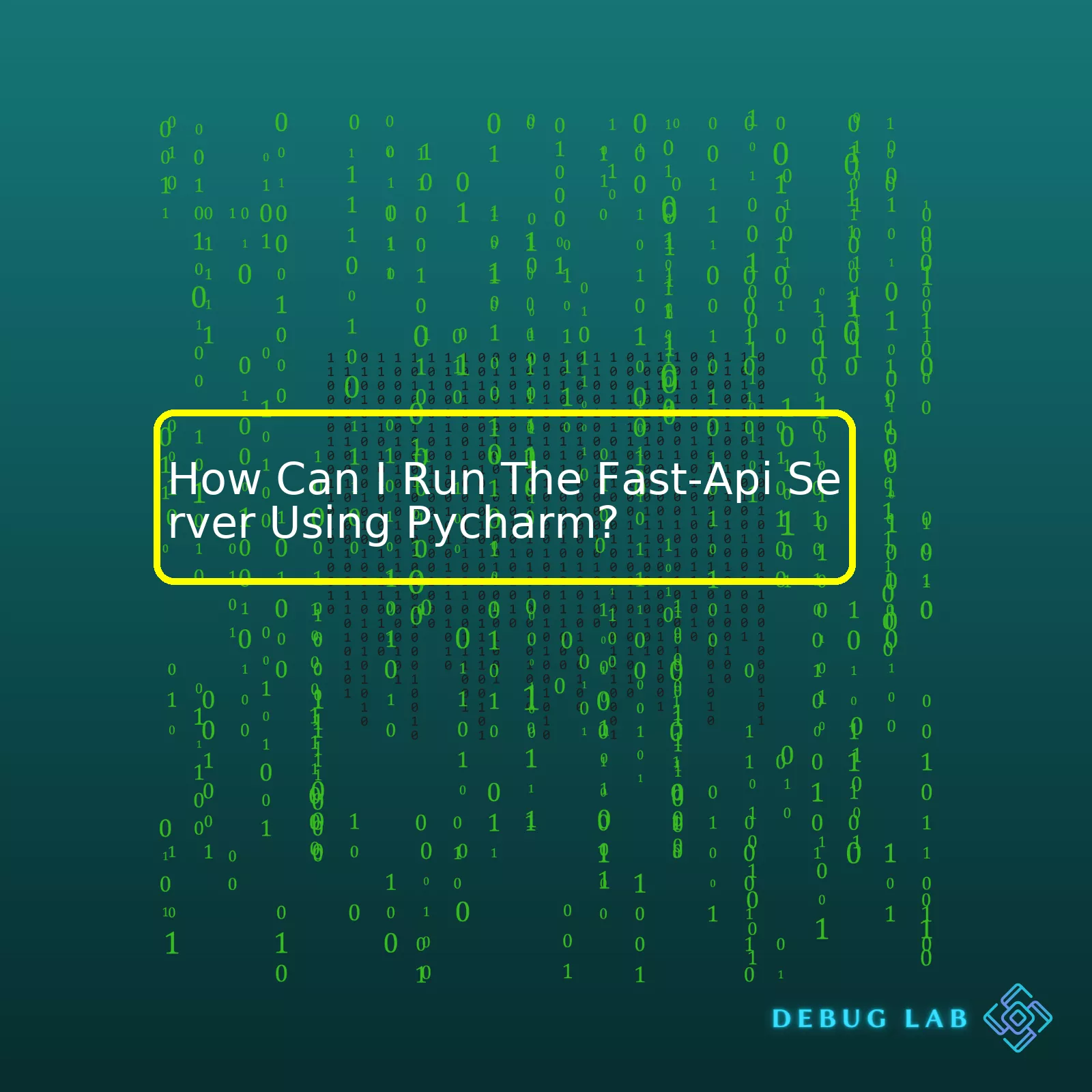
To kick things off, create a new Python file in PyCharm (such as `main.py`) with the following minimal FastAPI application:
from fastapi import FastAPI
app = FastAPI()
get("/") .
def read_root():
return {"Hello": "World"}
Next, install FastAPI and Uvicorn using the following pip command in PyCharm’s terminal:
xxxxxxxxxx
pip install fastapi uvicorn
Now in setting up how to run this server, let’s head back to PyCharm. Here we configure the project interpreter to use a Python environment where both FastAPI and Uvicorn are installed.
