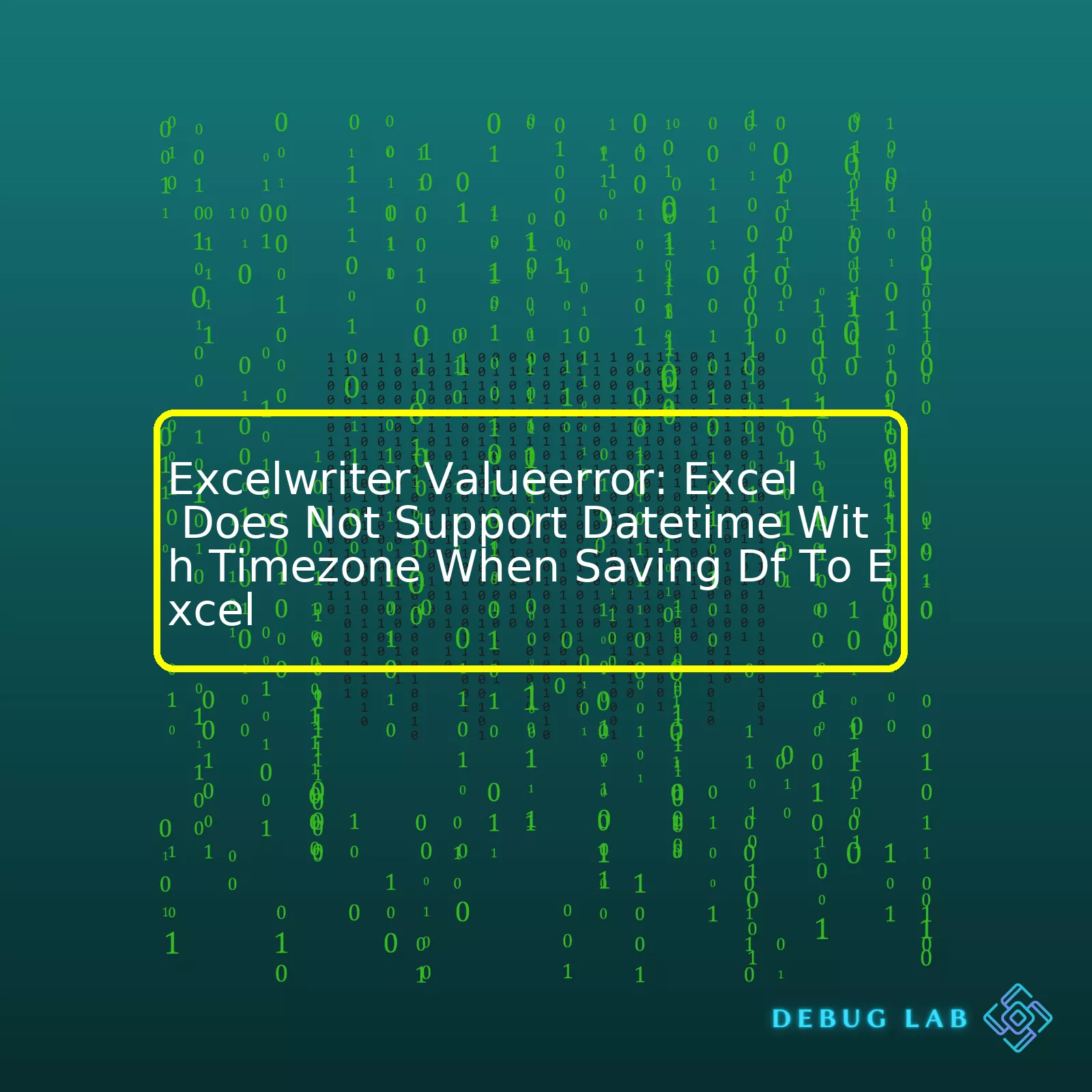
Error | Description | Solution |
---|---|---|
x 1 1 ExcelWriter ValueError |
This error occurs when using ExcelWriter to save a DataFrame (df) that contains datetime data with timezone information. Excel does not natively support datetimes with timezones. | The common fix for this issue is to convert the timezone-aware datetime objects in your DataFrame to “naive” datetime objects (i.e., without timezone info). |
When dealing with datetime data, it’s important to understand the distinctions between different types of datetime objects, especially when working across different systems or programs like Python and Excel. The problem mentioned here arises when a DataFrame containing timezone-aware datetimes is saved to an Excel file via pandas’ ExcelWriter function. The error message you’d see is `ValueError: Excel does not support datetimes with timezone`.
