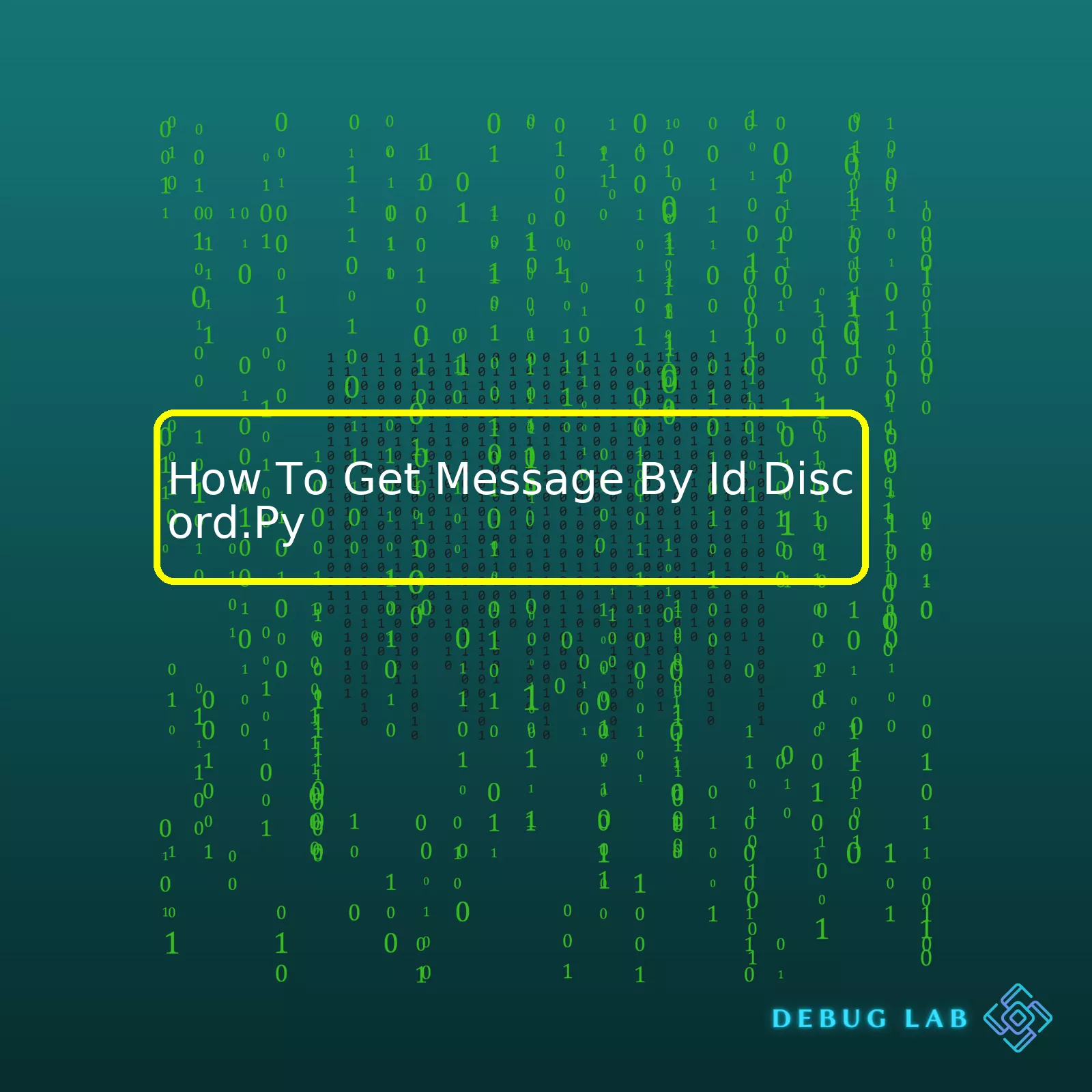
fetch_message()
. The script should look as follows:
xxxxxxxxxx
<?python
# Assuming you're inside an event
channel = client.get_channel(12324234183172)
message = await channel.fetch_message(123456789012345678)
print(message.content)
?
>
In this python snippet, we’re first obtaining the desired channel with the unique ID “12324234183172.” Subsequently, we are then utilizing the
xxxxxxxxxx
fetch_message()
function to extract the specified message using its unique ID “123456789012345678”. Lastly, the
xxxxxxxxxx
print()
function is partially responsible for outputting the content of the message.
