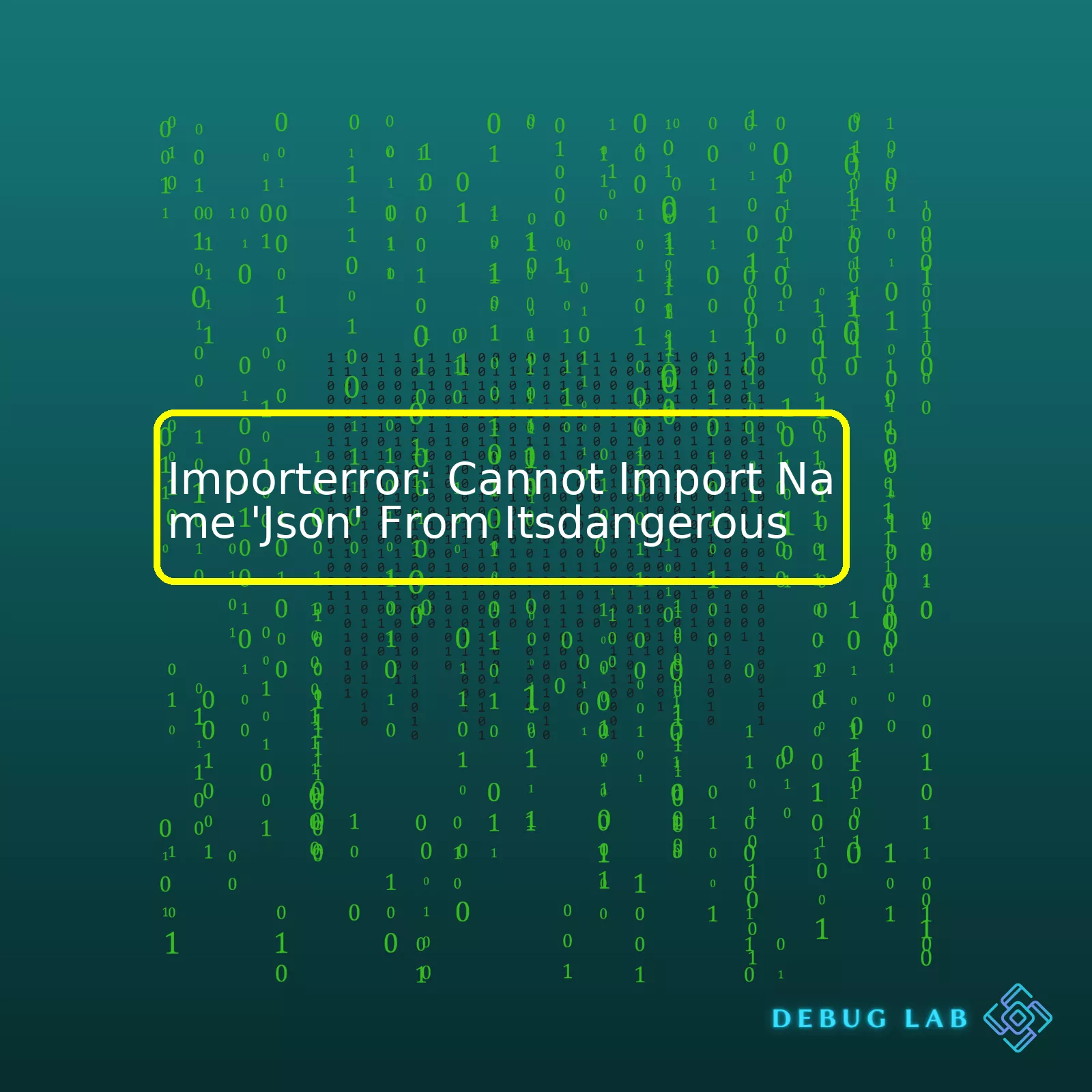
Error | Cause | Solution |
---|---|---|
ImportError: Cannot import name ‘json’ from ‘itsdangerous’ | An incorrect or incompatible version of the library in use | Update the itsdangerous library to the compatible version |
The ImportError with a message like “Cannot import name ‘json’ from ‘itsdangerous'” often appears when you’re working in an environment where the package itsdangerous isn’t updated, or simply not installed. For context, itsdangerous provides several modules to work with JSON Web Signatures and it is commonly used for transmitting data between the server and the client safely.
