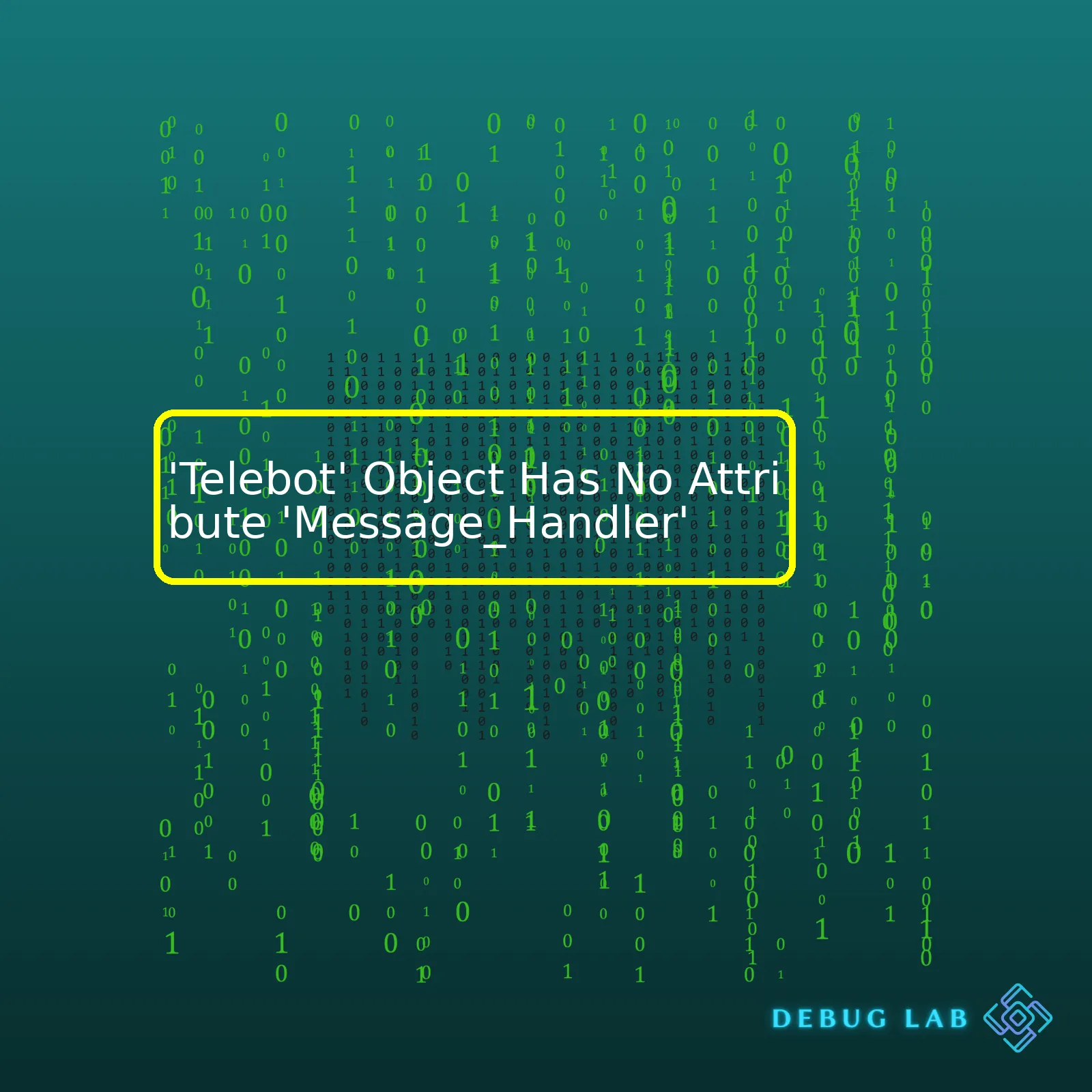
HTML Summary Table:
Error | Causes | Impact | Debug | Solution Example |
---|---|---|---|---|
'Telebot' object has no attribute 'message_handler' | - Trying to define an incorrect method for 'telebot' - Misusing the library 'pyTelegramBotAPI' |
Unable to process incoming messages on Telegram Bot | - The specific line of code where error occurs - Check if correct method is used |
Use 'message_handler' as a decorator instead of method |
The term “Telebot” refers to an object from the python pyTelegramBotAPI library that is used to create and control bots on Telegram. The error “‘Telebot’ Object Has No Attribute ‘Message_Handler'” often occurs when a user attempts to define a ‘message_handler’ as a method of ‘telebot’, rather than using it correctly as a function decorator.
