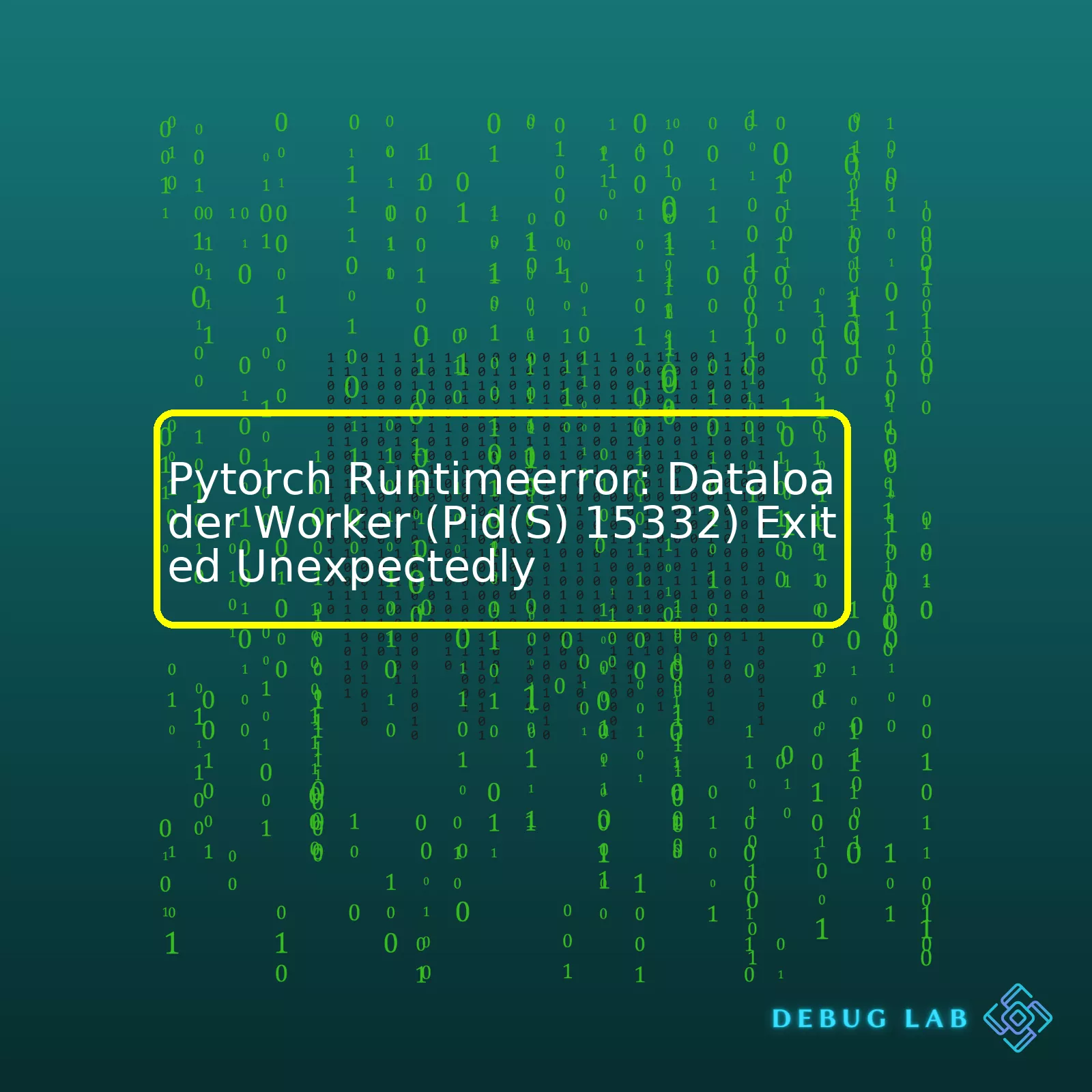
Let’s look at some common reasons for this error occurring and their respective fixes in HTML table format.
