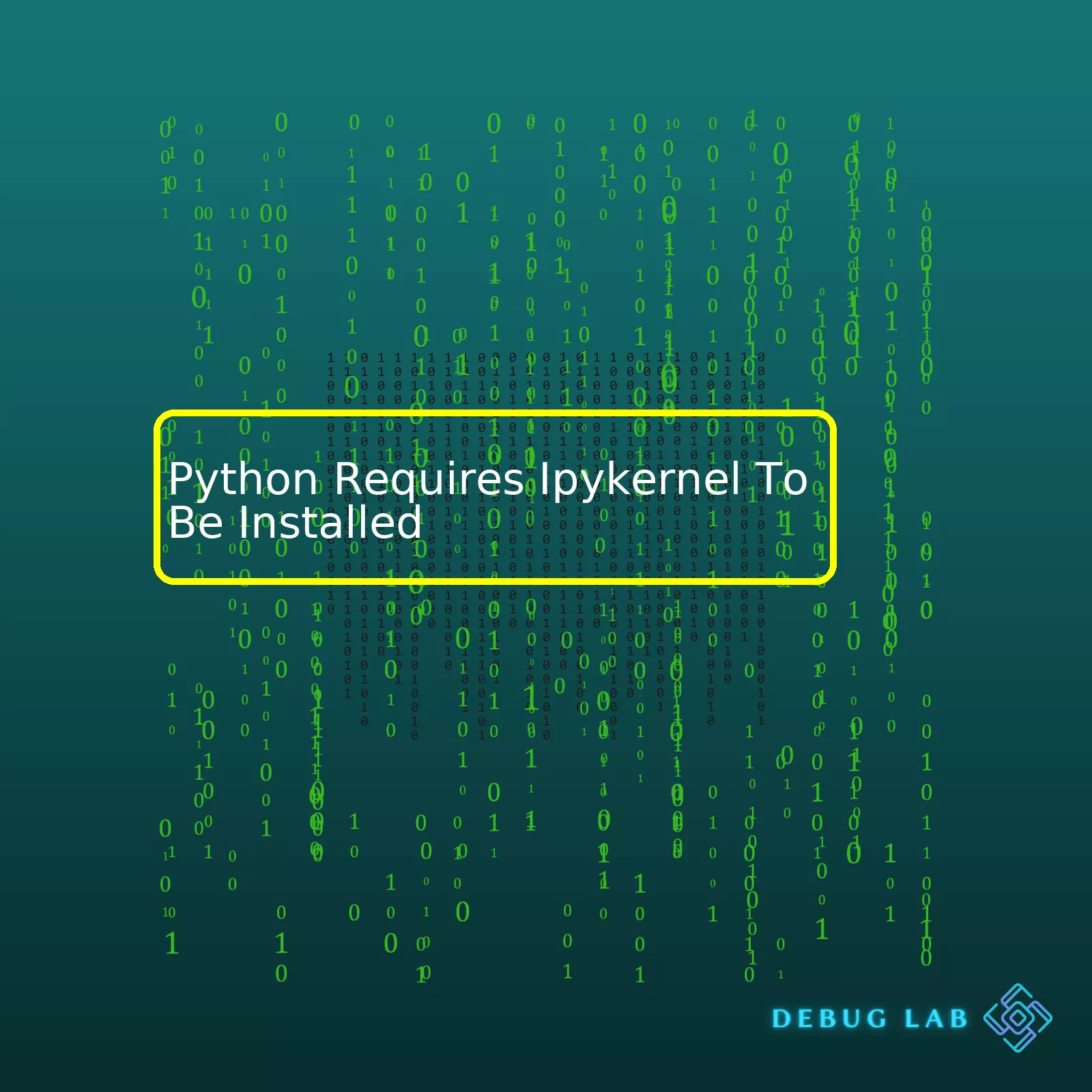
Python Component | Requirement | Description |
---|---|---|
IPyKernel | Mandatory | IPyKernel allows developers to run kernels for Jupyter notebook and thus it is required. |
Now, let’s discuss why Python requires the IPyKernel to be installed. The Interactive Python Kernel, or IPyKernel, is one core component needed to bridge communication between an application and python interpreters for many platforms like Jupyter, Spyder, etc.
