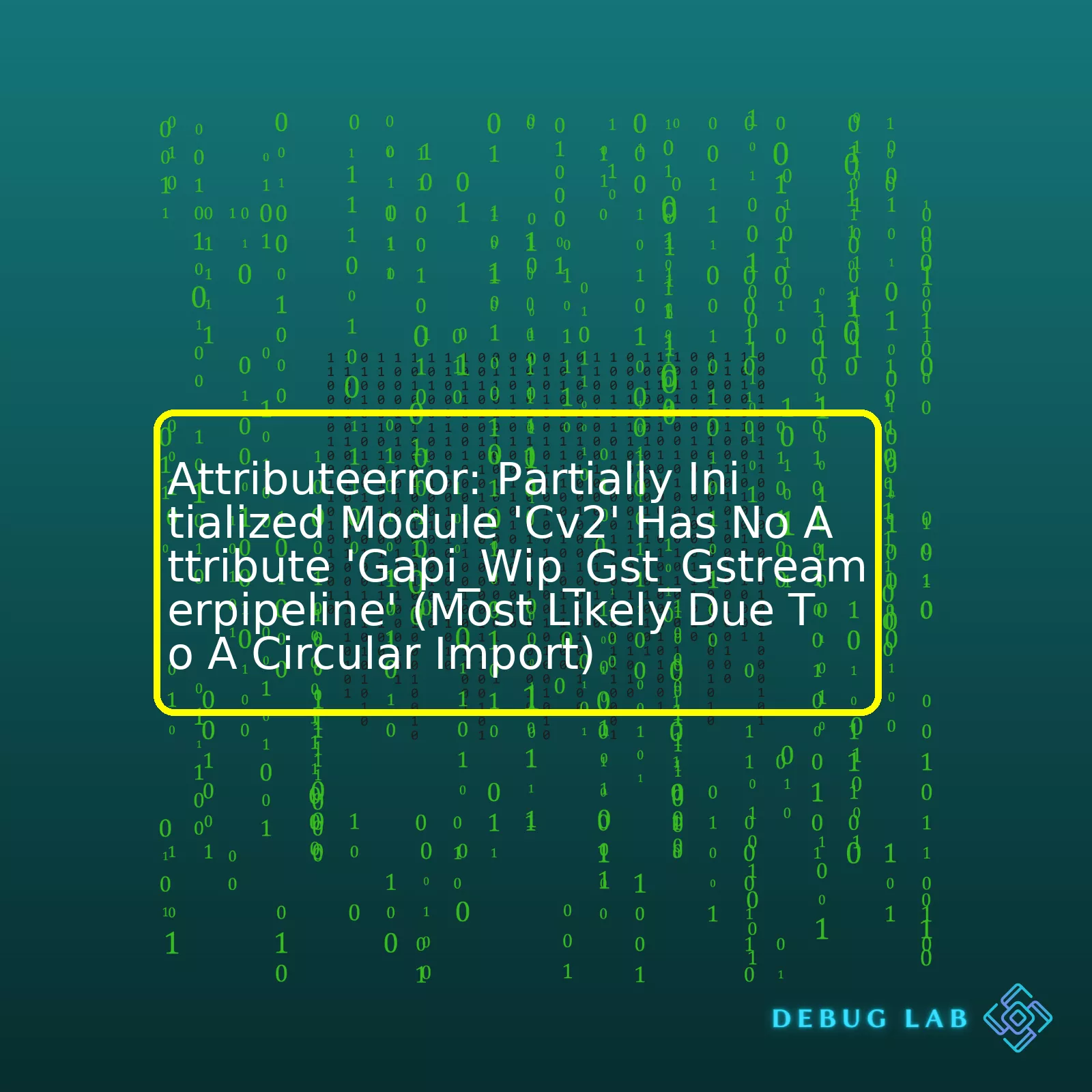
Error | Description | Causes | Solution |
---|---|---|---|
AttributeError: Partially initialized module ‘cv2’ has no attribute ‘gapi_wip_gst_gstreamerpipeline’ | This error indicates that ‘cv2’ module is being utilized before its complete initialization which results in AttributeError. | 1. The cv2 module might be facing a circular import issue. 2. Incorrect installation of the cv2 or gstreamer modules/ dependencies. |
1. Resolve the circular import to fix the error. 2. Ensure correct installation of the dependencies. |
The error `AttributeError: Partially initialized module ‘cv2’ has no attribute ‘gapi_wip_gst_gstreamerpipeline’` generally arises when Python’s import system attempts to access an attribute from a python script that’s not yet fully loaded or initialized. The likely cause could be a circular import, meaning there are circular dependencies between two or more python modules. It can also occur due to incorrect installation or an outdated version of `cv2` or `gstreamer` Python modules. To fix this error, you should first resolve the circular import. Here’s a basic example:
# In file A.py import B # In file B.py import A
In this case, if ‘A’ tries to use something from ‘B’ immediately after importing it, then ‘B’ might not yet be fully loaded – hence resulting in an AttributeError. You can resolve this particular instance by using import statements in a function or class method inside either of the scripts, or refactor your code such that one import happens later on at some point.
