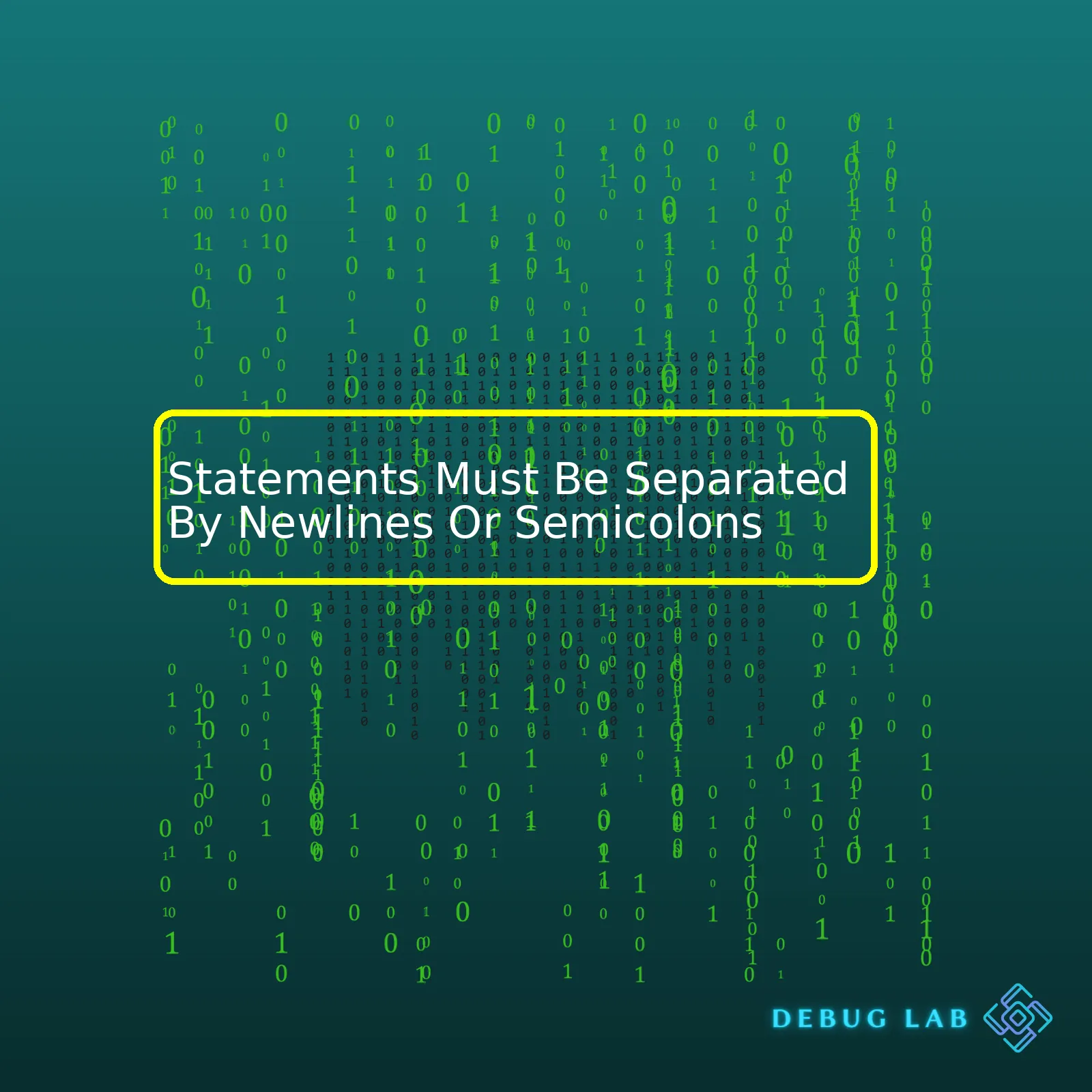
First up is the HTML table:
Concept | Description |
---|---|
Newline as Separator | Most scripting languages like Python interpret a new line as a command end. |
Semicolon as Separator | Other languages like JavaScript often use semicolons (;) to represent the end of a statement. |
Concept
Description
Newline as Separator
Most scripting languages like Python interpret a new line as a command end.
