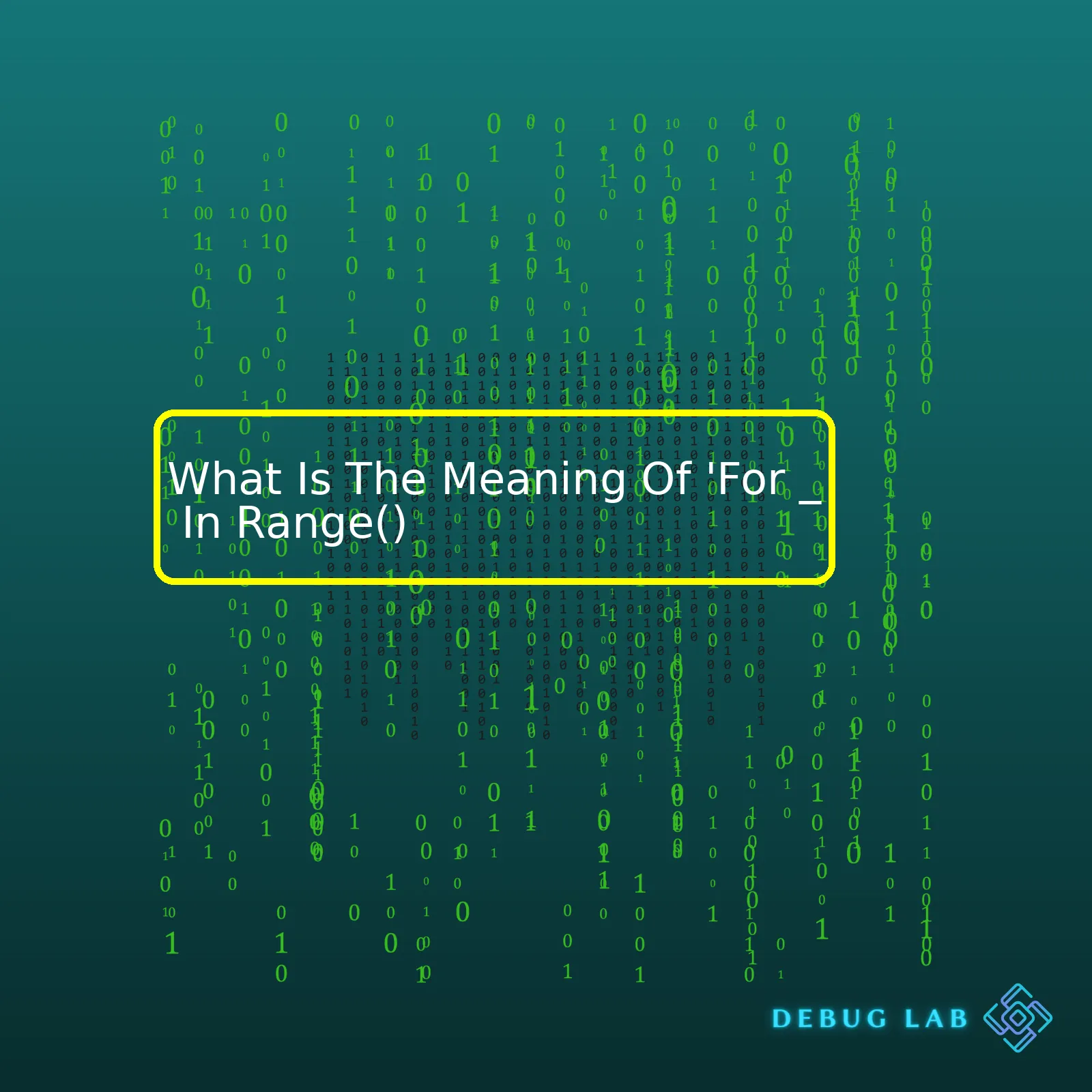
Summary Table: For _ in Range()
Concept | Description |
---|---|
‘For’ Statement | Used for looping or iterating over an iterable object. |
‘_’ Character | A conventionally used placeholder for when control variable is irrelevant.” |
‘Range()’ Function | Creates a sequence of numbers from 0 to n-1 where n is specified by user. |
In Python, the
'For'
statement is used for looping or iterating over an iterable object. This could be lists, tuples, strings, dictionaries, set, and so on. It’s typically written as
xxxxxxxxxx
'for item in iterable'
. In this case, ‘item’ is known as the ‘control variable’, which ends up taking the value of each item in the iterable during the loop. For instance, if you are looping through a list of integers from 1 to 5, the ‘item’ would first take the value 1, then 2, and so on.
