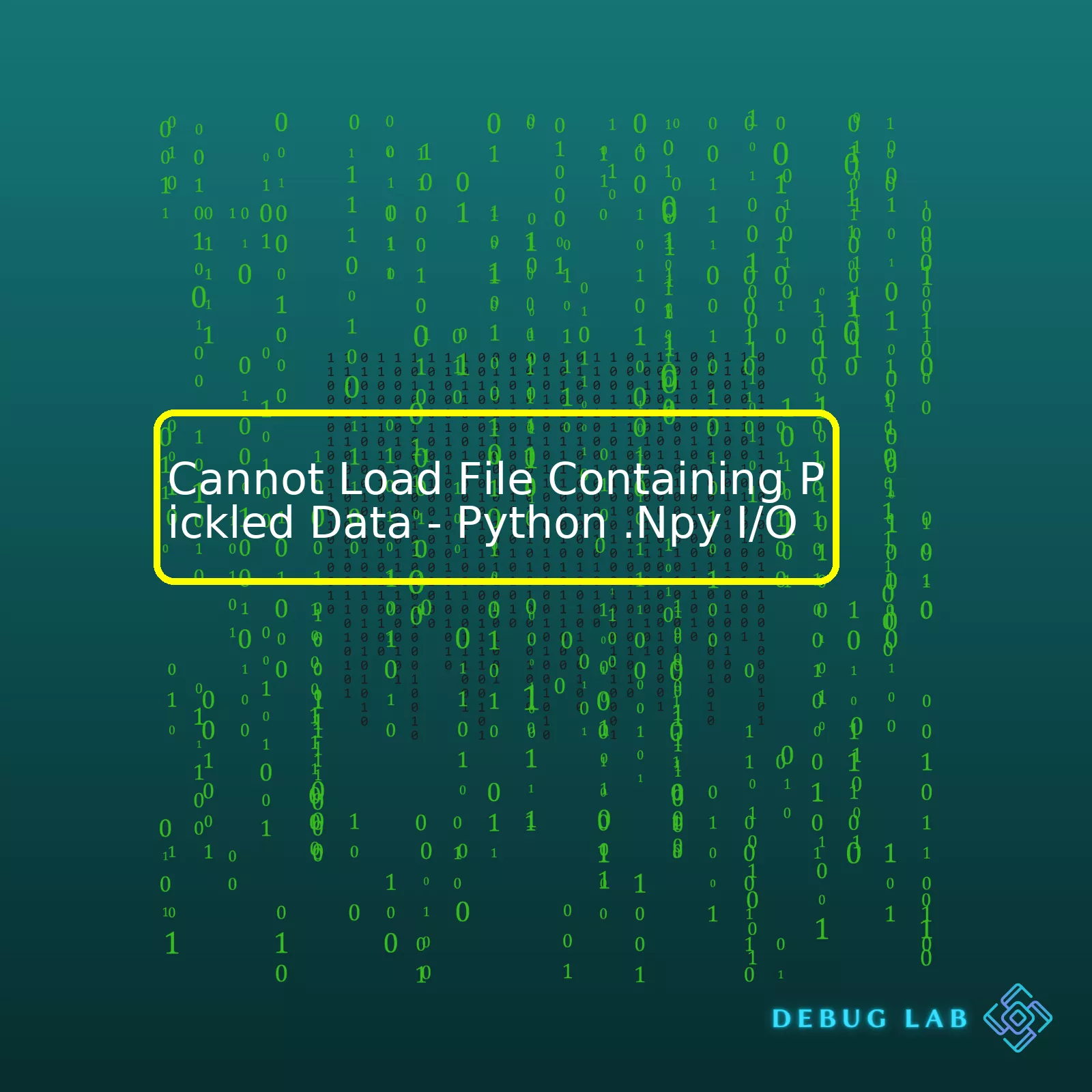
Error Message | Cause | Solution |
---|---|---|
Cannot Load File Containing Pickled Data | Mismatched numpy versions between creation and loading of .npy file | Ensure the same numpy version at both ends or recreate the npy file with the newer version of numpy |
Error Message
Cause
Solution
Cannot Load File Containing Pickled Data
Mismatched numpy versions between creation and loading of .npy file
Ensure the same numpy version at both ends or recreate the npy file with the newer version of numpy
The pickling process is a mechanism for serializing and de-serializing python object structures. When a python object is pickled, the object’s data is efficiently converted into a byte stream, which then can be saved to a disk file. This is how the numpy library saves arrays, using the .npy format.
