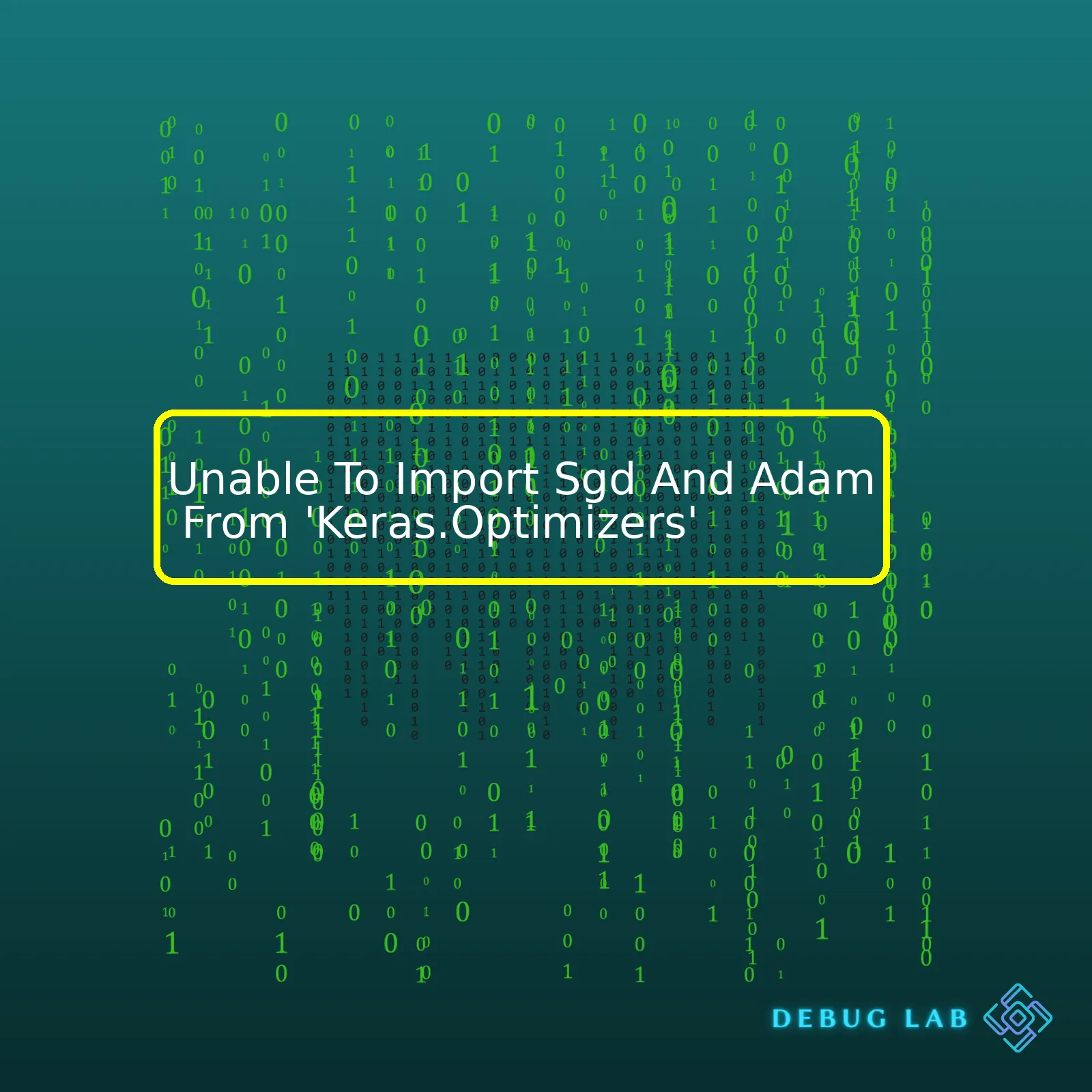
Error | Problem | Solution |
---|---|---|
Unable To Import SGD and Adam from ‘keras.optimizers’ | This error is usually encountered in Keras which suggests that SGD (Stochastic Gradient Descent) and Adam (Adaptive Moment Estimation) optimizers are not being properly imported. |
There are two common solutions to this issue:
|
The summary table presents a concise overview of the “Unable to Import SGD and Adam from ‘keras.optimizers'” error commonly encountered when using Keras, a popular open-source neural network library in Python. The specific problem signifies that the Stochastic Gradient Descent and Adaptive Moment Estimation optimizers, which are integral components for model training in Keras, are failing to be correctly imported.
