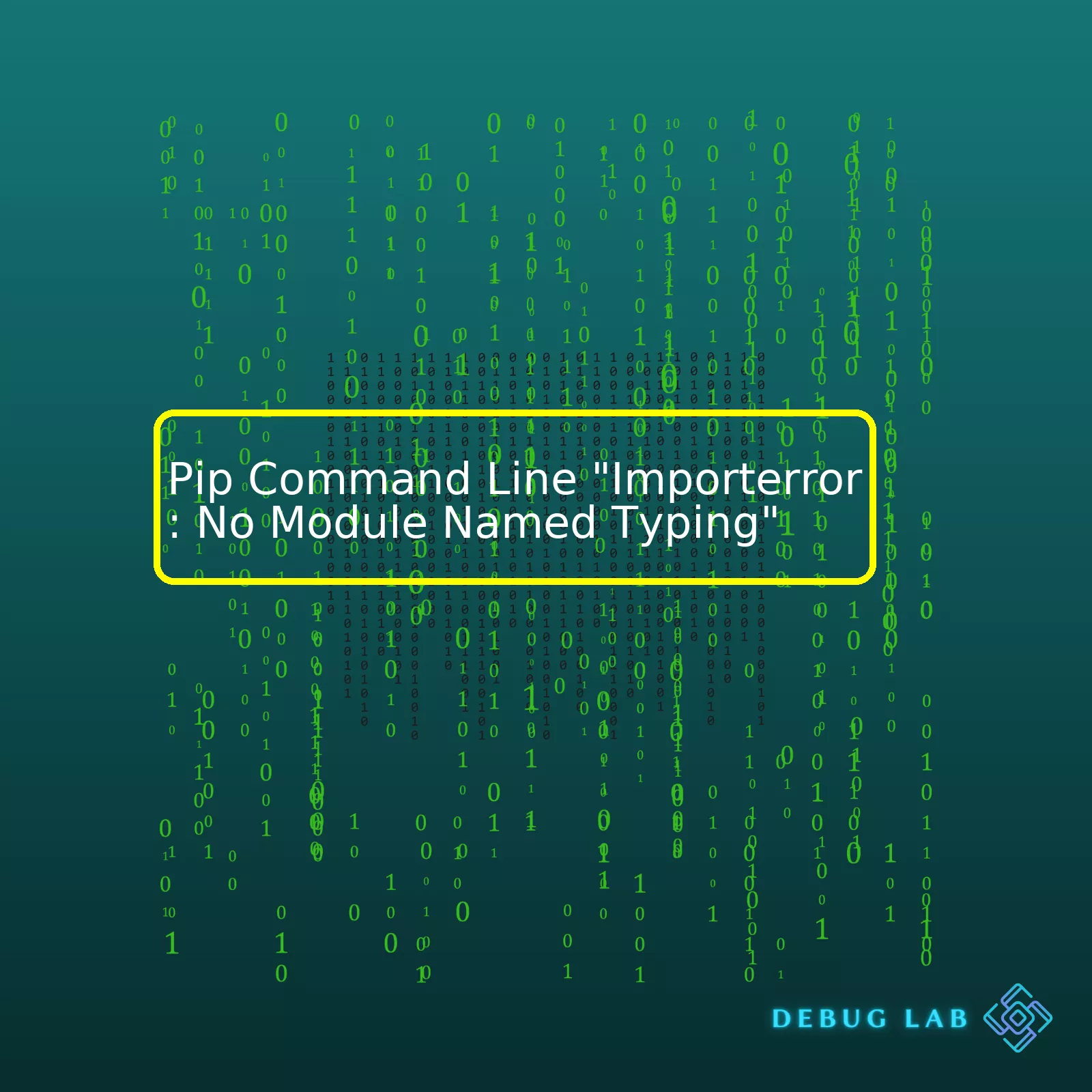
PIP Command | Description |
---|---|
x 1 1 pip install typing |
This command will download and install the Typing module from PyPI (Python Package Index). |
xxxxxxxxxx 1 1 1 python -m pip install typing |
An alternate way to use pip to install the Typing module. Helpful if multiple Python versions are installed. |
xxxxxxxxxx 1 1 1 pip show typing |
This command gives all the information about the installed ‘typing’ module including its version, location, etc. |
xxxxxxxxxx 1 1 1 pip uninstall typing |
This command is supposed to safely remove or uninstall a Python module. |
When using Python 3, you may come across an error like “Importerror: No Module Named Typing”. It occurs because your python environment does not have the ‘typing’ module. The ‘typing’ module is an integral part of Python that provides runtime support for type hints. In other words, it is not a third-party module you have to install explicitly. It comes with Python itself when we install it.
