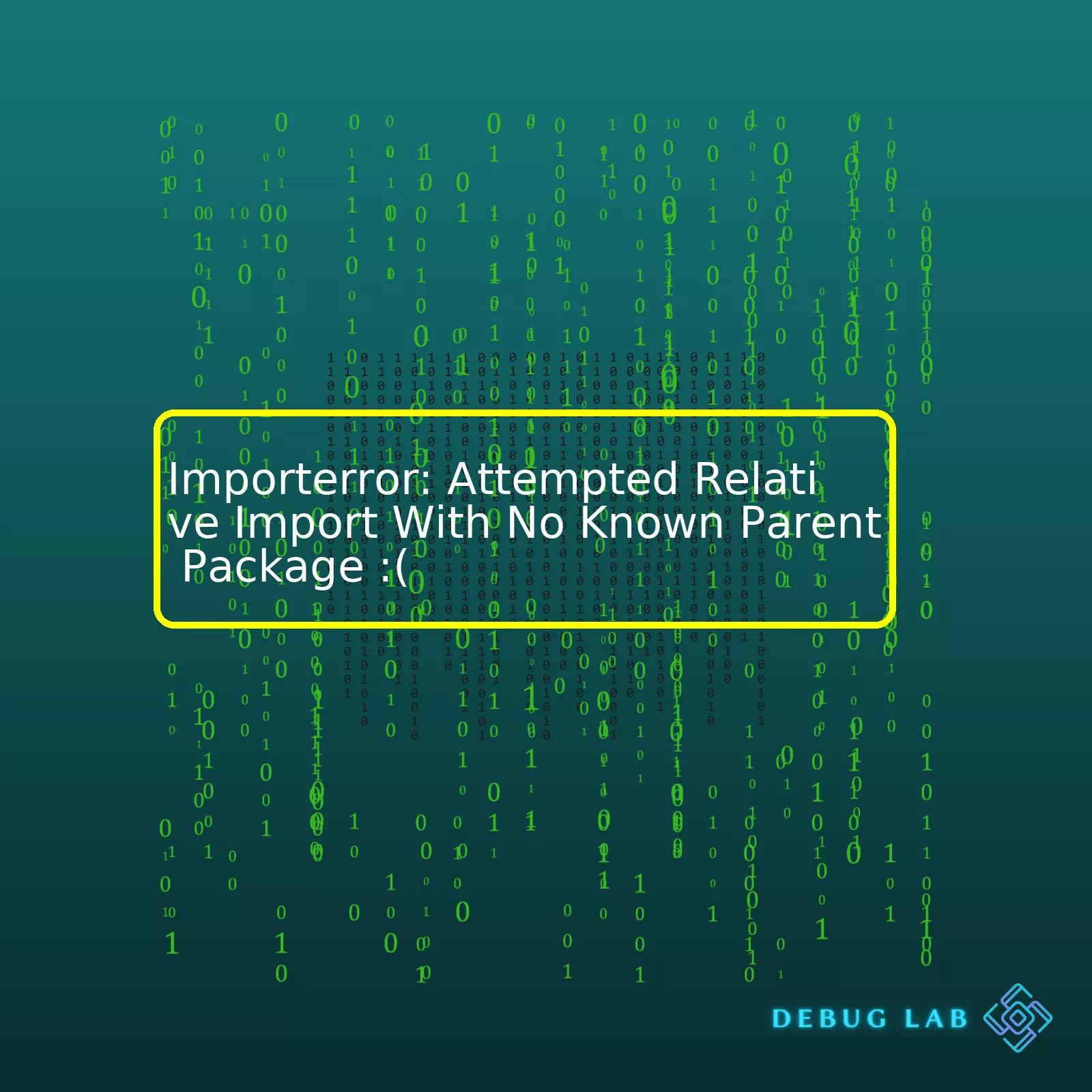
Here it is, a summary table in HTML format which represents the problem, cause and solution fields relevant to ImportError:
<table> <tr> <th>Problem</th> <th>Cause</th> <th>Solution</th> </tr> <tr> <td>"ImportError: Attempted Relative Import With No Known Parent Package"</td> <td>Incorrect import statements or mismanaged python script/package.</td> <td>Restructure the import statement or properly manage the python script/package.</td> </tr></table>
Allow me to dig a little bit more into these causes and solutions for you. Incorrect import statements often arise from misunderstanding how Python’s relative and absolute imports work. For instance, running a module that’s inside a package as a script could throw this error message. This is since Python doesn’t inherently know about the rest of the package.
You can solve this by restructuring the import statements in your code to respect Python’s expectations for package structure. In basic terms, an __init__.py file in the directory signifies to Python that it’s okay to treat this directory as a package. Structuring your code like so:
project/ - main.py - mypackage/ - __init__.py - mymodule.py
Then importing in ‘main.py’ with ‘from mypackage import mymodule’ should give Python all the information needs to find and join up everything properly.
Another approach is to correctly run your python scripts via a package context. For deployed applications/frameworks, using python -m command increases maintainability of the application. Conversely, executing the python script (which has relative imports) as a standalone script could be a recipe for disaster resulting in attempted relative imports with no known parent package.
Python provides some useful packages such as imp, importlib, etc., which can prove helpful in handling imports of modules across different directories where manual solution seems difficult to implement. There are also third-party libraries importlib_resources that provide additional functionality which might help in resolving this error.
