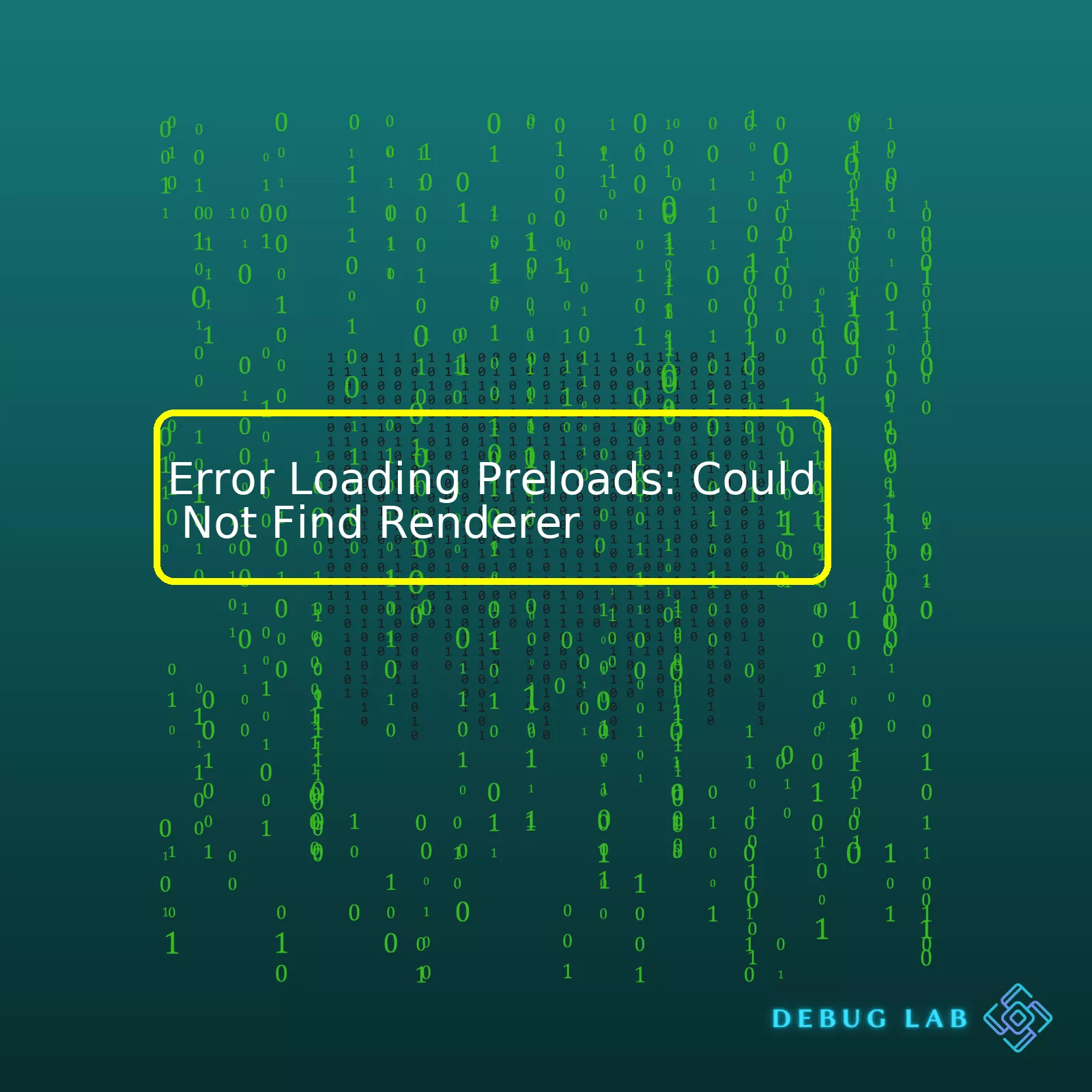
Error Type | Problem Description | Possible Solution |
---|---|---|
Error Loading Preloads: Could Not Find Renderer | This error typically occurs when the application isn’t able to locate the renderer file, which is a crucial part of rendering views in your application. | To resolve this, make sure the renderer file is present in its correct location and also properly referenced in your code. |
The “Error Loading Preloads: Could Not Find Renderer” is one of the common issues faced by developers. This error generally pops up when the system fails to locate the renderer file, a pivotal component for effectively presenting the visual elements or ‘views’ in an application. A renderer file essentially dictates how data or user interface behaves on your browser screen. When the renderer goes missing or ambiguously referenced, the application fails to execute and thus exhibits the said error.