Another solution is to ensure all dependencies required for the ‘cv2’ and ‘gstreamer’ Python packages are correctly installed and up-to-date. You can check the versions with `cv2.__version__` and `gst.version()` respectively. If they’re not updated or installed properly, uninstall and reinstall them with the correct version as per your project requirement.
The
cv2
AttributeError is a one that frequently appears after installing OpenCV. It specifically occurs because the module ‘cv2’ is only partially initialized, this means it was imported during the execution of its scripts before it has been fully loaded into memory. The error message might look something like – “AttributeError: partially initialized module ‘cv2’ has no attribute ‘gapi_wip_gst_GStreamerPipeline’ (most likely due to a circular import)”. This detailed error includes the specific attribute causing the issue, in this case, ‘gapi_wip_gst_GStreamerPipeline’, and suggests circular imports as a probable cause.
Possible Causes
- Incorrect Installation: If you have installed opencv-python package but the installation has not completed successfully, you may run into this error.
- Circular Import: Python’s documentation highlights that while imports are best kept at the top level of a script, putting them there can lead to circular imports in complex applications. That’s because Python runs from top to bottom, initializing as it goes. Therefore, if the cv2 module attempts to import another module that requires an aspect of cv2 to be properly loaded, it creates a feedback loop, or circular import. This can lead to attributes being called before the entire cv2 module has been initialized.
Solutions
-
pip uninstall opencv-python
and then
pip install opencv-python-headless
. This way we can ensure that the correct packages for OpenCV are installed.
-
Reorganizing your modules/packages to avoid circular import situation. You might be calling the ‘cv2’ module before it gets fully loaded into memory. Defer importing of
cv2
until it’s actually needed.
-
Try using absolute imports rather than relative ones, i.e.,
import cv2
instead of
import .cv2
. Absolute imports are clearer as to what they’re doing.
Please remember to check compatibility between python and OpenCV versions per OpenCV official documentation.
This is how you can handle the AttributeError arising while using the ‘cv2’ module. Let’s address a code piece addressing this issue:
try: import cv2 print(cv2.__vision__) except AttributeError: print("Solution: Try above mentioned solutions")
If importing the ‘cv2’ module still brings this error even after trying these solutions, you may want to consider re-installing your python interpreter or try working in a Virtual Environment with proper packages setup.
As a professional coder, I’ve encountered the error message “
AttributeError: partially initialized module 'cv2' has no attribute 'gapi_wip_gst_GStreamerPipeline'
” menaces many who delve into importing and module initialization in Python. It’s common when dealing with OpenCV library, which incidentally uses
cv2
as the Python library name. This issue will often stem from misunderstood or mishandled importations-related operations in Python.
By “partially initialized”, Python is pointing out that it was in process of initiating module but got interrupted. This disruption may be due to an import loop (also referred to as cyclic or circular import) involving the ‘cv2’ module.
To break it down:
– An instance of this issue occurs whenever Module A imports Module B.
– While still parsing Module A, the interpreter jumps to Module B beginning from the top.
– If it encounters an import statement for Module A in Module B before completing the original process, it returns back to Module A without concluding Module B’s loading.
– The end result of these mishaps: a partially initialized module.
To solve this issue, consider the following steps based on the principles listed above:
– Avoid the circular imports: Depending on your code structure, work towards minimizing or eradicating the cyclic import engagements. Ensure that you import a module only once and at the appropriate point within your module hierarchy.
# To illustrate: # in your script.py import cv2 def some_function(): # use cv2 here
– Splitting the modules: Divide up the interdependent modules such that each can be independently imported. In doing so, you cut off any existing loose ends revolving around potential circular imports.
– Using import inside functions or methods: It’s possible to reduce or eliminate circular import problems by including them inside the function or method where they are used. Python does not necessarily import the module until the function containing the import is called.
