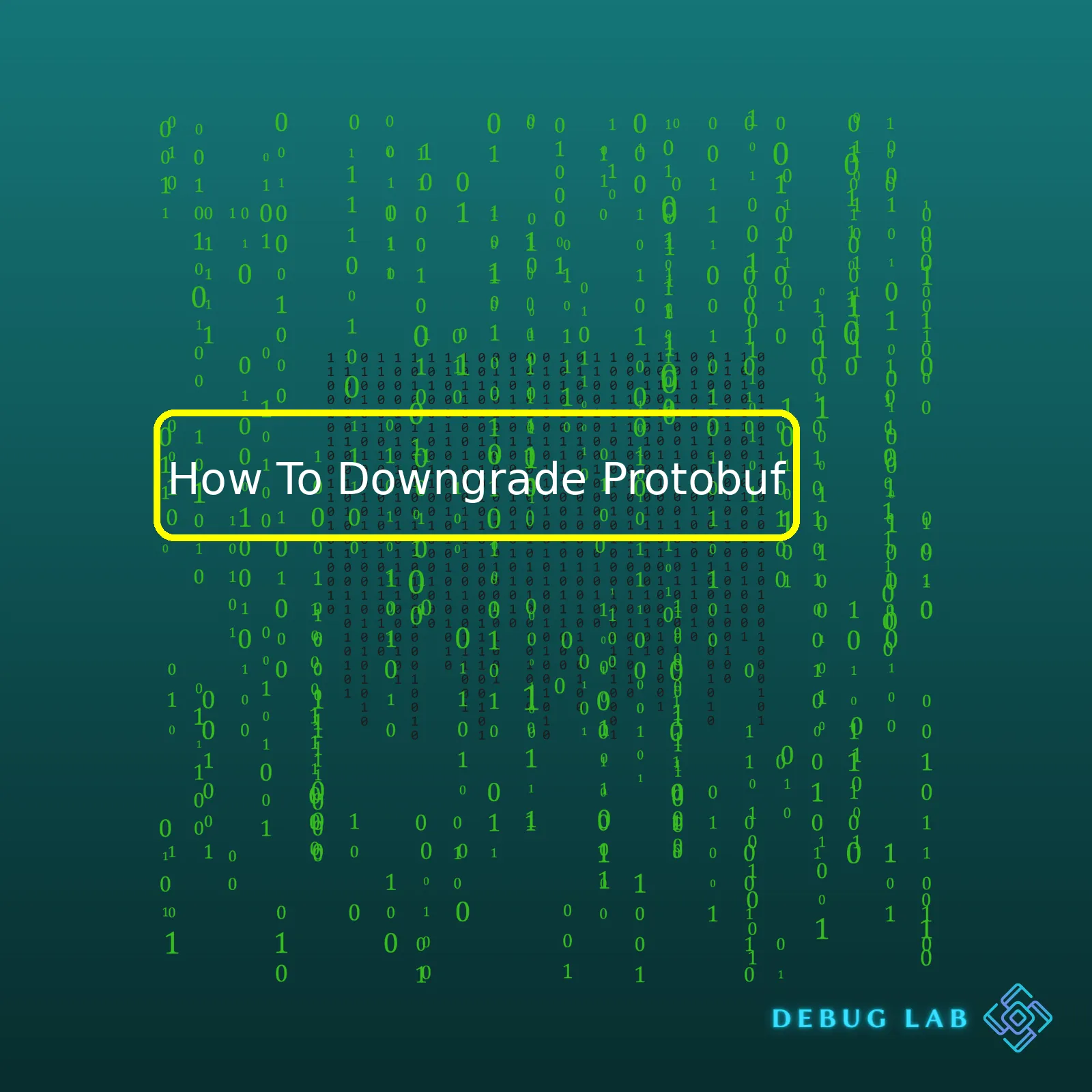
Step | Action | Description |
---|---|---|
Check Current Version |
x 1 1 pip show protobuf |
This command lets you know the current version of Protobuf installed in your system. |
Uninstall Existing Protobuf Version |
xxxxxxxxxx 1 1 1 pip uninstall protobuf |
The existing package is removed to make way for the required one. |
Install Desired Protobuf Version |
xxxxxxxxxx 1 1 1 pip install protobuf==3.6.0 |
A specified version of Protobuf is installed, replace ‘3.6.0’ with your desired version. |
Verify Installation |
xxxxxxxxxx 1 1 1 pip show protobuf |
Re-running this command enables you to confirm that the desired version has been installed correctly. |
When using the Google Protocol Buffers (protobuf) development environment and encountering potential version compatibility issues, the need to downgrade protobuf may arise. This process is straightforward and can be achieved through a sequence of commands entered into the terminal.