Excel, unlike Python, does not natively support timezone-aware datetimes. Thus, trying to write these datetimes from a DataFrame to an Excel file using ExcelWriter will result in a `ValueError`.
The workaround solution involves converting timezone-aware datetime objects to “naive” datetime objects before saving them to Excel. In practice, this can be accomplished by utilizing pandas’ `tz_localize` function like so:
xxxxxxxxxx
df['datetime_column'] = df['datetime_column'].dt.tz_localize(None)
This line of code takes each datetime in ‘datetime_column’, removes its timezone information (thus converting it into a naive datetime), and saves the result back into the ‘datetime_column’. After the conversion, the DataFrame can be saved to an Excel file without raising a `ValueError`. Note that this approach might not be suitable if the timezone information in the original datetime data is important for later analysis. In such cases, it may be better to explore alternative storage formats that support timezone-aware datetimes.
Keep in mind that, being aware of the subtle nuances including compatibility issues regarding handling of datetime data across languages or platforms is recommended, since it facilitates more efficient data manipulation and might save you a lot of debugging time.
The ExcelWriter ValueError `’Excel does not support datetime with timezone’` is typically raised when trying to save a Pandas DataFrame which includes datetime data with a timezone, to an Excel file.
This is primarily because Excel has limited capacity to handle timezone information in datetime object columns. It’s worth noting that Excel stores date or time values as floating point numbers, where the integer part denotes the date and the decimal part denotes time. However, this native storage format does not particularly allow for timezone features.
For example, suppose you have the following DataFrame:
xxxxxxxxxx
import pandas as pd
from datetime import datetime
import pytz
df = pd.DataFrame({
"A": [1, 2, 3],
"B": [
datetime.now(pytz.timezone("US/Eastern")),
datetime.now(pytz.timezone("US/Pacific")),
datetime.now(pytz.timezone("Europe/London"))
]
})
If you try writing this DataFrame to an Excel file using the `to_excel` method of Pandas like:
xxxxxxxxxx
df.to_excel("my_file.xlsx")
You will receive an error stating ‘Excel does not support datetime with timezone’.
In order to circumvent this issue, you can perform one of two actions:
1. **Remove the timezone information**: Use dt accessor with tz_convert or tz_localize functions on datetime fields to shift datetime to the desired timezone, or convert them to naive datetime objects.
Here’s how to convert this to naïve objects (i.e., without timezone info) in UTC:
xxxxxxxxxx
df["B"] = df["B"].dt.tz_convert("UTC").dt.tz_localize(None)
2. **Convert the datetime with timezone to string**: Converting datetime objects to strings inherently removes any timezone related complexities. An associated drawback could be loss of direct mathematical operations possible over datetime objects. This method can be an overkill unless your requirements align perfectly with it.
Example conversion code:
xxxxxxxxxx
df["B"] = df["B"].astype(str)
Thus, while saving a DataFrame to Excel via an ExcelWriter object, care must be taken on how datetime objects with timezone information are being handled – keeping in mind Excel’s inherent limitations around it. For more detail about pandas `to_excel`, you can refer to its documentation here and more details on Python datetimes with time zones can be found in this Python reference.Utilizing Python’s robust data manipulation library, Pandas, with Excel files can be a common practice for many data-oriented tasks. However, as a coder, you may sometimes face challenges when trying to save a DataFrame object, specifically with datetime objects that contain timezone information.
