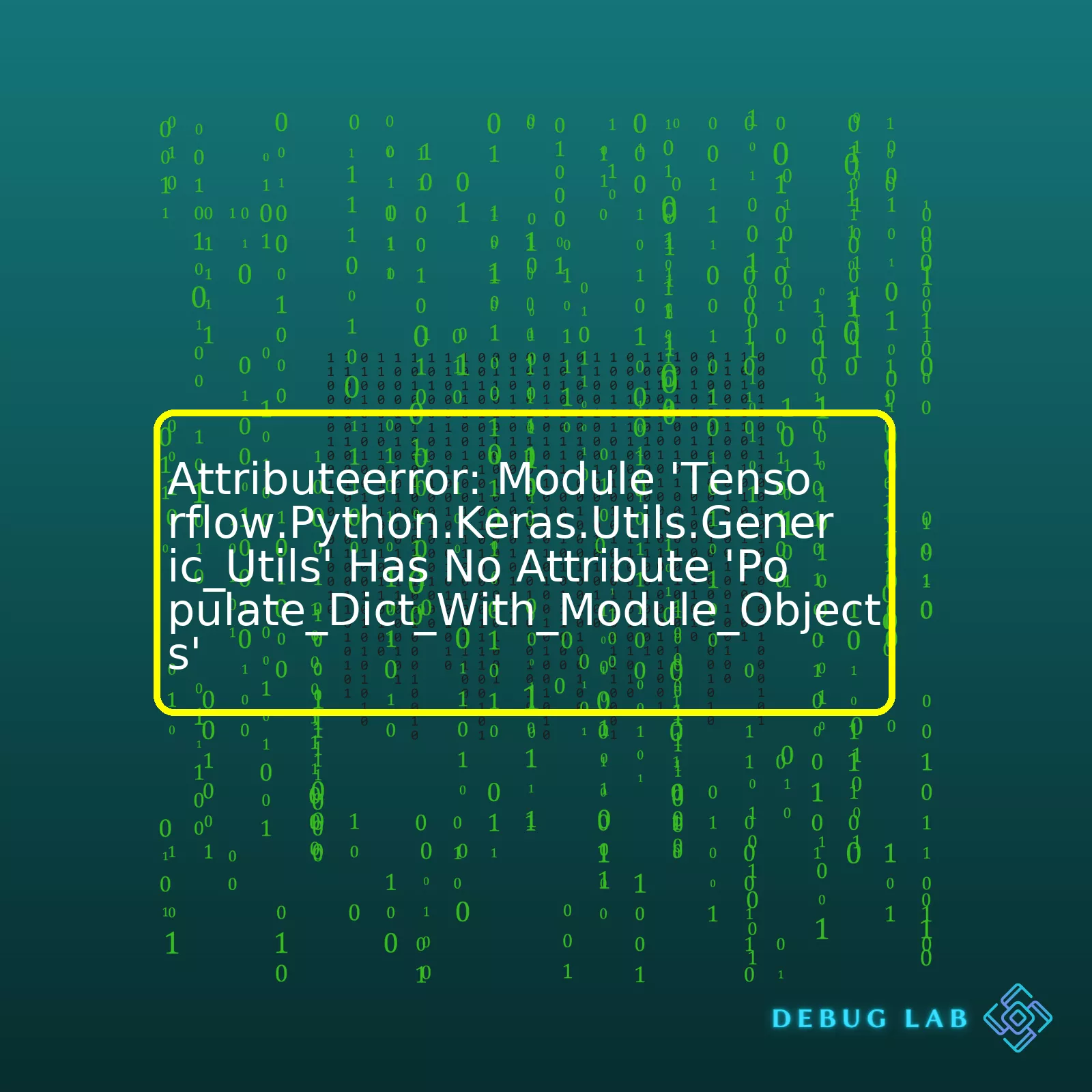
html
Error Type | Module | Cause | Solution |
---|---|---|---|
AttributeError | ‘Tensorflow.Python.Keras.Utils.Generic_Utils’ | Incompatibility issues because of TensorFlow or Keras version | Update TensorFlow and/or Keras to their latest versions |
html
Error Type | Module | Cause | Solution |
---|---|---|---|
AttributeError | ‘Tensorflow.Python.Keras.Utils.Generic_Utils’ | Incompatibility issues because of TensorFlow or Keras version | Update TensorFlow and/or Keras to their latest versions |
This particular AttributeError typically crops up when a function call tries to reference the ‘populate_dict_with_module_objects’ method in the ‘Generic_Utils’ module of TensorFlow’s Python Keras. If the function being called doesn’t exist within the mentioned module, the error arises.