Firstly, establish your currently installed protobuf version through the
xxxxxxxxxx
pip show protobuf
command. If it becomes clear that a downgrade is essential for compatibility, start the process by uninstalling the existing version. This action can be performed by typing and executing the
xxxxxxxxxx
pip uninstall protobuf
command.
The next step allows you to install the specific protobuf version tailored to your needs. Let’s say you want to install protobuf version 3.6.0, this can be executed by running
xxxxxxxxxx
pip install protobuf==3.6.0
. Simply replace ‘3.6.0’ with your desired version number.
The final step, and an important one for maintaining code integrity, is verification. With the
xxxxxxxxxx
pip show protobuf
command, we can confirm that the targeted downgrade was carried out successfully. Now, your program should operate smoothly without any previous protobuf-related version issues.
Remember, your specific use case and dependencies might demand you to perform these operations in a virtual environment to isolate your work. Bear in mind to always backup your current working setting to avoid potential data loss or corruption during the transition.Sure thing. Protocol Buffers, often referred to as Protobuf, are a popular method utilized by well-known tech giants such as Google, Uber, and others for efficient serialization. This technology offers a flexible, effective, and automated mechanism for serializing structured data.
To understand Protocol Buffers, one must first grasp the concept of data serialization. Serialization is essentially the conversion of data into a format that can be transferred over a network or stored in some type of storage system. On the other side of this transfer or storage, the data can subsequently be deserialized back into its original format.
Protobuf differs from other types of serializers like XML, JSON, etc., because it’s simpler, smaller, faster, and more robust. It’s comprised of two unique parts:
– Language-agnostic schema definitions: These describe how to interpret the serialized data.
– Libraries: Available in a variety of languages, they use the schemas produced above to convert objects in their respective language into serialized byte streams and vice versa.
Why do these matter? Let me illustrate with an example. You have data in one system or architecture (like JSON), and you need to send it to another (like MongoDB). The receiving infrastructure can’t comprehend JSON data directly, so you serialize it using protobuf, which translates it into a common language both architectures can understand.
However, there can be scenarios where one may need to downgrade protobuf versions due to compatibility issues or specific feature usage of older versions not present in newer ones.
The process of downgrading Protobuf involves modifying versions in tool-specific configuration files and rebuilding those tools. For instance, if we’re using Maven as our build tool, we would update the version in our pom.xml file like so:
xxxxxxxxxx
<dependencies>
<dependency>
<groupId>com.google.protobuf</groupId>
<artifactId>protobuf-java</artifactId>
<version>YOUR_LOWER_VERSION</version>
</dependency>
</dependencies>
And rebuild our project. But be cautious as protos generated by a higher version of Protobuf compiler “protoc” are incompatible with lower versions. Hence when downgrading, you’ll also need to regenerate any .proto files using a compatible version of protoc.
Here’s an example of downgrading protoc:
Let’s assume you initially had protoc-3.14.0 and want to downgrade to protoc-3.5.1.
– Download protoc-3.5.1 from the official GitHub repository.
– Remove current version: `sudo rm /usr/local/bin/protoc`
– Unpack the version you need: `sudo unzip protoc-3.5.1-linux-x86_64.zip -d protoc3`
– Move protoc to /usr/local/bin/
xxxxxxxxxx
sudo mv protoc3/bin/* /usr/local/bin/
– Now your project works with protoc-3.5.1.
