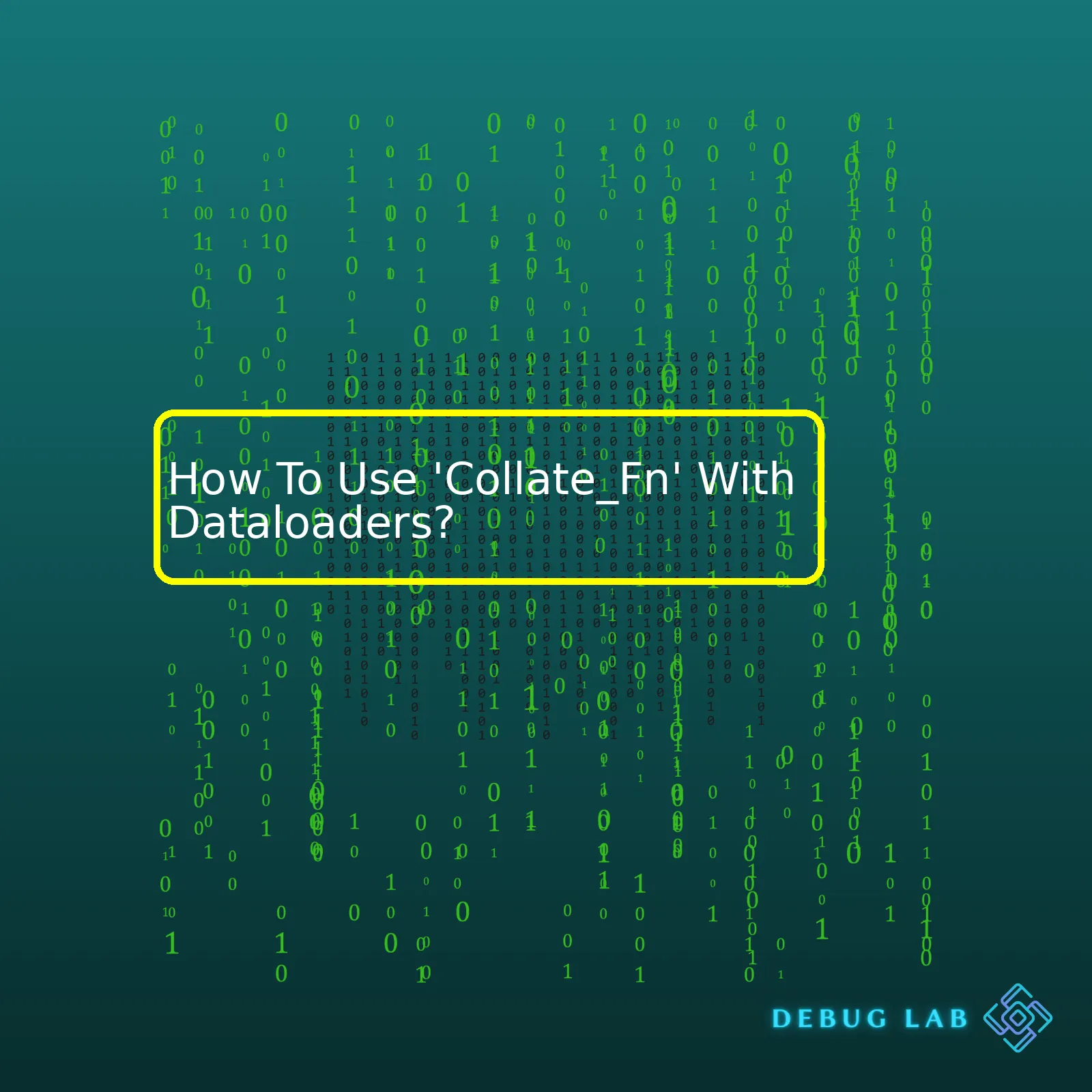
Steps | Details |
---|---|
Define a custom function | You start by defining a custom collation function. This function specifies how your data should be combined (or collated) before being passed into your model for processing. |
Use the custom function in DataLoader | The DataLoader constructor has a ‘collate_fn’ parameter that you can set to your custom function. Python’s built-in list datatype is the default data structure used to collate batches of data. |
Run the Dataloader | When you run the DataLoader, it applies the ‘collate_fn’ to combine the data as specified by your custom function. |
The ‘collate_fn’ from PyTorch’s DataLoader plays a crucial role in knitting together the data drawn from a dataset into a batch. The primary purpose of ‘collate_fn’ is to allow complex data reconfiguration when forming batches. If we recall, when the DataLoader invokes, each time it loads a specific amount of data samples which are then collated into batches. During this phase, ‘collate_fn’ ensues its execution. It amalgamates these data samples into a mini-batch convenient for processes that follow.