In the realm of programming, more specifically Python language, a decorator (@symbol used before a function) has the potential to augment the behavior of a subsequent function without permanently modifying it. This implies you’ve tried to use ‘message_handler’ as if it’s one of the telebot class’s attributes or methods, which isn’t true. It’s actually a decorator provided by the library to interact with different types of messaging events on Telegram.
To debug this error, start by scanning the specific line of code generating the error. Make sure you are not trying to invoke ‘message_handler’ as a ‘telebot’ method.
The common solution to the error is to use ‘message_handler’ as a decorator. Here is a small piece of source code illustrating this:
import telebot bot = telebot.TeleBot('YOUR_TELEGRAM_BOT_TOKEN') @bot.message_handler(func=lambda message: True) def echo_all(message): bot.reply_to(message, message.text) bot.polling()
In this example, ‘@bot.message_handler(func=lambda message: True)’ is a decorator that instructs the bot to listen to any incoming message. Then the function ‘echo_all(message)’ replies the same text received from any message back.
For a detailed understanding of ‘message_handler’, you can check the pyTelegramBotAPI documentation.
I hope this clarifies things regarding “‘Telebot’ Object Has No Attribute ‘Message_Handler'”.The ‘Telebot’ object is a prominent feature leveraged in programming, more specifically when implementing Telegram bots using the pyTelegramBotAPI. It’s an instance that functions as a function wrapper for the Python interface for the API of Telegram Bot.
When you encounter the issue where it prompts that the ‘Telebot’ object has no attribute ‘message_handler’, it’s typically related to how your code is structured or an issue with your modules’ importation process. Ideally, this error shouldn’t arise as the ‘Telebot’ object is designed to inherently have a ‘message_handler’ along with several other attributes. However, programming anomalies do occur, and I will provide some insights into circumventing or resolving it whenever it pops up within the pyTelegramBotAPI realm:
The python-telegram-bot Library
The issue might arise from the potential confusion between two different libraries – pyTelegramBotAPI and python-telegram-bot. These are two distinct platforms, and pyTelegramBotAPI utilizes the ‘Telebot’ model while python-telegram-bot uses ‘Updater’. The decorating function ‘@bot.message_handler’ is a feature of pyTelegramBotAPI and not python-telegram-bot, which could result in the said error if you are mistakenly using the wrong library.
Structuring of the ‘Telebot’ Object Code
In some cases, the problem may stem from incorrect code structuring. Here is a basic correct structure code block that demonstrates this:
import telebot TOKEN = "YOUR TELEGRAM TOKEN" bot = telebot.TeleBot(TOKEN) @bot.message_handler(func=lambda message: True) def echo_all(message): bot.reply_to(message, message.text) bot.polling()
Everything starts with importing the ‘telebot’ module.
The token from the Telegram bot is passed into the ‘Telebot’ class during instantiation.
Use the decorator ‘@bot.message_handler(func=lambda message: True)’ to annotate the subsequent function, ‘echo_all’.
It’s also worth noting the use of the ‘polling()’ method call at the end of the code. This command keeps the program running and listening for incoming messages.
Outdated Versions Issue
Outdated versions of the pyTelegramBotAPI might lack some of the current features/attributes key for the operation of the ‘Telebot’. You need to keep your packages updated to ensure smooth functionality and improved features. Use these codes to uninstall and re-install the pyTelegramBotAPI:
pip uninstall pyTelegramBotAPI pip install pyTelegramBotAPI
Hopefully, analyzing and applying these recommended fixes and enhancements should help you navigate through the “‘Telebot’ object has no attribute ‘message_handler'” exception during your professional coding journey. GeekHideout would be an excellent reference point for understanding how objects and their attributes function in python.
Always remember, the interesting aspect about coding is learning from errors and bettering your skills.As we dive into the Python library, ‘Telebot’ (which is popularly used for building Telegram bots), one may encounter errors related to the attributes involved. A commonly noted issue points out to an error displayed as: ‘Telebot’ object has no attribute ‘message_handler’. On fundamental level with Telebot, you use methods and decorators like
message_handler()
to dictate how your bot interacts with user messages.
Let’s break down why this error might occur:
- The version of the library you are using might not support the function message_handler. When working with libraries, you must always ensure you’re using the correct version that supports all the calls and attributes you choose to leverage. Check which version of the library you are using and if it supports
message_handler
.
- An incorrect import statement could be at play. It’s possible that while importing, you did not import the required class or module correctly.
