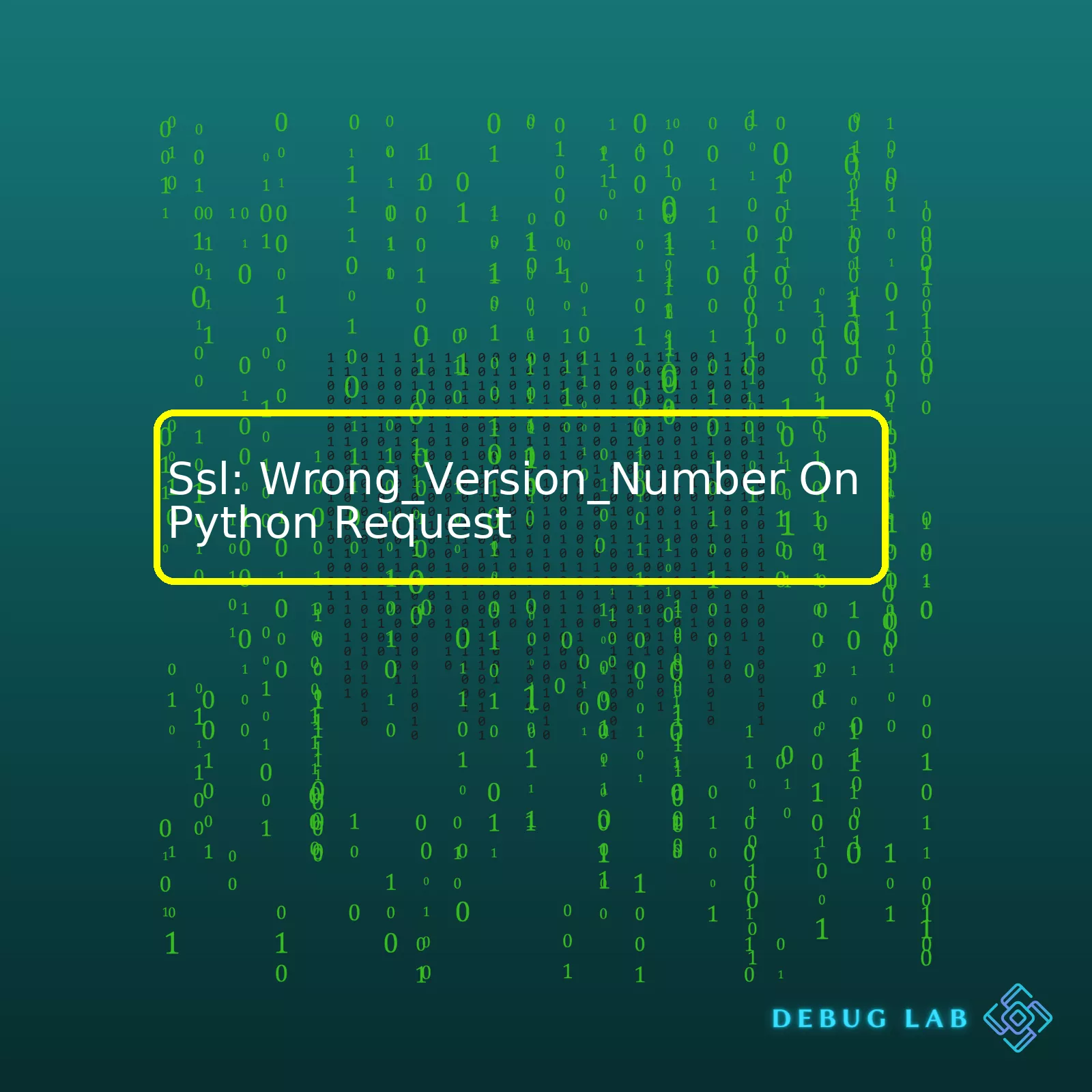
Error | Description | Solution |
---|---|---|
Ssl: Wrong_Version_Number On Python Request | This error typically arises when the server you are trying to connect to is not supporting the SSL/TLS protocol version that you specified in your request. | If the error persists, it might be advisable to either downgrade the version of your SSL/TLS or upgrade the server’s SSL/TLS version. Furthermore, it is highly recommended to validate any proxies or firewalls that could be possibly causing this issue. |
A deeper interpretation of this table would mean understanding what exactly ‘ssl: wrong_version_number’ on Python Request implies. When this error occurs, it primarily means that there’s a mismatch between the Secure Sockets Layer (SSL) or Transport Layer Security (TLS) versions that the client (in this case the Python Request library) and the server use to mutually authenticate each other and create an encrypted connection.