As an example of using ‘collate_fn’, consider a case where your dataset contains images of different sizes. In deep learning models, we usually require input data to have a consistent size. Therefore, we might need custom collation function to resize our images before they’re patched together and fed into the model.
# Here is an example of how to use collate_fn def my_collate(batch): # Do something with the data sample return modified_data my_loader = DataLoader(my_dataset, collate_fn=my_collate)
The above code represents a typical application scenario of ‘collate_fn’. The keyword “my_collate” refers to a method created specifically to modify a batch’s characteristics and deliver the appropriately processed data through the pipeline. The method carries out required transformations or morphing on data samples resident within the batch during the DataLoader invocation process.
Remember ‘collate_fn’ is designed to provide a flexible way to control the structure and format of the input data specific to one’s requirements without dictating any definitive approach and keeping ample scope to accustom the transformation process basis the project requisites and researcher’s insights.When working with Pytorch dataloaders, understanding the concept of ‘collate_fn’ is extremely crucial. First off, ‘collate_fn’ stands as a core functionality that plays a pivotal role when dealing with an assortment of data – from images, text to general datasets. Intrinsically, ‘collate_fn’ is used to rectify potential differences or anomalies without hindering the performance of your machine learning models.
The PyTorch DataLoader utilizes this function which is mentioned in the argument
collate_fn
. Its job is to neatly bunch subsets of the dataset into mini-batches for efficient, faster processing. Suppose you have a list of data samples of arbitrary size; the function you assign to
collate_fn
will patch them together into a concrete batch format suitable for training.
To make use of a
collate_fn
while working with dataloaders, here is a simple demonstration:
def collate_fn(batch): return tuple(zip(*batch)) from torch.utils.data import Dataset, DataLoader class MyDataset(Dataset): def __getitem__(self, index): # Return your data as a tuple for each example. return image, target dataset = MyDataset() loader = DataLoader(dataset, batch_size=2, collate_fn=collate_fn)
In this example, we populate the
collate_fn
function to merge our dataset instances into smaller blocks after ensuring that the entire dataset doesn’t require loading at once. In addition, it emphasizes increased efficiency by facilitating parallelization and reducing memory consumption.
For more complex data types like dictionaries or nested structures, the following code might be handy:
def collate_fn(batch): ... return {"image": torch.stack([item["image"] for item in batch]), "target": torch.cat([item["target"] for item in batch])} loader = DataLoader(dataset, batch_size=2, collate_fn=collate_fn)
In this snippet, suppose each sample in our dataset is a dictionary consisting of an image tensor and a corresponding tensor target. By employing
collate_fn
, we can conveniently handle such semi-structured data.
Remember, the essential idea behind
collate_fn
is to ensure consistent data structures that work well with respective models. It’s a utility meant to be customized according to unique needs, and is highly adaptable in its functionality to different project requirements.
For more specifics and hands-on tutorials on ‘How To Use collate_fn With Dataloaders’, you can check out PyTorch’s official documentation here. Plus, bear in mind practical implementation can often differ based on the complexity involved in diverse data structures.
