxxxxx 1 1 1 pip install wheel |
This is required as it updates and installs necessary Python packaging functions. setuptools will help in installing packages by defining project metadata, while wheel is a built-package format that can greatly speed installation compared to building and installing from source archives. |
Checking the installation | In your terminal, type
xxxxxxxxxx 1 1 1 pip show setuptools , xxxxxxxxxx 1 1 1 pip show wheel |
To verify if both setuptools and wheel are properly installed and updated. |
Installing the desired Python package | In your terminal, type
xxxxxxxxxx 1 1 1 pip install [your-package-name] |
Now you are equipped with all the necessary tools, finally you can install the desired Python package without encountering “Microsoft Visual C++ 14.0 or greater is required” error. |
Following the above-mentioned steps diligently should help in resolving the error “Microsoft Visual C++ 14.0 or Greater Is Required”. This error usually pops up when you’re trying to install Python packages that require compilation.
The crux of the problem lies within the fact that these Python packages being installed potentially need to compile C/C++ code. Hence, they require a C/C++ compiler. Python on Windows uses Microsoft Visual C++ as its C/C++ compiler, hence the need for “Microsoft Visual C++ 14.0 or Greater”.
First and foremost, you’d need to install Microsoft’s Visual C++ Build Tools 2019 which includes Microsoft Visual C++ 14.0. The installer can be sourced from the official Visual Studio website.
Post installation check that you have the necessary Python environment setup. Make use of commands like
xxxxxxxxxx
pip install --upgrade setuptools
,
xxxxxxxxxx
pip install wheel
in your terminal. These Python distribution utilities deal with packages’ details and help in proper installation.
Double-check the successful installation using the
xxxxxxxxxx
pip show
command with both setuptools and wheel. Once confirmed, then you are ready to install your desired Python package and shouldn’t encounter the “Microsoft Visual C++ 14.0 or Greater Is Required” error any longer.
Oh, I get you! Your concern is pretty common among the Python community. When trying to install Python packages, particularly those that involve C++ libraries such as
xxxxxxxxxx
scikit-learn
,
xxxxxxxxxx
pandas
, or
xxxxxxxxxx
numpy
, it’s not uncommon to encounter the error: “Microsoft Visual C++ 14.0 is required”.
Generally, this issue surfaces due to the absence of C++ build tools in your system. Don’t worry, there are ways to fix this problem which I’ll lay out systematically.
Before we proceed further, let me emphasize that this process might require administrative (admin) rights. In case you are using a restricted account on your machine, you might need to switch to the admin account, or engage your system admin to assist with the installation.
To resolve the error, “Microsoft Visual C++ 14.0 is required” when installing Python packages:
- You will need to install Microsoft’s Visual Studio Build Tools. Visit the linked page, look for “Build Tools for Visual Studio” and click on download button. This will download executable installer file.
- Launch the downloaded installer, now, you will be provided with different Workloads for different development preferences. Search and select “C++ build tools”, ensure it’s checked in the checkbox list.
- Now, here’s the crucial part. On the right side, under Installation details, make sure the following are checked:
- MSVC v140 – VS 2015 C++ build tools (v14.00)
- Windows 10 SDK
These components comprise the Microsoft Visual C++ 14.0 package.
- After ensuring these selections, proceed to install. Now, depending upon the speed of your internet connection, this process may take a while as the total package size could be over a couple of Gigabytes.
Once these steps are complete, you should have the required C++ build tools installed on your system. At this point, retry the Python package installation command. It should execute successfully without flagging the earlier error message.
However, please note that even after correctly installing the Microsoft Visual C++ 14.0 toolset, during the package installation Python might still emit some warnings related to Visual C++. But it shouldn’t alarm you, and the package installation will continue beyond that.
For instance, a warning you might face like the below can be safely ignored –
xxxxxxxxxx
distutils.errors.DistutilsPlatformError: Unable to find vcvarsall.bat
Getting your Python environment configured correctly with all the needed extensions and libraries is intimidating especially if you’re just starting out. However, little troubleshooting steps like this one, forms an integral part of a coder’s life, enhancing learning by practical exposure.
