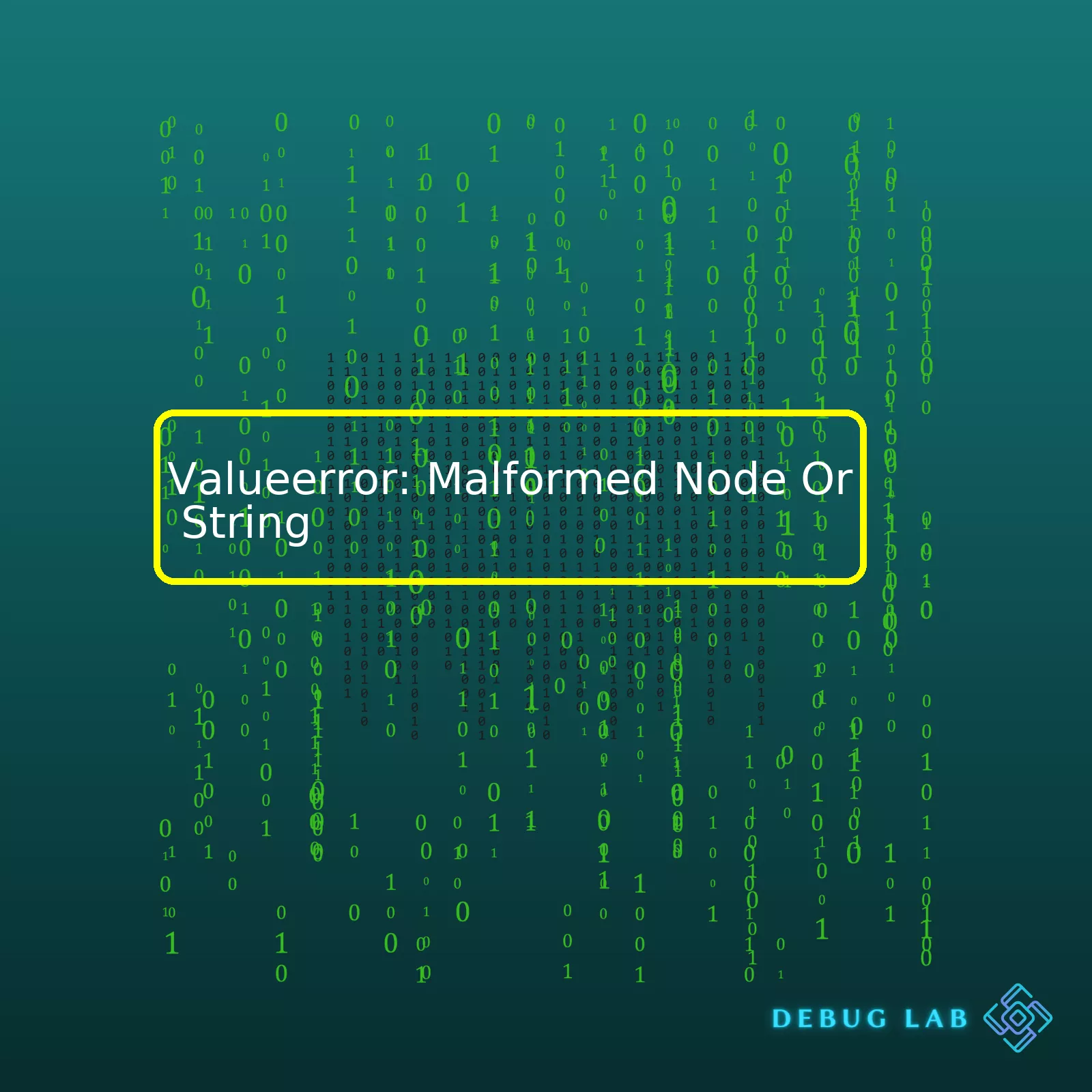
`, and ` | `.