But remember, Protocol Buffers offer backward compatibility but not forward compatibility, that simply means the old version of .proto files can be read by the new version libraries/runtime but the new version of .proto files can’t be read by old version libraries/runtimes. So make sure that your decision of downgrading protobuf is feasible with this aspect of Protocol Buffers.
On a concluding note, while Protocol Buffers provide simplicity, efficiency, and consistency in structuring and manipulating data, understanding their behavior across different versions is crucial for maintaining interoperability amongst various systems in an application environment.In the domain of data serialization formats, Protocol Buffers, also known as Protobuf, has staked its claim as a highly robust and efficient system. The current version of Protobuf boasts several compelling features, including:
- It provides schema evolution, allowing you to modify your data structure over time with maintained forward and backward compatibility. This flexibility is crucial in evolving applications where changing requirements can affect the data structures.
- Protobuf is language agnostic which means it’s able to support multiple languages like Java, C++, Python, etc
- The current protobuf version allows for nested message types and enum types inside other message types, giving users richer data utilizations.
- The generated code from Protobuf schema is generally faster and more efficient compared to other serialization libraries such as JSON or XML.
With these sophisticated characteristics on display, one may question why there would be any desire to downgrade Protobuf. However, suppose you’re facing compatibility issues, encountering bugs specific to the latest version, or working with systems or libraries dependent on an older protocol version. In those situations, downgrading becomes a necessity.
Let’s delve into how you can perform this downgrading process for Protobuf.
To begin with, uninstalling your current protobuf version depends on two main factors: a) the way you initially installed protobuf (e.g., from source, a pre-compiled package, or package manager) and b) the operating system. On Linux, for instance, using apt (advanced package tool), an uninstall would look something like this:
xxxxxxxxxx
sudo apt-get remove protobuf-compiler
Once uninstalled, reinstall the desired, lower version. This requires fetching the older protobuf release from their Github releases page. After acquiring the correct .tar.gz archive (the version needed), extract its contents and install this older version.
Here’s a sample sequence of commands that describes this process:
xxxxxxxxxx
wget https://github.com/protocolbuffers/protobuf/releases/download/v3.11.4/protobuf-all-3.11.4.tar.gz
tar xzf protobuf-all-3.11.4.tar.gz
cd protobuf-3.11.4/
./configure
make
make check
sudo make install
sudo ldconfig # refresh shared library cache.
After running all these commands, verifying your installation is equally necessary. Execute the below command:
xxxxxxxxxx
protoc --version
This command will return the currently installed version of protobuf compiler.
Although navigating version control can be a tricky task with its fair set of challenges, understanding both the perks of your current Protobuf version and the backup of how to downgrade when things don’t go as planned, should lay the groundwork for a more smooth coding experience. Remember, Protobuf is a powerful tool, but its effective utilization lies in the hands of us coders – and how well we understand and navigate its pieces.
Protocol Buffers, also known as Protobuf, is a system developed by Google for serializing structured data. It’s widely used because of its flexibility and lightweight nature but sometimes, you might face scenarios where you need to downgrade Protobuf in your set up. The reasons for downgrading are numerous, but the most common include:
- Dependency Compatibility: Older versions of some libraries or frameworks can have compatibility issues with newer Protobuf versions. If your project relies heavily on such a library or framework, it can be necessary to downgrade Protobuf.
- Stability: Newer versions, while bringing additional features and fixes, could potentially introduce bugs or instability. When prioritizing stability over new features, some developers may opt to use an older version of Protobuf.
- Performance Solutions: In few cases, certain performance problems can occur with upgraded versions of the Protocol Buffers, which might be solved with downgraded, more stable releases.
- Complexity: Sometimes, the advanced and sophisticated features present in the latest Protobuf versions may add unnecessary complexity to a simple and straightforward project.