Remember, Stackoverflow Python tag is a great resource for Python related queries and clarifications posted and solved by excellent worldwide Python community.The error message “Microsoft Visual C++ 14.0 or greater is required” generally appears when the system fails to find the correct versions of Microsoft Visual C++ Build Tools while installing Python packages. To resolve this problem, you should follow these steps:
Install Microsoft Build Tools
The first and foremost risk-free solution is installing the Microsoft Build Tools on your own. You can download it from the official Microsoft website in this link.
Please remember: You must choose the version that matches with your Python’s version. For example, for Python 3.x, you will need Microsoft Build Tools for Visual Studio 2019 or later.
xxxxxxxxxx
python --version
If you still get the error, this means the path where your system is looking for requires the tools isn’t correctly pointed. Then, let me show you how to address specifically visual studio code to solve the error.
Addressing Visual Studio Code Specifically
Firstly, we are going to ensure if Microsoft Visual Studio Code is able to recognize the Build Tools.
xxxxxxxxxx
# Check python installation
python --version
# Check pip version
pip --version
Before moving forward, generate a ‘.json’ file for compiling in Python from MSVC (Microsoft Shared-Source CLI language System) by adding the following code:
xxxxxxxxxx
{
"tasks": [
{
"taskName": "echo",
"type": "shell",
"command": "echo Hello"
}
],
"version": "2.0.0"
}
Then, save the ‘.json’ file as ‘c_cpp_properties.json’ in the directory: .vscode
Now, verify if Visual Studio Code or other text editors properly identify the Microsoft Build Tools.
If those mentioned solutions don’t work out for you, then we have alternatives that bypass the requirement for the build tool altogether.
Bypassing Microsoft C++ Build Tools Requirement Through Binary Python Packages
Instead of using source packages which require compilation, you could opt for binary packages with precompiled binary contents. These binary distributions help to eliminate the need to have Microsoft C++ Build Tools installed.
For Windows, such binary packages are distributed as ‘wheel files’. You can utilize Unofficial Windows Binaries for Python Extension Packages to find ‘wheel files’ for many popular python packages. Make sure you select the correct ‘.whl’ file that matches your Python version and system architecture (32-bit / 64-bit).
Download the relevant ‘wheel file’, then use the pip command in terminal:
xxxxxxxxxx
pip install wheel_file_name.whl
Remember, in the above command replace ‘wheel_file_name.whl’ with the downloaded file’s actual name. This way helps bypass the traditional route that would have otherwise necessitated the use of Microsoft C++ Build Tools.
Though useful, keep in mind using wheel files might not always be practical for every Python package. But for common and frequently-used Python libraries like NumPy, SciPy, and pandas, they provide a suitable alternative.
Periodically, the engagement with software will be all about troubleshooting and overcoming difficulties on-the-go. Succeeding online forums and official documentation play a fundamental role in providing us aids and helpful insights in these moments. Always go through similar scenarios/people who have faced related issues before on platforms like Stack Overflow or GitHub. You may find what you’re seeking or even better – stumble upon new paths that take you to the answer.
Let’s dive in with a clear understanding of the issue at hand. When you get an error saying that
xxxxxxxxxx
'Microsoft Visual C++ 14.0 or greater is required'
, it is usually because you’re trying to install or build a Python package, from source, which includes C, C++, or Cython extensions.
For instance, when you are installing packages using pip, like lxml, greenlet, gevent, etc., you’re essentially downloading the source code and compiling it on your machine. Compilation of such packages requires certain dependencies to be installed.
Now, these errors occur because, this compilation process specifically needs Microsoft’s C++ Build Tools which includes compiler, linker, and other software development tools specific to Windows. If these aren’t installed on your machine, the compiler won’t be able to build the package, hence leading to the aforementioned error.
Here comes the important aspect as how we can solve this particular problem. You can mitigate the error
xxxxxxxxxx
'Microsoft Visual C++ 14.0 or greater is required'
by following the below mentioned steps:
Step 1 – Ensure that your system has the latest version of pip installed. If not, you can upgrade pip using below command:
xxxxxxxxxx
python -m pip install --upgrade pip
Step 2 – Now, navigate to the webpage for Microsoft’s C++ Build Tools. Click on the ‘Download’ button to download them.