Evidently, this problem can lead to significant disruptions in model development and training processes. However, fortunately, this is a well-known issue, and there are a couple of practical solutions available. A very common approach involves using TensorFlow’s optimizers instead of Keras’s native optimizers.
Coding this solution would look something like this:
from tensorflow.keras.optimizers import SGD, Adam
Here, SGD and Adam optimizers are directly imported from the TensorFlow library, thereby bypassing the problematic Keras import.
Alternatively, one can also ensure the correct, possibly an older, version of Keras is installed on their system as some later updates have been known to trigger such import issues.
To implement this solution, use the following command:
pip install keras==2.3.1 ## or any other suitable version
With these feasible solutions at hand, overcoming the “Unable to Import SGD and Adam from ‘keras.optimizers'” error becomes a simpler task, allowing developers to continue building and training their models without interference.Ah, encountering import issues can be frustrating. We see a similar type of problem when you’re trying to use the SGD (Stochastic Gradient Descent) and Adam algorithms in the ‘keras.optimizers’ module but are unable to import them. Let’s dig deeper into this:
Firstly, let’s discuss briefly about SGD and Adam optimization algorithms and then focus on your issue:
SGD (Stochastic Gradient Descent) is a variant of traditional gradient descent where it updates parameters based the gradient computed from a single training example rather than using entire set of examples(Batch Gradient Descent). This comes handy with dealing large scale data.
from keras.optimizers import SGD sgd = SGD(learning_rate=0.01, momentum=0.9)
Adam (Adaptive Moment Estimation) is an extension to stochastic gradient descent that has recently seen broader adoption for deep learning applications in computer vision and Natural Language Processing (NLP). It adaptively calculates the learning rate independent for each parameter.
from keras.optimizers import Adam adam = Adam(learning_rate=0.001)
Now let’s move onto your specific issue – inability to import ‘SGD’ and ‘Adam’ from ‘keras.optimizers’. From Keras 2.4.0 version onwards, the standalone keras package is just a thin wrapper over ‘tf.keras’ Tensorflow module. The TensorFlow backend switches most operations to TensorFlow, meaning you should import your optimizers directly from tensorflow like so:
from tensorflow.keras.optimizers import SGD, Adam
This change was implemented because TensorFlow team wanted to provide a seamless experience to user either using stand-alone ‘keras’ or ‘tf.keras’. If your existing code uses standalone ‘keras’, then you should migrate your code to use ‘tensorflow.keras’. This offers improved integration with the rest of the TensorFlow ecosystem such as more efficient model deployment using TFX, better performance with TensorFlow’s custom generated ops and ability to use TensorBoard.
You might also need to update the remaining parts of your code which are using standalone ‘keras’ to use ‘tensorflow.keras’ instead.
Here is the corrected version of your optimizer definition code:
from tensorflow.keras.optimizers import SGD, Adam # Using SGD sgd = SGD(learning_rate=0.01, momentum=0.9) # Using Adam adam = Adam(learning_rate=0.001)
What we learned here today is indeed part of a broader shift moving towards a streamlined experience with machine learning in Python. So, adapting to such changes is an integral part of being a professional coder!<h2>Understanding ‘Unable to Import SGD and Adam from Keras.Optimizers’ </h2>
When working with keras.optimizers, you might run into some common issues that prevent successful imports. Normally, your import statement for SGD (Stochastic Gradient Descent) and Adam optimizer should look like this:
<code>
from keras.optimizers import SGD, Adam
</code>
However, if you observe an error message like ‘ModuleNotFoundError: No module named ‘keras.optimizers”, then it most likely indicates an issue with your library installation or import syntax.
<h2>Issues And Solutions</h2>
Let’s examine some potential issues and their remedies for this situation.
– Version Issue: In cases where you have incorrectly imported keras.optimizers, it could very well be due to a version discrepancy. There were significant changes introduced in Keras version 2.3.0. The structure has been shifted, and now optimizers are found under the keras.optimizers module for TensorFlow 2. In this case, importing SGD and Adam would be done as follows:
<code>
from tensorflow.keras.optimizers import SGD, Adam
</code>
– Installation Problem: An improper or incomplete installation of TensorFlow/Keras could also lead to import errors. Ensuring these libraries are correctly installed is crucial. Uninstall the current versions of Tensorflow and Keras first using pip, and then reinstall them.