To mitigate this issue, there are few preliminary steps you can undertake:
- Firstly, ensure that the renderer file is not absent or inaccurately placed within the directory structure. Each framework has its conventional practices and conventions about the file-location – violating which could spark off unnecessary complications.
- Secondly, cross-verify if the reference links to the renderer file in your source code are correctly written. It includes exact naming (case-sensitivity matters in some environments) and relative path addressing. Inaccurate or broken references can often lead to “File not found” situations.
Learning from mistakes is an invaluable aspect of programming. Keep note of such errors for future preventive measures. Pay closer attention while setting file pathways and referencing them elsewhere in your code. Proper documentation and following standard coding practices can save a lot of debugging time and effort due to these types of errors. Keep exploring more ways to optimize error handling to foster a smoother development journey.
Here is a simple example of how a renderer might be defined and used in an Express.js framework:
// Requiring express as our web server
const express = require('express');
// Creating an express instance
const app = express();
// Registering ejs as the view engine
app.set('view engine', 'ejs');
// Route handler for GET /
app.get('/', function(req, res) {
res.render('index');
});
// Start server on port 3000
app.listen(3000);
In the above code segment, ‘ejs’ is set as the rendition engine using the ‘view engine’ property. We have declared a route-handler for GET request to root ‘/’, where ‘index’ view will be rendered upon any HTTP request to localhost at port 3000.
An online reference link: [Handling Errors in Express](https://developer.mozilla.org/en-US/docs/Learn/Server-side/Express_Nodejs/skeleton_website) offers comprehensive insight into handling errors in Express.js, a widely accepted Node.js framework. However, the understanding of handling errors like ‘Could Not Find Renderer’ flawlessly come with experience and persistent learning to identify, analyze and resolve such glitches efficiently.
When encountering an “Error Loading Preloads: Could Not Find Renderer”, there are a few potential causes to consider as a professional coder. For reference, we refer to ‘preload’ in coding language as scripts that load before your main script runs – it’s a chance for you to set configurations or customize certain process settings.
Let’s start with the primary cause, which is related to file path issues. If the file path to the renderer script defined in the preload attribute isn’t correct, this error message will most likely appear. It points to a 404 error when the system tries to retrieve the given script but fails because it doesn’t exist at the specified location. An example of specifying a renderer script within the context of preloading in HTML might look something like:
xxxxxxxxxx
<link rel="preload" href="/path/to/myScript.js" as="script">
In this instance, the href attribute specifies the location of the resource and “as” defines its type. If “/path/to/myScript.js” doesn’t point towards a valid destination, a “Could Not Find Renderer” error will be thrown.
Another possible cause of this error could be due to incorrect setup when using bundled JavaScript libraries (like Webpack or Rollup). These tools usually compile and bundle many JS files into one, and if the configuration is wrong, they might neglect to include the necessary renderer.
Even the order of loading scripts can cause problems, especially in relation to how the scripts correlate. Imagine your code is similar to this:
xxxxxxxxxx
<script src="renderer.js"></script>
<script src="main.js"<</script>
If ‘main.js’ depends on functions or elements from ‘renderer.js’, but the renderer script fails to load first, it would display an error “Could Not Find Renderer”.
If your project includes external dependencies, you’ll want to make sure that these are correctly installed and loaded. When those libraries are missing, not properly installed, or have incompatible versions, they might not run appropriately or even trigger errors that others might misinterpret as “Error Loading Preloads: Could Not Find Renderer”.
Finally, bugs or inconsistencies within the browser itself might also result in this error. Browsers interpret the HTML, CSS, and JavaScript that forms any web page, and their unique interpretations may lead to distinct behaviors. For instance, newer APIs or experimental features might not be fully supported across all browsers. This is why it’s always good practice to test your application on different browsers. Mozilla’s Cross Browser Testing Documentation provides more about this topic.
Remember, the proper investigation of such errors requires comprehensive knowledge of your project structure, applied technologies, and third-party libraries. Equally important is understanding the environment where your code runs, as the issue might emerge from the operating system, installed Node.js version or any other relevant external factor. You should focus on addressing these areas while investigating the root cause behind the error message “Error Loading Preloads: Could Not Find Renderer”. This meticulous analytic approach often aids in finding the origin of the problem swiftly and accurately.
In the world of web development, one of the common issues you may encounter is Error loading preloads: Could not find renderer. Understanding this issue entails a profound knowledge about renderers and the roles they play in browsers. Renderers are the core components of any browser that lay out and paint a web page for you to interact with. They are responsible for taking the HTML, CSS, and JavaScript code and turning it into a viewable webpage.