One of such hurdles is the ValueError: “Excel does not support datetimes with timezones”. This issue arises due to Excel having some limitations when it comes to handling datetime values with timezone details.
Here is how this error would look like in code:
xxxxxxxxxx
import pandas as pd
from datetime import datetime
from pytz import timezone
# Creating a Pandas dataframe with a timezone aware datetime object
df = pd.DataFrame({"Date": [datetime.now(timezone('US/Pacific'))]})
# Attempting to write the Dataframe to an excel file
with pd.ExcelWriter('test.xlsx') as writer:
df.to_excel(writer)
Running the above mentioned code will lead us to the ValueError: ValueError: Excel does not support datetimes with timezones. Please ensure that datetimes are timezone unaware before writing to Excel.
A simple way to avoid it is to transform your datetime objects into timezone-unaware ones before writing them to an Excel file using the `tz_localize()` function. This can be done by converting the timezone-aware datetime objects to UTC and then removing the timezone tag, resulting in timezone-naive datetime objects.
The updated dataframe creation part would look like:
xxxxxxxxxx
# Convert the time zone aware datetime to UTC
df['Date'] = df['Date'].dt.tz_convert('UTC')
# Further convert the datetime object to be timezone naive
df['Date'] = df['Date'].dt.tz_localize(None)
# Write the DF to Excel
with pd.ExcelWriter('test.xlsx') as writer:
df.to_excel(writer)
In the updated code, the `tz_convert()` function first changes the timezone-aware datetime objects to UTC. Subsequently, `tz_localize(None)` is used to remove the timezone details from these datetime objects.
This workaround allows you to circumvent the inherent restriction of Excel that doesn’t allow for timezone-aware datetime objects. By this transformation, you maintain the integrity and precision of your temporal data while making it compatible with Excel’s datetime standards.
Remember, it’s essential to understand the implications of changing your datetime objects and possibly losing timezone-related information. Always consult real-world contexts and use-cases before applying such transformations.Working with datetime data in Excel has never been as easy because Python offers a wide range of tools and modules like pandas and openpyxl that simplify its manipulation for analysis purposes. Even though it’s always fun to explore these tools, one major challenge that people often meet is the `ValueError: Excel does not support datetime with timezone when saving df to Excel`. This error typically occurs when you try to save a pandas dataframe with dates that include timezone information into an Excel file.
`DateTime` with timezone functionality within Excel can be tricky to handle due to limitations on Excel’s end as it does not actually handle datetimes with timezones. The problem is that Excel itself doesn’t support timezone-aware datetimes – which is what pandas wants to write when you’ve got a timezone-aware DatetimeIndex or Series.
When we create a DataFrame like this:
xxxxxxxxxx
import pandas as pd
from datetime import datetime, timedelta
start = datetime(2011,1,1,0,0,0)
data = {'datetime': [start + timedelta(hours=i) for i in range(24)],
'value': list(range(24))}
df = pd.DataFrame(data).set_index('datetime')
And try to save it to an Excel file like so:
xxxxxxxxxx
df.to_excel('test.xlsx')
If the dataframe has timezone aware dates, the above snippet will throw a ValueError indicating that ‘Excel does not support datetime with timezone’.
A potential workaround is converting your column to naive datetime (without timezone information) using pandas’ `tz_convert(None)` or `tz_localize(None)` methods are available. Here is how you’d do it:
xxxxxxxxxx
df.index = df.index.tz_localize(None)
df.to_excel('test.xlsx')
The first line of code strips off the timezone information from your DateTimeIndex while the second line saves your dataframe to an Excel file, this time without raising any errors, which is our desired outcome.