The process to downgrade Protobuf essentially involves uninstalling the current version and installing the desired version. Here’s an example of how you would do this:
xxxxxxxxxx
$ sudo apt-get remove --auto-remove protobuf-compiler
xxxxxxxxxx
$ sudo apt-get remove --auto-remove libprotobuf-dev
xxxxxxxxxx
$ wget https://github.com/protocolbuffers/protobuf/releases/download/v3.6.1/protobuf-python-3.6.1.tar.gz
xxxxxxxxxx
$ tar -xvf protobuf-python-3.6.1.tar.gz
xxxxxxxxxx
$ cd protobuf-3.6.1/ && ./configure
xxxxxxxxxx
$ make && sudo make install
xxxxxxxxxx
$ protoc --version
libprotoc 3.6.1
While it’s typically advised to use the newer and regularly updated versions due to the constant influx of security patches, performance improvements, and other benefits, there can undoubtedly be situations where it might be beneficial to use an older version of Protobuf.
Please remember that every setup and configuration comes with its challenges. Always double-check the dependencies before altering your Protobuf version. Be sure to analyze version compatibility with your system and any third-party libraries you’re using or intending to use. Downgrading should be your last resort after considering all other possible options and implications.
You can refer further to protobuf GitHub repo for more detailed instructions and understanding of various versions: https://github.com/protocolbuffers/protobuf.
Depreciating Protobuf to a previous version could be necessary for a variety of reasons: compatibility issues, outdated codebases, or specific project requirements. Here, I guide you through the process in a step-by-step manner.
Your first move would be to ensure your current Protobuf installation is correctly recognized by your system. Use the following command to verify this:
xxxxxxxxxx
protoc --version
The output should show the Protobuf compiler’s current version.
Secondly, uninstall your existing Protobuf version. To do this in a Unix-based operating system like Linux or MacOS, use the following commands:
xxxxxxxxxx
sudo make uninstall
sudo ldconfig
For Windows users operating within Cygwin, use:
xxxxxxxxxx
make install
Next, obtain your desired Protobuf version. Visit the Protobuf GitHub repository and pick your preferred version. After deciding on a version, download its source code which is typically in .zip format.
After downloading, extract the files into an appropriate directory on your device. You can generally use any archive software to handle file extractions. An example of a command line extraction using tar might look like:
xxxxxxxxxx
tar xvfz protobuf-x.y.z.tar.gz
Where x.y.z implies the Protobuf version that was downloaded.
At this point, prepare the package for building by executing the “configure” script included in the unzipped folder. Do this with the following command:
xxxxxxxxxx
./configure
You’ll then want to begin the build process. The ‘make’ command must be run after compilation to achieve this.
xxxxxxxxxx
make
Follow that up by checking the compiled code to make sure there are no errors. This can be done with ‘make check’.
xxxxxxxxxx
make check
Lastly, install the newly compiled Protobuf version system-wide. You will need administrative permissions for this part, so prefix your instruction with ‘sudo’ if you are on a Unix-based system.
xxxxxxxxxx
sudo make install
Now, when you run ‘protoc –version’, it should reflect the new (or rather old) Protobuf version that was just installed. Make sure you reinstall any dependent software packages, as they might have been impacted by the Protobuf downgrade process.
Although these steps work most times, keep in mind that other dependencies or project considerations may alter how straightforward this process will be for you. If you face any complications, don’t hesitate to refer back to the official Protobuf documentation or other relevant resources. Remember, having a thorough knowledge of both the installation and depreciation processes can help you navigate version-related obstacles in a more efficient manner.
Downgrading your Protobuf (Protocol Buffers) version can come with a slew of potential risks. It is crucial to understand these ahead of proceeding with the downgrade. We are going to delve further into this subject, and explain how you can manage the protocols buffers versions in a carefully manner in an attempt to minimize any negative consequences.
Potential Risks
- Loss of Newest Features: Higher versions of Protobuf often come with newer features and functionalities. When you downgrade, there’s a risk that you will lose access to these improvements. This can impede productivity or limit functionality for your project.
- Compatibility Issues: Not all your systems or software may be compatible with an older version of Protobuf. If there’s already a system established around the newer version, downgrading it might cause compatibility issues leading to system malfunctions or total failure.
- Safety Concerns: The newer versions of Protobuf usually come packed with security patches and updates figured from the previous versions. Hence, when you downgrade to a lower version of Protobuf, you potentially open yourself to security threats that are redressed in the newer versions.