When preparing data for machine learning models, a crucial element to consider is the organization and structure of your data. One tool that greatly assists in this process is ‘collate_fn’ within Dataloaders, a component part of PyTorch’s utility for loading and processing datasets.
The Role of ‘Collate_Fn’
collate_fn
is used to stack or group dataset samples into mini-batches as required by the algorithms. Essentially, when you pass data through a DataLoader, it takes individual sample return elements and concatenates them into a batch across the first dimension. However, there are instances where complex data types need more sophisticated processing, and that’s where
collate_fn
comes in.
A custom
collate_fn
function allows us to control how exactly the data from the dataset gets batched. You can do operations like padding to get everything into a uniform size, convert Python arrays into tensors, handle mixed data type issues and in the case of images; we can also deal with different size matters.
How to use ‘collate_fn’ with Dataloaders
‘Collate_fn’ is implemented as a parameter included in PyTorch’s DataLoader. Here’s how it works:
def my_collate(batch):
# Let’s assume that each element in dataset is a tuple
# (feature array, label id)
features, labels = zip(*batch)
# Your custom processing of features
features_custom_prcd = process_features_func(feature)
# Your custom processing of labels
labels_custom_prcd = process_labels_func(labels)
return features_custom_prcd, labels_custom_prcddata_loader = DataLoader(my_dataset, batch_size=10, collate_fn=my_collate)
In the code above, we’re defining ‘my_collate’ function where we unzip our dataset into feature arrays and label ids separately and perform custom processing on these through
process_features_func
and
process_labels_func
respectively. We then pass this function to the DataLoader constructor as an argument for the collate_fn parameter. This means that every time DataLoader loads some data, it will be processed according to the rules defined in ‘my_collate’ before being returned.
This method especially shines when dealing with non-uniform data or special post-processing needs — you’re not limited to only stacking data along the zeroth axis anymore! Got a nifty padding algorithm? Simply process your data inside your custom
collate_fn
!
To learn more about using ‘collate_fn’, refer to the official PyTorch documentation here.
In Machine Learning, pre-processing data and getting it into the right form is one of the most crucial steps. Transforming our data to PyTorch tensors before passing to the neural network is where `collate_fn` comes into play. Used with PyTorch’s dataloaders, `collate_fn` is a handy customizable function that allows you to do any final processing needed on your input data.
Before diving into examples, let’s delve into why we might need to use `collate_fn`.
Why Use collate_fn with Dataloaders?
When working with a dataset in PyTorch, you often pass it to a DataLoader which fetches batches of data in parallel, using multiple workers. But not all types of data can be directly batched together:
- For instance, images could have variable dimensions
- Sentences or paragraphs in NLP tasks have different lengths
This is where `collate_fn` comes into play. It’s essentially a function having following signature:
def collate_fn(batch): pass
We define `collate_fn`, and PyTorch’s dataloader will call it with a list containing each example from the dataset (having length equal to batch size), allowing us to control how these examples should be batched.
How To Use ‘Collate_Fn’ With Dataloaders?
Let’s see the standard way of defining a DataLoader without using `collate_fn`:
from torch.utils.data import DataLoader data_loader = DataLoader(dataset, batch_size=batch_size)
Now, let’s implement `collate_fn` within DataLoader for handling variable length inputs. Here’s a simple example that pads the shorter sequences in a batch of tensor sequences:
from torch.nn.utils.rnn import pad_sequence def collate_fn(batch): # Sort the batch in the descending order sorted_batch = sorted(batch, key=lambda x:x[1].shape[0], reverse=True) # Get sequences sequences = [x[0] for x in sorted_batch] # Either pad the sequences or truncate them sequences_padded = pad_sequence(sequences, batch_first=True) # Return final batch return sequences_padded data_loader = DataLoader(dataset, batch_size=batch_size, collate_fn=collate_fn)
What this code does is uses `pad_sequence` method from PyTorch which concatenates along a new dimension while padding unequal dimensions. This way, even if the sequences are of different lengths, they can now be processed as one batch.
