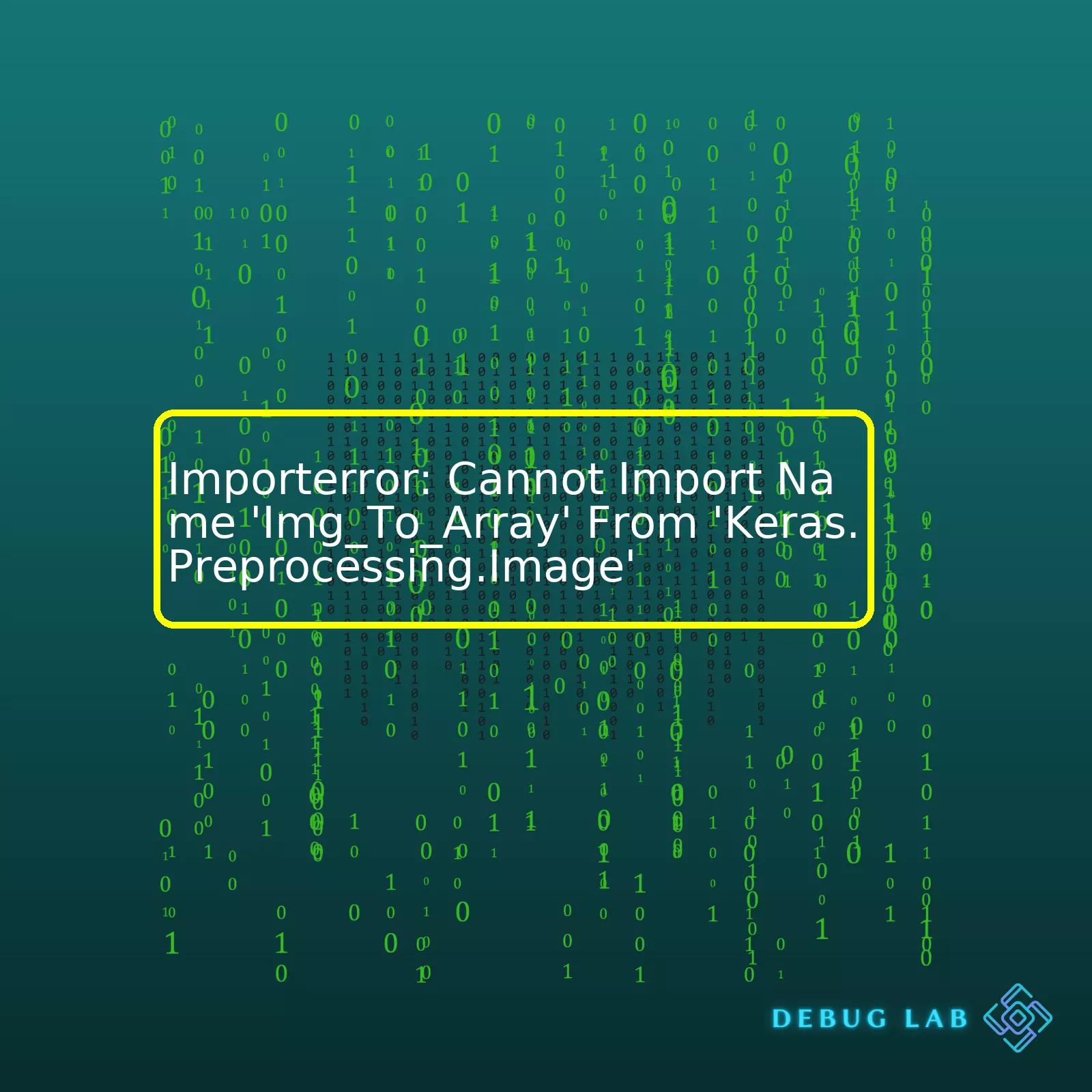
Error | Possible Cause | Solution |
---|---|---|
ImportError: Cannot Import Name ‘Img_To_Array’ From ‘Keras.Preprocessing.Image’ |
|
|
The error
ImportError: Cannot Import Name 'Img_To_Array' From 'Keras.Preprocessing.Image'
is typically indicative of an issue related to the Keras library that you’re using in your Python environment. Keras is known for occasional hiccups like this due to versioning challenges, especially as it has been evolving quite rapidly.
