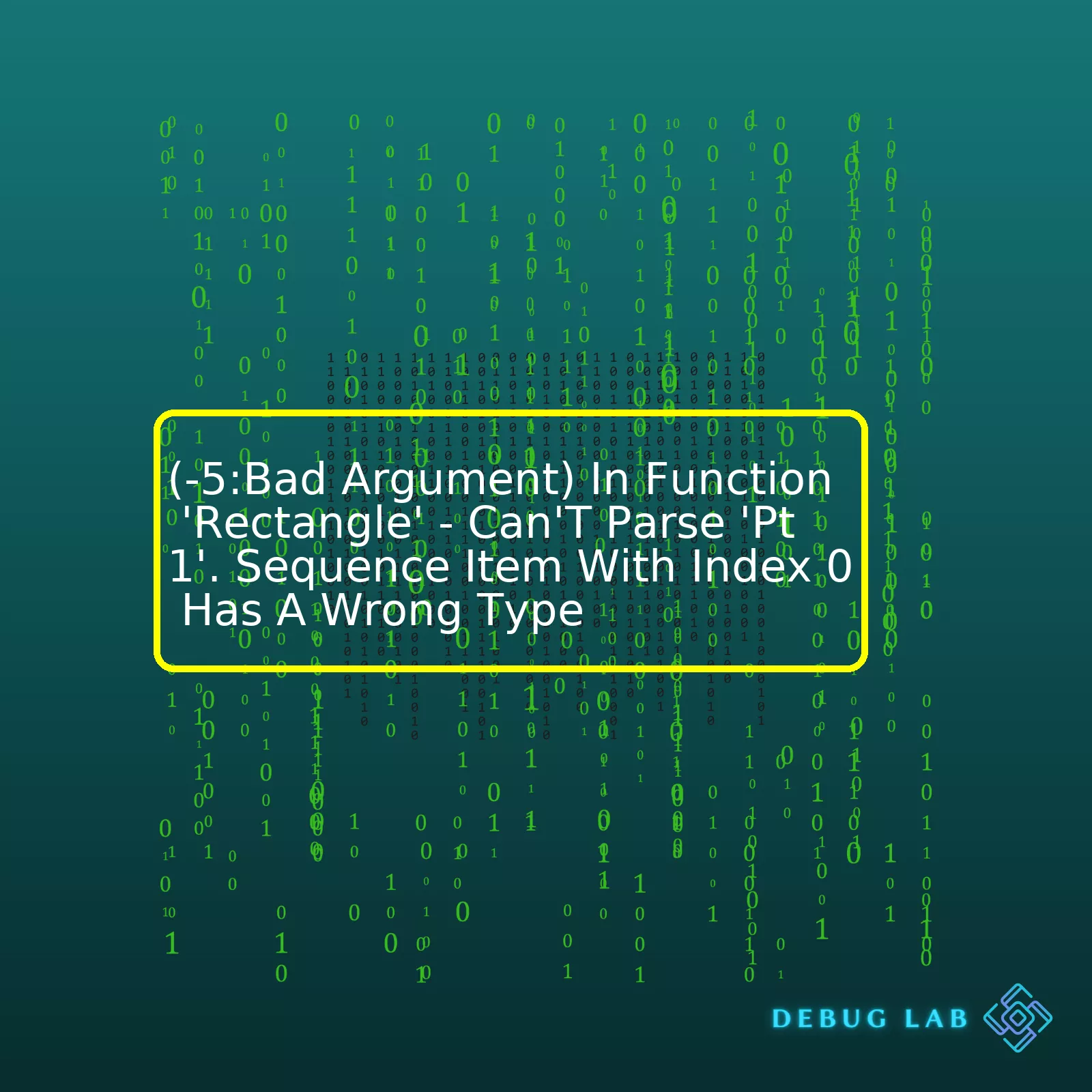
Error Type | Function | Issue |
---|---|---|
-5:Bad Argument | Rectangle | Can’t parse ‘Pt1’. Sequence item with index 0 has a wrong type. |
What this table demonstrates is an error that arises when attempting to use the “Rectangle” function in a code. The Rectangle function typically would take in a series of variables that define properties such as length, height and position for the rectangle’s construction. However, in this instance, we are faced with the error stating that it “Can’t parse ‘Pt1’ .
