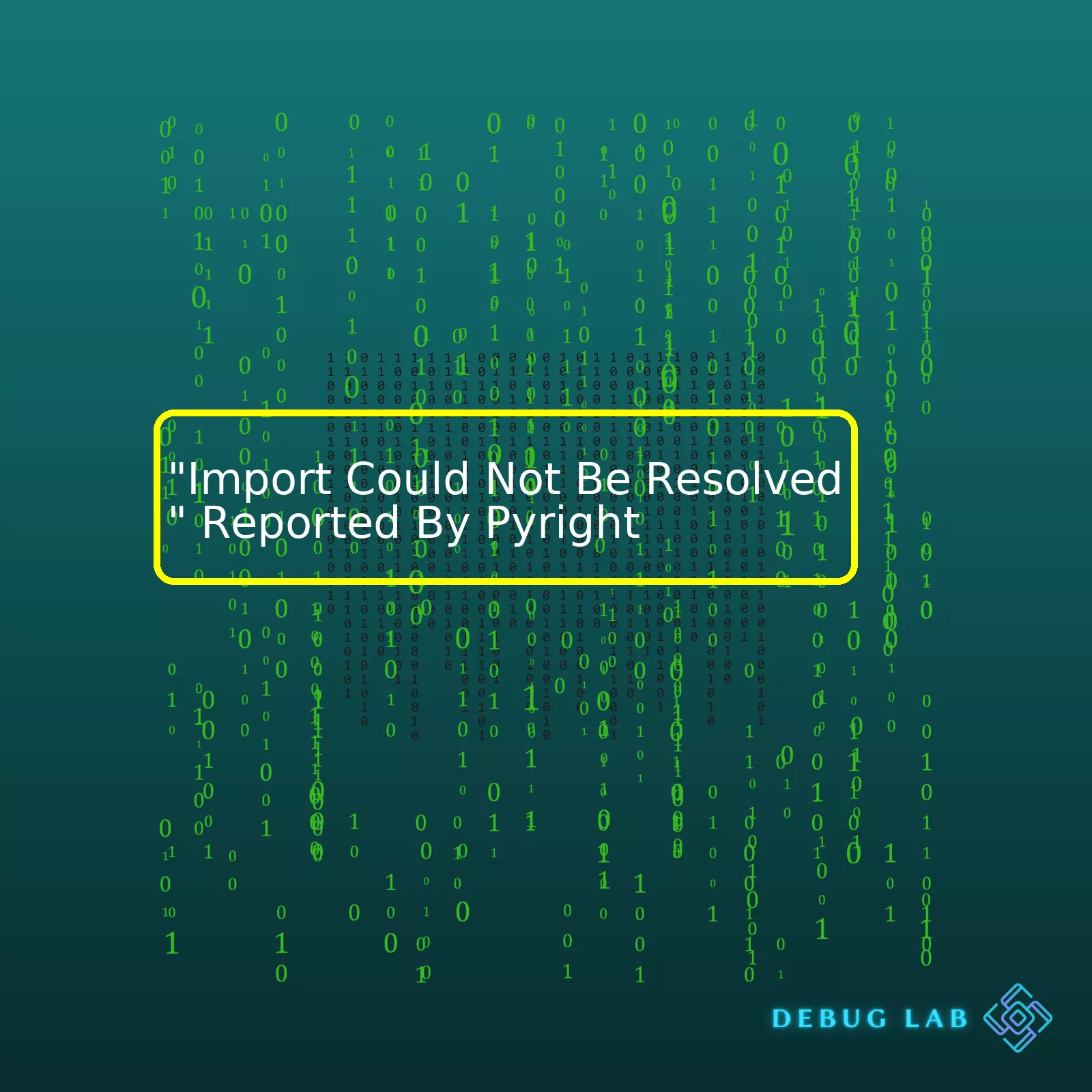
Possible Cause | Issue Description | Solution Approach |
---|---|---|
Incorrect File Path | Import statement might have an incorrect file path. | Check your directory structure and correct your import statements accordingly. |
Python Environment Mismatch | Pyright uses a different active Python environment than the one you’re coding in. | Ensure consistency between your development and Pyright’s environments. |
Issues with __init__.py files | Python usage of
x 1 1 __init__.py files has changed over versions, which might affect imports. |
If you’re using Python 3, ensure
xxxxxxxxxx 1 1 1 __init__.py is correctly implemented in every directory. |
Not in PYTHONPATH | The module isn’t within any directory specified by the PYTHONPATH. | Add your module’s location to PYTHONPATH or move the module within it. |
When you encounter such an error, the first order of business is to examine the import statement throwing this error. Confirm whether the relative path to the file or module is correct. For instance, if the current file is located at `source/app.py` but we tried to import a file (say `module.py`) located at `source/utils/module.py` without specifying its full or correct relative path like so:
xxxxxxxxxx
import module
This will definitely lead us into running into this error since the file path is not correctly referenced.
