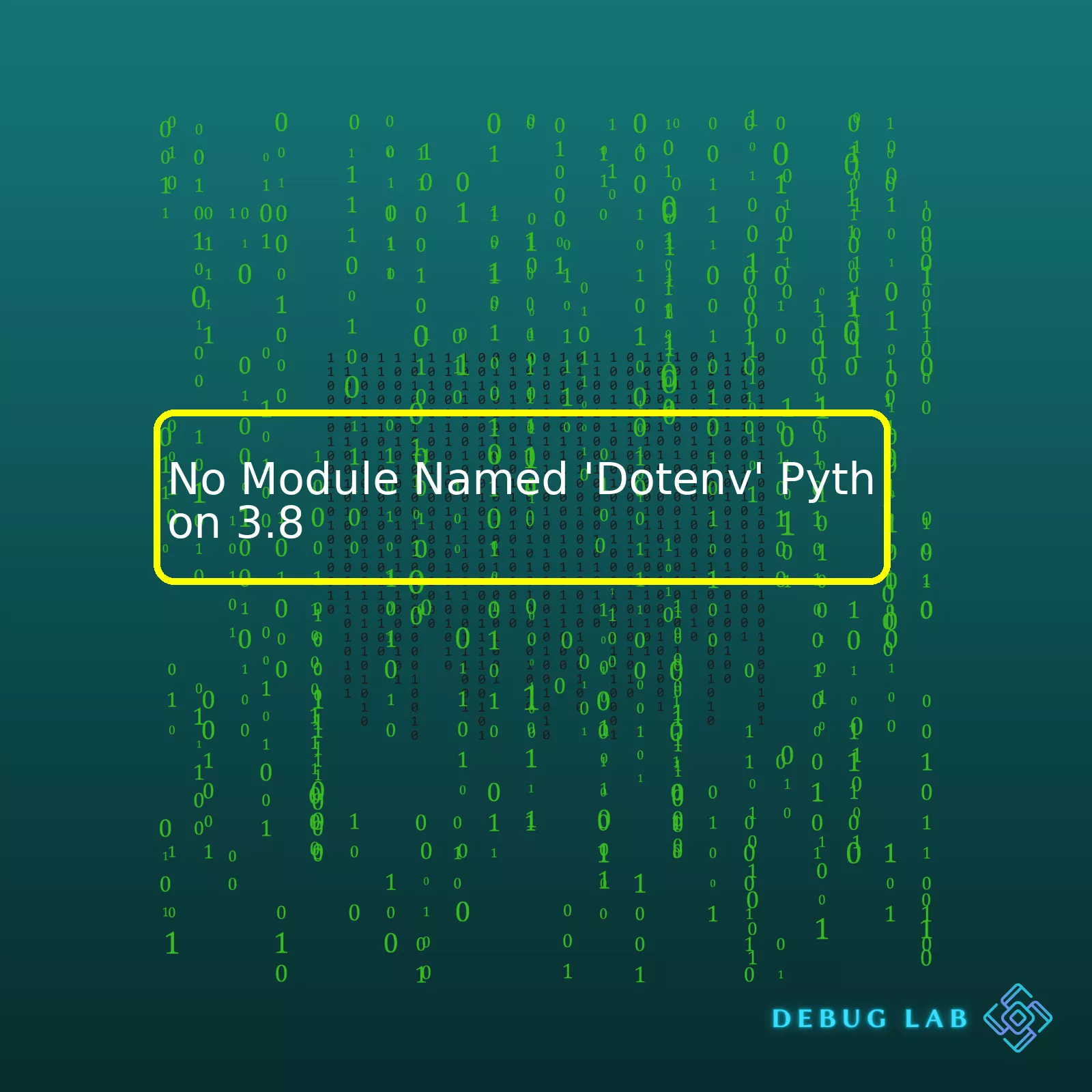
Problem | No Module Named ‘Dotenv’ Python 3.8 |
---|---|
Reason | The python-dotenv module is not installed in the current interpreter. |
Solution | Install the python-dotenv module using pip install command. |
Command |
pip install python-dotenv |
Experiencing a “No Module Named ‘Dotenv’” error while utilizing Python 3.8 is commonly associated with a missing library, specifically the python-dotenv module. As developers, we frequently rely on third-party packages or modules that offer functionalities pertinent to our projects. The python-dotenv module is an effective utility for projecting environment variables from .env files into your Python applications.