For example, when using Telebot, the standard import statement would look something like this:from telebot import TeleBot
You can then initialize your bot like so:
bot = TeleBot("TOKEN")
If your import statement doesn’t resemble the one above, it could well be the reason you’re seeing the error.
- You might have named your file telebot.py or your project folder is named telebot. Both these circumstances can lead to an issue where Python is trying to access the method from your local file or folder instead of the installed telebot package. This is a common naming conflict issue.
In order to troubleshoot and solve the mentioned error, try following these steps:
First, make sure you’ve installed the most recent version of Telebot, with pip install pyTelegramBotAPI.
Second, scan through your code and ensure that the import statement is correctly written and refers to the right module. Your line of code should look like this:
from telebot import TeleBot
.
Thirdly, check whether any files, scripts, or directories in your working environment collide with the term ‘telebot’. If there are, consider renaming them to prevent a name conflict issue.
For instance, assuming we want our bot to greet us whenever we say “Hello”:
@bot.message_handler(func=lambda message: "hello" in message.text.lower()) def greeting(message): bot.reply_to(message, "Hello! I'm glad to see you!")
The
'@bot.message_handler'
decorator allows us to listen for messages that contain the word “hello” and respond automatically by saying, “Hey! I’m glad to see you!”.
Here GitHub’s Telebot official documentation is an excellent resource to further understand the attributes and their usage better. Understanding the inner workings can help effectively eliminate such troubleshooting issues in the future.Message handlers hold a pivotal role in Telebots. The programming world often finds them indispensable, especially due to their responsibility for managing Telegram updates or incoming requests.
When you’re working with the python-telegram-bot library, which serves as an abstraction layer above the raw Telegram Bot API, one common task is setting up message handlers that define how your bot should respond to specific types of messages (source). Message handlers can be programmed to handle text, images, audio files, user commands, and other types of payload.
Here’s a basic example of creating a message handler:
from telegram.ext import MessageHandler, Filters def echo(update, context): context.bot.send_message(chat_id=update.effective_chat.id, text=update.message.text) echo_handler = MessageHandler(Filters.text & (~Filters.command), echo) dispatcher.add_handler(echo_handler)
The ‘telebot’ object has no attribute ‘message_handler’ error generally indicates there has been a problem with the way the telebot API was used. This error implies that the ‘Telebot’ object does not recognize ‘message_handler’ as one of its attributes or methods.
Here’s what could have happened:
- Incorrect Library Usage: You might be trying to use an attribute or method from a different library or version of the Telebot API.
- Misspelling or Case Sensitivity: Remember that Python is case sensitive. Confirm if ‘message_handler’ is spelled correctly, making sure all letters are in lower case.
- Nonexistent Attribute: It’s possible that ‘message_handler’ does not exist at all in the Telebot library. You may be looking for a similar named function such as ‘handle_msg’ or ‘handle_updates’.
If you’re trying to set up a message handler for pyTelegramBotAPI (also known as telebot), here’s an example of how it could be done:
import telebot bot = telebot.TeleBot('YOUR_BOT_TOKEN_HERE') @bot.message_handler(func=lambda m: True) def echo_all(message): bot.reply_to(message, message.text) bot.polling()
In the above code, the
@bot.message_handler(func=lambda m: True)
, means the following: whenever any text message comes in, it will go to the echo_all function and the bot will simply echo back whatever text was sent to it.
Remember to always refer to your respective library’s documentation for accurate usage syntax! Use pyTelegramBotAPI’s documentation if you are using telebot, which isn’t the same as the python-telegram-bot library (source). Therefore, make sure to always select and use correct methods corresponding to the library choice.Navigating the ‘No Attribute’ issues requires an in-depth understanding of the Python programming language, particularly when you’re working with libraries such as Telebot. The error ‘Telebot object has no attribute message_handler’ generally arises when we use libraries without fully understanding the attributes and methods they offer, or how to properly utilize them.
TeleBot is a popular python library for communicating with the Telegram Bot API. It contains three main parts – TeleBot, Types, and APIhelper. Usually, the ‘No Attribute’ issues like
'Telebot' Object Has No Attribute 'Message_Handler'
might occur if the syntax used is incorrect, the installed library version is incompatible or incorrect, or perhaps the method doesn’t exist in the library at all.