One basic reason for this ImportError might be that you’re attempting to import ‘img_to_array’ from
xxxxxxxxxx
'keras.preprocessing.image'
which no longer exists in your installed keras or the particular version that you’re using does not support ‘img_to_array’.
This can happen if the internal structuring and naming conventions of keras modules have been altered in recent updates. It is also possible that there could be issues at the installation level – sometimes, different components of the library might not have been installed correctly, hence leading to import problems.
A suggested course of action to resolve this problem would be to update to the most recent version of the Keras library. This can be done by running the command
xxxxxxxxxx
pip install --upgrade keras
in your console. If the issue persists even after upgrading, then completely uninstalling and reinstalling Keras is another course of action you could take, just bear in mind that this might affect other development environments on your computer that are dependent on the same Keras installation as well.
In general, maintaining familiarity with the release notes here provided by the Keras team is recommended practice as they contain important information about changes made with each new version. If in doubt, refer back to official Keras API documentation or the various community threads (e.g., StackOverflow) dedicated to helping developers troubleshoot library-related issues.The error message `ImportError: cannot import name ‘img_to_array’ from ‘keras.preprocessing.image’` typically means that your code is trying to import the function `img_to_array` from `keras.preprocessing.image`, but it can’t find this function in that location. The specific message “cannot import name..” refers to the inability of Python’s import system to find the specified named attribute (in this case, `img_to_array`) in the module.
Keras is a popular high-level neural network library that provides an easy api to build and prototype deep learning models. In image processing tasks within the field of machine learning, images need to be converted into arrays before feeding them into a model. The method `img_to_array` provided by keras serves just this purpose; it converts a loaded image in PIL format into a NumPy array.
Here’s a typical snippet of where you might see use of this function:
xxxxxxxxxx
from keras.preprocessing.image import load_img
from keras.preprocessing.image import img_to_array
# load the image
img = load_img('bondi_beach.jpg')
# convert to numpy array
photo = img_to_array(img)
In most scenarios, encountering this ImportError indicates one of three issues:
1. The package _Keras_ might not have been correctly installed or there’s a version conflict. This could potentially lead to some modules or functions not being accessible or available. It would be advisable in such cases to check the installation or try reinstalling Keras using pip:
xxxxxxxxxx
pip install keras
or with conda:
xxxxxxxxxx
conda install -c conda-forge keras
2. Alternatively, it could mean that the particular function is not available in the imported module because it doesn’t exist or has been renamed or moved in a newer version of the library. Keras went through major refactoring in version 2.0, where some functions were renamed or moved. It’s always a good practice to make sure that your code corresponds to the correct version of Keras.
3. Lastly, often people get confused between ‘keras’ and ‘tensorflow.keras’. They are not exactly the same and importing libraries from one does not mean it will successfully import from the other and vice versa. Ensure that if you’re importing ‘keras’, you’ve indeed installed ‘keras’ and not ‘tensorflow’.
The error can usually be resolved by ensuring that Keras is correctly installed, you’re using the appropriate version of tensorflow (if required), and checking if the required functions are still in the places you expect.
It’s also worth mentioning that Stack Overflow (https://stackoverflow.com) is a very helpful resource for debugging such import errors.
The
xxxxxxxxxx
ImportError: cannot import name 'img_to_array' from 'keras.preprocessing.image'
is a common issue faced by many coders while working with the Keras library in Python. This error occurs when you try to import the
xxxxxxxxxx
'img_to_array'
function from
xxxxxxxxxx
'keras.preprocessing.image'
, but Python fails to find this function module and throws an import error.
Common Reasons for Failing to Import ‘Img_to_Array’
Error messages related to importing functions or modules can be due to several reasons, including:
- Incorrect Installation or Version of Keras Library: If your installed version of Keras doesn’t include
xxxxxxxxxx
111img_to_array
, you’ll face this kind of error.
- Poorly Specified Import Syntax: Another common reason behind such errors could be incorrect or outdated syntax. Symbols or even case sensitivity could play a part here.