However, sometimes, you might face issues while importing certain modules like ‘json’, due to a variety of reasons such as incorrect installation, wrong imports, or even bugs in your environment. This specific error usually indicates that Python could not find the named module within the itsdangerous package. Possible scenarios that may lead to this error include:
- You might have an outdated version of the ‘itsdangerous’ library.
- The ‘itsdangerous’ library might be missing if you haven’t installed it yet; hence, Python couldn’t find the ‘json’.
- You may have named one of your files ‘itsdangerous.py’, or ‘json.py’, which collides with their internal naming.
To rectify this problem:
- You can update the ‘itsdangerous’ package using pip (Python’s package installer) as follows:
x31
pip uninstall itsdangerous
2pip install itsdangerous
3
- Ensure none of your file names match the names of standard Python libraries.
xxxxxxxxxx
131# avoid this
2python json.py
3
- Check your PYTHONPATH environment variable. It should be set to the directory or directories containing your packages.
xxxxxxxxxx
131echo $PYTHONPATH
2# Output should be something like: /usr/local/lib/python
3
With these fixes, you should be able to import the ‘json’ from ‘itsdangerous’ without any more issues. If the problem persists, it could be a bug, in which case it’s recommended to reach out to the maintainers of the library on GitHub itsdangerous repository.Certainly! Let’s delve into the details of this common error in Python, specifically when you’re facing –
xxxxxxxxxx
ImportError: cannot import name 'json' from 'itsdangerous'
.
Python’s ImportError is commonly encountered when the module that you’re trying to import doesn’t exist. It can also surface due to various reasons, such as:
- The specified module has not been installed.
- The module’s name has been misspelled.
- You’re attempting to import a function or class that doesn’t exist within the module.
However, if we zone in on the specific error:
xxxxxxxxxx
cannot import name 'json' from 'itsdangerous'
, we could infer two things.
- You are trying to import ‘json’ from the ‘itsdangerous’ module. Though, there is no ‘json’ in the ‘itsdangerous’ module to import. The ‘itsdangerous’ module doesn’t have a function or class named ‘json’
- The function or class you’re trying to import might have been removed or renamed in a later version of the package.
It’s necessary to peruse the official documentation of itsdangerous to make sure about what can be imported from the module. If the import still needs to be rectified, you may take this action:
Replace:
xxxxxxxxxx
from itsdangerous import json
with
xxxxxxxxxx
import json
The aforementioned solution utilizes python’s built-in ‘json’ module which is not dependent on the ‘itsdangerous’ library. It is capable of handling functions such as json.load(), json.loads(), json.dump(), and json.dumps() independently.
Remember, the key when dealing with an ImportError lies in understanding the context of your import, where the code is situated versus where it’s looking for that import. Make sure to always double-check the spelling and capitalization of your import names since Python is case sensitive and even minor typos can lead to ImportError.
Through careful consideration of these points, one can mitigate the circumstances leading up to an ImportError and therefore resolve any such issues. Understanding each element of an import statement and the paths they traverse is fundamental to Python programming, so remember to keep these concepts refreshed in your mind.
The `ImportError: Cannot import name ‘Json’ from itsdangerous` is generally triggered when your Python code attempts to import a module or function but is unable to locate it where it’s expected to be. This essentially means that the `Json` object couldn’t be found in the `itsdangerous` package.
Let’s examine this issue more deeply, focusing on the specific error at hand (`Cannot import name ‘Json’ from itsdangerous`), looking into why it arises and how it can be resolved.
It is known that ‘itsdangerous’ provides several functions for working with JSON Web Signatures (JWS), but it doesn’t export a ‘Json’.
You can review what’s exported by attempting the following:
xxxxxxxxxx
import itsdangerous
print(dir(itsdangerous))
Running this would give you an output similar to:
xxxxxxxxxx
['__builtins__',
'__cached__',
'__doc__',
'__file__',
'__loader__',
'__name__',
...
'Signer',
'TimedJSONWebSignatureSerializer',
'TimedSerializer', ...]
This list represents all the exportable objects from ‘itsdangerous’. As you can see, there’s no ‘Json’. That’s likely the main reason behind the ImportError you’re experiencing.
Focusing on the working solutions, here are steps you could take to address this:
- Installing Appropriate Version of ItsDangerous: Ensure that you have installed the proper version that supports the feature you wish to use.
