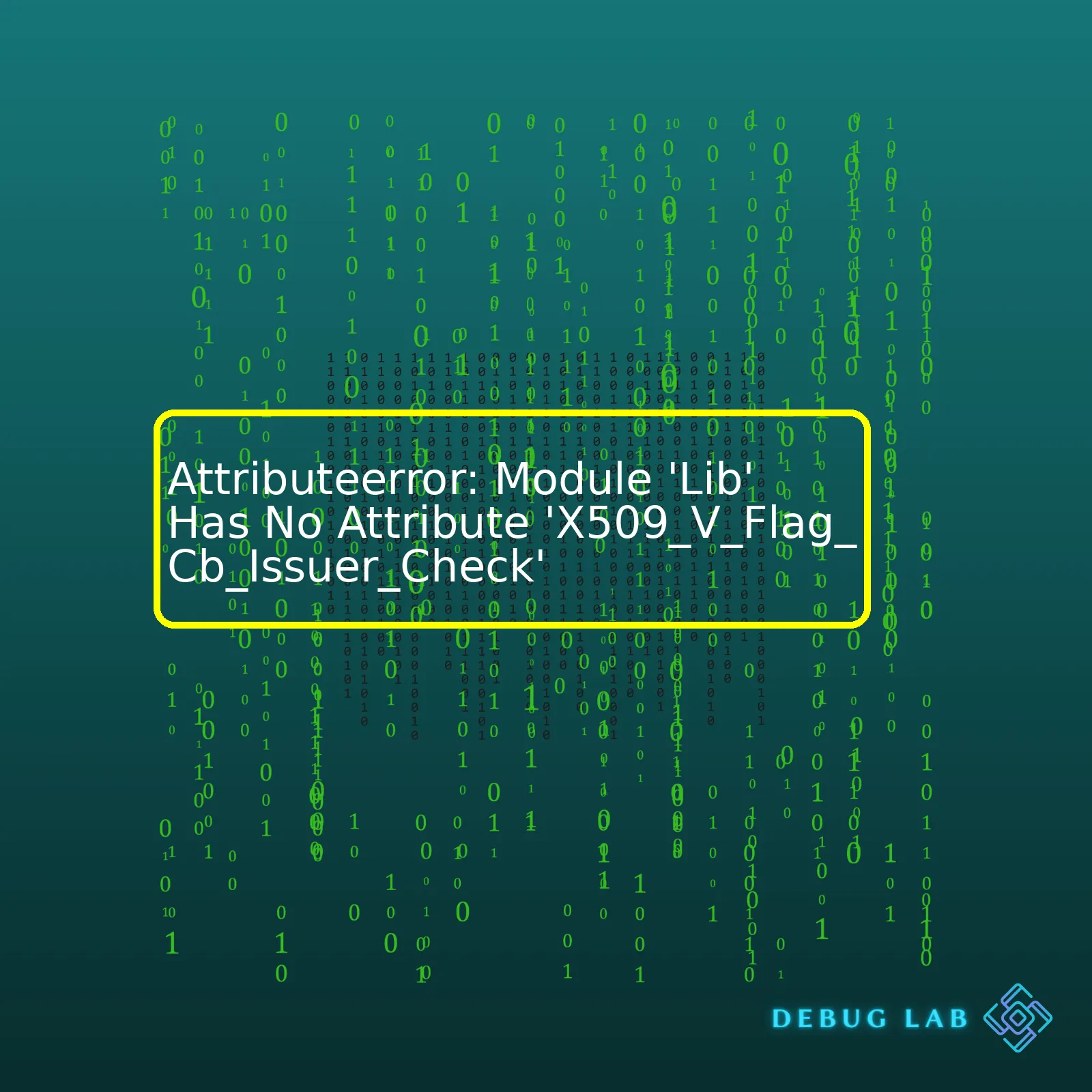
Error | Description | Reason | Solution |
---|---|---|---|
AttributeError: Module ‘lib’ has no attribute ‘X509_V_FLAG_CB_ISSUER_CHECK’ | This is a type of AttributeError that occurs when you try to access an attribute or method X509_V_FLAG_CB_ISSUER_CHECK in the ‘lib’ module which does not exist. | Usually happens due to updates in the OpenSSL library version that might have deprecated or removed ‘X509_V_FLAG_CB_ISSUER_CHECK’ | The resolution would be to update your python’s cryptography library or modify your code to avoid using ‘X509_V_FLAG_CB_ISSUER_CHECK’ |
The “AttributeError: Module ‘lib’ has no attribute ‘X509_V_FLAG_CB_ISSUER_CHECK'” error is one that Python programmers may come across when working with SSL and certificates in their applications. This issue usually hails from trying to tap into a method or attribute, precisely ‘X509_V_FLAG_CB_ISSUER_CHECK’, in the ‘lib’ module that doesn’t exist or has been removed from latest versions. Such errors are quite prevalent in coding scenarios where newer versions of libraries deprecate certain functions and methods.
