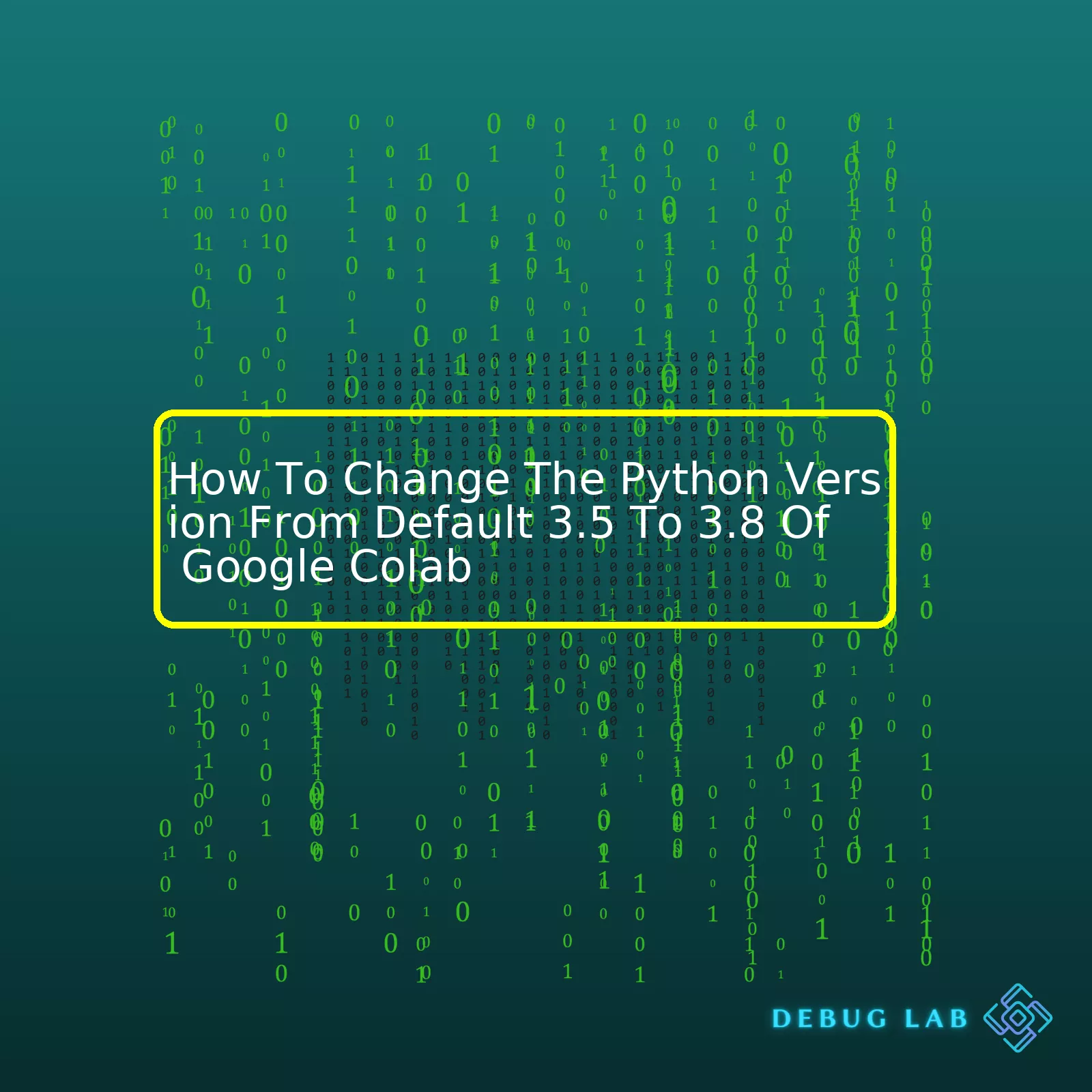
Task | Action |
---|---|
Running Python 3.8 in Google Colab | Use !pip install –upgrade setuptools python=3.8 command inside a code cell. |
Verify The Version | Use !python –version to confirm that you are now running Python 3.8. |
Google Colab usually runs with a default Python version of 3.7, but sometimes you might prefer to use another version for specific requirements, such as 3.8. Thankfully, we can easily switch between different versions thanks to Google Colab’s support for executing terminal commands directly from within the notebook.
