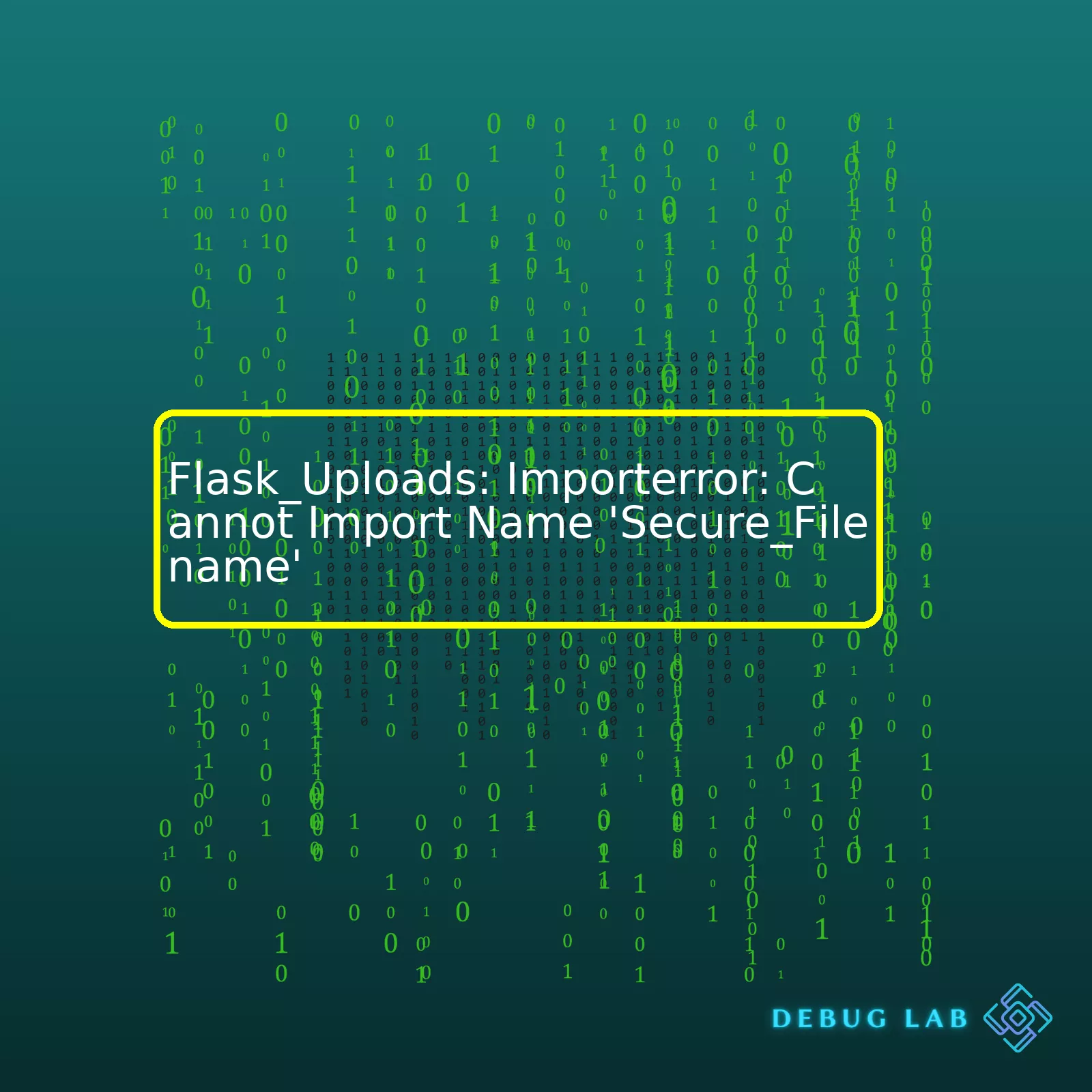
from flask_uploads import secure_filename
Attempting to import the ‘secure_filename’ function generates this concern. The error indicates that Python cannot locate this specific function (‘secure_filename’) within the ‘flask_uploads’ module.
Below is a summary table outlining the issue and possible solutions:
Error | Issue Origin | Most Likely Resolution Strategy |
---|---|---|
ImportError: Cannot import name ‘secure_filename’ | The Python interpreter is unable to find the ‘secure_filename’ function in the Flask_Uploads module. | Import ‘secure_filename’ from the correct module which is ‘werkzeug.utils’, not ‘flask_uploads’. |
It’s understandable how one can get confused because multiple Flask extension libraries deal with file uploads and names. However, the ‘secure_filename’ function doesn’t actually belong to the ‘flask_uploads’ module, which is driving the ImportError.
Instead, what you want to do is import it from the ‘werkzeug.utils‘ module:
from werkzeug.utils import secure_filename
The `secure_filename` utility comes as part of the Werkzeug package, a WSGI library used by Flask internally. It ensures that the filename is safe to use directly as a filename on your filesystem by removing potentially harmful characters.