In such scenarios, it’s critical to first identify the SSL or TLS versions supported by the server. Most Python libraries including
requests
have built-in capability to support multiple versions of the protocols right from TLSv1.0 to even the very latest TLSv1.3.
However, when an explicit version is set using
xxxxxxxxxx
ssl_context = ssl.SSLContext(ssl.PROTOCOL_TLSv1_2)
, then Python will only attempt to establish the connection using that specific protocol version. If the server doesn’t support it, you wind up with the ‘ssl: wrong_version_number’ error.
To solve this issue, you may want to:
– Try connecting without specifying a particular SSL or TLS version. This lets your
xxxxxxxxxx
requests
module decide on which version to use based on its negotiation with the server
– Or manually adjust the version you have specified to align with what the server supports.
In addition to the solutions mentioned in the table above, considering checking if any network securities like proxies or firewalls in place are contributing to the problem. Network securities are essentially fortification mechanisms designed to protect systems from threats over the internet.
However, their configurations can sometimes inadvertently block legitimate traffic, causing issues like the one we are discussing. They might, for instance, allow only certain versions of SSL/TLS through. It’s recommended then to check the accepted versions and make sure they jibe with what you are attempting to use in your Python code. Please refer to “Python’s official documentation on SSL” for more details.Sure, the “SSL: wrong_version_number” error typically occurs when you’re trying to make an HTTPS request using Python’s `requests` library but there’s a mismatch between SSL/TLS versions of the client and server.
Python’s requests module uses urllib3, which in turn employs python’s built-in ssl module for providing SSL/TLS support. The SSL/TLS version used is typically defined by the client or the server during the initial handshake. If the version defined by client isn’t supported by the server (or vice versa), this causes a miscommunication, resulting in the SSL: wrong_version_number error.
The SSL/TLS versions protocol can range from:
– SSL 2.0 (deprecated and insecure)
– SSL 3.0 (deprecated and insecure)
– TLS 1.0 (becoming deprecated)
– TLS 1.1 (becoming deprecated)
– TLS 1.2
– TLS 1.3
In certain cases where you’re dealing with systems still using outdated protocols due to legacy issues or strict corporate policies, you might encounter the “SSL: wrong_version_number” error.
Here’s a piece of code which illustrates a simple HTTPS request:
xxxxxxxxxx
import requests
def fetch_data(url: str):
response = requests.get(url)
return response.text
To troubleshoot and fix the “SSL: wrong_version_number” error in Python, follow these steps:
– Check your Python Version: Older versions of Python might not support newer SSL/TLS versions. At least Python 2.7.9 or Python 3.4 should be used because they introduced significant improvements to their ssl modules that included SNI (Server Name Indication) and hostname matching support.
– Adjust SSL/TLS Versions: Using tools like `pyOpenSSL`, you can adjust the SSL/TLS version used in the request. Note that reducing the SSL/TLS version may introduce vulnerabilities because older SSL/TLS versions have well known security flaws. Here’s how you can use pyOpenSSL to control the SSL version used:
xxxxxxxxxx
from requests_toolbelt.adapters import SourceAddressAdapter
from requests.packages.urllib3.util.ssl_ import create_urllib3_context
CIPHERS = (
'ECDH+AESGCM:ECDH+CHACHA20:DH+AESGCM:DH+CHACHA20:ECDH+AES256:DH+AES256'
':ECDH+AES128:DH+AES:ECDH+HIGH:DH+HIGH:RSA+AESGCM:RSA+AES:RSA+HIGH:!aNULL'
':!eNULL:!MD5'
)
class DESAdapter(SourceAddressAdapter):
def init_poolmanager(self, *args, **kwargs):
context = create_urllib3_context(ciphers=CIPHERS)
kwargs['ssl_context'] = context
return super(DESAdapter, self).init_poolmanager(*args, **kwargs)
Then add adapter to your session:
xxxxxxxxxx
s = requests.session()
s.mount('https://', DESAdapter('0.0.0.0'))
– Update OpenSSL: The problem may lie in an outdated OpenSSL library. OpenSSL is widely used in python to provide SSL support. Check the version of OpenSSL being used by your Python interpreter with `ssl.OPENSSL_VERSION`. You might want to update OpenSSL if its version is lower than 1.0.1 as versions below this do not provide complete support for TLS v1.2.