To effectively make sense of this in tabular format, let’s define the information as follows:
Function | Description |
---|---|
xxxxxxxxxx 1 1 1 get_channel |
This method retrieves the designated channel using a distinct ID. |
xxxxxxxxxx 1 1 1 fetch_message |
This asynchronous function provides a way to fetch the specific message using its unique ID. Discord retains messages little by little therefore, you are able retrieve past messages even if they aren’t cached. |
xxxxxxxxxx 1 1 1 print() |
The standard print function is utilized here to output or showcase the contents of the fetched message. |
In summary, fetching a message by ID in discord.py requires the use of the method
xxxxxxxxxx
fetch_message()
. This particular function operates asynchronously such that you can reach back into the history of a channel to find past messages, even ones that may have disappeared from the cache. Combined with the
xxxxxxxxxx
get_channel()
function to select the necessary environment and the standard
xxxxxxxxxx
print()
function to display retrieved data, these tools compile a toolkit of essential functions needed in interacting with the Discord API via Python.
For more detailed exploration of discord.py functionalities, check out the official manual available at the Discord.Py Documentation.
Getting a message by its ID is an integral part of working with the Discord API and Discord.py, a modern, easy-to-use, feature-rich, and async-ready API wrapper for Discord written in Python. If you already know how to use Discord.py, getting a message by its ID will be a walk in the park.
However, suppose you’re not familiar with Discord.py. In that case, it essentially serves as a way to interact with Discord’s APIs without having to worry about the nitty gritty details. It abstracts most of the complex things such as handling sharding and JSON payloads, allowing us developers to concentrate on creating the application features.
Knowing how to pull messages via their IDs can be especially useful when you’d like to manage individual comments or enable interactive reactions based on specific user inputs.
To get a message by its ID using discord.py library, you need to utilize fetch_message() method which is available under certain context objects. However, it’s important to note that in order to use
xxxxxxxxxx
fetch_message()
, the bot must have Read Message History permission. Here’s a simple code example:
xxxxxxxxxx
# Importing the Discord module
import discord
# Create an instance of a client object
client = discord.Client()
event .
async def on_ready():
channel = client.get_channel(12345) # Insert your desired channel ID here
message = await channel.fetch_message(67890) # Insert your desired message ID here
print(f'Message - {message.content}')
client.run('token') # Insert your bot token here
Here are the key parts in this simple script:
* The
xxxxxxxxxx
get_channel()
method gets the specified channel where we intend to retrieve a message.
* The
xxxxxxxxxx
fetch_message()
then retrieves the actual message via its ID.
* Finally, I just asked Python to print out the content of the message to make sure everything works as intended.
Once you run this script, it’ll print the content of the message with the specified ID in the console.
It’s possible that discord.py has no mechanism to verify whether the provided message ID exists within the given channel, so if there’s no record with that ID, it could fail silently or throw an exception – these are important edge cases to keep in mind when implementing this function.
Keep yourself updated with the latest Discord.py documentation, as new changes and improvements take place quite frequently. Happy coding!
Sources:
1. Discord.py TextChannel.fetch_message Documentation
2. Discord.py How To Get A Message By Its ID StackOverflow Discussion.
In
xxxxxxxxxx
discord.py
, an open-source Python library allowing interaction with the Discord API, retrieving messages by ID is a common operation. This can be part of monitoring content, programming reactions to specific messages, or mining data for research purposes.
To tackle this task, we require knowledge about fetching messages generally and targeting specific messages using IDs. Here’s how it works:
Finding Message by ID With fetch_message()
xxxxxxxxxx
fetch_message() is used in conjunction with the targeted channel and the message ID. The general syntax is:
message = await channel.fetch_message(id)
Example usage might appear as follows:
channel = client.get_channel(CHANNEL_ID)
message = await channel.fetch_message(MESSAGE_ID)
print(message.content)
By replacing
CHANNEL_ID
and
MESSAGE_ID
with actual values, you'd get the desired message out. Notice the use of
await
: discord.py 1.x.x makes heavy use of Python's asyncio library for asynchronous operations, meaning many commands are coroutine functions that need suspension before continuation.
Error Handling
You might want to include error handling for when an invalid message ID is inputted. Discord.py raises
HTTPException
when the GET request fails (as in the case of an incorrect ID), and
NotFound
if the message doesn't exist or isn't visible to the bot. To handle these incidents, include a try block:
try:
message = await channel.fetch_message(MESSAGE_ID)
except discord.NotFound:
print('Message does not exist.')
except discord.HTTPException:
print('Invalid ID')
Adding these checks ensures your program doesn't crash on encountering a non-existent message.