Reasons | Solutions |
---|---|
Data-related issues |
|
Shared Memory: | Increase shared memory or decrease number of threads. |
Termination signal: | Try setting num_workers=0 to do pre-loading on main process. |
There are cases where the issue stems from how your data is formatted, loaded or processed. For instance, if your dataset has some irregularities or inconsistencies – like missing files or inconsistent file formats, then cleaning or fixing your dataset might solve the issue. You might also encounter this error due to out of limits memory utilization, in which case reducing the batch size can be a solution.
Sometimes, limitations of resources such as shared memory might cause such problems. The DataLoader utilizes shared memory across different worker processes, and it might crash if it exceeds the shared memory limit. Increasing shared memory limit or decreasing the number of worker threads using the `num_workers` parameter in `DataLoader` can rectify the problem.
At other times, the worker is killed by a signal such as SIGKILL or others. One proposed fix to these cases is to disable the use of multiple worker processes and instead do the dataloading in the main process by setting `num_workers` to 0. Be cautious though, as this change could slow down data preparation and thus model training because the loaders now operate synchronously.
Here’s how to set `num_workers` in the `DataLoader` instance:
from torch.utils.data import DataLoader data = DataLoader(dataset, num_workers=0)
Please note that these solutions might not cover all causes of this error. Assess the specific circumstances, experiment with different approaches, and don’t hesitate to reach out to the community for help on platforms like [StackOverflow](https://stackoverflow.com/questions/tagged/pytorch) or [PyTorch Discussion Forum](https://discuss.pytorch.org/).
When using Pytorch’s data loader, you may encounter a runtime error that says “Dataloader worker (PIDs 15332) exited unexpectedly.” This is a common issue encountered in Pytorch, and it arises when the Dataloader’s workers die unexpectedly.
One reason for this problem could be that the Dataloader spawns multiple subprocesses to load data simultaneously. If any of these processes are interrupted or unable to complete their work, they cause an immediate termination and send a signal back to the main process, causing this error.
Scenarios Causing This Error
- Memory Issues: When your system does not have enough memory to handle the multiprocessing tasks desired, it could lead to such issues. This happens because as dataloader creates numerous worker threads, each thread can consume a substantial amount of memory.
- The coding environment: In certain cases, Jupyter notebooks might interact with multiprocessing in PyTorch, particularly leading to this error.
- Shuffling Data: If you’re using
num_workers>0
and shuffling data concurrently, there may be conflicts among the worker processes regarding data access.
Solutions to Prevent This Error
-
Adjust Number of Workers: Sometimes, reducing the number of workers for the DataLoader can solve the problem. If
num_workers
equals 0, it means data will be loaded in the main process.
loader = torch.utils.data.DataLoader(dataset, num_workers=0)
-
Disabling SHM: Another solution is to disable shared-memory. PyTorch utilizes shared memory to share data between processes. Setting
torch.multiprocessing.set_sharing_strategy('file_system')
before declaring the DataLoader disables use of shared memory.
torch.multiprocessing.set_sharing_strategy('file_system') loader = torch.utils.data.DataLoader(dataset)
-
Using pin_memory: By setting
pin_memory=True
, the data loader loads the Tensors in CUDA pinned memory, allowing faster data transfer compared to default pageable memory.
loader = torch.utils.data.DataLoader(dataset, pin_memory=True)
In summary, the main strategies for dealing with this error are troubleshooting your code or computing environment, reducing the number of worker processes, memory management, and considering how your data is being shuffled and loaded. Remember, the workaround would heavily depend on your specific situation and dataset. It might be necessary to try various combinations or methods to see what works best for your scenario.
These explanations, instructions, and possible solutions should help you understand and deal with the DataLoader worker’s unexpected exit issues better when working with Pytorch. More details about handling large-scale data in Pytorch and error fixing advice can be found at the official Pytorch website: https://pytorch.org/.
The Python programming language is globally recognized for its multi-paradigm operational nature that includes procedural, functional, and object-oriented styles. One of its standout features has to be the routines that create and control processes using the “os.fork()” technique.