When you see the error “Could not find renderer,” it usually implies that your application couldn’t locate the appropriate rendering context required by your preload script. Your preload script must be loading or looking for something that requires rendering context, such as WebGL or Canvas APIs, that doesn’t exist at the time it’s running.
To dive deeper, let’s take a look at JavaScript’s role in this process. When a website page loads, JavaScript files are loaded asynchronously and executed. Each file is highly reliant on the loading and execution order. The problem begins when you’re trying to use a piece of JavaScript that hasn’t been fully loaded and executed at that point – hence the error “Could not find renderer.”
- Incorrect path: One primary reason could be as simple as having an incorrect path specified for your preload. In this case, your app won’t find the `renderer.js` file because it’s looking in the wrong place. Make sure to provide the correct relative path.
- Different Environments: Different environments might have different ways of dealing with paths, including what they treat as the ‘root’ location. It’s always better to use absolute paths using something like
xxxxxxxxxx
111path.resolve()
rather than a manually typed string. This way, your program will determine the right path regardless of the environment in which it’s running.
- Compatibility issues: There’s also a possibility of compatibility issues between versions of Electron and your system or other installed software. Therefore, keeping your Electron version up-to-date can help circumvent such issues.
Here’s a sample code using absolute path, where __dirname refers to the directory from which the currently executing script is running:
xxxxxxxxxx
appWindow = new BrowserWindow({
width: 800,
height: 600,
webPreferences: {
preload: `${__dirname}/preload.js`
}
});
A major lesson here is that timing is everything in JavaScript. Everything has to load in the right order. So, ensuring proper sequencing and synchronization amongst scripts will help avoid such issues.
And lastly, another smart move is to watch your console. The Electron runtime often spits out sensible errors to guide you in the right direction towards the missing renderer. Check those error tracebacks if all else fails. Observe the type of exceptions that were thrown around the time of the error, conduct Google searches to understand these issues or visit developer communities like StackOverflow for additional guidance.
References
- ElectronJS Official Documentation
- StackOverflow discussion on similar error.
Problems with loading preloads often stem from the underlying intricacies of computer programming. The error “Could Not Find Renderer” may imply a variety of root causes to observe and investigate as part of your troubleshooting efforts. Rendering issues commonly arise due to software errors or compatibility issues which can be complex to resolve.
However remember, there’s no problem that can’t be resolved effectively using a systematic approach to troubleshooting. Following a concerted process enables us to isolate the issue systematically. Here are techniques you can employ while trying to overcome this common error:
Run an Update
The first step in tackling any error message is to ensure your software is current. If there’s been a new release since your last update, it could offer solutions to known issues causing your error. Use the
xxxxxxxxxx
npm update
command or equivalent to update your technology.
Check Your Code
At times, the problem may lie within your code. Minor oversights such as incorrect file paths, typographical errors, or missing dependencies could cause this error. Therefore, it’s essential to review your scripts meticulously to spot potential anomalies.
That includes examining your ‘renderer.js’ file if you have one.
xxxxxxxxxx
// Ensure the file structure for server invocation is correct
const server = require("/path/to/your/server");
server.listen(port, "localhost", function () {
….
});
Ensure Compatibility
There might be compatibility issues somewhere in your program stack; they can also trigger rendering issues. Make sure to check the compatibility between dependencies, not just against your operating system. Reducing the room for conflict helps in the smooth running of applications.
Diagnose With Developer Tools
Debugging tools offer a powerful way to diagnose problems in your application by letting you see ‘under the hood’. Modern browsers come with rich developer tools that provide deep insights into what’s happening inside your application.
To open these tools, typically you would do:
xxxxxxxxxx
// This sets up the debug environment
require('electron-debug')({showDevTools: true})
Often, a console log of the rendering process can flag where the issue lies. You may need to enable verbose logging options depending on the information required.
Remember, finding the right solution begins with understanding the problem correctly. Using in-depth monitoring, coupled with comprehensive error and performance data gives that understanding. Going through each potential cause systematically narrows down the likely reasons for your issue. Now you’re equipped with more knowledge than before about how to tackle standard rendering errors like “Could Not Find Renderer”.