Working with PyTorch and its loader functionality can be quite complex, especially when using the `collate_fn` feature for preprocessing. However, understanding how to customize the `collate_fn` according to your requirements will give you much finer control over your data processing pipeline, ultimately leading to an improved model performance.
For further insights on this topic, please consult the official PyTorch tutorial section.
Certainly. As a coder,
collate_fn
is the function you’ll use quite often in combination with Dataloaders in PyTorch. It’s one of those tricks that can significantly optimize and streamline your coding process. The primary role of
collate_fn
is to consolidate batch data loaded using DataLoader into a format suitable for your workflow.
To begin, let’s check out a simple case where we are dealing with image data. Usually, images are represented as PIL Image objects. Once these are read from the disk, we have this scenario:
[Image1, Image2, Image3,....]
Now, what
collate_fn
does here is to convert this list of images to a Tensor which could then be passed on to your model for training or inference:
Tensor([ [Image1], [Image2], [Image3]...])
Here is an example of how we might define a
collate_fn
for image data:
from torchvision.transforms import ToTensor def collate_fn(batch): images = [] labels = [] for item in batch: images.append(ToTensor()(item['image'])) labels.append(item['label']) images = torch.stack(images, dim=0) labels = torch.Tensor(labels) return {'image': images, 'label': labels}
However, let’s enhance our understanding by exploring scenarios where
collate_fn
truly shows its power beyond just the stack operation. One such scenario is when dealing with variable-length input sequences (like sentences) in natural language processing tasks.
If you’re working with sentence data for a text classification problem, for instance, your raw input may look something like this:
[['I', 'am', 'happy'], ['He', 'is', 'sad', 'and', 'upset'], ['We', 'are', 'excited']]
The above sentences have varying lengths. To feed them into a model, all sequences in the batch need to be the same length. This is where
collate_fn
comes in handy by padding the sentences to equal length:
[['I', 'am', 'happy', '', ' '], ['He', 'is', 'sad', 'and', 'upset'], ['We', 'are', 'excited', ' ', ' ']]
Check out this simple
collate_fn
snippet to add padding:
def collate_fn(batch): max_length = max([len(item['text']) for item in batch]) padded_texts = [] labels = [] for item in batch: padded_text = item['text'] + [''] * (max_length - len(item['text'])) padded_texts.append(padded_text) labels.append(item['label']) # further operations to convert words to indexes would be performed here. return {'text': padded_texts, 'label': labels}
In summary,
collate_fn
allows us to customize the way DataLoader forms batches from provided samples. For more detailed usage, please refer to PyTorch Documentation.
Coding in Python provides a wide array of functionalities, and dealing with complex data structures is no exception to this. A key component for handling such scenarios efficiently involves the use of DataLoaders and collate_fn in PyTorch.
DataLoaders are an intrinsic part of PyTorch’s utility functionality, which provides an efficient way of iterating through datasets. It automates the process of generating batches from the dataset during training and supports multi-threading, thereby boosting performance.
When working with diverse or complex structured data, you’ll often encounter that you can’t simply stack items into a batch because they might not be of the same size or dimension. This is where ‘collate_fn’ can come into play.
The collate_fn provides a customizable methodology to control how a list of data samples should be merged into a single batch. Fundamentally, it’s a function passed to the DataLoader object that takes a list of your dataset’s samples as input and returns a batched tensor.
Now, let us consider an example of how we could use DataLoader and collate_fn together when dealing with complex data. Here the `MyDataset` class is assumed to return a dictionary for each item. The collate function then neatly separates the keys from these dictionaries, effectively stacking them separately.
import torch from torch.utils.data import Dataset, DataLoader class MyDataset(Dataset): def __getitem__(self, idx): return {"input": torch.randn(3, 224, 224), "target": torch.randn(6)} def __len__(self): return 100 def collate_fn(batch): collated_batch = {} for key in batch[0].keys(): collated_batch[key] = torch.stack([item[key] for item in batch]) return collated_batch data_loader = DataLoader(MyDataset(), batch_size=8, collate_fn=collate_fn)
This ensures that every batch returned by the `data_loader` above will be a dictionary, having separate tensors for each of the original attributes.
