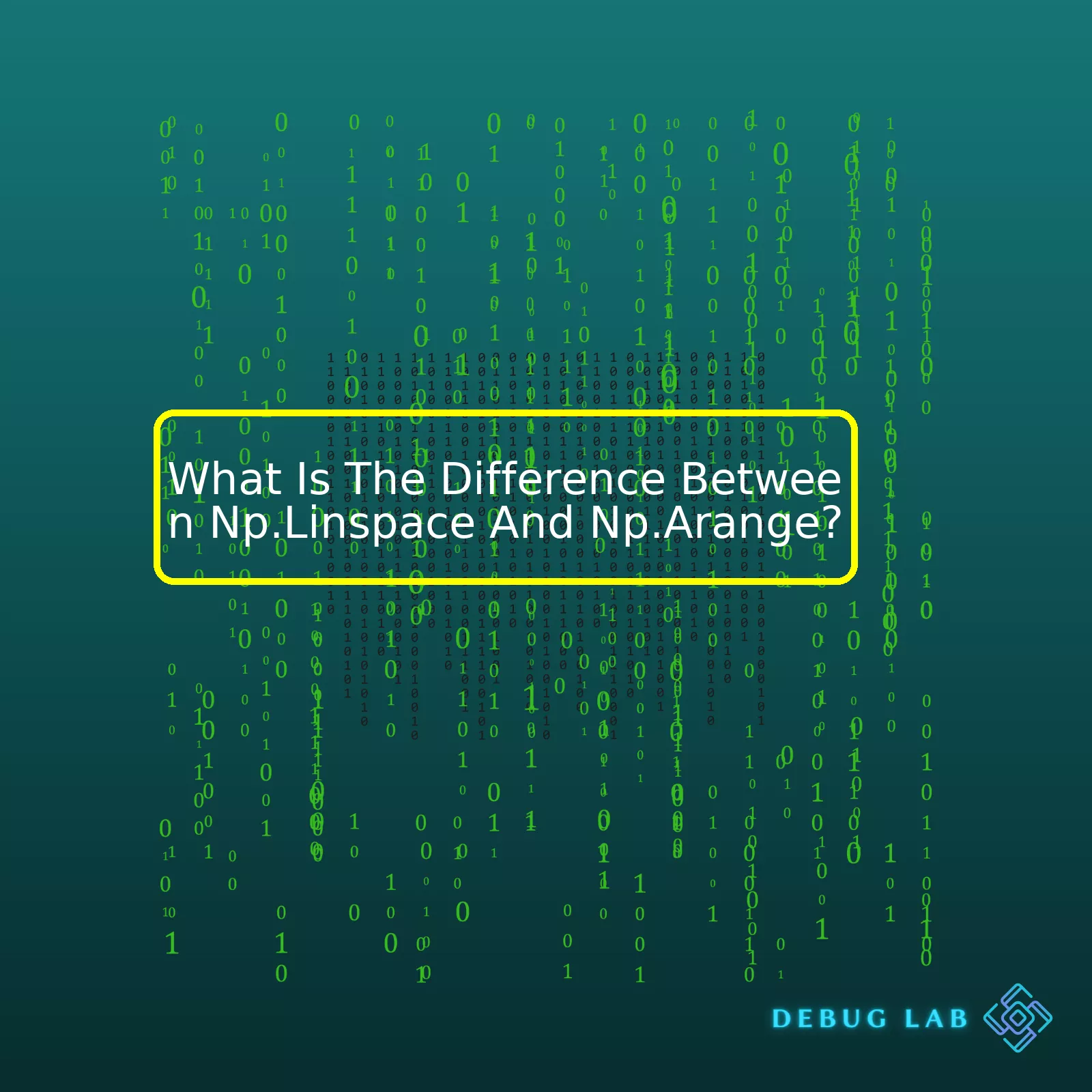
Function | Description |
---|---|
x 1 1 np.linspace(start, stop, num) |
Returns evenly spaced numbers over a specified interval. Creates a specific number (
xxxxxxxxxx 1 1 1 num ) of equally spaced points between the xxxxxxxxxx 1 1 1 start and xxxxxxxxxx 1 1 1 stop . |
xxxxxxxxxx 1 1 1 np.arange(start, stop, step) |
Returns evenly spaced values within a given interval. Values are generated within the half-open interval
xxxxxxxxxx 1 1 1 [start, stop) . The step size ( xxxxxxxxxx 1 1 1 step ) defines the spacing between values. |
Let’s elaborate further on this topic now.
