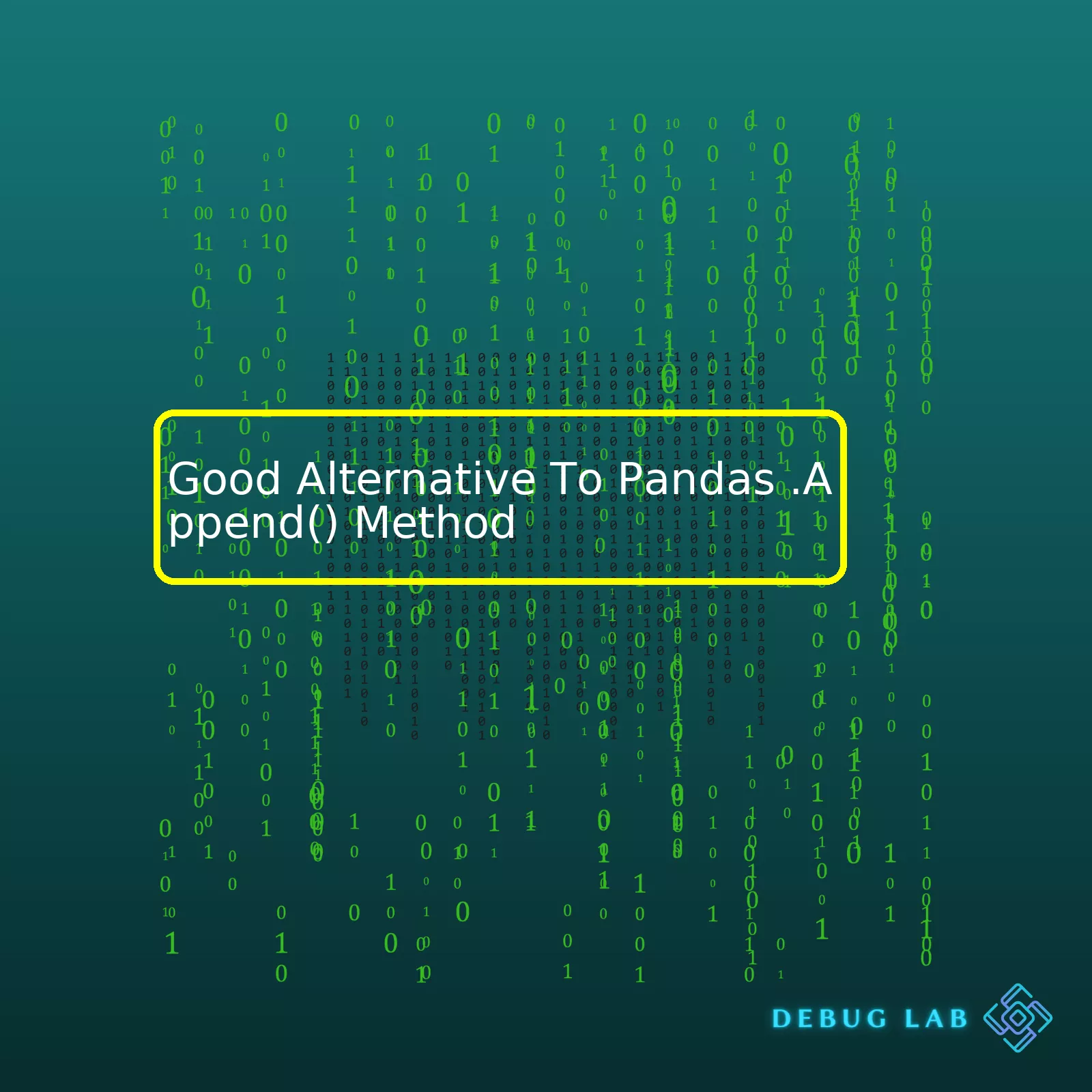
Pandas is a robust and widely used data analysis library in Python. The
.append()
method from this library is commonly used to add rows at the end of the DataFrame. However, it may not always be the most efficient solution due to its slow processing speed especially when you’re dealing with large datasets. A faster alternative is using
xxxxxxxxxx
pd.concat()
or list comprehension. These solutions are capable of performing faster concatenation which enhances code efficiency.
