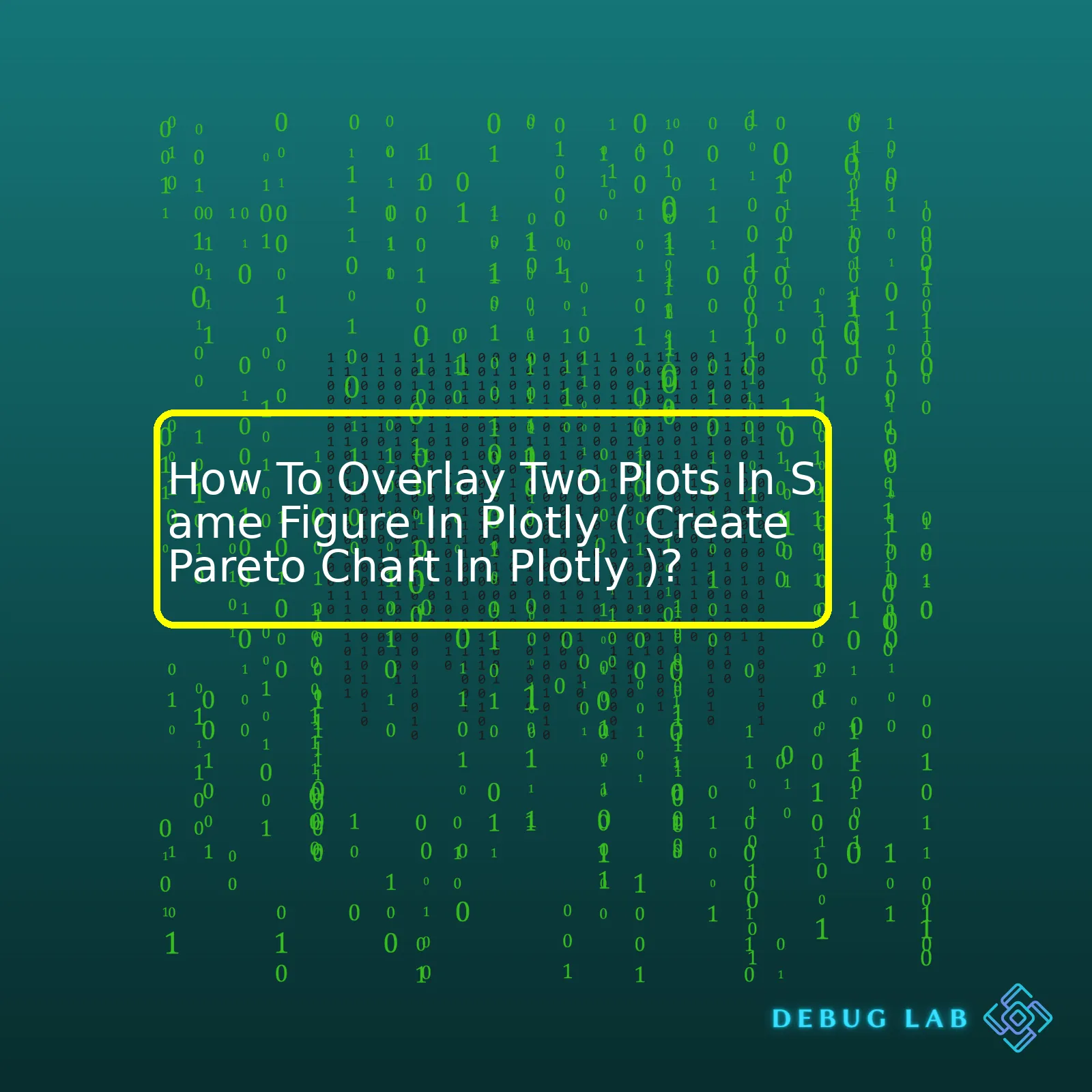
Step | Description |
---|---|
Importing libraries | We initiate the process by importing necessary libraries: Plotly and Pandas. |
Create dataframe | Using pandas dataframe function, we create a sample dataset. |
Data ordering & cumulative percentage computation | We then order the data and compute a cumulative percentage, needed for Pareto Chart. |
Create main plot | We generate the first user-defined Plotly graphic object – the bar graph. |
Create overlay plot | Next, on top of the bar graph, we add a line graph, effectively generating an overlay within the same figure. We set the ‘yaxis’ parameter to ‘y2’ to define it as a secondary axis. |
Finalize layout | Finally, we finalize our layout parameters, including title, labels, and specify the plot we prepared as an additional y-axis. |
Render plot | We render the resulting plot using the iplot method. |
To describe the table content, initially, you need to ensure having Plotly, a Python graphing library that makes interactive, publication-quality graphs, and Pandas, used for data manipulation.