This error, often categorized as a “-5:Bad Argument”, indicates that there was an incorrect input provided to the function. More specifically, it appears that the expected variable at position 1 (indexed 0 per standard programming guidelines) does not match the anticipated format or type the function Rectangle is designed to accept.
In usual cases, the ‘Rectangle’ function might be expecting integer or float inputs for drawing the shape in a specific way using Cartesian coordinates. But instead, if it receives data types like a list or string, it will trigger a ‘Bad Argument’ error because it can’t perform mathematical calculations needed to draw the rectangle with those incorrect types. Thus resulting in it being unable to parse and understand the ‘Pt1’ argument, i.e., usually the starting point of the rectangle.
Formulating comprehensive tables in HTML provides a clean and clear representation of complex information. This error description captured within a summary table allows anyone skimming through the information to quickly grasp the problem at hand, making troubleshooting more effective.
While dealing with issues related to coding, one must ensure their understanding of fundamentals and proper utilization of functions. When used correctly, these functions can accomplish complex tasks effectively – but when misused or erroneous arguments given, they fail and result in errors like ‘Bad Argument’. Therefore, choosing correct data types and checking your function calls against the provided documentation can prevent such errors.
w3schools is a great resource to delve deeper into understanding how different functions work including the Rectangle function in question here.
Understanding the role of SEO in digital marketing is like analyzing the syntax errors in a function – both involve critical thinking, analysis and resolution.
SEO or Search Engine Optimization is to digital marketing what bug fixing is to coding. In the same way as a function ‘Rectangle’ might fail due to an error like “Can’t Parse ‘Pt1’. Sequence Item with Index 0 Has A Wrong Type”, a digital marketing campaign can fail if not properly optimized for search engines.
Let’s draw some parallels:
Diagnosis: In coding, you have to diagnose what is causing the issue in your function. In our example, the error message is giving us a clue: “Can’t Parse ‘Pt1’. Sequence Item with Index 0 Has A Wrong Type”. This tells us that something is wrong with the type of data we’re trying to parse. Similarly, in SEO, we need to diagnose issues affecting a website’s visibility in search engines. Tools such as Google Search Console can provide insight into problems such as broken links or slow loading times.
Resolution: Once the problem has been identified, the next step is to resolve it. In coding, this might involve recasting a variable to the correct type. In our example, the resolution may look something like this:
def rectangle(pt1, pt2):
if isinstance(pt1, tuple) and isinstance(pt2, tuple):
# rest of function code follows
In SEO, resolution might involve actions such as optimizing webpage meta tags, improving site speed, or cleaning up broken links.
Evaluation: After implementing your solution, it’s important to evaluate whether it has worked. In coding, this involves testing your function to ensure it works without throwing errors. In SEO, this could involve tracking your website’s rankings in search engine results over time, or using an analytics tool to monitor changes in site traffic.
Understanding how SEO fits into digital marketing comes down to understanding these steps – diagnosis, resolution and evaluation – and knowing how they are applied to optimize a website for search engines. The key takeaway here is that just like a malfunctioning function can bring a whole program down, poor SEO can drastically reduce the effectiveness of a digital marketing campaign. Hence, it holds a crucial role in the field of digital marketing.
By diving deep into the mechanisms behind the process, from the implementation of keywords to backlink generation, you are not just coding algorithms; you’re coding your way to success.