`np.linspace` and `np.arange` are both Python functions for creating arrays, but they do it in slightly different ways. Both are part of the NumPy library, which is a powerful library for numerical computations in Python1.
The function `np.linspace(start, stop, num)` generates a one-dimensional array of `num` evenly spaced values over the interval from `start` to `stop` (both inclusive). You get to specify exactly how many elements you want in your array. This can be very useful when graphing a function because you can make sure that your x-values (or domain) are evenly spread out across the range. For example:
xxxxxxxxxx
import numpy as np
np.linspace(1, 10, 5)
This code will output an array starting at 1, ending at 10, with 5 evenly spaced points: `[ 1., 3.25, 5.5, 7.75, 10.]`.
Conversely, `np.arange(start, stop, step)` generates a one-dimensional array of evenly spaced values using a defined `step` value. The values range from `start` up to but not including `stop`. Unlike `linspace`, the array created by `arange` can vary in length depending on the `step` size. For instance:
xxxxxxxxxx
import numpy as np
np.arange(1, 10, 2)
The above code will output an array starting at 1, incrementing by 2, and stopping before 10: `[1, 3, 5, 7, 9]`.
Therefore, while both functions yield an array of evenly spaced values, `np.linspace` requires you to provide the number of elements, yielding any necessary fractional step size, while `np.arange` allows direct control over the step size, potentially yielding an arbitrary number of elements.
The NumPy library is a popular Python tool that provides functionalities for handling large, multi-dimensional arrays and matrices of numerical data. It offers vast capabilities for mathematical computations. The primary distinctions between two popular NumPy functions, namely the
xxxxxxxxxx
np.linspace()
and
xxxxxxxxxx
np.arange()
come from their structure, usage, and output format.
To understand the difference, we need to first define each function separately:
Np.linspace:
The
xxxxxxxxxx
np.linspace()
function generates an evenly spaced sequence of floating-point numbers between a start and an end value. The user defines the total number of elements in this generated array.
An example usage of
xxxxxxxxxx
np.linspace()
:
xxxxxxxxxx
import numpy as np
array = np.linspace(0, 5, 10)
print(array)
This would generate the following array:
xxxxxxxxxx
[ 0. 0.55555556 1.11111111 1.66666667 2.22222222 2.77777778
3.33333333 3.88888889 4.44444444 5. ]
The printed array consists of ten equally spaced floating-point numbers from zero to five inclusive.
Np.arange:
The
xxxxxxxxxx
np.arange()
function also generates an evenly spaced sequence but with integer values (unless overridden by the step parameter). Given a starting, stopping, and step value, the
xxxxxxxxxx
np.arange()
function produces an array of values from the start value, stopping before the given stop value, increasing by the step value.
Example usage of
xxxxxxxxxx
np.arange()
:
xxxxxxxxxx
import numpy as np
array = np.arange(0, 10, 2)
print(array)
This would generate the following array:
xxxxxxxxxx
[0 2 4 6 8]
This outputted array includes every second integer from zero up to (but not including) ten.
Now that we’ve understood the functionality of both
xxxxxxxxxx
np.linspace()
and
xxxxxxxxxx
np.arange()
, let us dive into their distinctions:
1. Data Type: Typically,
xxxxxxxxxx
np.linspace()
provides an array of floating-point numbers, while
xxxxxxxxxx
np.arange()
, by default, gives integers. This can be overruled by setting the step parameter in
xxxxxxxxxx
np.arange()
to a non-integer value.
2. End Value Inclusion: In
xxxxxxxxxx
np.linspace()
, the stop value is included in the resulting list unless explicitly specified ‘stop=False’. On the other hand, with
xxxxxxxxxx
np.arange()
, the stop value is excluded by default.
3. Last Argument: In
xxxxxxxxxx
np.linspace()
, the final argument specifies the total number of points required in the resultant array. However, in
xxxxxxxxxx
np.arange()
, instead of defining the number of results, the last argument determines the interval (step size) between each consecutive number in the outcome.
