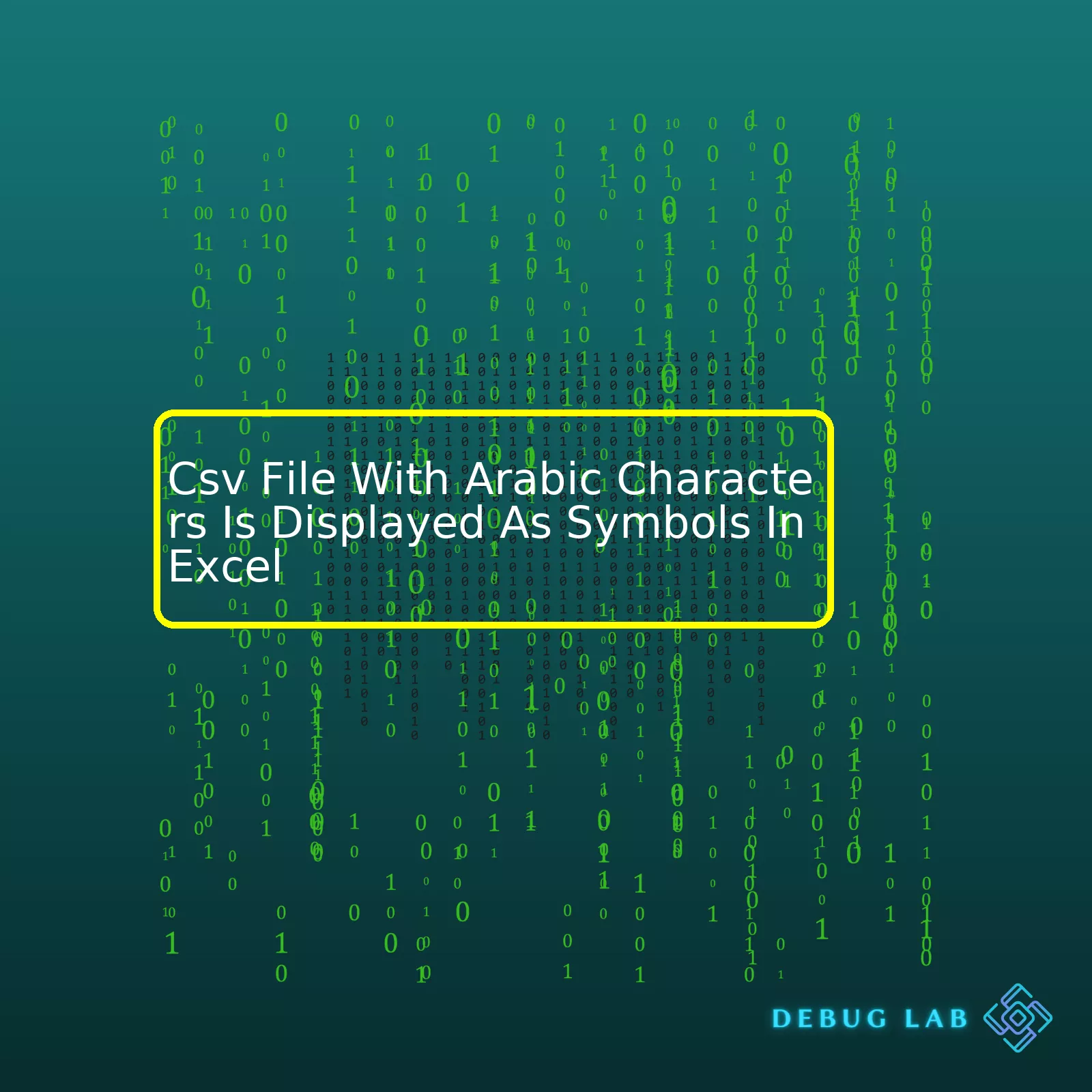
This issue often arises from encoding mismatches where your data is in one character encoding and the software you’re viewing it on assumes another. Especially, the problem becomes more prominent while using languages that have unique alphabets not used in English, including Arabic.