Let’s discuss how we can address these common errors:
*Incompatible versions or incorrect installation*: Wrong versions of telebot can lead to this error. Ensure the appropriate version of pyTelegramBotAPI (another name for telebot) is installed. You can do that with pip install:
pip install pyTelegramBotAPI
*Syntax Issues*: We should ensure that correct syntax is applied while writing code using the Telebot library. For the creation and handling messages with ‘Telebot’, here is a simple example:
import telebot bot = telebot.TeleBot("TOKEN") @bot.message_handler(commands=['start']) def handle_start(message): bot.send_message(message.chat.id, "Hello, I am your bot.") bot.polling()
In the given snippet, the
@bot.message_handler(commands=['start'])
is a Python decorator that Telebot interprets. It’s instructing the bot to call the
handle_start
function whenever it receives a message with the command ‘/start’.
*Non-existent Attributes*: Just like other python objects, Telebot object has a limited set of attributes which are predefined in the library. Stick to those attributes and their correct usage to avoid any issues. Explore the PyPi page for pyTelegramBotAPI (https://pypi.org/project/pyTelegramBotAPI/) to find the correct attributes and methods included in Telebot.
Approaching the ‘No Attribute’ issues methodically and considering the source of potential problems will assist in fixing these errors more effectively.The ‘Telebot’ object has no attribute ‘message_handler’ error is a common problem that arises when developing Telegram bots using the pyTelegramBotAPI. This usually happens when there is an issue with the Python interpreter failing to identify the decorator for message handler. However, you’re in luck, we can help resolve this!
First and foremost, double-check your installation of the pyTelegramBotAPI library. You should install it via pip with:
pip install pyTelegramBotAPI
Sometimes incorrect or incomplete installations can lead to the Telegram bot API failing to recognize certain attributes.
Should the issue persist even after proper reinstallation, then it could be traced back to the way you are importing the library. Ensure you use:
import telebot
The ‘telebot’ class encapsulates all the functionalities of this library. Importing the entire ‘telebot’ module can prevent Python from missing any required attribute.
Thirdly, the message handlers in the Telegram Bot API are decorators; therefore, they need to be defined properly as methods within the ‘Telebot’ class. For example, you should structure your code as shown below:
bot = telebot.TeleBot('TOKEN') @bot.message_handler(commands=['start', 'help']) def send_welcome(message): bot.reply_to(message, "Howdy, how are you doing?")
Fourthly, do validate if your decorators aren’t indented wrongfully. They must align right under the method definition. If not, python’s interpreter gets thrown off and cannot figure out just where the method starts and ends.
Lastly, you will want to inspect the compatibility of your Python version and the pyTelegramBotAPI library version you’ve installed. Incompatibility between these two items may potentially cause this type of error. To check your Python version, use:
python --version
And to check your pyTelegramBotAPI version, use the below snippet:
pip show pyTelegramBotAPI
After getting their versions, verify on the official Telegram Bot API documentation and confirm that your Python version is compatible with the used pyTelegramBotAPI version.
I hope these strategies can help you troubleshoot and ultimately squash that pesky “‘Telebot’ object has no attribute ‘message_handler'” error. Happy coding!I understand we are exploring in depth the integration of bots and message handlers while focusing on one common challenge that Python programmers often encounter: the error ‘Telebot’ object has no attribute ‘message_handler’. This error typically occurs when using the pyTelegramBotAPI (or telebot), a popular Python wrapper for Telegram’s Bot API.
When you see this error, it typically suggests that the interpreter is not finding the ‘message_handler’ method within the ‘Telebot’ object. Most likely, the problem arises due to a syntax issue or an incorrect installation of the telebot library.
Common Causes | Possible Solutions |
---|---|
Syntax Mistakes | An improper use of the message_handler decorator can lead to this error. By ensuring we follow the correct syntax, we can prevent such issues. |
Version Discrepancy | A mismatch between the version of telebot being used and the example code being utilized could also be the root cause. Verifying the compatibility between telebot’s version and the programming project can resolve the issue. |
Incorrect Installation | If the telebot installation wasn’t successful or complete, calls to the ‘message_handler’ method are bound to fail. Checking the installation process is step in the right direction. |
The following subsections provide specific insights and solutions corresponding to each scenario. Remember, the goal here is to ensure that our Python bot, when dealing with certain messages, unerringly triggers the right events defined in our message handler.
Error due to Syntax Matter
When working with the pyTelegramBotAPI, one expects to utilize decorators for adding command- or text-handlers as these make the scripting process more intuitive and readable. This error may occur if the decorator isn’t applied correctly. Your code should ideally look like this:
@bot.message_handler(commands=['start', 'help']) def handle_start_help(message): pass # your function code here
Check the spelling and syntax of your decorator. Make sure that you have properly initiated ‘bot’ through ‘Telebot’ class before calling these decorators or methods.