A second scenario relates closely to mismatches in the Python environment being used. Pyright may be utilizing a different Python interpreter hence it does not recognize some modules or packages installed in another Python environment. As a solution, making sure that both Pyright and your active environment match each other could help eliminate this problem.
Moving forward, if it happens that the Python version you’re working on uses `__init__.py` for folder initializations, make sure all folders contain a rightfully defined `__init__.py`. This file lets Python know that the directory should be treated as a package as such making all scripts within it accessible to external modules.
Lastly, check your PYTHONPATH – this is an environment variable listing paths where Python looks for modules. If the module you’re trying to import isn’t located in any directory within the PYTHONPATH, either add the module’s directory to PYTHONPATH or move it to a directory already included.
For further reading, here is a link redirecting you to Pyright’s official documentation regarding the configuration and set up.The ‘
xxxxxxxxxx
Import could not be resolved
‘ error in Pyright is a common hitch that Python developers often encounter. This typically arises when the Python interpreter cannot locate the module or the package you’re striving to import into your script. Pyright, a static-type checking tool for Python and also an accomplished Language Server Protocol (LSP) implementation, accurately reports this red flag.
But why does this error occur? Various reasons come into play:
– The coding environment may not be well configured, meaning Pyright isn’t correctly signposted to where your packages are stored.
– The required Python libraries haven’t been installed in the environment Pyright is referencing.
– Your code may try to leverage a Python library or script that doesn’t exist or has not been explicitly defined in your project.
Now, let’s peek at how we can sway this. Resolving the ‘
xxxxxxxxxx
Import could not be resolved
‘ alert reported by Pyright involves understanding of the error-causing contexts and adopting effective ameliorative strategies accordingly:
─ Install Missing Libraries:
To begin with, ensure all needed Python libraries have been installed in your current working environment. Use Pip, Python’s go-to package manager, to verify and install dependencies as follows:
xxxxxxxxxx
pip install name_of_missing_library
Also, remember to adjust your import statements accurately if they include any typographical errors.
─ Properly Configure Your Coding Environment:
If the Python interpreter can’t find the Python files you’re trying to import, it throws an ‘import could not be resolved’ error. To set up the correct path, configure your settings.json file. Reference your virtual environment and acknowledge the correct Python interpreter:
xxxxxxxxxx
"python.pythonPath": "path_to_your_virtual_environment",
Pyright also facilitates the use of configuration files in JSON format like pyrightconfig.json to specify paths. For instance:
xxxxxxxxxx
{}
"venvPath": "path_to_virtual_environment",
"venv": "name_of_virtual_environment"
Ensure these configurations are accurate and reflect the exact paths.
─ Check Your PYTHONPATH:
PYTHONPATH is an environment variable used by Python to determine the location of modules to load. If your libraries reside outside the standard library directories, ensure your PYTHONPATH points to them. Update it as follows:
For Windows:
set PYTHONPATH=%PYTHONPATH%;C:\my_python_lib
For Linux/Mac:
export PYTHONPATH="${PYTHONPATH}:/my/path/to/module"
In essence, the '
Import could not be resolved
' message in Pyright prompts you to thoroughly cross-check your coding environment, the installation of the necessary libraries, and the correctness of resource allocation paths. Moreover, leverage the usage of tools such as Pylance, which works concurrently with Pyright to provide more precise type information. Understanding the error and its remedies will undoubtedly render Pyright more optimally productive for you.