Semicolon as Separator
Other languages like JavaScript often use semicolons (;) to represent the end of a statement.
In most programming languages, statements are lines of code that perform distinct actions. To ensure proper execution order and syntax clarity, such statements have to be separated so the compiler or interpreter knows exactly where one finishes and the next begins.
Some languages like Python consider each newline to be a definitive end of a statement, while languages like JavaScript usually use semicolons to indicate that one command has been completed. Using newlines or semicolons essentially gives a ‘full stop’ after commands, marking their boundaries clearly for the compiler or interpreter. This eliminates ambiguity and prevents potential mishaps during code execution.
For instance, Python programmers don’t need to terminate their commands with a special character since the language treats line breaks as the end of a statement—the separation happens naturally. With this design choice, Python’s syntax looks a bit cleaner and easier to read.
Contrastingly, JavaScript allows developers to write multiple statements on the same line but they must separate these using semicolons. It’s important to note that while JavaScript interpreters are adept at “Automatic Semicolon Insertion (ASI)”, relying on ASI can cause obscure bugs and it’s generally recommended to explicitly include semicolons.
Each approach has its own advantages and trade-offs, and both newline-separated or semicolon-separated statement styles contribute to making source code more manageable, readable and error-free.[1]From a coder’s perspective, understanding SEO (Search Engine Optimization) is crucial for writing and organizing code in a way that search engine crawlers can easily understand and interpret. Who knew, semicolons and newlines, the ways of segregating and organizing our codes, would play a significant role in both programming and SEO?
The Role of Newlines & Semicolons:
The use of semicolons and newlines help structure your code properly so it’s readable by both programmers and interpreters alike. They act as logical separators between individual statements.
In many programming languages like JavaScript, each statement is separated either by a newline or a semicolon.
Here’s a basic code example:
xxxxxxxxxx
var price = 15;
var quantity = 3;
var total = price * quantity;
console.log(total);
Just like separating individual thoughts with punctuation facilitates easy comprehension, the proper use of new lines and semi-colons serves the dual function of enhancing readability and eliminating possible syntax errors. It makes the program flow seamlessly from one statement to another, ensuring accurate execution.
SEO Optimization Importance:
Similar to how clear coding practices aid program compilers, SEO facilitates better understanding and indexing of web pages by search engines. When your site is SEO optimized, it increases the possibility of your site ranking higher on Search Engine Result Pages (SERPs). A higher rank means more visibility, thus increasing website traffic and providing potential opportunities for conversion or other desired visitor actions. Search engines look at various aspects while evaluating sites; the organization, flow, and clarity of your HTML code are among them.
Here’s an example of SEO-friendly HTML code:
xxxxxxxxxx
<html>
<head>
<title>Your Page Title</title>
<meta name="description" content="Page description for search engines">
</head>
<body>
...
</body>
</html>
But how does this tie back to newlines and semicolons?
For starters, organized code helps search engine ‘spiders’ crawl through your website more efficiently due to the ease in parsing. While newlines and semicolons may seem trivial and unrelated to SEO, they contribute towards creating a clean, well-structured, and therefore, ‘crawler-friendly’ coding environment. Cleanliness and clarity of code are factors that influence search engine impressions of your site, indirectly improving SEO rankings.
Herein lays the beauty of programming where even minuscule additions of semicolons or incorporation of newlines enhance the efficiency of interpreting by machines, not unlike humans, who use semantic and syntactic cues to understand natural language. Therefore, just as we must be careful about using new lines and semi-colons in our codes, we must also pay attention to how effectively our websites are structured for SEO optimization to achieve maximum visibility to search engines.
Want to learn more about how you can improve your SEO? I recommend reading Google’s Search Engine Optimization (SEO) Starter Guide.