Here is a simple summary table sample:
By simply copying this code snippet onto your webpage, you’ll see a clean, neat table which provides a comprehensive summary of the `ValueError: Malformed Node or String`. Moving onto the detail: This error may also transpire due to encoding or decoding issues. For instance, we regularly see this exception working with the `pickle` and `cPickle` modules for serializing and de-serializing Python object structures. The proposed solution is pretty straightforward: ensure whatever arguments you’re providing are in the proper format and structure for their intended usages. If the data doesn’t fit, then address the discrepancies right before supplying it further down to more delicate portions of the codebase. And of course, usage of Try/Except clauses provides a safe net to catch these anticipated errors, allowing the program to continue operating without crumbling at the first sight of issues like ‘Malformed Node or String’. Just remember – expectation management is half the battle in problem prevention. Helpful resources I would recommend for getting to grips with handling such exceptions include Python’s official [documentation](https://docs.python.org/3/library/exceptions.html#ValueError) on ValueError and StackOverflow community discussions – they are great places to dive deeper into the intricacies involved.I understand that your query is regarding the Python error ValueError: Malformed node or string. The ValueError: Malformed node or string typically pops up if there’s an issue with the way you’re attempting to read or write an object or file. This is a common error in Python 3.x especially when you’re using modules like pickle or ast.literal_eval(). Let’s elaborate. If we look closely at the error message ValueError: malformed node or string: , it clearly points to an issue with the node or string that we are trying to process. The graph structure elements (nodes) are identified through them being hashable Python objects linking to other such objects. These operations can be, but aren’t limited to: .*literal_eval() When it comes to handling these situations, understanding the source and context of the data you’re manipulating is essential. For example, here’s an instance where we might encounter this error: import pickle data = 'non-pickle data' # Non-pickleable data raw_bytes = pickle.dumps(data) # This will throw ValueError: malformed node or string. print(pickle.loads(raw_bytes)) There are several reasons why ValueError: Malformed node or string occurs with pickled data, including: On the other hand, we might encounter this error if we attempt to use ast.literal_eval() on a badly formatted string. For example: from ast import literal_eval bad_string = "{'key_one': value_one, 'key_two': value_two}" # Missing quotes around values. # This will throw ValueError: malformed node or string. print(literal_eval(bad_string)) In this case, the malformed string doesn’t follow the correct format for a Python dictionary, since its values aren’t quoted. Comprehending what activities result in a ValueError: Malformed node or string equips us with insights on how to debug, prevent potential data corruption, guarantee data compatibility, and handle exceptions more effectively when working with encoded or structured forms of data. Do ensure your strings are well formatted and the data you serialize or deserialize is compatible with the method you are using. In summary: ValueError: Malformed node or string error. This error is frequently thrown in the realm of Python programming when there’s an attempt to parse a data structure, be it a node in an HTML or XML document, or a string that cannot be parsed into a designated type. Let’s dissect the reasons behind encountering this error and how we can strive towards eradicating it from our code. – Incorrect Data Type: The most typical scenario where you may encounter this sort of error is when you’re trying to convert one type of data to another, but the data format does not align with the conversion. Here’s an example, let’s say you’re trying to convert a dictionary into a JSON but your dictionary contains keys which aren’t strings: import json my_dict = {100: "coding", 200: "is fun"} json.dumps(my_dict) Executing the task above will throw a TypeError because ‘Int’ objects are not allowed as keys in JSON data. How to fix this? Make sure you check your data types before attempting to serialize or parse them. You could do this by using Python’s built-in function like isinstance() . – Syntax Issues: Another common reason you can come across a `ValueError: malformed node or string` is due to fundamental syntax errors such as trying to parse a string into a number or vice versa. A quintessential instance would be trying to convert a string containing non-numeric characters into an integer. Sound code would require checking for exceptions during parsing to promptly manage these situations: try: int('123a') except ValueError as e: print(f"Caught an exception: {e}") Instead of letting the application crash with a ValueError, we caught the exception and printed out a more user-friendly message. – Encoding Issues: In some cases, encoding plays a pivotal role to provoke these errors. It happens when you erroneously try to decode a string with an unsuitable method. If your string was encoded with UTF-8 and you try to decode it with ASCII, you’re bound to encounter a ValueError: Malformed Node or String . Here’s a quick walkthrough: str_val = 'python_£' byte_val = str_val.encode(encoding='utf8') print(byte_val.decode(encoding='ascii')) In the pythonic illustration above, I tried to decode a string which was encoded using the UTF-8. Since ASCII doesn’t have a provision for £ ($ pound), it