For more details, you can refer to Beginner’s Guide to SEO by Moz, where detailed explanation is given about these processes and their implementation in your digital marketing strategy. Also, you may want to check out Moz‘s other guides on todays issues and resolutions concerning coding, SEO and digital marketing.Keywords are a critical part of any SEO strategy, especially in situations where you’re dealing with complex coding issues such as running into bad argument errors in the ‘Rectangle’ function. The error message encountered, “-5:Bad Argument In Function ‘Rectangle’ – Can’T Parse ‘Pt1’. Sequence Item With Index 0 Has A Wrong Type”, suggests that we’re dealing with incorrect data type being used to sequence item indexed at 0.
[When doing keyword research](https://www.semrush.com/blog/keyword-research-the-ultimate-guide/), key aspects to consider include:
Relevancy: Keywords need to be relevant, both to the content being produced and to user queries. In our case, keywords around “debugging”, “data type error”, “Rectangle function” or “’Pt1′ parsing error” would be highly relevant, attracting coders looking for solutions to this problem.
Search Volume: It’s crucial to target keywords which users frequently use in search queries. High volume keywords can bring more traffic, yet also have higher competition.
Keyword Difficulty: This parameter computes how hard it would be to rank for specific keywords. Ideally, choose those with lower difficulty scores but a decent amount of expected traffic to maximize reach while also considering your website’s domain authority.
To illustrate how one might correct the “Rectangle” function parsing error using Python, the keyword researched may be clustered into a conceptually related subset and presented in an HTML code format:
xxxxxxxxxx
if isinstance(pt1, tuple):
x, y = pt1
else:
raise TypeError("'Pt1' is not a tuple.")
This python code snippet checks if ‘pt1’ is a tuple before proceeding to parsing it further. If not, it raises a TypeError with information that ‘Pt1’ should be a tuple. By ensuring the right data type for ‘Pt1’, we eliminate the mentioned error message and improve the functionality of the ‘Rectangle’ function.
While focusing on these elements, it’s recommended to leverage SEO tools that provide insights about keyword performance, competition, user intent, and other useful metrics. Examples of such tools could include Google Keyword Planner, SEMRush, Moz, or Ahrefs, all of which can aid in optimizing a website’s performance in the face of algorithm changes.
If one understands the intricacies of keyword research, it goes a long way in attracting quality traffic to a site, even when discussing occasionally arcane subjects like debugging data type errors in a given function.
References
- [SEMrush – Ultimate Guide to Keyword Research](https://www.semrush.com/blog/keyword-research-the-ultimate-guide/)
- [Python Documentation – Error Types](https://docs.python.org/3/tutorial/errors.html)
When programming, you could encounter the error
xxxxxxxxxx
-5:Bad Argument
In Function ‘Rectangle’ followed by a message like Can’T Parse ‘Pt1’. Sequence Item With Index 0 Has A Wrong Type. This is often due to an inappropriate value or data type passed as an argument in the function. However, this particular problem seems disjointed from our topic of “Robust Link Building Strategies for Better Ranking”. Let’s handle each topic independently.
Starting off with the Rectangle function issue, the likely culprit here is that the argument being passed to the ‘Rectangle’ function in Python doesn’t meet the expected requirement. Usually, the OpenCV rectangle drawing function expects an argument in the form of a tuple containing coordinates for two points that define the rectangle.
The general syntax for a rectangle function in python is:
xxxxxxxxxx
cv2.rectangle(img, pt1, pt2, color[, thickness[, lineType[, shift]]])
Where:
–
xxxxxxxxxx
img
– your source image on which rectangle has to be drawn.
–
xxxxxxxxxx
pt1
and
xxxxxxxxxx
pt2
– Vertices of the rectangle. The rectangle is drawn from top left (First point) to bottom right (Second point).
–
xxxxxxxxxx
color
– Rectangle color or brightness (grayscale image). For colored images, it must be a tuple such as (255, 0, 0) for Blue.
–
xxxxxxxxxx
thickness
– Thickness of lines that make up the rectangle. Negative values makes it a filled rectangle.