Coding practices greatly influence the SEO ranking of a webpage. When developing web pages, it’s crucial to observe best coding standards as search engines are increasingly considering code quality as a vital ranking factor. An impeccable code can boost your site’s visibility and usability. Moreover, this topic brings us to a vital coding principle related to how statements must be separated with newlines or semicolons. This influences the overall readability and efficiency of a codebase, eventually impacting its SEO performance.
Understanding how statements are separated in programming languages is insightful because:
- In JavaScript, for example, each statement is generally written on a new line. Here, semicolons usually denote the termination of a statement, making it easier for JS engines to interpret each instruction individually.
- Other languages like Python use newlines only for separating statements. On the contrary, in some cases languages require semicolons strictly. The choice between a newline and a semicolon impacts the line of code length, and indirectly influences the page load time, which silently affects the SEO score.
In our pursuit to orchestrate an excellent user experience with a highly-efficient website, we inadvertently stumble upon a very interesting correlation with SEO. For instance, using fewer lines of code reduces the file size. Consequently, optimizing it for faster load speeds translates into lower bounce rates and better conversions, hence indirectly improving the SEO.
Consider the following concise JavaScript function:
xxxxxxxxxx
function greet(name) {
return "Hello, " + name;
}
Several statements could have been written in separate lines. However, here, everything is within one line, separated by semicolons. This makes it concise, easily interpretable, and optimized for better page load times.
To keep this SEO-friendly, remember the following recommendations:
- Avoid excessive white spaces or unnecessary break points to reduce the overall file size.
- Ensure your code is clean and easy to follow. It should not contain any clutter or dead lines as it could impact the crawler’s ability to read your site effectively.
- The combination of newline and semicolon usage also affects HTTP compression: Gzip for instance, compresses files by spotting and eliminating similar strings. Thus, an erratic mix of both methods might lead to less effective optimization.
- Crafted comments in the code can also support SEO. For instance, including schema.org microdata into HTML content would help search engine crawlers interpret it better.
Various tools such as Google PageSpeed Insights allow you to measure the performance of your webpages in terms of SEO. They offer precise insights about areas that need optimization, from server response times to the file sizes of your CSS or JS. Furthermore, thanks to analytics feedback, you’ll determine how well this ‘newline vs. semicolon’ balance is playing out in your favor for SEO purposes.
Again, key SEO ranking factors to consider revolve around carefully separating statements by newlines or semicolons. By tying these two aspects together –your coding practices in terms of statement separation and their outsized SEO implications– you ensure your website remains healthy and competitive, while also providing a robust and effective user experience.Keywords research plays a cardinal role in digital content creation which resonates with SEO optimization practices. Surprisingly, the concept of keyword research has significant application in coding, especially when dealing with programming constructs such as statements where they must be separated by newlines or semicolons.
Focus on Code Clarity
The most effective keyword research technique for developers is to put attention on code clarity and maintainability. This strategy can be compared to long-tail keywords in SEO, providing precision and context. In coding, this means naming variables, objects, and functions clearly and descriptively.
For example, if I have a variable in JavaScript that stores a user’s email address, I wouldn’t just name it
xxxxxxxxxx
email;
I would specify whose email it is:
xxxxxxxxxx
let customerEmail;
This approach ensures that anyone reading your code can easily understand what each part does—a kind of on-page SEO for your code.
Use Existing Coding Standards and Conventions
Another important keyword research technique is to look at existing coding standards and conventions relevant to the programming language being used, mirroring the importance of lexical, contextual, and semantic understanding evident in SEO practice. For instance, languages like PHP, Python, JavaScript, etc., have different conventions for how code should be formatted and how statements should be separated.