– Use HTTP instead of HTTPS: If it’s for testing purpose and the server also supports HTTP traffic, you might try replacing https with http in your URL. This is only a temporary solution and is not recommended for production workloads because data sent over HTTP is not encrypted.
If none of the above solutions work, it might be best to reach out to the server administrators to check what SSL/TLS version is supported by their system.
Source: [Python request documentation](https://docs.python.org/3/library/ssl.html), [official openssl documentation](https://www.openssl.org/docs/man1.1.1/man1/ciphers.html)The outbreak of “ssl: WRONG_VERSION_NUMBER” error, especially in Python requests, indicates that you’re trying to create an HTTPS connection with a server expecting a different SSL or TLS version. It’s crucial that both client and server agree on which protocol version to use during the SSL/TLS handshake process. This error arises due to the following reasons:
– SSL protocol mismatches: Such mismatches could result from outdated protocol versions handled by servers. For instance, if a server still uses SSLv3 and the client tries to establish a connection using TLS 1.2, this results in a disagreement causing the “ssl: WRONG_VERSION_NUMBER” error. Secure HTTP requests require up-to-date versions of SSL or TLS.
– Firewall or antivirus interference: Firewalls and antivirus systems might interfere with network traffic or modify it, leading to such errors. They may interrupt the SSL/TLS handshake process, presenting incorrect SSL/TLS versions to either client or server.
You can tackle these challenges in several ways:
– Updating your server-side SSL/TLS version: If you experience these mismatches and you have access to the server, updating the SSL/TLS version might resolve the issue. Most modern servers abide by the latest TLS version. However, servers dealing with sensitive data should support multiple versions for backward compatibility.
– Forcing your request package to use a specific SSL/TLS version if there is no option of updating the server-side SSL/TLS. Here’s one way to do this using `pyOpenSSL`:
xxxxxxxxxx
import requests.packages.urllib3.contrib.pyopenssl
requests.packages.urllib3.contrib.pyopenssl.inject_into_urllib3()
ssl_context = OpenSSL.SSL.Context(OpenSSL.SSL.SSLv23_METHOD)
ssl_context.set_options(OpenSSL.SSL.OP_NO_SSLv3)
https_pool = urllib3.connectionpool.HTTPSConnectionPool('somehost.com', port=443, ssl_context=ssl_context)
Here, we force the client to start the handshake with one specific SSL or TLS version using pyOpenSSL.
– Switching off your Antivirus/Firewall: If you are sure of the safety of the server-side application you are interacting with, temporarily switching off your antivirus/firewall to perform this network operation can bypass the problem. This is not recommended unless absolutely necessary due to security concerns.
The solution depends primarily on personal circumstances, including whether or not you have control over the server and how secure your client needs to be. Note that deprecated protocol versions have known vulnerabilities; therefore, don’t sacrifice security for functionality.
For example, SSL 2.0 and 3.0, as well as TLS 1.0, have been found insecure rfc-editor.org. If possible, aim for a TLS version of at least 1.2 or later, as they offer more advanced encryption algorithms and improved security features.
Remember, having the same SSL/TLS protocol on both sides is paramount. Regular server maintenance and keeping your Python libraries up-to-date will save you from a lot of these hassles.When you encountered an “ssl: WRONG_VERSION_NUMBER” error during your Python request workings, it is often due to SSL/TLS handshake failure. This could be caused by a mismatch between the SSL/TLS version utilized by the client (in this case, Python requests) and the one supported by the server.
To prevent these SSL version errors, the following are some best practices:
TLS Version Checking
Check which versions of Secure Socket Layer (SSL) or Transport Layer Security (TLS) your client and server support. Python Requests uses the SSL module from Python’s standard library, which then utilizes OpenSSL. The Python version used, and how it was compiled, can influence the available versions of SSL/TLS. Some servers don’t support older versions due to security vulnerabilities.
Here appears a minimal Python code to check the version:
xxxxxxxxxx
import ssl
print(ssl.OPENSSL_VERSION)
Upgrade Outdated Components
Ensure that your Python interpreter, Requests library, and OpenSSL are updated to reasonably recent versions to support modern and secure protocols like TLS 1.2 or 1.3. Specific versions would depend on your project requirements but as per OpenSSL, version 1.1.1 or later supports up to TLSv1.3 and is currently recommended for use.
