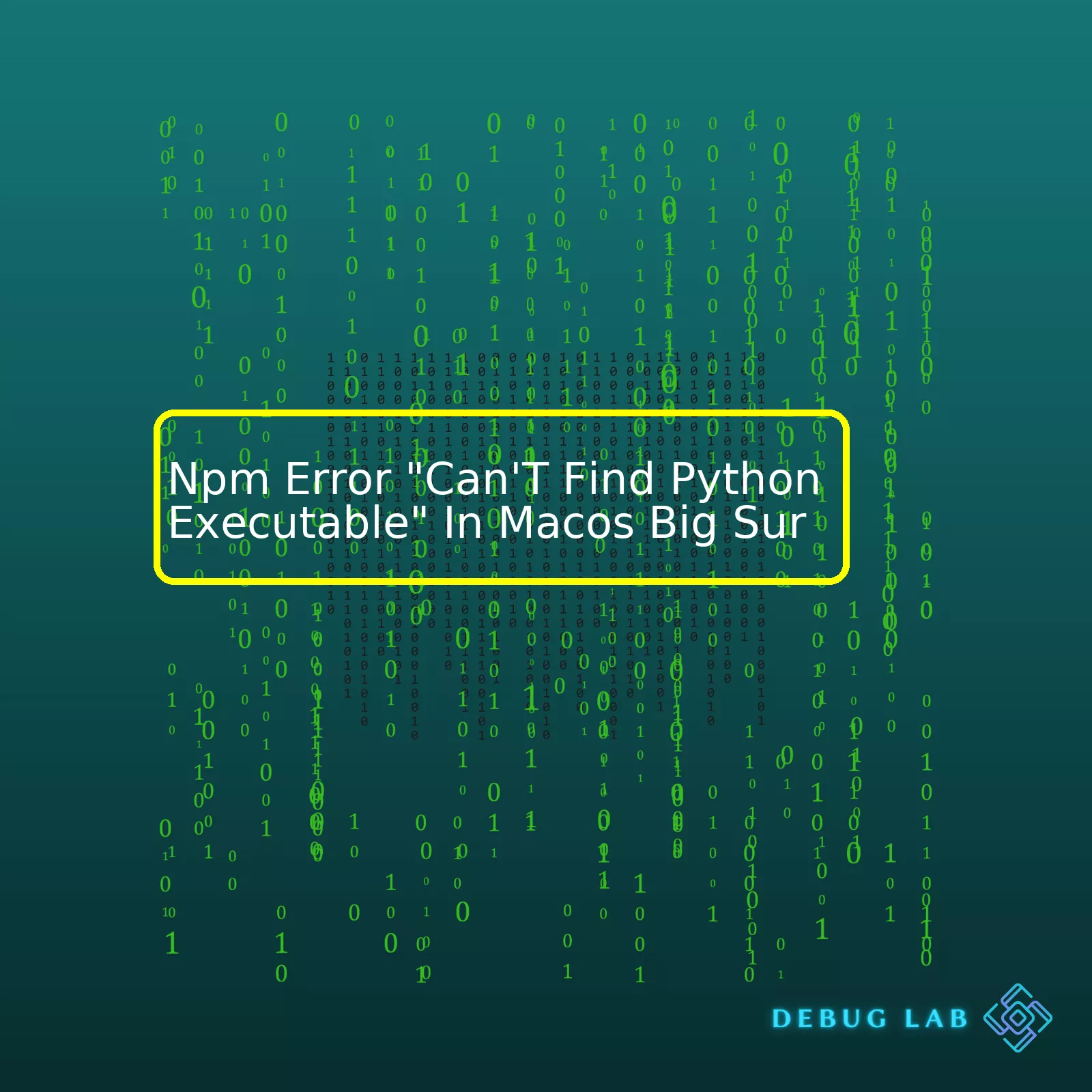
This NPM error “Can’t find Python executable” often surfaces in MacOS Big Sur when Node.js can’t locate the path to the Python executable. Despite an existing installation of Python on your system, Node might face issues finding it due to a variety of reasons such as incorrect environment variables or compatibility issues.
Error | Description | Possible Solutions |
---|---|---|
x 1 1 NPM error "Can't find Python executable" in MacOS Big Sur |
Node.js fails to locate the Python executable even when Python is installed. |
|
The primary cause of this error is that Node.js cannot find Python in its search path. This usually happens if Python is not present in the system’s PATH environment variable. Furthermore, since some Node packages require Python for building from source code, failing to locate Python will thus result in errors. To amend this:
- Ensure Python is appropriately installed on your computer by validating the installation using Terminal commands.