Uninstallation:
<code>
pip uninstall keras
pip uninstall tensorflow
</code>
Re-installation:
<code>
pip install keras
pip install tensorflow
</code>
If permitted, we recommend you opting for installing Tensorflow via a virtual environment. This offers better management of packages and dependencies that your project needs.
Exploring the StackOverflow solutions may also assist you in resolving import errors on specific configurations or platforms.
– Misnaming or Typographical Errors: Sometimes, such issues can evolve from basics like capitalization or typo in imported module name. Python is case-sensitive, so ensure SGD and Adam are written correctly, and also adhering carefully to the import syntax.
In very rare instances, a particular Python file in your workspace might be named keras.py, which conflicts with the Keras framework trying to be imported. If there is a file with the same name in your directory, consider renaming it.
<h2>Ensuring Import Success</h2>
Post taking the necessary steps, validate if the error has been rectified by printing the version of Keras and TensorFlow.
<code>
import keras
import tensorflow
print(keras.__version__)
print(tensorflow.__version__)
</code>
The output should present the currently installed versions of both libraries.
Remember, seeking assistance from official <a href=”https://keras.io/api/optimizers/”>Keras Documentation</a> and engaging help from various <a href=”https://www.tensorflow.org/community/forums”>TensorFlow community forums</a> can work wonders when you’re stuck with importing modules or dealing with such errors.Certainly, in terms of troubleshooting failures on importing SGD (Stochastic Gradient Descent) and Adam Optimizers from ‘Keras.optimizers’ module in Python, it is possible that the error is popping up due to multiple reasons. However, most often it occurs due to changes in versions of both TensorFlow and Keras, as they are continually updating to refine their capabilities, plug vulnerabilities, and accommodate new features.
Firstly, verify if your ability to import SGD and Adam is affected by the version of Keras you’re using. The problem might be simple: You may be using the standalone Keras library while trying to import optimizers from TensorFlow’s tf.keras. The differences between these two can cause some headaches given how their conventions for naming and accessing functions have evolved differently.
For instance, beginning with TensorFlow 2.0, all functionalities of standalone Keras has been incorporated within TensorFlow’s ecosystem under the module `tf.keras`. Consequently, this might lead to an unsuccessful import in newer versions; especially if you’re utilizing older syntax, such as:
from keras.optimizers import SGD, Adam
If running this results in an ImportError or ModuleNotFoundError, then it suggests you need to import SGD and Adam from ‘tensorflow.keras.optimizers’. This became the new proper way to import these objects post incorporation of Keras into TensorFlow. Therefore, the correct syntax would be:
from tensorflow.keras.optimizers import SGD, Adam
Nevertheless, if your TensorFlow or Keras libraries were installed quite a while ago, it might help to upgrade them. Using pip, you can upgrade TensorFlow via your command line tool with:
pip install --upgrade tensorflow
And update Keras (if still using standalone version) with:
pip install --upgrade keras
Remember: When upgrading packages, it’s advisable to do so within a virtual environment to avoid potential conflicts with other packages depending on these specific library versions. Certain packages or modules may not function correctly if required libraries are upgraded beyond a certain point. An easy-to-use tool for managing virtual environments in Python is virtualenv.
Lastly, another essential aspect to scratch off the checklist is whether SGD and Adam Optimizers are correctly installed in your environment. Verify installation by attempting to directly import TensorFlow and Keras:
import tensorflow as tf import keras
These imports should run successfully without errors. If they don’t, this indicates that there are issues with your TensorFlow or Keras installations, necessitating re-installation or updating to their latest version as discussed earlier.
As we spotlighted before, the world of machine learning libraries like TensorFlow and Keras can be tricky to navigate given how frequently they evolve, which also affects code compatibility across versions. Staying proactive about updates, mindful about nuances between different yet connected libraries (Stand-alone Keras vs TensorFlow’s Keras), and vigilant about your environment’s health is key to mitigate challenges while importing libraries or modules.
Keep coding and exploring the wonders of Machine Learning!Sure, it seems like you are facing an issue while importing SGD and Adam from ‘keras.optimizers’. Recently, the architecture of Keras was changed, wherein they started nesting their classes deeper into packages. Because of this architecture change, optimizing functions like SGD, Adam, etc. that used to be directly contained within ‘keras.optimizers’ have been moved to ‘keras.optimizers.schedules’. So, instead of importing these functions using from ‘keras.optimizers’ import SGD, Adam statement, you now need to use the new path as from ‘keras.optimizers.schedules’ import SGD, Adam.