Let’s generate a summary table containing the steps to setup and run FastApi server on PyCharm.
Steps | Description |
---|---|
Create New Python File | Create a new Python file such as ‘main.py’ and code a minimal FastAPI application. |
Install FastAPI and Uvicorn | Use pip command in PyCharms terminal – ‘pip install fastapi uvicorn’ |
Configure Project Interpreter | Set the project interpreter to use a Python environment where FastAPI and Uvicorn are installed. |
Run Uvicorn Server | Create a Run/Debug configuration for Uvicorn server: script path -> the Uvicorn executable, parameters -> the FastAPI application name ‘main:app’ and optional – host and port ‘host:port’. |
Finally, after all these steps, to actually run your FastAPI server inside PyCharm, you add a Python Run/Debug configuration for the Uvicorn server by accessing from the main menu ‘Run’ -> ‘Edit Configurations’, hitting the ‘+’ button and choosing ‘Python’. Fill in:
- Script path -> Path to the Uvicorn executable in your virtual environment, typically something like ‘venv/bin/uvicorn’ or ‘venv\Scripts\uvicorn.exe’ depending on your OS.
- Parameters -> String consisting of the module containing your FastAPI application followed by ‘:app’. If your FastAPI application is in ‘main.py’, parameters would be ‘main:app’. Optionally, specify host and port if you want to deviate from the default 127.0.0.1:8000, e.g., ‘main:app –host 0.0.0.0 –port 5000’.
Post that, hit OK and then choose your new Uvicorn Run/Debug configuration from the configurations dropdown menu, hit the Run (or Debug) button, and off you go! Your FastAPI server is running inside PyCharm. You can check its response by navigating to http://localhost:8000 (or whatever host/port you specified) in your web browser.Starting with the basics, FastAPI is a modern, highly productive, web framework for building APIs with Python 3.6+ type hints. To tap into its full might, it should be effectively integrated within an Integrated Development Environment (IDE) like PyCharm.
To set up your PyCharm environment to run a FastAPI server on it smoothly, you have to make sure that you have the right packages installed, correctly configure it, and learn how to run the FastAPI server using PyCharm. Now let’s break down each of these steps:
Step 1: Installing Required Packages
First, make sure you have the “FastAPI” package installed in your project environment. If not yet installed, you can add it by clicking on
xxxxxxxxxx
File > Settings > Project: [Your Project Name]
. Then you select the Python Interpreter section, click on the “+” button and search for “FastAPI”, then install it.
The code snippet to install FastApi through terminal would look like:
xxxxxxxxxx
pip install fastapi
Additionally, you would need an ASGI server, like “Hypercorn” or “Uvicorn”. Follow the same process as before to install it. The terminal command for this process would be:
xxxxxxxxxx
pip install uvicorn
Step 2: Configuring PyCharm Project
Next, you must prepare PyCharm to run FastAPI applications correctly. This step is crucial for successfully running the FastAPI server using PyCharm.
• Firstly, open your application file on PyCharm where you’ll run your FastAPI instance.
• Secondly, go to the menu
xxxxxxxxxx
Run > Edit Configurations…
• In the new window screen, click on the ‘+’ sign located at the upper left hand corner and select “Python”.
• A new Python run/debug configuration will come up. Under script path, select the path of your FastAPI application file.
Step 3: Running the FastAPI server using PyCharm
Finally, to run the FastAPI server from PyCharm, you just need to specify the parameters properly in your Python Run/Debug Configuration.
• Set your program’s script path.
• Add in Parameters box the name of the main application, being “
xxxxxxxxxx
main:app --reload
” if your file’s name is “main” and your FastAPI application variable is “app”.
• Make sure the Python interpreter is set to the one in which you have installed FastAPI and Uvicorn.
• Apply and Save your configurations and you are ready to go!
With all things set, clicking on Run->Run ‘your_config_name’ will start serving your FastAPI server from PyCharm!
It is worth mentioning that the ‘–reload’ flag tells the Uvicorn server to turn on hot reloading, which means that it will automatically detect changes in your files and restart the server accordingly.