For more discussions around handling this issue, visit online coding forums such as Stack Overflow and search for similar troubleshooting threads. Don’t hesitate to reach out to your community when you need help. There’s a possibility someone else has faced the same issue and they may have documented their solution online. This approach works wonders not only for dealing with coding errors but developing your technical skills overall.Errors can significantly impact the smooth running of any application. When dealing with preloads, encountering issues such as “Error loading preloads: Could not find renderer” could disrupt performance drastically, affecting the user experience negatively.
There are various reasons why you might encounter the “Could Not Find Renderer” error when loading preloads.
Error Causes:
- Inaccurate Path Specification: The preset path may be incorrect or non-existent which can lead to a failure in finding the renderer.
- Incorrect Module Exports: If the export and import of modules is handled improperly, this type of error can occur.
- Faulty or Missing Dependencies: If certain prerequisites required by the preload are missing or faulty, this could result to assertion of this error.
To give us context on ‘Missing Preload’, we should first understand what a preload is. A preload is a function that allows content to be loaded before your application runs. It’s primarily used within the Electron framework for desktop apps built using web technologies like
xxxxxxxxxx
HTML
,
xxxxxxxxxx
CSS
, and
xxxxxxxxxx
JavaScript
. You’d use preloads in Electron when an operation has to be executed before the window of the app is ready (Electron.js).
Solution
The solution to address this error would largely depend on the cause. However, here is a general guide:
- Validate the preload path specification: Ensure that the preload file path referenced in code is correct. This involves validating the associated directory and filename.
- Review module exports: Check your syntax when exporting and importing modules. Some frameworks have very specific requirements that, if not met, could lead to errors.
- Inspect dependencies: Confirm all dependencies necessary for the renderer to operate effectively are installed and working properly. Updating or reinstalling malfunctioning dependencies could help resolve the problem.
For instance, assuming your issue arises due to inaccurate path specification, you might rectify it using the following approach if you’re using Electron’s
xxxxxxxxxx
BrowserWindow
and
xxxxxxxxxx
webPreferences
:
xxxxxxxxxx
const { app, BrowserWindow } = require('electron')
const path = require('path')
function createWindow () {
const mainWindow = new BrowserWindow({
width: 800,
height: 600,
webPreferences: {
preload: path.join(__dirname, 'preload.js')
}
})
mainWindow.loadFile('index.html')
}
app.whenReady().then(() => {
createWindow()
app.on('activate', function () {
if (BrowserWindow.getAllWindows().length === 0) createWindow()
})
})
app.on('window-all-closed', function () {
if (process.platform !== 'darwin') app.quit()
})
By tentatively ensuring accurate path specification, reviewing module exports, inspecting dependencies, and making the necessary corrections, you should hopefully resolve any instances of “Error loading preloads: Could not find renderer”. However, always validate the potential underlying causes of the issue because the appropriate solution varies from one situation to another.When we talk about “Error Loading Preloads: Could Not Find Renderer”, it points to an occasion where your program is trying to load a renderer that it does not have access to or does not exist in the first place. This results in an error, because programs require specific renderers to visually display content or graphical user interfaces.
Understanding what exactly a renderer does can make it easier to appreciate the nature of these errors:
- Renderer: In technical terms, a renderer is a software component responsible for interpreting data and converting (or rendering) into images, video, or animations.
Typically, if you see this error, it is likely you are working on something involving a graphics-based UI (in a web browser, game engine, etc.). Now, depending on the specifics of your environment and codebase, the causes can range from configuration mistakes, missing dependencies, incorrect file paths, among others.
Analyzing Common Causes
- Incompatible System: Sometimes the issue arises if you’re trying to use a renderer that isn’t compatible with your system. For instance, hardware constraints can limit which renders you can use.
- Misconfiguration: In other cases, it might be that the settings meant to initialize the renderer are not correctly configured.
- Non-existent or Wrongly Specified Renderer: If a renderer has been specified but doesn’t exist or has been wrongly specified, that could also lead to errors.
- Missing packages or libraries: In some scenarios, there could be packages or libraries that need to be installed or updated to use the specific renderer.
A Few Troubleshooting Solutions
xxxxxxxxxx
// Code snippet suggesting how to declare and initialize a renderer
let renderer = new THREE.WebGLRenderer();
* Check the compatibility of the renderer with your system. Look up your tool or library’s documentation for details. Here is an example with Three.js WebGL Renderer documentation.
* Ensure your configurations and declarations are consistent with recommended methodology. Compare your code to samples provided by the library or framework you’re using.
* Verify that you have correctly specified the path to the renderer and that it indeed exists. Preload paths could sometimes be relative or absolute, so check for both.