Below is a summary table created in HTML illustrating the problem and its potential solution:
Problem | Solution |
---|---|
Csv File With Arabic Characters Is Displayed As Symbols In Excel | Convert the encoding of the CSV file to UTF-8-BOM |
And now let’s elaborate further .
When you export data to CSV format and try to view it in excel specifically, Excel expects the CSV file to be in a specific encoding, which is Windows-1252 or ISO-8859-1 (used by default in Excel). Since these encodings do not cover Arabic characters, you end up seeing question marks, boxes or other symbolic representations instead.
However, we can correctly display Arabic characters by converting the encoding of the CSV file to UTF-8-BOM (Byte Order Mark). UTF-8-BOM informs Excel about the encoding being used in the file, hence ensuring Arabic characters are rendered correctly.
Here’s how it can be achieved in Python:
import codecs
# Open the file in binary mode
with open("your_csv_file.csv", "rb") as f_in:
contents = f_in.read()
# Write out in 'utf-8-sig' mode
with codecs.open("new_csv_file.csv", "w", "utf-8-sig") as f_out:
f_out.write(contents.decode("utf-8"))
In this Python snippet, we first open our existing CSV file (“your_csv_file.csv”) in ‘rb’ (read binary) mode. This extracts the contents of the file conserving their original encoding. Then we write those contents into another CSV file (“new_csv_file.csv”) using ‘utf-8-sig’ (an alias for UTF-8-BOM). On opening this new file in Excel, the Arabic characters appear correctly instead of symbols.
So remember, understanding and adjusting to requisite encoding methods is crucial when dealing with multilingual text data to ensure accurate representation and interpretation of information across different softwares or systems.Certainly, I’m glad to delve into this topic. It’s often a challenge when Arabic characters in CSV files are not displayed correctly in Excel and instead, they show up as symbols or other unintelligible text. This is usually due to an encoding issue, where the software does not correctly interpret the character set.
CSV files are plain text files that represent tabular data in a simple format. When we input data with non-Latin characters such as Arabic into this file type, we might run into problems because of data encoding differences. Encoding is essentially a set of rules that dictate how character sets are represented in binary format.
The primary reason for the problem becomes evident when Excel opens these files using ANSI encoding by default. However, Arabic characters are typically written in UTF-8 encoding. This mismatch in encoding can cause Arabic characters to appear as unfamiliar symbols or gibberish.
But no need to worry as there’s a solution for it. Excel’s importing feature allows us to specify the correct encoding which solves this problem.
Follow these steps:
- Open Excel, go to the
xxxxxxxxxx
111Data
tab, then choose
xxxxxxxxxx
111From Text/CSV
.
- Select the CSV file you want to open.
- In the pop-open window, click on the drop-down list under
xxxxxxxxxx
111File Origin
. Here, try different encodings.
- For Arabic characters specifically, select
xxxxxxxxxx
111Unicode (UTF-8)
. But if that still doesn’t render the characters correctly, experiment with other Unicode options.
- Next, click on the
xxxxxxxxxx
111Edit
button. Now, you will be able to see your data with the correct characters in the preview area.
- Finally, load the data into your spreadsheet by clicking on the
xxxxxxxxxx
111Load
button.
While this method works more often than not, there may still be times when we encounter issues with displaying Arabic characters in CSV files. In such cases, one alternative we could consider is changing the system’s language settings. For example, if we’re using Windows, we can go to the Control Panel -> Region -> Administrative and then change the system locale to Arabic. Or perhaps, consider using tools dedicated to handling CSV data that comes with encoding support like LibreOffice or Google Sheets.
Even after all these detailed steps, it’s important to note that sometimes CSV just isn’t suitable for handling multi-language data. This is where JSON or XML could be a better alternative. They support UTF-8 encoding by default and don’t suffer from these same types of character display issues.
For further references please follow these links: Microsoft Docs – Connect to a Text or CSV file (Power Query), Microsoft Support – Import or export text (.txt or .csv) files Resolving character encoding problems in Excel, particularly when dealing with CSV files containing Arabic characters, is a challenge that can be tackled through multiple avenues. Excel has issues with certain encodings of non-ASCII languages like Arabic due to the different characters sets they use, which leads to the characters getting jumbled up and displayed as symbols.