However, an issue arises when attempting to load a .npy file generated by an older version of numpy with a newer one, or vice versa. There could be incompatible features within the object structure, or different ways of handling the data, causing numpy to fail when trying to unpickle it.
The most optimal way to rectify this situation is to ensure that when you’re saving and loading .npy files, the version of numpy should be consistent. This means that if you’ve used numpy version 1.15.4 to create and pickle your .npy file, use the same numpy version 1.15.4 to load it back. However, if you can’t control the versions on each end (for instance due to other dependencies), you may need to recreate the npy file with the newer version of numpy, since .npy is chiefly meant for temporary storage.
Remember, even though numpy provides backward compatibility, the principles stated above hold vital in the case of transitioning from major version changes where significant modifications occur. For more detailed information about numpy’s functioning, you can check out their official documentation available at https://numpy.org/doc/. Also, there are numerous questions related to this topic on StackOverflow like this one.The Python
xxxxxxxxxx
.npy
file format is a simple format for saving numpy arrays to disk with the full information about them. This includes data type, shape, and the data itself. When you load this data back from the file (or “unpickle” it in Python terms), you obtain exactly the same array as it was at the moment of saving.
However, you’re finding that you cannot load a
xxxxxxxxxx
.npy
file containing pickled data into your Python program – let’s delve into possible causes and solutions for this issue:
1. Incompatible Python or Numpy versions:
When Python pickles its objects, it writes binary data that includes detailed meta-information like the object’s class and any attributes it has. So if you are trying to load the data with different Python interpreter or a significantly different version of NumPy library, the unpickling process may fail to interpret the metadata correctly and throw an error.
2. Incorrect Data Path:
Always ensure that your file path is correctly specified and exists. A common mistake made by many coders including myself, is misspelling the file name or not providing the full path to the file. It’s better to use absolute paths or verify your relative path correctness. For instance:
xxxxxxxxxx
import numpy as np
data = np.load('/absolute/path/to/your/file.npy')
3. File Might Be Corrupted:
This might happen due to factors like improper shutdowns, software crashes, power failures during the write process etc. In such a case, you need to create and save your file again to resolve the loading issue.
4. Loading a Non-Pickled File:
While trying to load an array from a file using
xxxxxxxxxx
np.load()
, you should make sure that the file indeed contains a pickled Python object. If it was saved via some other way, you might need to use another function like
xxxxxxxxxx
np.loadtxt()
or
xxxxxxxxxx
np.genfromtxt()
.
To avoid any potential issues, always check what kind of data you’re writing into the file, its size, and format.
Finally, while searching for your issue online, always append the word “Numpy” to focus more on the related concerns. Explore official documentation, Stackoverflow threads and if still unresolved, ask questions in these forums with detailed explanation and code examples for better solutions.
Remember, diving into issues like this doesn’t only help you learn better coding practices but also contribute towards cleaner, bug-free codebases. Continue coding, continue exploring!Running into the “Cannot Load File Containing Pickled Data” error in Python could be frustrating. This exception is often raised while trying to load .npy files, which are NumPy array files. These files are used to store arrays of numbers, strings and objects, etc. In Python, .npy files can be read using
xxxxxxxxxx
numpy.load()
method. If you come across this hick-up, it’s possibly due to a variety of reasons, each with a different troubleshooting approach:
1) Incompatibility:
Python’s pickle module serializes and de-serializes objects for storage or transportation. But unfortunately, these pickled formats can sometimes differ between Python versions creating cross-compatibility issues.