- Maintenance and Support: Older versions may have reduced maintenance assistance and support availability from the provider’s end. Providers often reduce support to previous versions once they successfully deploy newer ones.
How To Downgrade Protobuf
Despite these potential risks, specific situations may compel you to downgrade Protobuf. Here is a general walk-through on how you can do it:
The process of downgrading Protobuf involves uninstalling the current version and replacing it with the older one. Let’s take Linux for instance:
xxxxxxxxxx
$ sudo apt-get remove protobuf-compiler
$ sudo apt-get install protobuf-compiler=2.6.1-1
You also need to verify the new version by using the following command:
xxxxxxxxxx
protoc --version # Ensure the output matches 'libprotoc 2.6.1'
The above procedure may differ based on your operating system. Nevertheless, it requires careful attention to detail to ensure no parts of the newer Protobuf versions remain, as they may interfere with the older version.
To mitigate the potential risks discussed, make extensive use of code and system backups before downgrading. That way, you could always revert changes in case of unforeseen malfunctions or incompatibilities. Always evaluate whether the downgrade is worth the potential security threats, and if possible, seek alternative approaches to accomplish what the downgrade is intended to solve.
Remember, Google’s official documentation for Protocol Buffers (Protobuf) provides in-depth information about differences between various Protobuf versions, which is useful during transition phases like downgrading. You should spend time understanding these before continuing.
The point is, while you can downgrade Protobuf and there might be convincing reasons to do so, you must approach it with caution considering the mentioned possible situations.
Now, let’s discuss the impact of downgrading Protobuf on system performance.
Protocol Buffers (Protobuf) is a long standing, widely used method of serializing structured data. Developers often opt in favor of this method due to its efficiency and speed; however, sometimes it may be necessary to downgrade Protobuf to an older version for reasons such as compatibility or integration with other components.
xxxxxxxxxx
# Typical installation command
pip install protobuf==version_number
In doing so, there could be several potential impacts on system performance.
Firstly, it’s important to understand that upgrading between major versions of Protobuf usually involves significant changes, which could include:
- Changes in serialization/deserialization speeds: Newer versions often have improved algorithms so downgrading could possibly lead to slower serialization/deserialization.
- Alterations to memory usage: Older versions might take up more memory. This can impact overall system performance if resources are limited.
- Varying support for programming languages: For example, if one is using Python, newer versions of protobuf support advanced features like type checking in python 3.x which older versions do not.
In addition to these, trade-offs could be involved in terms of compatibility, community-support, security, and bug fixes.
Factors | New Version | Old Version |
---|---|---|
Security patches | More frequent patches | Less frequent patches |
Bug Fixes | Up-to-date fixes | Potential delay |
Community Support | A larger community | Potential decrease over time. |
Compatibility | Incompatibility issues with older software | Compatibility with older software |
So, while making the decision of downgrading Protobuf, consider these factors in context of your application’s requirements and infrastructure capabilities. Also, test carefully post-downgrading to ensure the actual performance impact meets expectations.
xxxxxxxxxx
# Upgraded code example
message SearchRequest {
string query = 1;
int32 page_number = 2;
}
xxxxxxxxxx
# Downgraded code example
message SearchRequest {
required string query = 1;
optional int32 page_number = 2;
}
As depicted above, the syntax would differ across versions – replace “string query = 1;” from a newer version with “required string query = 1;” when downgrading.
If you’re looking for more extensive information about Protobuf, I’d recommend going through the official Google documentation. Here, you’ll find detailed descriptions about different use-cases and versions to better inform your downgrading decisions.The release notes available on the official GitHub repositories are also worth considering as they provide insightful changelogs.
After a successful downgrading operation of the Protobuf library, it’s crucial that we examine and verify its stability. The primary reason is to ensure that the existing services or applications dependent on Protobuf continue to function optimally, without any disruptions.
Protobuf, or rather Protocol Buffers, are Google’s language-agnostic and platform-neutral extensible mechanism for structuring data. It’s applied in several software development tasks, including RPC systems and interprocess communication.
