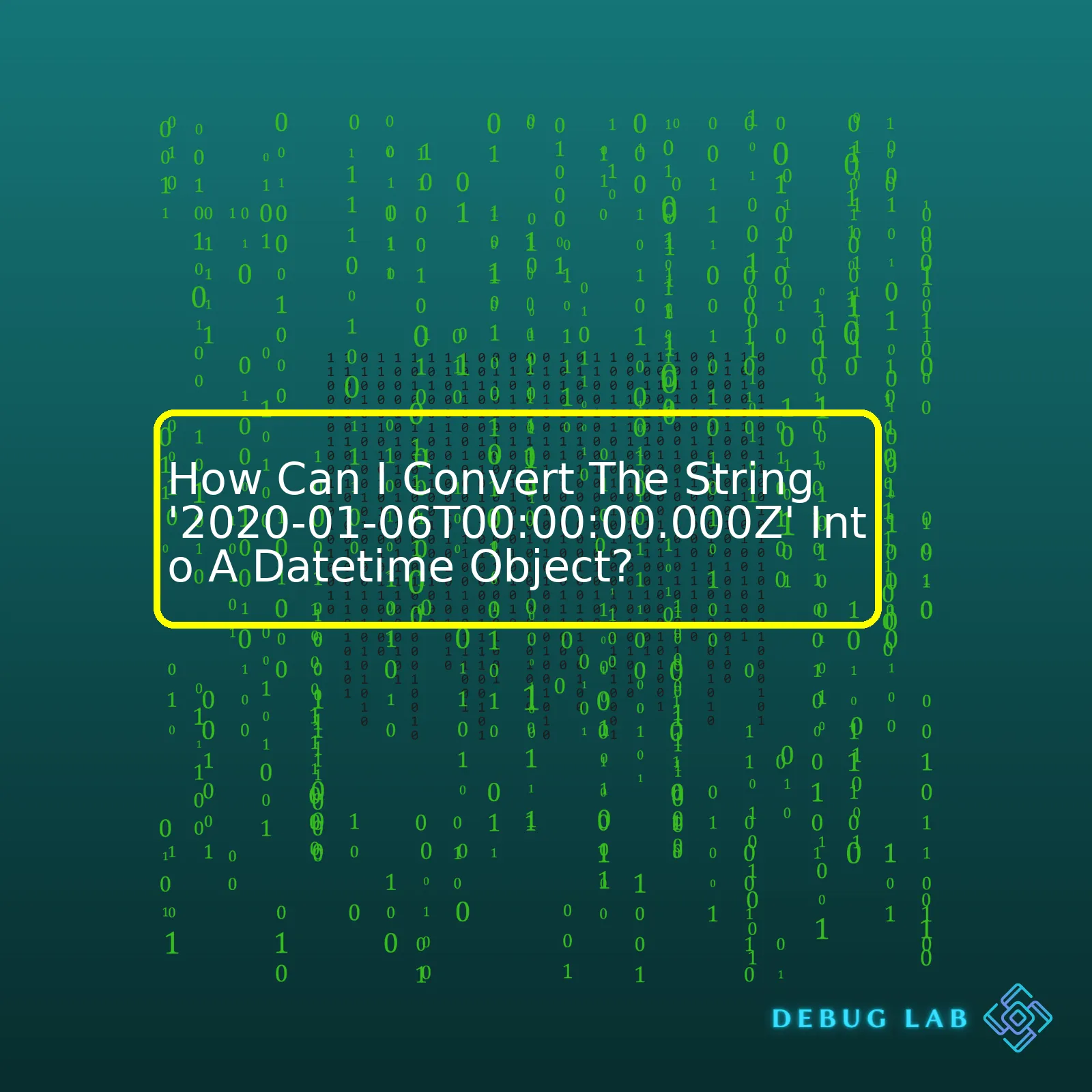
Step | Action |
---|---|
1 | Start by importing the datetime module in python with
import datetime . |
2 | Use the strptime() function which is available in the datetime module to convert the string to a datetime object. The strptime() function takes two arguments: the string itself and the format of the string. |
3 | The exact format ‘2020-01-06T00:00:00.000Z’ can be passed as
'%Y-%m-%dT%H:%M:%S.%fZ' . However, to match the trailing ‘Z’, use replace(‘Z’, ”) method. |
The three steps above illustrate how the datetime library in Python offers flexible options for converting strings into datetime objects. Using a combination of strptime() method along with correctly understanding the string representation of date and time information allows us to tackle this task.