Suppose, you get the above-mentioned error indeed due to version incompatibility, you could try using a lower protocol version while saving the .npy file. The default protocol uses the highest protocol version available, so specify a lower one like 2 if your use case allows.
Here’s how you save an array ‘a’ using a certain pickle protocol:
xxxxxxxxxx
import numpy as np
a = np.array([1, 2, 3])
np.save('filename.npy', a, allow_pickle=True, fix_imports=True)
And here’s how you’d load it:
xxxxxxxxxx
import numpy as np
data = np.load('filename.npy', allow_pickle=True, fix_imports=True)
The parameters
xxxxxxxxxx
allow_pickle
and
xxxxxxxxxx
fix_imports
are optional and should be set to True if you require Python 2/3 compatibility.
2) Corrupt .npy File:
If your .npy file got corrupted, you will also face the same error. You might want to check your file for corruption, especially if it was moved or copied from elsewhere.
Regenerating the file could solve the issue if you have access to the data source. To validate this, you might need to inspect the structure of the file using binary viewers or even simpler methods like viewing the content in Notepad or similar text viewers (be careful with large files).
3) Pickle Vulnerability:
Pickle isn’t secure against erroneous or maliciously constructed data. It’s documented that “Never unpickle data received from an untrusted or unauthenticated source.”source While working in collaborative environments, always ensure the data source is trusted.
4) Improper Use of numpy.load():
When loading .npy files with numpy.load(), consider passing
xxxxxxxxxx
allow_pickle=True
as the function argument. By default, it’s
xxxxxxxxxx
False
due to security reasons, thus not allowing loading of pickled objects.
That would look like this:
xxxxxxxxxx
import numpy as np
data = np.load('filename.npy', allow_pickle=True)
It is important to identify the specific case causing this loading error first to determine an appropriate resolution. Understanding these under-the-hood functioning and potential bottlenecks can minimize future encounters of similar errors improving your code efficacy and efficiency.When you encounter an issue like “Unable to Load .Npy Files” or “Cannot Load File Containing Pickled Data,” this often points to issues with the data loading mechanism such as:
– `numpy.load()` function might be misused.
– The .npy files might not be correctly pickled.
– File may be corrupted or not available in the expected directory.
– Non-matching Python or numpy version.
– The program doesn’t have suitable reading permissions.
Let’s elaborate upon each one.
Misuse of numpy.load() function:
Numpy provides a built-in
xxxxxxxxxx
numpy.load()
function to load the .npy file but sometimes you may use it incorrectly. The syntax should look like this:
xxxxxxxxxx
numpy.load(file, mmap_mode=None, allow_pickle=False, fix_imports=True, encoding='ASCII')
Mistakes in filename or filepath usage in the ‘file’ param can lead to failed loading.
.Npy files not correctly pickled:
A pickling error could occur if the .npy files are not serialized correctly when saved. You should ensure that you’re using
xxxxxxxxxx
numpy.save()
or
xxxxxxxxxx
numpy.savez()
and check filepaths during saving as well.
File Corruption:
If the .npy file was transferred between systems, it might have corrupted due to incomplete transfer or differing operating systems. It is suggested to verify the integrity of the file.
Non-matching Python or Numpy versions:
Sometimes, different Python or numpy versions can cause issues. If the .npy files were created in a different environment, there might be version mismatches causing file loading problems. Always check for compatibility.
Reading Permissions:
Without adequate permissions, your Python program won’t be able to read the file. Ensure the program has proper reading permission to avoid such issues.
To resolve these scenarios:
You can navigate through directories using os module, provide the correct path to the
xxxxxxxxxx
numpy.load()
function. Check if pickling was done correctly or repeat the serialization process. For Python or numpy version problem, prefer creating virtual environment so the application works in an isolated space.