When dealing with OpenSSL related tasks in Python, if you face this Attribute error it typically indicates that your current version of the OpenSSL library might not support ‘X509_V_FLAG_CB_ISSUER_CHECK’. The most probable cause being, either it has been deprecated, or you’re using an older version of the python cryptography library that still uses it, thereby causing the issue.
Clearing the air, to resolve this Attribute Error, an effective approach would be to keep your python’s cryptography library up-to-date by running
pip install --upgrade cryptography
. This operation ensures that your cryptography library aligns well with the current OpenSSL standards, which tend to remove or update some of their functions over different versions. Alternatively, you might want to alter your original code for it not to use ‘X509_V_FLAG_CB_ISSUER_CHECK’. Thereby avoiding the possibility of conflicting with updated versions.
When you’re dealing with Python, specifically the ‘lib’ module, encountering an
AttributeError
can be quite a common occurrence. These types of errors occur when you call on an attribute or method that doesn’t exist within the targeted module or class. In this particular scenario, we’re talking about the
AttributeError: Module 'lib' has no attribute 'X509_V_FLAG_CB_ISSUER_CHECK'
.
First things first, let’s talk about what an attribute error really means. Picture a warehouse (which we’ll liken to our ‘module’) packed to the brim with goods and objects (the attributes or methods). When somebody asks for an item in the warehouse, the workers can only hand them over if they actually exist in the warehouse inventory. If someone requests an item not available in the warehouse, it inevitably causes confusion and delays. An attribute error in the world of Python is just that – the system’s way of saying “I’m sorry, but that item doesn’t exist here”.
The part of our error message stating
X509_V_FLAG_CB_ISSUER_CHECK
is specifying the missing attribute from the ‘lib’ module. The X509_V_FLAG_CB_ISSUER_CHECK, generally utilized within OpenSSL, is not recognized as part of the ‘lib’ package.
Understanding this, we can then infer that the root cause of the issue is that the code references
X509_V_FLAG_CB_ISSUER_CHECK
, which it assumes is part of the ‘lib’ module, when in actuality it isn’t.
So, how do we go about fixing this?
There are two main steps you may need to take:
Step 1: Check your installation
Have a good, thorough check through your package installation history. It could be that you haven’t correctly installed or loaded the necessary module or package that contains this method. Python has many packages that handle SSL certificates like pyOpenSSL, so make sure you have installed the right one.
pip install pyOpenSSL
Step 2: Scopes and Namespaces
One of the more common reasons behind attribute errors is that you’ve either made the function call in the wrong place, where the scope does not include that attribute, or simply mistyped the attribute name. Ensure that you’re calling the attribute from the correct namespace and in the right context.
Step 3: Update Your Code
If the method no longer exists due to a newer version of the library, you may need to update your code to reflect these changes. Always keep yourself updated with changes to libraries – reading up on their documentation is key.
Another thing to always remember is asking for help – community platforms like StackOverflow are invaluable resources, and chances are that somebody else ran into the same problem and found a solution.