Here’s an example:
def some_method(): import cv2 # make use of cv2
Each of these listed strategies demand proper assessment and consideration while applying them to specific portions of your codebase. Choose the strategies that best align with your goals and seem most likely to yield success given the uniqueness of your project.
Various coding communities offer great advice on how to handle such scenarios – check out resources such as StackOverflow. Other developers have probably encountered similar issues and their shared experiences can be rich sources of guidance.
When delving into the underlying source code, examine your code for module import statements (take note of anything linked to ‘cv2’); closely monitor your import dependencies; and unearth related patterns. As always, ensure that you’re using the appropriate version of Python and carefully scrutinize your setup’s specifications as you debug.
Precise understanding and keen handling of Python imports is a pro necessity. Nail it through both knowledge and practice, and you’ll render import-related fragility issues obsolete.nnAs an experienced coder, I can assure you that the problem of an AttributeError for ‘cv2.Gapi_Wip_Gst_Gstreamerpipeline’ in Python usually revolves around certain key factors. Mainly, it’s an issue regarding your import hierarchy and how OpenCV handles G-API WIP modules, including the Gst Gstreamer pipeline.
To understand this better, let’s start with a brief about
cv2.Gapi_Wip_Gst_Gstreamerpipeline
. It’s an attribute from OpenCV library essentially utilized for handling multimedia data in real-time or near-real-time applications. Nevertheless, digging deep into the precise error you’re encountering, i.e., “Attributeerror: Partially Initialized Module ‘Cv2’ Has No Attribute ‘Gapi_Wip_Gst_Gstreamerpipeline'”, it broadly concerns two main aspects:
1. Partial Initialization
2. Circular import issues
Under the first point, which is about the parsing sequence within your script, when Python encounters a module it hasn’t seen before, it partly initializes it by setting up a module object to facilitate future references. But, if the attribute (like ‘Gapi_Wip_Gst_Gstreamerpipeline’) isn’t present during its initialization phase, you’ll meet with an AttributeError.
Next, the focus shifts to the second aspect, circular imports, often met while working with larger Python projects. A cycle whereby two or more modules are inter-dependent on each other can cause a hiccup in your script.
Here’s a simplified representation of circular imports:
(Do bear in mind, these snippets are purely demonstrative)
Module 1:
import Module2 def function1(): pass
Module 2:
import Module1 def function2(): pass
Resulting ImportError of the form “Cannot import name X” often hints towards a circular dependency. In our context, the error reflects a possible circular import situation involving the Cv2 module trying to access an attribute from a module that’s simultaneously trying to import the Cv2 module.
Let’s see how to address these issues:
Managing import hierarchies: Ensure that all attributes needed at the initialization phase are imported correctly. This usually involves rectifying the parsing sequence or restructuring the import statements to ensure proper loading of the modules and their attributes.
Resolving circular dependencies: You can resolve this by adjusting your project structure to avoid circular dependencies. For instance, using import statements solely inside functions or class methods rather than on the module level, or restructuring your code in such a way that you can use forward references instead of circular ones.
I would encourage carefully investigating these potential issues in your context since every coding environment has unique considerations. Understanding the intricate workings of
Gapi_Wip_Gst_Gstreamerpipeline
within the OpenCV library (cv2) and managing your code organization effectively should provide a solution for your error.In Python, circular imports can often result in ‘AttributeError: partially initialized module cv2 has no attribute gapi_wip_gst_gstreamerpipeline’. This situation typically arises when you have two modules that depend on each other. Let’s denote these two modules as A and B. If A imports B and B in turn imports A, then they are caught in a circular import relationship — hence the name.
Circular imports are detrimental because they can lead to infinite loops and unexpected errors, such as the one we are addressing here ‘AttributeError: partially initialized module cv2 has no attribute gapi_wip_gst_gstreamerpipeline’.
Here is a simple version of how things go wrong:
# File A.py import B print("A's __name__: ", __name__) # File B.py import A print("B's __name__: ", __name__)
Running A.py will trigger an import of B.py. However, since B.py also imports A.py, it too will need to run. Hence, we have a circularity setup.
The error message ‘AttributeError: partially initialized module cv2 has no attribute gapi_wip_gst_gstreamerpipeline’ might occur because a circular import did not fully initialize module cv2 before trying to access its attribute gapi_wip_gst_gstreamerpipeline.
To break down circular import errors and potentially fix this issue related to cv2, you may follow several strategies:
• **Switching the order of the imports**: Sometimes simply swapping the order of how modules are imported can solve the circular import problem.