Remember, more often than not, many errors come down to syntactical misunderstandings or minor overlooks. Take a closer look at the module documentation if the functions don’t behave as expected or even throw outright errors as in our case here.Flask-Uploads is an incredible extension for handling file uploads in Flask-based applications. However, issues may arise such as `ImportError: Cannot import name ‘Secure_Filename’`. This problem frequently occurs when the importing package itself has errors or there’s a mishap in the library or module setup. Luckily, these problems can be addressed quite readily.
To start, it’s essential to ascertain that you have correctly installed Flask_Uploads via pip by typing
pip install flask-uploads
in your terminal or command prompt.
Once the installation process is confirmed, the next step is to validate your import statements in your Python code. The most common cause of this error is attempting to import the module ‘Secure_Filename’ from the Flask_Uploads package, instead of its correct source which is Werkzeug.
In your case, change:
from flask_uploads import secure_filename, UploadSet, IMAGES
to:
from werkzeug.utils import secure_filename from flask_uploads import UploadSet, IMAGES
As you see, the ‘secure_filename’ is correctly imported from ‘werkzeug’, whereas ‘UploadSet’ and ‘IMAGES’ (only if required in your code) are imported from Flask_Uploads package.
If after performing this correction, you still encounter the ImportError, it could also be due to conflict between Flask-Uploads and Flask-Upload, two different packages that share similar naming but come from different sources and have different functionality. If Flask-Upload is accidentally installed instead of Flask-Uploads or vice versa, it may create confusion leading to errors. Verify the correct package needed for your application, then uninstall the incorrect one with the pip uninstall command ensuring you only have the right library. For more details about these modules, you can visit their official documentation herelink1 or link2.
Finally, make sure your environment does not contain any old or conflicting packages which could interfere with functionalities. You may want to use a virtual environment to provide a clean working space, isolating your workspace from global dependencies. Both venv and conda are excellent choices for python virtual environments.
# creating a venv
python -m venv env
# activating env on Unix/Mac os
source env/bin/activate
#activating env on Windows
.\env\Scripts\activate
In the virtual environment, you can manage your packages locally, without affecting your system-wide settings. This separation reduces the risk of conflicts between packages and promotes organized workflows.
To wrap up, several factors may contribute to the error `ImportError: Cannot import name ‘secure_filename’`. Mastering these solutions would involve correcting your import statement, verifying the installed packages are what is intended, and resorting to usage of a virtual environment.
Certainly!
The error message “ImportError: Cannot import name ‘secure_filename'” may occur when you try to import the secure_filename function in Flask. Specifically, this might happen when using the Flask module called Flask-Uploads. The
secure_filename
utility function is useful for ensuring that uploaded file names are secured and cannot cause damage.
Brief Note on Flask_Uploads:
Flask-Uploads is a module built to handle the uploading of files in Flask. It provides wide range capabilities such as converting images or restricting uploads to certain types of files.
However, the
secure_filename
function is not a part of Flask-Uploads, rather it belongs to another Flask module called Flask-WTF which deals with forms. So when we specifically call
from flask_uploads import secure_filename
Python won’t be able to find the requested resource hence throwing an ImportError.
So how do we solve this?
The problem here lies within Python’s order of execution and structure — it’s simply looking for our specified filename in the wrong area. We’ll have to direct it to the exact module where it resides.
Fixing the Error:
To address the error issue about Secure_Filename, we make the necessary code corrections. The function secure_filename should be imported from Werkzeug.
Werkzeug is a comprehensive WSGI web application library. It began as a simple collection of various utilities for WSGI applications and has evolved into one of the most advanced WSGI utility modules. It includes a powerful debugger, full-featured request and response objects, HTTP utilities to handle entity tags, cache control headers, HTTP dates, cookie handling, file uploads, and more.
Hence, we should replace:
from flask_uploads import secure_filename
with:
from werkzeug.utils import secure_filename
This way you’re importing the function from the correct location. After doing so your import error should vanish, and you can continue coding your Flask application!
You really need to ensure you take note of the specific locations of Python functions when trying to import them, especially when working with framework extensions in Python.
Make sure to consult the Flask-WTF Documentation if you come across similar problems again. Documentation always provides comprehensible clarity regarding any uncertainties or complications on the topic being discussed. Here you will be able to verify both the origin and functionalities of utilities such as
secure_filename
. Remember, Coding is trial and error filled with rigorous mastery.Flask_Uploads is a highly efficient library in Flask framework that’s mainly used to handle file uploads in a user-friendly way. However, it can sometimes generate an ImportError: “cannot import name ‘secure_filename'”, even after importing secure_filename from Flask’s werkzeug.utils module. Here’s what you need to know:
The function
secure_filename()
comes from the Werkzeug utility for Python-based web applications. What this function does is ensure that your uploaded file has a safe and suitable name to be stored in the server filesystem.