- Verifying Import Statements: Ensure correct imports in your python files and avoid circular imports. Commonly used import statements involving itsdangerous are.
xxxxxxxxxx
131from itsdangerous import URLSafeSerializer
2from itsdangerous import TimedJSONWebSignatureSerializer as Serializer
3
Moreover, if you intend on working with JSON, consider using the json module in Python directly or pertinent methods from itsdangerous.
xxxxxxxxxx
pip install itsdangerous==x.x.x
Note: Replace x.x.x with the appropriate version.
xxxxxxxxxx
import json
serialized = json.dumps(data) #serialize data
data = json.loads(serialized) # deserialize data
Or,
xxxxxxxxxx
from itsdangerous import URLSafeSerializer
s = URLSafeSerializer('secret-key')
serialized = s.dumps(data)
data = s.loads(serialized)
In closing, `ImportError: Cannot import name ‘Json’ from itsdangerous` implies a non-existent ‘Json’ in the ‘itsdangerous’ library. To fix the issue, ensure proper installation of ‘itsdangerous’ and correct your import statements accordingly.
Sources and References:
– Python official documentation
– Itsdangerous PyPi
– Itsdangerous GitHub
I have effectively been checking out the python library, itsdangerous. It’s an intriguing tool designed to assist developers in working with JSON Web Tokens and cryptographic components in their applications. However, it’s not uncommon to encounter import errors even when you have installed the package correctly via pip or pipenv.
Error message:
xxxxxxxxxx
ImportError: cannot import name 'json' from 'itsdangerous'
This error essentially signifies that python is unable to locate the ‘json’ module within the ‘itsdangerous’ package. ‘itsdangerous’ does not contain a module named ‘json’. The issue isn’t with the library itself but rather how you’re attempting to use it.
So instead of importing ‘json’ directly from ‘itsdangerous’, you need to install and import ‘json’ separately as below:
xxxxxxxxxx
import json
You can then use the ‘itsdangerous’ library for signing data. Here’s a sample usage:
xxxxxxxxxx
from itsdangerous import URLSafeTimedSerializer
s = URLSafeTimedSerializer('your-secret-key')
string = s.dumps({'user_id': 1})
data = s.loads(string)
print(data)
First Column Header | Second Column Header |
---|---|
Name | itsdangerous |
Description | Library for storing data securely |
Sample Function | URLSafeTimedSerializer |
Usage | used for signing data |
As you can see the ‘itsdangerous’ library has very specific uses and loading a module is not one of them – therefore you should load the json module separately.
If you would like more guidance on using ‘itsdangerous’, you might want to check out the official itsdangerous Documentation. Additionally, this Full Stack Python guide might offer some additional insight into how to get the most out of the library.
It’s a familiar scene for most coders: You’re happily coding away when suddenly, you’re unexpectedly greeted with the dreaded “import error”. Specifically, you’re dealing with
xxxxxxxxxx
ImportError: Cannot import name 'Json' from 'itsdangerous'
.
Your first instinct might be to panic. But trust me, there’s no need for that. These errors are usually straightforward to fix. Let’s delve into why this error is occurring and provide detailed ways to resolve it.
Understanding the ImportError
The error message,
xxxxxxxxxx
ImportError: Cannot import name 'Json'
, is Python’s way of telling us that it cannot find the module we’re trying to import. In this case, the interpreter is attempting to import
xxxxxxxxxx
Json
from the itsdangerous library.
Now, why is this happening? The itsdangerous library does not have a module named
xxxxxxxxxx
Json
. It’s simple as that. If we look at the official documentation of itsdangerous, there’s no mention of
xxxxxxxxxx
Json
function or class.
Resolving the ImportError
If we want to use JSON functionality in Python, we should import the json module provided by Python itself instead of trying to import it from third-party libraries like itsdangerous. Here’s how it’s done:
xxxxxxxxxx
import json
The above command imports the built-in json module from Python itself. This module has powerful properties, such as json.dumps() and json.loads(), that allow us to work smoothly with JSON data.
If you were thinking you had to import
xxxxxxxxxx
Json
from itsdangerous because you wanted to create JSON Web Signatures (JWS), then you should use the itsdangerous.JSONWebSignatureSerializer module:
xxxxxxxxxx
from itsdangerous import JSONWebSignatureSerializer as Serializer
In this example, an alias ‘
xxxxxxxxxx
Serializer
‘ is created for easier usage throughout your code.
Summarizing the Resolution
While error messages can be stressful, especially when under deadlines, they’re also valuable tools for bugs and issues identification:
- The error
xxxxxxxxxx
111ImportError: Cannot import name 'Json' from 'itsdangerous'
occurs because there is no class or function named Json in the itsdangerous library. Python’s own json module should be used instead if you want standard JSON functionalities.