Method | Description |
---|---|
xxxxxxxxxx 1 1 1 pandas.DataFrame.append() |
This function appends rows of another DataFrame to the end of the given DataFrame, returning a new object. Columns not present in the original DataFrame are added as new columns. |
xxxxxxxxxx 1 1 1 pandas.concat() |
Concatenation is much more efficient than other methods like append. It combines pandas objects along a particular axis quickly and can also handle duplicate indices. |
List Comprehension | An extremely fast approach that harnesses the speed of underlying C/Fortran code of Python’s raw libraries. It involves creating a list of dictionaries and converting it directly to DataFrame. |
The usage of
xxxxxxxxxx
pandas.concat()
over
xxxxxxxxxx
pandas.DataFrame.append()
brings a significant speed advantage while handling large datasets. For instance, if you are intending to concatenate multiple DataFrames together,
xxxxxxxxxx
pandas.concat()
allows you to do this in one go, unlike
xxxxxxxxxx
DataFrame.append()
which sequentially adds each DataFrame. This reduces overhead and considerably improves performance.
On the other hand, the method involving list comprehension pushes efficiency even further. Instead of appending a row one by one using loops, you can write a loop inside a list and form a list of dictionaries, where each dictionary represents a row of data. Afterwards, convert the complete list into a DataFrame in one stroke. This reduces the time-complexity from quadric (O(n²)) in case of
xxxxxxxxxx
.append()
to linear (O(n)) in this method, making it exceptionally faster for larger datasets.
Source Code Example:
Suppose we have two DataFrames as shown below.
xxxxxxxxxx
# Import pandas library
import pandas as pd
# Generate sample data
df1 = pd.DataFrame({'A': ['A0', 'A1'],
'B': ['B0', 'B1']})
df2 = pd.DataFrame({'A': ['A2', 'A3'],
'B': ['B2', 'B3']})
If you had to use the append method, this is how you’d proceed.
xxxxxxxxxx
df_append = df1.append(df2)
print(df_append)
In order to enhance your coding efficiency through the concat method, your process would look like this.
xxxxxxxxxx
df_concat = pd.concat([df1, df2])
print(df_concat)
Lastly, to utilize the fastest approach via list comprehension, follow this procedure.
xxxxxxxxxx
data = pd.DataFrame([{'A': x, 'B': y} for x, y in zip(list('AABB'), list('1122'))])
print(data)
The above examples distinctly illustrate these methods’ implementation and how similar their outcomes are despite the varying levels of complexity and speed. Each method has its perks, but remember: understanding your requirements and dataset size plays an important role in choosing the right approach.
You can further read about these alternatives in the official Pandas documentation.
Pandas `.append()` method is often a lifesaver when it comes to appending rows in a DataFrame. However, it’s not always the most efficient way, especially when dealing with large data sets. For this, I’m going to explore some alternatives that you might find useful.
The Concatenation Method
Pandas `concat()` function can be used as an alternative to the `.append()` method. In my experience, `concat()` performs better than `.append()` when dealing with a larger data set. It is important to note though, that the index will be duplicated if not reset after using the `concat()` function.
Take a look at the following usage:
xxxxxxxxxx
import pandas as pd
df1 = pd.DataFrame({'A': ['A0', 'A1'], 'B': ['B0', 'B1']})
df2 = pd.DataFrame({'A': ['A2', 'A3'], 'B': ['B2', 'B3']})
df_concat = pd.concat([df1, df2], ignore_index=True)
There are options like `ignore_index` that prevent index duplication, which makes your result more clean and organized.
List of Dictionaries Method
Building a list of dictionaries first and then converting the list into a DataFrame is another approach if performance is concerned.
Here’s an example on how it works:
xxxxxxxxxx
list_of_dict = [{'A': 'A0', 'B': 'B0'}, {'A': 'A1', 'B': 'B1'},
{'A': 'A2', 'B': 'B2'}, {'A': 'A3', 'B': 'B3'}]
df_from_dict = pd.DataFrame(list_of_dict)
This method saves time by eliminating the need for continuously reallocating and copying data from the memory.
Using Dictionary of Series Method
Lastly, a dictionary of series can be used as an option. This method treats the keys of the dictionary as column headers and the values of the dictionary as Series, which become the actual values in the dataframe.
Look at the following code snippet for a clearer understanding:
xxxxxxxxxx
dict_of_series = {'A': pd.Series(['A0', 'A1', 'A2', 'A3']),
'B': pd.Series(['B0', 'B1', 'B2', 'B3'])}
df_from_series = pd.DataFrame(dict_of_series)
Which method suits you best depends on your situation, but these alternatives to `.append()` provide some solid options. Do check the Pandas Documentation to learn more about each method and its benefits.