One approach we can use to fix this involves:
Step 1: Open the CSV file containing Arabic characters in Notepad++ or Sublime Text editor. These editors have better support for using and understanding diverse character sets.
xxxxxxxxxx
Notepad++ > Encoding > Convert to UTF-8
After converting the file to UTF-8 character encoding, save it. This action helps ensure that the binary representation of the character set aligns with that which Excel uses: Unicode.
Step 2: Import your newly saved CSV file into Excel. Rather than directly opening the file, using the ‘Import’ functionality gives you more control over how Excel handles the data.
Choose the option to import text data, then navigate to the saved file.
xxxxxxxxxx
Excel > File > Open > Import > CSV File
Now, during the import process, you can specify details about the file type, delimiters (like commas in a CSV file), and the all-important encoding.
Step 3: Select the correct encoding during import – Here, select UTF-8
xxxxxxxxxx
File Origin > UTF-8
Specifying that the file uses UTF-8 encoding allows Excel to correctly interpret the bits into characters, showing your Arabic characters as expected rather than as regrettable symbols.
Another method plays nicely within Excel’s domain, leveraging ‘Microsoft’s Power Query’ feature.
Step 1: With your CSV file open in Excel, click on the Power Query tab in the top Ribbon. The ribbon is the banner at the top of the Excel window where various commands reside.
xxxxxxxxxx
Power Query Tab > From File > From CSV
This command opens a new dialog box where you’ll navigate to your saved CSV file.
Step 2: In the next screen, you’ll see an option called File Origin.”
xxxxxxxxxx
File origin > UTF-8
utf-8 encoding leads the pack for web and email traffic statistics demonstrating its effectiveness across use cases including Excel’s import scenarios.
These practical steps should help restore the legibility of your Arabic-laden CSV files within Excel. It’s worth noting that UTF-8 is widely used for encoding different scripts, making these steps not just a one-off but a general-case solution for similar encoding woes. While global codification of language is still an abstract ideal, these strategies ensure our data reflects how richly diverse our world is.
In my coding experience, I’ve frequently come across a common issue when it comes to importing CSV files: dealing with non-English characters, such as Arabic ones. Specifically, these characters often get displayed as symbols or gibberish in Excel.
### Why it happens:
The root of this problem lies within the encoding used when saving the CSV file. CSV files are typically saved in ASCII format, which only supports basic Latin characters. When you have characters extending beyond that range like Arabic, Russian, French, and other such languages, those characters can’t be properly recognized, resulting in them being displayed as symbols.
Consider the following example. You have a CSV file containing Arabic text:
شارع 1, بيت رقم 2
When imported into Excel, it appears something like this:
عمان ، رقم بيت 2
### Solutions:
There’s no one-size-fits-all solution to this problem, because it would depend, to some extent, on how your computer and Excel are set up. However, I can offer some potential routes you may use to explore and solve this issue.
#### Solution 1: Import the data into Excel, instead of opening it directly
Excel can handle various encodings during the import process. By effectively instructing Excel to import data from your CSV file, you can specify the encoding. Here’s how it’s done:
* Open Excel
* Go to ‘Data’ -> ‘Get External Data’ ->’From Text’
* Select your CSV file
* In the Text Import Wizard, select ‘Delimited’, then ‘Next’
* Check ‘Comma’, then ‘Next’
* Choose the correct encoding from the dropdown menu (You can try UTF-8 or Windows-1256 for Arabic)
* Click ‘Finish’
The exact path may vary a little based on your version of Excel, but the general method is similar.
#### Solution 2: Save your CSV with a BOM (Byte Order Mark)
If you’re generating your CSV files programmatically: an alternative way would be to add a UTF-8 BOM at the start of your file. Most modern text handling software will understand this as “This text should be read as UTF-8”. In Python, you’d do something like:
xxxxxxxxxx
import codecs
with open('arabic.csv', 'w', encoding='utf-8-sig') as f:
writer = csv.writer(f)
writer.writerow(['شارع 1', 'بيت رقم 2'])
This code uses the ‘utf-8-sig’ encoding to ensure the creation of a BOM.