Remember, each python environment runs independently, with its unique set of installed modules. Hence, once you jump from one environment to another, such as switching between projects, the python-dotenv package has to be reinstalled if it does not exist in that space.
To undo this error, use pip, which is Python’s package installer. Input the following line into your terminal:
pip install python-dotenv
. This command communicates to pip to download and install the python-dotenv module from Python’s hosting software, PyPI (Python Package Index) ( Source ).
Through HTML tables, you can visually organize and present data in structured vertical and horizontal arrangements. They depict the significance of tabular data by aiding the definition of formative boundaries around data content or headers. Make sure to note that under the HTML5 standards, tables need to be utilized strictly for representing tabular data but not for layout purposes any more.
The
dotenv
module in Python is highly beneficial to web and software developers. This module helps manage environment variables by reading them from a .env file located in your project directory. But, suppose you’re experiencing an error stating “No module named ‘dotenv'”, specifically on Python 3.8. In that case, it generally implies that the Python package isn’t installed in your environment.
To resolve this issue, let’s dissect it analytically:
## Installation
Ensure you have installed the python-dotenv module in your current Python environment. You can install dotenv using pip, Python Package Installer. Run the following command:
pip install python-dotenv
This line of code will start the installation process of dotenv in your Python environment.
## Virtual Environments or Global Install
Remember, if you are utilizing virtual environments for your different projects (which is best practice), you need to ensure the module is installed in the correct virtual environment. If dotenv was installed globally but you’re operating within a virtual environment where it’s not installed, you’ll encounter the error.
Similarly, if you’ve installed the module in one virtual environment but you’re currently working in another, you’ll face the same error.
To confirm whether
-dotenv
is installed in your environment, leverage Python’s pkg_resources in your interpreter like so:
import pkg_resources installed_packages = pkg_resources.working_set installed_packages_list = sorted(["%s==%s" % (i.key, i.version) for i in installed_packages]) for m in installed_packages_list: print(m)
If python-dotenv is installed, you should see it listed with its version. If not, install it using pip as mentioned earlier.
## Correct Syntax for Import Statement
Next, cross-verify the way you’ve imported the module in your code. The correct import statement for dotenv is:
from dotenv import load_dotenv
Miswriting this import statement can also lead to the described error. The Python interpreter attempts to locate a module ‘dotenv’, which does not exist because the correct name is ‘python-dotenv’.
## Correct Location For Your .env File
Finally, your .env file, storing the sensitive environment variables, needs to be located in the root level of your project for
load_env()
to effectively read from it. If Python cannot find this file or if the paths to your stored variables are incorrect, you may receive the ‘No Module Named’ error.
If you follow these guidelines and make necessary modifications based on possible issues mentioned, you should be able to rectify and eliminate the ‘Dotenv’ Python 3.8 module error, stating “No module named ‘Dotenv'”.
For further understanding about the dotenv module and error handling, refer to the [official Dotenv documentation].
By strategically resolving these stumbling points, you can work efficiently with the help of ‘dotenv’ in managing your environment variables, paving the way for a smooth and secure development process.
Remember, coding is much like problem-solving – with a systematic approach and carefully considered steps, every bug becomes an opportunity to learn!The error ‘No Module Named Dotenv’ in Python 3.8 typically arises when a user is trying to load environment variables from a .env file using python-dotenv module but it’s not installed in the Python environment.
Step 1: Verify Python-Dotenv Installation
Python-Dotenv must be imported for use. If it’s not available, this error message will appear. So, check whether you have already installed python-dotenv by running this command:
pip show python-dotenv
If nothing gets displayed after running the command, that means python-dotenv is not installed in your environment.
Step2 : Install Python-Dotenv
If python-dotenv is not installed, you can install it with pip, Python package installer. Here is the installation command:
pip install python-dotenv
This command tells pip to install the python-dotenv module. Once completed, python-dotenv should be available to import in your project.