References:
1. Pyright Configuration
2. Pylance - PyPIWhen using Pyright, a static type checking tool for Python, you might occasionally encounter error messages that state "Import Could Not Be Resolved". This error typically signifies that Pyright cannot discover the specified module or package in your project’s Python environment. Several factors may cause this issue. These include:
Table of Contents
Toggle
Issue In The Python Environment ConfigurationA Missing Or Incorrectly Installed PackageType Stubs Are Missing For Third-Party LibraryIncorrect Import Statements1. Add the Specific Path2. Use the ‘extraPaths’ Feature3. Explicitly Enable ‘venv’Understanding and Correctly Using Absolute and Relative ImportsAvoiding Circular DependenciesProperly Structuring Your ProjectPyright and the “Import Could Not Be Resolved” ErrorExample
Issue In The Python Environment Configuration
A common cause of unresolved imports is a configuration problem with the Python environment being used. If the module is installed locally but not globally recognized, problems can arise. An example of this is when using virtual environments. To resolve this, it's crucial to ensure Pyright utilizes the correct Python interpreter and the appropriate virtual environment.
This is usually set by creating a
pyrightconfig.json
file in the root of the workspace.
{
"venvPath" : "./Your-Venvs-Folder",
"venv": "Your_Venv_Name"
}
It will configure Pyright to use the Python interpreter installed in the indicated venv.
A Missing Or Incorrectly Installed Package
The unresolved import issue could also result from a missing or incorrectly installed Python package. So, verify that all required packages are correctly installed in your Python environment. You can do this through the pip list command:
pip list
If the required package isn't listed, install it using the pip install command:
pip install package-name
Remember here that if you're working with a virtual environment, you need to activate the environment first before executing these commands.
Type Stubs Are Missing For Third-Party Library
Third-party libraries sometimes lack typings, or type stub (.pyi) files. Therefore, Pyright might not be able to resolve imports from them. To work around this, it would help if you could:
Create your own type stubs: Herein, you'd write a ".pyi" file that provides type information about the functions, classes, and variables defined in the corresponding ".py" file.
Using any available "^py.typed^" directory: Some third-party libraries provide this as part of their library specific to typing. When a library includes a
py.typed
file, it shows it has provided inline types or type stubs.
Ignore the missing imports: Another workaround involves configuring Pyright to ignore missing imports from specific modules via
"ignoreImports"
option in your
pyrightconfig.json
Incorrect Import Statements
Incorrect import statements can also lead to unresolved import errors. Ensure that you've accurately named all your files, directories, and subdirectories and that your import statement is correctly following the hierarchical structure of your project.
For example, suppose you'are importing a function named `my_function` located in a file named `my_script.py`, which is stored inside a directory named `my_directory`. Your import statement should be:
from my_directory.my_script import my_function
Explore these potential causes when dealing with an "Import Could Not Be Resolved" error. Running through these steps should, in most cases, help you identify and rectify the issue swiftly.One of the most common issues you might encounter as a Python developer is that Pyright often reports "Import could not be resolved". Before we dive into the resolving methods, let's explore the root causes of this problem.
1. Absolute vs Relative Import: You might have incorrectly referenced your packages/modules using an absolute import instead of a relative one or vice versa.
2. Environment Mismatch: Your local development environment might not match with the environment in which Pyright is running.
3. Python Interpreter: Pyright might be using a different Python interpreter from the one you intended for use.
4. Error in extraPath settings: Incorrectly configured paths in your settings may lead to the issue.
Here are some quick fixes and tips on how to resolve these import issues when they're reported by Pyright:
Use Correct Path Reference
This serves as a major solution since errors usually occur due to wrong references. Python provides us two ways to reference modules, absolute and relative.
- Absolute Reference: This is where you specify the complete path of the module from the project’s root folder. Here is an example:
from my_package.my_module import my_function
- Relative Reference: Here, you can use dots to specify the location of the module. Each dot represents the parent directory. Here is an example:
from ..my_module import my_function
Remember, figuring out when to use absolute or relative import depends on your project’s structure.
Matching The Environments
Ensure that Pyright runs in the environment identical to your local development environment. Use a tool like Pipenv, Poetry, or virtualenv to create reproducible environments. Once you have these tools setup, Pyright should be able to recognize any libraries installed within these environments.
Setting Python Interpreter
Pyright automatically detects the Python interpreter based on your system PATH. If it's wrongly detected, you could either change the system PATH or specify the Python interpreter manually via the `"python.pythonPath"` setting in your settings.json file.