Method
Description
fetch_message()
Fetches the message with the provided ID from a TextChannel.
get_channel()
Returns a channel with the specified ID.
print()
Prints the message content to your console
Of course, observe standard Discord bot practices like respecting user privacy and adhering to Discord's Terms of Service - avoid violating any rate limits to prevent your bot from getting banned.
Remember that the ability to get messages by ID opens up a world of possibilities for interaction with your Discord community. Use it creatively!
To read more on the topic, here's a helpful link to the discord.py documentation. Remember: the more familiar you become with the docs, the easier it'll be to navigate the complexities of coding a Discord bot.The 'Get Message By ID' feature is one of the essential functionalities in any messaging system, Discord being no exception. In the context of discord.py, this feature allows you to retrieve a specific message using its unique ID.
To use this, it’s crucial to understand that each message sent on Discord has an associated unique ID, a string of numbers that identifies it within the system—analogous to how each person has a unique social security number.
In discord.py, fetching a message would look like this:
channel = client.get_channel(1234567) # replace with actual channel ID
message = await channel.fetch_message(7654321) # replace with actual message ID
print(message.content)
In the code above, we first retrieve the Channel object via the client.get_channel() method, which takes as argument the intended channel's ID. We then call fetch_message() on the Channel object, passing the desired message ID. Finally, we print the content of the retrieved message.
This seems straightforward; however, a couple of considerations are worthwhile:
- To fetch a message by ID using discord.py, your bot needs the ‘Read Message History’ permission in the server or channel, from where the message should be fetched.
- The fetch_message method triggers an API call. Extreme caution should be taken to not spam too many requests within a short time-frame to mitigate the risk of being rate-limited by the Discord API.
Using 'Get Message By ID' isn't simply about retrieving messages; it's also about managing content effectively and understanding specific communication contexts accurately.
Remember always to comply with Discord's terms of service when writing bots and scraping data, especially regarding privacy and user data handling. It's worth noting that relying heavily on message IDs for important functionality can be risky due to potential message deletion or unavailability due to various reasons.
To sum it up, the 'Get Message By ID' function is an essential feature bringing great utility in creating effective interactions in discord.py, given careful consideration to the discussed factors.When dealing with the Discord.Py API, you might often find yourself needing to fetch a message by its ID. Fetching a message by its ID is especially useful when moderating chats or identifying the source of certain messages.
To get a message by its ID in Discord.Py, the method
fetch_message()
is used. However, this operation may sometimes run into errors and exceptions that need to be properly handled. One common exception is the NotFound error, which gets thrown when a message with the specified ID does not exist. It should also be noted that only the messages sent after the bot started can be fetched directly using the ID.
Here's an example of how to handle such exceptions:
"""
Remember, 'bot' is your instance of commands.Bot or bot.Bot
'channel_id' is an int containing the channel id
'message_id' is an int containing the message id
"""
async def fetch_message_by_id(bot, channel_id, message_id):
try:
channel = bot.get_channel(channel_id) # retrieves the channel
message = await channel.fetch_message(message_id) # attempts to retrieve the message
except discord.NotFound: # handles the exception if the message does not exist
return "Message not found."
except discord.Forbidden: # handles the exception if the bot doesn't have proper permissions
return "Bot does not have permissions to access the message."
else: # runs if no exceptions were raised
return message.content # returns the message content
In this code snippet above, the
fetch_message_by_id()
function aims to fetch a particular message from a specified channel with given IDs.
* The
get_channel(channel_id)
function of the 'bot' object retrieves the desired channel, and the
fetch_message(message_id)
function of the 'channel' object retrieves the message.
* It is crucial here to handle any foreseeable exceptions to prevent application breakdowns. With the inclusion of the
discord.NotFound
exception, the program ensures there is proper handling when the message ID doesn't exist in the channel. Additionally, the
discord.Forbidden
exception manages cases where the bot lacks necessary permissions to read the message or channel history.
These are just some ways to minimize surprise errors during your interaction with Discord.Py. Exception handling is paramount for delivering a fluid user experience. Furthermore, it brings about robustness in your applications.
For more detailed information, consider visiting the official Discord.Py Documentation.Fetching messages by ID in Discord.py allows for efficient and more targeted conversation analysis, moderation activities, or bot message responses within a Discord server.
Table of Contents
Toggle
Fetching Messages Using Discord.pyAdvanced Techniques In Fetching Messages
Fetching Messages Using Discord.py
When working with Discord.py, you will often need to fetch messages by their ID. Messages in Discord are assigned a unique identifier that can be used to retrieve them later. Let's start with the basic understanding of how we can get a message by its ID using discord.py.