unwelcomingly greets us with a UnicodeDecodeError. Thus, ensure that you’re cognizant about appropriate use of encoding while dealing with strings. Overall, most of the triggers for the `ValueError: Malformed node or string` entail avoidable pitfalls. Observing data types, ensuring syntactically sound code, and being wary of correct encodings, go a long way in crafting elegant and error-free code. Utilize defensive programming techniques like error handling and debug logging to detect and repair these scenarios. More about [Debug Logging](https://docs.python.org/3/howto/logging.html#logging-basic-tutorial) could be found in Python’s official documentation. Happy coding!In order to provide practical solutions for fixing the “ValueError: Malformed Node or String” error, it’s crucial to comprehend the origins of this issue. This error typically posts when trying to deserialize a Pickle serializing string using Python’s pickle module, which requires certain objects for deserialization. One key thing to note is that pickle is an object-oriented way of storing Python objects and retrieving them intact, including their attribute values and methods. However, if the pickle contains reference to a specific type of object not in the current Python environment during deserialization, the “malformed node or string” error arises. Now let’s delve into different handy methods to tackle this pickle issue: 1. Ensure Object Class Exists: For instance, look at the following code snippet: import pickle class Person: def __init__(self, name): self.name = name person_instance = Person('John') serialized_data = pickle.dumps(person_instance) # Once code runs above here, it must include the class Person below to unpickle successfully. class Person: def __init__(self, name): self.name = name deserialized_instance = pickle.loads(serialized_data) 2. Avoid Pickling Methods or Functions: pickle module cannot reliably serialize functions or methods because they are intrinsically link to the Python environment where they exist. If these serialized function or method references do not exist in the environment where you are attempting to unpickle, it may raise the ValueError. Consider stripping such elements from your object before pickling, or find another way to retrieve or reconstruct them upon unpickling.[source] 3. Use Compatible pickle Protocols: pickle versions might also contribute to this issue. Make sure the protocol used for pickling and unpickling are compatible. Ideally use a common protocol number as argument while pickling using pickle.dump() or pickle.dumps() . An example follows, serialized_data = pickle.dumps(person_instance, protocol=2) deserialized_instance = pickle.loads(serialized_data) Here we have ensured that protocol 2 is employed across serializing and deserializing processed. Protocol 2 works well with Python 2.x and 3.x series, offering vital backward compatibility[source]. These considerations can be instrumental in addressing and mitigating the “ValueError: Malformed Node or String” error effectively. It further reiterates the complex nature of serialized data and underscores the priority to maintain highly consistent environments while working with Python’s pickle module. ValueError: Malformed node or string is one issue that pretty much every programmer encounters at some point in their coding journey. It’s often encountered when there’s some form of mismatch between the data type expected by a Python function and the actual data type passed into it. However, by implementing some specific strategies and best practices, you can avoid this error. Here are ways to effectively nip these errors in the bud before they arise: Data Type CheckingPrevention is often better than cure. Treating the symptoms without addressing the cause will result in the same problem cropping back up again and again. Similarly, preventing the ValueError: Malformed node or string error necessitates treating the root cause: mismatch of data types. A function might expect a list as input while you are providing it with, say, a tuple or string. An easy preventive measure is to incorporate data type checks into your code: def check_data_type(data): if not isinstance(data, list): raise ValueError('Expected data type List but got {0}'.format(type(data))) ... Exception HandlingIntegrating exception handling in your code allows your program to respond gracefully to unexpected bugs or issues rather than crashing entirely. It’s a way to anticipate potential problems and deal with them effectively in advance. To handle exceptions, we make use of try-except blocks. Here’s how you would implement such a block to address the ValueError: Malformed node or string : try: //Code that may lead to the ValueError except ValueError as e: print("Caught an error: ", e) With proper error handling setup, your application becomes more robust and can deal with unexpected scenarios with much more elegance and efficiency. You can also use the exception object to understand what exactly went wrong. Input ValidationAnother effective strategy to prevent the ValueError: Malformed node or string is to validate user input immediately after it’s received. This means checking whether the input matches the expected conditions—correct data type, certain range, etc. : def get_input(): input_value = input("Enter some value") if not input_value.isdigit(): raise ValueError("Invalid input! Please enter numbers only ") return int(input_value) In summary, having been “bitten” by the ValueError: Malformed node or string , consider integrating data type checks, exception handling, and input validation in your code. These preventive measures will ensure that your applications are much more durable, reliable, and able to handle