In the context of PyTorch, a popular open-source library used for diverse computations like tensor manipulation and creating deep neural networks, understanding PIDs (process IDs) take on a new level. The evolution of machine learning libraries is associated with enhanced coping mechanisms for operating system constraints and seamless process creation and interruption, but nevertheless, errors like
PyTorch RuntimeError: DataLoader worker (PID(s) 15332) exited unexpectedly
are quite common.
A PID, or process ID, is a unique identifier assigned by the operating system to each process. Whenever we run error messages like ‘DataLoader worker (PID 15332) exited unexpectedly’, it relates to the application’s subprocess, represented by PID 15332 in the error message. This recursion usually indicates an issue springing from either a broken pipe or memory-related problems caused when workers (parallelly working parts of your code designed to speed up the input data feeding process) fail to allocate the required memory.
How can you resolve this? Here are some actionable change propositions:
– Amplify Shared Memory Availability: In applications involving significant parallel processing, shared memory often falls short, hence enhancing it could potentially resolve the problem. To increase shared memory at runtime, use the following command
docker run --shm-size 1G image-name /bin/bash
– Limit the Number of Worker Processes: Spreading the load overa fewer number of worker processes minimizes resource exhaustion risks. Rework the model structure to not overload the DataLoader. If multiple GPUs are available, Data Parallelism is a usable option.
torch.nn.DataParallel
– Reduce Batch Size: A large batch size also can lead to insufficiency of shared memory. Reducing it not only lessens the memory requirement but also refrains from compromising too much on model performance.
trainloader = torch.utils.data.DataLoader(trainset, batch_size=5, shuffle=True)
– Apply Garbage Collection: Regularly call Python’s garbage collector during execution to clear up space.
import gc gc.collect()
Remember, PyTorch extensively uses shared memory for DataLoader duties to boost memory efficiency. But if the DataLoader exceeds the default limit, it would trigger
RuntimeError: DataLoader worker (PID(s)) exited unexpectedly
. The proposed solutions aim to narrow down this discrepancy and make your learning models more efficient. For more details, you can always refer to the official PyTorch documentation. They provide extensive guides and tutorials for managing and diagnosing common errors in PyTorch. Additionally, consistently revisiting coding strategies and staying abreast with discussions in PyTorch’s forum, can supplement your understanding as user issues tackled in these forms provide insightful resolvable techniques.The “RuntimeError: DataLoader worker (pid(s) 15332) exited unexpectedly” error in Pytorch might be linked to a few factors. These may include inadequate memory space, incompatible system configurations, or complications from using shared file systems, especially in distributed environments.
Let’s take an analytical view of these potential causes and how you can solve them:
- Memory Issues:
- Incompatible System Configurations:
- Shared File System Issues:
Pytorch places data into Shared Memory using `multiprocessing.Queue`. When the Shared Memory is full and cannot house any more items, it could result in the ‘Dataloader worker has exited unexpectedly’ error.
A potential fix is to lower the value of `num_workers` when initializing the data loader:
data_loader = Data.DataLoader(dataset, batch_size=BATCH_SIZE, shuffle=True, num_workers=2)
Here, have set `num_workers` to 2 which reduces the number of child worker processes that are used. Lowering this value also decreases the amount of shared memory utilized by the loader.
The exception can also be generated due to incompatible system configurations. For example, the DataLoader utilizes multi-threading, where each thread could be executed on different cores of the processor. If the hardware isn’t compatible with such configurations, then it might lead to process failure.
Therefore, setting the `num_workers` attribute to 0 might help in this case, forcing the data loading to be done in the main process:
data_loader = Data.DataLoader(dataset, batch_size=BATCH_SIZE, shuffle=True, num_workers=0)
In distributed environments, DataLoader might malfunction or exit unexpectedly due to issues associated with shared file systems like Network File System(NFS). This is because many Network File Systems do not support file handling with the ‘mmap’ mode, which is used to open files by torch.utils.data.DataLoader.
To resolve this, avoid using mmap by setting `DataLoader`’s argument `pin_memory=False`. By default, `pin_memory=True` and the loaded data is placed into CUDA pinned memory.
data_loader = Data.DataLoader(dataset, batch_size=BATCH_SIZE, shuffle=True,num_workers=2, pin_memory=False)
Please note, disabling `pin_memory` could result in a slight drop in training efficiency since the data transfer from host to GPU device will not be asynchronous anymore.
