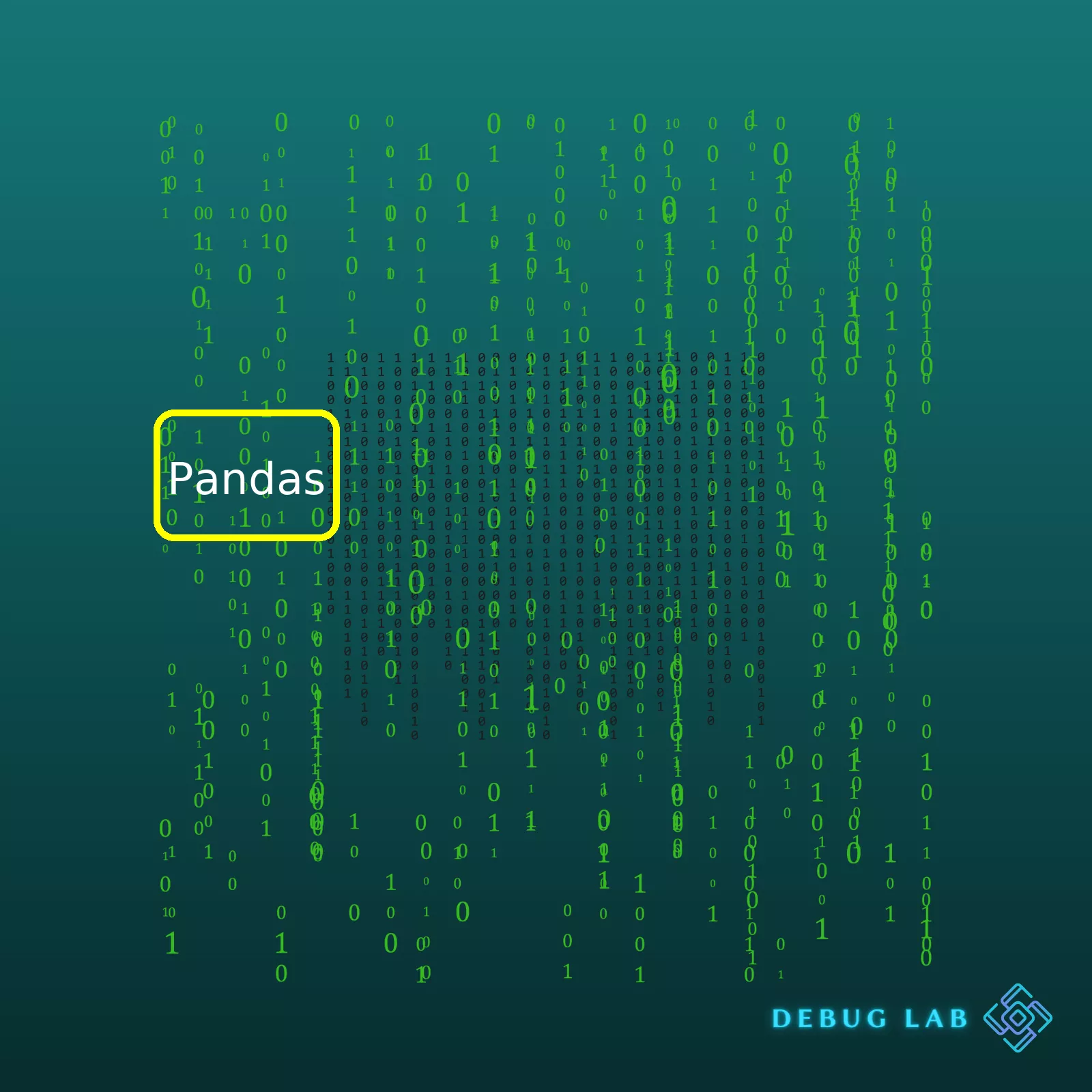
Function | Description |
---|---|
DataFrame() |
Two-dimensional, size-mutable, potentially heterogeneous tabular data. |
Series() |
One-dimensional ndarray with axis labels. |
read_csv() |
Read a comma-separated values (csv) file into DataFrame. |
describe() |
Generate descriptive statistics of DataFrame or Series. |
head() |
Return the first n rows. |
tail() |
Return the last n rows. |
merge() |
Merge DataFrame objects by performing a database-style join operation by columns or indexes. |
The above table demonstrates some core functions you’ll encounter in the Pandas library. For instance, the
DataFrame()
function lets you create a two-dimensional, heterogeneously-typed data structure wherein you can store data with columns that can be different types, like integers and strings. The
Series()
function acts similarly but is one-dimensional, meaning it’s perfect for single column data.