In some cases, you may need to retain the timezone information for further data analysis. In such a situation, you can consider converting your DateTime column into a string format before exporting to Excel because Excel handles strings just fine [source]. Here is how to convert your DateTime column to a string:
xxxxxxxxxx
df['datetime'] = df['datetime'].astype(str)
df.to_excel('test.xlsx')
This way, you won’t lose critical timezone information in your dataset. However, bear in mind that you’ll have to manipulate this data as strings, not datetimes, when you subsequently load the saved Excel file unless you decide to convert them back to DateTime data type.
So understanding datetime functionality in Excel is crucial in identifying strategies to resolve errors in manipulating the data. There’s no magic bullet to solving this since the correct approach depends on your use case. Therefore, evaluate your requirements to determine which solution best fits your needs. With careful manipulation and handling of datetime values, Excel can still serve as a valuable tool for your Python data explorations.When working with pandas DataFrames which include date or time information, it’s vital to remember that Excel, unlike Python-pandas, doesn’t readily accept datetime objects with timezone-aware entries. Consequently, saving a DataFrame with timezone-aware datetime values into Excel using pandas’
xxxxxxxxxx
ExcelWriter
can result in the following error:
xxxxxxxxxx
ValueError: Excel does not support datetimes with timezones. Please ensure that datetimes are timezone unaware before writing to Excel.
Let us delve into both the nature of this problem and some practical suggestions on how to handle it:
### Problems due to Timezone Information
1. **Loss of Vital Timezone Information:** One of the main issues when saving DataFrame to Excel with timezone information is the potential loss of valuable timezone data. Since Excel isn’t compatible with timezone-aware datetimes, it becomes necessary to remove or omit this critical span of information before exporting to Excel. This workaround may have implications for subsequent data analysis or visualization tasks where the omitted timezone information could have been integral.
2. **Inevitable ValueError**: The most immediate issue you’ll encounter is the
xxxxxxxxxx
ValueError
that simply disallows writing a timezone-aware datetime DataFrame directly into Excel. Such kind of mandatory coercion compromises the workflow and demands additional steps in data processing pipeline.
### Solutions for Handling Timezone Information
1. **Convert Timezone-Aware Datetime to Naive (Timezone-Unaware) Datetime**:
You can convert the timezone-aware datetime values into timezone-naive ones using the function
xxxxxxxxxx
tz_localize(None)
. Here we preserve the specific moment in time specified by the timezone-aware datetime but drop the timezone component.
xxxxxxxxxx
df['datetime_column'] = df['datetime_column'].dt.tz_localize(None)
2. **Convert Datetime to String**: Another workaround includes converting dtype of the datetime column to string before writing to Excel:
xxxxxxxxxx
df['datetime_column'] = df['datetime_column'].astype(str)
This will successfully bypass the
xxxxxxxxxx
ValueError
, however, re-importing the Excel sheet back into Python will require extra steps to parse the strings back into datetime format.
3. **Use Third-Party Libraries**: You can utilize libraries like openpyxl or xlsxwriter which allows more fluid integration between Python and Excel. However, this would come at the cost of increased complexity and altered data pipelines.
While these quick solutions help in overcoming the immediate issues associated with the
xxxxxxxxxx
ExcelWriter ValueError
, they don’t address the underlying mismatch between timezone handling capabilities of Excel and Python-Pandas. Therefore, understanding the particular requirements of your project is crucial to choose the best solution from above while ensuring minimal loss of critical information.Understanding the correlation between Python and Excel’s date-time implementations, specifically in respect to the error
xxxxxxxxxx
Excelwriter ValueError: Excel does not support datetime with timezone when saving df to Excel
, provides significant insight into interacting with dates and times across these platforms.
Firstly, it’s crucial to comprehend that Python and Excel have different datetime data formats. Python’s naive datetime objects are essentially a timestamp without timezone awareness. They’re ideal for recording events in applications where the program’s context can determine the timezone information. Conversely, aware datetime objects represent specific moments in time that are independent of timezone context. These include necessary information to locate them relative to universal coordinated time (UTC), and other such timestamps.