Here’s a simple snippet of how loading a .npy file should look like:
xxxxxxxxxx
import numpy as np
data = np.load('/path/to/your/file.npy')
Moreover, make sure that the file exists at the given path by using
xxxxxxxxxx
os.path.exists()
method.
Regarding the issue of reading permissions, you can use
xxxxxxxxxx
os.chmod()
method to modify the permissions of the file.
References:
Numpy Load – Documentation for numpy load function.
Numpy Save – Documentation explains the correct way to save arrays to .npy files to avoid pickling errors.
Remember, the clarity of your data I/O operations greatly affects the success rate of loading and manipulating .npy files. I hope this comprehensive breakdown helps you navigate and rectify any future difficulties you might encounter while dealing with .npy files.When working with NumPy’s
xxxxxxxxxx
np.load()
function, it’s common to run into issues like “Cannot Load File Containing Pickled Data”. This error arises when you’re trying to load an .npy file that was saved with pickle(data serialization module in python), but the data isn’t being unpickled correctly when you try to load it. Let’s get into the finer details of how to resolve this issue.
Firstly, the
xxxxxxxxxx
np.load()
function is a significant feature in NumPy – a Python library for performing mathematical and logical operations. It’s useful for robust multi-dimensional array computations and stands out in data analysis. You might save arrays into .npy files for efficient storage and retrieval using the
xxxxxxxxxx
np.save()
function (Documentation here). However, it becomes troubling when you now want to load this data with the
xxxxxxxxxx
np.load()
function and it gives a ‘Cannot Load File Containing Pickled Data’ error.
NumPy’s
xxxxxxxxxx
np.load()
defaults to not allowing pickled file loading because of potential security risks. If you trust the source of your .npy file, then the error can be remedied by adding the argument
xxxxxxxxxx
allow_pickle=True
. Here’s an example:
xxxxxxxxxx
import numpy as np
data = np.load('filename.npy', allow_pickle=True)
Now, if the argument
xxxxxxxxxx
allow_pickle=True
fails to solve the problem, the .npy file might be either corrupt or incompatible. In the case of corruption, you might need to re-generate the .npy file. If it’s an issue of version incompatibility, then updating your NumPy library could be the solution. Use the following pip command to upgrade NumPy:
xxxxxxxxxx
pip install --upgrade numpy
While we’re at it, let’s discuss more on NumPy’s
xxxxxxxxxx
np.load()
in terms of its efficient data handling benefits contrary to pure python.
The advantages of NumPy’s binary format over python are as follows:
– Binary format results in smaller files: If you compare the size of the same data saved in .txt (using python) and .npy (using NumPy), npy files take less space.
– Faster to read/write files: The I/O process (reading/writing file from/to the disk) is quicker with npy than txt due to the former’s binary format.
Table: Comparison between NumPy and Python in handling data
Aspects | Python | NumPy |
---|---|---|
Data Format | Text (.txt) | Binary (.npy) |
File Size | Larger | Smaller |
Read/Write Speed | Slower | Faster |
In essence, the ability to load .npy files containing pickled data is a valuable technique for fast and efficient data-driven coding practices in Python. When utilized correctly, it allows you to operate complex data structures beyond simple numerical arrays, including dictionaries, tuples, lists, and combinations thereof – all while addressing potential hiccups and ensuring secure handling through appropriate use of the
xxxxxxxxxx
allow_pickle
parameter.Firstly, let’s break down the problem for better understanding. If you’re having issues with loading a .npy file that contains pickled data, it most likely means Python can’t decode the serialized information. Numpy has its own way of storing arrays in a binary format with
xxxxxxxxxx
.npy
extension. When it tries to open a file not saved via numpy’s methods, it possibly won’t be able to understand the format.