#### Solution 3 – Save the file in UTF-16
You could also save the data in your CSV file with an encoding that Microsoft Excel reads correctly by default. UTF-16 or unicode – Little Endian, for instance.
xxxxxxxxxx
with codecs.open('arabic.csv', 'w', encoding='utf-16') as f:
writer = csv.writer(f, delimiter='\t')
writer.writerow(['شارع 1', 'بيت رقم 2'])
Bear in mind, though, that not all software handles UTF-16 well, so this approach might cause compatibility issues with non-Microsoft tools. Additionally, for larger datasets, this will take up considerably more space than UTF-8.
#### Additional Tips
It may be worth noting that there are some disadvantages to using a BOM, particularly in UTF-8 encoded files. For one thing, many applications don’t expect a BOM in UTF-8 files and might treat it as an unprintable character. Weigh these considerations against the needs and structure of your particular project.
Also, remember that if you tweak your code to use a specific encoding, other parts of your software stack need to be able to handle that encoding. Encoding issues can get really complicated really fast!
I hope these solutions were helpful. As always, it’s essential to test different approaches and choose the right solution depending on the specific needs of your project –Every developer’s journey!To display Arabic characters correctly in Excel, especially when dealing with a CSV file that has been displaying as symbols, we need to follow certain steps. Each step requires attention to detail and proper execution for the most optimal results.
1. Open a blank workbook: Instead of directly opening the CSV file in Excel, which often leads to improper character encoding, it’s more practical to create a new Excel file first.
Operations like this are important when dealing with encoding issues:
xxxxxxxxxx
NewWorkbook = xw.Workbook()
The Python package `xlwings` allows you to create a new Excel workbook using the above code.
2. Import data using ‘From Text’ tool: From your newly created Excel workbook, proceed to the ‘Data’ tab and select ‘From Text’. A window will pop up where you can browse and select the problematic CSV file with Arabic characters.
In this context, ‘From Text’ functions as a very handy tool, helping you perform actions like:
xxxxxxxxxx
DataImport = NewWorkbook.from_text('Path_to_CSV_File')
3. Select correct file type and encoding: While importing, make sure to select ‘65001: Unicode (UTF-8)’ from the ‘File origin’ dropdown menu under ‘Data preview’. This step helps to maintain proper encoding of the Arabic characters.
4. Delimit Columns: Ensure the right delimiters are used for separating columns. If you know that the CSV is comma-separated, choose the comma delimiter.
5. Load data: Finally, finish the wizard to load the data onto Excel. The Arabic characters should now display correctly.
These steps are crucial components to address this problem effectively.
Remember, issues related to incorrect display of special characters such as Arabic ones often arise from poor handling of character encoding. Properly indicating UTF-8 encoding during the import process into Excel saves everyone from a number of headaches.
Unfortunately, Excel does not automatically detect the correct encoding method for CSV files, which requires us to manually input it ourselves. But, understanding these steps and being able to correctly implement them, allows for smooth display of Arabic characters without them turning into meaningless symbols.
Aside from resolving the current issue, following these steps also ensures preserving data integrity to keep our work correctly representative of the original contents. The extraction of data from encoded files isn’t a mundane task but rather a technical one demanding awareness about the interpretive mechanism of different applications, such as Excel, towards character sets.
Sources:
– [Comma-Separated Values (CSV) Files](https://docs.microsoft.com/en-us/sql/integration-services/import-export-data/import-or-export-data-in-comma-separated-values-csv-format?view=sql-server-ver15)
– [Reading and Writing Excel Files in Python using Pandas](https://www.geeksforgeeks.org/reading-excel-file-using-python/)Overcoming Excel Language Barrier Issues
The scenario you described seems to happen quite often, especially when working with non-western characters like Arabic in CSV files. Fortunately, there are several tools and techniques to overcome this.
Excel’s ‘Get External Data’
This feature in Excel is something I personally use regularly. Instead of opening your CSV file directly, import it using ‘Get External Data’ function:
xxxxxxxxxx
Data -> Get External Data -> From Text
During the importing process, Excel will prompt you to specify the file type and encoding. Here, you need to select 65001: Unicode (UTF-8). This setting helps Excel understand how to decode the Arabic characters.