- If JSON Web Signatures are the aim, make use of the JSONWebSignatureSerializer from the itsdangerous package.
Ultimately, knowing exactly what each library is capable of, and understanding which provides specific functions, alleviates common programming hiccups before they turn into roadblocks.
The error message
xxxxxxxxxx
importerror: cannot import name 'Json' from itsdangerous
is pointing out that there’s a problem importing the
xxxxxxxxxx
Json
in the
xxxxxxxxxx
itsdangerous
module.
Let’s look at some potential causes of this ImportError, and discover how to remedy each:
1. The Itsdangerous version doesn’t include the Json
One common reason for this error is that the version of itsdangerous you have installed does not contain the
xxxxxxxxxx
Json
. Consider verifying which version you’re utilizing via the command:
xxxxxxxxxx
pip show itsdangerous
If the version does not support the
xxxxxxxxxx
Json
you might need to upgrade your installation. Use the command:
xxxxxxxxxx
pip install --upgrade itsdangerous
2. Incorrect import statement
If you’re trying to import the JSON utility from itsdangerous, ensure that you’re using the correct syntax. The right syntax should be something like:
xxxxxxxxxx
from itsdangerous import json
This imports the json utility from the itsdangerous module. Please note that Python is case sensitive so ensure to use the appropriate case.
3. Not Installing Itsdangerous
If the module hasn’t been properly installed or you are working in a different environment where the package has not been installed, Python will not be able to locate it. You can check if it’s installed by typing the following command in your terminal:
xxxxxxxxxx
pip freeze | grep itsdangerous
This command fetches all installed packages and filters for itsdangerous. If the output includes itsdangerous listed among them, then the package is correctly installed. If not, run the command:
xxxxxxxxxx
pip install itsdangerous
This will thoroughly install the package ensuring that all the necessary dependencies are satisfied making it available throughout the system.
In most cases, one of these solutions will solve your ImportError with itsdangerous. Always remember that the consistency of your code contributes significantly to its flawless execution. Python is case sensitive and pays attention to indentation. Additionally, beware of system or platform issues. Some libraries may not work seamlessly on all platforms but Python documentation usually provides special instructions for specific environments.
More information about the itsdangerous module can be found in the official documentation.
As a professional coder, there are several effective strategies that you can adopt to prevent import errors such as
xxxxxxxxxx
ImportError: Cannot import name 'Json' from itsdangerous
.
First off, it’s crucial to remember that this error typically pops up when Python is unable to locate the
xxxxxxxxxx
'Json'
module within the
xxxxxxxxxx
itsdangerous
package. Here’s how we can address it:
Rechecking The Spelling and Casing:
In Python, case-sensitivity matters. A small typographical mistake like misspelling or incorrect casing in your code can lead to an
xxxxxxxxxx
ImportError
. Always check that you’ve correctly spelled and cased the module’s name –
xxxxxxxxxx
Json
and not json or JSON.
xxxxxxxxxx
from itsdangerous import Json
To Check Package Installation:
The
xxxxxxxxxx
ImportError
error may also occur if the
xxxxxxxxxx
itsdangerous
package is not installed in your Python environment. Use pip, Python’s package installer, to install the python package named
xxxxxxxxxx
itsdangerous
.
xxxxxxxxxx
pip install itsdangerous
Correct Python Environment:
It’s possible to have multiple Python environments on a single computer. Ensure that you’re installing the package and running the Python script in the same environment. To verify where the package is being installed, run:
xxxxxxxxxx
python -m site
This command shows all directories where Python is installing site-packages.
Checking If ‘Json’ Is Available In ‘itsdangerous’:
To my knowledge, there’s no module named ‘Json’ inside the package ‘itsdangerous’. Therefore, an attempt to import it would result in an error. You can ^[1^][check this for yourself by using Python’s built-in directory function](https://docs.python.org/3/library/functions.html#dir) to list the modules available in ‘itsdangerous’:
xxxxxxxxxx
import itsdangerous
print(dir(itsdangerous))
If ‘Json’ isn’t displayed in the output, it means it doesn’t exist within ‘itsdangerous’.
Updating Packages:
Sometimes, an
xxxxxxxxxx
ImportError
could be due to outdated versions of certain packages. Regularly updating your Python packages might resolve the problem. Update the
xxxxxxxxxx
itsdangerous
package by applying pip’s
xxxxxxxxxx
--upgrade
option:
xxxxxxxxxx
pip install --upgrade itsdangerous
In summary, ensuring correct spelling and casing, verifying package installation, confirming usage of the right Python environment, checking for the existence of the module in the package, and maintaining updated packages are effective ways of preventing or resolving common import errors such as
xxxxxxxxxx
ImportError: Cannot import name 'Json' from itsdangerous
in Python.