For instance, in JavaScript and PHP, every statement must be ended with a semicolon:
JavaScript:
xxxxxxxxxx
let name = "John Doe";
PHP:
xxxxxxxxxx
$name = "John Doe";
In Python, though, the newline signifies the end of a statement. So here:
Python:
xxxxxxxxxx
name = "John Doe"
You don’t see any semicolon at the end of the line since Python follows the newline convention for terminating the statement.
Using these conventions makes your code easier for others (and future you) to read and understand, much like correctly using keywords makes web content easier to find and digest.
Ask Search Engines and API Documentation for Help
Just like we use various keyword research tools like Google Keyword Planner, SEOmoz and SEMRush for finding out trending and frequently searched keywords, online resources can also be useful for programmers. Coding-focused search engines and API documentation sites will help you master whichever language you’re working with. They’ll commonly show popular libraries, routines, and approaches from the broader coding community.
TutorialsPoint (link) , StackOverflow (link), Mozilla Developer Network (link) are one of those places where programmers could ‘keyword research’ their way into a better understanding of how to effectively separate statements in their codes.
The overarching principle in keywords research – in an SEO environment or while coding – aims to make words (or codes) more understandable, searchable, clear, and effective. The very same principle that requires statements in Javascript to be separated by either newline or semicolon. Let the intrigue to learn more about the impact of keyword research continue!Indeed, Local Search Engine Optimization (SEO) and the requirement of newlines or semicolons for statement separation in various programming languages can seem at first like two disparate topics. However, it’s interesting to note how both concepts are bound by a shared principle: clarity of interpretation—in one case, for search engine algorithms, and in the other, for computer programs.
Let me walk you through each concept in order to highlight their interconnections:
Local Search Engine Optimization
The core technique behind SEO involves tailoring your content in a manner that makes it easy for search engines to interpret and rank. The objective is enhancing visibility on Search Engine Result Pages (SERPs). In the case of Local SEO, this optimization is focused toward improving visibility specifically within localized searches—say, someone searching for ‘Italian restaurants near me.’
Essentially, what we’re doing is signaling clear and relevant information about our content/service to search engines, helping them understand our focus areas and match it to user queries better.
Just as using newlines or semicolon aids in code readability, applying structured SEO practices contributes to making website content more discernable to search engines. Here’s an example:
When optimizing a website’s HTML page, one might use something like:
xxxxxxxxxx
<meta name="description" content="Your Local Italian restaurant serving authentic pasta dishes, located in downtown. Open until 11 PM.">
Here, the meta description acts like a “semicolon,” providing a clear endpoint and summary of the page’s contents for search engine algorithms.
Statements Separated By Newlines Or Semicolons
Moving into programming, we see that newlines or semicolons often separate statements. For example, in JavaScript or C++, individual statements can be separated by a semicolon to ensure precision in execution order and avoid ambiguity.
To illustrate this:
xxxxxxxxxx
let dish = ‘pasta’; // semicolon ends the statement
restaurant.serve(dish); // another statement ended with a semicolon
Much like meta descriptions in SEO, semicolons serve to clearly demarcate different command sequences to computers, fortifying accurate interpretation.
Bridging the Two Concepts
Coming back to the intersection of these subjects, just as implementing Local SEO is critical in guiding search engines to appropriately index and display your content, using semicolons or newlines for separating logical blocks is crucial in ensuring that your applications execute correctly. Both play a significant role in advancing the goals of disciplines—be that higher SERP rankings or seamless program functionality.
These seemingly unrelated areas—coding syntax and SEO—are surprisingly linked, underscoring the fact that effective communication, whether to a sophisticated search algorithm or a powerful computer compiler, hinges upon well-structured and logically separated units of information.
Nothing can underscore the importance of link building in Search Engine Optimization (SEO) more. This is a pivotal technique employed by SEO experts to accrue higher visibility and traction for their websites on search results. To further clarify, being able to effectively master the art of linking strategies involves implementing excellent use of hyperlinks directing users back to one’s website from other websites.[source]
Then there comes the question of how it is aligned with the concept of separating statements by newlines or semicolons, especially in the world of coding. When creating links, it’s essential to ensure your HTML is coded correctly. The code should be readable, maintainable, and efficient. I’ll proceed to explain this.