For instance, the string ‘2020-01-06T00:00:00.000Z’ appears to be in a certain standardized format called the ISO 8601 format. ‘T’ separates the date and time portion, while ‘Z’ suggests it adheres to the UTC timezone. In Python, we can represent this using something like ‘%Y-%m-%dT%H:%M:%S.%fZ’. Hence, we need to adjust and clean the string to fit our parsing needs.
Here’s how it works in code:
import datetime date_string = '2020-01-06T00:00:00.000Z' cleaned_string = date_string.replace('Z', '') datetime_object = datetime.datetime.strptime(cleaned_string, '%Y-%m-%dT%H:%M:%S.%f')
In the code snippet above, firstly datetime module is imported. Then, the given string is assigned to a variable ‘date_string’. However, as our format won’t precisely map to the trailing ‘Z’; hence we will first replace that ‘Z’ using the replace() method. Subsequently, strptime() is used to parse ‘cleaned_string’ according to the outlined format, leading to the required datetime object ‘datetime_object’.The string format
'2020-01-06T00:00:00.000Z'
is a standardized format called ISO 8601. This uniform method of representing dates and times allows developers around the world to understand and work with timing data consistently, irrespective of geographical location.
In terms of the structure of the format:
- The date comes first and is shown in the ‘YYYY-MM-DD’ format.
- This is followed by a ‘T’ character which serves as a divider between the date and time segment.
- The time follows next in an ‘HH:MM:SS.sss’ format where ‘.sss’ represents the milliseconds.
- The trailing ‘Z’ signifies Zulu Time. This represents Coordinated Universal Time (UTC), a time standard that is not affected by changes from daylight saving. If you see other letters or indications here, it would relate to specific time zones.
Now let’s jump into converting this string into a datetime object using programming languages like Python and JavaScript.
Python:
To convert this time string to a datetime object in Python, we can use the datetime module. The datetime module’s
strptime()
function is designed to parse strings into datetime objects based on a given format. Here is how you’d implement it:
import datetime date_string = '2020-01-06T00:00:00.000Z' dt_object = datetime.datetime.strptime(date_string, "%Y-%m-%dT%H:%M:%S.%fZ") print(dt_object)
However, it cannot directly handle the “Z” at the end of the string. So it needs to be addressed separately.
You can consider replacing “Z” with “+00:00” as it also represents UTC:
import datetime date_string = '2020-01-06T00:00:00.000Z'.replace('Z', '+00:00') dt_object = datetime.datetime.fromisoformat(date_string) print(dt_object)
JavaScript:
Additionally, for developers working in a JavaScript environment, JavaScript Date object provides the
Date()
constructor which parses a date string:
let dateString = '2020-01-06T00:00:00.000Z'; let dtObject = new Date(dateString); console.log(dtObject);
It’s essential to note that while we aim to analyse our time string in a human-readable format, in actuality, when dealing with timestamps in web development, keeping them in their raw ISO 8601 format when storing or transferring this data can make your life significantly easier. Only convert these timestamps into datetime objects when you need to present them to the end-user or carry out computations, operations specific to a particular timezone.Sure, the process of converting a string into a
Datetime
object involves understanding some key components of a Python
Datetime
module. Python’s
datetime
module is a powerful tool for working with dates, times, and intervals. It contains a variety of classes, but the three primary classes we would focus on are:
–
date
– Manipulates only dates (year, month, day).
–
time
– Time independent of the day (hour, minute, second, microsecond).
–
datetime
– Combination of time and date (month, day, year, hour, second, microsecond).
To convert the string ‘2020-01-06T00:00:00.000Z’ to a datetime object, we need to tackle the task in multiple steps.