Choosing the right tool largely depends on the specifics of the problem at hand – the size of the data, the complexity of the operation, and the particular requirements of your project.When you’re dealing with data frames in pandas, there are many ways to combine them. One common approach is using the
xxxxxxxxxx
.append()
method. However, while
xxxxxxxxxx
.append()
may be intuitive and straightforward to use when concatenating or appending rows of a DataFrame, it may not always be the most efficient solution, especially if you’re combining large datasets.
An excellent alternative I’d recommend is the
xxxxxxxxxx
pd.concat()
function in pandas.
Compared to
xxxxxxxxxx
.append()
,
xxxxxxxxxx
pd.concat()
is more powerful because:
- It can concatenate along either axis (rows or columns).
- It provides various options for handling indexes.
However, what truly sets
xxxxxxxxxx
pd.concat()
apart in terms of efficiency is its speed. Purely from a computational perspective, using
xxxxxxxxxx
pd.concat()
to combine multiple DataFrames tends to be faster than repeatedly calling
xxxxxxxxxx
.append()
.
Let’s illustrate this with an example where we are trying to append two dataframes together:
Using append:
xxxxxxxxxx
df_append = df1.append(df2)
Using concat:
xxxxxxxxxx
df_concat = pd.concat([df1, df2])
Running these commands on large datasets, you would quickly notice that
xxxxxxxxxx
pd.concat()
runs significantly faster. The efficiency of
xxxxxxxxxx
pd.concat()
stems from the fact that it avoids creating a new index and data buffer for each addition operation. Instead, it performs a single concatenation operation on all dataframes at once, leading to a significant speed boost.
Therefore, as a professional coder dealing with large datasets, switching to
xxxxxxxxxx
pd.concat()
can help improve your code performance and processing times. However, keep in mind that
xxxxxxxxxx
pd.concat()
requires all the dataframes to be concatenated simultaneously in a list, so it might not work in scenarios where dataframes become available sequentially or are too big to be held in memory simultaneously.
Also, make sure you ensure the correct alignment of indices and handle null or missing values appropriately, as this varies between the
xxxxxxxxxx
.append()
and
xxxxxxxxxx
pd.concat()
methods. For instance,
xxxxxxxxxx
.append()
and
xxxxxxxxxx
pd.concat()
align their inputs differently:
xxxxxxxxxx
.append()
resets the index on the result dataframe [(*source*)](https://pandas.pydata.org/pandas-docs/stable/reference/api/pandas.DataFrame.append.html), while
xxxxxxxxxx
pd.concat()
preserves the original indices by default [(*source*)](https://pandas.pydata.org/pandas-docs/dev/reference/api/pandas.concat.html).Understanding Join and Merge as Good Alternatives to Pandas .Append() Method
In terms of the context of data optimization, considering efficiency at both memory and computation time is vital. Although
xxxxxxxxxx
Pandas .append()
serves as a straightforward method for combining Series or DataFrame objects, it’s not necessarily the most efficient, cost-effective solution when it comes to memory usage and computational duration.
Instead, the following alternatives may be better options: the
xxxxxxxxxx
Pandas .merge()
, and
xxxxxxxxxx
Pandas .join()
. Let’s inspect these alternatives.
Pandas .merge() as an alternative
The
xxxxxxxxxx
Pandas .merge()
function provides a substantial remedy to append. It consolidates DataFrame objects by conducting database-style merge operations. You can use this under different scenarios, such as connecting two data frames based on one (or more) common key(s).
A typical example:
xxxxxxxxxx
# Example DataFrame
df1 = pd.DataFrame({'key': ['A', 'B', 'C', 'D'],
'value': np.random.randn(4)})
df2 = pd.DataFrame({'key': ['B', 'D', 'D', 'E'],
'value': np.random.randn(4)})
# Merging df1 and df2
merged = pd.merge(df1, df2, on='key')
print(merged)
This will result in an output that merges based on the common key:
xxxxxxxxxx
key value_x value_y
0 B 1.23 -0.56
1 D -0.99 1.25
2 D -0.99 -1.50
Pandas .join() as an alternative
Another noteworthy alternative is the
xxxxxxxxxx
Pandas .join()
function. This method is excellent for combining DataFrames along either columns or indices.