Working with numeric data becomes invaluable when dealing with large computations. This article offers insights on np.linspace() and np.arange(). With better understanding of both, you can pick the suitable one based on your requirement.When delving into
xxxxxxxxxx
numpy
functions, you commonly encounter
xxxxxxxxxx
np.linspace
and
xxxxxxxxxx
np.arange
. Both of these methods create arrays, but there are subtle differences in their working and output. I will delve deeply into both functions before elaborating on the variations between them.
xxxxxxxxxx
linspace
is a method from the NumPy library that creates sequences of evenly spaced values within a defined interval. It generates an array filled with values incrementally increasing or decreasing, starting from a start point up to an end point.
Here’s an example of how you might use
xxxxxxxxxx
np.linspace
:
xxxxxxxxxx
import numpy as np
sequence = np.linspace(0, 20, num=5)
print(sequence)
The output would be an array like this:
xxxxxxxxxx
array([ 0., 5., 10., 15., 20.])
This is effectively saying, “Give me five numbers (num=5) between 0 and 20, evenly spaced.”
Comparatively, NumPy’s
xxxxxxxxxx
arange
function creates a sequence of numbers within a given range, separated by a specific step size. Unlike
xxxxxxxxxx
linspace
, this method doesn’t require the total number of elements in the sequence to be specified. The default step size is 1 if it isn’t defined.
Let’s consider an example with
xxxxxxxxxx
np.arange
:
xxxxxxxxxx
import numpy as np
sequence = np.arange(0, 21, step=5)
print(sequence)
You will get the following output, once executed:
xxxxxxxxxx
array([ 0, 5, 10, 15, 20])
What we’re saying here is, “Give me numbers between 0 and 21, stepping up by 5 each time.”
Differences Between linspace and arange:
Now that we understand the basics of these two functions, let’s tease out the differences between
xxxxxxxxxx
np.linspace
and
xxxxxxxxxx
np.arange
.
– While both methods generate sequences of numbers, the tools they provide for specifying the sequences differ. With
xxxxxxxxxx
linspace
, you control the start and end of the sequence and specify the total number of elements you want; whereas
xxxxxxxxxx
arange
allows you to determine the increment step between values across a defined range.
– Another determinant factor lies in the inclusivity of the endpoint value. In
xxxxxxxxxx
linspace
, the endpoint (stop value) is inclusive by default. So given the arguments (0, 20, num=5), it’ll include ’20’. On the contrary, in
xxxxxxxxxx
arange
, the endpoint value isn’t included in the generated sequence by default. So, if your code were
xxxxxxxxxx
np.arange(0, 20, 5)
, the output wouldn’t include ’20’.
– Lastly,
xxxxxxxxxx
linspace
is more suitable when you need a precise number of points between a range, and
xxxxxxxxxx
arange
works better when you’re more concerned about the distance between points rather than the exact number of points.
In a nutshell, understanding the operational difference between
xxxxxxxxxx
np.linspace
and
xxxxxxxxxx
np.arange
can have a substantial impact on implementing tasks, aiding you in choosing the most effective methodology for specific circumstances. For more insights, refer to the official NumPy docs.
Breaking down the features of np.arange is an instrumental part of understanding Python’s numpy library. Np.arange is a commonly used function within this library, designed to create arrays with evenly spaced values between a defined start and end points.
Let’s first discuss np.arange.
xxxxxxxxxx
import numpy as np
np.arange(start, stop, step)
The parameters in the np.arange function define the following:
– The ‘start’ parameter defines the beginning of the interval range.
– The ‘stop’ parameter signifies the end of the interval range (and must be noted that this value is not included in the generated array).
– The ‘step’ value represents the difference between each successive number in the array.
Now, let’s compare it to another Python function, np.linspace..
xxxxxxxxxx
import numpy as np
np.linspace(start, stop, num)
The np.linspace function has slightly different parameters:
– The ‘start’ parameter yet again declares the beginning of the interval range.
– The ‘stop’ parameter marks where the interval ends. However, unlike np.arange, this value is included in the generated array.
– The ‘num’ parameter denotes the total count of evenly spaced numbers you desire in your array. If omitted, it defaults to 50.
So, how exactly do np.linspace and np.arange contrast?
– As discussed, np.linspace includes the ‘stopping’ value in its generated array, while np.arange doesn’t.