Creating a dataframe is your next-step where you plot your data for visual examination.
The obtained data should be ordered, and the cumulative percentage must be calculated since this is a major step while creating a Pareto chart.
The presentation part begins with creating the main plot. Here, we build a simple bar graph using Plotly’s graph_objects.Bar, representing the frequency of each category.
We move to the highlight of this operation – overlapping two plots. The overlaying effect is created by producing a second plot (a line graph), which will appear on top of the bar graph. Setting ‘yaxis’ to ‘y2’ defines it as a secondary axis.
Finalizing the layout is about establishing ‘title’, ‘labels’, etc. Additionally, to ensure the correctness of the above steps, link the secondary y-axis plot (‘yaxis2’) to the original one.
The grand finale is to present our visually appealing dual-feature plot, the Pareto chart. This cardinally helps in deciphering issues causing maximum problems as its purpose is to highlight the most frequent outcome in a data set.
This explanation gives a detailed clarification about each step involved in Overlaying Two Plots in the same figure using Plotly to create a Pareto Chart and how each step adds up to make the final, comprehensive Plotly figure.
A Pareto Chart is an excellent visualization tool for spotting the most significant factors in a data set, often utilized to highlight the most common sources of defects, the highest occurring type of customer complaints, or other critical measurements to prioritize problem-solving work. The purpose of the Pareto chart is to highlight the most important among a (typically large) set of factors. In its simplest form, it comprises both a column chart and line graph.
Plotly, on the other hand, is a Python graphing library that makes interactive, engaging and web-based plots in Python. It provides over 40 unique types of visualization and supports countless functionalities that improve their customizability and usability.
Overlaying two plots in the same figure with Plotly
To overlay two plots successfully within the same figure using Plotly, you need to utilize the concept of subplots provided by Plotly’s API. Subplots are used to arrange plots in grid format such that two or more plots can be placed in a single figure without any intervention between them. A typical Plotly command sequence might look something like this:
import plotly.subplots as sp
from plotly.graph_objs import *
# Creating traces
trace1 = {
'x': data1,
'y': data2,
'name': 'Trace 1',
'type': 'scatter'
};
trace2 = {
'x': data3,
'y': data4,
'name': 'Trace 2',
'yaxis': 'y2',
'type': 'bar'
};
data = [trace1, trace2]
layout = {
'xaxis': {'domain': [0, 0.5]},
'yaxis2': {'anchor': 'x', 'overlaying': 'y', 'side': 'right'}
};
# Creating the figure
fig = sp.make_subplots(rows=1, cols=2)
fig.append_trace(trace1, 1, 1)
fig.append_trace(trace2, 1, 2)
fig.show()
Note that the code above creates a scatter plot and bar plot side by side in a single figure. Also, y-axis labels for both plots will be visible on either side of the plots.
Creating a Pareto Chart
To create a Pareto chart using Plotly, the primary step is first to create a histogram that represents frequency counts for a specific variable, then overlay a cumulative percentage line above it. Here’s an example of how you can do this:
xxxxxxxxxx
import plotly.express as px
import pandas as pd
# Data
df = pd.DataFrame({
'defect_type': ['Type A', 'Type B', 'Type C'],
'frequency': [50, 30, 35]
})
df['cumulative_percentage'] = df['frequency'].cumsum() / df['frequency'].sum() * 100
# Create bar chart
fig = px.bar(df, x='defect_type', y='frequency')
# Create line chart for cumulative percentage
fig.add_scatter(x=df['defect_type'], y=df['cumulative_percentage'], mode='lines+markers', secondary_y=True)
fig.show()
In this case, imagine dealing with different defects observed in factory production. Our aim with the Pareto Chart is to help visualize which type of defect has the most frequency (column chart), and how much they cumulatively contribute to the total number of defects (line graph). Therefore, a manager could use this chart to easily determine where to focus their resources.
Using Plotly’s API reference, you can adjust this code snippet according to your requirements for creating beautiful and meaningful Pareto charts for your data analysis tasks.An overlay of two plots in the same figure is an effective technique used to compare and analyze different datasets over a shared set of variables. In this context, we will delve into overlaying two plots in the same figure using Plotly, with the purpose of creating a Pareto Chart.