– Other parameters are optional.
For example,
xxxxxxxxxx
import cv2
Img = cv2.imread("path/to/image.jpg")
cv2.rectangle(Img, (100, 50), (200, 150), (0, 255, 0), 2)
This will draw a green square bounded by points (100,50) and (200,150) onto the given image. If you aren’t supplying arguments in this format, then you’ll encounter issues.
Now, switching gears, let’s talk link-building strategies for better search engine ranking. We keep this strictly in the context of coding and search engine optimization (SEO). Here are a few coding-centered tips:
– Use Anchor Text Efficiently: In HTML, we often use anchor tags
xxxxxxxxxx
<a>
to create hyperlinks. When creating such links for SEO purposes, make sure that the text is descriptive and includes the keywords you want to rank for. Also, the
xxxxxxxxxx
href
attribute should direct to a relevant and valuable URL.
– Entity & XML Sitemap creation: An XML sitemap is a simple list of URLs that you can submit to Google to tell them about your site structure. You can create an XML sitemap using different libraries available in Python like sitemap-generator.
– Employ “Dofollow” and “Nofollow” tags: Not every link you produce needs to influence your search engine rankings. When trying to influence page ranks, use
xxxxxxxxxx
rel="dofollow"
. When you want a hyperlink that doesn’t impact the ranking, use
xxxxxxxxxx
rel="nofollow"
.
– Implement Structured Data: HTML is great for understanding what the elements are on a page but it doesn’t tell much about what those elements represent. That’s where structured data comes in. Utilize JSON-LD or Microdata to allow search engines to better understand – and therefore display – your content.
While these link-building strategies help, without relevant, keyword-rich, quality content, they may not dramatically lift rankings. Always remember, content still plays a pivotal role in effective SEO.Local Search Engine Optimization (SEO) is an exceptional practice to amplify online visibility, especially for businesses aiming to create a significant impact on their local demographic. This tactic works harmoniously with the programming expectation implied by your error message: ‘
xxxxxxxxxx
-5:Bad Argument In Function 'Rectangle' - Can't Parse 'Pt1'. Sequence Item With Index 0 Has A Wrong Type
‘.
Even though it seems unrelated at the first glance, when we delve deeper into these aspects, a correlation can be throttled out from their intrinsic attributes.
At its core, both Local SEO and writing efficient code demand a thorough understanding of the designated protocols, specifically pivotal in avenues like function calls in coding or keyword research in SEO. Considering your error message, this signifies a concern experienced while passing arguments into the ‘Rectangle’ function. Here, the argument intended to be parsed as ‘Pt1’ perhaps couldn’t satisfy the prescribed type condition, which was expected by the function. My presumption is that you’re dealing with something similar to:
xxxxxxxxxx
def Rectangle(Pt1, Pt2):
# function body - It is expecting Pt1 to be in a certain format/type
Just as fixing this error demands comprehending the required parameters and making sure all input data fits those stipulations, Local SEO necessitates catering content around geographical keywords and structuring web pages (its every element) to equip local relevance.
Remember, the goal is always to ensure computational ease in case of programming (which could eliminate errors like the aforementioned), and in case of Local SEO, to enable search engines to effortlessly comprehend your business and its context.
Here’s a few ways Local SEO can enhance online visibility:
- Google My Business Listing: This is similar to a comprehensive snapshot of your business in Google’s perspective. An optimised listing provides critical information promptly to customers searching in your vicinity. It fulfills necessary schema conditions (akin to type requirements in coding).
- Targeted Keywords Research: If your website and contents are not optimized for the location and services you provide, chances are high that even well-structured functions wouldn’t save you from the obscurity. Specific local keywords guide crawlers to your site increasing its visibility.
- Accurate NAP Consistency: Parallel to requiring correct arguments for each function call, ensuring accurate Name, Address, and Phone number (NAP data) across all platforms is crucial to rank higher in local searches.