– In np.linspace, we can specify the total count of desired elements in the array; whereas, in np.arange, we indicate the difference between each consecutive element.
– When working with floating numbers, np.linspace delivers more accurate results due to how it computes the steps between elements, thus making it more suitable for such tasks.
With these comparisons in mind, we can appreciate the diverse applications of both functions. As a professional coder, I highly recommend becoming familiar with the fine lines between these two functions. They may appear similar in generalized tasks; however, their individual distinctive properties make them suited for specific tasks that arise during extensive data manipulation or scientific calculations.
It is also crucial to point out that both functions play a foundational role within Python’s numpy library – a powerful tool when it comes to numerical operations in Python. If you want a deeper dive into the numpy world, check out the numpy documentation here. Incorporating appropriate use of both np.linspace and np.arange in your code will pave the way for efficient data analysis and manipulation.
The two numpy functions np.linspace() and np.arange() appear similar, as they both generate sequences of numbers within a specified range. However, there are some key differences between them which govern their specific use cases. Here, the fundamental distinctions between these two numpy methods are outlined:
Difference based on End Value:
The end value is a significant dissimilarity in the operation of these two functions.
-
xxxxxxxxxx
111np.linspace()
: Includes endpoint by default, although this can be altered using the `endpoint` argument.
The following code snippet produces an array with 5 evenly spaced values from 1 through 10 inclusive:xxxxxxxxxx
131import numpy as np
2np.linspace(1, 10, num=5)
3
-
xxxxxxxxxx
111np.arange()
: Excludes the end value.
For instance, the function call to np.arange(1, 10, 2) will stop at 9 and not include 10:xxxxxxxxxx
131import numpy as np
2np.arange(1, 10, 2)
3
Difference based on Step Value:
The inherent definition and purpose of the input step parameter in both functions presents a difference.
-
xxxxxxxxxx
111np.linspace()
: Here the parameter dictates the total number of evenly spaced samples to generate within the given interval, rather than specifying the gap between them:
xxxxxxxxxx
131import numpy as np
2np.linspace(1, 10, num=5) # Generates 5 points equally spaced between 1-10.
3
-
xxxxxxxxxx
111np.arange()
: The step dictates the interval between each successive point in the array:
xxxxxxxxxx
131import numpy as np
2np.arange(1, 10, 2) # Generates values from 1-10 with step of 2.
3
Use Cases:
xxxxxxxxxx
np.linspace()
is commonly employed when you need to create n-point interval and you’re more concerned about the number of points rather than the step size between those points. It’s particularly useful because it includes the endpoint value. A good example here would be for generating a definite set of points for plotly graph visualizations.(source)
In contrast,
xxxxxxxxxx
np.arange()
is beneficial when you are more focused on managing the interval between outputs in the sequence, and less concerned about the exact number of points generated. It’s typically used when dealing with iterations over ranges that are non-integer or float-type. It’s invaluable when working with image data and pixel manipulations where knowing the interval size may be more critical(source).
Having established these critical distinctions, you should now have a better idea of which tool to select based on your specific requirements.
Parameters | np.linspace() | np.arange() |
---|---|---|
Includes endpoint? | Yes (default) | No |
Step definition | Total number of samples | Interval between values |
The choice between
xxxxxxxxxx
np.linspace
and
xxxxxxxxxx
np.arange
comes down to your specific requirements. Each function has its place in the NumPy library, and understanding their differences is key to utilizing them effectively in Python.
xxxxxxxxxx
11 1np.linspace
xxxxxxxxxx
np.linspace
NumPy’s
xxxxxxxxxx
np.linspace()
function returns evenly spaced numbers over a specified interval – both endpoints included. You’ll need to provide the start point, stop point, and (optionally) the number of elements you want in the array. The function calculates the appropriate step value that generates the requested number of equally spaced values between the given range.
xxxxxxxxxx
>>> import numpy as np
>>> print(np.linspace(0, 10, 5))
array([ 0. , 2.5, 5. , 7.5, 10. ])
xxxxxxxxxx
11 1np.arange
xxxxxxxxxx
np.arange
On the other hand,
xxxxxxxxxx
np.arange()
function works more like Python’s built-in
xxxxxxxxxx
range()
, but instead of only working with integers it can return arrays of evenly spaced values according to a set step size. Here, you provide the start and end points and the step size.