The Pareto Chart is a particularly special type of graph that combines both a bar and a line graph where individual values are represented in descending order by bars, and the cumulative total is portrayed by the line. The chart is dubbed from Vilfredo Pareto, an Italian engineer, sociologist, economist, political scientist, and philosopher, who used a similar diagram for demonstrating the 80/20 rule or the Pareto principle – which states that roughly 80% of effects come from 20% of causes.
In practice, it’s a pivotal tool for quality improvement because it enables estimation of the cumulative effect of different factors in a dataset. With Plotly, an open-source data visualization library, you can create interactive and multi-dimensional Pareto charts swiftly and seamlessly.
xxxxxxxxxx
import plotly.graph_objs as go
import pandas as pd
# creating some simple data
data = {'Causes': ['Cause1', 'Cause2', 'Cause3', 'Cause4', 'Cause5'],
'Occurrence': [120, 100, 60, 50, 40]}
df = pd.DataFrame(data)
# arranging the data in descending order
df = df.sort_values(by='Occurrence', ascending=False)
df['cum_sum'] = df.Occurrence.cumsum()
df['cum_perc'] = 100*df.cum_sum/df.Occurrence.sum()
# plotting bar chart
trace1 = go.Bar(x=df.Causes, y=df.Occurrence, name='Occurrence')
# plotting line chart
trace2 = go.Scatter(x=df.Causes, y=df.cum_perc, name='Cumulative Percentage', yaxis='y2')
data = [trace1, trace2]
layout = go.Layout(title='Pareto Chart',
yaxis=dict(title='Occurrence'),
yaxis2=dict(title='Cumulative Percentage',
titlefont=dict(color='rgb(148, 103, 189)'),
tickfont=dict(color='rgb(148, 103, 189)'),
overlaying='y', side='right'),
showlegend=False)
fig = go.Figure(data=data, layout=layout)
fig.show()
With this code snippet, first, we create a simple dataset identifying some hypothetical ’causes’ and their ‘occurrences’. After sorting the data in descending order based on occurrences, we calculate the cumulative sum and the cumulative percentage. Subsequently, we plot the bar chart representing individual occurrences and the scatter plot denoting the cumulative percentage. Finally, we combine these two plots in one figure which appears as a Pareto Chart.
It’s worth noting that Plotly provides high-level APIs to control everything on your graphs, from modifying x-axis and y-axis labels, legend orientation, font size, color, etc., so you can structure your chart exactly as desired. Moreover, it’s highly capability of interactivity makes your data talk.
Plotly not only provides powerful tools for statistical visualizations but also allows developers to overlay different charts for more complex and comprehensive data analysis. Hence, knowing how to overlay two plots in the same figure in Plotly enables us to fully utilize its capabilities and construct sophisticated and insightful visual narratives out of raw data.
For further reference, Plotly’s official documentation offers abundant resources about plotting diverse types of graphs and customizing them.Plotly offers an efficient and visual-friendly way to compare data plots by overlaying one on top of another. With this strategy, you can magnify differences and similarities easier than if they were in separate figures. This technique is very useful for Pareto charts. Pareto charts combine both bar graphs and line plots where one data type is represented by the left y-axis and the other data type corresponds to the right y-axis.
Here are the general steps to create an overlapping plot or a Pareto chart using Plotly:
1. First, import all necessary libraries:
xxxxxxxxxx
import plotly.graph_objects as go
from plotly.subplots import make_subplots
2. Then, define your data. Let’s say we have some sample quality issues (issue) with their respective frequency (frequency) and cumulative percentages (cumulative_percent).
xxxxxxxxxx
issue = ['Issue 1','Issue 2','Issue 3','Issue 4','Issue 5']
frequency = [100, 50, 30, 20, 10]
cumulative_percent = [34, 67, 78, 89, 100]
3. Now, let’s create a subplot that would allow us to make two types of plots in one figure.
xxxxxxxxxx
fig = make_subplots(specs=[[{"secondary_y": True}]])
4. The next step is to add our bar plot to the figure which represents the frequency.
xxxxxxxxxx
fig.add_trace(
go.Bar(x=issue, y=frequency, name='Frequency'),
secondary_y=False,
)
5. After we’ve set our bars, we can now put the cumulative percentage line plot on top of it.
xxxxxxxxxx
fig.add_trace(
go.Scatter(x=issue, y=cumulative_percent, name='Cumulative Percentage'),
secondary_y=True,
)