"python.pythonPath": "/path/to/your/python"
Configure extraPaths
To ensure all your modules are discoverable, you can use the `extraPaths` setting. `extraPaths` tells Pyright where to find your Python source files.
In your pyrightconfig.json, it would look like:
"python.analysis.extraPaths": [
"./additional_path",
"./another_additional_path"
]
Ensure you replace "additional_path" with your actual directory paths.
It's important to keep these considerations in mind while developing in Python, as neglecting them may cause unnecessary headaches down the road. Remember, even experienced developers can overlook these key points, leading to problems such as 'Import Could Not Be Resolved'.
Above all, understanding your codebase structure, keeping a well-managed and clean coding environment, and reading error messages attentively will eventually lead to fewer coding issues. And once you become proficient at debugging, these types of situations become less of an obstacle and more of a minor setback.
For further read, check out:
Pyright Configuration,
Python Best PracticesThe role of PYTHONPATH in Python is pivotal in managing how your program imports modules. Each time you use the
import
statement, Python goes through a list of locations defined in
PYTHONPATH
to search for the module or package.
PYTHONPATH works essentially like a path in a file system. A path identifies the location of a file on a computer. Similar to how different paths will lead to different files, different PYTHONPATH settings will make Python look for modules in different places.
When Pyright, the static type checking tool by Microsoft, reports an "Import Could Not Be Resolved" error, it generally means it failed to locate the module you were trying to import. This missing link often occurs due to inconsistencies between your project's structure and the PYTHONPATH environment variable.
There are multiple ways to tackle this issue:
1. Checking and Updating PYTHONPATH:
Start by examining your PYTHONPATH. You can do this in a Python shell using:
import os
print(os.environ['PYTHONPATH'])
To add a new path to PYTHONPATH, you'd write:
import os
# Suppose we want to add '/my/new/path' to PYTHONPATH
new_path = '/my/new/path'
if 'PYTHONPATH' in os.environ:
os.environ['PYTHONPATH'] += ':' + new_path
else:
os.environ['PYTHONPATH'] = new_path
2. Using Absolute Imports:
Instead of changing your PYTHONPATH, you can revise your module structure and utilize absolute imports.
For instance, suppose we have a directory structure like:
- my_project
- src
- main.py
- util.py
In
main.py
, an absolute import would look as follows:
import my_project.src.util
PEP 8, Python's official style guide, recommends absolute imports because they’re usually more readable and less prone to errors than relative imports.
However, remember that certain programs or scripts running directly (not imported) might not correctly resolve absolute imports. It’s always necessary to keep track of your PYTHONPATH when dealing with import resolution errors.
3. Using .env Files:
If updating the system's PYTHONPATH isn't your thing or viable, consider using a library such as python-dotenv. Libraries like this read the key-value pairs from the
.env
file and add them to environment variables.
Consider reading the documentation on how to use these libraries and create .env files corresponding to various environments in which your Python program is likely to run.
Remember, whenever you face any import issues, make sure your module structure, PYTHONPATH environment variable, Python interpreter, and actual script location align seamlessly. More importantly, ensure the referred module exists and works as expected.
Dealing with PYTHONPATH can be precarious, and cautious handling is advised. Nonetheless, understanding this environment variable's working will help you in robust code development.If you're a Python developer that utilizes Microsoft's static type checker, Pyright, you might have stumbled upon the error "Import Could Not Be Resolved" at some point. This usually occurs when Pyright is unable to locate an imported module in the specified files or directories.
The root cause for this discrepancy will likely be linked back to your Pyright configuration setup. Properly setting up your `pyrightconfig.json` file can significantly improve your workflow and save you from continuously run-ins with this error in the future.
Before we start, it's critical to know that the Pyright configuration file (known as pyrightconfig.json) stores settings for a particular workspace or project directory. If you don't already have one, simply create a new JSON file called `pyrightconfig.json` within your project directory. Now it's time to examine possible fixes.
1. Add the Specific Path
Pyright tends not to search the entire directory, which implies that if your module exists outside of the given path, the "import could not be resolved" error might arise. In these situations, I'd suggest explicitly adding the required paths to the Pyright configuration.