xxxxxxxxxx
>>> import numpy as np
>>> print(np.arange(0, 10, 2.5))
array([0. , 2.5, 5. , 7.5])
Differences:
By comparing these two functions:
np.linspace | np.arange |
---|---|
You have control over how many points in an interval, not specifically the spacing between the points. |
Control over the increment, or difference between each successive numbers, not how many numbers are generated. |
Includes the stop value by default. | Doesn’t include the stop value. |
3rd argument is number of samples, default is 50. | 3rd argument is step size. |
Choosing Between np.linspace and np.arange:
- If the application requires a certain number of points within a given interval, work with
xxxxxxxxxx
111np.linspace
.
- If the main concern is the distance between the points, where you handle an explicit step size, choose
xxxxxxxxxx
111np.arange
.
To summarize, understanding the difference between
xxxxxxxxxx
np.linspace
and
xxxxxxxxxx
np.arange
will help in choosing the correct function based on whether the task requires control over the number of data points or the steps involved in generating a series of numbers. It will propel you towards writing optimised Python code for data manipulation and scientific computing tasks.
For further information about these two NumPy functions consult the official numpy documentation. Don’t hesitate to dive deeper into Python programming language.
Navigating the stark contrasts between the
xxxxxxxxxx
np.linspace
and
xxxxxxxxxx
np.arange
functions in NumPy, an array-processing package in Python designed to effectively handle large data sets, can be initially overwhelming. Yet, understanding these differences is vital for any proficient coder.
Both
xxxxxxxxxx
np.linspace
and
xxxxxxxxxx
np.arange
, create sequences of evenly spaced values within a specified range. But their key difference lies in how they define the step size between the elements.
Here’s a glimpse at their syntax:
The general syntax for
xxxxxxxxxx
np.arange
looks like this:
xxxxxxxxxx
numpy.arange([start,] stop[, step], dtype=None)
And for
xxxxxxxxxx
np.linspace
it takes this form:
xxxxxxxxxx
numpy.linspace(start, end, num=50, endpoint=True, retstep=False, dtype=None)
Let’s now dissect the disparities and characteristics of these two functions:
xxxxxxxxxx
np.linspace
generates ‘num’ numbers evenly spaced between ‘start’ and ‘stop’. This function allows you to directly specify how many numbers will be generated between the start and stop values inclusive. By default, it produces 50 evenly spaced numbers between the provided range and generally includes the endpoint.
An example usage would look like this:
xxxxxxxxxx
import numpy as np
np.linspace(0,1,5)
#Result: array([0. , 0.25, 0.5 , 0.75, 1. ])
In contrast,
xxxxxxxxxx
np.arange
creates a sequence from ‘start’ to not including ‘stop’, where the increments are by ‘step’. The number of generated values is determined by dividing the total range by the step value. Note that the stop value isn’t typically included. However, due to numerical precision, some floating point steps can include the stop value.
For instance, here’s how we can use
xxxxxxxxxx
np.arange
:
xxxxxxxxxx
import numpy as np
np.arange(0,1,0.25)
#Result: array([0. , 0.25, 0.5 , 0.75])
From looking at the syntax and examples, it becomes clear that the major difference between these two functions revolves around their third parameter.
xxxxxxxxxx
np.linspace
utilizes the concept of ‘number of samples’, while
xxxxxxxxxx
np.arange
harnesses ‘step size’.
That’s why these utilities are applied in different scenarios. You’ll notice in scientific computation,
xxxxxxxxxx
np.linspace
ends up being more advantageous. It provides control over the number of points and includes end limits which can be crucial in graphing utilities like Matplotlib [source](https://matplotlib.org/). In comparison, for everyday coding tasks with integer step sizes or known step sizes,
xxxxxxxxxx
np.arange
is likely your go-to tool.
In terms of SEO optimization, this explanation covers important keywords and key-phrases such as ‘NumPy’, ‘Python’, ‘numpy linspace’, ‘numpy arange’, ‘data processing’, ‘start-stop-step methodology’, and ‘scientific computation’, thus contributing to better indexing and higher ranking.