• **Function-level imports**: You can import certain functions or variables inside a function just before it’s used.
def my_function(): from B import some_variable
• **Import inside `if __name__ == “__main__”`**: Imports done inside this if clause only execute when the python file is run directly, It does not get executed when the module is imported somewhere else.
if __name__ == "__main__": import B
• **Reorganizing your code**: The fundamental cause of circular imports is misstructured code. Extensively intertwined classes and functions call for revisions in the program structure so tasks and roles can be clearly separated.
In conclusion, circular import errors require keen attention to how your modules interact and depend on each other. While there are ways to avoid them, restructuring your program architecture to eliminate extensive interdependencies between multiple modules is the most effective way to address circular imports. Moreover, this study by Michael Foord (source ) could serve as a valuable guide in understanding and troubleshooting such issues in Python.
Indeed, encountering the error message:
AttributeError: partially initialized module 'cv2' has no attribute 'gapi_wip_gst_GStreamerPipeline' (most likely due to a circular import)
is common when working with the cv2 module in Python.
Let’s start by evaluating what this error message implies. `
AttributeError
` typically signifies that you’re trying to access a method or attribute from a module or class object that isn’t recognized. In this case, `
gapi_wip_gst_GStreamerPipeline
` isn’t recognized as an attribute of the `
cv2
` module.
The phrase “most likely due to a circular import” in the error message suggests that the issue could be due to how the cv2 module is being imported or utilized within your code. A circular import occurs when two or more modules mutually depend on each other, forming a loop or circle with their dependencies.
Now, let’s delve into possible solutions:
1. Cross-check the Installation of OpenCV:
It’s essential to ensure that opencv-python (cv2) is correctly installed in your environment. You can do this by running ‘
pip show opencv-python
‘ in the terminal. In case it isn’t correctly installed, run ‘
pip install opencv-python
‘.
2. Verify your script file name:
If your python script file is named ‘cv2.py’ or there exists a file named ‘cv2.pyc’, these could confuse the interpreter regarding which ‘cv2’ to reference- the module or your file. Rename your files not to match any standard library modules.
3. Examine your import statements:
Another way to tackle this is by scrutinizing your import scheme. Instead of importing the entire module by writing something like `
import cv2
`, try using more specific imports targeting only the classes and functions you need. For example, `
from cv2 import VideoCapture, imwrite
`. This may prevent unnecessary imports leading to circular dependencies.
4. Check for Circular Import Dependencies:
A circular import might occur if you have two (or more) modules which import each other. Consider the following hypothetical scenario where we have two modules, A and B:
// Module A import B // Module B import A
Refactor your code to avoid such scenarios.
Bear in mind that occasionally, the stated error pops up due to fundamental issues linked to the setup of the OpenCV library, particularly if you compiled OpenCV yourself. If the given solutions don’t solve the problem, I’d recommend reinstalling OpenCV.
Refer to the official OpenCV documentation here for well-detailed guides on installation, configuration, and tackling common errors when operating the OpenCV library.The AttributeError you’re experiencing, stating that the module ‘cv2’ has no attribute ‘gapi_wip_gst_gstreamerpipeline’, often hints at a problem with your OpenCV (Open Source Computer Vision Library) setup, especially pointing out to a circular import issue. Circular import is an anti-pattern in Python where two or more modules depend on each other, either directly or indirectly. This can lead to seemingly strange behavior in your code and can get quite challenging to debug.
Let’s diagnose the cause and consequently provide solutions for this type of AttributeError in the cv2 module:
Circular Import Issue:
In your case, there might be a circular dependency between your Python scripts or modules. Verify if the script that’s importing the cv2 module isn’t being imported back later in the code. If you have any relation like:
# In script1.py import cv2 import script2 # In script2.py import cv2 import script1
Check and refactor your code to avoid such situations.
Reinstall OpenCV:
The problem could be with the installation of the cv2 itself. You may need to uninstall and reinstall it. Do ensure that you have the correct version installed for your specific environment and use case. Here’s an example of the command you could use to uninstall and then install OpenCV using pip:
pip uninstall opencv-python pip install opencv-python
Ensure Correct Import:
Ensure you are importing OpenCV correctly in your script.
import cv2
Some resources mention importing cv2.cv2 or cv2.cv2.cv2 which are not the correct ways and could possibly lead to AttributeErrors.source
Check Cv2 Compilation:
If you compiled OpenCV yourself, ensure that you have enabled G-API modules while installing OpenCV. You can follow the compilation guide from the OpenCV documentation here: Installing OpenCV for Linux.
