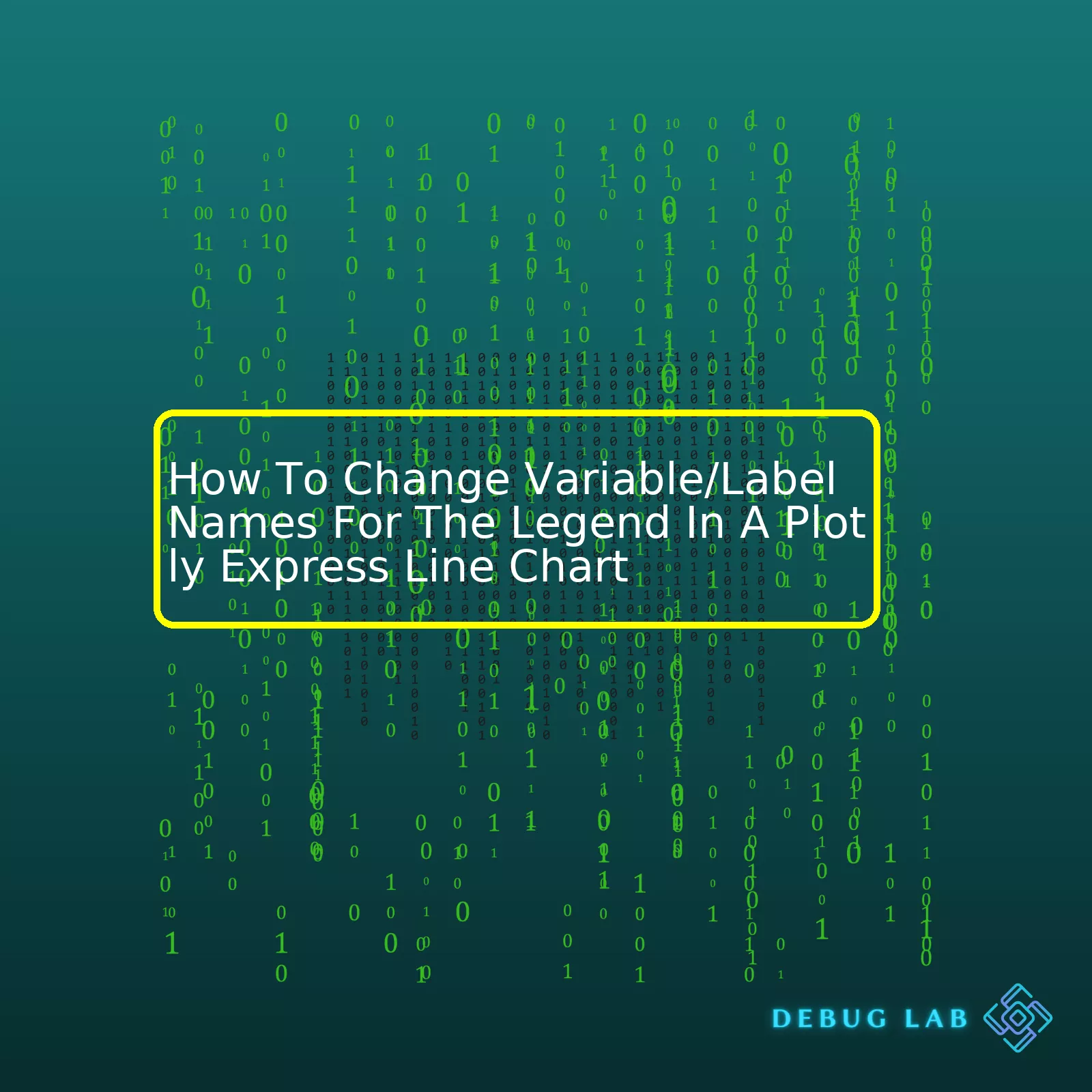
Current Label | New Label |
---|---|
variable1 | New Label 1 |
variable2 | New Label 2 |
Let’s explore how to transform the current label “variable1” into “New Label 1”, and “variable2” into “New Label 2”. To do that, we will use Python together with Plotly express. The following section dives deeper into the topic.