Google Spreadsheets
Another way is to upload your CSV file to Google Spreadsheets. Google Sheets has remarkable support for a variety of character sets including Arabic. After uploading your file, you can then download it as an Excel file:
xxxxxxxxxx
File -> Download -> Microsoft Excel (.xlsx)
This way, you won’t have trouble opening the file in Excel with correct display of Arabic characters.
Notepad++ and UltraEdit
Both are excellent text editors. You can use them to change the Encoding of the CSV file to UTF-8-BOM and save the changes. Later, when you open the CSV file in Excel, the Arabic characters should appear correctly.
Here’s how to do it in Notepad++:
xxxxxxxxxx
Encoding -> Convert to UTF-8-BOM -> Save
And in UltraEdit:
xxxxxxxxxx
File -> Save As -> Encoding -> UTF-8 -> Check "Add BOM (Byte Order Mark)" -> Save
Pandas library in Python
If you’re comfortable with coding, you might find value in using Python’s Pandas library. With just a few lines of code, you can load the CSV data, specifying the correct encoding.
Here’s a basic example of how you could do that:
xxxxxxxxxx
import pandas as pd
df = pd.read_csv('yourfile.csv', encoding='utf_8')
df.to_csv('newfile.csv', index=False, encoding='utf_16_le')
In this sample Python script, we’re importing the pandas library, reading a CSV file (‘yourfile.csv’) with ‘utf_8’ encoding, and writing it back out with ‘utf_16_le’ encoding, which Excel understands as Unicode.
A combination of these tools and techniques should help you navigate language barrier issues in Excel and ensure your Arabic characters display as they should. Remember to experiment and find what works best for your particular situation.
For more in-depth information about Character Encoding, you may refer to the IBM documentation. To learn more about pandas library in Python, the official Pandas Documentation is a good resource.When encountering a CSV (Comma-Separated Values) file with Arabic characters displayed as symbols in Excel, this usually means that the file is encoded in UTF-8 but Excel is not properly interpreting it. UTF-8 is an extension of ASCII and can display characters from all languages, including Arabic. However, Excel often still uses ANSI or ISO 8859-1 as its default encoding, leading to misinterpretation of non-Latin character sets.
To solve this issue, you will have to convert your Arabic UTF-8 encoded CSV file into a format that Excel can display correctly. There are two primary methods that I recommend: one using an online tool and another using Python programming.
xxxxxxxxxx
#Using an Online Tool:
There are various free online tools, such as EditPad or SublimeText, that allow you to open a file and save it in a different character encoding. Follow these steps:
xxxxxxxxxx
- Open the CSV file in the text editor
- Select 'UTF-8' for the decoding
- Save the document again under 'File' > 'Save with encoding' > 'Unicode - A little endian (UTF-16)'
- Re-open the now Unicode-encoded file in Excel
This gives Excel the hint that it’s opening a Unicode file instead of an ANSI/ISO 8859-1 file, and Excel should then interpret the characters correctly.
xxxxxxxxxx
#Using Python:
Python’s built-in CSV reader can handle UTF-8 encoded data, making it ideal for this task.
The pandas library simplifies the conversion process to just a couple of lines:
xxxxxxxxxx
import pandas as pd
dataframe = pd.read_csv("UTF8_encoded_file.csv", encoding='utf-8')
dataframe.to_csv("new_unicode_file.csv", index=False, encoding='utf-16')
In the Python code above:
– The first line imports the pandas library.
– Next, we read our UTF-8 encoded CSV file.
– Then we write the DataFrame containing our data back out to a new CSV file, specifying \n “UTF-16” encoding to make it compatible with Excel.
– The argument `index=False` prevents pandas from writing out row indices.
The resulting CSV file will be double-byte Unicode, and Excel will display the Arabic characters correctly.
It’s important to remember that Excel also supports loading data directly from a Python script via the pandas DataFrame to_excel function. This could negate the need to handle CSV files and their potential encoding issues. Remember, it depends on the requirements at hand!