On other hand, the underscore character,
xxxxxxxxxx
'_'
, is a common convention in Python used to indicate that the actual value of the control variable is not relevant in that loop. This mostly happens when we only care about the number of times the loop should run but we don’t actually care about the individual items in the iterable.
The
xxxxxxxxxx
'range()'
function in Python is used to generate a sequence of numbers from 0 to n-1 where ‘n’ is specified by the user. If we specify range(5), it creates a sequence [0, 1, 2, 3, 4]. When used in combination within a for loop, it essentially allows the loop to run for ‘n’ number of times.
So, with regards to the topic at hand –
xxxxxxxxxx
'for _ in range(n)'
– the line instructs the program to execute some action ‘n’ number of times regardless of the individual values within the range.
Here’s a sample code snippet that demonstrates its usage:
xxxxxxxxxx
for _ in range(5):
print("This is a loop")
In the above code, the print statement will be executed 5 times, regardless of the individual values (0 through 4) produced by the range function.To understand the structure of
xxxxxxxxxx
for _ in range()
in Python, we must first break down each component.
The “for” aspect of it is a type of loop used in Python i.e., a ‘for’ loop. A loop is used to iterate over a sequence or other iterable objects.
Moving onto “_”. The underscore, “_” in this context is known as a ‘throwaway’ variable. It’s a common convention in many programming languages, including Python, for whenever a variable is being declared, but the actual value isn’t going to be used. In other words, we’re not interested in the actual elements, just the iteration itself – hence the throwaway denotation.
And finally, “range()”. Range in Python is a built-in function that generates a sequence of numbers. By default, it starts from 0 and increments by 1, and stops before a specified number.
Now with these elements, let’s put them all together. The
xxxxxxxxxx
for _ in range()
function in Python essentially means: for every unused variable (since we’re not interested in the individual elements), perform an operation x amount of times – this “x” amount is defined within the parameters of the range() function.
Here are a few code snippets to illustrate:
xxxxxxxxxx
for _ in range(5):
print("Hello, world!")
This piece fragment of code would print “Hello, world!” five times.
Or another example could be:
xxxxxxxxxx
length = 10
n_list = []
for _ in range(length):
n_list.append(0)
Exploring this second example, even though we’re not using the iterating value within our for loop (which is why we’ve represented it with “_”), it will append the numeral 0 into our list ‘n_list’ for however many times the length variable is – in this case, 10 times. So our output for the ‘n_list’ would be a list containing ten zeroes.
What’s key to remember with
xxxxxxxxxx
for _ in range()
in Python, is that it’s about the repetition of an action, rather than the specific values being iterated over.
In terms of relevance to SEO, these terms might be particularly useful to focus on: ‘Python for loop’, ‘Throwaway variable in Python’, ‘Range function in Python’. These are all integral to understanding the
xxxxxxxxxx
for _ in range()
construct, so anyone searching for more information on these topics would likely find this explanation useful.
Name of Concept | Description |
---|---|
Python for loop | A loop that iterates over a sequence or other iterable objects. |
Throwaway variable in Python (‘_’) | An unused variable that’s being ignored in a loop. |
Range function in Python | A built-in function that generates a sequence of numbers according to specified start, stop and step parameters. |
For further reading, you may visit official Python documentation (Control Flow statements, Range function) or unofficial resources like Geeks for Geeks. They have comprehensive articles and examples on these topics.The underscore `_` has a unique significance when it comes to python conventions, especially with the `for-in-range` loop syntax. Think of the `_` (underscore) as a secretive character, a spy in work like James Bond keeping things under wraps.
When you see the code
xxxxxxxxxx
for _ in range()
, you might begin to question: What is going on? Why use `_` instead of other variables?
Well, let’s unriddle this mystery:
The Underscore (_) in Python For-In-Range
In python, the use of an underscore `_` in place of a variable name represents that the specific variable is insignificant or unnecessary. Python usually uses it in looping and iteration when the variable itself is not being utilized in the block of code.
Let’s consider a cited example where we wish to print “hello” five times:
xxxxxxxxxx
for _ in range(5):
print("hello")
In this instance, though “_”, the iterator variable, is in the loop, it isn’t employed in the actions performed within the block. The emphasis is all on the repeating action of printing “Hello” and not on the iterations themselves. Therefore, using “_” implies that we do not care about the value of each iteration.