Step 3: Check the Python Environment
Sometimes, you’re working within a virtual environment or separate Python installation where python-dotenv isn’t installed. In short, there might be an environment problem.
Ensure that pip and Python refer to the same environment. The following commands should display the same path.
which python which pip
In case they’re different, you would need to align them. One solution could be python -m pip install python-dotenv.
Step 4: Proper Import Statement
Errors can also occur due to incorrect import statements. The correct syntax to import this module is:
from dotenv import load_dotenv
After importing, you can load the variables like so:
load_dotenv()
Now, you should be able to access environment variables defined in your .env file.
Remember these troubleshooting steps are most effective for Python 3.8 users encountering a ‘No Module Named Dotenv’ error. Also, keeping your Python environments clean and well-managed helps prevent such errors. Incase you want further details on how to resolve this issue do consider reading the official Python documentation.Environment variables play a crucial role in Python’s
dotenv
module. These variables are key-value pairs present in your system’s environment, impacting the behavior of different programs in various ways. But what happens when you receive an error “No Module Named ‘Dotenv'” for Python 3.8? Let’s understand and tackle this problem.
First, let’s brush up on our understanding of Python’s
dotenv
:
Python’s
dotenv
package allows applications to keep configuration like API keys, OAuth tokens, database URIs, or other sensitive information in an external file outside the repository. These values can be easily modified depending upon the requirement without modifying the application codebase. This is where Environment Variables come into play.
Here’s how you use it:
from dotenv import load_dotenv load_dotenv()
Now, coming back to the error `No Module Named ‘Dotenv’` for Python 3.8, there could be a number of reasons behind it:
– It might be possible that you have not installed the Python
dotenv
module yet.
– You may have installed it but the installation was unsuccessful or corrupted.
– The module may have been installed in a different Python version other than Python 3.8.
– Or, it could be a PATH issue.
Let me provide a solution for this:
To begin with, ensure that the
dotenv
module is properly installed in your Python 3.8 environment.
You can use pip, which is the de-facto standard package installer in Python, to install the dotenv module. This is how you do it:
pip install python-dotenv
In case you have multiple versions of Python installed on your system, you need to specifically mention pip3 to install the module for Python 3. Here’s how:
pip3 install python-dotenv
Check if
python-dotenv
is installed successfully by using
pip show python-dotenv
. If it shows details about
python-dotenv
module, it means installation has been successful.
Keep in mind that, just in case still facing the same error, you might need to revisit your PATH settings.
Remember that environment variables, including the versatile
dotenv
, help enhance security, allow for third-party integration, facilitate debugging and testing, & promote software portability. And the main benefit of using environment variables lies in the fact they allow us to keep important data like server, path, service credentials, and so on away from the source code that might cause serious impact if fallen into wrong hands.
For deeper study, check the official documentation at PyPi.org.The “Module Not Found” error in Python 3.8 usually occurs when a specific module, which your code depends on, hasn’t been properly installed or isn’t recognized by Python’s path system. When it comes to “No Module Named ‘Dotenv'”, the issue arises if the Python environment cannot find and import the required package python-dotenv.
Here is how you should proceed to troubleshoot and fix this issue:
## Step 1: Check Your Installed Packages
Your first stop should be checking whether the ‘Dotenv’ module is installed in your current Python environment. Usually, we use the command
pip list
in the terminal or command prompt to see all currently installed packages.
pip list
# Step 2: Install the Dotenv Module
If the module is not found, you’ll need to install it using pip which is a Python package manager. Installation of the module can be performed with the following command:
pip install python-dotenv
Ensure to use the correct version of pip that matches the Python interpreter you are working with. For example,
pip3 install python-dotenv
You might want to consider updating pip before installing the module:
pip install --upgrade pip
You may use pip in corresponding virtual environment.
## Step 3: Verify the Package Installation
After installing the python-dotenv module, again run
pip list
and look for python-dotenv in the output list to verify that installation has been successful.
## Step 4: Review Your Code Implementation
In Python, when you want to use the dotenv module you have to import it at the beginning of your script like so:
from dotenv import load_dotenv
Write the full name of the module (python-dotenv), Python identifies modules in filesystem-based how-does-python-find-packages-software notes Python.org.