Regardless of the method you choose, your desired results: readable Arabic characters in Excel, should be achieved!Working with CSV files that contain language data such as Arabic might sometimes lead to the file being displayed as symbols when viewed in programs like Excel. The reason behind this is often an encoding issue. When you save a file, it’s written in a specific format or encoding. Excel may not automatically identify the correct data encoding for languages that use non-Latin characters, like Arabic. Thus, the information will appear as unrecognizable symbols instead of the intended text.
In order to fix this issue, the solution could be to change the encoding to UTF-8 while reading or saving the CSV file. UTF-8 (8-bit Unicode Transformation Format) can handle any character in the Unicode standard which includes different scripts such as Arabic.
To illustrate, let’s consider Python as an example. Here is a possible code snippet showing how to explicitly set the encoding to ‘utf-8’ during the reading and writing process:
xxxxxxxxxx
import pandas as pd
# Read the .csv file and specifying the encoding
df = pd.read_csv('filename.csv', encoding='utf-8')
# Save the DataFrame into a new .csv file with specified encoding
df.to_csv('new_filename.csv', encoding='utf-8', index=False)
Here, Pandas read_csv() method reads the CSV file into a DataFrame and specifies the file’s encoding as ‘utf-8’. Similarly, to_csv() method writes the DataFrame back into CSV format using utf-8 encoding, making it easier to view in programs like Excel.
Apart from programming, manual conversion is also possible by importing the CSV file directly into Excel:
– Open the Data menu in Excel.
– Select ‘From Text/CSV’.
– Choose your CSV file.
– The file preview won’t show the content correctly. On the File Origin dropdown, select ‘65001: Unicode (UTF-8)’.
– Excel will now import your CSV file, and it should display the Arabic characters correctly.
In general, if there are encoding differences between systems or applications used to view/open/edit the data, compatibility issues may arise. Therefore, those working around CSV and Excel need to be aware of potential encoding issues, particularly when processing non-Latin character text like Arabic.
It is also important to note that other factors could come into play, depending on use case specifics such as operating system, local language settings, versions of software being used among others. Adjustments might be required accordingly. As is often mentioned, test everything and make backups!
As far as online resources go, [W3C Internationalization] offers a comprehensive guide on changing encodings.
Also, this StackOverflow question provides helpful guidance in resolving the issue: [Python pandas, Error tokenizing data].
Dealing with a CSV file that has Arabic characters displayed as symbols in Excel can be an annoying experience, especially if you don’t understand why it happens and how to solve it.
In the realm of text encoding, certain settings can cause distortions like these unwanted symbols. These symbol substitutions occur due to the mismatch between the character encoding of the CSV file itself and Excel’s default encoding.
To resolve this, we need to look into the specifics of CSV files and Excel’s role in reading them:
- Understanding Encoding: Text encoding is a system where each letter, number, or symbol is termed by a specific numeric code. Because various systems handle this differently, inconsistencies and misinterpretations happen, leading to gibberish or symbolic text. Arabic text often suffers in such scenarios due to its unique set of characters. Look for UTF-8 encoding while saving Arabic content in Excel.
- CSV File Settings: As plain-text files, CSVs don’t contain font or style specifications. Any application opening them will interpret the text based on default settings. If the CSV was saved using one type of character encoding–like UTF-8–and another program uses a different default–such as ASCII–it causes the unusual symbols.
xxxxxxxxxx
121your_dataframe.to_csv('file_name.csv', encoding='utf-8') # Python
2
- Excel Settings: When you open a CSV in Excel, it doesn’t ask which encoding to use. It picks ANSI by default, not best suited for Arabic. A workaround is to import the data via the Data Import widget and select UTF-8 there.
Convert this mishmash into clear Arabic by matching the character encoding across all interfaces that engage with your CSV. Save your CSV file in UTF-8 format, and when importing to Excel, ensure it’s interpreted in the same way. Alternatively, consider switching to other platforms that offer better support for encoding, like Google Sheets or tech-friendly environments like Python or R programming.
More depth on encoding can be found at this source.
DataFrame Code To Save CSV In UTF-8 (Python) | How to Adjust Encoding in Excel |
---|---|
xxxxxxxxxx 1 1 1 your_dataframe.to_csv('file_name.csv', encoding='utf-8') |
Data Tab -> From Text -> Delimited -> 65001: Unicode (UTF-8) |