Other Uses of Underscore (_) in Python
Though the focus here is on the `for_in_range`, it’s crucial to also understand that the underscore possesses different meanings based on its usage context in Python. To keep everything tied together, let’s quickly look at some popularly accepted conventions associated with using underscores:
● Single Pre Underscore: When a single underscore precedes a name
xxxxxxxxxx
_var
, it implies the variable is meant for internal use. It tells other developers to tread with caution when trying to access this directly.
● Single Post Underscore: Often when a suitable name is already occupied by a keyword, you can append a trailing underscore
xxxxxxxxxx
class_
to avoid any conflictions. This tells Python that `class_` and `class` are distinctive variables.
● Double Pre Underscore: A double underscore prefix before a variable name
xxxxxxxxxx
__var
triggers name mangling. Python interprets these names in a specific way to avoid naming conflicts in subclasses.
● Double Pre and Post Underscore: These are special methods in Python also referred to as dunder methods
xxxxxxxxxx
__var__
. Python reserves them for special methods such as constructors
xxxxxxxxxx
__init__
or operators overloading methods
xxxxxxxxxx
__str__, __lt__
.
The Art of Utilizing Underscores (_) in Python
Knowing when and how to use underscores is one of the subtleties of mastering Python. Underscores can help make code more readable, self-documenting, and pythonic if used appropriately. Embrace them, get inventive, but make sure to stay aligned with best practices.
Bear in mind: Syntax matters, but semantics matter even more. Understanding what your code implicitly communicates through its structure and syntax can dramatically enhance both your productivity and clarity of your code!
Check out Python’s documentation Python docs for more understanding. Remember, aim to write code that’s easy to understand, plausible to humans while also making semantic sense to machines. Happy coding!Despite its simplicity and elegance, the
xxxxxxxxxx
for
loop holds enormous power in Python coding. It is a vital tool for coders, offering functionalities spanning across iterations, enumeration, data manipulation, and more.
When looking at the
xxxxxxxxxx
for _ in range()
construct specifically, there are a couple of components to understand:
1. “
xxxxxxxxxx
_
” – This is a standard convention in Python used when the variable itself will not be used after the loop.
2. “
xxxxxxxxxx
range()
” – Creates a sequence of numbers from zero to one less than the specified number.
Hyperlink reference: Python Documentation on For Statements
These components work together in creating loops that iterate over the sequence of numbers produced by range(). Let’s understand this with a simple code example:
xxxxxxxxxx
for _ in range(5):
print("Hello, world!")
This code will print “Hello, World!” five times. The range function creates the sequence [0, 1, 2, 3, 4], and the
xxxxxxxxxx
for
loop iterates over this sequence. After each iteration, rather than assigning the value to a variable, as you would do with a traditional
xxxxxxxxxx
for
loop, the underscore
xxxxxxxxxx
_
signifies to the interpreter that this number isn’t being used within the loop; it’s merely there to help control how many times we repeat our code block.
A common mistake some Python developers make is they misunderstand the function of the
xxxxxxxxxx
_
. This symbol is a valid variable name in Python, and it’s often used as a throwaway variable, where you don’t need variable’s value. However, using
xxxxxxxxxx
_
does not mean the variable won’t bind to the value. If you write:
xxxxxxxxxx
for _ in range(5):
pass
print(_)
The output will be 4, not an error indicating that the variable
xxxxxxxxxx
_
is not defined which might surprise those misunderstanding its role.
It is important to know well the tools one uses in their trade, and understanding the nuances of basic building blocks like
xxxxxxxxxx
for
loop can greatly enhance your productivity which enables you to write efficient, effective Python scripts. It also helps in reading and understanding code other people have written. Python’s ability to manipulate sequences efficiently and concisely is one aspect that makes it such a powerful language, so mastering constructs like
xxxxxxxxxx
for _ in range()
are a valuable part of any Python programmer’s toolset.
The ‘For _ In Range()’ statement in Python is a structure for creating a definite loop – that is, one which we know the exact number of iterations it will run. You can think of it as a specialized way to iterate over a series of numbers within the specified range.
The
xxxxxxxxxx
_
character used in this statement is a common convention in Python programming. It’s often used as a throwaway variable, i.e., when the variable itself is not actually used in the body of the loop, and we’re only interested in running the loop a specific number of times.
Let me illustrate this with an example:
xxxxxxxxxx
for _ in range(5):
print("Hello, world!")