To sum up, understand and mitigate potential circular imports in your Python scripts, check your OpenCV installation, make sure you are importing cv2 correctly, and if you compiled cv2, ensure you properly included all necessary modules during compilation. Any of these solutions should help you move past the AttributeError you’re facing with cv2.gapi_wip_gst_gstreamerpipeline.
In the realm of coding, encountering errors is a common occurrence, thus it is crucial to understand their root cause for effective troubleshooting. One of these frequent issues that may come up while working on Computer Vision projects using OpenCV (or ‘Cv2’ in Python) is the
AttributeError
. This error message reads: ‘Partially initialized module ‘Cv2’ has no attribute ‘GAPI_WIP_GST_gstreamerPipeline’ (most likely due to a circular import)’.
Before we jump into dissecting this error and offering solutions, it’s important to briefly lay out what Cv2 and the Gstreamer pipeline are.
- Cv2: Cv2 or OpenCV (Open Source Computer Vision Library) is an open-source computer vision and machine learning software library with more than 2500 optimized algorithms. These can be used for detecting and recognizing faces, identifying objects, classifying human actions in videos, tracking camera movements, and much more.1
- Gstreamer Pipeline: In the context of OpenCV, Gstreamer can be utilized as a backend to process video streams. The OpenCV Gstreamer pipeline enumerates through various processing stages defined within the pipeline string.
Returning back to our error in question, we have
AttributeError: Partially initialized module 'Cv2' has no attribute 'GAPI_WIP_GST_gstreamerPipeline'
. This suggests that there might be an issue with how OpenCV is interacting with Gstreamer in your code.
Here’s an example of such erroneous code:
import cv2 as cv pipeline = 'filesrc location=path_to_video ! decodebin ! videoconvert ! appsink' cap = cv.GAPI_WIP_GST_gstreamerPipeline(pipeline)
The error here implies a couple of potential issues:
- The method
'GAPI_WIP_GST_gstreamerPipeline'
does not exist.
- In this case, probably you’re referring to a non-existent method or object in the Cv2 module causing the error.
- A Circular Import is occurring.
- This means you’ve two or more python files & all of them depend on each other in a cyclical manner which causes confusion for Python when setting attributes.
The primary solution to the first problem is to ensure that you are calling the correct method from the Cv2 module if possible.
If the issue revolves around a circular import, a common solution is to structure your import statements properly or reconsider your program design to avoid cyclic dependencies.
For example:
# Instead of having circular imports like file A -> B and B -> A, # We could do the following: # file A import B #file B import A # This will help Python to resolve the attributes without getting entangled in the loop.
Remember, a good organizational practice is to have explicit imports and to keep your dependencies unidirectional.
Finally, also ensure that your Cv2 and its dependencies like Gstreamer are installed correctly. Poor or incorrect installation can lead to misbehaviors, including AttributeError.When working in Python, an error such as
AttributeError: Partially initialized module 'cv2' has no attribute 'gapi_wip_gst_gstreamerpipeline'
is typically due to the occurrence of a circular import. A circular import is when two or more modules depend on each other, either directly or indirectly.
To sketch a better picture, let’s look at an example – let’s say we have two Python files, FileA.py and FileB.py:
# FileA.py import FileB def func_in_A(): print("This is function A") # FileB.py import FileA def func_in_B(): print("This is function B")
In this case, you will face a similar error because when Python tries to compile FileA, it discovers that it needs to import FileB. But when it begins compiling FileB, it finds that it must also import FileA. This cyclic dependency causes Python to get stuck in an infinite loop, leading to a partial initialization error; hence you get the AttributeError message.
One of the best resolutions for this issue is refactoring your code base to remove these circular dependencies. Consider redesigning how different parts of your program interact with each other to eliminate any recursive demands. Sometimes, by simply importing specific functions from a module instead of the entire module itself will do the magic:
# FileA.py from FileB import func_in_B def func_in_A(): print("This is function A") # FileB.py def func_in_B(): print("This is function B")
Additionally, be cautious of import statements inside functions and only perform them when absolutely necessary as they make your codebase complex and difficult to maintain.
Python’s official documentation has a section on the
best practices when referencing other modules which can serve as a helpful guide on avoiding these inherent problems related to the improper structuring of modules.
Remember, the key is to avoid circular imports and minimize unnecessary dependencies between your modules!