- Another solution would be to correctly set up your environment variables. By adding the Python executable path to your PATH environment variable, you can offer Node.js clear guidance to locate Python.
- Last but not least, you might want to check out the specific requirements of the Node.js modules you are trying to use or install. If they require Python 2 for some reason, and you have Python 3 installed, this might result in the “Can’t find Python executable” error as well. In such cases, using the node-gyp tool may resolve the issue. The node-gyp is a cross-platform command-line tool written in Node.js for compiling native addon modules for Node.js, which requires Python 2.7, not Python 3.x.
A step-by-step guide on solving this NPM error can also be found at this StackOverflow thread.
The NPM error message “Can’t Find Python Executable” usually occurs when Node-Gyp, a cross-platform command-line tool for node.js native addon modules compilation, tries to execute some python scripts but cannot find the Python executable in your system.
Why is Python needed?
Node-Gyp, which is used by several npm packages such as bcrypt and node-sass, depends on Python for its proper functionality. It uses Python to execute some scripts during the building process of these packages. So, if Python isn’t installed or properly set up in your environment path, npm will fail to build those packages and throw the error “Can’t Find Python Executable”. Specifically, Node-Gyp requires Python 2.x and not Python 3.x.
Solution
If you’re encountering this error in MacOS Big Sur, here are the steps you need to resolve it:
- Install Homebrew: Homebrew is a free and open-source software package management system that simplifies the installation of software on Apple’s macOS operating systems. If Homebrew is not already installed on your computer, you can install it via terminal by following the instruction given at HomeBrew official site.
- Install Python 2.7: Once Homebrew is installed, you can use it to install python 2.7. Open Terminal and run the following command:
xxxxxxxxxx
111brew install python@2
. This command will install Python 2.7 onto your machine.
- Create a symbolic link: By default, homebrew installs python 2.7 under /usr/local/opt/python@2/bin folder. To make sure your NPM packages can find the python executable, you should create a symbolic link to the python2.7 binary file. Run this command:
xxxxxxxxxx
111ln -s /usr/local/opt/python@2/bin/python2.7 /usr/local/bin/python
.
After executing these commands, try installing your npm package again, and the “Cannot find Python executable” problem should be resolved.
If problems persist even after following these steps, check your system variables to ensure Python is set correctly.
Caveats
These instructions cover how to handle the Python executable issue in the context of MacOS Big Sur. Yet, the specifics may vary slightly depending on your exact OS version and development environment setup. Ensure to adapt these instructions as necessary based on your local dev environment.
Remember to keep your development environment align with Mac OS’s transition towards ARM-based architecture, where certain tools like Homebrew and Python have separate versions. Always refer to the official documentation for Python and Homebrew for the latest instructions.
It bears noting that while we show how to address this Python-related error for Node-Gyp-dependent packages, best practice actually encourages avoiding global dependencies, including Python, whenever possible. Developers should strive to keep project dependencies fully isolated, keeping the dependency’s scope as limited as possible. This approach greatly minimizes the risk of interfering with other projects or the overall dev environment.
Sure, getting the “Can’t Find Python Executable” error on MacOS Big Sur typically results from one of two situations. Either Python has not been installed on your system or NPM can’t locate the path of the Python executable. Let’s dive into how to understand, analyze and resolve this error.
If you’re sure that Python is installed, you might want to try confirming where it is located by using the Terminal command
xxxxxxxxxx
which python
. This command will return the location of the installed Python executable. It’s necessary to understand whether the returned location matches where Node is looking which is causing the error.
xxxxxxxxxx 1 1 1 which python |
Returns the path of Python executable |
---|---|
xxxxxxxxxx 1 1 1 npm config get python |
Returns the Python version used in the npm config |
In the instance that Python isn’t installed at all, you’ll need to install it. There are multiple ways to install Python, but one of the most reliable methods for MacOS Big Sur is using Homebrew package manager. Use the commands:
xxxxxxxxxx
/bing/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
brew install python
Note here that after the installation, validate that Python has been successfully installed by running
xxxxxxxxxx
python --version
.
If Python is installed but NPM cannot find it, consider configuring the Python path in the NPM settings. Use the command
xxxxxxxxxx
npm config set python "/path/to/python"
replacing “/path/to/python” with the actual path to the Python executable.