Remember to perform proper debugging to find the cause of the issue before implementing a solution. In most cases though, modifying attributes like ‘num_workers’ or ‘pin_memory’ in the DataLoader class would work fine. (source)Let’s start off with a deeper understanding of the error message, “
RuntimeError: DataLoader worker (pid(s) 15332) exited unexpectedly
“. The meaning is pretty straightforward – one or more of your Dataloader workers (the processes which handle loading data into your model during training) have unexpectedly terminated.
This kind of PyTorch RuntimeError can indeed be quite frustrating. Fortunately, there are several strategies we could employ to mitigate this issue:
– Error Tracking: In order to determine the most effective workaround for your specific problem, it’s vital to understand what exactly causes these workers to exit unexpectedly. Using Python’s built-in traceback utility can help provide crucial details about your runtime error.
To do this, wrap your main code with a try-except block and print out the traceback if an exception occurs:
import traceback try: # Your code here... except Exception as e: print("Traceback below:") traceback.print_exc() print("Exception message:") print(str(e))
– Adjusting the Number of Workers: The number of DataLoader workers is set by the ‘num_workers’ parameter within DataLoader. If you’ve added too many workers for your system to handle, it can lead to overload and cause them to crash.
You might consider reducing the number of workers. While this will slow down your data loading speed, it can potentially prevent unexpected crashes:
torch.utils.data.DataLoader(dataset, num_workers=2)
– Handling Multiprocessing Issue: Another cause can be the interplay between DataLoader and multiprocessing on some systems. This can be particularly prevalent if your dataset involves reading large files from disk, something that isn’t uncommon in machine learning tasks involving images or videos.
One way to solve this issue is to use the ‘
worker_init_fn
‘ argument in DataLoader. This forces each worker to use a different seed for initialising random number generation, thereby preventing potential conflicts:
def seed_worker(worker_id): worker_seed = torch.initial_seed() % 2**32 numpy.random.seed(worker_seed) random.seed(worker_seed) torch.utils.data.DataLoader(dataset, num_workers=4, worker_init_fn=seed_worker)
Remember to test each of these strategies one by one, and track whether they’re having the desired effect of fixing the RuntimeError in question. Debugging can often involve plenty of trial and error, but perseverance and a systematic approach usually pays off in the end!
Also note that it is always helpful to stay updated with the latest version of PyTorch. A runtime error you are experiencing might be due to a bug in PyTorch itself, which could already be resolved in a newer version.
Lastly, PyTorch has a fairly responsive discussion board where you can post your issues and seek help from other members of the community who may have faced similar problems. It’s always good to turn to such resources when struggling with complicated issues like runtime errors.
When dealing with PyTorch and encountering the RuntimeError: DataLoader worker (pid(s) 15332) unexpected exit, it’s important to understand the root cause of this issue. This particular error usually comes as a result of trying to load data in parallel using
DataLoader
in PyTorch. The error is more likely to occur while running PyTorch on Windows as its process spawning mechanism differs from Unix.
Several factors can contribute to this error:
– Inefficient memory management
– Faulty hardware
– Unstable software
To effectively resolve these issues, here are several solutions that have proven effective:
Reducing the number of workers in the DataLoader
One simple solution to this problem could be reducing the number of workers in your DataLoader if it is currently set to a high number. You may be creating more subprocesses than your system can handle leading to an overutilization of resources.
DataLoader(dataset, num_workers=1, **kwargs)
Setting the “worker_init_fn” to a random seed
The use of shared memory objects like cryptographic random number generators could cause the DataLoader issue. Resetting the random seed for each worker in “worker_init_fn” could efficiently solve this problem.
Set your random seed using numpy or any other compatible library in Python’s standard library.
def worker_init_fn(worker_id): np.random.seed(np.random.get_state()[1][0] + worker_id) DataLoader(dataset, num_workers=num_of_workers, worker_init_fn=worker_init_fn, **kwargs)
Setting “spawn” as the start method
Issues might arise when using “fork” as the start method. If you’re working in a Unix-like environment, setting the global start method to “spawn” before creating the DataLoaders can make a difference. However, this only applies to Unix systems as Windows doesn’t support the “fork” method.