Step 3 – Once the installer starts running, make sure you select the “Workload” tab, then scroll down and check the ‘C++ Build Tools’ checkbox.
Step 4 – Navigate over to the right and under Installation details, ensure that under “Optional” the ‘MSVC v142’ and ‘Windows 10 SDK’ options are selected.
Step 5 – Finally, finish the installation by clicking on the ‘Install’ button.
It is noteworthy that your system may require a reboot after the successful installation.
After completing the above-mentioned steps, go back to your terminal or command prompt and try reinstalling the Python package that previously failed due to this error. It should now install successfully as all necessary components are available for your use now.
For example,
xxxxxxxxxx
pip install packageName
Keep in mind, however, that while this procedure will likely solve most issues stemming from this error, there might still be some variations depending on factors such as your operating system, Python environment configuration, and the specific package you’re attempting to install.
Also, remember that it is always good practice to have a virtual environment setup while working with Python projects. This helps avoiding any package conflict issues that may arise due to different project dependencies. The Python venv module makes it easy to create lightweight “virtual environments” with their own site directories, optionally isolated from system site directories.
In short, understanding why you encounter the
xxxxxxxxxx
'Microsoft Visual C++ 14.0 or greater is required'
error and how you can solve it, paves the road for seamless execution and installation of Python packages. Happy coding!
We’re diving into a common issue Python developers often face when working with the Windows platform: the dreaded “Error: Microsoft Visual C++ 14.0 or greater is required” when trying to install Python packages, especially those involving compilation of C or C++ code like pandas, numpy, or lxml.
Fortunately, you don’t need to churn your brain gears too much as correcting it might be simpler than you think. The main reason behind this error is the absence of the required build tools, compiler, and libraries, specifically, Microsoft Visual C++, which is essential for compiling a Python extension.
Using pip
The first solution I’d recommend using is
xxxxxxxxxx
pip
, Python’s built-in package manager. Versions starting from 19.0 can detect if the build dependencies are missing and prompt the user with a more helpful error message.
If you see an error prompting you to install Microsoft Visual C++ 14.0 or greater, we can instruct pip to use wheel files, precompiled binary packaged versions of the library so that it does not require any additional compilation.
First, update pip to its latest version by running this command in your command terminal:
xxxxxxxxxx
python -m pip install --upgrade pip
Then we instruct pip to prefer binary wheels over source distributions with this command:
xxxxxxxxxx
pip install --prefer-binary <package-name>
Just replace <package-name> above with the Python package you want to install, e.g numpy.
Windows Build Tools
If the earlier solution does not work or the package you’re going to install does not provide wheel files, we need to set up build tools manually. There is a Python package called setuptools which helps in this scenario. It will download and install all requirements for building extensions.
First, install the setuptools package using pip:
xxxxxxxxxx
pip install setuptools
Next, install the necessary build tools:
xxxxxxxxxx
pip install setuptools setuptools_scm wheel
Visual Studio Build Tools
As a last resort, if previous attempts have failed, you should consider directly installing Visual Studio and its associated build tools. Specifically, you would need the C++ build tools that come with Visual Studio. This can be a bit more tedious in terms of both time and disk space but ensures proper setup of all the required components.
Follow these steps to set up the environment:
- Head over to the official Visual Studio downloads page.
- Select ‘Build Tools for Visual Studio’ and proceed to download.
- Run the installer and choose the “C++ build tools” workload. Make sure to check the options for the latest MSVCv142 – VS 2019 C++ x64/x86 build tools and Windows 10 SDK.
- Finally, click ‘Install’
xxxxxxxxxx
Note:
Make sure you have adequate disk space (about 5-6GB) to run the installer and complete the setup.
After successfully setting up Visual Studio and its C++ build tools, try running the Python package installer again.
Addressing the “Microsoft Visual C++ 14.0 or greater is required” error order: update pip and prefer binary packages, leverage setuptools to handle requirements, or manually add Visual Studio build tools.
Remember, every system configuration is unique. So don’t be discouraged if the first attempt doesn’t resolve it – subsequent solutions may be required depending on what’s missing from your local development environment.