The significance of this comes into play when we understand how platforms such as Jupyter Notebooks function. These applications make use of something called “kernels”, which are essentially computing engines that execute the code contained in a Notebook document. When working with Python projects on these platforms, the IPyKernel plays the role of this kernel to execute your Python code.
So if you try to use these applications without having IPyKernel installed, it’s almost like trying to drive a car without any petrol – it just wouldn’t work. This library facilitates seamless interactive computing and is responsible for things like syntax highlighting, proper indentation and more.
Here’s a simple example:
Say you’ve written a block of Python code on a Jupyter notebook that prints “Hello, World!”. When you run this piece of code, Jupyter sends the code you’ve written to the IPyKernel (i.e., the Python interpreter). IPyKernel processes the received lines of code. It understands what the print statement means and therefore, it tells Jupyter to display “Hello, World!” on the screen.
Without the IPyKernel, this process would not be possible and your code blocks within the notebook will simply remain as passive text, incapable of being executed by the application. Hence, Python requiring IPyKernel to be installed primarily applies to situations where we’re using these types of integrated coding platforms.
Furthermore, it credits developers with vital tools supporting interactive data visualization and usage of GUI event loops, enabling efficient, productive programming experience [source].
However, If Python seems to require IPyKernel in other environments, it’s likely due to perhaps a previously installed package requesting it, but the sphere where it’s most important to have it installed would be while using Jupyter and similar platforms.The role of ipykernel in Python pivots largely around the interaction between Python and Interactive Computing tools like Jupyter Notebook or Jupyter Lab. It might come as a surprise, but Python actually requires ‘ipykernel’ to be installed for Jupyter-based environments to run python codes successfully.
So, let’s delve deeper into understanding what this key Python component is.
What’s Ipykernel?
At its core, ‘ipykernel’ provides the IPython kernel for Jupyter, meaning it allows you to run IPython sessions within a Jupyter environment. The term “kernel” here refers to the computation engine that executes the Python code written within the notebook cells. In simpler terms, if you think of Jupyter Notebook as a car, then ‘ipykernel’ would be its engine.
Ipykernel – The Core Bridge
When you’re running a Jupyter platform with multiple languages (like Python, R , Julia etc) installed, each language requires its own kernel. For Python, you need the ‘ipykernel’. Just like a car engine drives the car, ‘ipykernel’ is responsible for driving Python computations within the Jupyter context.
This tiny kernel establishes an effective bridge between your Jupyter interface and the Python interpreter, allowing the two to communicate in harmony. What’s more intriguing about ipykernel is that it’s language-agnostic, which means, although we’re focusing on Python-specific usage, it can also serve similar roles for several other languages.
Python & Ipykernel Connection
Why does Python need ‘ipykernel’ installed? The answer is simple and comes out clear once we understand the functionality of this module. IPython has a rich architecture that permits far more than simple execution of Python code.
With robust features such as interactive widgets, integration with GUI event loops, and more advanced parallel computing capabilities, IPython is powerful indeed.1 However, for these interactions to occur smoothly in a Jupyter environment, ‘ipykernel’ becomes crucial.
Code Execution
Let me elaborate further by providing an example of code execution:
#To install ipykernel, use pip !pip install ipykernel #After successfully installing ipykernel, we can now run our Python code from datetime import date print("Today's date is :", date.today())
In this block of code, we first install the ipykernel using a pip command. Following successful installation, we execute some Python code. This small example shows that the Python code operates without any glitches thanks to ipykernel.