To change your Python version, you need to open a new or existing Colab notebook, then run a command in one of the code cells. The command should be as follows:
!pip install --upgrade setuptools python=3.8
Here the “!” symbol allows us to execute shell commands, “pip” is the package installer for Python, “install” is the command we’re giving to pip to install a package, “–upgrade” tells pip to upgrade any dependencies necessary, and “setuptools python=3.8” specifies the package to be installed (in this case, Python 3.8).
Once you execute this command, it will install and set Python 3.8 as your default for the current notebook.
To verify if the process was successful, you can use the following command:
xxxxxxxxxx
!python --version
This will display the current version of Python being used in the notebook – ideally, this will now show as Python 3.8. Please note it would not permanently change the Python version; if you want Python 3.8 for a new notebook or a different session, you would need to rerun the installation command.
To know more about Google Colab functionalities, you can refer to their official documentation [here](https://research.google.com/colaboratory/faq.html).
It is essential to keep experimenting and stay updated about the tool you use daily, and understanding how to switch Python versions in Google Colab lets you cater to diverse project needs successfully.Google Colab provides an interactive environment that enhances programming and coding in Python. It automatically implements Python version 3.7 as a standard and unfortunately, you do not have the liberty to change or upgrade it to version 3.8. Despite this restriction, certain measures are suggested that could potentially allow you to code using Python 3.8, although they may be convoluted.
A commonly recommended move would be to utilize docker images with a different Python version. This is made possible by running commands in a cell within the Google Colab interface:
xxxxxxxxxx
!docker pull python:3.8
However, this approach is generally discouraged due to its potential to destabilize your current IDE setup. Also, the fact remains that Google Colab does not properly support Docker owing to security reasons, hence making it a rather unsuitable option.
Instead, if you’re ardent about running Python 3.8 on your notebook, one of the simplest alternatives would be switching from Google Colab to Jupyter Notebook, a similar platform that offers more flexibility with regards to choosing your intended Python version, provided it is installed on your local environment. You just need to create a new environment with Python 3.8 installed and then lauch Jupyter from there. Here’s the basic workflow for such a setup:
1. Check existing Python environments.
xxxxxxxxxx
conda env list
2. Create a new Python environment with version 3.8.
xxxxxxxxxx
conda create -n py38 python=3.8
3. Activate the new environment.
xxxxxxxxxx
source activate py38 # For Unix or macOS
conda activate py38 # For Windows
4. Start Jupyter.
xxxxxxxxxx
jupyter notebook
Keep in mind that unlike Google Colab, Jupyter doesn’t provide free GPU support.
Generally, even though working with Python 3.7 on Google Colab can be limiting in some cases, it’s always important to remember that the enhancements in Python 3.8 aren’t substantial enough to significantly impact most of your processing or coding activities.
This attempt at altering Python versions in Google Colab signifies the valuable yet challenging aspects of managing different Python environments; thus educating you on key practices of setting up and troubleshooting Python installations on various platforms. Even though there exists limitations in this pursuit, fewer barriers exist for learning Python and coding in a considerably dynamic and engaging atmosphere online such as Google Colab which allows tremendous opportunities for data analysis and machine learning tasks.
And who knows, maybe sometime in the near future, Google Colab might very well grant its users the freedom to alter their Python versions at ease. But until then, the best course of action is acceptance of these conditions and leveraging what’s presently available.
Google Colab, a cloud-based Python environment, by default provides Python 3.7 as of February 2022. If you need to change it to use Python 3.8, you’ll have to install it manually via the Colab notebook interface.