However, if you face such an error, there is a straightforward solution. The PIP commands mentioned in the table above can help you handle this problem effectively. For instance, you can simply execute the command ‘pip install typing’ in your command line interface to ensure that the module is downloaded and installed in your environment from PyPI (Python Package Index).
Sometimes, especially after switching between different Python environments or versions, you might still get the same error. If this happens, try uninstalling the ‘typing’ module and reinstalling it again with the respective commands in our table.
Likewise, if you have multiple Python versions installed, it’s also possible that the ‘typing’ module was installed on one version but when you switched to another, the module wasn’t there. To avoid confusion in such cases, you can use the command ‘python -m pip install typing’ instead of just ‘pip install typing’. This ensures that the module gets installed in the relevant Python environment.
Apart from installation, you can also verify whether the ‘typing’ module is correctly installed and its version by running ‘pip show typing’ in your terminal. Consequently, understanding and exploiting these PIP commands will allow you to handle “Importerror: No Module Named Typing” effectively and keep your coding journey smooth and uninterrupted.
The
xxxxxxxxxx
ImportError: No Module Named Typing
is a common error message that typically denotes the absence of the
xxxxxxxxxx
typing module
in your Python environment.
Now, allow me to unwrap this error and find an amicable solution using the pip command line.
In the grand scope of Python programming, we work with various data types such as strings, integers, and floats. In more complex scenarios when dealing with user-defined classes or built-in data structures (like lists), we would ideally want to specify what kinds of values these composite objects should contain. This is where the typing module available in Python 3.5 and later versions comes to our aid by enabling support for type hints.1
Our focal point, the TypeError can likely ensue if:
– You are trying to use the feature on a version before Python 3.52
– The
xxxxxxxxxx
typing module
is simply not installed in your Python environment
As programmers, it’s pivotal to have a grip over which of your projects has a requirement for this module; hence segregating virtual environments becomes crucial.
When faced with the
xxxxxxxxxx
ImportError: No Module Named Typing
error, a quick performable fix we could implement involves installing the package via pip. To attempt this quick fix, open a terminal and run:
xxxxxxxxxx
pip install typing
However, this generalized command assumes that you only have one Python environment. In situations catering to multiple environments, you’ll want to receive assistance from a specific version of pip. For instance,
xxxxxxxxxx
python3.6 -m pip install typing
which helps you ensures that the
xxxxxxxxxx
typing module
is being installed for python3.6.
If you’re juggling multiple virtual environments, you’ll need to activate yours prior beginning the installation3. This can be accomplished by running:
xxxxxxxxxx
source venv/bin/activate
After the activation step, install the typing module:
xxxxxxxxxx
pip install typing
Bear in mind that if you embark upon this route, remember to replace
xxxxxxxxxx
venv
with the name of your virtual environment.
To confirm whether you’ve successfully won the battle against this error, import the typing module and check:
xxxxxxxxxx
>>> import typing
>>>
A lack of error messages confirms that the
xxxxxxxxxx
typing module
has been successfully installed for your Python environment, banishing the
xxxxxxxxxx
ImportError: No Module Named Typing
into oblivion. Whether you’re utilizing a standalone Python or con-jointly managing several applications using unique versions with virtual environments, pip commands can prove to be a certified lifesaver, eclipsing these errors without any hassle.Before diving into the core problem, let’s first understand the key elements in play – Python, Pip, and the module named ‘typing’.
Python is a high-level programming language widely used for various applications ranging from web development to data science. It’s praised for its ease of learning and readability aided by a syntax that emphasizes clarity.
Pip, on the other hand, is a de facto tool for managing Python packages, installations, and dependencies. With pip, you can easily download and install Python packages hosted on the Python Package Index (PyPI) and other indexes.
Now, what’s the deal with ‘Typing’? Basically, typing is a built-in Python library from Python 3.5 onward. The typing module is mainly used to support type hints. Type hints are a new feature since Python 3.5 via PEP 484 that allows developers to annotate their functions and methods so they can specify which data types function parameters can be.