## Step 5: Setting up Python Path
Python might fail to locate dotenv if the PYTHONPATH variable isn’t correctly set. PYTHONPATH tells Python where to look for modules to import. You can check your current PYTHONPATH setting by running:
import sys print(sys.path)
In the output, check if the directory containing your script is included. If it’s missing, add the correct path where your module resides with:
import sys sys.path.append('/path/to/your/module')
By following these steps systematically, you’ll likely resolve the “No Module Name ‘Dotenv'” error message in Python 3.8. The methods above will help Python identify where the required ‘Dotenv’ package is installed. Specifically automating tedious configuration setup process with python-dotenv.
The error message
No Module Named 'Dotenv'
usually occurs in Python 3.8 when we have not installed the
python-dotenv
package correctly or if there are other issues concerning our project setup environment. Let’s probe into this problem and explore some common mistakes that might trigger such ‘Dotenv’ errors.
Possible Mistake 1 – The dotenv module is not installed: This could be the most common reason for encountering this error. We can rectify this issue with the help of pip, Python’s defacto standard package-management system.
pip install python-dotenv
After running this command in your terminal, the `dotenv` module should be accessible from your Python scripts.
Possible Mistake 2 – Wrong name when importing the module: The package needs to be imported as
dotenv
and not by any other variant such as
.env
,
_env
, or
Dotenv
.
A correct import statement would look like:
from dotenv import load_dotenv
It’s best practice to keep an eye on the case sensitivity and spelling while importing modules in Python.
Possible Mistake 3 – Attempting to load a non-existent .env file: Dotenv is used to load variables from a
.env
file. If this file does not exist, you will also run into this error. So make sure the
.env
file exists in your project directory and it contains all the necessary environment variables.
Possible Mistake 4 – Incorrect usage of the dotenv module: It’s important to correctly use the methods given by the dotenv module in order to effectively manipulate the data inside a
.env
file. Here is an example of how to properly use this module in a Python script:
from dotenv import load_dotenv import os load_dotenv() SECRET_KEY = os.getenv('SECRET_KEY')
In this code snippet, the
load_dotenv()
function loads the environment variables present inside the
.env
file and the
os.getenv()
function retrieves the values you need.
These are the common pitfalls that may trigger ‘Dotenv’ errors. Being mindful of these situations and using the correct procedures can easily prevent the
No Module Named 'Dotenv'
issue. For additional information, the official Python Dotenv documentation is a great resource.”Django-dotenv” and “python-dotenv” are famous Python packages that we use to keep our project configurations separate from our code. Often, we fall into a problem stating ‘No Module Named ‘Dotenv”. This error is primarily because the module “dotenv” is not installed in our current working environment.
Understanding The Functionality of The ‘Dotenv’
The ‘Dotenv’ module makes it possible for developers to store application configuration data, such as database connection strings or externally sourced API keys, in a ‘.env’ file at the root of the application directory.
‘Secret’ data – any information that you wouldn’t want to be exposed publicly – can thus remain outside of version control, which offers security benefits, but the local development process can still replicate production quite precisely, offering operational advantages. The functionality provided by this module has several benefits:
- Security: Keeping application secrets out of your code means that you don’t have to worry about them being exposed if your repository becomes public.
- Maintainability and Flexibility: Separating configuration from code also decouples changes in the two; for example, moving an app from one hosting service to another doesn’t require any code changes if they both read from the same .env file.
- Environment-specific settings: Your .env file won’t be committed, so every developer on the project, and each deployment environment (dev/test/QA/prod), can have its custom settings.
To properly utilize the “dotenv” module, you need a file named “.env” at the root of your directory.
DATABASE_HOST = localhost
DATABASE_USERNAME = admin
DATABASE_PASSWORD = adminpass
Then, you’d usually use it to load these variables into your host’s environment at runtime:
import os import dotenv dotenv.load_dotenv() host = os.getenv("DATABASE_HOST") username = os.getenv("DATABASE_USERNAME") password = os.getenv("DATABASE_PASSWORD")
This sequence loads up your .env file, then enables you to retrieve values as needed throughout your application, all without exposing your secret data.