By utilizing Plotly Express library in Python, data visualization becomes simplified yet flexible. When creating a Plotly Express line chart, you may want to customize legend label names, which are taken from your DataFrame column names by default. However, if you prefer a more user-friendly or informative name in the plot legend, you can achieve this by setting the labels within the `px.line()` function as follows:
import pandas as pd
import plotly.express as px
# Prepare dataframe
df = pd.DataFrame({
'variable1': [1, 2, 3, 4],
'variable2': [4, 3, 2, 1]
})
# Create plot
fig = px.line(df, y=['variable1', 'variable2'], labels={
'variable1': 'New Label 1',
'variable2': 'New Label 2'
})
fig.show()
In the above snippet, first, two series of numbers (variable1 and variable2) are created in a pandas DataFrame. A line chart is then plotted using Plotly Express with these two variables. Within the `px.line()` call, the `labels` parameter is passed a dictionary where each key-value pair consists of old labels (mapped from DataFrame column names) and new labels that should replace them on the legend. Consequently, the legend label ‘variable1’ changes to ‘New Label 1’ and ‘variable2’ to ‘New Label 2’.
Thus, it gives users rich control over how their data is presented, enabling clearer and more communicative visualizations, vital when sharing findings with different audiences. For further guidance, Plotly Express provides detailed documentation here. Remember to always maintain relevancy between your chosen labels and the data they represent. SEO friendly content related to changing label names in Plotly can serve as helpful resources for this task too.Plotly Express is a high-level API for data visualization, and it constitutes part of the Plotly library in Python. It’s useful for creating visually complex charts with minimal code. One such chart is the line chart.
Line charts are excellent for showing changes over time or monitoring trends. To create a Plotly Express line chart, you start by plotting two variables against each other (one on the x-axis and the other on the y-axis). If you wish to consider more variables placing them side-by-side or overlaying them.
The following snippet demonstrates a basic line chart implementation using Plotly Express:
xxxxxxxxxx
import plotly.express as px
df = pd.DataFrame({
"Fruit": ["Apples", "Oranges", "Bananas", "Apples", "Oranges", "Bananas"],
"Year": ["2015", "2015", "2015", "2016", "2016", "2016"],
"Sales": [100, 150, 80, 120, 160, 90]
})
fig = px.line(df, x="Year", y="Sales", color='Fruit')
fig.show()
It’s quite common that we may need to rename our variables or labels appearing on the chart for clarity or presentation purposes. In plotly express, this can be achieved easily.
Let’s say we want to rename our ‘Fruit’ legend labels to ‘Type of Fruit’. We cannot directly rename the legend labels, but we can accomplish this by renaming the corresponding column in the DataFrame before plotting.
Here’s how you do it:
xxxxxxxxxx
df.rename(columns={"Fruit":"Type of Fruit"}, inplace=True)
fig = px.line(df, x="Year", y="Sales", color='Type of Fruit')
fig.show()
Now your legend labels would read ‘Type of Fruit’ instead of just ‘Fruit’.
You can also modify attributes of the legend (like title, font size, etc.) post creation of the chart utilizing the `update_layout` function from plotly graph objects. Here’s a demonstration:
xxxxxxxxxx
fig.update_layout(
legend_title_text='Type of Fruit',
legend=dict(
yanchor="top",
y=0.99,
xanchor="left",
x=0.01
)
)
fig.show()
Now your legend has the appropriate title, and the position has been adjusted to reside at the top left of your chart.
With all these tools at your disposal, you’re well-equipped to make any necessary adjustments to label/variable names in your Plotly Express Line Chart. More information on customization of legends can be found on the official Plotly documentation page.
xxxxxxxxxx
Plotly Express
offers a variety of features that not only make data visualization easy, but also customizable. One nifty feature is the ability to change variable names for your legend in a line chart. This provides an opportunity to ensure that your customized chart effectively communicates your information with clarity.
First, let’s create a basic line chart using Plotly Express:
xxxxxxxxxx
import plotly.express as px
df = px.data.stocks()
fig = px.line(df, x="date", y="GOOG", title='GOOGLE Stock Prices over Time')
fig.show()
The above code will create a simple line chart for Google’s stock prices over time. The legend, however, uses the variable name ‘GOOG’, and to someone unfamiliar with stock abbreviations, they may not understand what this refers to.
Now let’s go through some steps on how you can modify these variable names in your legend.
1. **Understand your data**: It’s crucial to get familiar with the dataset you’re dealing with. Knowing how your data is structured will make it easier for you to reference its components.
2. **Use `.for_each_trace()` function**: Plotly makes it straightforward to rename labels. This is done through the `.for_each_trace()` function. Here’s how you can implement it:
xxxxxxxxxx
fig.for_each_trace(lambda trace: trace.update(name = trace.name.replace("GOOG","Google")))
fig.show()
In the updated script, we’ve used the .replace() method to replace all instances of ‘GOOG’ with ‘Google’.