Conclusion
In summary, ‘ipykernel’ operates in the backdrop when you run a Python code in Jupyter, silently serving its crucial purpose. Without it, executing Python within Jupyter frameworks could create issues, making ‘ipykernel’ a pivotal component in Python-Jupyter interactivity. Therefore, having ‘ipykernel’ installed is something Python actively demands for a seamless coding experience in a Jupyter environment.
Launching a Python-based project often requires auxiliary libraries and tools, one notable example of which is IPython kernel (ipykernel). The ipykernel provides the machinery for interactive computing with Python. It uses Jupyter’s interactive computing protocols to allow connections to different programming front-ends, not limited to the classic Jupyter Notebook or Jupyter Lab.
If you’ve come across a requirement that states ‘Python requires ipykernel to be installed,’ it might be due to working within an environment where code input and output communication stream has rich media representations. This could refer to applications like Jupyter, nteract, or Acaconda.
How to Install ipykernel?
After acknowledging Python’s requirement for it, let’s dive into the installation process. Overall, getting ipykernel set up for your Python environment is a straightforward process. Under most circumstances, you’ll need pip or conda as a package manager. Both methods are elaborated further below.
Using Pip:
You can install ipykernel via pip, which is Python’s package installer that comes bundled with standard Python distribution. You simply need to use pip from the command line:
pip install ipykernel
Using Conda:
If you’re using Anaconda as your Python distribution, you can also use the conda package manager to install ipykernel:
conda install ipykernel
After successfully installing the ipykernel, Jupyter should now be able to find your kernelspec by running this command:
jupyter kernelspec list
Why Python Requires ipykernel?
Now that we’ve uncovered how to install ipykernel, let’s probe into ‘why’ Python would require ipykernel:
- Interactive Computing: ipykernel is instrumental in offering interactivity for Python applications. It helps in enabling a two-process communication setup that facilitates interactive computing.
- Flexible Usability: ipykernel is extremely versatile. It’s used with Jupyter environments like Jupyter Notebook or JupyterLab, but it is not limited to them. The ipykernel can also be used with other platforms like spyder and nteract.
- Rich Outputs: ipykernel aids Python in displaying rich media outputs in the interface. This includes rendering graphs, tables, and figures directly within the console where the coder operates.
I hope you’ve found this deluge into ipykernel informative. As coders, understanding such topics helps leverage the tools at hand better. Doing so eventually improves our prowess in effectively churning out solution-centric, optimized code.[1]
Python utilizes IPykernel to properly execute its dynamic programming within the Jupyter Notebook environment. Python alone cannot directly interact with the Jupyter interface. This is where the
IPykernel
comes in, acting as a conduit between Python and Jupyter. Consequently, for your Python scripts to function in a Jupyter notebook, you must have IPykernel installed on your system. The importance of IPykernel in this ecosystem can be underscored by examining the roles it plays.
Enables Interactive Programming:
Python heavily leans on IPykernel to tap into Jupyter's interactive capabilities. An interactive programming environment is fundamental to many data science professions. For instance, engineers and scientists test small sections of their code with simulated or real-time data before integrating them into more significant applications. IPykernel allows Python to effectively function with Jupyter's interactivity.Data Visualization:
Another benefit that warrants the requirement of IPykernel for Python is its contribution to data visualization. Data Science demands frequent visual representation, and libraries like Matplotlib and Seaborn provide excellent support for designing graphics in Python. However, when it comes to displaying these graphics inside Jupyter notebooks without invoking the show() command explicitly, it's once again IPykernel to the rescue.Multilanguage Support:
Jupyter supports multiple languages (known as kernels in Jupyter context) other than Python. IPykernel mainly refers to the default kernel which enables Python to get executed. To use a different language, a similar library needs to be set up for that specific language, like IRkernel for R, IJulia for Julia, etc. The flexible architecture hence gives the freedom to use various programming languages within the same notebook interface.