A couple of possible solutions could be to:
– Double-check your method of data saving:
Don’t pickle your data before using numpy.save(). Instead, you’d save your numpy arrays directly with np.save():
xxxxxxxxxx
import numpy as np
# creating an array
array = np.arange(10)
# saving array
np.save('saved_array', array)
Now, you can load this data back into memory with np.load():
xxxxxxxxxx
loaded_array = np.load('saved_array.npy')
print(loaded_array)
– Look at the method you’re using to load the data:
There are two reasons you might end up trying to load pickled data from a .npy file. You could either have saved pickled data to a file with a .npy extension, or your data could’ve been saved with numpy.save() but you’re now attempting to load it with numpy.load(). If the former is true, make sure to use python’s pickle module to load the data:
xxxxxxxxxx
import pickle
file = open('file.npy', 'rb')
data = pickle.load(file)
If the latter is true, try setting allow_pickle=True:
xxxxxxxxxx
data = np.load('file.npy', allow_pickle=True)
Keep in mind though, as of numpy version 1.16.3, loading .npy files with pickled data was disabled by default due to security concerns. So, while enabling pickling might solve your immediate problem, it’s generally recommended to avoid pickling if possible.
– Finally consider re-installing Numpy:
A corrupted installation of Numpy could cause issues when trying to open .npy files. A fresh installation could fix any underlying issues with the library. Uninstall the current installed version and reinstall using pip:
xxxxxxxxxx
pip uninstall numpy
pip install numpy
Each of these steps provides potential fixes for the issue that you’re experiencing with ‘.Npy I/O’. Understanding how pickling works (Python’s official pickle documentation) and the nature of numpy’s save and load mechanics will further help in debugging such issues.
Every software error takes patience and perseverance to address. With these steps, identifying issues and implementing targeted solutions becomes an achievable goal. Happy coding!Typically, the numpy (`.npy`) file format is used to store large arrays efficiently in Python programs, thanks to its capability to save on necessary overhead that comes with other data storage methods. Nevertheless, there might occur a situation where an error message pops up indicating a problem loading a `.npy` file containing pickled data. This issue usually arises due to missteps in the saving and loading process of `.npy` files in Python.
To start saving and loading `.npy` files correctly in Python, follow these practical tips:
– Consider using the `numpy.save()` function to save your .npy files. This will make sure that large numerical datasets take up less space and I/O time. Once you have your data stored properly, it tends to load faster when you later require it in your code. Here’s how to do this:
xxxxxxxxxx
import numpy as np
# Create a numpy array.
array = np.array([1, 2, 3, 4, 5])
# Save the numpy array as .npy file.
np.save('filename.npy', array)
– To effectively load `.npy` files back to NumPy array structures, make use of the related `numpy.load()` function. This method loads `.npy` forms back into your workspace for manipulation. See the example below:
xxxxxxxxxx
import numpy as np
# Load the numpy array from .npy file.
array = np.load('filename.npy')
print(array)
If errors pop up during the process, be sure to check each of the following aspects:
– Corrupt File: The ‘Cannot Load File Containing Pickled Data’ error message may surface because of corrupt or incomplete `.npy` files. If the system crashed while writing the data or if it was manually stopped midway, the file could become corrupted. To prevent file corruption, ensure that the operation completes before closing the script or shutting down the computer.
– Incompatible Pickle Protocol: pickle protocol can affect how your data is serialized and deserialized. An incompatible pickle protocol may trigger this error. It’s worth noting that different versions of Python use different pickle protocols, so be consistent with the Python versions if you’re sharing `.npy` files across different environments.
– Outdated Library: Sometimes, this error occurs due to the existence of outdated numpy library. Upgrading the numpy library may fix this issue.
For further details on numpy’s methods, refer to the official documentation of numpy here.