An example would look like this:
xxxxxxxxxx
# Example DataFrame
df3 = pd.DataFrame({'A': ['A0', 'A1', 'A2'],
'B': ['B0', 'B1', 'B2']},
index=['K0', 'K1', 'K2'])
df4 = pd.DataFrame({'C': ['C0', 'C1', 'C2'],
'D': ['D0', 'D1', 'D2']},
index=['K0', 'K2', 'K3'])
# Joining df3 and df4
joined = df3.join(df4)
print(joined)
The output provides a column-wise combined DataFrame:
xxxxxxxxxx
A B C D
K0 A0 B0 C0 D0
K1 A1 B1 NaN NaN
K2 A2 B2 C1 D1
These methods –
xxxxxxxxxx
.merge()
and
xxxxxxxxxx
.join()
– generally outperform
xxxxxxxxxx
.append()
since they are both designed to combine data across either columns or rows in a highly optimized way, similar to how SQL handles JOIN operations. However, when choosing between these functions and the simple
xxxxxxxxxx
.append()
operation, it mostly boils down to the nature of your dataset and what sort of merging or joining you require.
For additional details on
xxxxxxxxxx
.merge()
and
xxxxxxxxxx
.join()
, refer to the official pandas documentation.
xxxxxxxxxx
pandas
library in Python for data analysis, chances are that you’ve repeatedly used the
xxxxxxxxxx
.append()
method to combine datasets. It’s intuitive and seemingly straightforward. However, the
xxxxxxxxxx
pd.concat()
function is often found to be a more efficient and powerful substitute for combining datasets than
xxxxxxxxxx
.append()
.
xxxxxxxxxx
pd.concat()
is a function in pandas that concatenates two or more pandas objects along a particular axis with optional set logic along the other axes. Its scalability, flexibility, and better performance make it ideal when dealing with large datasets.
Performance:
xxxxxxxxxx
pd.concat()
outperforms
xxxxxxxxxx
.append()
, especially while dealing with large databases. With every use of the
xxxxxxxxxx
.append()
method, a new object has to be created, which slows down the process considerably. On the contrary,
xxxxxxxxxx
pd.concat()
completes the task far more quickly and efficiently. Hence, it’s better in terms of speed and performance.
Scalability:
The append function only allows you to concatenate along the row axis (
xxxxxxxxxx
axis=0
). With
xxxxxxxxxx
pd.concat()
, you have the option to concatenate along columns too (
xxxxxxxxxx
axis=1
), offering greater scalability.
How to use pd.concat():
You simply pass a list of DataFrame objects to
xxxxxxxxxx
pd.concat()
:
xxxxxxxxxx
import pandas as pd
# creating two data frames
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2', 'A3'],
'B': ['B0', 'B1', 'B2', 'B3'],
'C': ['C0', 'C1', 'C2', 'C3'],
'D': ['D0', 'D1', 'D2', 'D3']},
index=[0, 1, 2, 3])
df2 = pd.DataFrame({'A': ['A4', 'A5', 'A6', 'A7'],
'B': ['B4', 'B5', 'B6', 'B7'],
'C': ['C4', 'C5', 'C6', 'C7'],
'D': ['D4', 'D5', 'D6', 'D7']},
index=[4, 5, 6, 7])
#concatenating
pdf = pd.concat([df1, df2])
print(pdf)
The output DataFrame `pdf` would now contain rows from both `df1` and `df2`. We can concat along the column axis (axis = 1) by:
xxxxxxxxxx
pdf_columns = pd.concat([df1, df2], axis=1)
print(pdf_columns)
In conclusion, when considering flexibility, performance, and scalability,
xxxxxxxxxx
pd.concat()
is an ideal substitute to pandas’
xxxxxxxxxx
.append()
method for data concatenation. Therefore, I’d go as far as suggesting making it your first choice when looking to combine datasets whilst coding with pandas.
References:
1. Pandas documentation: pd.concat()
2. Performance comparison: How to make your pandas loop 71,803 times faster?
3. Examples: Pandas: Merge, Join, and Concatenate Certainly! When dealing with large datasets and needing to concatenate, the
xxxxxxxxxx
pandas.DataFrame.append()
method can be time-consuming and inefficient. Instead, a powerful alternative is adopting Python’s native list append process combined with transforming the final list into a DataFrame at the end.
Let’s delve deeper into this approach and compare it with Panda’s
xxxxxxxxxx
.append()
operation.
Pandas DataFrame.append():
Firstly, let me share an example of pandas
xxxxxxxxxx
.append()
in use:
xxxxxxxxxx
import pandas as pd
df1 = pd.DataFrame({
"Age": [30, 20, 40],
"Name": ["Alice", "Bob", "Cathy"]
})
df2 = pd.DataFrame({
"Age": [50, 45],
"Name": ["Dave", "Eve"]
})
final_df = df1.append(df2, ignore_index=True)
While panda’s
xxxxxxxxxx
.append()
method may seem straightforward for concatenating DataFrames, there is a caveat – each
xxxxxxxxxx
.append()
results in a full copy of the data, which reduces performance significantly when working with larger datasets. Each repeated call multiplies the time taken, leading to an inefficient use of resources.