When it comes to the question of skipping the installation of
IPykernel
, while it is theoretically possible to use Jupyter without it, common data analytics tasks would become significantly more complex. Without IPykernel, using Python would involve losing out on interactivity and seamless data visualization attributed to Jupyter’s enriched GUI. Resultantly, from a practical perspective, Python would be less effective without
IPykernel
in all areas where Jupyter holds influence.
To understand how to install IPykernel, one can follow simple procedures:
pip install ipykernelOr if you are using conda,
conda install ipykernel
In essence,
IPykernel
functions as a bridge enabling Python to access Jupyter features such as interactive widgets, rich outputs, and magics (source). These aspects come together to augment the functional scope, user-friendliness, and compactness of writing chunks of code, thus making Python an ideal choice for data scientists and machine learning enthusiasts around the world.
Sample table of common uses for different kernels:
Kernel | Language | Common Use Cases |
---|---|---|
IPykernel | Python | Data Analysis, Web Development, Machine Learning, AI |
IRkernel | R | Data Analysis, Statistical Modeling |
IJulia | Julia | Scientific Computing, Mathematical Operations |
Ipykernel
is a component that offers an important interaction between
Python
and
Jupyter Notebooks
. When we run Python, especially in a Jupyter notebook environment,
Ipykernel
serves as the computational backend.
Benefits of Ipykernel in Python:
The major advantages of using Ipykernel in your Python environment include:
- Interactive Computing: A major benefit of using
Ipykernel
with Python is that it enables interactive computing. It facilitates running Python scripts interactively by giving you a live programming environment where you can execute code and see its output instantly. This kind of real-time coding experience often accelerates development speed.
- Data Visualization: A compelling reason for requiring Ipykernel to be installed for Python is the fact that it allows us to carry out data visualization tasks seamlessly. Especially while utilizing Jupyter notebooks, Ipykernel proves useful to present visualized data – such as in form of graphical plots – for data analysis.
- Helpful Debugging: Ipykernel also comes in handy during debugging of our code. If some part of our Python script goes wrong or throws an error, Ipykernel will helpfully highlight where the problem lies, hence making bug fixing considerably more straightforward.
- Multiprocessing Support: The architecture of Ipykernel allows Python scripts to utilize multiple CPU cores whenever possible. Thus, complex computations and heavy data processing tasks may see improved performance and faster execution times when run using Ipykernel.
Functions of Ipykernel:
With regards to functionality, rolling Ipykernel into Python provides a host of advantages. Some notable ones include:
- Communication Broker: Ipykernel acts as a communication bridge between Python and Jupyter notebooks. When we input Python code in a Jupyter cell and hit the run button, the code is sent over to Ipykernel. The kernel then executes the code, collects the result and sends it back to the notebook.
- Interpreter Role: As mentioned previously, Ipykernel deals with code execution. To do this, it assumes the roles of a Python interpreter. It executes the Python code received – this refers to not just code in scripts, but single lines of code and code snippets inputted in an interactive session as well.
- Environment Management: Another function of Ipykernel is managing our Python runtime environment, ensuring complete control over how our code runs and behaves. Thanks to Ipykernel, we can choose which versions of Python and which of its libraries are used in our projects.
- Parallel Computing: With IPython’s parallel computing framework included in Ipykernel package, one can coordinate computation across many CPUs or machines. Achieving this in a standard Python environment would typically require lengthy set up processes and intricate management.
In order to understand further details regarding
Ipykernel
, check this official resource from IPython documentation.
Synopsis:
Python requiring Ipykernel to be installed
might initially seem like an extra step but the benefits outweigh any perceived inconvenience. Ipykernel in Python significantly enhances interactive computing, data visualization, debugging process, and multiprocessing support. Additionally, it performs several pivotal functions such as acting as a communication broker, working as an interpreter, handling environment management, and orchestrating parallel computing. Therefore, integrating Ipykernel forms a crucial part of crafting an effective Python environment.