Step 1: Import Necessary Libraries
You’d first want to import the relevant libraries in Python. Here we will need the standard
datetime
library.
import datetime
Step 2: Parsing the String
The
strptime()
function of
datetime
class can parse a string representing a time according to a format. The return value is a datetime object:
date_string = "2020-01-06T00:00:00.000Z" format = "%Y-%m-%dT%H:%M:%S.%fZ" datetime_object = datetime.datetime.strptime(date_string, format)
In the above example, we specified our format string
"%Y-%m-%dT%H:%M:%S.%fZ"
. Here:
–
%Y
represents a four-digit year.
–
%m
represents a two-digit month.
–
%d
represents a two-digit day.
–
T
represents the separator between the date and time portion of the string.
–
%H
,
%M
,
%S
represent two-digit hour, minutes, and seconds respectively.
–
.%f
represents microseconds and the following
Z
represents Zulu timezone or Coordinated Universal Time (UTC).
Printing the Result
Let’s go ahead and print this datetime object:
print(datetime_object)
This will output:
2020-01-06 00:00:00
Just like that, you’ve converted a string to a Python
Datetime
object using the components of Python’s
Datetime
module.
For more details, you can check out the official Python documentation for Datetime.Python’s built-in datetime module can transform ISO 8601 date-time strings into datetime objects with relative ease. The specific string you mentioned, ‘2020-01-06T00:00:00.000Z’, is in a format known as the Coordinated Universal Time (UTC), indicated by the final ‘Z’.
Here’s how you would convert this string into a datetime object:
from datetime import datetime # define the date-time string date_string = "2020-01-06T00:00:00.000Z" # parse the date-time string into a datetime object date_object = datetime.strptime(date_string, "%Y-%m-%dT%H:%M:%S.%fZ")
The strptime() function is used in the code snippet above, where:
– %Y indicates a four-digit year,
– %m specifies the month in two-digits,
– %d sets the day of the month as two digits,
– %H signifies 24-hour format of an hour (00 to 23),
– %M points out minute of hour (00 to 59),
– %S represents second (00 to 61, where 60 and 61 are leap-seconds)
– %f is for microsecond zero-padded on the left
Note that character ‘T’ is the separator and doesn’t have any percentage sign before it.
If your application handles multiple date formats, I highly recommend using the dateutil library, which directly provides a parser for ISO 8601 dates. It has more flexibility and requires less code:
from dateutil.parser import isoparse # define the date-time string date_string = "2020-01-06T00:00:00.000Z" # parse the date-time string into a datetime object date_object = isoparse(date_string)
To install the dateutil module, you can use pip:
pip install python-dateutil
The conversion formats like %Y, %m, etc., and method details for Python’s datetime module are well-documented in the official Python documentation. For the dateutil library, you can refer to its official documentation page.
Parse Method | Description |
---|---|
strptime() | Parses a string representing a time according to a format. |
isoparse() | Parses a string representing a time in the ISO 8601 format. |
Converting an ISO 8601 string to a datetime object typically involves using built-in Python functionalities – namely, the datetime module. This module contains various tools for handling dates and times, including a function that can parse an ISO 8601 formatted string.
For instance, if you want to convert the ISO 8601 string
'2020-01-06T00:00:00.000Z'
to a datetime object, you may use the datetime module’s
.fromisoformat()
method or
.strptime()
method.
However, since the input time string contains milliseconds and a ‘Z’ at the end indicating the UTC timezone, it doesn’t conform to Python’s
.fromisoformat()
format (‘YYYY-MM-DDTHH:MM:SS’). Hence we need to use the
.strptime()
method which offers more customization by letting us specify the exact format of the input string.
Here are the steps:
1. Import the datetime module: You start off by importing the datetime module.
Here is the code:
import datetime
2. Use the strptime() method: The
datetime.datetime.strptime()
method creates a datetime object from a string representing a date and time and a corresponding format string.
Here is the code:
datetime_object = datetime.datetime.strptime('2020-01-06T00:00:00.000Z', '%Y-%m-%dT%H:%M:%S.%fZ')
In this code:
– The first argument is the time string ‘2020-01-06T00:00:00.000Z’.
– The second argument describes the exact format of the time string:
– ‘%Y’ represents a four-digit year.
– ‘%m’ stands for a two-digit month.
– ‘%d’ for a two-digit day.