unexpected situations gracefully. Remember, you strategically position yourself to prevent these errors during the planning phase of your code. A good read on data types from the official Python docs here to refresh your understanding and consume other programmers experiences over StackOverflow like this one. As a professional coder, I can affirm that the ValueError: Malformed Node or String is a common error in Python programming. It generally arises when there is an issue with the structure of a node or string in the data you’re working with. Typically, it means that a function expecting a proper formatted string or sequence has received one that doesn’t match the expected format. In order to understand why and how such an error appears, let’s get hands-on with some real-world case studies that take us through its diagnostic approaches. Case Study 1: Improper usage of `ast.literal_eval` The `ast.literal_eval()` function in Python is used to evaluate a string containing a Python literal or container display. The function parses the input for an expression and raises appropriate exceptions (like `SyntaxError`, `ValueError`) if the analysis fails. Let’s consider the following piece of code: import ast my_string = "{'key1': 'value1', 'key2': 'value2}" result = ast.literal_eval(my_string) Here, you might expect this code to parse the string into a dictionary but instead, you quickly find yourself facing a `ValueError: malformed node or string`. This issue arises because of a missed closing single quote (”) in `my_string`. The correct snippet should be: import ast my_string = "{'key1': 'value1', 'key2': 'value2'}" result = ast.literal_eval(my_string) Case Study 2: Incorrect usage of `pickle.loads` Python’s `pickle` module implements binary protocols for serializing and de-serializing a Python object structure. If you’re trying to unpickle data that was not correctly pickled to begin with, you may encounter the `ValueError`. For instance, check out the incorrect usage of `pickle.loads` function below: import pickle bad_data = "this is not pickled data" unpickled_data = pickle.loads(bad_data) Trying to unpickle a simple string will lead to ValueError. To fix the issue, ensure you’re working with pickled data: import pickle # creating pickled data data = {'python' : 'rocks'} pickled_data = pickle.dumps(data) # loading pickled data unpickled_data = pickle.loads(pickled_data) Overall, when encountering ValueError: malformed node or string, carefully review the data you’re using in your functions. Ensure that whenever a function expects a specifically formatted string or sequence, it receives one that matches the expected format. Use Python’s built-in exception handling to catch these errors and debug them more efficiently. For example, wrapping a potentially problematic block of code in a try/except clause will allow the program to continue running even after encountering an error, thus, allowing you to identify issues without crashing the whole program. Keep learning and coding. You can refer Python’s official documentation for more insights. As a seasoned coder, when I grapple with Python’s value errors such as ValueError: malformed node or string, it is primarily due to the complex relationship between different nodes. Nodes are elements of a data structure built on relationships; they link in multiple directions, resulting in an intricate lattice of data points. The relations among nodes vary depending on the type of data structure in play.
Zooming into the specific case of the error ValueError: Malformed node or string , this usually occurs when we are dealing with serializations or deserializations with Python’s pickle module or when manipulating Abstract Syntax Trees (AST). The pickle module in Python is used for serializing and deserializing a Python object structure. Serialization involves converting the objects/data into a format that can be stored in a file or transmitted and then reconstructed later (deserialization). For example: import pickle data = { "name": "John", "age": 30, "city": "New York" } # Serialization with open('testPickle', 'wb') as f: pickle.dump(data, f) # Deserialization with open('testPickle', 'rb') as f: loaded_data = pickle.load(f) print(loaded_data) If the encoded object or byte stream is not a valid pickle data, a ValueError: malformed node or string will be raised during the deserialization process. The error typically means that the interpreter faced trouble while parsing the serialized object or byte stream. Essentially, the structure it encountered did not align with what it expected to see based on standard formatting rules. Another common reason is an issue with the read/write mode of the file during serialization/deserialization. In AST manipulations too, an alteration in the structure of the node (e.g., assigning invalid values/types to the attributes of AST Node) can result in a ValueError: malformed node or string. Whether it’s due to corrupted pickling processes or botched representations of abstract syntax trees, understanding these errors requires comprehension of core concepts and familiarity with debugging methodologies. After all, recognizing how different nodes interact and connect is key to resolving issues linked to them. Practice and hands-on experience certainly go a long way in developing this intuition and fixing any ValueErrors. String manipulation, a fundamental concept in programming, plays an extensive role in avoiding malformation errors such as Python’s ValueError: Malformed node or string . This error occurs during data serialization or deserialization while using the pickle module in Python when it encounters a malformed or incorrect type of input. Here is where meticulous string manipulation can