A common instance of utilizing secure_filename in a Flask application happens like this:
from flask import Flask, request from werkzeug.utils import secure_filename app = Flask(__name__) @app.route('/upload', methods = ['GET', 'POST']) def upload_file(): if request.method == 'POST': f = request.files['file'] f.save(secure_filename(f.filename))
In this example, we’re using
f.save(secure_filename(f.filename))
to store uploaded files directly in the application root directory with a sanitized filename.
Although the error might occur, essentially, because Python cannot locate the ‘secure_filename’ within the werkzeug library scope. Before delving into the solution, it’s interesting to understand this scenario.
Werkzeug, being WSGI web app library, provides a collection of utilities vital for developing web services. One such utility includes a secure handling method securing filenames associated with file-uploads.
However, there are potential reasons behind the stated error:
– If you’re using an outdated version of Werkzeug (0.15 or below), you might experience the ImportError, as it might lack the ‘secure_filename’ function.
– The installation of Flask or Werkzeug could have patched incorrectly due to network issues, corrupting certain files, thereby causing the error.
For these reasons, if you come across this error, consider the following solutions:
– Reinstall Werkzeug.
– Upgrade your Werkzeug version to 0.16 or later.
Install/upgrade Werkzeug using pip:
pip install --upgrade Werkzeug
After these steps, make sure to clear Python’s cache before restarting your application.
Please note:
Before using
secure_filename()
, always remember, although it sanitizes your filenames, doesn’t ensure their uniqueness on the filesystem. So take care to prevent overwriting of files. You can add a timestamp or unique id to the filename before saving them.
Understanding the role and significance of secure_filename in Flask_Uploads, and actioning the solution to this ImportError, aids tremendously in seamlessly managing your file-upload feature within a Flask application. I hope this discussion has useful for you. Read More about Flask File Uploads.Without a doubt, import errors are one of the common plights that we Python coders have to tackle in our day-to-day coding lives. Among those, ‘ImportError: cannot import name X’ tends to pop up quite frequently. This error message is indicative of either missing modules/packages or incorrect usage of the import statement. With reference to the prompt at hand, the error ‘Cannot import name Secure_Filename’ predominantly arises when you are working with Flask Uploads, a flexible and efficient upload handling module for Flask.
Let’s take a moment to analyze this issue in more depth. Typically, Flask-Uploads allows you to easily handle file uploading in your application. To use it, however, you need to import it properly. Here is how the correct import statement should look:
from flask_uploads import UploadSet, configure_uploads, IMAGES, patch_request_class
From the error message, there seems to be an ImportError caused by the unsuccessful import of ‘Secure_Filename’. Most probably, you might have tried to import ‘Secure_Filename’ straight from `flask_uploads`. While ‘Secure_Filename’ is indeed a useful utility function used to secure a filename before storing it directly on the filesystem, it does not belong to the `flask_uploads` package. Instead, it belongs to the `werkzeug.utils` package of Werkzeug, a WSGI web application library.
Here’s the correct import for ‘secure_filename’:
from werkzeug.utils import secure_filename
In an attempt to clearly demonstrate this, let’s take into account the snippet of code below where I correctly import and use ‘secure_filename’ in a very basic Flask app:
from flask import Flask, request from werkzeug.utils import secure_filename app = Flask(__name__) @app.route('/upload', methods=['GET', 'POST']) def upload_file(): if request.method == 'POST': f = request.files['the_file'] f.save('/var/www/uploads/' + secure_filename(f.filename))
In the given example, the `secure_filename` function is imported from `werkzeug.utils`, not `flask_uploads`.
Occasionally, these kind of import errors may also suggest that the module/package is not installed in the current environment. Make sure that both Flask-Uploads and Werkzeug are correctly installed by running:
pip install Flask-Uploads pip install Werkzeug
By avoiding these common pitfall through following proper importing practices, understanding the packages/modules necessary for your work and ensuring that they are installed in your environment, you’ll save yourself from unwanted headaches and ensure that your python programming journey becomes smooth sailing. Just remember, the next time Python tells you that it “cannot import name X”, take a closer look at where you’re trying to import X from – Python is very likely telling you that X simply doesn’t live there! For more information about imports in Python, visit Python’s official documentation.The problem you’re facing – “ImportError: Cannot import name ‘Secure_Filename'” – is commonly encountered when working with Flask. The error occurs when your Python Flask environment attempts to import the ‘secure_filename’ method from the ‘flask_uploads’ module instead of from its original source, the ‘werkzeug.utils’ module.
To explain this relationship, let’s dive into Flask-Uploads and secure_filename.