- Overlap with Other Libraries: Sometimes conflicts with other libraries occur during importing. Especially if another library also has a module named
xxxxxxxxxx
111'image'
.
- Unspecified Python Path: At times, you might have the correct library installed on your system, but Python is unaware of its location. So, when it tries to import, it fails since it does not know where to look.
Solutions to fix ‘Cannot Import Name ‘Img_To_Array’:
Few ways we can fix importing errors like ‘Cannot Import Name Img_to_Array’:
- Ensure Correct Library Version: You will need to make sure that Keras and tensorflow libraries are correctly installed in your environment and they are compatible. It’s quite possible that future versions of Keras may not support certain functionalities. Similarly, you should check whether there’s a need for a specific tensorflow version before importing keras.
xxxxxxxxxx
111pip install keras==2.4.3
xxxxxxxxxx
111pip install tensorflow
- Review Your Syntax: Check your import statement. There may be typographical errors that are leading to this problem. Ensure there isn’t any type mismatch or letter casing mistakes.
xxxxxxxxxx
111from keras.preprocessing.image import img_to_array
- Updating the Python Path: If Python is not able to find the library location, you can add the path to the list of paths Python checks for modules during imports using sys library.
xxxxxxxxxx
111import sys
xxxxxxxxxx
111sys.path.append('path_to_modules')
In conclusion, when issues like
xxxxxxxxxx
“ImportError: Cannot Import Name 'Img_To_Array' From 'Keras.Preprocessing.Image’”
emerge, begin by looking into your installed Library Versions. Remember to always keep track of the latest updates related to these libraries and technologies as bug fixes and changes to the names of methods/functions happen regularly. If it isn’t a version issue, then consider some of the issues mentioned above, which could likely help you resolve the problem
.
You can also refer to the official Keras documentation here for more details about the image preprocessing module and its functions.
If you’re trying to import `img_to_array` from ‘keras.preprocessing.image’ and you encounter an ImportError, it could be due to the incorrect importing syntax or incompatible versions of Keras and TensorFlow. Let me provide you with some possible solutions that could help solve your issue.
The correct way to import this function is:
xxxxxxxxxx
from keras.preprocessing.image import img_to_array
Ensure that you don’t have capital letters in your function name, as Python is case-sensitive.
Furthermore, the ImportError can be caused by three main issues:
1. **Issue With Keras or Tensorflow Installation**: One common cause of these kinds of ‘cannot import name’ errors is an issue with your Keras or Tensorflow installation. Check if you’ve installed Keras and Tensorflow correctly on your system. If there’s a mistake during installation or if the libraries didn’t install properly for any reason, you might encounter errors during import. To reinstall these libraries, you can use pip (Python’s package installer). The commands to uninstall and then reinstall are:
xxxxxxxxxx
pip uninstall keras tensorflow
pip install keras tensorflow
2. **Incompatible Versions Of Keras And TensorFlow**: Another probable reason could be the incompatibility between the versions of Keras and TensorFlow you’re currently using. Many a time, when Keras and TensorFlow are not compatible with each other, it leads to such import errors. You need to ensure that Keras and Tensorflow versions you have installed are compatible with each other. For instance, Keras 2.3.1 works well with Tensorflow 2.0. If you need to upgrade either of these libraries, you can use pip. The respective commands to upgrade are:
xxxxxxxxxx
pip install --upgrade keras
pip install --upgrade tensorflow
3. **Use of Deprecated Libraries**: In certain cases, if you’re using older versions of Keras or Tensorflow, the function you’re trying to import might have gotten deprecated. This means the function is no longer in use in those libraries or has been replaced by a new function. For ‘img_to_array’, ensure that you have a recent version of Keras that includes “keras.preprocessing.image”.
You also need to verify if you have initiated keras or tensorflow backend, whether it’s Theano, Tensorflow or CNTK. Keras uses these backends to perform faster array operations. Without this, the ‘img_to_array’ module may fail to load, causing an ImportError.