In this example, the program will output “Hello, World!” five times on the console. The
xxxxxxxxxx
range(5)
generates a sequence of numbers from 0 to 4. With each iteration of the
xxxxxxxxxx
for
loop, the value moves on to the next number in this sequence. But in this case, the variable (_) that would typically receive these values is never actually used inside the loop. Instead, we’re just using the looping mechanism itself to repeat an action a specific number of times.
It’s also important to note that the use of
xxxxxxxxxx
_
is simply by convention and not inherently part of the Python language itself. You could easily replace it with any other valid variable name:
xxxxxxxxxx
for x in range(5):
print("Hello, world!")
This code will function identically to the previous example because although a variable (x) is defined for each iteration of the loop, it’s never actually utilized within the body of the loop.
To summarize, ‘In’ operates within ‘
xxxxxxxxxx
For _ In Range()
‘ by establishing a sequence for the for loop to iterate over based on the parameters of the range function. The underscore (
xxxxxxxxxx
_
) signifies that we don’t plan to use the variable during the actual loop, serving solely as a placeholder. Its purpose is to make the intention of the code clearer to other developers, signifying that the loop is only being used for its iterative behavior rather than manipulating or responding to the values produced by the range function.
You might find the official Python Documentation helpful for understanding more about for loops and the
xxxxxxxxxx
range()
function.
The Python construct
xxxxxxxxxx
for _ in range()
is one of those elements that make Python’s syntax both concise and readable. The underscore (_) in this statement is a convention, mostly used by Python developers when the loop variable is not required or used. It signifies to another coder reviewing your code that you’re intentionally ignoring the variable.
To delve further into understanding the practical applications and examples of
xxxxxxxxxx
for _ in range()
, let’s first appreciate the functionality of
xxxxxxxxxx
range()
. This built-in Python function generates an immutable sequence of numbers from 0 (by default) up to (but not including) the number specified within its parentheses. If two parameters are provided,
xxxxxxxxxx
range()
operates from the first parameter up to (but not including) the second parameter.
For instance,
xxxxxxxxxx
range(5)
will produce: 0, 1, 2, 3, 4.
Whereas,
xxxxxxxxxx
range(1, 5)
will give: 1, 2, 3, 4.
Let us now highlight some key examples relating this construct to various scenarios:
1. Performing a Task Repeatedly
Assuming we need to print “Hello, World!” ten times consecutively but do not wish to waste time writing the same line of code ten times. Handily,
xxxxxxxxxx
for _ in range(10)
presents itself as a perfect and elegant solution:
xxxxxxxxxx
for _ in range(10):
print("Hello, World!")
This indicates to the computer to execute the print statement ten times, without intereset on each specific iteration number.
2. Initialising a List With Same Values
If there is need to initialise a list with the same value, such as creating a list of ten zeroes. Here’s how you could approach it:
xxxxxxxxxx
zeroes = [0 for _ in range(10)]
This builds a list where ‘0’ is repeated ten times.
Let’s remind ourselves once more, while the underscore (_) convention promotes cleaner coding practices given that it signals intentional disuse of the loop variable, you can substitute it with any other variable name if you end up needing access to the iterator value. You’ll find numerous instances where tracking iteration numbers is necessary. However, for cases like the ones above, making use of
xxxxxxxxxx
for _ in range()
results in neater, compact code — worthy of industry-grade Python.
Do read further on advanced Python loop control mechanisms on the official Python Documentation. Though understanding these basics will provide one a solid foundation to begin their coding journey.The ‘For _ in Range()’ syntax is a very important structure in Python, providing an efficient way to navigate through loops. This expression allows us to iterate over a sequence of numbers produced by the built-in function `range()`.
Here’s a basic example using it:
xxxxxxxxxx
for _ in range(5):
print("Hello, World!")
This will output “Hello, World!” five times. Here, `_` is behaving as a variable that changes with each iteration; however, we aren’t actually using this value within our loop. Using `_` is a convention followed to indicate that the variable is not going to be used.
But why use ‘For _ in Range()’ and what makes it so efficient? Let’s dive into this.
• Firstly, python’s `range()` function generates a sequence of numbers starting from 0 up to n-1 where ‘n’ is the number passed as argument. When combined with `for`, it provides a simple and clean way to repeat a code block for ‘n’ number of times without having to worry about incrementing the loop counter manually. Simply put:
xxxxxxxxxx
for _ in range(n):
# Code block
Here, the code block executes ‘n’ number of times.