We often encounter the error message
xxxxxxxxxx
Error: Microsoft Visual C++ 14.0 or greater is required
when attempting to install Python packages such as `pandas`, `numpy`, `scipy`, etc. This is usually because these libraries rely on specific functionalities provided by MSVC++ which is not installed in our local system. Here are the step-by-step guidelines to solve this issue:
1. Installation of Microsoft Build Tools:
Start with downloading and installing the latest version of Microsoft Build Tools. It’s a software package that provides the necessary components which are used for building C++ applications. Here’s an example code to download the exe file using Windows Powershell:
xxxxxxxxxx
$url = "https://go.microsoft.com/fwlink/?LinkId=691126"
$output = "$env:USERPROFILE\Downloads\ms_build_tools.exe"
Invoke-WebRequest -Uri $url -OutFile $output
& $output
2. Configuration of Microsoft Build Tools
During installation, ensure that you include
xxxxxxxxxx
"C++ build tools"
, and they should be checked along with the latest versions of
xxxxxxxxxx
"MSVCv142 - VS 2019 C++ x64/x86 build tools"
and
xxxxxxxxxx
"Windows 10 SDK"
.
3. Installing Python Packaging Tools:
Ensure that you have updated versions of `setuptools`, `wheel` and `pip`. Use the following commands on your command line or terminal to upgrade:
xxxxxxxxxx
python -m pip install --upgrade pip setuptools wheel
4. Environment Variables Update:
To make sure that Python can find and load the newly installed library, we must update the PATH environment variable:
xxxxxxxxxx
[System.Environment]::SetEnvironmentVariable('Path', $env:Path + ';C:\path\to\your\cpp\build\tools', [System.EnvironmentVariableTarget]::User)
Replace
xxxxxxxxxx
C:\path\to\your\cpp\build\tools
with the path where you’ve installed the C++ build tools.
5. Re-install The Python Packages:
After accomplishing all the above-mentioned steps, try reinstalling the packages that were initially causing the errors. For instance, if you were trying to install `pandas`, you would use this command:
xxxxxxxxxx
pip install pandas
Completing these steps should help to resolve the
xxxxxxxxxx
Error: Microsoft Visual C++ 14.0 or greater is required
error that was obstructing the installation of Python packages. This guide is centered on setting up your environment appropriately so as not to run into this error while working with these commonly used Python libraries. By adequately setting up your environment, you’ll enhance its operational efficiency and thereby boost your productivity.As a seasoned coder, I’ve often encountered various types of error messages while installing Python packages. One common error message that frequently crops up is “Error: Microsoft Visual C++ 14.0 or Greater is Required”. This error message can occur due to the absence or incompatibility of the existing version of Microsoft Visual C++ on your computer system. However, don’t worry as this problem can be easily fixed by following the comprehensive troubleshooting steps outlined below.
## Using Windows Binary Packages
The “Microsoft Visual C++ 14.0 or Greater is Required” error primarily occurs when you are trying to install Python packages which require compilation against C++ libraries provided by MSVC. `pip`, the most commonly used package manager for Python, tries to do this natively and fails if the required components aren’t found.
The simplest workaround, if the package supports it, is to use precompiled binary packages. Here’s how to do it:
xxxxxxxxxx
pip install --only-binary :all: PACKAGE-NAME
Just replace `PACKAGE-NAME` with the package you’re trying to install.
## Install Build Tools
In case the above method doesn’t work, the next step is to install the Microsoft Visual C++ Build Tools. You can download the latest version directly from Microsoft’s webpage.
After downloading, run the installer and select “Workloads” then check the box that says “C++ build tools.” The installation may take some time as it downloads over 1GB of build tools.
It’s important to note that you should restart your command prompt or PowerShell after the Microsoft Visual C++ Build Tools installation process has been completed successfully.
## Install Older Versions Of C++
Sometimes, the error may still persist after installing the C++ build tools. This happens if the Python package you’re attempting to install depends specifically on older versions of Microsoft VC++. Here’s how you can install an older version of VC++:
Here is an example for installing microsoft visual studio 2015:
1. Visit the Older Downloads page on the visual studio website.
2. Sign in and scroll down to find “Redistributables and Build Tools.”
3. Find and download “Microsoft Visual C++ 2015 Redistributable Update 3.”
After installing this, your package should install smoothly.