As an example, upgrading Python, with Linux, can be done using the following command in terminal:
xxxxxxxxxx
sudo apt-get upgrade python3
While upgrading Python Requests can usually be achieved with pip:
xxxxxxxxxx
pip install --upgrade requests
Be Explicit on TLS Version When Possible
If you have control over the client and server, specify the exact version of SSL/TLS to use when making requests instead of relying on automatic negotiation, which may fail. In some libraries, like `requests`, this process can get complicated because it uses `urllib3` under the hood, which doesn’t provide an easy way, albeit possible, to change the SSL version used.
Ready the Client to Fallback to Lower Versions
Prepare your client to fallback to lower versions if higher versions fail in cases where the server still operates on an older version. You should do this cautiously, though, falling back to much older versions, such as SSLv3, exposes your system to potential security vulnerabilities.
Utilize Proxy as Bridge
Use a proxy, e.g., Nginx or HAProxy, that supports various SSL/TLS versions as a bridge between your client and server. The idea is to connect Python requests to the proxy using a mutually supported SSL/TLS version, then the proxy connects to the server on its supported SSL/TLS version.
The above tactics should help prevent `ssl: WRONG_VERSION_NUMBER` errors during your implementation of Python requests. However, bear in mind never to sacrifice security for compatibility. Procedures involving deprecated protocols endanger your data integrity and confidentiality. Make sure any downgrade decisions are temporary and well justified while pushing for upgrades on all client-server interactions.
Diagnosing and Debugging SSL Version Error in Python
An SSL version error suggests there is an issue with the installed OpenSSL libraries on your machine or the Python installation. To diagnose and debug the SSL version error in Python, you need to inspect:
1. **Your Python and OpenSSL versions**: The root of the problem could be a mismatch between them.
Python provides `ssl` module that allows you to handle encrypted connections created using OpenSSL library.
You can check your Python version by typing
xxxxxxxxxx
python -V
in your console. If you’re using different versions of Python, be sure to use the right command for the version (e.g., python2 or python3).
To check your OpenSSL version, try
xxxxxxxxxx
openssl version
.
If your Python version is outdated, consider updating it to its most recent version from the official Python website. The same goes for OpenSSL. Updates often correct these kinds of compatibility issues.
2. **Your SSL/TLS configuration status**: Misconfigurations in your SSL/TLS settings can lead to ssl errors in Python. Refer to relevant documentation to establish standards proposed by authorities like IETF (Internet Engineering Task Force) for guidance on setting up SSL/TLS connections.
3. **The certificates being used**: Ensure you’re using only valid and current certificates as expired or invalid certificates might trigger errors.
Finally, while experimenting with possible fixes, remember to take network and other security considerations into account to avoid new challenges down the line.
Let’s assume that our checks have confirmed that our Python and OpenSSL versions are compatible and configurations are perfect. But we’re still encountering SSL WRONG_VERSION_NUMBER error.
**Fixing ‘ssl.WRONG_VERSION_NUMBER’ Error**
Outputting full exception details will help understand what’s happening under the hood. This should be done by handling exceptions in your requests code block.
Here’s an example:
xxxxxxxxxx
import requests
from requests.exceptions import SSLError
try:
response = requests.get('https://example.com')
except SSLError as e:
print("SSL Error:", e)
If the issue persists, you might consider forcing client to use TLS instead of SSL or vice versa. While this may seem counterintuitive, the error message ‘WRONG_VERSION_NUMBER’ refers to a protocol version mismatch between the client and the server, not necessarily that one is more recent than the other.
You can achieve this by adding `ssl_version` flag in your code like this:
xxxxxxxxxx
import requests.packages.urllib3.util.ssl_
requests.packages.urllib3.util.ssl_.DEFAULT_CIPHERS += ':HIGH:!DH:!aNULL'
try:
requests.packages.urllib3.contrib.pyopenssl.DEFAULT_SSL_CIPHER_LIST += ':HIGH:!DH:!aNULL'
except AttributeError:
# no pyopenssl support used / needed / available
pass
This forces Python to make requests using the prescribed SSL/TLS version. Be sure to revert back all changes if they don’t improve the situation. Make careful decisions as incorrect flags may expose your application to security threats.
By following these steps, you should be able to accurately diagnose and resolve your SSL version error in Python.Sure, to fix the “SSL: wrong_version_number” problem when using Python’s requests library may sometimes involve going through a few key steps. I am going to cover these steps one by one, starting with checking the SSL/TLS version, proceeding through validating your certificates, and finally, configuring Python requests properly.