In essence, the AttributeError: Module ‘lib’ has no attribute ‘X509_V_FLAG_CB_ISSUER_CHECK’ basically translates into your Python interpreter telling you, “You told me to find X509_V_FLAG_CB_ISSUER_CHECK in the lib module, but I just couldn’t find it!” So get to examining our warehouse (module) and the goods (methods or attributes) being stored there carefully to ensure everything matches up correctly before moving forward.The `X509_V_FLAG_CB_ISSUER_CHECK` attribute often generates the error message “AttributeError: Module ‘lib’ has no attribute ‘X509_V_FLAG_CB_ISSUER_CHECK.'” In Python, specifically when dealing with cryptographic libraries such as OpenSSL.
Such a problem is primarily from one of two potential sources:
– An outdated version of the Python library PyOpenSSL
– An environment issue involving incorrect system paths.
Optimizing for search engines, this answer will guide you on how to validate your OpenSSL installation and highlight the importance of ensuring that all related software packages (Python, OpenSSL, etc.) are properly installed and updated.
PyOpenSSL and OpenSSL:
You should first check your OpenSSL and PyOpenSSL versions using command line:
openssl version # check openssl version python -m pip show cryptography pyOpenSSL # check PyOpenSSL version
If you’re using an outdated version of PyOpenSSL, I suggest updating it to a newer version which may resolve the AttributeError issue:
pip install --upgrade pyOpenSSL
To explain, PyOpenSSL is a Python wrapper for OpenSSL and is designed to expose most of OpenSSL’s functionality while remaining compatible with the Python standard library. Basically, the `X509_V_FLAG_CB_ISSUER_CHECK` attribute is tied to a specific functionality within OpenSSL. However, if PyOpenSSL isn’t up to date, this could result in error messages like the one mentioned earlier.
System Paths:
If OpenSSL and PyOpenSSL aren’t causing the issue, you might be experiencing an environment problem. A common root cause of this error could be due to the location of the OpenSSL library files. The system might not be able to find these libraries resulting in the observed attribute error.
In Linux systems, you may have to add OpenSSL’s library path to the LD_LIBRARY_PATH environment variable.
Let’s assume the OpenSSL library files are at /usr/local/lib. You can modify the LD_LIBRARY_PATH environment variable using these commands:
export LD_LIBRARY_PATH=$LD_LIBRARY_PATH:/usr/local/lib sudo ldconfig
Just replace “/usr/local/lib” in the code above to match your actual OpenSSL installation directory.
Understanding the Error Message:
The error “AttributeError: Module ‘lib’ has no attribute ‘X509_V_FLAG_CB_ISSUER_CHECK'” indicates that the Python interpreter cannot locate the attribute named `X509_V_FLAG_CB_ISSUER_CHECK`. This can come up whenever your Python interpreter doesn’t have access to that specific attribute within the required library – a sign that either the library or your path setup is faulty.
Resolving such AttributeErrors often involves correctly installing all relevant packages within the correct directories. For example, you should ensure that all necessary OpenSSL library files are reachable by the operating system and that PyOpenSSL can effectively communicate with the underlying OpenSSL library. This verifies that Python scripts utilising OpenSSL’s capabilities are directed to the right place and have access to the correct resources.
References:
1. PyOpenSSL’s official documentation
2. StackOverflow discussion on checking PyOpenSSL and OpenSSL versionsDiving into Python’s X509 Verification Flags, we understand that they are associated with the certificate validation process. These flags help in setting specific constraints during the validation, ensuring awareness of any discrepancies that may arise when interacting with SSL connections.
Specifically addressing your issue,
AttributeError: module 'lib' has no attribute 'X509_V_FLAG_CB_ISSUER_CHECK'
, it is indicative of a problem in the OpenSSL lib used for SSL and cryptography functions in Python. You’re attempting to access a specified attribute,
'X509_V_FLAG_CB_ISSUER_CHECK'
, which doesn’t appear to exist in the referenced library module – ‘lib’.
This particular flag:
'X509_V_FLAG_CB_ISSUER_CHECK'
is not defined in Python’s OpenSSL binding `cryptography` package that provides cryptographic recipes and primitives to Python developers. Hence, this attribute assignment seems to be raising an exception.