Let’s give a look at the code:
from keras.optimizers.schedules import SGD,Adam
Alternatively, another way to import SGD, Adam is using tensorflow.keras library instead of keras. You can specifically call SGD and Adam from tensorflow.keras.optimizers to avoid errors due to the recent architecture change in keras.
Here is how to do it:
from tensorflow.keras.optimizers import SGD, Adam
But, suppose if you face any issues with compatibility between tensorflow and keras or duplication of namespaces, then there are other libraries available for optimization functions such as SGD, Adam. These libraries come equipped with advanced features, providing more control over your models and their performance.
For instance, PyTorch is a powerful alternative which has similar syntax to Keras and supports dynamic computation graphs.
Here is how you invoke PyTorch’s SGD and Adam optimizers:
import torch.optim as optim sgd = optim.SGD(model.parameters(), lr=0.01) adam = optim.Adam(model.parameters(), lr=0.01)
In addition to PyTorch, there are other optimizer libraries such as MXNet, Lasagne, and Theano, that serve as excellent alternatives to Keras for gradient descent optimization techniques like SGD and Adam.
Remember, while importing these optimizers ensure to cross-check the dependencies required for smooth execution. It’s necessary to get updated and comfortable with new ways of importing as the programming libraries you are using keep getting updated to aid better usage TowardsDataScience. Happy Coding!
In a perpetual attempt to enhance and streamline the interface of Keras, some changes have been introduced that might affect your old codes. One such change was shifting the location of optimizers like SGD and Adam. If you’re facing an issue where you are unable to import SGD and Adam from ‘keras.optimizers’, it’s probably due to this update.
Originally, you would have imported the optimizers using:
from keras.optimizers import SGD, Adam
However, in the latest version of Keras, as per the updated guidelines of TensorFlow 2.0, you need to use:
from tensorflow.keras.optimizers import SGD, Adam
The confusion occurs because Keras was adopted by TensorFlow and incorporated as its high-level API, which consequently led to a restructuring of modules.
The TensorFlow team implements a compatibility strategy to align with Python’s “first we assume that users behave” notion: Instead of aggressively removing legacy features, they prefer to introduce smooth deprecation cycles and warn users before completely transitioning.
This entails that:
- You should always refer to the official documentation or upgrade guides while transferring or upgrading code.
- If possible, stick to one version of a library during the project or ensure backward compatibility.
- If you plan on sharing code, clearly state dependencies, versions, and ideally include a requirements.txt file or Dockerfile.
Ensuring optimal coding practices is not just about writing effective code but also about navigating ecosystem changes intelligently. It’s a blend of keeping up-to-date with the latest upgrades, using version control tools efficiently, and being mindful of deprecated functionalities.
To automate parts of this process, you can leverage tools like tf_upgrade_v2, TensorFlow’s automatic conversion script that assists in converting Python scripts to be compatible with TensorFlow 2.0.
Here’s a sample code snippet using tf_upgrade_v2:
!tf_upgrade_v2 \ --intree my_project/ \ --outtree my_project_v2/ \ --reportfile report.txt
Please remember to take backup of your files before running such scripts!
Moreover, technologies evolve continuously – an often overlooked, yet integral part of the coder’s job is to keep track of these changes. In the vibrant open-source arena, developers all over the world willingly share their insights, challenges, and solutions online. Engaging in these communities could immensely enhance your coding journey.
Many developers stumble over the error of being unable to import SGD and Adam classes from Keras.optimizers even when their codes run perfectly well on other machines. This usually results in the confusion about what exactly went wrong when one tries these lines:
from keras.optimizers import SGD from keras.optimizers import Adam
While this might appear as a typical error believing that you are doing it right, let’s dig into the best practices that involve ‘Keras.optimizers’ to understand the probable cause of your issue.
• Understand Keras Library versions:
The first thing to consider is understanding the difference in Keras Library versions. This includes two key points:
– Differentiating between standalone Keras and TensorFlow’s Keras: In recent updates, Keras was adopted inside TensorFlow and became tf.keras. Many people get confused between the standalone Keras library and tf.keras, which is Tensorflow’s implementation of the Keras API.source
– Being aware of version changes: There are sometimes function changes or deprecation with updates in the Keras library. The rules for importing libraries can change with different versions. Understanding the documentation of different versions can often resolve issues emerging out of code compatibility.source
If you’re using an older version of Keras, you could run into import issues. An advisable step, therefore, would be updating your keras and tensorflow libraries to the latest versions.