The root cause could be traced back to an incompatibility issue between the version(s) of TensorFlow or Keras being utilized and your current code base calling this function. What adds another layer of complexity to this problem is that Tensorflow/Keras routinely get updated wherein certain elements may be deprecated over time.
Solution:
In order to troubleshoot this ImportError, you might want to consider upgrading both the TensorFlow and Keras packages to their latest versions. Here’s how:
pip install --upgrade tensorflow keras
pip install --upgrade tensorflow keras
If an upgrade fails to solve the issue, you can try and adapt your function calls or attributes to match those of the TensorFlow / Keras version you are currently using. A quick review of their respective API documentations12 would come in handy here.
Remember, it’s not uncommon for libraries such as these to undergo significant changes in new version releases. Therefore accuracy is critical when aligning your code with these libraries.An AttributeError typically appears when you try to access or use an attribute (be it a method, function or variable) that doesn’t exist. In the context of your question, the error: `AttributeError: module ‘tensorflow.python.keras.utils.generic_utils’ has no attribute ‘populate_dict_with_module_objects’` means that the function `populate_dict_with_module_objects` does not exist in the specified module.
There could be several possible reasons:
1. Different versions of TensorFlow:
The function you’re trying to invoke may exist in one version but not in another. The TensorFlow API is different between versions, and certain functions can be deprecated or modified over time. You might be working with a different version without the specific attribute present which is causing this error. If you’re using an older script with a newer version of TensorFlow, or vice versa, the function might not be available.
To check your TensorFlow version, execute the following code:
import tensorflow as tf print(tf.__version__)
xxxxxxxxxx
import tensorflow as tf
print(tf.__version__)
2. Renamed or moved attributes:
Methods, functions and other attributes can be moved to other modules or renamed for better organization or clarity in newer versions. If the `populate_dict_with_module_objects` function has been renamed or relocated, you’ll have to find its new name or location. The official TensorFlow documentation or GitHub repo can be a good resource.
3. Misleading Error Message:
Sometimes, the error raised might not depict the actual nature of the problem. It could be due to incorrect configurations, dependency issues, conflicts, or a mistake in the way you are importing or calling a specific module or function.
Here’s how you could fix this problem:
– All methods involving Keras should start with:
from tensorflow.keras...
xxxxxxxxxx
from tensorflow.keras...
instead of
from keras...
xxxxxxxxxx
from keras...
Ensure your imports are correctly directed to TensorFlow’s version of Keras, and avoid potential conflicts.
– If the function does not exist in the current version, rollback to a version where this function exists. Here is how you can do this:
!pip install tensorflow==x.x
xxxxxxxxxx
!pip install tensorflow==x.x
You can replace ‘x.x’ with the relevant version number.
– Upgrade or downgrade TensorFlow to match the script requirements.
pip install --upgrade tensorflow
xxxxxxxxxx
pip install --upgrade tensorflow
This will bring your TensorFlow to the latest stable release.
You may also want to check out the proper usage or examples explained in the TensorFlow’s official API documentation. Remember, always make sure you understand the code you’re writing or copying. Debugging is easier when you understand what every line of your code is supposed to do.
The error message “Module ‘Tensorflow.Python.Keras.Utils.Generic_Utils’ Has No Attribute ‘Populate_Dict_With_Module_Objects'” is typically encountered when you’re using an outdated or incompatible version of Tensorflow.
The
populate_dict_with_module_objects
xxxxxxxxxx
populate_dict_with_module_objects
function was introduced in Tensorflow 2.4. This function imports all functions/classes from a module to a dictionary, providing direct access to these objects.