For handling CSV files, the
read_csv()
function does exactly what you’d expect—it reads your CSV files and translates them into a Dataframe. Interpreting and analyzing your loaded data gets easier with the
describe()
function which generates descriptive statistics of your Dataframe or Series, including mean, median, mode, and more.
To get a quick peep of your DataFrame, use
head()
to show the top n records, and
tail()
for the last n records. Lastly, if you need to combine multiple datasets into one comprehensive DataFrame,
merge()
can bring your separated datasets together with a database-style join operation.
Mastering these key functions can get you well on your way towards leveraging the full power of the Pandas library.Pandas, which stands for Python Data Analysis Library, is an open-source library providing high performance, easy-to-use data structures and data analysis tools. Pandas in official documentation. One of the great features of Pandas is its ability to parse a wide variety of data formats directly into DataFrame objects.
Pandas has two main data structures:
1. Series: A one-dimensional labeled array capable of holding any data type
2. DataFrame: Two-dimensional, size mutable and heterogeneous tabular data structure with both rows and columns labels.
You can create a series object with the following code snippet.
import pandas as pd s = pd.Series([1, 3, 5, np.nan, 6, 8]) print(s)
Dataframes are broadly used, and you can think of them like a spreadsheet or SQL table. Here’s a simple way to create a dataframe:
df = pd.DataFrame({ 'A': pd.Timestamp('20130102'), 'B': pd.Series(1, index=list(range(4)), dtype='float32'), 'C': pd.Series(1, index=list(range(4)), dtype='float64'), 'D': np.array([3] * 4, dtype='int32'), }) print(df)
Beyond just data manipulation, Pandas offers functionalities for handling missing data, merging datasets, reshaping datasets, aggregating or transforming data with a powerful group by feature, slicing, indexing, and subsetting large datasets. A typical example showing how pandas handle missing data is as follows:
# Assuming df is your DataFrame and it contains null values # To remove rows with NaN df_no_nan = df.dropna() # To replace NaNs with a standard value (in this case 0) df_nan_replaced = df.fillna(0)
For slicing, indexing, and subsetting of large datasets, please see the code snippet below:
df = pd.DataFrame(np.random.randn(10, 4)) # slice including upper and lower bounds df.loc[1:4] # using position df.iloc[1:4]
Note that while
df.loc[1:4]
includes the rows with indices from 1 to 4 inclusively,
df.iloc[1:4]
will include the rows from the first up to but not including the fourth row (i.e. indices 1, 2, and 3).Pandas is a powerful open-source data analysis tool in Python that provides flexible and dynamic data structures designed to make working with structured or relational datasets easy and intuitive. Here are some primary benefits of using Pandas for data analysis:
Efficient Data Handling
Pandas can ingest diverse types of data (like .csv, .json files, SQL databases), thereby offering compatibility with various formats. Additionally, it has
DataFrame
and
Series
objects that allow you to work with wide-range data: from simple 1-dimensional series to complex multi-dimensional datasets.
Effective Data Cleaning
With pandas, various tasks such as identifying and handling missing data, detecting outliers or changing data types become easier. Use methods like
dropna()
to get rid of rows containing null values or
fillna(value)
to fill missing values with a default value.
Data Manipulation
Pandas provides you with an array of tools for the manipulation of structured data. Using the
groupby()
,
merge()
, and
join()
functions, among others, helps aggregate and combine data efficiently.
Data Analysis
Pandas transforms raw, messy datasets into tidy and intuitive ones that are ready for visualization and analysis. With functionality to handle time-series operations, mathematical computations and summarizing data, it conveys meaningful insights from the data at hand.
Data Visualization
Though the pandas library doesn’t offer any data visualization capabilities by itself, it works well with Matplotlib and Seaborn to create visual interpretations of data. This aids in visually understanding patterns, trends, and correlations in your data.