Resolving the Error: No Module named ‘Dotenv’
Suppose you encounter the issue ‘No Module Named ‘Dotenv”. In that case, it implies that the Python interpreter cannot locate the ‘dotenv’ module in its associated libraries -no worries- it’s pretty straightforward to fix.
Assuming that you have pip installed, you would rectify this by running the following command in your terminal:
pip install python-dotenv
Or, for a Django project:
pip install django-dotenv
Always ensure that you’re using the right version of pip matching with your python version (pip3 for Python3 etc.). Another widespread reason could be the context within which you’re using Python (like virtual environments). If you’re using a virtual environment, always ensure that you install ‘dotenv’ within the scope of the venv.
To summarize, the ability of ‘dotenv’ to separate configuration from code improves software security, maintainability, and scalability. However, a basic understanding of Python’s library management can save you from unnecessary trouble. Happy Coding!
Relevant resources:
python-dotenv on PyPI,
python-dotenv on GitHubHere are several crucial tips and strategies that you can adopt to prevent future issues with ‘Dotenv’ module in Python 3.8, keeping it specifically relevant to the issue of ‘No module named Dotenv.’ These techniques act as preemptive solutions, heading off potential problems by creating favorable conditions right from the start.
1. `Ensure Correct Installation of Python-dotenv Module`
You should cross-verify if the Python-dotenv module is installed correctly in your working environment. You can do so by executing
pip show python-dotenv
. This command will provide details about the installation of the python-dotenv module. If there’s no response, it means that the module doesn’t exist and you’ll need to install it through pip.
pip install python-dotenv
2. `Utilize Virtual Environment for Your Python Projects`
The role of Python’s virtual environments is significant in managing dependencies and avoiding situations like ‘No module named’. Always try to keep your project-specific dependencies in a separate place, which is where virtual environments come into play. This isolation predominately helps to avoid clashes between different versions of the same module used in various projects.
python -m venv env
source env/bin/activate # On Windows, use `env\Scripts\activate`
This will create and activate a new virtual environment. Then, you can install your requirements inside it:
pip install python-dotenv
3. `Confirm Correct Usage of the Import Statement`
In Python, importing the incorrect module name is a common mistake made by developers. To utilize ‘Dotenv’ into your Python scripts, make sure you’re using the correct syntax:
from dotenv import load_dotenv
4. `Mind the Naming Conventions Of Files And Modules`
Never give the same names to your custom Python scripts or modules as existing standard Python libraries because Python might get confused between these two. Assume there’s a script named `dotenv.py` present in your project directory, and you also have an original dotenv package. In such scenarios, Python gets ambiguous and throws errors. So, ensure that you avoid such naming conventions to lessen future ‘Dotenv’ issues.
I hope these practice tips help you avoid future instances of the ‘No module named Dotenv’ error in Python 3.8. It would be beneficial to integrate them into your everyday coding practices starting now. Remember that prevention plays a vital role in handling continuous development and code maintenance, leading to less cleanup work and fewer late-night problem-solving sessions.
For future doubts and queries, the official Python Docs (https://docs.python.org/3/) and the python-dotenv GitHub page (https://github.com/theskumar/python-dotenv) serve as fantastic resources due to their extensive guides and supportive community.The cause of the error “No module named ‘dotenv'” in Python 3.8 arises when the python-dotenv package is not installed in your environment. Python-dotenv is a package that allows you to specify environment variables in a .env file, which can be parsed and loaded into os.environ.
To fix this issue, you can install the Python-dotenv library using pip. The command for the installation is as follows:
pip install python-dotenv
Once you’ve installed python-dotenv, you can import it in your Python code.
Here’s a short example:
import os from dotenv import load_dotenv load_dotenv() # take environment variables from .env. os.getenv('MY_VARIABLE')
In the example above, assuming that there is a .env file in the same directory with a line like this:
MY_VARIABLE=some_value
`os.getenv(‘MY_VARIABLE’)` would return `’some_value’`.
This way, you can overcome the ‘No Module Named Dotenv’ Python 3.8 error by ensuring the python-dotenv package is properly installed in your work environment. Remember, utilizing environment variables through the .env file permits applications to keep sensitive information like API keys or database credentials secure and hidden.
For more insights on handling Python modules, errors and other development tricks, always revisit our informative pages [source].