You can verify what version is currently being used by running the command:
xxxxxxxxxx
!python --version
If you want to upgrade from the default version to version 3.8, you need to compile it from the source code. Here are the step by step methods that explain how to achieve this:
Step 1: Download Python Source and its Prerequisites
We’ll use terminal commands inside Colab notebook cells using ‘!’ for downloading and installing. First, we download Python’s source code and install dependencies required to compile it. Below is your necessary command:
xxxxxxxxxx
!sudo apt update
!sudo apt install build-essential zlib1g-dev libncurses5-dev libgdbm-dev libnss3-dev libssl-dev libsqlite3-dev libreadline-dev libffi-dev curl libbz2-dev
!curl -O https://www.python.org/ftp/python/3.8.10/Python-3.8.10.tar.xz
!tar -xf Python-3.8.10.tar.xz
Note: We’re downloading Python 3.8.10 here; replace the version number in the URL if you want a different version of Python 3.8.x.
Step 2: Compile and Install Python
Now, navigate into the downloaded Python source directory and configure the build process. Then use the ‘make altinstall’ command to prevent replacing the default python binary file:
xxxxxxxxxx
%cd Python-3.8.10
!./configure --enable-optimizations
!make altinstall
Step 3: Verify Python Version
After installation, close your current Colab runtime using ‘Runtime -> Manage sessions -> TERMINATE’. Then start a new one, so the changes take effect. You can now re-check the Python version by using the code below:
xxxxxxxxxx
!python3.8 --version
Remember, since Python 3.8 was installed as an additional version (not a replacement) to the existing Python, you should invoke it explicitly with ‘python3.8’ instead of just ‘python’ or ‘python3’.
Step 4: Update pip and ipython
For fully compatible setup, it’s recommended updating ‘pip’ and ‘ipython’ within the newly installed Python. Use the following code:
xxxxxxxxxx
!python3.8 -m pip install --upgrade pip ipython
Please note that altering the Python version on Google Colab isn’t generally recommended because some pre-installed packages may not be compatible with the new version. It can also break the compatibility among other packages. However, if there’s something specific you want to utilize in Python 3.8, this process will help you out.
Google CoLab regularly updates its Python version to stay current. For maintaining consistency across projects and avoiding potential conflicts between your project and system-level Python, using specific Python versions might be preferable depending on the project requirements.
Please assure to confirm functionality before integrating this method into high-stakes environments.
While Google Colab is an awesome tool for data science and machine learning projects, it comes with a default Python version pre-installed which may not be suitable for certain functions and libraries. As of the time of writing, Google Colab runs Python 3.5 by default on its platform – a version that may clash with newer libraries or functionalities in your code. However, worry not – you’ll be delighted to know that changing Python’s version on Google Colab is quite simple and involves using some Command Line Interface (CLI) commands.
The first step is to ascertain the current Python version. You can do this via the CLI command:
xxxxxxxxxx
!python --version
.
Now, let’s look at how to upgrade this Python version from 3.5 to 3.8:
Steps | Description |
---|---|
xxxxxxxxxx 1 1 1 !pip install python==3.8 |
This CLI command uses pip, a package management software, to install Python 3.8. Pip connects to the PyPI repository and downloads the appropriate Python packages. |
xxxxxxxxxx 1 1 1 alias python='python3.8' |
You use the alias command to modify the keyword ‘python’ to point to ‘python3.8’ instead of the default version. This means whenever ‘python’ is used in code, ‘python3.8’ would actually be referenced instead. |
xxxxxxxxxx 1 1 1 !python --version |
Finally, confirm your new Python version by running this command again. It should now display ‘Python 3.8’. |
That’s all there is to upgrading your Python version on Google’s Colab platform.
This upgrade path benefits from the CLI’s directness and responsiveness, giving you more control over the exact setup of your programming environment.