* Confirm that all the necessary packages or libraries needed by the renderer are present and up-to-date.
While this analysis should provide you with a robust start to understanding and resolving the ‘Error loading preloads: could not find renderer’, remember that like many coding and debugging processes, it could vary based on individual project architectures and configurations.While coding professionally, there are several renderer solutions that can be adapted to meet different project requirements. However, at times, you might face an error like ‘Error Loading Preloads: Could Not Find Renderer’. This primarily means that the application fails to find a module or fails to render a function as expected.
Let’s unearth some common causes of this problem:
– **Failed import**: It’s crucial to double-check all your import statements, ensuring that they correctly refer to the required rendering modules. The inconsistencies could be as small yet significant as case discrepancies.
– **Non-existent File/database entries**: If the database entries or files you’re referring to don’t exist, it will prompt the ‘Could Not Find Renderer’ error.
– **Pathing Problems**: Pathing issues often crop up if the referred module resides in a separate folder. Facile navigation through directories and understanding the difference between absolute and relative paths is paramount during code development.
To fix these, we need to apply specific solutions accordingly:
1. **Rationalizing Imports**:
Examine your import statements carefully. Ensure that the names align with those in the exported files – both case-wise and spelling-wise.
xxxxxxxxxx
//Bad import
import { myModule } from './myModulle';
//Good import
import { myModule } from './myModule';
2. **Ensuring Files Exist**
Before running the code, confirm that all the files and database entries indeed exist. If they don’t, you might need to generate or fetch them before letting the render function accessing them.
3. **Correct Pathing**
If the files reside in different folders, ensure that the routing is correct. You can use built-in NodeJS
xxxxxxxxxx
path
module to handle file paths.
xxxxxxxxxx
const path = require('path');
// Incorrect path
const myModule = './module/myModule';
// Correct path
const myModule = path.resolve(__dirname, 'module/myModule');
Knowing what typically causes the “Could Not Find Renderer” error and how to fix it will make us better equipped at troubleshooting similar problems in the future. At the end of the day, we must remember that these errors are not something to forge ahead against but learn from by coming up with creative solutions. A complete understanding of the coding intricacies will help us keep the problems at bay.
Kindly refer to Mozilla’s comprehensive [“JavaScript Modules”](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Modules) guide for further details in regard to loading modules in JavaScript.Without a doubt, the error “Could Not Find Renderer” can be frustrating. This error often occurs when you try to load your preloaded items in your code, especially when handling advanced functionalities of Web-based applications or graphics intensives software. Let’s delve into the nuts and bolts of this issue.
Primarily, consider, what does ‘Preload’ mean? It refers to the process of loading some data into cache before it’s needed. It’s a way by which we prepare our application to launch more quickly and efficiently, thereby enhancing the user experience. When you encounter an error loading preloads such as ‘Could not find Renderer,’ it usually indicates a failure in finding the rendering process file1.
You might face this issue when:
- The path specified for the renderer is incorrect.
- The renderer file itself is missing or corrupted.
- There are permission issues that prevent access to the renderer file.
- Your system lacks sufficient resources to start the renderer.
To resolve this error, here are several steps you can take:
- Ensure the path to your renderer in your codebase is correct. You could do this with the
xxxxxxxxxx
111fs.access()
function in Node.js to check if a file is accessible or use any other similar functions for different languages.
- If that works fine, ensure that the Renderer file itself exists and isn’t corrupted. You could perhaps re-download or rebuild it.
- Check your user permissions to ensure you have access to the required directories.
Another snippet is: - Lastly, always ensure to clear your cache after making these changes, as they might not reflect immediately due to cached data.
An example snippet would look something like this:
xxxxxxxxxx
const fs = require('fs')
fs.access('/path/to/renderer', (err) => {
console.log(err ? 'No access!' : 'Can read/write')
})
xxxxxxxxxx
const fs = require('fs')
fs.access('/path/to/renderer', fs.constants.R_OK | fs.constants.W_OK, (err) => {
console.log(err ? 'no access!' : 'can read/write')
})
Errors such as ‘Could not find Renderer’ often stem from path or accessibility issues, and troubleshooting them involves understanding the preload feature and its complexity. Moreover, they point towards keeping your application well-prepared and efficient enough to deliver top-notch performance using preloading techniques.
Remember to go through the given solutions systematically. With some perseverance and a methodical approach, you’ll overcome the hurdle swiftly. No mistake should discourage you as a coder. On the contrary, they serve as opportunities to improve and learn. Happy coding!2