HTML:
xxxxxxxxxx
Checking the SSL/TLS Version
Start by checking whether the SSL/TLS version used by the client matches that of the server. Tools such as nmap can help determine what type of SSL/TLS version you are running:
$ nmap --script ssl-enum-ciphers -p 443 www.example.com
If you receive an error, it could be an indication that your client is attempting to use a deprecated or unsupported version of SSL/TLS. In this case, consider upgrading.
Validating Certificates
The next step would be certificate validation. If the server's certificate is expired or invalid, the connection will not be established correctly. This can be checked easily in the browser,
Go to site -> Click on lock symbol (address bar, usually left) -> View Certificates
If existing certificates are valid and still you're facing this issue, then acquire a new SSL certificate from authorized providers like Letsencrypt, GoDaddy etc.
Configuring Python Requests Properly
If you've confirmed your SSL/TLS version and certificates are correct, you may need to adjust your Python requests configuration.
In some cases Python’s Request library will throw “SSL: wrong_version_number” if it doesn’t have access to necessary libraries for creating a secure connection. To bypass this issue, you might use HTTP instead of HTTPS in your request (if it's acceptable regarding data sensitivity), or turn off verification using Python request like this:
requests.get('https://www.example.com', verify=False)
This will bypass SSL checks, but remember it's generally not recommended except for specific debugging situations because it makes connections less secure.
If none of the above steps works, remember there can be other problems causing this issue such as bad Server Name Indication (SNI) or an incompatible cipher suite between the client and server.
In order to solve it on the server side, strong ciphers should be enabled and weak ciphers should be disabled. You could refer to this guide for more information.
I hope this gives you a clear direction of how to approach a "SSL: wrong_version_number" error while working with Python. Remember, security should never be undermined.
Remember, each problem is unique, so it may require a unique solution. Always make sure to follow the appropriate regulatory guides and handle sensitive customer data with care.The
xxxxxxxxxx
ssl: WRONG_VERSION_NUMBER
error in Python request is a common issue which may occur if a hostname attempts to talk HTTPS over an HTTP connection or vice versa, creating a mismatch. There could be various causes for this anomaly, such as the Python environment running incompatible Secure Socket Layer (SSL) version, issues with network configurations, and more.
Several solutions can be implemented to resolve this issue:
Solution 1: Disable SSL Verification
A Recommended workaround could be disabling the SSL verification by using the
xxxxxxxxxx
verify=False
parameter of
xxxxxxxxxx
requests
. Here’s an example of how to do it:
xxxxxxxxxx
requests.get('https://your-url.com', verify=False)
Please note that disabling SSL verification may pose security risks and isn’t recommended for production environment.
Solution 2: Bundling Certificates
We might face SSL errors when requests cannot verify our hostname. One way around this is to bundle our certificates using the
xxxxxxxxxx
certifi
package. Example:
xxxxxxxxxx
import certifi
requests.get('https://your-url.com',
cert=certifi.where())
Here,
xxxxxxxxxx
certifi.where()
returns the path to your bundled certificates.
Solution 3: Manually Setting SSL Version
If the error stems from an SSL version mismatch, you can manually dictate the SSL version used by requests in your code using the
xxxxxxxxxx
ssl
library.
xxxxxxxxxx
import ssl
try:
_create_unverified_https_context = ssl._create_unverified_context
except AttributeError:
# Fallback to default SSL context
pass
else:
ssl._create_default_https_context = _create_unverified_https_context
This configuration allows your requests to bypass certification verification.
To find out which one works best for you, consider these angles before picking a solution for your feature.
1. **Project Scope:** You must understand your project requirements before going forward with any of solution. For instance, if your project calls for high-class security measures, then you shouldn’t opt for disabling SSL verification.
2. **Objective Judges Everything:** Every approach aims to accomplish its specific goal. If your goal aligns with safely installing packages without worrying about whether the connection is secure or not, perhaps manually dictating the SSL version would be best.
3. **Environment Formation:** Last but crucial! You have to visualize your development and production environment before choosing. If you are working on local development, disabling SSL could save time but for production, bundling the certificates may give you the desired safety.
In software development, rarely does a one-size-fits-all solution exist. Instead, we should always think critically and evaluate each option based on its merits and drawbacks, considering all relevant factors to make the best decision for your specific situation.