Digging deeper, let’s analyze how we can troubleshoot and dissect such problems:
• Firstly, always verify the installed versions of Python and ‘cryptography’ packages you are using. Make sure they’re updated to the latest stable release. This will ensure that you’re on track with the developer’s security patches and new bindings if introduced.
import sys from cryptography import __version__ as crypto_version print("Python Version:", sys.version) print("Cryptography Package Version:", crypto_version)
To install or upgrade ‘cryptography’, use:
pip install --upgrade cryptography
• If updating didn’t resolve the issue, reassess the code. Ensure that references to
'X509_V_FLAG_CB_ISSUER_CHECK'
is critical. It might be worth considering alternatives given some flags sometimes have very specific use cases and might not be necessary for general scenarios.
In case you’re interested in custom issuer checks, create a validation callback function and register it with the context. Here’s how to implement it:
import OpenSSL def verify_cb(conn, cert, errnum, depth, ok): # Custom Certificate Issuer check # If False is returned, the certificate verification fails return ok ctx = OpenSSL.SSL.Context(OpenSSL.SSL.TLSv1_METHOD) ctx.set_verify(OpenSSL.SSL.VERIFY_PEER|OpenSSL.SSL.VERIFY_FAIL_IF_NO_PEER_CERT, verify_cb)
In this snippet, a custom callback function ‘verify_cb’ performs your certificate issuer checks while returning a boolean status of the validation.
• Going through similar questions on respected forums like [StackOverflow](https://stackoverflow.com/) can give insights about alternative solutions or workarounds suggested by other developers from the Python community.
• The [official OpenSSL documentation](https://www.openssl.org/docs/manmaster/man3/X509_VERIFY_PARAM_set_flags.html) serves as an excellent resource for understanding the scope and purpose of these X509 Verification Flags.
Errors involving libraries and packages have their foundations lying in package management, version control, or inherent limitations of a method. Thorough investigation helps pinpoint the source of issues efficiently.Handling errors involving Python’s ‘lib’ module can certainly be a formidable task, particularly when you encounter the “AttributeError: module ‘lib’ has no attribute ‘X509_V_FLAG_CB_ISSUER_CHECK'” issue. To truly understand this error and devise detailed strategies to tackle it, let’s first take a closer look at what this particular error implies.
The “AttributeError” in Python occurs when you attempt to use a non-existent attribute for a module, class, instance, or any other object that does not have that attribute. In this case, ‘X509_V_FLAG_CB_ISSUER_CHECK’ is the attribute that Python cannot find in the ‘lib’ module. The presence of such an error generally signals that the attribute is either missing from the ‘lib’ module or is incorrectly called.
Before we proceed to the fix, note that ‘X509_V_FLAG_CB_ISSUER_CHECK’ largely stems from the OpenSSL library in Python (more specifically, the
ssl
built-in Python module) – meaning it’s closely associated with SSL/TLS connections which primarily involve secure web communications.
Let’s explore several probable solutions to rectify “AttributeError: module ‘lib’ has no attribute ‘X509_V_FLAG_CB_ISSUER_CHECK'”.
Proper Installation and Verification of OpenSSL
Firstly, ensure that the ‘OpenSSL’ library is correctly installed in your environment.
To check if OpenSSL is correctly installed, execute the following command in your terminal.
$ openssl version -a
If OpenSSL is properly installed, this command would return information about its version, built date, etc. If not, you’d need to install it using:
For Ubuntu:
$ sudo apt-get install openssl libssl-dev
For CentOS/RHEL:
$ sudo yum install openssl openssl-devel
Using the Right Python OpenSSL binding module
You might also be using an old or incompatible Python OpenSSL binding module which does not have the ‘X509_V_FLAG_CB_ISSUER_CHECK’ feature. Python provides a number of modules as OpenSSL bindings like pyOpenSSL (source), but, in your case, I recommend using Python’s built-in
ssl
module.
As an additional measure, ensure that your Python version itself is up-to-date. An outdated Python version may lack the necessary built-in library features to handle SSL/TLS connections.
Ensuring Correct Attribute Names are Used
In some cases, your problem might be as simple as a typo. Ensure that the attribute name is spelled correctly and exists in the specific ‘lib’ module that you’re trying to import it from. You can usually verify this by examining the official Python library documentation or source code of the module.
