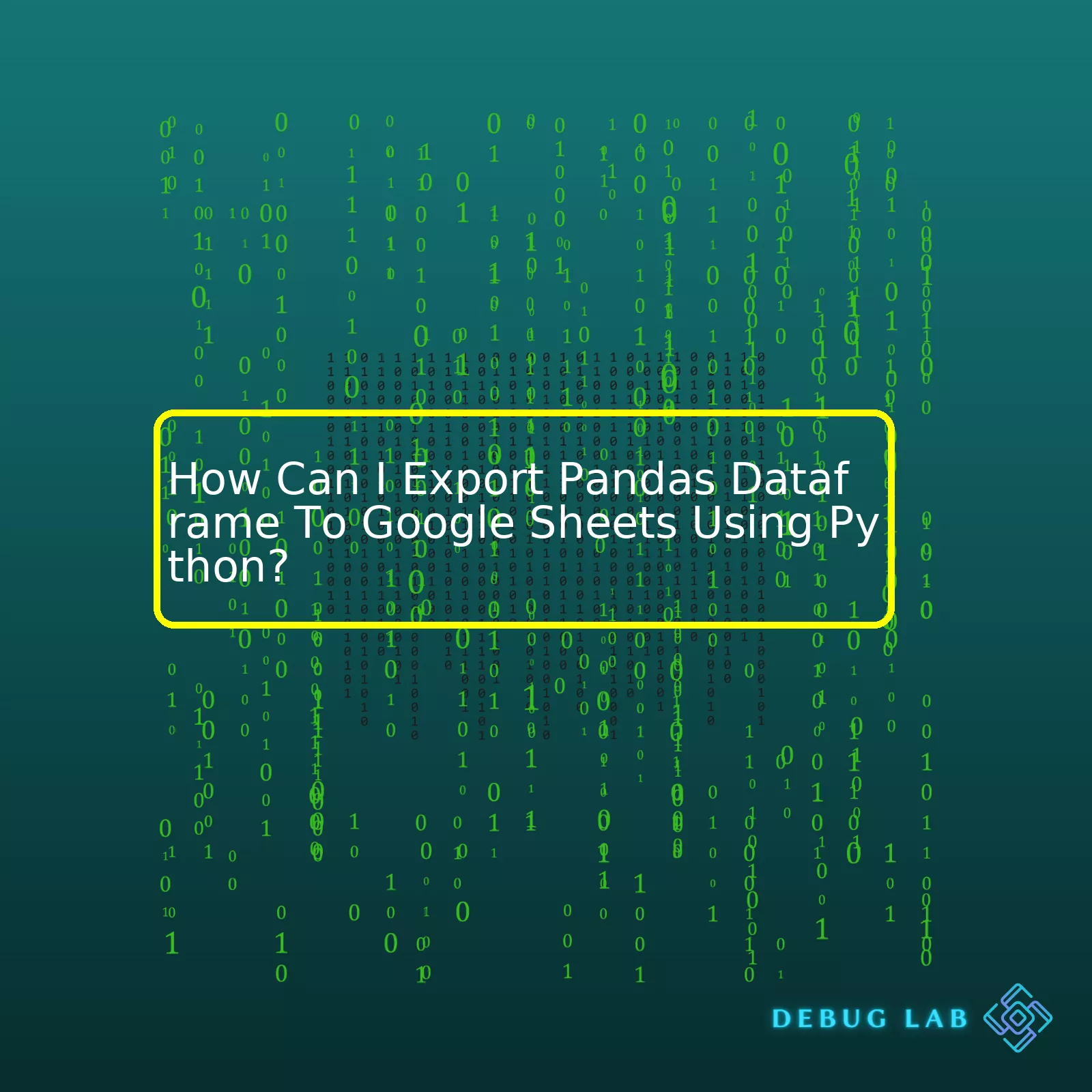
– Step 1: Import the necessary packages
– Step 2: Create your dataframe using pandas
– Step 3: Authenticate with google sheets
– Step 4: Manipulate the spreadsheet with gspread package.