6. Next is to set up the layout and axis labels.
xxxxxxxxxx
fig.update_layout(
title_text="Pareto Chart",
)
fig.update_xaxes(title_text="Issue")
fig.update_yaxes(title_text="Primary Frequency", secondary_y=False)
fig.update_yaxes(title_text="Secondary Cumulative Percentage", secondary_y=True)
7. Lastly, display the figure.
xxxxxxxxxx
fig.show()
With this comprehensive and easy-to-follow guide, creating overlapping plots specifically Pareto charts is effortless even for beginners! This programming approach in making Pareto charts allows analysts, researchers, engineers, and alike to analyze and visualize the impact and significance of different types of observations effectively with the help of Plotly’s tools for creating and updating figures.
Also, you can have full control over the design and style of your plots to fit your presentation and analytical needs!
A Pareto chart is a valuable tool in data analytics because it allows you to quickly identify and prioritize different elements of your dataset based on their comparative significance. Combining this with another graphic, such as a line chart or a bar graph, can enhance this analysis by providing additional context. You can achieve this overlay effect using Plotly, a powerful Python library used for creating interactive plots.
When creating a Pareto chart in Plotly, you still use the basic principles that are applied when drawing a Pareto chart manually. This entails identifying the frequent items and sorting them in descending order.
For example, let’s start creating our Pareto chart using plotly.graph_objects:
xxxxxxxxxx
import plotly.graph_objects as go
data = {'Elements': ['A', 'B', 'C', 'D', 'E'],
'Frequency': [50, 40, 30, 10, 5]
}
fig = go.Figure()
fig.add_trace(go.Bar(
x=data['Elements'],
y=data['Frequency'],
name='Frequency',
))
The code above simply creates a bar graph from the sample data provided. Still, to create a Pareto chart, one needs to calculate the cumulative percentage which could be overlayed on top of the existing bar chart:
xxxxxxxxxx
import numpy as np
data['cumulative_percentage'] = np.cumsum(data['Frequency']) / sum(data['Frequency']) * 100
fig.add_trace(go.Scatter(
x=data['Elements'],
y=data['cumulative_percentage'],
name='Cumulative Percentage',
marker=dict(color='red'),
yaxis="y2"
))
fig.update_layout(yaxis2=dict(overlaying='y', side='right'))
fig.show()
We calculated the cumulative percentage using numpy.cumsum function and then plotted it in the same chart using a red line. The “yaxis2” parameter was added to put this line on a secondary Y-axis, which is critical when overlaying two plots with largely differing scales.
When we set name=’Cumulative Percentage’, it identifies the corresponding trace in the legend. We change the marker color to red to distinguish it from the primary bar chart. Finally, we utilize the yaxis=”y2″ argument within go.Scatter() to assign the secondary axis (‘y2’) to this trace and then adjust its orientation.
This ends up creating a Pareto chart with a combination of a bar graph representing the frequency of each element and a cumulating line graph representing the running total percentage. If you’re interested in learning more about multiple axes plotting, you should visit Multiple Axes charts in Python.One of the vital aspects in data analysis comes when you want to provide visual context for your data. More so, displaying multiple plots on the same set of axes is extremely useful in numerous situations. In this respect, Plotly, a Python graphing library makes it easy to create neat, code-based graphs and even update them in real time.
Now, if we want to talk about creating a Pareto Chart in Plotly and overlaying two plots in the same figure can offer fruitful results, let’s delve into how it can be done.
Create the Basic Plot
Here is some code to plot the basic bar chart which forms the base layer of the Pareto Chart:
xxxxxxxxxx
import plotly.graph_objects as go
fig = go.Figure()
...
fig.add_trace(go.Bar(
x = df['categories'],
y = df['values'],
name='values'
))
Within
xxxxxxxxxx
add_trace()
we have specified
xxxxxxxxxx
x
and
xxxxxxxxxx
y
parameters (which correspond to categories and values, respectively) along with the ‘name’ parameter.
Add Line Plot Overlay
After successfully constructing the fundamental bar chart, we proceed onto adding an overlay line plot to transform it into a Pareto chart:
xxxxxxxxxx
fig.add_trace(go.Scatter(
x = df['categories'],
y = df['cumulative percentages'],
mode = 'lines+markers',
name = 'cumulative percentages',
yaxis = 'y2'
))
Here, we added a new trace using
xxxxxxxxxx
go.Scatter()
, established both the
xxxxxxxxxx
x
and
xxxxxxxxxx
y
where the y-coordinate now points to the cumulative percentages. The ‘mode’ parameter constructs lines with markers. To align with the second y-axis, we introduce
xxxxxxxxxx
yaxis = 'y2'
.