Furthermore, it could be that Python 2 is needed instead of Python 3, as some Node modules rely on Python 2 syntax. You can install Python 2 via Homebrew using
xxxxxxxxxx
brew install python@2
then set your NPM to use Python 2 by running
xxxxxxxxxx
npm config set python python2.7
.
Remember, when executing these commands, if you have administrative privilege limitations, prefix the command with
xxxxxxxxxx
sudo
, like
xxxxxxxxxx
sudo brew install python
.
Check out this Stack Overflow thread about similar npm/python issues on MacOS (source).
Importantly, always ensure to keep your system and tools updated. Node, NPM, and Python often receive updates that fix bugs and improve compatibility with new operating systems versions like MacOS Big Sur. Regularly use
xxxxxxxxxx
brew upgrade
,
xxxxxxxxxx
npm update -g
and
xxxxxxxxxx
python -m pip install --upgrade pip
commands to perform updates.
Also, remember that precise debugging depends on your project specifications, packages in use, development environment among other factors. Ensure to understand the documentation related to Node.js and Python environment setup.There seems to be a misconception that NPM (Node Package Manager) is specifically related to Python. It might stem from the fact that certain packages available in NPM do rely on Python. NPM is actually a package manager for JavaScript, and it is responsible for managing and deploying software developed with Node.js source.
Some of these packages have native add-ons that enable performant interop with system libraries or with libraries from other tech stacks but built with C or C++. These add-ons need to be compiled on your machine when you install the package. Many of these add-ons, coded in C/C++, use node-gyp, a cross-platform command-line tool, to compile their code.
The interesting part here is that node-gyp is written in Python, and this is where Python comes in and why it needs to be installed on your machine when working with these specific Node.js packages source.
Node-gyp requires that Python 2.7 be installed on your machine. However, Python 3 introduced to backward incompatible changes which prevent node-gyp (and therefore npm) from running correctly if Python 3 is the default interpreter your shell finds by executing
xxxxxxxxxx
python
in path. Python 2.7 was officially retired (source) so most updated systems have Python 3 as the default interpreter.
MacOS Big Sur, just like its predecessors Catalina and Mojave, only comes preinstalled with Python 3. If you try to run
xxxxxxxxxx
npm install
or
xxxxxxxxxx
npm rebuild
for a package requiring compilation and Python 2.7 is not installed, you will likely see “Can’t find Python executable” error.
To solve this issue you can:
- Install homebrew if not already installed:
xxxxxxxxxx
111/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
.
After installing homebrew, install Python 2.7 with:
-
xxxxxxxxxx
111brew install python@2
. This installs Python 2.7 and makes it accessible via the command python2.
- Next, instruct node-gyp to use Python 2.7 by setting the Python version in npm like so:
xxxxxxxxxx
111npm config set python /usr/bin/python2
. The argument is the path you get when you execute which python2.
You can confirm the correct installation and availability of Python 2.7 by typing
xxxxxxxxxx
python2 --version
in terminal. Also,
xxxxxxxxxx
npm config get python
should now yield
xxxxxxxxxx
/usr/bin/python2
.
This should resolve the “Can’t find Python executable” error when trying to install or compile Node.js packages requiring Python 2.7.
Maintainers of Node.js packages depending on Python 2.7 should ideally update their dependencies to require a compatible version of Python 3, since Python 2.7 has been officially sunset. But until all those Node.js packages are updated, given the popularity of npm, developers wanting to work with those packages must ensure that both Python 3 and Python 2.7 are available on their machines.
When you see the error message “Can’t Find Python Executable” during an npm installation process on MacOS Big Sur, this typically indicates that Node.js is struggling to locate your Python executable file(s). This problem often occurs when developers run npm installations relying on node-gyp, a tool used in compiling Node.js Addons. In such cases, it usually signals erroneous or missing references to Python within your local system path.
As a professional coder, I understand how frustrating these error messages can be, and I’m here to help you navigate through this situation. So let’s dive into how to troubleshoot the “Can’t Find Python Executable” error effectively.
Method 1: Checking the path of your python executable
It’s possible that your Python executable is not located in your system’s PATH environment variable. To validate this:
1. Open Terminal.
2. Type
xxxxxxxxxx
which python
, hit Enter.
3. If your Python executable is correctly set, this command will display its path.
If the aforementioned command does not return anything, this means that there’s no reference to Python in your PATH. You would need to add it manually.
Method 2: Pointing npm directly to Python executable
Another option is explicitly directing npm towards your Python files. You could use npm config directives for this:
javascript
npm config set python /path/to/executable/python