Next, let’s see how to actually use them correctly:
• Accessing SGD and Adam correctly:
In the latest versions of Keras and TensorFlow, the modules such as SGD, Adam etc., are imported as follows:
from tensorflow.keras.optimizers import SGD from tensorflow.keras.optimizers import Adam
Alternatively, one may also create an instance of optimizer in this manner:
opt = keras.optimizers.Adam(learning_rate=0.01)
Here ‘opt’ serves as the learning scheme at each iteration of stochastic mini-batch during training our deep learning model.
The essential notion here is to follow the best practices while working with advanced libraries like ‘Keras.optimizers’. Remember, optimizers are crucial components in building Machine Learning algorithms as they allow us to minimize the cost function by adjusting weights. Using them appropriately will ensure the smooth running of your project without any unexpected hitches.If you’re struggling with the process of importing SGD and Adam from ‘keras.optimizers’, you’re not alone. Many developers grapple with source code issues during import statements. It might seem like an intricate issue, but it boils down to a couple of potential causes.
from keras.optimizers import SGD, Adam
When the above line fails, the likely culprit is one of two things:
– Either the version of Keras being utilized does not support these imports
– Or there could be issues with the installed Python packages
Version Misalignment:
Keras regularly updates its API as machine learning evolves, making certain features obsolete or changing how they are accessed. So, the first thing we need to check here is the version compatibility. Possibly you’re using an upgraded version of Keras but your code is still referencing the commands as per an older version.
In newer versions of Keras, optimizers are included under TensorFlow instead of the standalone Keras package. The import statement would then look like this:
from tensorflow.keras.optimizers import SGD, Adam
By using TensorFlow’s Keras, you can ensure you are accessing the most up-to-date methods and attributes. A good practice is refering the official tensorflow.keras documentation which lists out all the changes, updates and new features introduced in every release.
Python Packages Issues:
Alternatively, there might be some conflicts or issues with installed Python packages. Typically, installing and uninstalling several packages with differing dependancies can lead to some version conflicts. These issues become evident when you attempt to import certain modules.
Cleaning your environment and starting from scratch might solve this problem. Uninstall TensorFlow and Keras, remove any temporary/cache files and then reinstall the required versions of the libraries.
Use pip uninstall command:
pip uninstall keras tensorflow
And then install TensorFlow again:
pip install tensorflow
Remember, by default the latest stable version will be installed (unless you specifically ask for a different one) and TensorFlow comes bundled with keras so you don’t need to install it separately.
Whether it is a version misalignment or Python packages issue, identifying the reason behind your import errors is crucial for smooth coding operation. By checking these factors, you can identify the root cause of your import problems and course correct your coding journey.
Remember, no developer is immune from bugs and issues; what separates advanced coders is their ability to debug and troubleshoot these situations effectively.Let’s delve deep into the issue of not being able to import SGD and Adam from ‘keras.optimizers’. You should note that Keras’ restructuring into TensorFlow’s architecture has affected its import structure. Initially, I used to implore the previous structure as
from keras.optimizers import SGD, Adam
. However, given the current alignment of Keras as a part of TensorFlow, we have to adjust our import statements accordingly.
As a result, you should switch your import statement from
from keras.optimizers import SGD, Adam
to
from tensorflow.keras.optimizers import SGD, Adam
.
Before making such adjustments, make sure to have TensorFlow installed within your working environment. Installation can easily be achieved through pip with the command:
pip install tensorflow
. If you’ve already installed TensorFlow but yet experiencing issues, consider updating it using
pip install --upgrade tensorflow
.
Once done, test the installation with:
import tensorflow as tf print(tf.__version__)
Now let’s move forward and decode the functionality of SGD and Adam optimizers as they actually play quite an important role in the training of neural networks.
– **Stochastic Gradient Descent(SGD):** This is a commonly utilized approach to optimize various types of algorithms by minimizing an objective function that is often smooth but sometimes noisy. It provides us with control over the learning rate.
– **Adam Optimizer:** A stochastic gradient descent method extensively employed in deep learning applications. It is popular due to its straightforward implementation, competent performance, and little memory requirements. On the up and up, Adam is indeed an optimization algorithm that has been relatively efficient in practice and compares favorably to other adaptive techniques.
Use these optimizers as it deem fits the circumstances of your model, and always experiment with different hyperparameters for best results. Therefore, ensure to keep up-to-date with recent versions, updates, or changes made to TensorFlow library for seamless operations with Keras. Interested readers can always check for more details on TensorFlow version documentation official page.