Finally, remember that when dealing with libraries or packages that aren’t built directly into Python, always cross-verify that these resources are updated and fully supported. Outdated resources or those no longer maintained are often prone to compatibility issues or bugs that lead to these unexpected errors.The error message ‘
AttributeError: module 'lib' has no attribute 'X509_V_FLAG_CB_ISSUER_CHECK'
‘ is an indication that the Python code you’re trying to run is accessing an attribute of the
lib
module named
X509_V_FLAG_CB_ISSUER_CHECK
which doesn’t exist. This is most likely happening in a context where SSL/TLS certificates are being handled using OpenSSL, and this flag specifically is expected to be used as an option in verification procedures.
Unfortunately, if we take a closer look into the official Python documentation we won’t find
X509_V_FLAG_CB_ISSUER_CHECK
. Could the absence of
X509_V_FLAG_CB_ISSUER_CHECK
cause this error? Very likely! As part of the OpenSSL library, it should act as triggers for specific certificate verification callbacks, but seems not to be available under the lib used by Python or your current environment.
This problem could be caused by:
- Your current environment is having an older version of OpenSSL which doesn’t support that flag.
- This flag isn’t implemented yet in the OpenSSL binding/libraries utilized by Python, such as pyOpenSSL or cryptography. If it is not defined there, it is not accessible for Python language to use.
You can determine the actual cause this way:
Use
print(lib.OPENSSL_VERSION)
to print out your OpenSSL version for the current environment.
Also, check the source code of the module lib you’re importing to see if
X509_V_FLAG_CB_ISSUER_CHECK
is actually defined.
If you need to use this or other advanced characteristics from OpenSSL not incorporated in built-in Python features, you can consider encapsulating C-based libraries with functions in Python. Another viable option includes looking for third-party alternatives adjusting to these specific requirements.
Here’s an example of how to utilize ctypes to wrap this flag from OpenSSL:
from ctypes import cdll, c_long lib = cdll.LoadLibrary('libssl.so') # load OpenSSL shared library X509_V_FLAG_CB_ISSUER_CHECK = c_long(0x20).value # Define the flag
Remember to replace `’libssl.so’` with the path to your OpenSSL dll file in case it’s located at a different location on your machine.
In-depth discussions regarding OpenSSL and its Python bindings can also be beneficial for better understanding of the left-out attributes and their working solutions, like this thread on StackOverflow.
Bear in mind though, careful consideration is needed when dealing with SSL/TLS certificates due to the critical security implications involved. Ensure to follow best-practices for handling cryptographic material.
Lib attributes in Python are made accessible via import statements, which allows you to utilize various functions and classes defined in the module. When working with a lib attribute such as
X509_V_FLAG_CB_ISSUER_CHECK
, it is crucial to remember that this particular attribute belongs to the OpenSSL library, not the lib module. Hence, if you try to import this from lib, the compiler will raise an ‘AttributeError,’ stating that the ‘lib’ module doesn’t have an Attribute named ‘X509_V_FLAG_CB_ISSUER_CHECK’.
The error message looks something like this:
AttributeError: module 'lib' has no attribute 'X509_V_FLAG_CB_ISSUER_CHECK'
This error arises because of trying to access an Attribute from a module where it does not exist. The reason being, your reference is aimed at the wrong module.
To work around this issue, you need to amend your import statement to fetch this particular attribute from the correct library, which in this case is OpenSSL. Here’s how you can do it using Python’s handy ‘import’ function:
from OpenSSL.crypto import X509_V_FLAG_CB_ISSUER_CHECK
What we’re doing here is importing the specific attribute (
X509_V_FLAG_CB_ISSUER_CHECK
) directly from the
OpenSSL.crypto
namespace where it lives, ensuring that we’re accessing the right resource from its appropriate source.
Once you’ve corrected your import statement, you can harness the power of SSL and X509 flag for issuer checks in your Python script without much hassle.
For more information on lib attributes and their uses in Python, I’d recommend checking out the official documentation of Python’s standard libraries [source] and OpenSSL library documentation [source], which provide comprehensive insights into these resources and their proper utilization.
When you find the error message
AttributeError: Module 'Lib' has no attribute 'X509_V_FLAG_CB_ISSUER_CHECK'
, it means that the Python program is trying to access a module or function in a library that doesn’t exist. This might be caused by different reasons, but mostly it’s traced back to a lack of proper installation or incorrect usage.