Running pip from the command line and encountering an error “ImportError: No module named typing” means the script you’re trying to run requires the typing module but cannot locate it to import into your Python script.
Let’s tackle this error
Here’s how you might possibly see the ImportError when installing or importing a Python package using the pip command:
xxxxxxxxxx
$ pip install
xxxxxxxxxx
ImportError: No module named typing
A fundamental reason for this error message is that the typing module is not found in your current Python environment, especially if you’re using a Python version earlier than 3.5 where the typing module is not preinstalled.
First, verify your Python version:
xxxxxxxxxx
$ python --version
If you’re indeed working with a version less than Python 3.5, upgrading to a newer version would be a beneficial move. Not just to resolve this error but also to leverage the various features and improvements embedded within newer versions:
xxxxxxxxxx
$ sudo apt-get upgrade python3
Another approach is to explicitly install the typing module via pip:
xxxxxxxxxx
$ pip install typing
Sometimes, you might encounter this error because your scripts do not correctly detect the active Python environment. Virtual environments help isolate your Python projects, and ensuring you’ve activated the appropriate environment may assist in resolving the issue. This becomes more crucial if you order different Python versions for separate projects.
You can activate a virtual environment using:
xxxxxxxxxx
$ source /path/to/your/env/bin/activate
Elevating the Understanding
It’s worth noting that Python, as with any technology, continues to evolve. Lapses in compatibility are not uncommon, however, understanding errors such as the one at hand provides invaluable insight into the intricacies involved in the orchestration of Python libraries, scripts, and various modules.
Should you wish to dive deeper into Python’s vast utility opportunities, Python’s official tutorial can deepen your understanding from scratch.
Furthermore, the Python Package Index’s official page of the typing module might illuminate for you more about its application and functionality.
Bear in mind that managing Python versions and packages could become cumbersome at scale. Advanced tools like virtual environments and Pipenv provide advanced capabilities helping handle multiple project environments and dependencies.
In summary, understanding the architecture and functionalities of Python and pip empowers us to troubleshoot errors seamlessly while maintaining neat and clean projects.
It’s absolutely possible that you’ve encountered the dreaded “ImportError: No Module Named Typing” while running your Python program using the Pip command line. The error message is self-explanatory – Python is unable to find the
xxxxxxxxxx
typing
module in its standard library. But let’s delve a bit deeper into the
xxxxxxxxxx
typing
module before addressing the potential fixes for this error.
The
xxxxxxxxxx
typing
library is part of Python’s standard libraries and was introduced in Python 3.5 as a provisional module. Due to its usefulness, Python decided to keep it permanently starting Python 3.7. This module enables clear type hints which allows static typing checkers, linters, or even your development environment understand better the usage of your code.
Here’s a very basic example:
xxxxxxxxxx
def greet(name: str) -> str:
return f'Hello, {name}!'
In this snippet, by using
xxxxxxxxxx
: str
after our variable
xxxxxxxxxx
name
, we’re telling a potential static type checker that name should be a string. The same happens with our special annotation
xxxxxxxxxx
-> str
after our function signature, we’re indicating that our function will return a string.
Now, let’s move onto resolving the error message. Since typing is included in Python 3.5 and up, the solutions are slightly divergent depending on your Python version.
For Python version 3.5 and above:
Since the
xxxxxxxxxx
typing
module comes out-of-the-box with Python 3.5 and up, encountering an ‘ImportError’ could mean that there is a problem with your Python installation. Here are a few things you can do to verify and fix this:
– Verify your Python version by typing
xxxxxxxxxx
python --version
in your terminal. If this command returns a version below 3.5, then consider upgrading your Python version. You can learn how to do so by visiting the official Python downloads page.
– Verify the integrity of your Python environment. It’s feasible that the Python execution environment or the environment where pip is installed has been corrupted, thus causing problems locating appropriate modules.
For Python version 2.x and versions below 3.5:
Users who are still using Python versions 2.x or even versions below 3.5 have two main options to access the
xxxxxxxxxx
typing
module:
1. Upgrade to a newer Python version. As already emphasized, Python 2 has reached its offcially end of life at 2020–1–1 and won’t receive any updates. Upgrading Python is usually the best course of action both for efficiency and security reasons.