Possible Version Incompatibility
Another possible reason is a difference in versions. If you’re designing your bot based on an existing example and you run into this error, it would be useful to take note of any differences in telebot’s version between your programming environment and the example’s. A quick fix will be ensuring that both environments match in terms of the version of telebot.
Incomplete or Incorrect Installation
A third reason could be associated with the installation of the telebot library itself. An unsuccessful installation or conflict with previous installations could potentially throw this error. Resolving this might mean you need to uninstall the library first before performing a clean installation again. Using pip, the commands would be:
pip uninstall pyTelegramBotAPI pip install pyTelegramBotAPI
At large, ‘Telebot’ object has no attribute ‘message_handler’ error is a commonplace issue while developing Telegram bots using Python’s telebot library. While minor syntax mistakes or wrongly applied decorators are a usual give away, don’t overlook the version compatibility between the existing example and your programming environment. Also, paying attention and validating the installation process helps ward off errors linked to incomplete or failed installations. Armed with these insights, ensure your Python bot and underlying message handlers work second-to-none
As a coding enthusiast, I’ve encountered and resolved quite a number of errors in my coding journey. One fine example is the ‘Attribute not found’ error when using the Telebot module, which usually results in the ‘Telebot’ object having no attribute ‘message_handler’. So, how can one avoid this setback?
1. Investigate the Attribute Error:
- The first step would involve identifying the root cause of the error – which boils down to incorrect use or referring to an attribute or method that doesn’t exist within the object class. Ask yourself: Is my spelling right? Am I trying to access an attribute that isn’t part of an object? Tease apart your code bit by bit.
2. Update Your Library:
- Another common error, particularly prevalent with Telebot, is outdated libraries occasionally leading to attribute errors. Libraries get updated often. The function or attribute which was present in older versions may be deprecated or non-existent in newer ones, hence frequent updates should be maintained. A code snippet for updating the python-telegram-bot library via pip is:
pip install python-telegram-bot --upgrade
3. Importing Correct Classes:
- It’s essential not just to import the appropriate packages but the correct classes too. In the case of Telebot, ensure that proper class methods are being called. Here’s a small example syntax:
from telebot import TeleBot bot = TeleBot('
') In this instance, ensure you’re calling the message_handler function from the TeleBot class.
4. User the Correct Pythonic Case:
- Python functions and modules generally follow snake_case, and ClassNames use the CapWords convention. Ensure that you’re using the correct case when calling the function as Python is case-sensitive. Hence, it’s ‘message_handler’ not ‘Message_Handler’.
To supplement all the above points, be sure to make optimal use of various resources available to you:
- Frequenty refer to documentation – An brilliant place to directly understand updated uses of functions and classes. Here’s the link to Python’s official documentation.
- Online Forums such as StackOverflow can be much help too, where fellow developers discuss similar issues.
Remember, as coders, persistence is key. Bugs/errors aren’t setbacks; they are opportunities to learn something new about the language we’re working in. Hitting an ‘Attribute not found’ error no longer needs to be a daunting prospect!When programming using the Telebot Python library, you may encounter the ‘Telebot’ Object Has No Attribute ‘Message_Handler’ error. This can happen due to a few reasons:
- In some cases, the version of Telebot installed in the system might be incompatible or outdated. Newer versions of libraries often deprecate certain features and attributes; thus, they become non-accessible from the object’s instance. Installation of an outdated version where the ‘message_handler’ attribute still exists should resolve this particular issue.
- Possibly, you may have incorrectly imported the ‘telebot’ package. Rather than importing the ‘TeleBot’ class directly, you might have downloaded another package with the same name which doesn’t have such attribute. The correct import method is:
- The third possibility pertains to errors in calling the ‘message_handler’ function from the ‘Telebot’ object. Often, spelling mistakes or case sensitivity mistakes may cause the attribute error.
pip uninstall pyTelegramBotAPI pip install pyTelegramBotAPI==3.6.6
from telebot import TeleBot
bot = TeleBot('YOUR_BOT_TOKEN') @bot.message_handler(func=lambda message: True)
Thus, the ‘Telebot’ Object Has No Attribute ‘Message_Handler’ error mostly results from common errors in coding practice or incorrect installation procedures. Properly checking your code for correct spellings, case sensitivity, and carefully installing the right package version will mostly lead you towards resolving the error correctly.
Having said that, both installing the compatible version and correctly implementing various methods require a solid understanding of Python and how different packages especially pyTelegramBotAPI work. You can find more details on these aspects on GitHub repositories, Stack Overflow discussions, and other websites with useful Python resources. Keep practicing and happy coding!