# Assuming you've already have your bot/client ready
async def get_message_by_id(channel_id: int, message_id: int):
channel = client.get_channel(channel_id)
if not channel:
raise Exception(f'Channel {channel_id} does not exist.')
message = await channel.fetch_message(message_id)
if not message:
raise Exception(f'Message {message_id} does not exist.')
return message
This function above will get a specified message given the channel id and message id. However, there might be times when you want to use more advanced techniques where a simple fetch won't do the trick.
Advanced Techniques In Fetching Messages
Let's dive deeper into some advanced retrieval methods:
Bulk Message Fetch:
You may need to bulk-fetch messages and parse each one for the desired message ID if the specific message is very far back in the channel's history. Here's how you can do this:
async def bulk_fetch_message(channel_id: int, message_id: int):
channel = client.get_channel(channel_id)
if not channel:
raise Exception(f'Channel {channel_id} does not exist.')
async for message in channel.history(limit=100):
if message.id == message_id:
return message
raise Exception(f"Message {message_id} not found in last 100 messages.")
In this `bulk_fetch_message` function, you're retrieving the last 100 messages from the specified channel and iterating through them to find the message with the desired ID.
Note: The
history()
method can be memory-intensive on large channels, so make sure you only use it when necessary and limit the number of messages you fetch. Also, you would likely want to handle exceptions more gracefully, but that will be application-specific.
Fetching From Different Channels:
In certain scenarios, you might need to fetch a message ID across multiple channels. This can be done by looping through all available channels and trying to retrieve the message:
async def fetch_from_different_channels(message_id: int):
for guild in client.guilds:
for channel in guild.text_channels:
try:
message = await channel.fetch_message(message_id)
if message:
return message
except:
continue
return None
In this `fetch_from_different_channels` function, we're looping over every text channel in every server(guild) that the bot is a part of and attempting to retrieve the message.
Please Note: If you're trying to search for a message across all channels, this can lead to many requests being made to Discord's API which could possibly get your bot temporarily rate limited.
Each of these advanced techniques offers different advantages adapted to various use cases - from fetching bulk messages, to searching across multiple channels. Use these strategies carefully, considering the benefits and potentially high resource usage.
Remember, all the messages sent in a server are recorded in the server logs; therefore, admins can always view complete logs for thorough audit or moderation purposes - making refined message retrieval an essential skill when building bots with Discord.py.Getting a message by its ID using discord.py can be a daunting process if you're not familiar with the Discord API or Python's async functionality. The message ID is a unique identifier given to every message whenever it's created. It's what allows us to target and interact with specific messages.
First, let me outline my understanding of how you should go about it.
- Configure your bot settings on Discord and fetch necessary details
- Install discord.py library
- Understand discord.py events
- Fetch the message via ID
Configuring your bot settings on Discord:
Before making use of Discord's API, one has to create and configure their application on Discord's Developer Portal. Once created, you can retrieve the BOT token which will allow your BOT to interact with the Discord servers. Do remember to give your BOT appropriate permissions.
Installing discord.py:
To install discord.py you can simply use pip:
pip install discord.py
Understanding discord.py events:
Events in discord.py are driven by asynchronous programming. Due to this, we're able to handle multiple activities at once, like getting messages, handling approvals, etc., all in real time. Executing synchronous operations would cause bottlenecks, which are prevented through async functionality.
One such key event that discords.py provides is
on_message
. This event triggers whenever a new message is received from any server your BOT is active on.
Fetching the message via ID:
Once we understand these points, we can proceed to fetch messages by their IDs. To accomplish this, Python's 'await' function can be used inside an async block until the operation returns a message object. Considering that retrieving individual messages could demand resources, there might be rate limits. Hence, use it efficiently.
Here's a code snippet demonstrating this process:
import discord
client = discord.Client()
event .
async def on_ready():
general_channel = client.get_channel(id)
msg = await general_channel.fetch_message(message_id)
print(msg.content)
client.run('insert-bot-token-here')
In this example, `id` is the channel id where your desired message resides, and `message_id` is the id of the message you want to get.
This is the basic implementation of getting a message by its id in discord.py. You might need to make adjustments based on your application (e.g., the bots permissions on the specified channel).
Remember, when working with Discord's API, it's important to be mindful of the rate limits set forth by Discord. They're implemented to ensure fair usage of the API for all developers (Discord API Rate Limits). So instead of frequently fetching a single message, consider setting up a cache of fetched messages to save resources.