For example, consider a directory structure like:
Project
│
├── src
│ └── main.py
│
├── lib
└── mymodule.py
In order to ensure that Pyright locates the ‘mymodule.py’ file located in the ‘lib’ folder, add the following code in `pyrightconfig.json`:
xxxxxxxxxx
17 1{
2 "pythonPath": [
3 "${workspaceFolder}/src",
4 "${workspaceFolder}/lib"
5 ]
6}
7
This advises Pyright to include both `src` and `lib` folders while checking for imports in the Python scripts.
2. Use the ‘extraPaths’ Feature
Occasionally, you may encounter issues when your Python interpreter differs from the Pyright version. In such cases, include the Python path manually into your `pyrightconfig.json` using ‘extraPaths’.
Fortunately, Pyright launched a feature known as ‘extraPaths’, designed to resolve files originating in different locations. Here’s how to include your Python path:
xxxxxxxxxx
16 1{
2 "extraPaths": [
3 "/home/user/path/to/python/interpreter"
4 ]
5}
6
The Pyright should now successfully resolve all the import statements coming from this Python interpreter.
3. Explicitly Enable ‘venv’
For developers who consistently leverage virtual environments via ‘venv’, it’s paramount to indicate the specific venv directory on your Pyright config. Pyright does not automatically detect virtual environments; hence, you need to specify the venv pathway explicitly.
xxxxxxxxxx
15 1{
2 "venvPath": "/absolute/path/to/venvs",
3 "venv": "name_of_venv_directory"
4}
5
Now, Pyright can easily register any libraries installed in this particular virtual environment.
Remember, the Pyright error message “import could not be resolved” typically signifies a configuration problem in relation to your project directory and file structure. By properly configuring the `pyrightconfig.json`, the issue can be quickly alleviated.
I would highly recommend perusing Microsoft’s official documentation on Pyright Configuration for a comprehensive look at how to manipulate these configurations to your advantage.To avoid the common pitfalls with module management in Python and specifically address the “Import could not be resolved” error reported by Pyright, a static type-checker for Python, complete understanding and correct execution of several key practices related to Python’s import system is necessary.
Understanding and Correctly Using Absolute and Relative Imports
An absolute import specifies the full path to the Python module or package relative to the project root, while a relative import uses the dot notation to specify location relative to the current module. Using absolute imports can help avoid some namespace collisions and confusion over where a module lives.
Alternatively, relative imports can cause issues if not handled correctly. These are especially problematic when importing from a module above the current one in the directory structure.
xxxxxxxxxx
14 1 # example.py
2 from . import my_module # This is a relative import
3 import my_project.my_module # This is an absolute import
4
Avoiding Circular Dependencies
A circular dependency occurs when two or more modules depend on each other either directly or indirectly. This creates infinitely recursive loops that result in runtime errors.
When Python encounters an import statement, it checks the module registry to see if the module has been imported before. If it hasn’t been previously imported, it proceeds to load the module. With cyclical dependencies, the module isn’t fully loaded before it’s called upon by another import statement which needs it to function correctly – generating the RuntimeError.
xxxxxxxxxx
14 1 # module1.py
2 import module2
3 ...
4
xxxxxxxxxx
14 1 # module2.py
2 import module1
3 ...
4
Properly Structuring Your Project
Python recognizes modules and packages based on the directory structure of your project. If your project is not properly structured, you might run into problems when trying to import modules. A recommended structure should look like this:
xxxxxxxxxx
17 1my_project/
2│ __init__.py
3├── module1.py
4└── subpackage/
5 ├── __init__.py
6 └── module2.py
7
In this setup, every directory having a
xxxxxxxxxx
11 1__init__.py
file is treated as a package, including the project root, and modules can be imported easily without errors. For integrating with Pyright, additional configuration may be needed. Check out the official GitHub repo for Pyright for more details.
Using these strategies, you can better manage your Python modules and avoid frustrating problems such as the “Import could not be resolved” error reported by Pyright. It’s important to have a firm grasp on Python’s approach to modules and packages, to maintain clean, error-free codebases!When using Pyright, a static type checker for Python, you may experience an “Import could not be resolved” error. This means that Pyright cannot locate an imported module or library. Typically, this happens when the necessary requirements have not been correctly installed or there are misconfigurations in your development environment.
Firstly, ensure that all imported modules have been installed within your project’s virtual environment.