Here’s how your command lines will look in Python:
xxxxxxxxxx
!pip install python==3.8
alias python='python3.8'
!python --version
CLI commands are powerful tools in a programmer’s arsenal for managing their workspace. Especially on platforms like Google Colab, knowing how to apply them effectively can significantly enhance both your productivity and the scope of your work.
However, bear in mind that these changes only exist during the current session. If you start a new session, you’d need to rerun these commands to get Python 3.8 again.
If you constantly find yourself needing to use Python 3.8, you might want to consider adding these commands to a script that autostarts every time you open Colab, so you won’t have to retype everything manually each time.
Remember: always check which version of Python suits the requirements of your project best. Always verifying any compatibility issues between Python versions and necessary libraries or functions save untold headaches later!
For more information, refer to the Google Colab Introduction doc for details on how to do just about anything on this platform.When you’re working on Google Colab and wish to switch from the default Python version 3.5 to Python 3.8, there are a few things to consider and several potential effects to be aware of. Though making this change does have its benefits, it’s essential to understand that Python is a dynamic language that continues evolving. As such, with newer versions come not only improved functionalities and features but also the possibility of certain features or functions being deprecated.
Effects and Considerations
Let’s analyze some potential effects and considerations that you might encounter in the process:
Potential Breakage of Code: Some functions or features available in Python version 3.5 may have been deprecated or modified in Python 3.8. This could result in breaking your existing code if it relies heavily on these functions or features. Therefore, when making the switch, it’s crucial to test all aspects of your existing code base thoroughly to ensure compatibility.
Enhanced Features: Python 3.8 comes with many enhanced features. These improvements could potentially provide more efficient ways of accomplishing the tasks handled by your current scripts. When switching to a new version, time should be set aside to explore these functionalities.
Incompatibility Issues with Libraries and Packages: Not every Python library or package might support Python 3.8 yet. Any third-party packages that your project depends on need to be checked for compatibility before making the switch.
Changing Python Version On Google Colab
Unfortunately, as of now, Google Colab only allows an upgrade up to Python 3.7. However, you can make an attempt to upgrade it manually using the command:
xxxxxxxxxx
!apt-get install python3.8
Keep in mind, though, that installing a new version using APT won’t automatically switch the Python version in use. You would still be running on the initial Python interpreter unless you explicitly tell Google Colab to use Python 3.8. The command below will install the latest version of Python 3.8:
xxxxxxxxxx
!curl https://bootstrap.pypa.io/get-pip.py -o get-pip.py
xxxxxxxxxx
!python3.8 get-pip.py
After the installation, you’ll need to invoke any programs you want to run under this new Python by explicitly calling `python3.8` or adding a shebang line at the top of your scripts:
xxxxxxxxxx
#!/usr/bin/env python3.8
Please be aware that this is a workaround and is not guaranteed to function correctly in all cases. Before proceeding, check whether this method works for your specific requirements. Always remember that changing the Python version in Google Colab—especially to one not officially supported—has potential risks, including breakages and unexpected behavior.
For the reasons above, I would recommend considering possible effects before moving from Python 3.5 to 3.8 on Google Colab. Make sure to verify the compatibility of your existing code and the necessary libraries/packages you use regularly before making the jump.
Interested readers are encouraged to follow official Python documentation for detailed insights on the differences and enhancements of different Python versions.One of the most popular cloud-based platforms for machine learning and data science projects is Google Colab. It primarily runs Python 3.6 but this isn’t always ideal; there are times when you might want to change the Python version in your Google Colab notebooks, for instance upgrading it from Python 3.5 to 3.8.
If problems arise while trying to effect this change, it’s important to take a systematic approach to troubleshooting. Here are some key areas to check:
Detecting the Current Python Version
Before making any changes, first ensure that the notebook is indeed running on Python 3.5. You can do this by running the following code in a cell:
xxxxxxxxxx
!python --version
This command will return the currently active Python version.