- Localized Content: Crafting content pertinent to local news, events, or sharing local customer testimonials may upsurge your connectivity quotient in that locality, encouraging visibility.
Consider a business as a complex function taking several arguments. To get optimal results (think low SERP ranking), it must ensure every parameter dealt with (like keywords research, targeted content, local backlinks) adheres to the Google’s algorithm requirements. The same way as in the coding scenario earlier, you have to streamline the input type definitions to comply with what the function expects.
Coding and SEO bear surprising similarities! For more insights into Local SEO, you might want to explore MOZ’s detailed guide. And a handy reference for managing function arguments in Python can be found in Python’s official documentation. Remember, debugging code or optimizing websites, getting the basics right ensures easier navigation through complex problems.
Mobile Optimization and Its Impact on SEO
There is a clear interrelation when discussing Mobile Optimization and SEO practices. However, relating it to the situation (-5: Bad Argument) In Function ‘Rectangle’ – Can’t Parse ‘Pt1’. Sequence Item With Index 0 Has A Wrong Type, involves considering how coding errors or incorrect function arguments could potentially impact the performance of a website, particularly on mobile devices, and consequently its SEO ranking.
Mobile Optimization for Improving SEO Ranking
Mobile optimization is critical as it directly influences the user experience on mobile devices. Websites well optimized for mobile experiences tend to gain more traffic, reduce bounce rates, increase dwell time, and garner better user engagement. All these factors contribute significantly to improve search engine ranking, motivating SEO experts to pay close attention to mobile optimization.
- A mobile-friendly design: This helps in scoring higher engagement metrics like time spent on page and reduces bounce rate, directly influencing the SEO.
- Fast load speed: Search engines like Google prioritize websites that provide faster user experiences, particularly on mobile devices.
- Responsive website coding: Platforms correctly coded to adapt and respond according to different devices will undoubtedly have an edge in terms of SEO rankings.
Implication of Coding Errors in Mobile Design
When designing a mobile-friendly site, the process often requires code that reacts differently according to the device used, aptly called responsive design. If there’re any coding errors during the responsive design or improper use of functions (like in the context of the given issue) this can cause problems in display or functionality on mobile which might not be present on a desktop view of the site.
For instance, incorrect type passed to a function such as ‘Rectangle’ instead of expected point coordinates (pt1, pt2) can lead to issues like elements not rendering correctly on the screen.
xxxxxxxxxx
Rectangle pt1, pt2; //Expected
Rectangle "pt1"; //Incorrect usage leading to error
This specific mistake illustrates a ‘bad argument’, meaning the function is getting an inappropriate input for the task it’s designed to fulfill. Situations like this degrade the overall user experience, especially on mobile devices with more limited processing capabilities than desktops. They can lead to longer loading times, elements appearing out of place or not at all, potentially increasing the site’s bounce rate negatively impacting the SEO score.
Google’s Lighthouse, for instance, provides tools for audit and identifies such issues, helping developers to rectify them thereby improving the website’s performance and indirectly, the SEO ranking.
Structured Data and SEO
Additionally, investing time into properly structuring the data contained within your web pages, including correct usage of types and prerequisites in your functions can also enhance your SEO rating as structured data allows search engines to better understand the content.
xxxxxxxxxx
<script type="application/ld+json">
{
"@context": "https://schema.org",
"@type": "WebSite",
"url": "http://www.example.com/",
"potentialAction": {
"@type": "SearchAction",
"target": "http://www.example.com/search?q={search_term_string}",
"query-input": "required name=search_term_string"
}
}
</script>
In summary, while mobile optimization plays an integral role in SEO practices, managing correct code implementation, including appropriate function calls and usage, contributes equally to the performance of websites, ultimately affecting their visibility and ranking on search engines. Hence, troubleshooting and resolving issues such as the bad argument error in the function ‘Rectangle’ is crucial for preserving mobile optimization’s impact on SEO.When it comes to the intersection of strategic user experience (UX) and Search Engine Optimization (SEO), it’s essential to understand how these two elements can mutually reinforce each other. On one hand, a carefully-crafted UX strategy can significantly enhance your SEO performance by attracting higher traffic, increasing engagement rates, and reducing bounce rates. On the other hand, effective SEO techniques can help boost your site’s visibility and draw more potential users, thus offering ample opportunities for improving UX.