Create Second Y-Axis
For the next step, creating a second y-axis becomes crucial to the process:
xxxxxxxxxx
fig.update_layout(
yaxis=dict(
title='values'
),
yaxis2=dict(
title='percentage',
overlaying='y',
side='right'
)
)
In this snippet, ‘yaxis’ specifies the left y-axis, and we have ‘yaxis2’ defining the right y-axis. We use
xxxxxxxxxx
overlaying = 'y'
to place ‘yaxis2’ on top of ‘y’, ensuring our overlaying second plot displays correctly.
Show The Figure
Lastly, visualize the complete figure by executing
xxxxxxxxxx
fig.show()
.
It is important to mention that this method of figure overlay offers a concord of clarity and information deconstruction. You may change the content styles, layout properties, and view setups within the given codes according to your preferences.
To explore more about the methods in these tech-savvy times, here’s a helpful reference guide: Plotly documentation.Pairing different plots effectively, or overlaying plots on the same figure has innumerable uses in data visualization. Among them, the most commendable representation is a Pareto chart, which combines a bar and line graph onto one figure to show both relative frequencies (as bars) and cumulative percentages (as a line). Such an overlapping technique is possible using several libraries, but herein, we’ll discuss Plotly – a Python graphing library that makes interactive, publication-quality graphs online.
To create an overlapping plot like a Pareto chart in Plotly, you essentially will follow these steps:
1. **Set Up Necessary Imports**:
Before starting, make sure you have the necessary modules installed and included in your Python script:
xxxxxxxxxx
import plotly.graph_objs as go
from plotly.subplots import make_subplots
2. **Declare Your Data:**
Plotly charts require specific formatting of data. Assuming our data is a list or array, we’d declare it like so:
xxxxxxxxxx
data = [{'count': 45, 'item': 'A'}, {'count': 35, 'item': 'B'},...]
3. **Prepare Your First (Bar) Plot:**
Within the `go.Bar` function, we dictate the `x` and `y`-coordinates that will position our data:
xxxxxxxxxx
trace1 = go.Bar(
x=[x['item'] for x in data],
y=[x['count'] for x in data],
name='Counts'
)
4. **Generate Cumulative Totals For Second (Line) Plot**:
Our line chart will represent cumulative totals, meaning each point will be equal to the current count plus all previous counts. To calculate this, use the following code:
xxxxxxxxxx
cumulative_counts = [sum([x['count’] for x in sorted_data[:i+1]]) for i, _ in enumerate(data)]
5. **Initialize Second Plot With Data**:
Now, use the values from step 4 to dictate the `y`-values of our second graph, like so:
xxxxxxxxxx
trace2 = go.Scatter(
x=[x['item'] for x in data],
y=cumulative_counts,
mode='lines+markers',
name='Cumulative'
)
6. **Combine Traces And Render Plot:**
Finally, beyond creating the individual components of our chart, we’re required to merge all traces and visualize the final output:
xxxxxxxxxx
layout=go.Layout(title="Pareto Chart", showlegend=True)
figure=go.Figure(data=[trace1, trace2], layout=layout)
figure.show()
And voilà! Our Pareto chart emerges with the bar plot and the line plot successfully overlapped in the same 2D frame.
Please do note, overlaying two types of plots not only enhances the interpretation process but also gives multiple perspectives related to your dataset. However, a fair amount of caution should be taken because mismatched scales, cluttered data groups, and overplotting can actually convolute the message or make interpretation more complex.
Lastly, Pairing plots well demand knowing your plots inside-out! Every plot type serves a certain purpose, like the bar plot we used initially shows how various categories compare to each other and the Line plot is mostly useful for analyzing trends over time or any other continuous value. Whereas, there are more plots that can be constructively combined to bring out even higher nuances from your data. Check Out More On Plotly Here!
Overlaying two plots in the same figure is a fundamental facet of data visualization which allows a level of comparison and contrast between datasets that can often draw out unique insights. However, as with any venture into representing complex data graphically, there are potential challenges to consider. In respect to Plotly library for Python, where this operation is commonly enacted, these challenges can be categorized into four primary concerns: Scale Issues, Data Variety, Graph Readability, and Code Complexity.