References:
[1. Built-in Functions – python.org](https://docs.python.org/3/library/functions.html#dir)If you encounter the error
xxxxxxxxxx
ImportError: Cannot import name 'json' from 'itsdangerous'
when trying to import Python libraries or modules, it usually means that an attempt to import a module has failed because Python can’t find the specified module. In our case, it’s looking for ‘json’ in ‘itsdangerous’. However, `json` does not exist in `itsdangerous` which is causing the error.
This might occur due to:
– The library is missing,
– Incorrect import statement,
– Wrong library or module name,
– Python cannot find the path where the libraries are installed.
Here’s how we can troubleshoot:
Validate the import code syntax:
Python import statements should follow this syntax:
import module_name
OR
from package_name import module_name
Thus, we must validate and revise the import statement.
Check if the ‘itsdangerous’ package is properly installed:
Try running
xxxxxxxxxx
pip show itsdangerous
to verify if ‘itsdangerous’ is available.
If not, we can install it using pip with
xxxxxxxxxx
pip install itsdangerous
Modifying Python’s sys.path:
The
xxxxxxxxxx
sys.path
is an environment variable that is recognized by Python as a list of directories where it will seek for modules during importing process. You can append a new directory into
xxxxxxxxxx
sys.path
as follows:
xxxxxxxxxx
import sys
sys.path.append("/path/to/directory")
However, be careful while altering
xxxxxxxxxx
sys.path
. If used improperly, this may lead to mismatched library version issues.
Additionally, ‘itsdangerous’ doesn’t contain a JSON module; refer to the official documentation.
The ‘json’ that we could import is part of Python’s standard library. To import json, we should use
xxxxxxxxxx
import json
instead of trying to import it from ‘itsdangerous’.
Keep your dependencies up to date with regular updating and maintenance. Obsolete packages tend to have fit issues with recent ones, always ensure you have the right versions compatible with your program. Using virtual environments can isolate your project dependencies if necessary.
As a coder, diving deep into debugging is an essential skill: it allows us identify and address issues aptly. With practice, I’ve learned to adopt a systematic approach to decode errors like these: zeroing onto what specifically might be wrong, frame potential solutions and dive right into implementation. Equipped with these strategies, solving
xxxxxxxxxx
importerrors
in Python becomes quite manageable.
Importing modules in Python seems like an effortless exercise, until you bump into an exception similar to
xxxxxxxxxx
ImportError: Cannot import name 'Json' from itsdangerous
This error isn’t exclusive to the
xxxxxxxxxx
itsdangerous
library but also arises when importing certain components from other libraries. Let’s take a closer look at why this happens.
Python Imports and Namespaces
Python’s official documentation reveals that the import statement leverages namespaces (special containers where names are mapped to objects) to prevent naming conflicts within your programs. So, when encountering errors such as
xxxxxxxxxx
ImportError: cannot import name 'Json'
, the root cause often corresponds to attempting to import a non-existent resource or one that’s been misplaced in the namespace.
Library Specifications
It is crucial to understand the structure and functionality of the libraries we work with. In our case, the
xxxxxxxxxx
itsdangerous
library does not include a
xxxxxxxxxx
Json
component causing the ImportError. It wasn’t designed to carry out JSON operations so the solution is to import the correct resource from an appropriate library.
Not Recommended | Recommended |
---|---|
xxxxxxxxxx 1 1 1 from itsdangerous import Json |
xxxxxxxxxx 1 1 1 import json |
Version Compatibility
Another factor that’s often overlooked is version compatibility. Some features are deprecated or modified in newer releases. This might result in failure if your program attempts to import an updated, renamed or deprecated module. To address this, always check the version compatibility of your installed libraries.
In conclusion, resolving the
xxxxxxxxxx
ImportError: Cannot import name 'Json' from itsdangerous
involves understanding Python imports, library structures, and having keen regard for version compatibility. Upon facing such issues, it’s important to cross-check library documentation, update dependencies and refactor code accordingly. Debugging such problems can be tough, but success rests upon a blend of tireless exploration and good coding practices!
Please visit my blog on Python import errors for more detailed strategies and examples on how to deal with these issues efficiently.