Python’s List append() and DataFrame Conversion:
A speedy alternative uses standard Python lists and their inherent
xxxxxxxxxx
.append()
method. Here’s how we can do it:
xxxxxxxxxx
list_data = []
list_data.append(["Alice", 30])
list_data.append(["Bob", 20])
list_data.append(["Cathy", 40])
list_data.append(["Dave", 50])
list_data.append(["Eve", 45])
final_df = pd.DataFrame(list_data, columns=["Name", "Age"])
Our strategy here entails keeping data in a list format as long as possible and converting it into a DataFrame only when necessary. Why is this efficient? Python lists are extremely optimized within the language’s core, making operations like appending to them almost instantaneous regardless of their size. Moreover, creating a DataFrame from a list is incredibly fast.
One crucial aspect to take note of is that if your data includes multiple different datatypes (i.e., numerical and object), you might want to create a structured array with
xxxxxxxxxx
numpy
before transforming it into a DataFrame. This method ensures an appropriate datatype assignment, which might not occur if you pass a basic list to the DataFrame function.
Pandas’ documentation itself offers valuable advice, suggesting the use of the more efficient
xxxxxxxxxx
pandas.concat()
over
xxxxxxxxxx
append()
for combining DataFrames along a particular axis. Similarly, the approach I’ve discussed allows you to harness the performance powers of Python’s core functionality, bypassing pandas until the final step of DataFrame conversion.
In summary, while
xxxxxxxxxx
pandas.DataFrame.append()
is still viable for small scale operations, efficiently handling larger datasets requires the adaptability and skill to leverage other strategies such as Python’s native list append process.
xxxxxxxxxx
combine_first()
method in Python’s pandas library provides a powerful tool for combining datasets, filling null values with respective data from the other dataframe. This allows coders to enhance their data manipulation and is a very good alternative to the more commonly used
xxxxxxxxxx
.append()
method.
The difference between the two is how they manage merging conflicts and missing data:
- The
xxxxxxxxxx
111.append()
method just glues the dataframes vertically (one below another), without considering if the appended rows perfectly match the main dataframe structure.
- In contrast, the
xxxxxxxxxx
111combine_first()
method acts a bit differently. It takes the row values of the first dataframe, and only if some are missing (NaN), it fills them with the values of the corresponding row in second dataframe.
Here’s an illustrative example on the usage of
xxxxxxxxxx
combine_first()
showcasing its benefits:
xxxxxxxxxx
import pandas as pd
df1 = pd.DataFrame({'A': [1, 2, None], 'B': [4, None, 6]})
df2 = pd.DataFrame({'A': [10, 20, 30], 'B': [40, 50, 60]})
result = df1.combine_first(df2)
print(result)
Output:
A | B | |
---|---|---|
0 | 1.0 | 4.0 |
1 | 2.0 | 50.0 |
2 | 30.0 | 6.0 |
You can see from the output that where
xxxxxxxxxx
df1
had null values in columns ‘A’ and ‘B’, these have been replaced with the corresponding values from
xxxxxxxxxx
df2
. Furthermore, where
xxxxxxxxxx
df1
had actual data – this has been preserved and hasn’t been overwritten by
xxxxxxxxxx
combine_first()
. As we conclude, this approach provides a better level of control for developers when dealing with appending data and managing NaN values – proving to be a fantastic utility method to append or blend data.
Incorporating methods like
xxxxxxxxxx
combine_first()
into your database handling can significantly boost your ability to clean, merge, and manipulate data – making it a handy addition to any developer’s toolkit.
When it comes to data manipulation and structure alteration, pandas is an intriguing Python library that shines bright. Its array of functions, such as
xxxxxxxxxx
append()
and
xxxxxxxxxx
update()
, provide impressive flexibility in handling DataFrame objects.
However, it’s noteworthy that the
xxxxxxxxxx
append()
method might not be the most effective approach when you want to modify existing items in a DataFrame. Excessive usage of
xxxxxxxxxx
append()
can lead to inefficient memory usage and longer execution times. An ideal alternative here would be the
xxxxxxxxxx
update()
function. So, let’s delve deeper and unveil the potential of the update() function which stands out as a good replacement for append().