Here’s an example of how you would load a CSV file using pandas and then proceed with cleaning and manipulating the dataframe:
import pandas as pd # Load the csv file df = pd.read_csv("file.csv") # Fill NA values df.fillna(0, inplace=True) # Groupby operation grouped_df = df.groupby('column_name') # Compute mean mean_df = grouped_df.mean()
On the other hand, take a look at this [link](https://pandas.pydata.org/docs/) for more examples and details about how you can utilize the extensive capabilities of pandas in your own data analysis.
Remember, harnessing the power of pandas can help tremendously in the effective interpretation of data, deriving valuable insights that can support evidence-based decision-making. The use of pandas can significantly streamline the data analysis process making it a must-have tool for any data scientist or analyst!Pandas is a proficient library in Python for data manipulation and analysis. It incorporates two primary data structures, Series (1-dimensional) and DataFrame (2-dimensional), to handle the vast majority of typical use-cases in finance, statistics, social sciences, and engineering.
The key functionalities of Pandas can be explored in five significant domains: Data Structure, Data Loading, Data Manipulation, Data Cleaning and Merging, and Data Visualization.
Data Structure
Pandas introduces two new data structures to Python –
Series
and
DataFrame
. Both are remarkably flexible to use.
According to Wes McKinney,
- Series: It is a one-dimensional labelled array adept at holding any type.
- DataFrame: A 2-dimensional labelled data structure with columns of potentially different types. You can think of it like a spreadsheet or a SQL table, or dictionary of Series objects.
Here’s an example of creating Series and DataFrames:
import pandas as pd # Creating pandas series ser = pd.Series([10, 20, 30, 40]) # Creating pandas dataframe df = pd.DataFrame({'A': pd.Series([1, 2, 3]), 'B': pd.Series([1.0, 2.0, 3.0])})
Data Loading
In order to run analysis on your data, it must first be loaded into the memory. Panda’s inbuilt functions allow you to load data from multiple file formats like csv, excel, json or even data scraped from a webpage directly into a DataFrame.
# Load csv format data_csv = pd.read_csv('file.csv') # Load excel format data_excel = pd.read_excel('file.xls') # Load json format data_json = pd.read_json('file.json')
Data Manipulation
Pandas provides a wide range of functions that help you to manipulate your data effectively. Some of these include mathematical operations, string processing as well as date and time conversions. For example,
# Mathematical operations df['variance'] = df['col1'] - df['col2'] # Date conversion df['date']= pd.to_datetime(df['date_col'])
Data Cleaning
Pandas offers tools for cleaning raw data which usually contains errors, missing values or inappropriate formats. This aspect is very crucial especially given that data scientists spend 50-80% of their time cleaning data (source). Pandas provides several methods for data cleaning, some of which include,
# Remove null values df.dropna() # Replace null value df.fillna(value) # Remove duplicates df.drop_duplicates()
Data Merging
Merging dataframes is a powerful feature in Pandas that enables combining data from various files.
merged = pd.merge(df1, df2, on='id', how='inner')
Data Visualization
You can explore data visually using the built-in plotting available in Pandas. Although not as flexible as Matplotlib, its ancestor, for quick exploratory purposes, it suffices.
df['col'].plot(kind='hist')
To explore Pandas to its full extent, visit the official documentation here.
The essence of the open-source Pandas library, and why I’m such an aficionado of it, lies in its extensive functionality. By undertaking every task from loading bulky datasets to high-performance data cleansing, merging and reshaping, to name a few, data analysis has never been so seamless. Including libraries like NumPy and Matplotlib in its foundational framework just extends its efficiency yet another notch higher. Just by importing this one simple library transparently bridges the void between data handling and data insight.As a professional coder, one of the most sought-after libraries I can vouch for is Pandas. For those dabbling with data manipulation in Python, this open-source data analysis and manipulation tool is nothing short of a boon. This power-packed library stands unrivaled in terms of flexibility and functional richness it provides to manipulate structured data.
import pandas as pd data = {'Col1': [1, 2], 'Col2': [3, 4]} df = pd.DataFrame(data) print(df)
This simple example above exhibits how effortlessly you can create DataFrames in Pandas – one of many bountiful features it beholds. DataFrames are a way to store data in grid that is easy to view and manipulate. Each row of these grids corresponds to measurements or values of an instance, while each column is a vector containing data for a specific variable. This means that a data frame’s rows do not need to contain, but can contain, the same type of values: they can be numeric, character, logical, etc.