Remember to globally set the start method to “spawn” in your main guard.
if __name__=='__main__': multiprocessing.set_start_method('spawn') ...
Keep in mind that there’ll be increased memory usage since all the model parameters need to be copied to each subprocess.
Reinstalling PyTorch
There could be instances where the installation of PyTorch becomes problematic. A corrupt or partial installation of PyTorch can give rise to this error. In such a case, reinstalling PyTorch might help:
pip uninstall torch torchvision pip install torch torchvision
These are some specific solutions that might address the DataLoader worker (pid(s) 15332) exit unexpectedly runtime error. It is advisable to thoroughly diagnose your code, dataset, and system specifications, such as available RAM and GPU before implementing these solutions. You should reference the official PyTorch documentation to figure out what best suits your environment and application requirements. Remember, creating an efficient machine learning environment requires a delicate balance between computational resources and coding efficiency.The
RuntimeError: DataLoader worker (pid(s) 15332) exited unexpectedly
is an issue you can often experience when training your deep learning models using PyTorch. This error typically arises due to issues with the multi-threading of PyTorch’s DataLoader and could result from any of:
+ Non-deterministic transforms
+ Standard input/output operations within the dataset items
+ Sharing CUDA tensors between processes
Here are some ways you can approach resolving these issues:
Using a Single Worker
You can avoid problems related to sharing data between different threads completely by setting num_workers as zero in your DataLoader, thereby forcing it to work synchronously:
data_loader = DataLoader(your_dataset, num_workers=0)
However, this may slow down the data loading and thus affect the training speed. This method only advisable for debugging purposes or very small tasks.
Ensure Determinism in Transforms
Ensure all custom transformations done on your dataset are deterministic. Randomness in transformations on the dataset when processed in multiple threads can lead to synchronization problems and subsequently crash the workers. For instance, if you’re using random scaling using torchvision.transforms, make sure to set a constant seed before calling them.
Remove Standard Input/Output Operations from Dataset Items
Avoid performing any print or file read/write operations within your dataset code, as concurrent standard input/output operations can cause conflicts between workers.
For example, instead of including print statements like:
class MyDataset(Dataset): def __getitem__(self, index): item = load_data(index) print("Loaded data", index) return item
It’s better practice to handle logging and exceptions outside the worker script.
Move Tensors to Shared Memory
If you’re sharing CUDA tensors between processes by returning them in your
__getitem__
method, they must be moved to shared memory:
def collate_fn(batch): return {key: torch.stack([d[key] for d in batch]).to('cuda') for key in batch[0]} data_loader = DataLoader(dataset, collate_fn=collate_fn)
Using workers_init_fn
PyTorch allows you to specify a function to initialize your dataloader workers through the workers_init_fn argument:
import os def worker_init_fn(worker_id): np.random.seed(np.random.get_state()[1][0] + worker_id) torch.utils.data.DataLoader(dataset, worker_init_fn=worker_init_fn)
For more information about the DataLoader worker error and Python multi-threading, you can refer to the official Pytorch documentation.
Remember, always use good coding practices such as handling exceptions correctly, not using global variables etc., to reduce the chance of runtime errors. Understanding the underlying parallel computing principles will also give you valuable insight into how to tackle such errors.The PyTorch error message:
RuntimeError: DataLoader worker (pid(s) 15332) exited unexpectedly
is not an uncommon one for professionals using the PyTorch Machine Learning library. This error can occur due to a variety of reasons, and understanding these root causes is essential in helping you debug and solve this problem efficiently.
One primary cause of this error message is related to memory issues. When PyTorch runs out of memory, it may forcefully terminate the worker processes involved in data loading. The termination of these workers then immediately triggers this error message. Code that demands a lot of memory, such as intensive computation scripts or large dataset handling, can push your system to its limits and trigger this RuntimeError.