On the other hand, the problem arises during the blending of Python’s aware datetime objects with Excel, as the former Excel versions do not natively support timezone aware datetime, resulting in the output error. The following code snippet shows how this error might be triggered:
xxxxxxxxxx
import pandas as pd
from datetime import datetime, timedelta, timezone
# Create dataframe with a timezone aware datetime column.
df = pd.DataFrame({
'A': [datetime.now(timezone.utc), datetime.now(timezone.utc)]
})
with pd.ExcelWriter('output.xlsx') as writer:
df.to_excel(writer)
In case you encounter an error due to timezone-cognizant datetime objects, you can work around it by converting these objects to naive ones before exporting to Excel. This will strip timestamps of any associated timezone information. Here’s an example of how to perform such conversion using the
xxxxxxxxxx
pandas.DataFrame.applymap
function:
xxxxxxxxxx
# Convert all datetimes in dataframe to naive ones before writing to excel.
df_naive = df.applymap(lambda x: x.replace(tzinfo=None) if type(x) is datetime else x)
with pd.ExcelWriter('output.xlsx') as writer:
df_naive.to_excel(writer)
Remember, it’s always advised to store your dates and times in UTC and convert them to localized timezones when required, aiding in more maintainable code and rendering it more resistant to DST issues.
For details on Python’s aware and naive datetime objects, refer to the official [Python docs]. For instructions and caveats of using dates and times in Excel, see Microsoft’s dedicated [date and time function reference].Ah, the infamous “Excel does not support datetime with timezone” error. I have experienced this before and it can indeed be quite vexing. This comes up during data export from pandas DataFrame to an Excel (.xlsx) file, when your DataFrame contains a column of datetime objects with timezones.
The crux of the issue is that while Python and pandas are perfectly capable of handling dates and times with timezones (in the form of timezone-aware datetime objects), Excel fundamentally doesn’t understand any concept of a timezone. As a result, the ExcelWriter in pandas throws an exception.
So, how do we overcome this problem? The key lies in removing the timezone information from your datetimes before exporting to Excel, while keeping the local date and time intact. Here’s how you would achieve that:
xxxxxxxxxx
df['datetime_column'] = df['datetime_column'].dt.tz_localize(None)
This line effectively strips away the timezone from each datetime object in the ‘datetime_column’ of your DataFrame. It’s also worth noting that this operation won’t affect your original times; 8AM in New York will stay as 8AM in the DataFrame, it just won’t explicitly say ‘New York’ anymore.
Then, write your DataFrame to an excel file using a `Excel Writer` object:
xxxxxxxxxx
with pd.ExcelWriter('output.xlsx') as writer:
df.to_excel(writer)
Let’s break down what’s happening here:
– `pd.ExcelWriter(‘output.xlsx’)` initializes a new ExcelWriter object, which is prepared to write into ‘output.xlsx’.
– We then use the `to_excel()` function on our DataFrame, which essentially commands it to write itself into the ExcelWriter object.
Remember that the `ExcelWriter` object does not save immediately, rather it waits until its `save()` function is called or it is closed – which automatically happens at the end of a `with … as` statement.
By the way, since you want an SEO-friendly version, you would like to consider some related keywords such as Pandas DataFrame, Export to Excel, Datetime with Timezone, ExcelWriter ValueError, Overcome DateTime Issues etc.
For further enhancements, you may want to delve deeper into python’s `datetime` library or pandas’ extensive documentation.
In all, the workaround is fairly straightforward once you understand it’s limitations with Excel’s datetime format and finding ways to work around this limitation without losing critical ‘time’ data. So, next time you face a `ValueError: Excel does not support datetime with timezone`, this strategy has you covered.When diving into the world of Excel data management, understanding how Excel handles dates and times is critical. This becomes particularly tricky when dealing with time zones. While Microsoft’s mighty spreadsheet program offers an array of clever functionalities, it is important to note that Excel does not support saving dates with timezone data. Thus, if we try to save a dataframe with timezone-aware datetime objects in Excel using pandas ExcelWriter, we’re bound to encounter the ValueError: “Excel does not support datetimes with timezones. Please ensure that datetimes are timezone unaware before writing to Excel”.