2. Install the
xxxxxxxxxx
typing
library manually by typing
xxxxxxxxxx
pip install typing
on your terminal. This library backports most of the new standard library
xxxxxxxxxx
typing
module functionalities to older Python versions.
HTML tables aren’t particularly appropriate for the information at hand, but here are some HTML snippets that demonstrate how to use them:
xxxxxxxxxx
<table>
<tr>
<th>Person</th>
<th>Email</th>
</tr>
<tr>
<td>John Doe</td>
<td>john.doe@gmail.com</td>
</tr>
</table>
To summarize, the error message ‘ImportError: No Module Named typing’ when running your Python application typically means that Python cannot locate the
xxxxxxxxxx
typing
module which is included in Python’s standard libraries starting from Python version 3.5. Depending on your Python version – if it’s below 3.5 or not – there could be different strategies you’d like to apply in order to resolve this issue.
The issue tagged “Importerror: No Module Named Typing” is one of the more common issues developers encounter when working with Pip, the Python package installer. This error can come up due to a few reasons:
– Incompatibility with your Python Interpreter: The first possible cause can be that your python interpreter isn’t compatible with the ‘typing’ module. Before Python 3.5, there wasn’t an in-built ‘typing’ module. So, if you’re utilizing a version of Python lower than 3.5, you’d have import errors when using packages that rely on it.
Solving this problem requires checking your Python version with the command:
xxxxxxxxxx
python --version
If the version showed is lower than 3.5, I recommend upgrading to a newer version of Python.
– Outdated pip: Pip’s outdated versions may have troubles installing or upgrading modules flawlessly, including the ‘typing’ module. Update pip using the command:
xxxxxxxxxx
pip install --upgrade pip
– Absence of the ‘typing’ module: If the ‘typing’ module isn’t installed in your current environment, you’ll certainly get an ImportError. Use pip to install the ‘typing’ module with the following command line since it’s available as a standalone library on PyPI:
xxxxxxxxxx
pip install typing
– Environmental discrepancies: There may be differences between your global Python environment and local project-specific environments, possibly leading to import errors at runtime. Either install the necessary packages in the right environment or align your development and deployment environments by using tools like Docker or virtual environments. With such tools, you can ensure that the correct versions of all dependencies, including the ‘typing’ module, are consistently available across various stages of your application life cycle.
xxxxxxxxxx
pip install virtualenv #for installing the tool
virtualenv venv #for creating the new virtual environment
source venv/bin/activate # for activating the environment in shell
runtime.txt #for specifying the Python version in case of using Heroku or other similar platforms
However, always remember to deactivate your environment after you are done with your work.
Taking proactive steps such as noting compatibility requirements and routinely updating your pip are effective ways of preventing recurring “ImportError: No module named typing” from showing up. If they do come up, coders can rely on the solutions outlined above to resolve them. To delve deeper into resolving pip-related import errors, check out official Python and Pip documentation online.
Remember, coding involves progressive learning and problem-solving. Even professional Python coders sometimes face debugging issues. But with clear understanding of Python, its packages, and a methodical approach, these hurdles can be overcome. Perhaps, now you might think twice about breaking your head over ‘import errors’. They might just be there to grant an improved version of Python or pip!The Python ImportError indicates that the Python interpreter could not find a specific module. In your case, this module happens to be the
xxxxxxxxxx
typing
. Notably, the
xxxxxxxxxx
typing
module was introduced in Python 3.5 as a provision for hints about the expected types of function arguments and return values. That being said, it’s compatible with these versions and beyond.
Here is how you can approach and resolve this particular ImportError: `No module named ‘typing’`.
Solution 1: Upgrading your Python to a compatible version
You might encounter this error because you are using an earlier version of Python, possibly below 3.5, which does not support the
xxxxxxxxxx
typing
module. Therefore, upgrading Python to a compatible version can fix the problem.
Firstly, check your Python version:
xxxxxxxxxx
python --version
If your Python version is below 3.5, consider upgrading Python to a higher version such as 3.7 or 3.8. Here is a useful guide from Python Docs on a successful upgrade.