Understanding Update Function
xxxxxxxxxx
DataFrame.update()
is an in-place method in pandas, which modifies the caller DataFrame with values from another DataFrame while aligning with the specified DataFrame index/ column. One crucial thing to note is that it does not return a new DataFrame but modifies the original one.
Here are some salient features of the
xxxxxxxxxx
update()
function:
+ It only updates values in the original DataFrame that are shared with the second DataFrame. Non-shared values are not affected.
+ Unlike
xxxxxxxxxx
append()
,
xxxxxxxxxx
update()
doesn’t increase DataFrame size.
+ This method also prioritizes maintaining the original DataFrame’s data type over updating its values.
Let’s have a glance at a simple example:
xxxxxxxxxx
import pandas as pd
df1 = pd.DataFrame({'A': [1, 2, 3],
'B': [400, 500, 600]})
df2 = pd.DataFrame({'B': [4, 5, 6],
'C': [7, 8, 9]})
df1.update(df2)
print(df1)
The output will be:
A | B | |
---|---|---|
0 | 1.0 | 4.0 |
1 | 2.0 | 5.0 |
2 | 3.0 | 6.0 |
Clearly, as observed, the values under column ‘B’ of df1 got updated based on df2, whereas column ‘A’ remained unchanged. Column ‘C’ was nowhere to be found because it doesn’t exemplify any commonality between df1 and df2.
Overwhelmed? Worry not! Pandas documentation serves as a great guide to understand more about how the
xxxxxxxxxx
update()
function works.
Moreover, in the long run, understanding the use-cases and effectiveness of each tool becomes vital. While pandas .append() method proves fruitful to concatenate along the rows axis, the
xxxxxxxxxx
update()
function tends to be a more time and memory-efficient way when your goal is to actually modify the existing data points instead of attaching new ones.
So, take some time, try out these approaches in different use cases and appreciate the versatility Pandas brings to our fingertips!If you’re using Pandas in your data analysis workflow, you’ll often find yourself needing to combine your DataFrames. The
xxxxxxxxxx
.append()
method might be your first go-to, but it’s not always the best choice. Depending on your situation, choosing an alternative method could deliver better performance and more flexibility.
One excellent alternative is the
xxxxxxxxxx
.concat()
function. This versatile tool can handle a variety of tasks that go beyond simple appending. Whilst
xxxxxxxxxx
.append()
merely attaches one DataFrame below another,
xxxxxxxxxx
.concat()
can merge them side-by-side as well.
Let me provide you with a code snippet example:
xxxxxxxxxx
import pandas as pd
# Create two sample dataframes
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2', 'A3'],
'B': ['B0', 'B1', 'B2', 'B3'],
'C': ['C0', 'C1', 'C2', 'C3'],
'D': ['D0', 'D1', 'D2', 'D3']},
index=[0, 1, 2, 3])
df2 = pd.DataFrame({'A': ['A4', 'A5', 'A6', 'A7'],
'B': ['B4', 'B5', 'B6', 'B7'],
'C': ['C4', 'C5', 'C6', 'C7'],
'D': ['D4', 'D5', 'D6', 'D7']},
index=[4, 5, 6, 7])
result = pd.concat([df1, df2])
Table format of the dataframe `df1` would look like this:
A | B | C | D | |
---|---|---|---|---|
0 | A0 | B0 | C0 | D0 |
1 | A1 | B1 | C1 | D1 |
2 | A2 | B2 | C2 | D2 |
3 | A3 | B3 | C3 | D3 |
Improved performance is another advantage of
xxxxxxxxxx
.concat()
over
xxxxxxxxxx
.append()
. As per the [Pandas documentation](https://pandas.pydata.org/pandas-docs/stable/user_guide/merging.html), .append() essentially executes a concat along axis=0, which means repeated calls are rather inefficient. If you know up front what you want to append, using
xxxxxxxxxx
pd.concat
on an iterable will yield much better performance results.
One final note: both of these functions create a new DataFrame by design, rather than modifying the original ones. This behavior might feel inconvenient at times but rest assured, it’s actually a vital safety measure that prevents unintentional modification of your source data.
Exploring alternatives to the commonly used methods in a library like Pandas is a time-tested strategy. By doing so, you gain extra tools for your kit, widening your ability to tackle different situations and enhancing your efficiency. So next time when you think about combining your DataFrame, remember: it’s not just about the
xxxxxxxxxx
.append()
, expand your horizons with the
xxxxxxxxxx
.concat()
function.