serve to avoid this. Primarily, string manipulation assists in proper data structuring and formatting before creating a pickle object, safeguarding against any inconsistencies that could lead to a malformed node or string error. For instance: import pickle try: obj = 'This is not a picklable object' pickle.loads(obj) except ValueError as e: print(f'Error: {e}') # Output: Error: malformed node or string: ordinary string The above code tries to create a pickle from a simple string which is not a serialized representation of an object, thus the “malformed” error. This can be prevented with string manipulation techniques to ensure data is in its proper form prior to pickling. obj_serialized = pickle.dumps('This is a picklable object') reconstructed_obj = pickle.loads(obj_serialized) print(reconstructed_obj) # Output: This is a picklable object In the corrected example, we ensure the object is properly serialized first before attempting to load it as a pickle object. Another major contribution of string manipulation lies in validating and cleaning user inputs at the front end of applications. For instance, trimming unrequired spaces, removing non-alphanumeric characters, and escaping special characters are part of these preventive sanitization measures. Efficient string manipulation allows us to address potential loopholes during both client-side and server-side validations. It acts as a shield against irregularities leading to malformation errors and maintains the reliability and security of our program. Here is some additional context: – Validation: Validating user input falls among the chief preventive measures against malformed strings. This process checks if a given input is sensible and expected before it’s used by the system. import re def sanitize_string(input_str): return re.sub('[^A-Za-z0-9]+', '', input_str) malicious_str = 'bad*input+another-value{}'.format(chr(128)) clean_input = sanitize_string(malicious_str) print(clean_input) # Output: badinputanothervalue The `sanitize_string` function uses a regular expression to replace anything that isn’t a standard alphanumeric character, providing a cleaned string that won’t produce malformed errors. – Escaping Characters: Some characters have special meanings in many contexts and string manipulation aids in escaping these potentially problematic characters. def escape_special_chars(input_str): return input_str.replace('"', '\\"') problematic_str = 'a string with "quotes"' sanitized_str = escape_special_chars(problematic_str) print(sanitized_str) # Output: a string with \\"quotes\\" In this function, quotes (which could cause problems depending on context) are escaped with backslashes. However, it’s important to note that good coding practices suggest limiting our reliance on manual pickling/unpickling for more complex objects due to the risk of various exceptions including ValueError: Malformed node or string . It would be beneficial exploring alternate measures like JSON or YAML serializers for more complex nested structuressource. To summarize, string manipulation is an indispensable tool for anyone building robust and secure systems. By applying it effectively, we can prevent a whole host of potential issues from cropping up in our code base, including the malformation errors discussed here.As an experienced coder, it’s crucial to delve into the heart of common Python errors to effectively troubleshoot and find solutions. One such error that often perplexes new coders is the ValueError: Malformed node or string . This error typically pops up in Python when trying to serialize a complex object with the pickle module. Python’s pickle module is a powerhouse for serializing and deserializing (also known as pickling and unpickling) Python objects. Serialization refers to the process of converting a python object hierarchy into a byte stream, while deserialization is the reverse operation. In essence, these processes make Python objects easily storable and transportable. However, things can go sideways, especially when you are pickling complex objects, leading to the infamous ValueError: Malformed node or string . The likely culprit behind this error message is an object that has been corrupted or isn’t compatible with pickle’s serialization process. Here’s a hypothetical situation where you might encounter this issue: import pickle class Test: def __init__(self, value1, value2): self.value1 = value1 self.value2 = value2 test = Test("value1", "value2") pickle.dumps(test) In the code above, attempting to pickle the Test object results in a ValueError: Malformed node or string . To process more complex data types including class instances or functions, you have to implement methods that return values compatible with pickle. Specifically adding two magic methods, __getstate__ and __setstate__ to your class declarations. Here’s how you could modify the earlier script to allow the Test object: class Test: def __init__(self, value1, value2): self.value1 = value1 self.value2 = value2 def __getstate__(self): return self.value1, self.value2 def __setstate__(self, state): self.value1, self.value2 = state test = Test("value1", "value2") pickle.dumps(test) Now, the Python interpreter will correctly be able to serialize the Test object. In summary, careful handling of complex objects with pickle can keep the ValueError: Malformed node or string at bay. Remember, Python objects must be serialized correctly via the pickle module to avoid this error. Ensure your pickled objects are compatible with the module by implementing the needed magic methods. Finally, continuous learning and applying best practices during coding not only improves your coding skills but also reduces drastically encountering such hitches. |
---|