I’ll guide you through the necessary steps and measures that involve:
- Checking and Updating Dependencies
- Running Tests
- Monitoring Performance
Checking and Updating Dependencies
First off, we need to ensure that all other libraries or services dependent on Protobuf are compatible with the newly downgraded version. This can be achieved by revisiting all dependencies and checking their Protobuf version requirement against the downgraded one.
Sample Python code snippet to verify Protobuf version:
xxxxxxxxxx
import google.protobuf
print(google.protobuf.__version__)
Running Tests
Upon confirming the compatibility with dependencies, initiate running all unit and integration tests again shortly after downgrade. Regression testing helps to identify if any functionality has been affected due to the new Protocol Buffers setup.
In java, here is an example of a simple test case using JUnit:
xxxxxxxxxx
import static org.junit.Assert.*;
import com.google.protobuf.*;
import org.junit.Test;
public class ProtoTest {
() {
String expected = "hello world";
YourMessage.Message message = YourProto.Message.newBuilder().setMessage(expected).build();
assertEquals(expected, message.getMessage());
}
}
Monitoring Performance
Beyond the initial testing phase, keep monitoring the performance of your applications and services. Espy for signs of unusual behaviors which could ensue as a result of downgrading Protobuf.
Check application logs frequently for any errors, increased latency or memory usage, and look into them immediately. Popular tools like ELK Stack or Splunk can be used to monitor and analyze system logs.
These steps will take you considerably closer to ensuring stability following a successful Protobuf downgrade. Even so, bear in mind that situations differ. And while this guidance provides a decent start line, it may not serve comprehensively. Hence, I advise always to accord an extra buffer period for close monitoring and quick remediation when necessary.
Frequently, downgrading Protobuf (Protocol Buffers) becomes a fundamental requirement to maintain the compatibility with older versions of libraries or frameworks which utilizes it. The task encompasses several specific steps that encapsulate both caution and accuracy.
The initial step demands your cognition of the current Protobuf version installed in your system. This serves as a key determinant in the overall downgrade process. It’s achieved with a simple command line
xxxxxxxxxx
protoc --version
. This tiny piece of code will help assess whether you have the compatible, desired version or should you indeed proceed with the downgrade.
This then prompts the important phase – installing a lower protobuf version according to your needs. You need to uninstall the existing version first. Using pip, you would use
xxxxxxxxxx
pip uninstall protobuf
. Now, despite this seeming apparent solution, you may suffer instances where the Protobuf version doesn’t get downgraded. Why? Some pre-installed packages have protobuf as a dependency, causing the failure of the straightforward uninstall/install method. Hence, you indeed to put a force-reinstall as follows:
xxxxxxxxxx
pip install protobuf==3.6.0 --force-reinstall
, assuming you wish to downgrade to version 3.6.0
But remember, no coding strategy is foolproof without constant validation and testing practices. A proactive coder would always verify each step of the execution process, including any changes to Protocol buffers framework versions. Similarly, here, always crosscheck your protobuf version after the downgrade using
xxxxxxxxxx
protoc --version
command to make sure it showcases the version you downgraded to, guaranteeing a successful operation.
Finally, if all else fails, remembering that sometimes, a complete wipeout of all Python-related installations from your system and starting fresh might be the only viable approach left. However, take caution as this should only be your last resort.
A successful Protobuf version downgrade can enable smoother testing and production deployment environments, ensuring maximum system compatibility. Thus, every careful step takes you closer to a better managed and structured coding ecosystem.
You may refer to the official TensorFlow GitHub issue thread [Downgrade Protobuf] that showcases relevant discussions regarding the said topic by seasoned coders and TensorFlow contributors alike.
In essence, here are some simple steps:
xxxxxxxxxx
$ protoc --version # check current Protobuf Version
$ pip uninstall protobuf # remove current installed version
$ pip install protobuf==[desired_version_number] --force-reinstall # replace [desired_version_number] with the version you want
$ protoc --version # validate downgrade
Employ these quick and easy steps to ensure an effective Protobuf downgrade. Happy Coding!