• Secondly, when working with lists or essentially any iterable objects, `for _ in range()` proves to be particularly handy. It can provide an index-based approach to iterating over elements in a list which gives more control during iterations:
xxxxxxxxxx
items = ['apple', 'banana', 'cherry']
for i in range(len(items)):
print(f'Item {i+1}: {items[i]}')
• Another powerful advantage `for _ in range()` offers is its compatibility with conditions and nested loops, making it fit for a variety of use cases.
For further reading refer to Python Official Documentation on range.
In summary, whether you’re looking to repeat an operation multiple times, traverse a list in a controlled way, or work with complex nested loops and conditions, `for _ in range()` could just be your go-to tool in Python.The Python `for _ in range()` is a very common and powerful construct used in various applications to perform loop iterations over a set of integers. It is primarily used when you need to repeat a block of code a defined number of times. Here, the underscore `_` serves as an ignored variable, indicating that it doesn’t matter what the actual value is; we are just interested in doing something repetitively.
Consider a very simple example:
Index | Value |
0 | Python |
1 | Ruby |
2 | JavaScript |
3 | Java |
xxxxxxxxxx
languages = ['Python', 'Ruby', 'JavaScript', 'Java']
for _ in range(4):
print(languages[_])
In this example, the `range(4)` generates a set of integer values from 0 to 3 which indicates the indices of our list (`languages`). Our for-loop runs that many times and prints out each element in the array.
However, like any other piece of code, it isn’t immune to errors. They can happen during its execution and if not handled properly, they can halt or break your application. In this context, here are strategies to handle errors when using `for _ in range()`:
* **Try/Except Block**: One way to safeguard against potential errors is by using a try/except block within the looping structure. Common areas where you might want exception handling are accessing elements beyond a list’s scope or dealing with null or inappropriate data types.
xxxxxxxxxx
try:
for _ in range(len(someList)):
# Some operation here
except IndexError as e:
print(f"Index Error: {e}")
except TypeError as e:
print(f"Type Error: {e}")
# You can add as many exceptions as you wish
* **Validating Input Data**: You should make sure the data being queried or manipulated within the loop is valid. This applies to both the elements of your data and the range over which you’re trying to iterate. If required, you can include data checking and sanitization step before entering the loop.
xxxxxxxxxx
if isinstance(n, int) and n > 0:
for _ in range(n):
# operation involving _
else:
print("Invalid input, please provide a positive integer.")
* **Logging Errors**: When your script encounters an error, simply printing an error message and continuing might not be enough – this is where logging comes into play. By logging errors, you can preserve a record of them that can be invaluable when troubleshooting problems after-the-fact.
xxxxxxxxxx
import logging
logging.basicConfig(level=logging.ERROR)
try:
for _ in range(n):
# some operation involving _
except Exception as e:
logging.error(f"Exception occured: {e}")
By effectively employing these methods, you can build robust and resilient codes capable of standing against possible abnormalities and exceptions while using the dynamic `for _ in range()` construct in Python. It helps in better error-detection and noticeably increases the maintainability of your programming scripts.
While diving deep into Python, the concept of ‘for _ in range()’ is often encountered. Simply put, this construction illustrates one of Python’s key concepts – iteration. In the context of a loop, it provides an effortless way to perform a certain action a specified number of times.
In Python,
xxxxxxxxxx
for _ in range(n)
means executing a set of statements ‘n’ amount of times. The
xxxxxxxxxx
_
, known as a “throwaway” variable, signifies that while we know something is being looped over, we do not require the actual data from the list or range it is looping over. This syntax makes coding less cluttered and more readable.
Code | Description |
---|---|
xxxxxxxxxx 1 1 1 for _ in range(5): print("Hello") |
This code signifies that the print statement will be executed 5 times, printing “Hello” 5 times on the console. The “_” symbol in this instance stands for each iteration but is disregarded. |
xxxxxxxxxx 1 1 1 for i in range(5): print(i) |
Unlike the previous example, this time the variable “i” is used to carry the current iteration’s value. This code will output the numbers 0 through 4 on the console. |
The use of ‘range()’ can also introduce additional functionality into your loops. With the ability to specify start, end, and step values, you have increased control over how your program iterates. For example, when
xxxxxxxxxx
for _ in range(1, 10, 2)
is stipulated, it means executing a set of statements every other integer from 1 up to but not including 10.
Thoroughly understanding the power of ‘For _ in Range()’ in Python can significantly increase the performance and readability of your code. Known for its ease of learning and versatility, Python continues to offer solutions to diverse tasks – iterating in loops being just a small part of why Python’s popularity is ever-growing among developers.