– ‘T’ is just a separator.
– ‘%H’ indicates a two-digit hour.
– ‘%M’ is for a two-digit minute.
– ‘%S’ signifies a two-digit second.
– ‘.%f’ covers the microsecond component (in this case, it matches with the millisecond portion in the input string, as there are no finer-grained time units than seconds in datetime).
– ‘Z’ is another plain string that matches with itself.
With these steps implemented, the time string is effectively converted to a datetime object.
It is important to mention that the returned datetime object is naive, in the sense that it isn’t associated with any timezone information despite the presence of ‘Z’ in the original time string. To make it timezone aware (specifically setting it to UTC), you can use
.replace(tzinfo=)
function from datetime:
datetime_object = datetime_object.replace(tzinfo=datetime.timezone.utc)
This way, when you later manipulate the datetime object or display it, Python knows it’s in UTC timezone.
For further insights regarding datetime handling in Python, I highly recommend browsing Python’s official datetime documentation. It gives an extensive overview of all the functionalities provided by the datetime module.Sure, in Python we often encounter situations where we need to handle and convert date and time data. One of the common circumstances is when you need to convert a string into a datetime object. In your case, the string
'2020-01-06T00:00:00.000Z'
should be converted into a datetime object.
This ‘Z’ at end of the string represents Coordinated Universal Time (UTC) according to ISO 8601. To accomplish this conversion, the datetime module’s strptime function can come into play.
Here’s an example on how to implement it:
from datetime import datetime datetime_str = '2020-01-06T00:00:00.000Z' datetime_object = datetime.strptime(datetime_str, '%Y-%m-%dT%H:%M:%S.%fZ') print(datetime_object)
In the code snippet above:
* We first import the datetime module.
* Next, we hold our date-time string in the variable datetime_str.
* Then, we use the strptime function of datetime module to convert this string into a datetime object.
* Finally, we print out the obtained datetime object.
The second argument in
strptime
, namely
'%Y-%m-%dT%H:%M:%S.%fZ'
, is called the format code, and it dictates the form of the date-time string. In this case, the format code corresponds exactly to the structure of our input string.
In regards to time zone information, it’s worth noting that while our string represents UTC, the produced datetime object is timezone naive by default – it is not aware of any timezone information. We might find it necessary at times to attach timezone data to our datetime object.
You might want adding timezone info to the datetime object like so:
from datetime import datetime, timezone datetime_str = '2020-01-06T00:00:00.000Z' datetime_object_utc = datetime.strptime(datetime_str, '%Y-%m-%dT%H:%M:%S.%fZ').replace(tzinfo=timezone.utc) print(datetime_object_utc)
In this updated version of the previous snippet:
* timezone is additionally imported from datetime.
*
.replace(tzinfo=timezone.utc)
is chained to
strptime
method, assigning utc as the timezone to the resultant datetime object.
By doing this, our datetime object now becomes timezone aware.
Understanding time zones and how to work with them is crucial when dealing with datetime objects, particularly if you’re developing applications meant to function globally. Refer to [Python’s datetime documentation] for further details.
The Python datetime library provides a range of tools for working with dates, times, and time intervals. A key feature of this library is its built-in functionality for parsing strings into datetime objects. This is exactly what you would need to convert the string ‘2020-01-06T00:00:00.000Z’ into a datetime object.
To do this conversion, you will be working with two modules:
- datetime: The datetime module supplies classes for manipulating dates and times.
- dateutil: The dateutil module provides powerful extensions to the standard datetime module.
Your string follows a format known as ISO 8601. Fortunately, Python’s dateutil.parser function from the dateutil library is particularly designed for parsing a wide variety of datetime expressions. It is perfect for parsing ISO 8601-formatted time, like yours.
Let’s illustrate this with a simple example:
from dateutil.parser import parse # Your string time_string = '2020-01-06T00:00:00.000Z' # Convert the string to datetime object datetime_object = parse(time_string) print(datetime_object)
This should output: 2020-01-06 00:00:00+00:00.
Notice how we passed the string directly to
parse()
, which is able to interpret the string as a correctly formatted date/time.