Incorrect Usage
It could happen due to incorrect usage. You might be trying to import a submodule or function directly from the parent module, which does not have such an attribute. In order to solve this issue, you first need to ensure that you’re using the correct import statement. If you were trying to use
X509_V_FLAG_CB_ISSUER_CHECK
like this:
from OpenSSL import crypto print(crypto.X509_V_FLAG_CB_ISSUER_CHECK)
That won’t work because
X509_V_FLAG_CB_ISSUER_CHECK
would not be found under the openssl.crypto module. Ensure that you are importing the correct method and object names when working with a library.
Module Not Installed
The error could also mean that the necessary libraries aren’t properly installed on your Python setup. Make sure to install the required libraries using the pip package manager like so:
pip install pyOpenSSL cryptography
Old Library Version
You may also face this sort of issue if you are using an old version of the lib module which doesn’t include the
'X509_V_FLAG_CB_ISSUER_CHECK'
attribute. You should update it by running:
pip install -U pyOpenSSL cryptography
This command will upgrade the installed version of PyOpenSSL and cryptography, including its dependencies.
It should be noted that running pip commands should be done within your project’s virtual environment (if one exists) to prevent conflicts with other projects or system-wide settings.
Re-importing the Module
Python tries to optimize resources by avoiding reloading modules that have already been imported. If you made changes and want them to reflect, you’ll need to restart the Python interpreter or force Python to re-import the library. One possible option to force a re-import could look like this:
import importlib importlib.reload(module_name)
Replace
module_name
with the name of the module you want to reload.
Environment Variables
Make sure the OS can find the modules. Sometimes, you might run Python in an environment where it cannot locate files and packages even though they are installed. You should check and adjust PATH variables on your operating system accordingly.
In case none of the above solutions resolve your problem, try checking the issue tracker for the library on its official GitHub repository or their community forum, as the error might be related to a known bug.
Diving into the crux of
AttributeError: Module 'Lib' has no attribute 'X509_V_FLAG_CB_ISSUER_CHECK'
, it represents an error message that Python developers commonly encounter. This error signifies that the Python interpreter is attempting to access an attribute or method on a module named ‘Lib’, which doesn’t possess the ‘X509_V_FLAG_CB_ISSUER_CHECK’ attribute.
Reflecting deeply on this, such errors generally surface when there is an attempt to access attributes or components that do not exist. There might be multiple reasons like:
- A typo in the attribute name.
- The required libraries aren’t imported properly.
- An outdated version of a library that does not support certain functionality.
Nevertheless, mitigating this hindrance calls for practical steps. Start by confirming the existence and continuity of the attribute you are trying to access. If the attribute exists in an external library:
- Examine if the library is up-to-date.
- Check its importation status; make sure import statements are correct.
Here is a simple example that gives an idea of the situation, considering ‘Lib’ as numpy module and ‘non_existent_attr’ as your X509_V_FLAG_CB_ISSUER_CHECK:
import numpy as Lib print(Lib.non_existent_attr)
Running this code will generate the same kind of
AttributeError
because ‘numpy’ does not have an attribute called ‘non_existent_attr’.
It’s crucial to bear in mind that attributive errors are part and parcel of coding in Python. As a twist to the usual approach, acknowledging the familiarity with essential modules and recurrent updating practices plays a key role to ensure smooth executions without hitches. Investing time to comprehend the anatomy of Python’s AttributeError and the skillful means to debug them can decidedly progress problem-solving efficiency in Python programming. Take a moment to delve into Python’s official documentation1 to learn more about attribute errors.
Through robust observation, meticulous debugging, acquaintance with Python’s libraries, and proper updation proficiency, navigating through such errors become less daunting and more of an expedition. Even though such roadblocks seem hindering, remember that every professional coder has been through this path. It’s all part of the journey!
Coding is not just about solving problems, but identifying them, structuring them, and systematically resolving them. So the next time when programming throws at you an
AttributeError
, rather than getting perplexed, scrutinize it. Every error is a step towards becoming a great coder!