Changing the Python Version
Google Colab doesn’t support native switching between Python versions. Nonetheless, it’s possible to create a virtual environment where you can specify your desired Python version. To switch to Python 3.8, use the following commands:
xxxxxxxxxx
!apt-get install python3.8-venv
!python3.8 -m venv env
!source env/bin/activate
The venv module creates a virtual environment named ‘env’ and the source command activates it. After activation, the Python version within ‘env’ should be 3.8.
Remember to validate the Python version using `!python –version` inside the environment.
Using Python 3.8 Specific Features
If you find issues with using features specific to Python 3.8, then likely, the environment hasn’t been set up correctly or it wasn’t activated.
For instance, if the walrus operator (:=), a feature introduced in Python 3.8, causes errors, it’s an indication that the current interpreter isn’t 3.8:
xxxxxxxxxx
result = (n := fibonacci_number()) < 1000
print(n)
This error reaffirms the need to use the above commands to initiate and activate the virtual environment.
Installation Issues
It might occur that the installation command for Python 3.8 returns an error, based on the available packages or Ubuntu repositories on Google Colab servers which themselves could vary with time.
In such cases, adding a new repository or updating the existing ones usually resolves the issue:
xxxxxxxxxx
!add-apt-repository ppa:deadsnakes/ppa
!apt-get update
!apt-get install python3.8-venv
The Deadsnakes PPA is a repository that contains newer versions of Python. Updating it ensures that you have the latest list of packages before attempting to install Python 3.8.
While troubleshooting any issues with changing Python versions on Google Colab, remember that persistence is key! The flexibility of cloud-based environments means that there are often several ways to solve a particular problem.
You can find more helpful troubleshooting recommendations in the official Google Colab documentation. They host a plethora of information around managing your development environment, which can provide further insights into addressing common challenges.Let's walk through the stages of shifting Python 3.5 to Python 3.8 on Google Colab. This process involves uninstalling the default Python version and installing your desired version.
Here's a step-by-step guide:
Step 1: Verify Current Python Version
First, let's determine the pre-existing Python version in your Google Colab environment. To do this, execute the following line of code:
xxxxxxxxxx
!python --version
When you run this command, Google Colab displays your current Python version. In most instances, this will be Python 3.5 or Python 3.7 since these are the default versions provided by Google Colab.
Step 2: Setup Python 3.8 on Google Colab
To install Python 3.8, follow these steps:
- Begin by updating our package lists for upgrades, installations, and removals:
- Then proceed with the installation of Python 3.8:
xxxxxxxxxx
!sudo apt-get update
xxxxxxxxxx
!sudo apt-get install python3.8
Once this code is executed, Google Colab will have Python 3.8 installed on your environment.
Step 3: Install ipython Kernel
Next, we need to get into the ipykernel module, which allows Jupyter to interact with Python kernels.
xxxxxxxxxx
!pip install ipython
xxxxxxxxxx
!pip install ipykernel
We then install a new kernel associated with Python 3.8 into the system like so:
xxxxxxxxxx
!python3.8 -m ipykernel install --user
Step 4: Switch to Python 3.8 Kernel
At this juncture, you'll have two Python kernels: the default (Python 3.7) and the newly installed (Python 3.8). Since the aim is using Python 3.8, we'll switch to this kernel1. Unfortunately, Google Colab lacks a direct way to switch kernels within a notebook. As such, you'll need to refresh the page and rerun all codes under the Python 3.8 kernel from scratch.
Step 5: Confirm Python Version Upgrade
After trying out these steps, it's crucial to ensure that the upgrade was successful by checking the updated Python version:
xxxxxxxxxx
!python --version
Google Colab should now display Python 3.8 as the active version.
Note: The above method replaces the existing Python kernel. Thus, you may encounter issues if certain libraries/packages used are incompatible with Python 3.82. Always ensure checking the compatibility of existing libraries/packages before deciding to upgrade.