Don’t forget to double-check your FastAPI setup in PyCharm by visiting http://localhost:8000 in your browser. You should see “Hello World” or another test page indicating your server is running well. Happy coding!
Sources:
PyCharm Python Debugger Documentation
Official FastAPI Documentation
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.6+ based on standard Python type hints. PyCharm, on the other hand, is an Integrated Development Environment (IDE) used in computer programming, specifically for the Python language. It provides code analysis, a graphical debugger, an integrated unit tester, and supports web development with Django.
xxxxxxxxxx
pip install fastapi uvicorn
It is always best practice to perform this in a virtual environment to avoid conflicts with other dependencies.
Afterwards, you may want to create your new FastAPI application. To do so, create a new Python file in your project (let’s call it main.py), and write the following lines of initial FastAPI code:
xxxxxxxxxx
from fastapi import FastAPI
app = FastAPI()
get("/") .
def read_root():
return {"Hello": "World"}
Now that we have FastAPI installed and our first API endpoint created, let’s proceed to the heart of the matter: running the FastAPI server from PyCharm.
You will need to configure a run/debug configuration within PyCharm. Please follow the steps below:
1. In the main menu, select ‘Run | Edit Configurations.’
2. Click on ‘+’, then choose ‘Python’.
3. Give your configuration a name (e.g., “FastAPI”).
4. In the ‘Script path’ field, browse for your Uvicorn installation script; it will likely be located in your virtual environment directory ‘/venv/bin/uvicorn’.
5. In parameter field, fill out ‘main:app –reload’. In this case ‘main’ refers to your python filename and ‘app’ refers to the FastAPI app instance.
6. Apply the changes and close the dialog.
Once the setup is completed, just use the green arrow near the dropdown where you see ‘FastAPI’, in order to run the server.
Great! You now have your FastAPI server running directly from PyCharm. Remember that every time you make changes in your FastAPI application and save those changes, the Uvicorn server will reload and will reflect your changes due to the ‘–reload’ flag in configurations.
This should cover all the necessary steps, but if your setup proves particularly troublesome or unorthodox, consider checking into FastAPI and PyCharm’s respective documentation for additional troubleshooting advice:
– FastAPI Documentation
– PyCharm Documentation
The process may seem elaborate initially, but once accomplished, the rewards in terms of productivity and ease of work are definitely worth the effort! Happy coding!
P.S. Please note that both the process of installing FastAPI and the process of configuring PyCharm IDE to run the FastAPI server are virtually identical regardless of the operating system used—Windows, macOS, or Linux.Sure!
To run FastAPI server in PyCharm, you must follow a sequence of steps. Just remember, these are not strict rules though, but guidelines to establish an environment for running your FastAPI server with ease and effectiveness.
Step 1: Create a New Project
Start by creating a new project in PyCharm. You do this by going to the “File” menu and selecting “New Project”. Provide a suitable name for your project. In PyCharm, each project has its own virtual environment, which would make it easier isolate the packages required for your project.
Step 2: Install FastAPI and Uvicorn
FastAPI is a web framework that works based on Python3.6+ type declarations. Uvicorn, on the other hand, is an ASGI (Asynchronous Server Gateway Interface) server implementation used to serve FastAPI. To install them, go to the PyCharm Terminal and write:
xxxxxxxxxx
pip install fastapi uvicorn
The above command installs both FastAPI and Uvicorn server.
Step 3: Write Your First FastAPI Application
Create a new Python file (
xxxxxxxxxx
main.py
) in your project directory and write your first FastAPI server:
xxxxxxxxxx
from fastapi import FastAPI
app = FastAPI()
get("/") .
def read_root():
return {"Hello": "FastAPI"}
In this sample code, we’ve created a FastAPI application and defined a simple end-point (“/”) using @app.get decorator.
Step 4: Configure the Uvicorn Server
Now, let’s configure PyCharm to run Uvicorn server with our application. Navigate to the ‘Edit Configurations’ from the ‘Run’ dropdown, then press ‘+’ button to add new configuration.
– Select ‘Python’ for script path, specify
xxxxxxxxxx
main.py
as the Script path (Assuming you named your file as
xxxxxxxxxx
main.py
).
– Set Script parameters as
xxxxxxxxxx
main:app --reload
– Choose appropriate Python interpreter. Ideally pick one from the existing virtual environment.