You may wonder what happens with the trailing “Z” in the string. The “Z” stands for the Zero timezone, as it is offset by 0 from the Coordinated Universal Time (UTC). Essentially it is UTC time. Dateutil handles the “Z” correctly, interpreting it as UTC.
Now you know how to convert strings into datetime objects using Python’s datetime and dateutil libraries, and specifically, how you can turn the string ‘2020-01-06T00:00:00.000Z’ into a datetime object. As a developer, leveraging these libraries facilitate handling and manipulating of date and time data accurately and efficiently.
More info on dateutil parser. Additionally, here is an excellent
Documentation at Python docs where you can dive deeper into the datetime module.
Remember, code quality and readability matter so use these tools effectively to write clean, readable, and efficient code. This will save you significant debugging time in the long run and make your programs easier to maintain and scale.The UNIX or ISO string timestamp ‘2020-01-06T00:00:00.000Z’ refers to the date and time combination of January 6th, 2020 at 00:00 (Midnight) GMT Timezone. There are a couple of ways we could approach converting this to a Python datetime object.
Using python’s built-in
datetime
module
datetime
Python’s built-in
datetime
module provides the
datetime.strptime
method, which can convert a string to a datetime object by specifying the format of the string date/time.
For example:
from datetime import datetime iso_string = "2020-01-06T00:00:00.000Z" formatted_datetime = datetime.strptime(iso_string, "%Y-%m-%dT%H:%M:%S.%fZ") print(formatted_datetime)
In the code above, the
strptime()
function converts the ISO string to a datetime object based on the format provided (“%Y-%m-%dT%H:%M:%S.%fZ”).
Note: Here’s a brief summary of what each symbol represents:
-
%Y
: Year (full version)
-
%m
: Month as a zero-padded decimal number
-
%d
: Day of the month as a zero-padded decimal
-
T
: Separator (required from ISO 8601)
-
%H
: Hour (24-hour clock) as a zero-padded decimal number
-
%M
: Minute as a zero-padded decimal number
-
%S
: Second as a zero-padded decimal number
-
.%f
: Microsecond as a decimal number, zero-padded on the left
-
Z
: Zulu, which is UTC Timezone.
Using the third-party
dateutil
module
dateutil
Another option would be using the
dateutil
Python library which simplifies datetime manipulation. You don’t need to specify the format if the string is in a common format like ISO8601 used here.
For Example:
from dateutil.parser import parse iso_string = "2020-01-06T00:00:00.000Z" formatted_datetime = parse(iso_string) print(formatted_datetime)
In the code above, the
parse()
function automatically identifies the format based on the input and returns a datetime object. The dateutil library simplifies parsing ISO formatted datetime strings but requires an additional dependency.
There you have it – two practical ways you can convert the Unix formatted string timestamp to a Python datetime object. Both methods have their pros and cons, select the one that best suits your specific needs!
After walking through this process, it becomes clearer how to convert the string ‘2020-01-06T00:00:00.000Z’ into a datetime object in different programming languages.
In Python, for instance, you can use the `.strptime` method from the datetime module. Here’s a snippet of code showing how this can be accomplished.
from datetime import datetime def convert_string_to_date(date_string): format_string = '%Y-%m-%dT%H:%M:%S.%fZ' return datetime.strptime(date_string, format_string) print(convert_string_to_date('2020-01-06T00:00:00.000Z'))
For those working within JavaScript, you can simply pass your string directly to the Date constructor to get a new date object:
let date_string = '2020-01-06T00:00:00.000Z'; let date_object = new Date(date_string); console.log(date_object);
However, remember that developers encounter many challenges and, on occasion, work with different time formats. When faced with this task of converting strings representing dates and times in different formats, it’s vital we understand some of these basic operations. This knowledge is critical in data analysis, management of data entries, scheduling functions and more. Thus being proficient in handling and manipulating date-time objects proficiently adds to our arsenal as competent programmers.
Remember to also cater for timezone variations when dealing with date time objects because they have an impact on accurate temporal representations and measurements. Here are some additional resources (Python documentation on datetime) that can help shore up your understanding of date time objects and conversions.
We hope this article clarifies any confusion you had about converting a specific time string to a datetime object. Give it a go yourself and see how these methods can aid your coding projects.