This can be confirmed through the use of
xxxxxxxxxx
11 1pip list
command which shows the packages installed within your current virtual environment. If a required package isn’t listed, install it through
xxxxxxxxxx
11 1pip install package-name
.
For instance, if your code includes
xxxxxxxxxx
11 1import numpy
and you’re getting the import error from Pyright:
xxxxxxxxxx
11010 1$ pip list
2/**
3Output:
4Package Version
5--------------- -----------
6pip 22.0.2
7setuptools 60.7.1
8wheel 0.37.0
9**/
10
As shown above numpy is missing. Install it with the command below:
xxxxxxxxxx
11 1pip install numpy
The error might persist even if the relevant packages are installed. In such cases, it’s likely due to the fact that Pyright doesn’t know where to find those packages. Directing Pyright on the correct path can be accomplished by defining the
xxxxxxxxxx
11 1python.analysis.extraPaths
attribute in pyright’s configuration file (pyrightconfig.json).
xxxxxxxxxx
14 1{
2 "python.analysis.extraPaths": ["./my_project_folder"]
3}
4
In this case, ‘./my_project_folder’ is the directory where your project files including the installed packages reside. Change this as per your project structure.
Additionally, you may need to set up the interpreter path property, `python.pythonPath`. It should be set to the path of the Python interpreter associated with the Python environment your workspace is utilising.
xxxxxxxxxx
14 1{
2 "python.pythonPath": "/usr/local/bin/python3"
3}
4
In the given example ‘/usr/local/bin/python3’ is the path of the available Python 3 interpreter on my system. Ensure to replace this value with the correct path on your machine.
If these strategies do not resolve the problem, or if there are issues with the configurations, working with a language service plugin compatible with your code editor such as Pylance (for VSCode) or Coc-python (for Vim) could mitigate these challenges. These plugins uses Pyright and provide features like auto-imports, type information and intelligent code completions which could improve your coding efficiency greatly.
Remember, Advanced Problem-Solutions With Pyright are achievable with a properly set up environment and understanding of its functionalities. The steps discussed will boost your programming efficiency and address common hitches encountered with Pyright like the dreaded “Import Could Not Be Resolved” error.
Often, programmers encounter the “Import Could Not Be Resolved” error when utilizing Pyright. This situation arises due to Python’s dynamic typing system not being able to comprehend the data type at compile time. It might also be due to the system not identifying specified modules or libraries during importation despite them residing in the correct directory.
Pyright and the “Import Could Not Be Resolved” Error
It’s key to understand that Pyright is a Microsoft product, developed to flag Python static typing issues within integrated development environments (IDEs) like Visual Studio Code. Its effectiveness makes it an attractive option for many Python developers. However, the error message “Import Could Not Be Resolved” creates a significant stumbling block.
The insightful solution here would involve checking a couple of things:
- Properly exploiting Pyright settings by using
xxxxxxxxxx
11 1python.analysis.extraPaths
in your
xxxxxxxxxx
11 1settings.json
- Making sure your mentioned libraries are installed and on Path
- Opting for absolute imports instead of relative ones
- Trying out a different but compatible linter or type checker like pylint, MyPy, among others
Example
Here’s how you can control Pyright settings:
xxxxxxxxxx
14 1{
2 "python.analysis.extraPaths": ["relative_path_to_directory"]
3}
4
The true resolve often lies in understanding the core issue. Analyzing the message “Import could not be resolved” might reveal that the problem consists of more than just missing importations. It might uncover insights regarding possible incompatible type checkers. Understanding this could help you employ better strategies to handle such situations in future Python development endeavors.
Additionally, note to always keep your modules or libraries up to date. Old versions may not be compatible with newer language features, leading to overlooked bugs or errors. It’s also essential to follow conventions when importing modules and to maintain consistency across individual files and projects. Further, code readability is critical, especially in large or complex systems; a neat and clear coding style can ease debugging and improve the overall performance.
Bridging the gap between what Pyright expects and what’s written in Python code mitigates instances of the “import could not be resolved” error. Offering simplicity, productivity efficiency, and speed to help you scale your Python Development processes.
For more detailed information about Pyright, and how to troubleshoot its common issues, refer to this
link to their official documentation.. A Stack Overflow post
addressing this particular query can be found here which provides useful developer-driven discussion on the topic.”