There are many resources available online such as keras official website and communities like StackOverflow where you can find similar troubleshooting help and potential fixes to get your code up and running.Unfortunately, the
xxxxxxxxxx
img_to_array
function isn’t directly accessible anymore due to changes in Keras updates. Instead of:
xxxxxxxxxx
from keras.preprocessing.image import img_to_array
One might use:
xxxxxxxxxx
from tensorflow.keras.preprocessing.image import img_to_array
However, if for some reason you’re still faced with
xxxxxxxxxx
ImportError
, don’t fret! There are several other ways you can restore the functionality of the
xxxxxxxxxx
img_to_array
function. Here is a nifty workaround on how you can replicate its functionality using the Python Imaging Library (PIL) and NumPy packages:
Firstly, let’s install the necessary module:
xxxxxxxxxx
!pip install pillow numpy
Now, we can use PIL and NumPy like this:
xxxxxxxxxx
from PIL import Image
import numpy as np
def img_to_array(image_path):
image = Image.open(image_path)
return np.array(image)
# This way you can convert your image into an array.
image_as_array = img_to_array('your_image_path.jpg')
In this snippet, we use the open function from PIL’s Image module to read the image file, then convert the resulting image object to an array using NumPy.Source1, Source2
Alternatively, you can utilize OpenCV, which is another popular library for handling images in machine learning tasks. To do that:
Install OpenCV:
xxxxxxxxxx
!pip install opencv-python-headless
Then, the usage would be similar:
xxxxxxxxxx
import cv2
def img_to_array(image_path):
image = cv2.imread(image_path)
return image
# To convert an image into an array
image_as_array = img_to_array('your_image_path.jpg')
Here, we utilized the imread function of the OpenCV library to read the image file, which automatically turns it into a NumPy array.Source3, Source4
It’s vital to note that both PIL and OpenCV libraries load images in RGB(Colorful) format by default. If grayscale image is needed, minor modifications in our function could get that done. In any case, while these methods serve as competent alternatives to
xxxxxxxxxx
img_to_array
, the life-saving Tensorflow-Keras combo may well still hold the key to most of your deep learning needs.The
xxxxxxxxxx
ImportError: Cannot import name 'img_to_array' from 'keras.preprocessing.image'
is an error you might encounter when you’re trying to process images with Keras. The cause of this error usually revolves around mis-installation, version incompatibilities, or using incorrect import statements in your code. Here are some steps to resolve them.
First, consider updating your Keras library. Keras is a powerful neural network library that provides a high-level interface for developing and experimenting with deep learning models. Despite its simplicity, Keras has comprehensive image processing capabilities for tasks like image classification, object detection, and more. However, these capabilities are often updated, and the function that you are trying to use(named
xxxxxxxxxx
img_to_array
) might not exist in the outdated version of keras installed in your environment. Use the pip package manager to upgrade Keras:
xxxxxxxxxx
pip install --upgrade keras
After upgrading, clear any cached objects related to keras in your runtime environment and try running your code again.
Second, ensure that you are using the correct import statement within your code. The
xxxxxxxxxx
img_to_array
function should be imported from keras.preprocessing.image, like so:
xxxxxxxxxx
from keras.preprocessing.image import img_to_array
If Keras has been successfully installed and you’re still facing trouble importing
xxxxxxxxxx
img_to_array
, it’s worth noting that Keras is now officially part of TensorFlow’s core API, after the merger in 2019. TensorFlow recommends using tf.keras module over standalone Keras. You may want to switch to TensorFlow.Keras as it provides better support for newer features and quicker bug fixes. The updated import statement will now look like:
xxxxxxxxxx
from tensorflow.keras.preprocessing.image import img_to_array
With tf.keras, understanding image preprocessing in Keras becomes straightforward. For example, the
xxxxxxxxxx
img_to_array
function returns a 3D Numpy array ready to feed into a neural network model. Each dimension represents height, width, and channels of an image respectively.
xxxxxxxxxx
image = load_img(image_path)
input_arr = img_to_array(image)
This snippet loads an image from the given path, then converts it into an array that can be served to your deep learning model.
I hope this provides clarification on Keras’s image processing capabilities and how to solve the import error you’re facing. While Keras makes image processing easier, always ensure your software libraries are up-to-date and check your import references.