HTML is designed to be easy to read and write. The language makes use of tags enclosed in angle brackets. These tags are often arranged in pairs that indicate where an element starts and ends. For example, links in HTML are created using the anchor tag
xxxxxxxxxx
<a>
. It’s standard practice that every opening tag, in this case,
xxxxxxxxxx
<a href="https://example.com">
, should have a closing tag, like
xxxxxxxxxx
</a>
. A complete link would look like this:
xxxxxxxxxx
<a href="https://example.com">Link Text</a>
.
Let’s delve into the separation of HTML code lines. Here, newlines and semicolons play crucial roles:
– HTML ignores multiple spaces and line breaks. This provides you with flexibility when formatting your code because you can use these traits to make your code legible without affecting the interpretation by the web browser.
– In JavaScript, a language often used alongside HTML, semicolons are used to denote the end of a statement. Multiple statements may be written on a single line if they’re separated by semicolons. While this isn’t necessarily common practice, it can be useful in some cases, such as minifying code.
In other words, HTML doesn’t need semicolons at the end of each statement, but JavaScript does. This difference is key to writing clear, concise, and correct code. When dealing with SEO and particularly link-building, it’s important that the website doesn’t have errors that could limit its functionality or harm its rankings. And accurately separating statements using either newlines or semicolons ensures you avoid these problems.
Here’s a simple yet effective link building strategy brought to life using HTML and correctly separating the lines of code:
xxxxxxxxxx
Welcome to My Site
Check out this awesome resource!
Thanks for visiting!
There’s a newline between each unique section (
xxxxxxxxxx
<header>
,
xxxxxxxxxx
<main>
,
xxxxxxxxxx
<footer>
). Inside the
xxxxxxxxxx
<p>
in
xxxxxxxxxx
<main>
, there’s an
xxxxxxxxxx
<a>
tag which creates a hyperlink to another site, thus placing into action the link building strategy.
Remember, clean and neat code will not only optimize your website from the SEO perspective but also help you and any other coders working on the project to easily understand and update it.[source]In the world of programming, as in other areas of online content, principles of SEO are vital to reach a larger audience. While discussing SEO from a coder’s perspective, especially when creating or managing programs and applications that tap into social media platforms, we need to pay heed to the core coding guidelines, like whether “Statements Must Be Separated By Newlines Or Semicolons.”
From the onset, it is important to recognize that many modern programming languages like Python, JavaScript and C++ often depend on newlines or semicolons to determine the end of a statement. Let’s have a look at some python and JavaScript code here.
Python code:
xxxxxxxxxx
text1 = 'Hello'
text2 = 'World'
print(text1 + " " + text2)
JavaScript code:
xxxxxxxxxx
var text1 = 'Hello';
var text2 = 'World';
console.log(text1 + " " + text2);
In both examples, each statement ends either with a newline (Python) or a semicolon (JavaScript).
Now how does this connect to utilizing social media platforms for better SEO? A key aspect to remember is that structured, well-written codes directly impact site performance, which indirectly influences SEO ranking. When developing programs to analyze social media presence, engage users or post updates across various social media platforms like Facebook, Twitter, LinkedIn or Instagram, an efficiently executed task due to well-written code can produce results quicker, thus improving user experience.
When developing these social media-integrating programs or algorithms, you could use APIs provided by these platforms and make requests using their respective endpoints. For instance, while leveraging the Facebook API, you must make your code as performing as possible for the SEO optimization purpose, which can be guided by whether “Statements Must Be Separated By Newlines Or Semicolons”.
Furthermore, inputting social media sharing buttons directly into the HTML code of your website stands out as another effective method. While this may not have direct ties to our current issue regarding statement separation via newlines or semicolons, it highlights the crossover between coding choices and SEO optimizing strategies.