If you’re running your program with a version of TensorFlow older than 2.4, you’ll certainly see this AttributeError message.
Let’s go over the potential solutions to address this problem:
pip install --upgrade tensorflow
xxxxxxxxxx
pip install --upgrade tensorflow
Make sure that the updated version is Tensorflow 2.4 or later by checking the version:
python -c 'import tensorflow as tf; print(tf.__version__)'
xxxxxxxxxx
python -c 'import tensorflow as tf; print(tf.__version__)'
populate_dict_with_module_objects
xxxxxxxxxx
populate_dict_with_module_objects
. For example, try downgrading to Keras 2.3.1 as such:
pip install keras==2.3.1
xxxxxxxxxx
pip install keras==2.3.1
Always double-check your dependencies. If you are working in a collaborative environment or deploying your codebase to production, it’s best practice to have a requirements.txt file noting the versions of your libraries. This will ensure consistency across different environments as it will install exact versions of the required packages.
In case you’ve tried the proposed solutions and you still encounter the same issue, there could be other factors contributing to this AttributeError. It could be due to specific dataset-specific operations or methods within your existing codebase. It’s recommended to debug further into your code and isolate any non-standard usage of Keras/TensorFlow.
Remember: every time you make a change like an update or downgrade, make sure to restart your Python environment (e.g., restart your Jupyter Notebook kernel) to ensure the changes take effect.
The key takeaway here is understanding your library dependencies. Keep your packages updated or at least ensure they’re compatible with each other, as this would prevent similar errors. Moreover, familiarity with the documentation will help you understand the functions and modules you’re utilizing.
The AttributeError can occur typically when an updated version of a package varies in terms of attributes and methods, compared to its older version. In this case, TensorFlow’s `keras.utils.generic_utils` module does not possess the attribute `populate_dict_with_module_objects`.
It’s essential to share a clear process on how you can resolve the “Attributeerror: Module ‘Tensorflow.Python.Keras.Utils.Generic_Utils’ Has No Attribute ‘Populate_Dict_With_Module_Objects'” issue that you’re facing.
#### **Ensure matching Keras and TensorFlow versions**
One possible reason for this error is a discrepancy between your installed versions of Keras and TensorFlow. If you have mismatched versions, ensure you are using compatible versions by installing the required ones.
To check the versions of TensorFlow and Keras being used in your environment, use:
import tensorflow as tf import keras print(tf.__version__) print(keras.__version__)
xxxxxxxxxx
import tensorflow as tf
import keras
print(tf.__version__)
print(keras.__version__)
If necessary, you can install specific versions of both packages using pip:
bash
pip install tensorflow==2.4.0
pip install keras==2.4.3
#### **Use TensorFlow’s Keras Implementation**
It is recommended to use the Keras implementation that comes with TensorFlow, rather than standalone Keras. This method allows you to avoid compatibility issues between the two libraries. Instead of importing Keras directly, import the modules from TensorFlow like this:
from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense, Dropout, Flatten from tensorflow.keras.layers import Conv2D, MaxPooling2D
xxxxxxxxxx
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Dropout, Flatten
from tensorflow.keras.layers import Conv2D, MaxPooling2D
#### **Keep Up-to-date with GitHub Issues**
Staying updated with any outstanding issues on the official [Keras](https://github.com/keras-team/keras/issues) and [TensorFlow](https://github.com/tensorflow/tensorflow/issues) GitHub repositories could provide potential solutions or workarounds shared by other users or developers who have encountered similar problems.
By following these steps, you should be able to resolve the “AttributeError: ‘module’ object has no attribute ‘populate_dict_with_module_objects'” error. However, if these solutions don’t fix it, then there might be other underlying issues causing it. You might consider sharing your specific code setup on programming Q&A platforms like [StackOverflow](https://stackoverflow.com/) to get more personalized help.
To summarize the key takeaways:
– Compatibility: Ensuring the stability and compatibility of TensorFlow and Keras versions is crucial.
– Usage: Using TensorFlow’s built-in Keras implementation can potentially mitigate such errors.