Solution 2: Explicitly installing the typing module via pip
Python might already be up-to-date, but the module has not been installed in your system yet. In this instance, you can install
xxxxxxxxxx
typing
with
xxxxxxxxxx
pip
, Python’s dedicated package installer. Running this command installs the latest wheel file for the module:
xxxxxxxxxx
pip install typing
Still experiencing the same error? It might be caused by an outdated pip installation. Updating pip can also solve the issue. To update pip, use the following command:
xxxxxxxxxx
pip install --upgrade pip
After pip finishes updating itself, try installing the typing module again.
Solution 3: Check the current working directory
Certain errors can materialize if there’s a conflict between your current working directory and the location where you’re trying to import the module from. Execute this command to figure out your pathway-specific problems:
xxxxxxxxxx
import os
print(os.getcwd())
This will provide you with the path of your current working directory. If your script is not in the same place as your modules, you have to alter your paths appropriately or shift your scripts.
It’s crucial to reiterate that while these solutions promise to be potent fixes, they won’t work universally, due to variations among different machines, operating systems, and Python environments. Always strive to understand your local dev setup and its potential peculiarities. Consider checking the official documentation periodically for changes related to importing specific modules like
xxxxxxxxxx
typing
. These steps would help you become more fluent in the process of debugging and module management in Python.
For extensive details and optimal practices in handling Python ImportErrors, consider exploring this practical guide Real Python: Understanding Python’s import. Remember, error messages are not roadblocks, they merely act as signposts pointing towards the right path.
A common obstacle when using Python’s pip command line is coming across an ImportError. Specifically, you may encounter this error:
xxxxxxxxxx
ImportError: No module named typing
This usually implies that the Python interpreter cannot find a module or package called ‘typing.’ The ‘typing’ module is normally standard in Python 3.5 and onwards, but if you’re using a lower version, you might face this error.
Let’s delve into some troubleshooting strategies to resolve this issue:
1. Update Your Python Version
The ‘typing’ module was included as a standard library from Python 3.5 onwards. Therefore, if you’re currently running on an older Python version, such as Python 2.x, you will likely face the
xxxxxxxxxx
ImportError: No module named typing
.
You can check your Python version by entering this on your terminal:
xxxxxxxxxx
python --version
To remedy this, simply update your Python version. You can find the latest Python versions at the official Python website (https://www.python.org/downloads/). Download and install the version suitable for your system.
2. Install the ‘typing’ Module
If you cannot update your Python environment due to certain restrictions, you can manually install the ‘typing’ module. To do so, use the pip install command:
xxxxxxxxxx
pip install typing
Afterwards, test your application again; it should work as expected.
3. Check Your Virtual Environments
Python programmers often make use of virtual environments to keep project-specific dependencies isolated. If you’re using a virtual environment, ensure you’ve activated the right one before running the application.
To activate a virtual environment:
xxxxxxxxxx
source env/bin/activate (on Unix or MacOS)
\path\to\env\Scripts\activate (on Windows)
Keep in mind, dependencies installed outside the active environment will not be accessible inside it.
4. Ensure Pip and Python Version Compatibility
It’s essential that your pip version matches your current Python environment. For instance, if you’re using Python 3.x, ensure you’re applying pip commands related to Python 3.x, which could be
xxxxxxxxxx
pip3
or
xxxxxxxxxx
pip3.x
depending on your setup.
5. Revisit Your Code
Lastly, verify your actual code imports. Ensure there are no typographical errors in the import statement producing the
xxxxxxxxxx
ImportError: No module named typing
.
For reference, here is what an import statement for the ‘typing’ module looks like:
xxxxxxxxxx
from typing import List
Approach problems systematically, and with these strategies, you should be able to resolve and prevent
xxxxxxxxxx
ImportError: No module named typing
effectively. Remember – keep your Python environment up-to-date and keep a keen eye on your import statements and virtual environments.
The Python programming language has become a global standard for everything, from data analysis to web development and beyond. Though Python’s simplicity and ease of use make it a powerful tool, developers can sometimes encounter challenges. Among these is a common error that developers may encounter:
xxxxxxxxxx
ImportError: No module named typing
.
This error usually occurs when the python interpreter encounters an import statement in your code and is unable to locate the module named “typing.” There could be many reasons for this, one being if the typing module isn’t installed properly or not at all. Another reason might be related to how you’ve structured or organized your code.