However, some problems may arise while attempting to code or develop the elements necessary for enhancing UX and SEO. A typical issue is encountered in code errors, such as the one described in the context (-5:Bad Argument) In Function ‘Rectangle’ – Can’t Parse ‘Pt1’. Sequence Item With Index 0 Has A Wrong Type. This error usually occurs when inappropriate argument types are passed in a function call or when parsing a certain object becomes complicated due to incompatible data type or structure.
In the given scenario, the error might be occurring within a
xxxxxxxxxx
Rectangle
function where ‘Pt1’, an expected sequence item, has been inputted with a wrong type. This could potentially undermine the on-site UX due to inconsistencies, malfunctioning features or even a broken layout if the function is linked to crucial website elements like sizing, layout management or event handling, which are integral parts of the total User Experience.
To fix this code error in Python, you should first ensure that the sequence items ‘Pt1’ passed have the correct type. For instance, if the
xxxxxxxxxx
Rectangle
function is expecting a tuple of coordinates (x,y), make sure that you are passing a tuple and not any other data type.
xxxxxxxxxx
# Below is an example of a correct syntax
Rect = Rectangle((x1, y1), (x2, y2))
Bear in mind that a failure to tackle such code problems swiftly may result in poor user experience due to non-functional interactive features or slow loading pages. This will inevitably affect your SEO rankings as search engine algorithms take into account metrics that reflect the quality of user experience, such as time spent on page and bounce rate.
Emphasizing strategic user experience isn’t just about aesthetics and interactivity. It’s equally important to craft a seamless UX that works hand-in-hand with the technicalities of good programming practices and efficient debugging techniques. Google’s Page Experience Update, for instance, is set to prioritize sites that offer excellent UX [Google’s Page Experience](https://developers.google.com/search/blog/2020/05/evaluating-page-experience).
As a developer, contemplating the user’s journey through debugging code errors offers an invaluable perspective. Through considering your own function ‘Rectangle’ problem, it’s easy to see why regular site audits and addressing errors quickly impacts positively on both UX and SEO. By refining the user experience strategically, troubleshooting common code issues, and optimizing for search engines, we can hit that sweet spot where UX and SEO truly complement one another.Structured Query Language (SQL) is an essential tool used by programmers in the database management systems. It’s frequently used for managing data in relational databases and executing CRUD operations, which stand for Create, Retrieve, Update, and Delete. Programmers prefer SQL because it provides them with the necessary tools to efficiently manage their data.
#### SQLException: “Bad argument in function ‘rectangle’ – can’t parse ‘pt1’. Sequence item with index 0 has a wrong type” error:
If you encounter the above-mentioned SQLException, it means there is a problem with the arguments passed in the ‘rectangle’ function. The function ‘rectangle’ is used to create rectangular shape in SQL spatial analysis. If the input isn’t readable as the expected type, you’ll get this exception. It happens due to:
– Incorrect datatype provided
– Wrong number of variables
– Faulty sequence or syntax
To rectify this issue kindly ensure, you give the ‘rectangle’ function the correct parameters it expects.
Here is how to declare a rectangle using its corner points in SQL:
xxxxxxxxxx
DECLARE @g geometry;
SET @g = geometry::STPolyFromText('POLYGON((0 0, 10 0, 10 10, 0 10, 0 0))', 4326);
SELECT @g.ToString();
In the above code, we’re creating a rectangular polygon using the STPolyFromText function. The polygon’s boundaries are specified in ‘POLYGON((0 0, 10 0, 10 10, 0 10, 0 0))’, where each pair represents a vertex of the rectangle.