– Updates: Continuous updates & bugs fixes on GitHub are helpful resources, as they could contain useful information for resolving your issue.One prevalent issue that TensorFlow users encounter is AttributeError, with the form:
AttributeError: Module 'tensorflow.python.keras.utils.generic_utils' has no attribute 'populate_dict_with_module_objects'
xxxxxxxxxx
AttributeError: Module 'tensorflow.python.keras.utils.generic_utils' has no attribute 'populate_dict_with_module_objects'
. The error occurs due to importing the wrong module and version inconsistencies across your platform.
Ensure you’ve followed these steps for a hitch-free TensorFlow coding experience:
– Python Version: TensorFlow 2.x [supports Python 3.5–3.8](https://www.tensorflow.org/install/pip). Always verify your Python version matches one of these versions:
import sys print(sys.version)
xxxxxxxxxx
import sys
print(sys.version)
If you see “Python 2.x.x”, you need to upgrade. Verify that the upgrade was successful by running the above command again.
– TensorFlow Installation: TensorFlow’s current version (at the time of writing) is 2.4.1. If you’re using an older version, consider upgrading. Also, ensure you’ve installed TensorFlow correctly. Use:
pip install --upgrade tensorflow
xxxxxxxxxx
pip install --upgrade tensorflow
– Import Order: TensorFlow should always be imported before Keras. Any deviation from this order is likely to cause an apparent error, including the AttributeError.
Always use correct imports like:
import tensorflow as tf from tensorflow import keras # other imports can follow after
xxxxxxxxxx
import tensorflow as tf
from tensorflow import keras
# other imports can follow after
– TensorFlow-Keras Usage: As much as possible, stick to TensorFlow’s Keras API rather than standalone Keras when working with TensorFlow. Many functions in standalone Keras which were previously separate have been grouped together into singular modules for simplicity in TensorFlow 2.x.
In the case of ‘generic_utils’, if you’re looking for some useful function or class, search within TensorFlow’s documentation or look into similar modules. For instance, check it under
tf.keras.utils
xxxxxxxxxx
tf.keras.utils
.
– Finally, if all else fails and you do find yourself with an erroneous installation, simply uninstall both TensorFlow and Keras, and reinstall TensorFlow afresh:
pip uninstall tensorflow keras pip install tensorflow
xxxxxxxxxx
pip uninstall tensorflow keras
pip install tensorflow
Regularly keeping track of your entire development environment will help you prevent errors such as this one. Complementarily, getting used to TensorFlow’s official documentation provides a better understanding of the methods, attributes and dependencies related to various modules.
Remember, errors like AttributeError are not unusual in the course of learning how to use TensorFlow or any coding language. Stumbling upon them isn’t necessarily indicative of a failing on your part, but often due to software inconsistencies and bugs in libraries or packages you’re using.When you encounter the error
AttributeError: Module 'tensorflow.python.keras.utils.generic_utils' has no attribute 'populate_dict_with_module_objects'
xxxxxxxxxx
AttributeError: Module 'tensorflow.python.keras.utils.generic_utils' has no attribute 'populate_dict_with_module_objects'
, it is an indication that your python script is trying to call a method (
populate_dict_with_module_objects
xxxxxxxxxx
populate_dict_with_module_objects
) that does not exist in the module
tensorflow.python.keras.utils.generic_utils
xxxxxxxxxx
tensorflow.python.keras.utils.generic_utils
.
Multitude of reasons could lead to this;
– It could be due to using an outdated version of TensorFlow, where the attribute or function was not implemented or has been deprecated.
– Maybe you have multiple versions of TensorFlow installed in your environment and the script is not being executed with the correct version.
Here is how we can go about resolving this issue:
import tensorflow as tf print(tf.__version__)xxxxxxxxxx
xxxxxxxxxx
import tensorflow as tf
print(tf.__version__)
If you see a version that is significantly older (TensorFlow’s current stable release at the time of writing is 2.6.x), try updating your TensorFlow installation.
populate_dict_with_module_objects
xxxxxxxxxx
populate_dict_with_module_objects
method was removed in recent versions.