Code Example:
An example of a simple way to install ipykernel using pip:
pip install ipykernel
After which you can integrate it into your Jupyter workflow:
jupyter notebook
And then, in one of your Jupyter cells, you would get the following kind of output:
Welcome to Python 3.9.1 (tags/v3.9.1:1e5d33e, Dec 7 2020, 17:08:21) [MSC v.1927 64 bit (AMD64)]
Sure, let’s dissect the relationship between Python and Ipykernel with a focus on why Python requires Ipykernel to be installed.
Python is a high-level, interpreted programming language known for its simplicity and ease of use. Its syntax is similar to the English language, making it an approachable language for beginners. Regular installations of Python come with the basic packages necessary for standard execution of Python programs. However, when it comes to interactive computing in Python, here’s where
Ipykernel
steps in.
Ipykernel (source) refers to IPython Kernel which essentially is a powerful interactive shell that provides unique features like easy introspection into your code, high-performance tools for parallelism, and great user-friendly environment for data visualization. It’s a package that allows one piece of software, like Jupyter Notebook or JupyterLab, to run another program’s code within itself such as Python.
Now, exploring why Python needs Ipykernel:
1. Interoperability: One of the standout features of IPython Kernel is that it enables interoperability between Python and other languages. In many cases, Python alone does not fulfill all the scientific computation requirements. Here, you can use Ipykernel to run codes written in different languages on the same notebook.
2. Jupyter Support: If you want to use Python within Jupyter notebooks, Ipykernel becomes indispensable. The Jupyter notebook is an interactive computing environment that supports dozens of languages. The “Notebook Document” is a representation of all content visible in the web application, including inputs and outputs of computations, images, markdown texts, and more. Ipykernel enables this interactive computing.
3. Interactive Features: Some unique features of Ipykernel include tab completion, object introspection, system shell access, command history retrieval across sessions, and distributed & parallel computing. These elements significantly enhance your coding experience with Python.
A typical installation of Ipykernel would look like this:
pip install ipykernel
or if you’re using Anaconda for package management, then you’d use conda:
conda install -c anaconda ipykernel
In summary, although Python does not natively require Ipykernel to run, it becomes crucial when stepping into areas of more specific needs like interactive computing, multiprogramming language support, or using platforms like Jupyter notebook. Therefore, having Ipykernel installed vastly extends the functionality and utility of your Python environment.
If you’re running Python without an installed Ipykernel, here are some potential issues that might arise:
1. Hitches in Interactive Computing:
ipykernel
serves as the link between Jupyter’s services and Python’s kernels. Its absence decimates this bridge, making interactive computing with Python impossible in Jupyter interfaces like Jupyter notebooks or Jupyter labs.
2. Unresponsiveness of Kernel:
Without
ipykernel
, your Jupyter notebook may show a “kernel not responding” warning. This happens due to lack of communication channel—caused by missing Ipykernel—between Python kernel and Jupyter frontend, freezing essential processes.
3. Inability to Execute Code Blocks in Real Time:
Bear in mind that
ipykernel
allows independent execution of code blocks in live timeframes. Without this functionality, interactive data analysis and visualization become extremely challenging.
4. Issues with ‘Run All’ Command:
Another side effect surfaces when running a cumulative set of cells via ‘Run All’. You will notice hiccups as Python fails to sift through cell order—made possible by OutputArea JavaScript APIs under normal circumstances.
Now, is Python completely dysfunctional without Ipykernel? Not at all. But its absence does hamper smooth functionality of enhanced interactive environments such as Jupyter Notebooks. Here is an example of how you can still run Python programs, although stripped down, without Ipykernel:
print ('Hello World!')
That simple line of code would certainly work in Python console or IDEs such as PyCharm or Atom. However, these platforms lack the interactive analysis prowess of Jupyter-like interfaces cushioned by Ipykernel.