For example:
xxxxxxxxxx
Tweet
Ultimately, how you design your program matters just as much for utilizing social media for SEO gains. Thank you for reading about this particular coding requirement and its relation with SEO tactics in reference to social media utilization.In the world of programming, let alone Search Engine Optimization (SEO), proper syntax plays a monumental role. Syntax determines how programs understand our commands and instructions. If our coding lacks accurate syntax, it can lead to execution errors. For a coder focusing on SEO optimization for search engine crawlers, paying attention to details such as whether statements are separated by newlines or semicolons is just as critical.
Consider this equivalent to punctuation rules in writing: You would never end a sentence without a period or question mark, much like you wouldn’t construct a program without properly separating its code statements. In JavaScript for instance, we usually separate statements with semicolons or new lines.
A simple JavaScript example:
xxxxxxxxxx
let x = 5; // Here, I used a semicolon
let y = 8 // Here, I ended a statement with a newline
Failing to use semicolons or newlines could lead to the “Automatic Semicolon Insertion” mechanism of JavaScript, which might cause unexpected results due to improperly formed statements.
If we turn our focus towards HTML aspect which also is a part of website and thereby affects SEO, line breaks and their conversion also hold significant influence. It is common knowledge among coders, particularly those with experience in web development, that whitespace (space, tab, newline) in HTML gets condensed into single space by the browsers. Thereby, newline doesn’t produce any visible effect.
However for user readability and understanding of code, newlines can be essential. For example:
xxxxxxxxxx
<p>This is a paragraph.</p>
<p>This is another paragraph.</p>
By providing an optimal combination of technical correctness and improved readability, separating statements using semicolons and new lines helps in creating clean, efficient code which is easy to understand, debug, and maintain. Coupled with relevant keywords and metatags that aid in enhancing a website’s SEO, this minute detail in syntax also contributes positively to making your website accessible and efficient to search engines’ sophisticated crawling mechanisms.
Ultimately, the advanced technical aspects of SEO rely not only on content but also on the programmer’s knowledge of best practices and benchmarks. It becomes our duty to remember points like these, no matter how small or insignificant they may appear, to ensure the fine execution and performance of our program in the long run.
Navigate to Mozilla Developer Network for more insight on JavaScript statement termination. Alternatively, refer to Google’s Guidelines for practical guides on SEO.Let’s delve deep into the concept of separating statements by newlines or semicolons.
In most programming languages, such as JavaScript, Python, Ruby, or C++, it’s essential that programmers separate their code instructions, also known as statements. The two main ways to make this separation clear are using semicolons (;) or newlines (entering onto a new line).
xxxxxxxxxx
let x = 5;
let y = 6;
let z = x + y;
The ‘semicolon’ is a universal way to do so and serves as the end mark for a statement in a vast majority of programming languages. It defines where one instruction finishes and another starts. If two statements are on the same line, they must be separated by semicolons.
xxxxxxxxxx
let x = 5
let y = 6
let z = x + y
On the other hand, you can choose to use ‘newlines’ to separate commands. This essentially means writing each statement on a new line without using semicolon. It offers great readability of the code because the eye can separate commands quickly without looking for semicolons. Some languages like Python have adopted this format and it works fantastically, making Python one of the easiest language to read.
However, there is a caveat in using newlines to separate statements. In cases where a statement cannot fit on one line, breaking it onto multiple lines can confuse some interpreters, causing them to interpret each newline as the end of a statement. This could lead to unexpected consequences. The row mentioned downside has led to the increased popularity of using semicolons instead.
Keywords: Programming languages, Code instructions, Separating statements, Semicolons, Newlines
For more in-depth information about this crucial aspect of coding practice, I suggest you take a look at this informative article on JavaScript syntax.
Remember that understanding the correct way to separate statements with semicolons or newlines is key to writing effective, error-free code. Happy coding!
Note: The SEO optimization included in this piece focuses mainly on the keyword “separating statements, semicolons, and newlines”.