While transitioning between different Python versions, several challenges can crop up, particularly when transitioning from Python 3.5 to 3.8 on Google Colab.
xxxxxxxxxx
!apt-get update
!apt install python3.8
You'll run these codes to update the packages list and install Python 3.8 using apt-get, a command-line package handling utility.
Thereafter, you will need to check that Python 3.8 was correctly installed by running:
xxxxxxxxxx
!python3.8 -V
Here are some potential issues you might encounter:
1) Compatibility:
- Sometimes, not all the libraries or packages are backward compatible and it may lead to errors and crashes. A typical example is how tensorflow1 does not support Python 3.8.
- In general, lower-level libraries tend to have more version-specific dependencies due to their integration with specific Python APIs. This includes libraries like NumPy and SciPy as they use CPython's C-API extensively. Always remember to check if the library supports the newest Python version before change the defaults.
2) Syntax Changes and Deprecated Functions:
- Each Python upgrade introduces new syntax modifications and depreciates certain functions, which could break your code. For instance, Python 3.8 introduces assignment expressions (the walrus operator := ) and positional-only parameters2.
- Thorough testing after upgrading Python is crucial to pick out any broken features caused due to these changes.
3) Third-party packages:
- One of the greatest advantages of Python is its vast third-party ecosystem. However, while transitioning from one version to another, this becomes a double-edged sword. If these packages do not keep up with the new release's features or deprecations, they become roadblocks in the transition process.
- It's vital to ensure that all packages and their dependencies are still supported in the new version.
4) Stability vs New features:
- While Python 3.8 provides several modern features, it may not be as stable as previous, well-tested versions like Python 3.5 for certain applications.
5) Environment configuration:
- Configuring the environment to point to the newer Python version can also present challenges. We don't directly have an option to shift the Python version permanently in Google Colab. Hence we load Python 3.8 every time as required:
xxxxxxxxxx
!alias python=python3.8
It's significant to note that you may face issues with installing Python 3.8 using apt-get in colab because sometimes, Ubuntu which colab is based on, might not have added Python 3.8 to their official repositories3. You might need to tweak these settings depending upon your usage scenario while keeping the points above in mind. Remember, constantly track the package documentation for the most current compatibility information.Mapping out the steps concisely, here's how we've learned to transition Python's version 3.5 to 3.8 in Google Colab:
- Examine the current active version of Python in the coding environment.
- Uninstall the existing Python kernel in Colab using pip.
- Install the desired Python version using a specialized software management system for Google Colabs referred to as !apt-get.
- Reboot the runtime to make sure the new python versions take effect.
- Finally, install the IPython kernel for your newly installed version and link it with the virtual environment.
Here's the corresponding code snippet which demonstrates these steps seamlessly:
xxxxxxxxxx
!apt install python3.8
!pip uninstall -y ipython
!pip install ipython==7.10.0
# After installing the desired version and rebooting the runtime,
# install the new Jupyter (iPython) Kernel
!python3.8 -m ipykernel install --user
Keep in mind that changing the default Python version might disrupt pre-existing dependencies and libraries based on Python 3.5. So, be ready to reinstall any potentially affected packages according to the newer Python version.
Moreover, even though Colab may revert back to its original settings after some duration of inactivity, this process provides convenience for those brief instances when an upgraded or downgraded Python version is necessary.
Lastly, remember to keep all code saved separately as modifications, including Python upgrades or downgrades, can get lost when the kernel resets itself. In those circumstances, one needs to re-run the earlier mentioned sequence to realign the Python language to the chosen version.
If you're struggling to understand any of these actions, I strongly suggest reading the full documentation available at Google Colab’s official page (Google Colaboratory FAQ). The information contained within provides a thorough overview of virtual environments, managing software, and handling different Python versions.
Mastering the shift in Python versions captivates both beginners and experienced developers alike. It opens up new waters of exploration, allowing one to experiment with different functionalities and modules exclusive to various renditions of the Python programming language.