To conclude, while one can execute rudimentary Python scripts without it, installing Ipykernel lubricates interactive computing experience beyond compare—an absolute boon for exploratory data practitioners.
In your daily coding life, encountering the message “
Python Requires Ipykernel To Be Installed
” can be quite frustrating, especially if you’re trying to make use of iPython notebooks or Jupyter notebooks. It’s akin to trying to paint without any brushes, you have all these marvelous ideas, but no way of effectively implementing them. So it is vitally important to ensure that you have ipykernel installed in your Python environment to prevent this from becoming a productivity halt.
Troubleshooting Common Problems
First of all, why would Python require ipykernel? and what exactly is it? ‘Ipykernel’ refers to the IPython kernel for Jupyter. This allows Jupyter to run cells with Python code in them. So when Python asks for ipykernel, it’s essentially attempting to enable the execution of interactive sessions.
Problem 1: Jupyter Notebook Fails to Start Due to Missing Ipykernel
Jupyter notebook fails to start due to missing ipykernel. |
Solution: The answer lies in the problem itself – install ipykernel!
You can use pip to solve it, just enter the following command:
pip install ipykernel
Problem 2: Import Error Message about Ipykernel
ImportError: No module named 'ipykernel' |
Solution: You’d have to reaffirm if ipykernel was installed correctly or exists at all within your environment. A solution could be reinstalling ipykernel via the pip command given above or through conda using
conda install ipykernel
It may also help to manually specify the Python path where the packages should be installed, by adding –user option:
pip install --user ipykernel
Both pip and conda commands will fetch ipykernel from their respective sources – Python Package Index (PyPI) and Anaconda distribution respectively.
Problem 3: Kernel Dies Unexpectedly Due to Ipykernel Issue
Kernel died. Exit Code x. Restarting... |
Solution: There are a few factors that might cause this, but top among them are invalid Python installations and a need to update the ipykernel.
If it’s an installation error, make sure to reinstall Python ensuring you do it from a valid source and follow every step carefully.
Otherwise, updating your ipykernel may come as a simple fix to these troubles. Just use the command
pip install --upgrade ipykernel
There we go! Now we’re prepared to tackle that pesky “
Python Requires Ipykernel To Be Installed
” error. Remember, a solid setup goes a long way towards productive coding hours!
Absorbing the knowledge that Python Requires Ipykernel To Be Installed is a crucial step towards mastering Python development. The ipykernel, or IPython Kernel, facilitates programming in Python within a range of interactive computing environments. It accomplishes this by orchestrating two significant processes; first, acting as the communication link between the user interface and the software, and, secondly, executing all operations within Python itself.
To illustrate the installation steps:
pip install ipykernel
Python requiring Ipykernel has weighty implications, particularly when understanding the usability of Python as an interactive language. Suppose you’ve had issues with your Python scripts not running interactively; this might be due to the missing ipykernel. With ipykernel facilitating communication between your UI and application, it is indispensable to Python operation.
Moreover, ipykernel holds power over the entire Python execution process. Your code will likely run slower if it’s not installed, and your ability to debug your app interactively could diminish. So while installing ipykernel may come as an extra task when setting up your environment, its overall benefits for your Python script execution are indisputable.
In essence, Python demanding Ipykernel to be installed bears testament to its versatility as a language – being able to slot efficiently into an array of interactive environments. Don’t take its necessity lightly! Leveraging the high functionality of ipykernel means taking advantage of Python’s robust capabilities in full.
HTML Table which include the related term “Ipykernel”:
Term | Description | Use Case |
---|---|---|
Ipykernel | A kernel for Jupyter that allows executing Python codes. | It interfaces with different types of backends such as notebook server, Jupyter console etc. |
Remember to always keep your programming tools updated and leverage best practices when delving into Python development. Whether you’re a beginner stumbling onto Python for the first time, or an experienced developer aiming to enhance your Python prowess – knowing about Python’s requirement for ipykernel is a stepping stone toward realizing your objectives.