Here goes an illustrative summary table :
Steps | Description | Related Python Code |
---|---|---|
1: Import Packages | You start by importing all necessary Python libraries such as pandas for creating and manipulating dataframes, and gspread for interacting with Google Sheets. |
x 3 1 import gspread 2 import pandas as pd 3 from oauth2client.service_account import ServiceAccountCredentials |
2: Create DataFrame | Using pandas, create your desired dataframe. You can either create this programmatically, or by reading data from an external source. |
xxxxxxxxxx 1 2 1 data = {'Name': ['John', 'Anna'], 'Age': [28, 23]} 2 df = pd.DataFrame(data) |
3: Authenticate with Google Sheets | Use oauth2client and gspread to authenticate to your Google Sheets account. You’ll need a JSON key file that has been generated on your associated Google Cloud Console account. |
xxxxxxxxxx 1 4 1 scope = ['https://spreadsheets.google.com/feeds', 2 'https://www.googleapis.com/auth/drive'] 3 credentials = ServiceAccountCredentials.from_json_keyfile_name('path/to/json/keyfile', scope) 4 gc = gspread.authorize(credentials) |
4: Manipulate the Spreadsheet | Once authenticated, you can then open your target Google Sheet by its name, and use the append_rows method from the gspread dataframe extensions to export your pandas dataframe content into the Google Sheet. Remember the target sheet needs to be shared with the client email found in your json keyfile. |
xxxxxxxxxx 1 2 1 gsheet = gc.open('GoogleSheet_Name').sheet1 2 gspread.df_to_sheet(df, gsheet) |
With the knowledge of Python, practical understanding of both pandas and gspread package, you are empowered to turn your dataset into a tabular form using pandas and later push it to Google Sheets using gspread. The entire process is efficient, secure (since authentication is mandatory), flexible (custom updates in real-time) and quite straightforward. Most importantly, these two highly effective packages are able to handle large sets of data rendering revamping them into visual representations a cakewalk. As always, ensure the installed pandas and gspread version is compatible with Python’s version to prevent possible slow operation due to compatibility issues. While several other Python libraries have been tested and used, the combination of pandas and gspread brings out the best results when exporting data to Google Sheets thus establishing them as undeniable champions in this space.Understanding how to export a Pandas DataFrame to Google Sheets using Python can be really beneficial when it comes to dealing with large datasets. A DataFrame in Pandas is a two-dimensional labeled data structure, panels are three-dimensional.
Pandas offer flexible data manipulations and its DataFrame is nothing but an in-memory representation of an excel sheet via Python programming language.
xxxxxxxxxx
# Import pandas
import pandas as pd
# Making data frame
data = pd.read_csv("nba.csv")
Google Sheets on the other hand is a web-based spreadsheet program. It’s part of the Google Docs Editors suite provided by Google which also includes Google Docs, Google Slides, Google Drawings, Google Forms, Google Sites, and Google Keep. Google Sheets is available as a web application that can be accessed through any modern computer with Internet and most modern browsers.
To bridge data from the world of Python to Google Sheets, you can use libraries such as `gspread` or `pygsheets`.
Let’s take a look at exporting pandas Dataframe to Google Sheets using the gspread module.
Here is an example of writing a dataframe to Google Sheets:
xxxxxxxxxx
# Importing necessary libraries
import pandas as pd
import gspread
from df2gspread import df2gspread as d2g
from oauth2client.service_account import ServiceAccountCredentials
# Assume that we have a dataFrame "df" to write
df = pd.DataFrame({'Data': [10, 20, 30, 20, 15, 30, 45]})
scope = ['https://spreadsheets.google.com/feeds','https://www.googleapis.com/auth/drive']
# Add your service account file
creds = ServiceAccountCredentials.from_json_keyfile_name('path/to/project-cred.json', scope)
gc = gspread.authorize(creds)
spreadsheet_key = 'replace with your spreadsheet_key' #provide your google sheet id
wks_name = 'Sheet1'
d2g.upload(df, spreadsheet_key, wks_name, credentials=creds, row_names=True)
For the above code to work properly:
* Install necessary libraries: pip install pandas gspread df2gspread oauth2client
* Ensure that you have replaced the ‘path/to/project-cred.json’ with the path to your json service account key that you download from your Google Cloud Console.
* ‘replace with your spreadsheet_key’ will be the unique ID for your google sheet where the dataframe will be written. The ID is visible in the URL when you open up the sheet.