Remember, stack traces are your best friend! They often contain valuable information that can help you narrow down the exact problem and find a viable solution. If the suggestions above didn’t resolve your issue, reread the error messages carefully for hints about what else might have gone wrong. Happy coding!I understand that you are experiencing an error message stating
xxxxxxxxxx
'Microsoft Visual C++ 14.0 or Greater is Needed'
when trying to install Python packages. This issue arises primarily due to the lack of necessary compiling resources on your system, which are required for some Python packages.
To help you resolve this problem, let’s delve into how to first verify and then rectify this situation.
[Step 1] Verify The Presence of Microsoft Visual C++ Redistributable Package:
The Microsoft Visual C++ Redistributable package includes library files needed to run applications developed using Visual C++, an integrated development environment from Microsoft. You should check if this package is already installed on your computer.
– Press
xxxxxxxxxx
Win + S
to bring up your computer’s search function, then type in ‘Add or remove programs’.
– Scroll through the list to see whether ‘Microsoft Visual C++ 2015-2019 Redistributable’ is available.
If not, it means you need to install it.
[Step 2] Install Microsoft Visual C++ Build Tools:
For certain Python packages like twisted, pyaudio etc., the key to resolving this error lies in installing a component named
xxxxxxxxxx
'Microsoft Visual C++ Build Tools'
. Here are the instructions:
– Visit the official Microsoft website to download Visual Studio 2019 Community Edition.
– Run the installer, select
xxxxxxxxxx
'Modify'
in the Visual Studio Installer window.
– Look for
xxxxxxxxxx
'Desktop development with C++'
workload.
– Check the box for this workload then click
xxxxxxxxxx
'Modify'
at the bottom right of the window.
This would set up all compilation resources needed for python packages installation including Microsoft Visual C++ 14.0.
[Step 3] Reinstall the Python Package:
After having installed the `Microsoft Visual C++ Build Tools`, you should be able to install the Python packages without encountering the problem now. Use pip command to install the package. For example, if you want to install the Twisted package, use:
xxxxxxxxxx
pip install twisted
Remember to replace
xxxxxxxxxx
'twisted'
with your target package name.
These steps should ideally resolve the error mentioning
xxxxxxxxxx
'Microsoft Visual C++ 14.0 or Greater is Needed'
. The process mentioned above helps create an environment where the necessary dependencies are available for the Python package being installed.
Remember, coding is about tinkering and troubleshooting. Issues crop up, but the process of resolving them enhances understanding. So keep this momentum going, and soon enough, these hitches will become second nature for you to resolve.Solving the “Error: Microsoft Visual C++ 14.0 or greater is required” issue when installing Python packages requires understanding of the various dependencies within the Microsoft and Python ecosystem.
When you encounter this error during package installation in Python, it’s due to the absence of an essential software dependency, namely Microsoft Visual C++. To rectify this, you need to install Microsoft Visual C++ Build Tools. After reaching the website, proceed with downloading and installing the software.
Here’s a glimpse of how the command line interface (CLI) error message might look:
xxxxxxxxxx
$ pip install [package-name]
Error: Microsoft Visual C++ 14.0 is required
The error blocker essentially stems from the fact that some Python libraries were written in C++, and require Microsoft Visual C++ for compilation.
In order to solve it, navigate to the build tools download page and then execute the installer. Follow these steps:
- Choose custom install
- Tick the box signalling the latest C++ build tools.
- Finish the installation process by following the prompts on-screen
Post-installation, your system needs to restart. Provide any confirmation if necessary and wait while the PC reboots. The next time you install Python Packages, the “Error: Microsoft Visual C++ 14.0 or greater is required” shouldn’t appear anymore.
As a sidenote, always keep your systems updated. It’s a great practice to regularly check softwares like Python and its libraries, avoiding the arising of such errors and ensuring a smooth coding experience.
I hope this can streamline your journey in Python package installations and grooms you to handle similar software dependency issues in the future. Feel free to check detailed troubleshooting guides and forums, like Stack Overflow thread, for more insights about such problems. Happy Coding!