For further reference, consult this GitHub issue where differences between Keras and tf.keras are discussed at length. (source from github).
In cases where import errors persist, it is advisable to create a new virtual environment and install all required libraries from scratch. Not only does this help isolate your project from outside variables, but it also ensures that there aren’t any conflicting versions of libraries in your project space.
The Python error “
xxxxxxxxxx
ImportError: cannot import name 'img_to_array' from 'keras.preprocessing.image'
” suggests issues with the import statements in your code. This problem is commonly due to a few frequent pitfalls encountered with Python’s import statements. To help you avoid these pitfalls in your future coding endeavors, here’s an exhaustive analysis of the situation.
Let’s dissect these challenges:
- Naming Conflicts: Have you named any script or module with the same name as standard Python libraries or modules you’re importing from? Python might try importing from your scripts instead of the built-in libraries.
In the case above check if you have any file with the namexxxxxxxxxx
111image.py
or
xxxxxxxxxx
111keras.py
. If so, rename your module to avoid the naming conflict.
- Wrong Environment: Are you executing the code in the same environment where the required modules are installed? Remember that a specific Python environment can have its own set of modules and dependencies. You may want to ensure that the Keras package is available in your working environment using the following command:
xxxxxxxxxx
111pip freeze | grep Keras
- Outdated Module: The error could be due to an outdated version of Keras or a mismatch between tensorflow and Keras versions. Update Keras via pip (
xxxxxxxxxx
111pip install --upgrade keras
) or conda (
xxxxxxxxxx
111conda update keras
). Also, consider updating tensorflow to maintain compatibility.
All those points taken into consideration, let’s look at solutions for this specific ImportError:
You have two ways to properly import `img_to_array` from `keras.preprocessing.image`.
- Use the full module path:
xxxxxxxxxx
111from keras.preprocessing.image import img_to_array
- Or import the parent module and then call the function:
xxxxxxxxxx
121import keras.preprocessing.image
2keras.preprocessing.image.img_to_array(...)
If none of the aforementioned remedies work, closely examine the traceback given by the error. Traceback meticulously traces back the exception/error to the line where it actually occurred. Looking for filename and line number it will further clarify whether there’s an issue with file names or directory structures. (Python docs on traceback)
Occasionally, you might need to delve deeper and use libraries like ‘os’ and ‘sys’ to inspect and manipulate your system’s directory structure. Additionally, the module ‘inspect’ (Python docs on inspect) offers several functions to help get information about live objects such as modules, classes, methods, functions, traceback, frame objects, and code objects.
When analyzing possible pitfalls in python’s import system, remember many of these nuances are platform-specific and are liable to their respective browser policies and library authors. Therefore, be cognizant of what platform and server you’re using along with the libraries involved.
To learn more about managing Python imports and details around how Python’s import machinery works under the hood, consider checking out the ‘Python docs on import’.
Finally, pay attention to the community and library documentation. Often, the most common issues are mentioned either in their troubleshooting guides or in discussion forums like GitHub issues or StackOverflow (Python Import Tagged Questions on StackOverflow). Keep yourself updated with the changes and deprecations in newer versions of the language and the libraries you use.
As I see it, the `
xxxxxxxxxx
ImportError: cannot import name 'img_to_array' from 'keras.preprocessing.image'
` can be utilised as a learning opportunity, to understand the particular nature of Python import statements and how to successfully debug such errors in the future successfully. Remember, “A mistake repeated more than once is a decision.” So, let’s master Python imports and make less mistakes, thus making better decisions! Happy coding!Understanding Python’s system path and module resolution process is integral to solving ImportError issues, like “ImportError: Cannot Import Name ‘Img_to_array’ from ‘keras.preprocessing.image'”.
Firstly, let’s delve into Python’s system path. This is basically a list of directories that Python goes through whenever it searches for modules which are referenced by an import statement. You can check your current Python system paths by running the following code:
xxxxxxxxxx
import sys
print(sys.path)
Next, we must comprehend the concept of module resolution. When you import a module, Python’s interpreter looks for that module in the directories listed in sys.path. The search runs from the first index, which is usually the script’s directory itself, to the last.