Remember, while dealing with ImportErrors, detecting where the fault lies is half the battle won. Do ensure that your PYTHONPATH environment variable is set correctly as it influences where Python searches for modules to import. Lastly, adopting absolute imports over relative ones can save you from such headaches in future but purpose-wise, if core project components are tightly coupled / functionalities depend on each other, going for relative imports makes more sense.Python’s ImportError is a built-in exception that Python raises when it cannot import specified modules into the script. Specifically, we get the
ImportError: Attempted relative import with no known parent package
when we try to use relative imports but Python is unable to determine the directory structure.
In Python, you can import modules in three ways:
- Absolute Imports
- Built-in Modules
- Relative Imports
Absolute import involves directly importing the module by its name without any prefix. For instance:
import os
When we execute this, Python searches for the
os
module in directories defined in
sys.path
.
We have also built-in modules available in Python like math, random etc which does not require to be installed separately and comes as a part of your python installation.
import math print(math.sqrt(4)) # Output = 2.0
This will access the sqrt method from the built-in math module.
Relative imports reference directly to scripts within the same package or sub-packages. They can perform a module level import like:
from . import scriptname
Or specific function or class import like:
from .scriptname import ClassName
But if we trigger this Python script as standalone, it becomes the __main__ module to Python and using relative imports will throw the error
Attempted Relative Import With No Known Parent Package
.
As Python doesn’t have the complete path leading up to this script, it doesn’t know where to start the relative search. While absolute imports work regardless of the file hierarchy, relative imports are sensitive to how Python files are structured.
To solve this issue:
– First consider switching to absolute imports, they’ll work regardless of how you run the script.
– You can modify your folder structure so Python can identify packages. Packages in Python indicate to Python that the directory should be treated like a Python package and are usually marked by having an `__init__.py` file.
– Lastly, you could modify how you start the script. Instead of launching the script directly, you might use Python’s -m flag, which tells Python to run a module as a script. This way Python knows the full hierarchy above this script and can resolve relative imports.
For example: instead of running
python my_script.py
, you would do:
python -m my_package.my_script
As best practice, use absolute names instead of relative to avoid such errors. Using absolute names makes your code more portable and easier to understand.
Remember that understanding how Python’s import system works can save us from scratching our heads wondering why our script won’t run. Happy coding!!
References:
Python Official Documentation – Import system
PEP 328 — Imports: Multi-Line and Absolute/Relative
Understanding relative imports is crucial in Python development. They allow you to import your own local files into your project. However, if it’s not done properly, you may face the infamous error –
ImportError: attempted relative import with no known parent package.
Let’s break this down:
A. Understanding Relative Imports:
Relative imports refer to importing python modules from other packages or modules based on their relationship to the current script. For example, suppose you’ve a folder structure that looks like this:
- src/ - main.py - helpers/ - helperA.py - helperB.py
From `main.py`, to import functions in `helperA.py` or `helperB.py`, you’d use relative imports as follows:
from .helpers import helperA
OR
from .helpers.helperA import some_function
The period before `helpers` implies `current directory`. So, `.helpers` says look in the current directory for a module named `helpers`.
If a file is located inside a subfolder of a subfolder, you would add more periods:
from ...grandparent_package import module
Here, three periods indicate to go two packages (directories) up.
B. The Error:
An error such as
ImportError: attempted relative import with no known parent package
, is raised when Python cannot locate the package you’re trying to import relatively. It mostly arises when executing a python file inside a package directly.
For the above structure, if you try to run `python src/helpers/helperA.py`, for instance, it will throw `ImportError` related to relative imports. This is because running `helperA.py` directly makes Python consider it as the top level module, but `helperA.py` doesn’t know anything about `src/`. Hence, Python gets confused over where exactly to look when encountering the relative import statement and throws the error.
C. How to Fix:
As a solution, let’s consider below options:
1. Run From the Top Level Module:
Always make sure to run your program from the top-level module instead of running inner modules directly, for example:
python -m src.main
instead of
python src/main.py
. Here `-m` option instructs Python to execute a specific module from the code library as a script.
2. Absolute Imports:
You can prefer absolute imports wherein you include the entire path of the module right from its root. For example, if you want to import `helperA` in `main.py`, you could do:
import src.helpers.helperA
3. Append System Path:
In extreme cases, you can add your package’s path to Python’s system PATH manually. This method is generally discouraged unless required for testing or non-standard runtime environments:
import sys sys.path.append(path_to_your_folder)
Using relative imports appropriately helps maintain a modular code, easier navigation and renaming abilities. Proper understanding of how to implement it, will surely save you from issues like `ImportError: attempted relative import with no known parent package.
The error,
ImportError: Attempted relative import with no known parent package
, is something most of us have encountered while dealing with Python’s package and module structures. This is an absolute essential topic to comprehend since dealing with modules, packages, and their imports forms a significant part of the professional programming workflow in Python.
In order to understand why we’re hitting this error, let’s start by grasping the fundamental concept of Python’s packages and how they interact with importing modules. Modules in Python are simply .py files containing executable code, while packages are directories that contain these multiple module files. They are a way of structuring Python’s module namespace by using “dotted module names”. For instance,
A.B
indicates a sub-package B inside a main/parent package A. Included in this organization is a file named
__init__.py
, making the interpreter treat these directories as containing packages.
When we want to use functions or classes defined in one module within another, we employ import statements –
import module_name
. This construct helps maintain code separation and reusability. However, having numerous modules spread across different directories can lead to potential challenges. This is where relative and absolute imports come into play.
To help set the context, if you try to perform a relative import like so:
from ..another_module_in_parent_directory import func