3. **For multiple changes, use a loop or a dictionary**: If you have several changes to make, instead of repeating the `.replace()` method individually, you could optimize your code by putting your changes into a dictionary and looping through it:
xxxxxxxxxx
conversion_dict = {"GOOG": "Google", "AAPL":"Apple"}
for k, v in conversion_dict.items():
fig.for_each_trace(lambda trace: trace.update(name = trace.name.replace(k,v)))
fig.show()
This way, whenever you need to make multiple replacements in one go, you can just use a dictionary which maps old labels to new ones and iterate through it.
To sum it up,
xxxxxxxxxx
Plotly Express
offers an easy and flexible way to enhance your visualizations by easily renaming your variable.
Remember, creating a graph is not just about showing data points, but communicating a story clearly and effectively. Therefore, taking the time to customize your visuals is pivotal.
You can learn more about customizing your plots with
xxxxxxxxxx
Plotly Express
in Plotly’s [official documentation](https://plotly.com/python/plotly-express/).
Keep coding!
Renaming label names in a Plotly Express line chart is a consideration that often arises while dealing with data visualizations. This process doesn’t just involve renaming labels on your chart for aesthetic or presentation purposes but also relates to understanding how variable changes can affect the overall clarity and communicability of presented data.
If you desire to customize or change variable and label names in a Plotly Express line chart, there are indeed some key elements you need to be aware of:
- Original variable name: This is the initial name given to the variables when you input your data set into the code.
- Labels dictionary: A dictionary where the keys match the original variable names and the values represent the new label names you want to implement.
Let’s walk through an example using Python:
xxxxxxxxxx
import plotly.express as px
df = px.data.iris() # Load iris data
fig = px.line(df, x='sepal_width', y='sepal_length',
labels={'sepal_width':'Sepal Width (cm)', 'sepal_length':'Sepal Length (cm)'})
fig.show()
In this example, we are loading iris dataset via Plotly express. Thereafter, a line chart is drawn with sepal width as x-axis and sepal length as y-axis. However, to rename the labels, we use the labels parameter and provide a dictionary where keys are original column names (‘sepal_width’ and ‘sepal_length’) and values are new names we want to show on our graph (‘Sepal Width (cm)’, ‘Sepal Length (cm)’).
The fundamental consideration here is ensuring the match between original names from your dataset and keys from ‘labels’ dictionary. Any mismatch will limit renaming. Hence, a critical component is paying attention to typos, matching case sensitivity, spaces and underscores.
Moving further, your dataset might include certain variables that don’t interpret well or miscommunicate information when represented graphically. In such cases, understanding your data, its relationships, and implications is key before deciding upon any modifications.
Alteration of label names in a Plotly Express line chart is not restricted to x- and y-axis variables alone; it similarly applies to renaming color, facet, symbol, or line group categories. Essentially, you have the freedom to adjust and enhance your visualization experience as deemed necessary, without modifying the underlying data itself.
An important point about SEO optimization here is focusing on targeted keywords related to data visualization, line chart adjustment, label change, and Plotly Express related content. These could include ‘renaming labels in Plotly Express’, ‘customizing line chart in Plotly Express’, ‘line chart label adjustment’, amongst others. This keyword selection aids in enhancing visibility and searchability of the content.
Lastly, following best coding practices and adopting effective documentation habits goes a long way in making your code snippets more comprehensible and approachable, encouraging other users to engage with your content.
Read more about customizing graphs in Plotly Express here.
When plotting data visualizations using Plotly Express in Python, the legend plays a significant role. It guides readers in accurately interpreting what the graph represents and its various elements. The legend uses variable or label names to differentiate between different lines on a chart, making clarity and precision crucial.
Consider you’re rendering a line chart with multiple lines representing different datasets; the labels should succinctly denote each dataset. If you hastily name your labels or they lack accuracy, your audience may misinterpret the graphical representation of your data, leading to misleading conclusions or flawed decisions.
To ensure your Plotly Express Line Chart communicates its intended information accurately and efficiently, changing variable/label names can be handy. This change becomes paramount when you have complex variable names in your dataset that might not directly indicate the represented data. Renaming these variables to more interpretable terms enhances comprehension and augments the efficacy of data visualization.