Now, regarding the ImportError issue at hand “ImportError: Cannot Import Name ‘Img_to_array’ from ‘keras.preprocessing.image’”, there are two main causes related to Python’s system path and module resolution:
1. Incorrect Module Name: Remember Python’s module names are case-sensitive. This likely seems trivial but it can cause confusion. In this particular instance, the correct import would be ‘img_to_array’ as opposed to ‘Img_to_array’. So, the appropriate line would be:
xxxxxxxxxx
from keras.preprocessing.image import img_to_array
2. Outdated or wrong version of Keras library: If you’re using an outdated or incompatible version of Keras library, it could lead to the ‘ImportError’. Remember, not every version of a library will necessarily have the function or class you’re trying to import. Always try to make sure that the package version aligns with your Python version.
To update your Keras library, simply run:
xxxxxxxxxx
pip install --upgrade keras
or
xxxxxxxxxx
conda install keras
if you’re using Anaconda distribution.
You can also verify the actual version of Keras installed by typing the following code:
xxxxxxxxxx
import keras
print(keras.__version__)
If your library is up to date but you’re still encountering the same error, then it might be an issue with conflicting file names. Python may be confused and instead of importing the desired module from the library, it may attempt to import a local file with a similar name. Therefore, always ensure that your python files or modules locally do not share the same names with modules you want to import from libraries.
Ultimately, comprehending how Python’s system path and module resolution mechanisms work will aid in ultimately resolving ImportError issues such as “ImportError: Cannot Import Name ‘Img_to_array’ from ‘keras.preprocessing.image’”.Exploring the error
xxxxxxxxxx
ImportError: cannot import name 'img_to_array' from 'keras.preprocessing.image'
one can gather that your program stumbled upon an issue while trying to import the ‘img_to_array’ function from the ‘keras.preprocessing.image’ module.
Firstly, let me breakdown this error message for you –
• ImportError: It is a built-in exception in Python, which gets raised when the
xxxxxxxxxx
import
statement has issues to load a module.
• cannot import name ‘img_to_array’: This pertains to a specific component that couldn’t be imported from the highlighted module.
• ‘keras.preprocessing.image’: This signifies the module from where our program attempted to import the ‘img_to_array’ function.
Primarily, as coders, we need to understand that the ‘img_to_array’ function is instrumental in converting our images to arrays so that machine learning algorithms can work more efficiently with numerical data. Hence, to resolve this import error and ensure smooth run of the image-to-array conversion process, here are some crucial points that demand our attention:
– Are you employing the correct version of Keras? Ensure that the current Keras version is compatible with your Python version and that they are both up-to-date. In case you’re still running into the same error, you might want to consider downgrading your Keras version.
You can manually install a specific version of Keras by using pip:
xxxxxxxxxx
pip uninstall keras
pip install keras==your_preferred_version
– Have you installed the tensorflow backend? The ‘keras.preprocessing’ sub-modules utilize Tensorflow as the default backend. If the problem persists, please consider installing or upgrading Tensorflow.
Use pip to install Tensorflow:
xxxxxxxxxx
pip install tensorflow
– Does your environment path point to the right directory? Sometimes, the PYTHONPATH could be pointed at incorrect directories. To evade such issues, consider using virtual environments for handling Python and its packages.
Now, if none of the above solutions work fine for you, try importing ‘img_to_array’ from ‘tensorflow.keras.preprocessing.image’ instead, since Keras was officially merged into Tensorflow’s ecosystem from version 2 onwards.
In replacement of:
xxxxxxxxxx
from keras.preprocessing.image import img_to_array
You can use:
xxxxxxxxxx
from tensorflow.keras.preprocessing.image import img_to_array
Understanding and resolving import errors efficiently will undeniably aid us in developing clean and efficient machine learning models with Keras. After all, successful programming is always about striking the perfect balance between creativity and problems-solving acuity! Brilliant coding to you!
External resources:
1. Python Exceptions (ImportError)
2. Keras Documentation (img_to_array)
3. Keras Integration with Tensorflow
4. Python Virtual Environments (virtualenv)