Next, let’s discuss one of the significant issues encountered when working with large datasets – handling missing data. More often than not, real-world datasets have missing data, and tackling them becomes inevitable.
df=pd.DataFrame({'A':[1,2,np.nan],'B':[5,np.nan,np.nan],'C':[1,2,3]}) df['states']="CA NV AZ".split() df.set_index('states',inplace=True) new_df=df.dropna(axis=1) print(new_df)
Pandas provides a plethora of options like filling the missing data, removing the instances of missing data (like illustrated above).
Often, in the process of manipulating data, we desire certain transformations on our datasets. Let me brief on some of those.
Data Transformation%
DataFrame functions | Description |
---|---|
pivot() | Reshape data (produce a “pivot” table) based on column values |
melt() | Unpivots a DataFrame from wide format to long format |
concat() | Concatenate pandas objects along a particular axis |
merge() | Merge DataFrame or named Series objects with a database-style join |
join() | Join columns of another DataFrame |
Additionally here is an example of merging two datasets,
left = pd.DataFrame({'key': ['K0', 'K1', 'K2', 'K3'], 'A': ['A0', 'A1', 'A2', 'A3'], 'B': ['B0', 'B1', 'B2', 'B3']}) right = pd.DataFrame({'key': ['K0', 'K1', 'K2', 'K3'], 'C': ['C0', 'C1', 'C2', 'C3'], 'D': ['D0', 'D1', 'D2', 'D3']}) result = pd.merge(left, right, on='key') print(result)
The datasets ‘left’ and ‘right’ are merged on the basis of a common attribute, ‘key’. The result is a new dataset.
Furthermore, the Panadas library comes equipped with a gamut of built-in mathematical functions that provide the potential to carry out desired operations on your datasets. There also advanced functionalities like groupby, dealing with date-time data, category datatype et al, which extend an umbrella of powerful features to proficiently deal with complex datasets.
Ergo, the versatility and prowess that Pandas offers in case of handling and manipulating data are truly admirable. It enhances the overall productivity and allows users to perform complex computations with simplicity.
For more comprehensive information on Pandas, look over the Pandas documentation.In optimizing performance in pandas, some factors are really important to consider due to the large memory load that can come with handling big data. Here are some tips on to how best optimize your code when using pandas:
Use vectorized operations:
Vectorization is a technique of applying operations to entire arrays instead of individual elements. It’s akin to broadcasting in NumPy. Most pandas methods and functions are designed to work with Series or DataFrame objects directly.
import pandas as pd import numpy as np data = np.random.randint(0, 100, size=(5, 2)) df = pd.DataFrame(data, columns=['A', 'B']) # Vectorized operation df['C'] = df['A'] + df['B']
Loading less data:
Only load the specific rows/columns you require for your analysis. You can specify which columns/rows to load when reading from a file using read_csv function’s usecols parameter.
columns_example = ['name', 'age'] df = pd.read_csv('sample_data.csv', usecols=columns_example)
Avoid using loops:
Loops can drastically slow down your computations. Pandas provide functions like apply(), map(), etc., which can often perform the same operation more quickly because they utilize C-based under-the-hood optimizations.
df['new_column'] = df['old_column'].apply(some_function)
Consider Using Categorical Data For Text Data:
You can save memory and speed up computations by converting text data into categorical form. Note this is only beneficial when the total number of categories is considerably smaller than the length of the DataFrame.
df['column'] = df['column'].astype('category')
Use Chunking If Data Doesn’t Fit Into Memory:
If your dataset is too big for your machine’s memory, you can still load it into smaller chunks, then process each chunk at a time keeping memory usage manageable.