#### The Power of analytics in SEO
The power of analytics in Search Engine Optimization (SEO) cannot be underestimated.
– Analytics provide valuable insights about users’ behavior on your website.
– SEO professionals can identify the most effective keywords, hence improving the website’s visibility in search engine results.
– User engagement metrics like CTR (Click Through Rate), bounce rate, and time spent on site can be measured and optimized.
– Analytics helps identify issues related to website speed, errors, and mobile optimization.
Google Analytics is a very useful tool in this domain. By using Google Analytics, keeping track of organic search traffic becomes simpler than everGoogle Analytics.
On-page SEO refers to optimizing individual webpages to rank higher in search engines. This practice primarily includes content and HTML source code. Incorporating relevant keywords and structuring the webpage’s HTML source for maximum visibility in search engine results are crucial strategies in SEO.
Implementing these SEO tactics help ensure a high-ranking placement in the search results page of a search engine. It increases visibility, driving more traffic toward your website, ultimately benefiting your online presence and business.
Remember, your website’s HTML structure must be excellent for better optimization. Home in your skills to ensure that every line of code contributes positively to your website’s rank in the search engine results. Review your process regularly so that no SQL exception could compromise your application’s performance.Sure, let’s delve deeper into this error:
xxxxxxxxxx
(-5:Bad Argument) In Function 'Rectangle' - Can't Parse 'Pt1'. Sequence Item With Index 0 Has A Wrong Type
. To appropriately handle this error message, we need to analyze it. Let’s dissect the error and pinpoint its causes.
Firstly, the
xxxxxxxxxx
-5:Bad Argument
indicates that there is an incorrect argument passed in the function. This typically occurs when you call a function with arguments of incompatible data type.
Secondly, the phrase ‘In Function ‘Rectangle” suggests that the issue arises specifically within the ‘Rectangle’ function. Being cognizant of where the error is occurring can assist us in rectifying it by narrowing down our focus to the aspects of our code related to this particular function.
The phrase ‘Can’t Parse ‘Pt1” means that the system was unable to understand or interpret the data item ‘Pt1’. This is possibly due to it being an incompatible data type or having an unexpected structure.
Finally, ‘Sequence Item With Index 0 Has A Wrong Type’ suggests that the first item (index 0) of your sequence (list, tuple etc.) is of an erroneous type i.e., the function expected it to be of one data type, but received a different one.
Here’s how you might resolve it:
To remedy this, verify the data types of the arguments that you are passing to the ‘Rectangle’ function. More than likely, ‘Pt1’ should be a sequence containing values of acceptable data type. Also double-check the datatype of the first item in your sequence.
Understand the requirements of the ‘Rectangle’ function, then ensure that the argument being passed (Pt1) adheres to these requirements. For instance, if the Rectangle function expects a two-item list/tuple where each item is an integer representing coordinates, ensure that Pt1 is structured correctly e.g., Pt1 = [x coordinate, y coordinate].
To further visualize what the corrected code might look like, refer to this:
xxxxxxxxxx
# ensure Pt1 is defined and populated correctly
Pt1 = [5, 10]
# where 5 and 10 represent x and y coordinates respectively
# define rectangle with Pt1 as argument
Rectangle(Pt1)
Even with carefully planned functions and syntax, sometimes errors still manage to creep through. They’re part and parcel of the programming journey towards creating more robust and reliable code. As you continue to debug and refine your functions, remember to use tools such as good editor software, resources like Python’s official documentation on sequence types and forums like StackOverflow to help untangle your coding quandaries.
By understanding the ‘-5:Bad Argument’ In Function ‘Rectangle’, mastering how to interpret these clues presented in the error message and applying the strategies as discussed, you will be better equipped at navigating around this and similar issues in programming. Happy coding!