You can further read about these issues on Python Requests Documentation and Python PEP 476.
The “SSL: wrong_version_number” error often occurs when there is a discrepancy between the SSL or TLS protocols that the client and server support. It’s essentially about a version conflict between your system OpenSSL libraries and the Python packages you are trying to use.
Here are some strategies for preventing future “SSL:wrong_version_number” issues in Python `requests`:
– Upgrade Your Python Packages: Keeping your Python software stack updated is always a good idea. By upgrading to the latest versions, you stand the best chance of having support for the latest network protocols and security fixes.
You can update `requests` library itself using pip:
xxxxxxxxxx
pip install --upgrade requests
– Monitor The SSL/TLS Version Used: If the server you’re interacting with enforces a certain version of SSL or TLS, you should ensure your system supports it. For this, inspect the default versions of SSL/TLS that Python’s ‘requests’ library tries to establish.
– Set The SSL Context Manually: If you have control over how `requests` are made in your code, consider setting the SSL context manually. This way, you define exact connection parameters and bring under control the SSL/TLS versions.
Below is an example on how we can set parameters manually using `ssl` module in Python:
xxxxxxxxxx
import requests
import ssl
# create a new SSL context with custom settings
context = ssl.create_default_context()
context.options &= ~ssl.OP_NO_SSLv3
response = requests.get('https://example.com', verify=context)
– Try with Different Versions of OpenSSL: In some cases, changing the version of OpenSSL your Python is linked against might resolve the issue, especially if your Python was compiled against a particularly old OpenSSL build.
You can verify the OpenSSL version of your Python installation by:
xxxxxxxxxx
from platform import python_version
import ssl
print("Python version: ", python_version())
print("OpenSSL version:", ssl.OPENSSL_VERSION)
– Avoid Ignoring SSL Verification: Disabling SSL verification would negate any benefits you get from using HTTPS. It essentially makes the communication channel insecure, which leaves you exposed to potential ‘man-in-the-middle’ attacks and undermines data integrity.source.
Remember however that while all these suggestions help in preventing “SSL: wrong_version_number” errors, you may sometimes face a complex network scenario where finding the root cause would require deeper investigation from your side.From a coding expert’s perspective, “SSL: WRONG_VERSION_NUMBER on Python Request” is a common error encountered when using the
xxxxxxxxxx
requests
or
xxxxxxxxxx
urllib
library in Python to make HTTPS requests. This error typically occurs when the SSL/TLS version used by the server does not match that of your client (in this case, Python).
xxxxxxxxxx
import requests
response = requests.get('https://example.com')
This is your standard HTTP request using the
xxxxxxxxxx
requests
module in python. If you’re facing the ‘SSL: WRONG_VERSION_NUMBER’ error, chances are there’s a mismatch between the SSL/TLS versions used by the Python client and the remote server. You might also encounter this issue if the server expects an SSL handshake whereas Python’s
xxxxxxxxxx
requests
module doesn’t offer one.
So how do we deal with this?
– Confirm SSL/TLS version compatibility: Verify the server-side configuration settings related to SSL/TLS versions. If possible, compare them with the SSL/TLS versions supported by the Python library you are using for your HTTPS requests.
– Using
xxxxxxxxxx
pyOpenSSL
: PyOpenSSL is a higher-level, more Pythonic API built on top of OpenSSL. It exposes some of OpenSSL’s internal details and may provide a quick solution. However, it’s important to have a clear understanding of its usage to avoid introducing security vulnerabilities.
xxxxxxxxxx
from requests.packages.urllib3.contrib.pyopenssl import inject_into_urllib3
inject_into_urllib3()
– Disabling SSL verification: In cases where the other solutions can’t easily be implemented, or for testing purposes, disabling SSL verification completely can be another option, though it should always be last resort due to the significant security risks it involves.
xxxxxxxxxx
import requests
response = requests.get('https://example.com', verify=False)
Therefore, while the ‘SSL: WRONG_VERSION_NUMBER’ in Python requests can be irritating, it’s often an indication of SSL/TLS version mismatches, which can usually be remedied with the tips provided. Nonetheless, bear in mind too that troubleshooting such errors will also require looking at the bigger picture—not just your Python code but the server configurations as well.
For those interested, check out the official [Python docs](https://docs.python.org/3/library/ssl.html#) or the documentation for [Requests Library](http://docs.python-requests.org/en/master/) for a more comprehensive read-through.