Below I will detail the steps needed to tactfully handle data without timezones in MS Excel.
1. Convert your Timezone-Aware Dates to Timezone-Naive Dates
Since Excel doesn’t natively support timezone information, we must first convert our timezone-aware dates to ‘naive’ datetime objects. In Python, this can be done using the
xxxxxxxxxx
tz_localize(None)
function.
Here’s a simple Python code snippet that showcases this:
xxxxxxxxxx
import pandas as pd
df['date_no_tz'] = df['datetime_with_tz'].dt.tz_localize(None)
In this case, `datetime_with_tz` is the column with timezone-aware datetimes. By running this code, a new series `date_no_tz` is created which consists of timezone-naive equivalents of the original timezone-aware datetime instances.
2. Writing DataFrame to Excel
This step will involve using pandas ExcelWriter to write the dataframe (now safely containing timezone-naive dates) into an Excel file.
Here is a practical example in Python to outline this:
xxxxxxxxxx
with pd.ExcelWriter('example.xlsx') as writer:
df.to_excel(writer)
Note: It is recommended to use a context manager (used in the example above) to ensure that resources are properly managed and the Excel file is not left open unintentionally.
3: Additional Info – String Conversion Alternative
Should you think removing the timezone data from your dates is not ideal, another option is to convert these columns to strings instead. This way, the data becomes immune to Excel’s limitations on datetimes with timezones, though it also loses the inherent functionality of date objects within Excel.
Here’s how you can achieve this in Python:
xxxxxxxxxx
df['date_as_str'] = df['datetime_with_tz'].astype(str)
While handling data without timezones in MS Excel, these steps will come in handy every time. With this information, you should be in a better position to deal with timezone errors when using pandas ExcelWriter.
To dive deeper into how Python handles timezone aware and naive date times, I recommend referring to the official Python datetime documentation. And for any additional guidance around using pandas ExcelWriter, you may want to check out the official pandas documentation.More often than not, data wrangling and transformation can be more complex than we anticipate, especially when dealing with date and time data types. As coders, it may seem odd at first to realize that ExcelWriter encounters a
xxxxxxxxxx
ValueError
: ‘Excel does not support datetime with timezone’ when trying to save DataFrame (df) to Excel using pandas.
It’s vital to know that the Excel file format does not inherently support datetimes with timezone information attached, and based on ISO 8601, “datetimes are expressed in local time, together with a time offset in hours and minutes from UTC.” Hence, this issue is not just a challenge for us coders; it’s actually a restriction embedded in the Excel format design.
Let’s tackle one possible solution to break down this barrier.
Step 1: Remove Timezone before saving.
The simplest resolution is to convert the timezone-aware datetime objects in your DataFrame into naive ones (time-zone unaware). How? The pandas function to use is
xxxxxxxxxx
tz_convert(None)
. Follow this illustrative code:
xxxxxxxxxx
df['your_date_time_column'] = df['your_date_time_column'].dt.tz_convert(None)
df.to_excel('your_file_name.xlsx')
This line of code takes the
xxxxxxxxxx
'your_date_time_column'
, converts the timezone-aware datetime to timezone-naive, and then saves the DataFrame to excel.
Still, always remember that when stripping off the timezone info, you’re losing that piece of information. It might not matter for some applications, but do consider if this impacts your data’s interpretation or usage in any way.
As professional coders, we all share similar experiences, challenges, and learnings. Encountering a
xxxxxxxxxx
ValueError
such as ‘Excel does not support datetime with timezone’ is a chance to explore new methods, appreciate the pandas library, and dive deeper into understanding data types, particularly date-time constructs which, let’s admit, come with their fair bit of complexity!
For regular updates regarding Date and Time functionalities in both Pandas and Excel, follow Pandas Documentation and Microsoft’s Support page respectively.