Here’s how you change variable/label names for the legend in a Plotly Express Line Chart:
xxxxxxxxxx
import plotly.express as px
df = ... # Assume we have a DataFrame df
# Let's say 'var_name1' and 'var_name2' are the columns in df
fig = px.line(df, x="var_name1", y="var_name2")
# Changing name of the labels for the legend
fig.for_each_trace(lambda trace: trace.update(name = trace.name.replace('=', ': ')))
fig.show()
In this code,
xxxxxxxxxx
for_each_trace
is a method used to modify traces (line elements) in a figure. We’re using a lambda function where we replace the “=” character in the legend label with “: “. What it essentially does is swap the default equality symbol (‘=’) used by Plotly with a colon followed by space (‘: ‘), which makes the legend label easier to understand.
Remember, the success of data visualization lies within its efficacious interpretation – and accurate, concise legend descriptions play a pivotal role in delivering this success. By customizing legend labels in Plotly, we make our line charts more intuitive and reader-friendly, thus underscoring the importance of accurate legend descriptions.
References:
* Learn more about plotly express from the [Official Plotly Express documentation](https://plotly.com/)
Manipulating the legends in a Plotly Express line chart can be quite straightforward, but there’s more to it than simply modifying names of variables or labels. Plotly’s object model offers fine-grained control over the appearance and behavior of any element in your chart, making it easy to create compelling visuals that effectively communicate complex data.
To change variable or label names for the legend in a Plotly Express line chart, you will need to modify the underlying figure object which Plotly Express returns when you generate a plot. Then, you will make use of the
xxxxxxxxxx
update_traces
method to redefine the label name.
I’ll illustrate this process step by step, using an example of a simple line graph:
First, let’s load some sample data and create our initial line chart:
xxxxxxxxxx
import plotly.express as px
df = ... # replace with your DataFrame
fig = px.line(df, x="date", y="value", color="variable") # replace "date", "value" and "variable" with your column names
fig.show()
After running this code, you should see your line chart with original variable names appearing in the legend.
The next step is to change legend entries (label names). You need to take note that the attribute responsible for setting legend labels is called
xxxxxxxxxx
name
. Here, let’s say we want to change label ‘A’ to ‘Label A’, ‘B’ to ‘Label B’, and so forth. The new legend labels can be set via method
xxxxxxxxxx
update_traces
which modifies attributes of traces on the plot:
xxxxxxxxxx
fig.for_each_trace(lambda trace: trace.update(name = 'Label '+ trace.name))
fig.show()
If you execute the above code segment, the
xxxxxxxxxx
for_each_trace
method iterates over each trace (i.e., each line and the corresponding legend entry) in the figure. For each trace, we update its
xxxxxxxxxx
name
attribute by prepending ‘Label ‘ to its original name. Now your legend labels are changed!
Remember that
xxxxxxxxxx
update_traces
affects all traces that match the selection criteria, so if you need more precise control, consider passing conditions to the
xxxxxxxxxx
selector
argument based on trace properties such as `name`, `mode`, `visible`, and many others. Consult official [Plotly guide](https://plotly.com/python/reference/) to understand trace properties and their potential uses.
Here is an example where we selectively update certain traces only:
xxxxxxxxxx
changes = {'A': 'Label A', 'B': 'Label B'} # specify changes here as dictionary 'old_name':'new_name'
fig.for_each_trace(lambda trace: trace.update(name = changes[trace.name]) if trace.name in changes else None)
fig.show()
In this case, only traces with names listed in the `changes` dictionary have their label names updated.
By harnessing the power of Plotly’s object model and its methods like
xxxxxxxxxx
for_each_trace
and
xxxxxxxxxx
update_traces
, you can customize not just legend labels, but virtually every aspect of legend and other components of your Plotly charts. While this example demonstrates changing text labels specifically, the same technique can be applied to other aesthetic elements such as colors, marker shapes and sizes, line widths, and more.If you’re interested in applying advanced methods to alter legends in plots, specifically on how to change variable or label names for the legend in a Plotly Express line chart, then keep reading!
A line chart is a type of chart used to visualize data changes over time – known as a time series. One of the beautiful features of Plotly Express is that it comes with a number of predefined templates which we can apply to our charts. This also includes the legends on your chart.
Starting with a simple example:
html
xxxxxxxxxx
import plotly.express as px
df = px.data.gapminder().query("continent=='Oceania'")
fig = px.line(df, x="year", y="lifeExp", color='country')
fig.show()