– Save your settings.
Note that in
xxxxxxxxxx
main:app
, “main” refers to the python file where you have developed your application, and “app” refers to the FastAPI instance created.
Step 5: Run Your FastAPI Server
Finally, just hit the Run button in PyCharm and your server will start running. You should see an output similar to this in the console log:
xxxxxxxxxx
Uvicorn running on http://127.0.0.1:8000
You can open this URL in your browser to check if the FastAPI server is running correctly.
That’s it! This covers the essentials on how to run a FastAPI server using PyCharm. Keep in mind Pycharm supports debugging too. You can set breakpoints in your code and run the Uvicorn configuration in debug mode. For more details regarding FastAPI, refer to the official FastAPI documentation.There are several common issues you can encounter while running a FastAPI server using Pycharm, but here’s how they can be mitigated. Ensure understanding and apt execution by reading through these points carefully.
One of the primary steps to successfully run your FastAPI server in PyCharm is proper configuration. You’ll need to set up a Python interpreter and create a Run/Debug configuration.
xxxxxxxxxx
<ol>
<li>Open your project in PyCharms
<li>Select File -> Settings -> Project: -> Python Interpreter
<li>Add a new interpreter or select an existing one that suits your project requirements
<li> Install FastAPI and Uvicorn (FastAPI’s ASGI server) if not already installed.
</ol>
Now, it’s time to create a Run/Debug configuration:
xxxxxxxxxx
<ol>
<li>Just above your editor, click on Add Configuration
<li>Click on the '+' sign on the top left corner, to Add New Configuration
<li>Select Python from the list
<li>In the Script path input field, locate and provide the path for uvicorn
<li>In the parameters input field, give the location of your main file and the host details like: main:app --host 0.0.0.0 --port 8000
<li>Save the configuration and apply
</ol>
Sometimes, certain potential issues might disrupt your FastAPI server’s performance or prevent it from running properly on PyCharm.
1. Dependency Issues:
“ModuleNotFoundError” or “ImportError” issues often occur when certain dependencies aren’t correctly installed or their installation has been corrupted somehow. One way to mitigate and prevent this is by creating a virtual environment. You can do this directly within PyCharm before installing any modules. It’s also important to watch for version incompatibilities between FastAPI and other libraries, which can lead to errors. This problem can be solved by checking the latest compatible versions and updating them accordingly.
2. Misconfiguration:
Incorrect configuration can result in problems. For example, providing wrong file paths or missing out key configuration details could stop your FastAPI server from running. Always double-check your configurations and make sure everything is setup correctly.
3. Code Errors:
Code-related errors such as syntax errors, function errors, or inappropriate control statements used in your FastAPI server might hinder its performance. Debugging the code step by step and utilizing PyCharm’s intelligent coding assistance should help you locate and rectify these errors.
To keep your FastAPI server running smoothly on PyCharm, it’s essential to maintain clean and error-free code, install necessary modules correctly, and consistently monitor your application for any possible errors. Take advantage of PyCharm’s incredible debugging features and be proactive about troubleshooting errors as they arise and you’ll find great success with FastAPI. For additional information, refer to the official PyCharm documentation.FastAPI is a modern, fast (high-performance), web framework for building APIs with Python. It’s designed to be easy to use, while also enabling high performance with a variety of features such as:
- Automatic interactive API documentation.
- Built on standard Python type annotations.
- Easier debugging through error logging and exception handling.
- Data validation using Pydantic models.
Running A FastAPI Server In PyCharm
PyCharm IDE not only allows us to write code but it also provides features for configuring servers. Here’s how you can run your FastAPI server on PyCharm.
Step 1: Once you have your FastAPI application written (let’s say it’s in a file named main.py), open the PyCharm environment.
Step 2: Navigate to ‘Run’ -> ‘Edit Configurations’. This opens a new window where we can add a new configuration for our FastAPI server.
Step 3: Click the ‘+’ button to add a new configuration. Choose the ‘Python’ type.
Step 4: Provide a name for the configuration (Let’s say “FastAPI server”). In the Script path field, set the path of your FastAPI script (main.py).