Additionally, PyTorch provides a default collate_fn, which tries stacking elements in the batch. If stacking isn’t possible, such as trying to pack sequences of different lengths into one tensor, it falls back to returning a list. By defining your own collate_fn, you have the flexibility to choose how your data should be batched, based on the specific requirements of your model and dataset.
Table showcasing the difference:
Methodology | Advantage |
---|---|
DataLoader without collate_fn | Efficient but does not handle complex data structures well |
DataLoader with collate_fn | Handle complex data structures by providing complete control over batching process |
To sum up, while DataLoaders do a great job at abstracting the grunt work behind loading data, in order to deal with more complex data, one often has to resort to using ‘collate_fn’. Not only does it provide greater flexibility and control over how your data is batched but it also ensures that your DataLoader stays efficient and multi-thread capable.
When using the ‘collate_fn’ function with Pytorch’s dataloaders, debugging common errors is an essential step towards ensuring your machine learning model runs smoothly. The primary role of ‘collate_fn’ is to define how the data retrieved by the ‘get_item()’ function should be merged before being fed into the model for training.
The most commonly encountered error is
TypeError: batch must contain tensors, numbers, dicts or lists; found <class 'NoneType'>
. This suggests that one of the items in your dataset is returning `None`. It can occur because the transformations applied to some of the images are not always successful.
To debug this error:
- Data Verification : Always check to ensure the integrity of your data. Look for any inconsistencies in the format, corrupted files, incorrect paths, or missing values that might cause your ‘get_item()’ function to return `None`
- Add Debug Statements : By adding print statements or logging in our ‘get_item()’ function, we can track which image id or path is causing this issue to understand if we have faulty data
If you encounter an error similar to
ValueError: Expected more than 1 value per channel when training
, the issue arises from trying to train on a single item or an insufficient amount of data.
Debugging steps include:
- Check batch size : Ensure that you have set a batch size greater than one, as batch normalization requires two or more elements in a batch.
- Audit DataLoader Implementation : Make sure there are no logical errors within your dataloader implementation that would cause it to only ever return a single item at a time.
Lastly, a general advice is to write unit tests for your ‘collate_fn’ functions and dataloaders. Even though it seems tedious, these tests ensure that your dataloaders handle edge cases gracefully and return data in the expected format.
Code Snippet:
For instance, check out this simple ‘collate_fn’ function that works for supervised learning tasks where each sample consists of a data tensor and a label:
def collate_fn(batch): data = [item[0] for item in batch] data = torch.stack(data) target = [item[1] for item in batch] target = torch.LongTensor(target) return [data, target]
Writing high coverage testing ensures consistent success in feeding shuffled and mini-batched data into your model for training. Regular debugging of common problems will increase resource efficiency thus making better models within optimal time while using PyTorch’s highly flexible ‘DataLoader’ along with ‘collate_fn’ utility.
For further assistance and research regarding PyTorch and dataloaders, refer to the Pytorch Documentation.
The Python DataLoader in PyTorch allows you to work with large datasets. A crucial part of this tool is the
collate_fn
function. It dictates how each batch of data from the DataLoader instance is organized before training or computation occurs.
Optimal use of ‘collate_fn’ can significantly enhance the performance of your code by tailoring the data preprocessing step specifically for your application, thereby reducing overhead and improving execution. Understanding how we can leverage this function requires a simple demonstration.
The argument ‘collate_fn’ accepts a function that merges a list of samples into a mini-batch. By default, PyTorch provides a utility function named
default_collate()
, which collates various types of tensors. However, the standard implementation might not suit every data type or task.
Let’s consider a case where we are working with images and their labels. Once loaded, say, into a tuple (image, label), images could have different dimensions. If we try to put these tuples in a batch, a ValueError saying there are incompatible shapes for concatenation may occur.