Another possible cause involves issues relating to multi-threading. If the DataLoader uses multiple worker threads to load data and any one of these threads crash, this error message will be prompted. Crashes are often caused by pushing the limits of system resources or flawed programming logic which results in fatal exceptions during runtime.
Here’s an example on how the “Dataloader” could be used:
from torch.utils.data import DataLoader, Dataset import big_dataset class BigDataset(Dataset): def __init__(self, big_data): self.big_data = big_data def __len__(self): return len(self.big_data) def __getitem__(self, idx): return self.big_data[idx] big_data_instance = big_dataset.load_data() dataset_instance = BigDataset(big_data_instance) dataloader = DataLoader(dataset_instance, num_workers=4)
Solving the problem generally involves two steps:
– Determining if it is a memory issue: Methods to tackle this include reducing batch sizes, simplifying models, or increasing your available system memory.
– Ascertaining if it’s a multi-threading problem: You can try reducing the number of worker processes or debugging the part of code to fix existent bugs.
Often enough, running out of memory on the GPU is the real culprit behind this error. Tools like torch.cuda.memory_allocated() allow you to command line check your PyTorch GPU memory usage.
Dealing with subtler issues, such as algorithm problems or bugs in third-party libraries, becomes easier when you’ve ruled out the common causes above. Proactively checking and managing memory usage, ensuring robust multi-threading practices, and employing diligent debugging can prevent the occurrence of this RuntimeError.
The PyTorch RuntimeError: Dataloader Worker (Pid(s) 15332) Exited Unexpectedly often arises because the DataLoader worker process ends prematurely. This problem predominantly crops up in situations involving multi-processing scenarios. Remember that Pytorch DataLoaders leverage multi-threading to load batches of data simultaneously, amplifying efficiency. Nevertheless, this proess isn’t without its complications.
from torch.utils.data import DataLoader loader = DataLoader(dataset, batch_size=8, num_workers=4)
In this code snippet above, we set num_workers to a value greater than zero. We’re employing multiple sub-processes for data loading, which can cause the error. The most common reasons behind this error are two-fold:
- Somewhere within your custom dataset’s code, you’re trying to use a functionality not supported by multi-threaded/multi-process operations. An example might be an attempt to read from a file or database simultaneously from different threads.
- Your system doesn’t have sufficient resources to handle numerous parallel tasks. When your system runs out of memory, worker processes get terminated and consequently end abruptly.
How to solve it? Solutions typically fall into one of three categories:
- Adjust DataLoader parameters:
You might want to run a tune-up on the number of workers. Normally, there isn’t a hard-fast rule to follow. You may decrease the workload per worker by raising the num_workers count, but don’t unknowingly overburden your system’s RAM. Running out of memory is recipe for catastrophe — more processes then equals less available memory per worker.
loader = DataLoader(dataset, batch_size=8, num_workers=1)
- Examining your custom Dataset code:
Inspect the getItem function within your custom Dataset class. If you’re using any non-thread-safe operations or shared resources across various worker processes, segregate them.
class CustomDataset(Dataset): def __getitem__(self, index): # Check any non-threadsafe operations here
- Use DataLoader’s worker_init_fn:
Inside your DataLoader, utilize the worker_init_fn, a notable function invoked upon each worker process’s init phase. Here, you could code actions that isolate resources for every worker process.
def worker_init_fn(worker_id): np.random.seed(np.random.get_state()[1][0] + worker_id) DataLoader(dataset, batch_size=batch_size, num_workers=num_workers, worker_init_fn=worker_init_fn)
Running your deep learning models smoothly without hiccups requires careful threading management when it comes to data loading. Thankfully, PyTorch provides us with the necessary tools to attain this feat.
Please note to use solutions relevant and applicable to your deployment scenario. It’s crucial to strike a balance among performance, complexity, and memory usage in handling PyTorch RuntimeErrors related to DataLoader workers. You can dive deeper into understanding these errors and solutions with PyTorch’s official documentation or consult their lively community forums for specific cases. To examine real world applications, comprehend source examples at the GitHub PyTorch projects repository.