chunk_size = 50000 chunks = [] cunky_iterator = pd.read_csv('large_data.csv', chunksize=chunk_size) for chunk in chunky_iterator: chunks.append(chunk) df = pd.concat(chunks, axis=0)
Optimize datatypes:
By default, pandas tends to load data types in a way that allows generality but this could be memory consuming. We can save substantial memory by paying attention to the dtype param during data loading.
optimized_df = pd.read_csv('datafile.csv', dtype={'column1':np.int8, 'column2':np.float32})
Saving to binary format:
Reading and writing from/to .csv files can take a lot of time. By saving your DataFrame in a binary format like .pickle or .hdf, you reduce file size and speed up your I/O operations.
df.to_pickle('/tmp/dataframe.pkl') pd.read_pickle('/tmp/dataframe.pkl')
Performance is critical due to pandas’ ability to handle large datasets. Understanding low-level details about how things are computed enables us to write faster programs as we avoid pitfalls and know where the hard limits will be. References: Real Python, Pandas Official Documentation.One of the most thrilling aspects of working with Python’s Pandas library is its multitude of advanced features. With a bewitching enchantment, they make data cleaning and exploration excitingly versatile and efficient.
Let’s unmask some of these features:
1) Chaining Assignments
Pandas supports chain assignments which allow you to perform multiple operations on a DataFrame within one statement. Basically, this involves combining more than one action in a single line of pandas code. For instance:
df = df[df['age']>25].assign(age_plus_one = df['age'] + 1)
2) Method Chaining
Method chaining allows us to call methods on an object one after another, each time acting on the result from the preceding method. The output here would be the final result generated by the sequence of method calls. This is a great way to condense many different operations into one line, making the code cleaner and easier to understand. Notably, the use of method chaining prevents intermediate variable creation thus saving memory.
Have a look at this snippet of code using method chaining:
(df.loc[:, ['B', 'A']] .rename(columns={'B': 'new_B', 'A':'new_A'}) .assign(A_plus_B = lambda x : x.new_B - x.new_A))
3) Multi-indexing or Hierarchical indexing
The terminology used for multi-indexing might feel confusing but it provides capability for high-dimensional data structure handling. A Pivot table function works wonderfully well on such data sets. See the example below:
data_multiIndex = pd.MultiIndex.from_tuples(list(zip(['bar', 'bar', 'baz', 'baz', 'foo', 'foo', 'qux', 'qux', 'bar', 'bar'], ['one', 'two', 'one', 'two', 'one', 'two', 'one', 'two', 'two', 'two'])), names=['first', 'second'])
Refer here for more about multi-indexing.
4) Categorical Data Handling
Pandas enables effective encoding of categorical data which optimizes the usage of memory and runs computations faster. We can convert columns into category type as shown:
df["grade"] = df["grade"].astype("category")
For further reading in-depth about handling categorical data, check this out.
5) Time Series Manipulation
Time-series analysis is made significantly simpler with pandas. You can resample time-series data, convert strings into timestamps, work with time periods, and more. For example,
date_rng = pd.date_range(start='1/01/2020', end='1/08/2020', freq='H') df = pd.DataFrame(date_rng, columns=['date']) df['data'] = np.random.randint(0,100,size=(len(date_rng)))
Find more insightful details here about manipulating time series.
Pandas indeed comes with a chunk of powerful features waiting for you to untangle them. By delving deep into Pandas, you will open up a whole new world of possibilities and capabilities when it comes to dealing with data. Being abreast of such advanced features empowers you to handle your data more effectively and adeptly. Shedding light on such complex functionalities should help you better appreciate what Pandas truly has to offer. Learning how to harness these tools can dramatically increase your productivity and effectiveness as a professional coder. It is all there waiting for you to aim for the moon. Happy coding!
Pandas is an open-source, extensive Python library. It allows for flexible data manipulation and analysis. While it’s a key tool in any data analyst or programmer’s toolkit, there are some common pitfalls that users often fall into when working with Pandas. Let’s dive in to discuss some of these typical issues and how to mitigate them.
1. Chained Assignments
Chaining assignments refer to scenarios where one does chained indexing like
df['col']['row'] = 'x'
. This can lead to unpredictable results and a common error known as the ‘SettingWithCopy Warning’. Since Pandas offers two ways to index data – with loc and iloc – it gets mixed up with chained assignments which follow neither of these.