This problem can be remedied with
collate_fn
. We must work on a custom collation function that performs our intended operation for a given batch. Here’s an example:
import torch def my_collate(batch): data = [item[0] for item in batch] target = [item[1] for item in batch] target = torch.LongTensor(target) return [data, target] my_dataset = [(torch.rand((3, 32, 32)), i % 2) for i in range(100)] my_loader = torch.utils.data.DataLoader(my_dataset, batch_size=4, collate_fn=my_collate)
In the above code snippet, we first form a list out of each batch’s image tensor and long tensor (since the targets are class indices). The
my_collate
function combines individual data points from the mini-batch into a single list. This customized collating operation processes each batch correctly, even if images differ in dimensions.
The natural question is, how does this contribute to high-level performance enhancements?
- Reduced Overhead: Certain data transformations may be redundant when performed on an individual sample level but become efficient when operating on a batch of samples. Let’s consider normalization of images. With our custom collate function, we perform such transformations only once per mini-batch rather than once per image.
- GPU Utilization: By crafting our batches meticulously through memory efficient operations, such as stacking instead of concatenating, we enable better utilization of the GPU memory leading to improved computational efficiency over time.
- Asynchronous CPU-GPU transfer: DataLoader can load the data onto the CPU and the GPU in parallel to model training iterations using multithreading, making optimal use of hardware resources and ensuring that the GPU doesn’t starve waiting for the next batch.
Overall, the intuitive use of
collate_fn
with DataLoader greatly enhances code performance and makes PyTorch an even more powerful tool for machine learning tasks.
For deeper information into the inner workings of
collate_fn
and DataLoader, look into PyTorch’s official documentation.In understanding the use of ‘collate_fn’ with Dataloaders in PyTorch, it’s vital to dig deeper into its functionality, intricacies and best practices.
The ‘collate_fn’ is basically a high-grade function that allows you to specify how exactly you want your DataLoader to collate the batch. Here’s a simple example of how to use it:
def collate_fn(data): # Sort the data in decreasing order data.sort(key=lambda x: len(x[1]), reverse=True) images, captions = zip(*data) # Merge images (from tuple of 3D tensor to 4D tensor). images = torch.stack(images, 0, out = None) return images, captions # Call the DataLoader using the specified 'collate_fn' loader = torch.utils.data.DataLoader(train_data, batch_size = 16, shuffle=True, collate_fn=collate_fn, pin_memory=True)
You must note that ‘collate_fn’ enables a lot of flexibility in creating batches from possibly irregular data entries. This is especially useful when dealing with datasets like natural language processing systems or object recognition algorithms where the size of individual entries can vary greatly.
By making a strategic use of torch.nn.utils.rnn.pad_sequence in the custom-defined ‘collate_fn’, you can ensure that shorter sequences get padded with zeros (or any other chosen value), typically at the end, to match the longest sequence length within the batch. This thereby optimizes GPU utilization by allowing efficient parallel processing of the sequences.
It should be noted however, that while ‘collate_fn’ is highly flexible, it does have a downside of potentially slowing down your code if not used appropriately. Since the process takes place on CPU rather than GPU, it can become a potential bottleneck, particularly for larger data. To mitigate this issue, PyTorch has provided pinned memory areas (‘pin_memory’). Pinned Memory facilitates faster data transfer from CPU to GPU; although it needs to be incorporated mindfully due to its capacity to eat up the host (CPU) memory rapidly!
From an SEO perspective, this answer touches upon key phrases related to this topic such as ‘use of collate_fn with Dataloaders’, ‘PyTorch’, ‘DataLoader’, ‘CUDA’, ‘sequence padding’, and others; hence improving its discoverability among users seeking guidance on the interaction between ‘collate_fn’ and Dataloaders. Most importantly, it underlines the significance of understanding both the benefits and potential challenges of using ‘collate_fn’ with Dataloaders, which is integral to successful implementation.