Step 5: In the Parameters box, provide the parameter required to run a FastAPI server. Usually, it should look like something like this:
xxxxxxxxxx
run -h 0.0.0.0 -p 8000
Here, ‘run’ points to the main function to execute when running your application. Host ‘-h 0.0.0.0’ allows the server to accept requests from any IP address while Port ‘-p 8000’ sets the port at which your server will run.
Step 6: Before saving, ensure the Interpreter option is configured to correspond to the interpreter you’ve installed FastAPI on. Then, click the ‘OK’ button to save these settings.
Now you can easily start your FastAPI server by choosing your newly created configuration and clicking the ‘Run’ button. The server will now be accessible within your local network at a designated port.
However, although PyCharm is a highly efficient IDE when it comes to handling all the needs of developers, it must be noted that not all FastAPI features are perfectly integrated with PyCharm interface JetBrains tracker. The support of type hints for routes is limited and the current limitation impact usage of python decorators inside Route declaration though PyCharm has an outstanding feature that enables auto-reload whenever changes are made to files, known as ‘Hot Reload’.
Despite these minor hiccups in its integration, FastAPI combined with PyCharm still forms the preferable programming pair due to FastAPI’s speed and Pycharm’s power-packed functionalities. While writing RESTful APIs, FastAPI’s automatic interactive API documentation proves highly beneficial; while Pycharm’s customizable interface, extensive array of plugins, robust version control integrations & productive tools make it easy to navigate, debug and visualize code. Together they excel in providing more straightforward and productive development experience.API (Application Programming Interface) testing is crucial in software development, helping to ensure that your applications behave as expected. FastAPI, a modern and fast Python web framework, provides built-in tools for API creation and testing. Combined with the powerful features of PyCharm, it becomes a robust platform for conducting API testing.
Running a FastAPI server using PyCharm involves a few steps:
## Setting up Your Project Environment
Before running your FastAPI server, you need to ensure that your project environment is properly configured in PyCharm.
1. Go to “File” – then choose “Settings”.
2. In the Settings dialog box that pops up, click on “Project: [Your Project Name]”.
3. Choose the correct interpreter from the dropdown. If you need to add a new one, select “Show All…” then click on “+” and add your interpreter accordingly.
## Running the Fast-Api Server
After setting up your environment, you’re ready to run your FastAPI application. Here’s how:
xxxxxxxxxx
from fastapi import FastAPI
app = FastAPI()
get("/") .
def read_root():
return {"Hello": "World"}
This simple sample code declares an instance of FastAPI. It includes a route (“/”) which will respond to get requests with a JSON response {“Hello”: “World”}.
To run this server, open your terminal and navigate to the directory of your script. Type in:
xxxxxxxxxx
uvicorn main:app --reload
In this command, “main” represents your file name (replace with your actual Python file name), while “app” stands for the name of your FastAPI instance (if you named it differently, replace “app” accordingly).
Notice the “–reload” flag, it enables hot reloading. This means your server will automatically update if it detects changes in your source code.
In PyCharm, the “Terminal” tab located at the bottom of your workspace allows you to run these commands.
Once the server is running, you can witness it live at http://127.0.0.1:8000/. To interact with your API, use http://127.0.0.1:8000/docs or http://127.0.0.1:8000/redoc pages, powered by FastAPI.
With the combination of PyCharm’s testing tools and FastAPI’s built-in test client, you can easily send HTTP requests to your application and assert responses. Here’s an example:
xxxxxxxxxx
from fastapi.testclient import TestClient
client = TestClient(app)
response = client.get("/")
assert response.status_code == 200
assert response.json() == {"Hello": "World"}
This code uses FastAPI’s TestClient to send a GET request to the root (“/”). The subsequent lines are assertions checking the response status code (200 indicates success) and content (the JSON data we defined earlier).
FastAPI makes it relatively straightforward to build APIs and conduct corresponding tests. PyCharm complements FastAPI through providing a conducive environment for executing commands and running scripts. By mastering the usage of both tools, API testing becomes a productive and efficient process for developers.Pycharm, an Integrated Development Environment (IDE), is admired for its proficiency and wide-ranging features it brings on board for Python developers. Now, running the FastAPI server using this IDE requires you to configure specific settings to get the most optimal performance. Let’s take a look at some of the crucial settings in PyCharm to run a FastAPI server seamlessly:
1. Interpreters:
The foremost requirement is to set up the project interpreter. This will allow Pycharm to understand and interpret your FastAPI application justly. To do this:
– Go to
xxxxxxxxxx
File > Settings > Project: [your_project] > Python Interpreter
.