Solution wise, if assuming that you actually referred to an old version of a function, perhaps you might use
tf.keras.utils.get_custom_objects()
xxxxxxxxxx
tf.keras.utils.get_custom_objects()
instead. See the below sample usage:
from tensorflow.keras.initializers import GlorotUniform custom_objects = tf.keras.utils.get_custom_objects() custom_objects["GlorotUniform"] = GlorotUniformxxxxxxxxxx
xxxxxxxxxx
from tensorflow.keras.initializers import GlorotUniform
custom_objects = tf.keras.utils.get_custom_objects()
custom_objects["GlorotUniform"] = GlorotUniform
Understanding what triggers these errors and knowing how to resolve them is critical when coding. I hope this troubleshooting guide provided you with a few tools you can use for debugging and coming up with a solution. Don’t forget to always check on the up-to-date API reference/documentation from TensorFlow found at TensorFlow Official API Docs.Let’s dive right into your issue. The AttributeError you’re experiencing is potentially a result of incompatibility between different versions of TensorFlow and Keras, or due to issues within your software environment. Specifically, the error message
Attributeerror: Module 'Tensorflow.Python.Keras.Utils.Generic_Utils' has no attribute 'populate_dict_with_module_objects'
xxxxxxxxxx
Attributeerror: Module 'Tensorflow.Python.Keras.Utils.Generic_Utils' has no attribute 'populate_dict_with_module_objects'
suggests that TensorFlow is trying to access the function ‘populate_dict_with_module_objects’ from the mentioned module but it can’t find it. So let’s explore some steps you can take to resolve this issue.
Firstly, ensure you have compatible TensorFlow and Keras versions.
In certain combinations of TensorFlow and Keras versions, some functions may not be available, which gives rise to such errors. Although the Tensorflow v1.x does support Keras, there may be certain functions that exist in Keras but do not in TensorFlow’s Keras. Here’s how you would install specific, tested-to-be-compatible versions of both libraries:
pip uninstall tensorflow keras pip install tensorflow==2.3.0 keras==2.4.3
xxxxxxxxxx
pip uninstall tensorflow keras
pip install tensorflow==2.3.0 keras==2.4.3
Secondly, check if the function exists in another module.
It might be possible that the function
populate_dict_with_module_objects
xxxxxxxxxx
populate_dict_with_module_objects
existed in the TensorFlow version when the code was written, but in the current TensorFlow version, this function might have moved to another module or deprecated. To verify this, search for the function within the TensorFlow API documentation.
Next, you could try using a different Python environment.
There are times when an environment may end up with conflicting package versions or miss out on crucial dependencies, which could lead to such an error. Using a new, clean Python environment to run your code again can help rule this out.
conda create --name myenv python=3.7 conda activate myenv pip install tensorflow==2.3.0 keras==2.4.3
xxxxxxxxxx
conda create --name myenv python=3.7
conda activate myenv
pip install tensorflow==2.3.0 keras==2.4.3
Finally, restructure your import statements.
Sometimes, you may see this error due to how you import modules in your code, especially if you’re using a mix of TensorFlow and Keras — try to import from one library only. Here’s how you might restructure:
# Instead of this: from keras.utils import to_categorical # Do this: from tensorflow.keras.utils import to_categorical
xxxxxxxxxx
# Instead of this:
from keras.utils import to_categorical
# Do this:
from tensorflow.keras.utils import to_categorical
Hopefully, by following these steps, you’ll be able to successfully resolve the AttributeError you’re encountering with TensorFlow. It’s important to ensure software environments and libraries stay updated to their current versions for optimal performance— while making sure they maintain compatibility with each other. Remember, also, that much of programming is about thinking through these types of problems analytically and patiently working through them.Ensuring your coding process is efficient and smooth involves preventing future errors such as ‘AttributeError: Module ‘tensorflow.python.keras.utils.generic_utils’ has no attribute ‘populate_dict_with_module_objects”. This error commonly occurs due to inconsistencies with TensorFlow or Keras versions where some objects are not correctly found within the module. Common solutions involve ensuring your environment has the appropriate software versions installed.