In addition, when working with discord.py, be sure to keep yourself updated with changes. As of late 2022, discord.py has announced discontinuation so you may have to switch to alternative libraries (Official GitHub Issue stating the discontinuation).
The above should provide you with enough knowledge about getting a message using its id with discord.py while also scoping into best practices with Discord's API and discord.py effectively.Mastering security measures is crucial to any task involved in programming, including retrieving messages by ID through Discord.Py.
When working with Discord, it's necessary to have a good understanding of python and the discord.py API wrapper. Here's how you can retrieve message by id in Discord.Py:
event .
async def on_message(message):
if message.content.startswith('!get'):
channel = message.channel
msg_id = int(message.content.split(" ")[1])
my_msg = await channel.fetch_message(msg_id)
print(my_msg.content)
In the above script, we're creating an event `on_message` that gets triggered each time a message is sent in a channel where the bot has access. The bot will check if the message starts with '!get'. If the above condition is true, we split the input, cast the second argument (the message id) to integer, fetch the message with this specific id in the current channel, and print its content.
Security Measures
While security measures can often seem auxiliary to primary development processes like retrieval of messages, they are vital components to secure and robust applications, especially when dealing with sensitive information such as user data.
Discord security can be managed at several different levels: authenticating requests, managing permissions in servers and channels, using modern HTTPS to provide secure internet connections, and ensuring best coding practices to prevent dangerous errors or vulnerabilities like cross-site scripting (XSS), Injection attacks or Denial-of-service (DoS) attacks.
Here are some things to consider:
• Token Security - Your bot token is essentially your bot’s password. You should never reveal your bot token to anyone else. Never push code containing your bot's token into a public repository on Github, nor offer it to services without prior knowledge about them. It provides full access to your bot and may result in malicious uses if found by others.
TOKEN = 'your_token'
client.run(TOKEN)
• Rate limiting And Best Practices - Be observant of the rate limits. Aggressively making API requests one after the other can result in penalties or even a blacklist for your application. Always implement logic to deal with [API-specified rate limits](https://discordpy.readthedocs.io/en/latest/api.html#discord.Intents). It's also recommended to use the bulk versions of certain actions whenever possible, like `ctx.channel.purge()`, instead of deleting messages one by one.
• Error handling - Try-except blocks or error handlers (`on_command_error`) allow you to catch any errors that may occur during the execution of your code, preventing the bot from crashing and giving you control over flow of the program.
event .
async def on_command_error(ctx, error):
if isinstance(error, commands.CommandNotFound):
await ctx.send('Invalid command used.')
• Authentication Checks - Put checks in place before taking action on a request to ensure the person requesting data or actions holds the appropriate permissions. You wouldn't want someone with ill intent manipulating your bot simply because they asked it to perform an action. Use discord permissions and decorator checks (`@commands.has_permissions`) to make sure only allowed users can use a specific command.
Remember, mastering security measures is practical and, most importantly, safeguards the integrity of your application or bot and its user's data.Achieving the goal of retrieving a message by its ID in discord.py can be accomplished efficiently. The
get_channel
and
fetch_message
API calls come into play to facilitate this process, as you're calling these parts of the Discord API directly.
If you would like to get a specific message by its Id using discord.py library, working with the following bits of code should help:
# This is a sample code snippet, it might not work standalone. Add it within your application flow.
async def get_message_by_id(client, channel_id, message_id):
# Fetching the channel context.
channel = client.get_channel(channel_id)
try:
# Fetching the message using provided message_id.
message = await channel.fetch_message(message_id)
except:
message = None
return message
In this example, you don't require looping over all messages inside a channel, which could significantly improve performance if the channel contains large volumes of messages. However, bear in mind that you need administrative permissions to carry out this action. It's because the bot needs the 'Read Message History' permission to retrieve message history in text channels.
What you should keep in mind when getting a Discord message by its ID using the Python library, discord.py:
• One critical point is that IDs are unique integers and they're not interchangeable between types: i.e., a message ID cannot refer to a channel.
• It's crucial to remember that despite the invulnerability of deleted messages to this method, it cannot be used to access private messages or messages sent in direct message channels.
Refer to the [`discord.py`](https://discordpy.readthedocs.io/en/latest/api.html?highlight=fetch_message#discord.TextChannel.fetch_message) official documentation for more examples.
Now that you know how to retrieve a discord message by its id using discord.py, feel free to experiment with other functionalities that discord.py provides. This knowledge is translatable and flexible to allow you to manipulate message objects, enabling you to create comprehensive discord bots. Happy Coding!