To combat these “No Module” issues, let’s walk through some Python best practices:
Installing modules:
Many people will turn to pip (which is Python’s package manager) to install packages and modules. To use pip, you need to structure your command following the syntax outlined here:
xxxxxxxxxx
pip install typing
However, modern versions of Python (3.5 and later) already come with this built-in module, so this step may not be necessary unless you’re working on older Python versions.
Consider using virtual environments:
Virtual environments allow you to manage separate package installations for different projects, creating isolated spaces for them to avoid collisions between package dependencies and versions. Virtual environments keep your projects tidy, especially if they have conflicting dependencies.
To create a virtual environment:
xxxxxxxxxx
python3 -m venv tutorial-env
And activate it with:
xxxxxxxxxx
source tutorial-env/bin/activate
You can then use
xxxxxxxxxx
pip
to install any packages unique to this environment without affecting others.
Check your PYTHONPATH:
The Python interpreter uses the PYTHONPATH variable to decide where to look for modules to import. If the place where your ‘typing’ module resides isn’t included in this path, you will get an ImportError.
Add paths using the following command in Linux/Mac:
xxxxxxxxxx
export PYTHONPATH=$PYTHONPATH:/path/to/your/module/
For windows use:
xxxxxxxxxx
set PYTHONPATH=%PYTHONPATH%;C:\path\to\your\module\
Remember to replace /path/to/your/file/ with the actual path where your ‘typing’ module file is located.
Organize your code files:
Ensure your Python files are well-structured and ordered. When importing modules, there is a hierarchy the interpreter follows, and if your files aren’t structured correctly, it can lead to “No module named…” errors.
Keep the following order when calling the
xxxxxxxxxx
import
function:
– Standard library imports.
– Related third-party imports.
– Local application/library-specific imports.
By adhering to these best practices for Python coding, you will reduce the occurrence of future “No module named” errors in your code.
Finally, stack overflow discussions1 often offer quick solutions and hacks contributed by experienced developers facing similar problems. If your problem pertains to a specific library or package, GitHub repositories often maintain FAQs and community discussion forums where your issue may find resolution. Also, the official Python documentation2 and typing — Support for type hints3 offer comprehensive guides and troubleshooting steps.
Best Practices | Key Action Points |
---|---|
Installing Modules | Use PIP to manage package installations |
Use Virtual Environments | Create separate installation spaces for different projects |
Check Your PYTHONPATH | Add paths for interpreter to locate your packages/modules |
Organize Your Code Files | Adhere to import hierarchy while calling the import function |
From the perspective of a professional coder, when you find yourself face to face with the
xxxxxxxxxx
Importerror: No module named 'typing'
, it suggests that the Python Typing Module is missing from your environment. The typing module essentially provides runtime support for type hints as specified by PEP 484, such as
xxxxxxxxxx
List[int]
and
xxxxxxxxxx
Any
. It’s a fundamental feature of coding in Python, especially if you’re dealing with complex projects that require explicit declarations of data types.
Perhaps, at this point, you might be asking “How did I end up here?”. Well, the most plausible explanation is simply that your Python version doesn’t include the typing module. Typing was added as part of the standard library since Python 3.5. If you’re using an earlier version of Python, you won’t have access to this module, triggering the import error message.
So what’s the solution? Consider upgrading your Python version to something later than 3.5. However, if changing the Python version isn’t an option (maybe because of dependencies on your legacy systems), you can install the typing module separately using pip. Pip, or Pip Installs Packages, is a package management system that simplifies software package installation. Here’s how to use pip to install the python typing module:
xxxxxxxxxx
pip install typing
Remember to do this in your command line interface. The above command tells pip to fetch the latest version of the typing module from PyPi (Python Package Index) and install it into your relevant Python environment. Crucially, this could resolve the node module import error effectively.
Looking ahead, we all know that challenges like this are part of a coder’s life. Errors such as ImportError: No module named ‘typing’ are roadblocks we occasionally stumble upon while traversing paths in the expansive Python universe. But it’s through overcoming these obstacles that we come to truly appreciate the intricate dynamics of coding. And remember, despite initial struggles, once resolved, errors often yield additional knowledge, let them become bricks in your wall of Python proficiency, not stumbling stones.
For further understanding, insights on pip command lines or even specifics on other ImportError messages, online platforms such as StackOverflow, Python documentation site, and others have a wealth of information available that offers in-depth discussions, practical solutions, and real-world examples to help you along your coding journey. Keep coding and keep learning!