Flask-Uploads
Flask-Uploads simplifies the process of uploading files in your Flask application. With Flask-Uploads, you can configure where the uploaded files will be stored, the specific file types that are permitted for upload, and limit the size of uploads. It’s efficient and useful in controlling how users can engage with your web application.
However, it’s important to realize that Flask-Uploads doesn’t contain the ‘secure_filename’ function internally. This function belongs to another Flask-related module, Werkzeug.
Werkzeug and secure_filename
Werkzeug is a WSGI web application library. It’s one of the building blocks on which Flask rests. Among many other utilities, Werkzeug provides the ‘secure_filename’ function. This function is critical because it ensures safe and appropriate filenames for user-uploaded files. It accomplishes this by removing any potentially unsafe characters and replacing them with underscores, thus mitigating security risks such as directory traversal.
Now, let’s take a look at how these two modules should work together, to solve your ImportError issue.
from flask import Flask, request from werkzeug.utils import secure_filename app = Flask(__name__) @app.route('/upload', methods=['POST']) def upload_file(): file = request.files['theFile'] filename = secure_filename(file.filename)
In the code snippet above, secure_filename originates correctly from werkzeug.utils, which prevents an ImportError when executing the script.
Do not forget not to try to import secure_filename from flask_uploads. For instance:
from flask_uploads import secure_filename # Wrong!
This throws an ImportError because flask_uploads doesn’t contain a function named secure_filename.
Finally, it bears mentioning that the error message may arise for other reasons such as a misconfigured virtual environment. That is particularly true if you migrated from older versions of Flask-Werkzeug to new ones where some dependencies require updates.
See the official Flask documentation for precise information about handling file uploads and consider exploring the wider online community for troubleshooting assistance on platforms like Stack Overflow.Sure! Fixing import errors in Flask, particularly the ‘ImportError: cannot import name ‘secure_filename” issue from Flask_uploads commands your attention to a couple of strategies. I’m laying down a step by step big picture first before diving deeper.
Here’s the overview of our problem-solving:
– Diagnose the Problem Accurately
– Tweak Your Project Structure
– Check for Casing Issues
– Use the Right Version of Libraries
– Inspect Your Dependencies
Now let’s unravel each of these strategies with their respective elaborate explanations.
Diagnose the Problem Accurately
Do some diagnostic work on your code. Find out if you are getting any specific error messages and use them to trace back to the line(s) that could be causing the trouble. In the case of “ImportError: cannot import name ‘secure_filename'”, this typically means Python can’t find the module or function you’re trying to import.
For instance, ensure that the library is correctly installed using pip:
pip install Flask-Uploads
Remember to retain your virtual environment active while installing.
Tweak Your Project Structure
The project structure has to be organized in such a way that python recognizes the file it needs to import. If you have modules spread haphazardly, it might cause issues with importing.
In the context of Flask and particularly with “Flask_Uploads”, here is an example on how to properly import ‘secure_filename’:
from werkzeug.utils import secure_filename
‘h2’Check for Casing Issues’/h2’
Python is case-sensitive, which means ‘Secure_Filename’ and ‘secure_filename’ would be treated as different entities. Do cross-check all instances where ‘secure_filename’ is used in your code.
Use the Right Version of Libraries
Incompatibility between versions of Flask, Flask-Uploads or even other libraries in your project might cause problems. Make sure you’re using compatible versions.
For instance, secure_filename is available in Werkzeug version 0.5 and later. Be sure that you have at least that version installed. You can check or upgrade with:
pip show werkzeug
Or,
pip install --upgrade werkzeug
Inspect Your Dependencies
Take a good look into your project dependencies. If two (or more) libraries are dependent on different versions of another common library they may clash leading to import errors. In such a scenario, find an alternative library or adjust your code so that you can standardize the version of the common dependent library across your project.
Also, you can refer to the official Flask documentation for extensive details about dealing with import errors and detailed understanding on Flask_Uploads:Importerror.
Positioning all these strategies, you’ll recognize that our key focus is to articulate the relation of the error message received with the appropriate analytical response. These strides towards debugging will allow your codebase to regain it’s harmony with the Flask_Uploads.The Flask Uploads ImportError that reads ‘
ImportError: Cannot import name 'secure_filename'
‘ is a pretty common issue. It can arise due to various conditions and can be resolved through multiple pathways.
First, let’s understand the cause behind this error. Secure_filename is a function provided by the Werkzeug utils module, primarily used to ensure that file names are secure before they are passed onto the Flask framework for processing. Therefore, an error importing it from the Flask Uploads means a missing or improperly addressed function in your Python environment.
Let’s address how we can tackle this error in different scenarios:
Misplaced Import Statement:
Python runs scripts from the top to the bottom. If you happen to use the function before importing it, Python throws an ImportError. Make sure you have placed the import statement at the top of your modules; ideally after installing all the modules.
from werkzeug.utils import secure_filename