Remember, carefulness in managing your `.npy` files will mitigate any unwanted scenarios such as data loss or failure to load the data when required by your Python programming tasks.Certainly, I’d be more than happy to review how we can check if we’re ‘unpickling’ our code correctly, and more specifically, help you troubleshoot a problem where you can’t load .npy files.
The first thing to remember is that
xxxxxxxxxx
pickle
isn’t a universal data interchange format. It’s designed to serialize and deserialize complex Python objects, and it does that job perfectly. However, problems can arise because of this narrow focus on Python.
While NumPy uses its own binary format for .npy files, we can use pickle compatibility to load these files, but there are some precautions we have to take.
So let’s get straight into it:
If you’re struggling with loading your pickled data from a .npy file, which is the issue at hand, there’s a possibility that your file has been inadvertently damaged, or the object wasn’t pickled correctly in the first place.
To load a numpy array stored as a .npy file, you should ideally be using
xxxxxxxxxx
numpy.load()
like this:
xxxxxxxxxx
import numpy as np
arr = np.load('my_array.npy')
However, we run into trouble when the original data was not serialized using NumPy’s methods. If instead, it was pickled using Python’s native
xxxxxxxxxx
pickle
, then we need use
xxxxxxxxxx
pickle
for unpickling:
xxxxxxxxxx
import pickle
with open('my_array.npy', 'rb') as f:
arr = pickle.load(f)
It’s crucial to open the file in binary mode (‘rb’ stands for read binary). Regular text modes may change line endings or other special bytes, resulting in corrupted data.
Now, if you’re still getting an error while trying to unpickle data, it could be due to differences in the Python environment wherein the object was pickled and the current one you’re trying to unpickle it. Here there are a few things you can check:
* Python version: Pickle files created in Python 2.x cannot necessarily be read by Python 3.x without changing the encoding.
Like this:
xxxxxxxxxx
with open('my_array.npy', 'rb') as f:
arr = pickle.load(f, encoding='bytes')
This forces Python 3 to interpret the bytestream as it was created in Python 2.
* Check Library Versions: The libraries used to construct objects (like NumPy or pandas) can sometimes affect the ability to unpickle objects serialized with different versions of those same libraries.
* Security Considerations: By default, pickle executes bytecode as part of its deserialization process which may lead to security threats. Always trust the source where you get your pickle files from.
Checking these areas, alongside verifying that your files haven’t been corrupted and you’re using the correct reading functions, are some of the key steps for ensuring that you’re unpickling data as intended, and certainly when working with .npy files created for Python.
Sometimes, you might be using different Python environments for saving and loading the .npy files. In such scenarios, compatibility issues can arise, especially if different versions of numpy are used in these environments. One way to overcome this is by ensuring that you are using the same Python environment consistently. Alternatively, you could work towards making your code compatible across different versions. Here’s how:
xxxxxxxxxx
import numpy as np
# Save numpy array as .npy file
np.save('my_array.npy', my_array)
# Load numpy array from .npy file
my_array_loaded = np.load('my_array.npy')
In other instances, you might encounter pickle errors because the object you’re trying to load includes a custom class or function that is not available in your current scope. Importing these classes or functions before loading the file should fix the issue. If these methods don’t resolve your problem, you may have to consider potential installation issues.
If you believe your installation might be corrupt or incomplete, reinstalling numpy might just solve your problem. A clean install ensures you have all necessary dependencies and nothing is broken or missing. Here’s how to uninstall and reinstall numpy:
xxxxxxxxxx
# Uninstall numpy
pip uninstall numpy
# Reinstall numpy
pip install numpy
To avoid any further similar issues, ensure that your Python environment is up-to-date, and packages like numpy are correctly installed. Good coding practices such as regularly updating your environment and maintaining compatibility in your code will go a long way towards error-free programming.
Remember, working out minor bugs and errors is part of the magic of programming—it turns haphazard lines of code into a well-oiled machine. It’s through tackling these challenges you’ll truly hone your skills as a coder. Happy coding!