Avoiding this pitfall: use Pandas inbuilt functions like
.at[], .iat[]
or
.loc[], .iloc[]
instead of relying on chained indices. These are more predictable and performant. For example:
df.loc['row', 'col'] = 'x' df.at['row', 'col'] = 'x'
2. Not Using Inplace Parameter Correctly
In many Pandas operations you’ll see an ‘inplace’ attribute in functions. This has both advantages and drawbacks depending on the exact situation. The primary use of ‘inplace=True’ is its capacity to modify the original DataFrame. However, using ‘inplace=True’ may enforce type consistency across columns leading to unexpected outputs.
Avoiding this pitfall: Wise usage of ‘inplace’ attribute and reassigning the modified DataFrame to a new variable e.g.:
df_modified = df.dropna()
3. Ignoring Data Types
Even though Pandas is very convenient at managing different types inside a data structure, developers often ignore the data types leading to increasing memory usage and slower computation times. It is especially crucial when dealing with large amounts of data.
Avoiding this pitfall: Looking at the datatype of each column and using appropriate datatypes, also converting objects to category whenever necessary will help.
print(df.dtypes) #convert if object type and only few unique variables df['column_name'] = df['column_name'].astype('category')
4. Misusing the apply function
Apply is used for applying a function across an axis (row/ column) of a DataFrame. However, it is known to be a bit slow. When working with larger datasets, resorting to apply function every time can significantly slow down the execution time.
Avoiding this pitfall: Try to refrain from using the apply method excessively, resorting to in-built pandas methods, vectorized operations or list comprehensions where possible.
# Vectorized operation example df[col] = df[col] * 2 # Apply function equivalent df[col] = df[col].apply(lambda x : x*2)
5. Memory Usage
Without properly considering memory usage while dealing with large datasets, developers might run out of memory causing a system crash or excessive usage of resources.
Avoiding this pitfall: Firstly, optimize your data types as mentioned before. Second, consider loading chunks of data instead of the whole dataset at once.
chunk_iter = pd.read_csv('large_file.csv', chunksize=1000) for chunk in chunk_iter: process(chunk)
Nonetheless, one has to always remember that learning to effectively navigate a tool like Pandas requires both practice and patience. Mistakes are not always failures, they can also provide a valuable learning experience. However, avoiding these common pitfalls would enhance efficiency and productivity while developing code with Pandas.
References:
The power of Python’s library, Pandas, cannot be overstated when it comes to data manipulation and analysis. Expert and novice programmers alike find its versatility and ease-of-use invigorating, and it is widely trusted in industries far and wide, from science to finance.
To illustrate this, consider some common tasks easily tackled with Pandas:
- Loading data into a usable format: With just a single line of code, Pandas allows for easy import of major file types like CSVs, JSON, and SQL databases.
- Data cleaning: Null values, duplicates, and outliers can obfuscate your true findings. Thankfully, Pandas offers straightforward commands to reveal and handle them.
- Data exploration: The real magic of data analysis lies in the discovery. With Pandas, high-level summaries (mean, median, count) are easily accessible, while more meticulous digging (cross-tabulation, pivot tables) are possible too.
import pandas as pd df = pd.read_csv('yourfile.csv')
df = df.dropna()
df.describe() pd.crosstab(df['col1'], df['col2']) df.pivot_table(index='col1')
And remember, this absolutely scratches the surface of what’s available. For those interested in digging deeper, the official Pandas documentation is an excellent starting point as it is detailed, chock-full of examples, and ever-evolving just like the open-source community behind it.
As our digital world becomes more intricately entwined with data, libraries like Pandas will increase in importance. Now, more than ever, it’s important to arm oneself with the best tools for processing, cleansing, and dissecting that data – Pandas stands firm amongst these options. To stay ahead in the race, both businesses and individuals need to understand how to work with data efficiently, and for that, learning Pandas really does matter.
Evidently, not limited only to the scope of technical individuals, Pandas and similar data analytics tools ought to be absorbed and employed across spectrum – from novel learners commencing their coding journey to skilled professionals already immersed in executing complex data operations. As the mantra goes, ‘Knowledge has a beginning but no end. The next level awaits.’