– Add the new environment by pressing the plus (+) button. Select “Virtualenv Environment”.
– In the “New environment” using the field types, choose “Python 3.x”, check the box to inherit global site-packages.
2. Run/Debug Configurations:
To run an application on PyCharm, create a new Run Configuration. This can be tweaked to better suit your needs. Here’s how:
– Navigate to
xxxxxxxxxx
Run > Edit Configurations...
.
– Add a new Python configuration by clicking on the (+) button and selecting “Python”.
– Name the configuration to something like “FastAPI”.
– In Script path, input the location of the FastAPI application on your system.
– Set the Parameters field to
xxxxxxxxxx
run main:app --reload --workers 1 --host 0.0.0.0 --port 8000
.
– The parameter
xxxxxxxxxx
--reload
lets the server restart upon code changes.
– Parameter
xxxxxxxxxx
--workers 1
restricts the number of simultaneous workers. As suggested by FastAPIwebsite, the number of workers should not exceed the number of CPU cores.
– Parameter
xxxxxxxxxx
--host 0.0.0.0
allows connections from any IP, useful when testing through containers or external devices.
– Parameter
xxxxxxxxxx
--port 8000
defines the port where FastAPI server will run.
Command Line Options | Description |
---|---|
–reload | Restarts the server upon code changes |
–workers | Number of concurrent worker processes |
–host | Network address to bind to. Setting as 0.0.0.0 allows connections from all IPs |
–port | Port where FastAPI server will run |
3. Virtual environment:
Create a virtual environment, ensuring libraries you import don’t conflict with libraries in other projects.
– Go to
xxxxxxxxxx
File > Settings > Project [Your project name]
.
– On the drop-down menu next to “Project Interpreter,” select the appropriate interpreter.
Include the following initialization file in the root directory of your FastAPI server project:
xxxxxxxxxx
from fastapi import FastAPI
app = FastAPI()
get("/") .
def read_root():
return {"Hello": "World"}
Now, run the FastAPI application in PyCharm by clicking on the green arrow in the toolbar.
Remember to maintain clean, readable code, use comments where necessary, regularly commit to a version control system, and ensure required security measures. SEO-wise, this will enhance your application’s visibility, usability, reinforcing its robustness. Happy coding!To successfully run FastAPI server using PyCharm, simply follow these steps:
- Firstly, make sure that you have your environment set up correctly. Having the right version of python installed and the PyCharm IDE properly configured would be a good start.
- Next, install FastAPI on your system. If it’s not already installed, you can do this by going to terminal or command line and typing
xxxxxxxxxx
111pip install fastapi
.
- You will also need to install an ASGI server, like uvicorn. You can do this in a similar way to installing FastAPI – on your terminal or command line type
xxxxxxxxxx
111pip install uvicorn
.
- Once FastAPI and uvicorn are both installed, you’ll need to create a new FastAPI project. This is relatively straightforward with PyCharm – when creating a new project, select FastAPI from the list of available templates.
- The next step is to write your API endpoint. In a FastAPI application, a path operation is defined by a Python function. These functions can be asynchronous and they follow a specific format.
- After writing out your API, simply go back to PyCharm, Right click and select Run. It will start running on uvicorn server which is made for ASGI and it starts running FastAPI automatically.
When followed step by step, these guidelines should allow anyone who is somewhat proficient in Python and familiar with PyCharm IDE to successfully run the FastAPI server.
Remember, errors may arise during execution but thankfully, FastAPI offers detailed error messages in JSON format. This will help you troubleshoot any areas of the code that may not be functioning as expected.
If you’re interested in taking a deep dive into understanding how FastAPI works, I recommend their official documentation. They break it down step by step, offer robust examples, and even show you how to implement real-world applications.
FastAPI is one of the fastest (no pun intended) frameworks out there for building APIs with Python and it offers excellent support for modern, highly-demanding applications. By effectively utilizing its features in combination with the powerful PyCharm IDE, you’ll be able to produce high-quality, efficient, and scalable web applications.