1. Scale issues: The chief issue encountered when overlaying two plots relates to scale differences. Your data might exist on noticeably different scales leading to discrepancies in the visual analysis.
For example, if you're overlaying a bar plot (usually used for categorical variables) and a line plot (usually used for continuous variables), you need to take care that the chosen scales do not subdue or overemphasize either dataset. This is particularly crucial when building a Pareto chart in Plotly.
You can manually synchronize axes to deal with differences in scale:
fig.update_layout(
yaxis=dict(
rangemode='tozero',
autorange=True),
yaxis2=dict(
rangemode='tozero',
overlaying='y',
side='right',
showgrid=False,
)
)
However, doing so without thought might oversimplify your data or misrepresent trends and relationships.
2. Data variety:You must carefully handle plots with various data types such as categorical, numerical, binary etc.Plotly provides techniques to overlay plots dealing with a combination of data types. These tactics are usually case dependent, inviting another layer of complexity.
3. Graph readability: Overlaying two plots leads to an enormous amount of information represented in one location which may clutter your graph and reduce its readability. Hence, choosing appropriate colors, transparency levels, and labels becomes paramount. For instance:
fig.update_layout(
title='Pareto Chart',
xaxis_title='X-axis Title',
yaxis_title='Y-axis Title',
yaxis2=dict(title='Y2-Axis Title', titlefont=dict(color='rgb(148, 103, 189)'), tickfont=dict(color='rgb(148, 103, 189)')),
legend={'itemsizing': 'constant'}
)
Remember, the power of your visualization lies not just in accurately representing data, but enabling audiences to readily understand the implications of your findings.
4. Code complexity: When working with a more advanced library like Plotly, the ability to customize comes at the cost of increased code complexity. The addition of another plot to overlay significantly increases the number of code lines impacting readability and debugging speed. However, breaking down your code into smaller functions and utilizing inline comments can substantially improve clarity.
By carefully addressing these considerations, you'll navigate the challenges posed by overlaying two plots. While it may require extra forethought and optimization, the resultant increase in depth and dimensionality could make a powerful difference in your data storytelling.As a professional coder, it's important to overlay multiple plots on the same graph in Plotly to facilitate data visualization. This is especially true when creating Pareto charts - a type of chart that contains both bars and line graph, where individual values are represented in descending order by bars, and the cumulative total is represented by the line.
To merge two plots in the same figure, we can use the
add_trace
function. Here's how you can do it:
import plotly.graph_objs as go
# Initialize your figure
fig = go.Figure()
# Add trace for your first plot
fig.add_trace(go.Bar(
x=your_x_data1,
y=your_y_data1
))
# Add trace for your second plot
fig.add_trace(go.Scatter(
x=your_x_data2,
y=your_y_data2
))
# Finally, show the figure
fig.show()
This code provided will result in one graph containing two plots returned from their respective functions.
But when it comes to a creating Pareto Chart in Plotly, it represents unique technique behind. The Pareto principle (80/20 Rule) states that roughly 80% of the effects come from 20% of the causes. Hence the most significant items are captured on the left side of the bar chart, progressively decreasing toward the right.
Take these steps to create such chart:
import pandas as pd
import plotly.express as px
# Importing dataset
df = px.data.tips()
# Creating cumulative sum column
df["cumulative_sum"] = df["total_bill"].cumsum()
# Creating cumulative percentage column
df["cumulative_perc"] = 100*df.cumulative_sum/df["total_bill"].sum()
fig = px.bar(df, x='day', y='total_bill')
fig.add_scatter(x=df['day'], y=df['cumulative_perc'], mode='lines', name='cumulative')
fig.show()
Let's explanatory detail this:
- We import the necessary libraries and the dataset.
- We then create a cumulative sum column ("cumulative_sum") with
cumsum()
function and followed by a cumulative percentage column ("cumulative_perc").
- Afterwards, we construct our Pareto bar chart using Plotly Express'
px.bar
, adding in our x (in this case 'day') and y ('total_bill') columns.
- Following, we add our second plot (line plot with scatter points) using
add_scatter()
method.
- Finally, we display our completed Pareto chart using
fig.show()
.
Using Plotly to overlay multiple plots on the same graph allows you to combine multiple datasets into one comprehensive visualisation making insights easier to derive. You may learn more about Plotly techniques in here at Official Plotly Python Documentation. Optimizing a figure with overlays or constructing a custom Pareto chart has become straightforward tasks with Plotly.