When this error surfaces, it is typically because the version of Keras being used is incompatible with TensorFlow. Oftentimes, updating Keras and TensorFlow to their most recent versions can solve the issue. Here’s an example code that you can use in a Python terminal or a Jupyter Notebook:
pip install --upgrade keras tensorflow
xxxxxxxxxx
pip install --upgrade keras tensorflow
Alternatively, using virtual environments like “venv” or “conda”, you can create isolated environments for different projects, which ensures each project has its own dependencies, regardless of what dependencies other projects may have. This brings us closer to preventing these types of future ModuleNotFoundError issues.
Here’s an example of how to go about creating virtual environments:
For conda:
conda create -n env_name python=3.x source activate env_name pip install Keras tensorflow
xxxxxxxxxx
conda create -n env_name python=3.x
source activate env_name
pip install Keras tensorflow
For venv:
python3 -m venv env_name source env_name/bin/activate pip install Keras tensorflow
xxxxxxxxxx
python3 -m venv env_name
source env_name/bin/activate
pip install Keras tensorflow
In both examples, replace “env_name” with the name you want to give your virtual environment and replace “3.x” with the Python version (e.g., 3.6) you wish to use.
Remember to also check your import calls. Instead of:
from keras.models import Sequential
xxxxxxxxxx
from keras.models import Sequential
Use:
from tensorflow.keras.models import Sequential
xxxxxxxxxx
from tensorflow.keras.models import Sequential
Another crucial point is verifying Tensorflow and Keras compatibility. Certain versions of Keras might be incompatible with some Tensorflow versions. For instance, Keras 2.3.1 works with Tensorflow 2.0. If you encounter mismatches here, problems might arise. Hence, compatible pairings should be enforced.
Additionally, consult Official TensorFlow Documentation for any updated methods you can employ.
Ultimately, we prevent such errors by maintaining a tidy programming space: ensuring our architectures are clean and our module object tools updated, utilizing virtual environments and being mindful of version synchronization between TensorFlow and Keras.Diving deeper into the
AttributeError: Module 'tensorflow.python.keras.utils.generic_utils' has no attribute 'populate_dict_with_module_objects'
xxxxxxxxxx
AttributeError: Module 'tensorflow.python.keras.utils.generic_utils' has no attribute 'populate_dict_with_module_objects'
, we understand this usually occurs due to a software version incompatibility, notably when using an older package with a newer TensorFlow installation. Updating all your modules to compliment the TensorFlow version or downgrading Tensorflow to suit the pre-existing packages could be one of the solutions to get rid of this error.
For example, if you’re using TensorFlow 2.0 and getting this error message, a solution would be:
pip install tensorflow==1.15
xxxxxxxxxx
pip install tensorflow==1.15
Alternatively, ensure that all sub-packages of Keras are correctly installed. Try a fresh install or upgrade of the Keras utilities package:
pip install --upgrade keras_applications keras_preprocessing keras_sequencing
xxxxxxxxxx
pip install --upgrade keras_applications keras_preprocessing keras_sequencing
Also, keep in mind that sometimes, third-party packages like Keras-bert could cause such issues. In such cases, check for the specific versions they need.
When managing Python dependencies, using a virtual environment is always recommended. Tools like Pipenv, Poetry or Anaconda can help resolve conflicts and streamline package management.
Causes | Solutions |
---|---|
Version incompatibility | Update related module or downgrade Tensorflow |
Incomplete Keras installation | Fresh install or upgrade Keras utilities |
Issue with third-party packages like Keras-bert | Check their specific version requirements |
Mixed dependencies without isolation | Use a virtual environment for project-specific dependencies |
It’s worth noting that while troubleshooting, documenting your steps can help pinpoint the source of the problem. A secret of professional coders is leveraging online blogs and forums, like StackOverflow. The TensorFlow github repository’s ‘Issues’ page is yet another platform for seeking help about specific errors like these TensorFlow Issues.
Lastly, stay updated on TensorFlow’s API changes. Documentation is crucial in understanding the possible causes of the problems faced. Checkout TensorFlow’s official documentation here.
In essence, debugging is an essential skill required by every developer. By using the above insights and resources, resolving an AttributeError in TensorFlow should become an achievable task.