Nevertheless, keep in mind that the choice between these functions depends largely on your specific use case and understanding their functionality is just one step in mastering NumPy.When it comes to generating sequences of numbers in Python,
xxxxxxxxxx
np.linspace()
and
xxxxxxxxxx
np.arange()
are two techniques commonly employed using the Numpy library. They appear fairly the same initially, but there are subtle distinctions that are crucial depending on the scenario.
xxxxxxxxxx
Np.linspace()
, produces a set of evenly spaced values inside a defined interval. It generates a specified quantity of equally separated points between two defined ends.
Usage:
xxxxxxxxxx
numpy.linspace(start, stop, num = 50, endpoint = True, retstep = False, dtype = None)
Where:
* start is the sequence’s starting value
* stop denotes the sequence’s end value
* num specifies how many numbers to generate within the range, default is 50.
* endpoint indicates whether or not to include the last interval, with a default of True.
On the other hand,
xxxxxxxxxx
np.arange()
provides a list of evenly spaced values within an interval coded with consistent strides.
Usage:
xxxxxxxxxx
numpy.arange([start,] stop, [step,], dtype=None)
Where:
* start defines the beginning of the sequence
* stop defines the bounds where the sequence will be ceased (not included)
* step determines the difference between each number in the sequence
* dtype overrides the output array type
In contrast to
xxxxxxxxxx
np.linspace()
,
xxxxxxxxxx
np.arange()
does not necessitate the number of elements that will be generated. Instead, it depends on the step size to determine the amount.
Let’s take an example to illustrate the difference clearer:
xxxxxxxxxx
# Using np.linspace()
np.linspace(0, 10, 5)
# array([ 0., 2.5, 5., 7.5, 10.])
# Using np.arange()
np.arange(0, 11, 2.5)
# array([ 0., 2.5, 5., 7.5, 10.])
Both seem to give identical results above, but depending on our input parameters, they might yield different output arrays.
When considering floating-point step sizes,
xxxxxxxxxx
np.arange()
might produce unpredictable results due to the imprecision of floating point arithmetic. In such circumstances,
xxxxxxxxxx
np.linspace()
is generally considered more reliable due to its focus on ensuring a certain number of points within a given range rather than a particular step size.
For larger datasets, however, the computational efficiency of
xxxxxxxxxx
np.arange()
can often make it a preferred choice.
Knowing the details and implications of these functions can hugely influence your approach in Python coding. Therefore, understanding when to use
xxxxxxxxxx
np.linspace()
over
xxxxxxxxxx
np.arange()
, and vice versa, is fundamental for efficient and optimal code.
References & Further Reading:
Numpy Linspace Documentation,
Numpy Arange Documentation.
Diving deep into our discussion of the differences,
xxxxxxxxxx
np.linspace
and
xxxxxxxxxx
np.arange
, these are two fundamental functions in the NumPy library, particularly beneficial for generating sequences of numbers. Their core difference resides in the parameters they accept and the way they generate these number sequences.
Starting with
xxxxxxxxxx
np.linspace
, this is a function that creates sequences of evenly spaced values within a specified range. The terminology “linspace” itself stands for ‘linear spacing’, hence providing equidistantly separated values. For instance,
xxxxxxxxxx
import numpy as np
print(np.linspace(0, 10, num=5))
This code returns:
xxxxxxxxxx
[0. 2.5 5. 7.5 10.]
Quite literally creating four equal segments between 0 to 10, thereby filling it with five evenly spaced points.
Contrarily,
xxxxxxxxxx
np.arange
operates differently. It takes a less direct approach to sequence generation, by accepting three arguments: starting point, end point, and step size. Instead of computing the exact number of steps to precisely fit within a defined interval, it iterates over the start-end interval with a defined step size. Here’s an example,
xxxxxxxxxx
import numpy as np
print(np.arange(0, 10, 3))
This block outputs:
xxxxxxxxxx
[0 3 6 9]
Here, notice how it starts at zero, incrementing by 3 until reaching a number less than 10.
These functions prove vital in creating sequences, especially while manipulating arrays or matrices in Python. What sets them apart, essentially, is their method of sequence generation – uniform step sizes (
xxxxxxxxxx
np.arange
) versus exact division of ranges into even segments (
xxxxxxxxxx
np.linspace
).
Mastering these nuances can elevate your skills in numerical computation, proving especially useful in data analysis, machine learning, or any field dealing with extensive numerical data.
For additional background on both functions, consult the official NumPy linspace documentation and NumPy arange documentation.