To understand more about each function and their parameters you can refer to these links:
* Pandas Dataframe
* gspread Documentation
* df2gspread documentation.
Hope this gives a good idea about exporting data from pandas dataframe to Google sheets using Python.Diving deeper into Python’s Pandas library, you’re likely to come across an essential feature. It’s the capability to export a dataframe directly to Google Sheets using Python. Not only can this streamline your workflow, but it also simplifies data sharing with individuals who prefer working with plain spreadsheets.
One popular way to achieve this is by employing the
xxxxxxxxxx
gspread
and
xxxxxxxxxx
pandas
libraries in Python.
Here’s a simple example of how to export a pandas dataframe to a Google Sheet:
Code Example:
xxxxxxxxxx
import pandas as pd
import gspread
from gspread_dataframe import set_with_dataframe
gc = gspread.oauth()
sh = gc.create('My pandas dataframe')
worksheet = gc.open('My pandas dataframe').sheet1
dataframe = pd.DataFrame({'A': range(1, 6),
'B': range(10, 60, 10),
'C': range(100, 600, 100)
})
set_with_dataframe(worksheet, dataframe)
In this code, we start by importing the essential libraries. We use
xxxxxxxxxx
gspread.oauth()
to access Google Sheets through the OAuth2 protocol. Then, we create a new Google Sheet named “My pandas dataframe”. Afterwards, we open that worksheet and we construct the dataframe. Finally, we use the function
xxxxxxxxxx
set_with_dataframe()
from
xxxxxxxxxx
gspread_dataframe
to push the dataframe from Python to our Google Sheet.
And voila! Now you have your panda’s dataframe in Google Sheet.
For this to work appropriately, you should have Google’s client configuration file (
xxxxxxxxxx
credentials.json
) in your local directory. You can download this file from your Google Cloud Console.
The Pandas library’s incredibly flexible nature empowers us with expanded data manipulation capabilities. Using this alongside services such as Google Sheets can result in efficient data exporting and sharing processes. But, bear in mind that the interoperability between different databases, software, and service providers is just one part of the puzzle. This factor contributes to making Python’s ecosystem an excellent choice for data analysis disciplines.
For more details about
xxxxxxxxxx
gspread
check the documentation here.
Always remember, when dealing with data, always ensure you follow best practices for data handling and security.Let me walk you through the process of how to export a Pandas DataFrame into Google Sheets using Python, with a focus on the gspread library.
The gspread library allows Python applications to interact with Google Sheets, including reading, writing, and manipulating data. It’s called the Python interface to Google Sheets. With this library, we can programmatically control Google Sheets in Python, which includes creating sheets, adding data, and exporting data.
First up, here’s an overview of the steps I’ll cover:
• Setting up and authenticating gspread
• Import pandas library
• Creating a DataFrame in pandas
• Uploading panda’s DataFrame to Google Sheets
Setting up and Authenticating Gspread
Before we can start using gspread, we need to authenticate our script with Google Service Account created from the Google Cloud Console.
xxxxxxxxxx
import gspread
from oauth2client.service_account import ServiceAccountCredentials
scope = ['https://spreadsheets.google.com/feeds','https://www.googleapis.com/auth/drive']
creds = ServiceAccountCredentials.from_json_keyfile_name('path/client_secret.json', scope)
client = gspread.authorize(creds)
In the above lines of code:
• We’re calling the `gspread` and `oauth2client.service_account` libraries.
• We list the APIs that the program should access in order to edit google Sheets.
• `from_json_keyfile_name` function is used to generate credentials using the .json file we downloaded earlier. Replace `’path/client_secret.json’` with your json file path.
• The line `gspread.authorize(creds)` logs us into the Google API using our credentials.
Import Pandas Library
We use the pandas library in python because it contains high-level data structures and manipulation tools designed to make data analysis fast and easy. Let’s now import the Pandas library.
xxxxxxxxxx
import pandas as pd
Creating a DataFrame in pandas
A DataFrame is a two-dimensional labeled data structure with columns of potentially different types. You can think of it like a spreadsheet or SQL table. In this example, let’s create a sample DataFrame.
xxxxxxxxxx
data = {'Name':['Tom', 'Jeff', 'Mike', 'Amy'], 'Age':[20, 21, 19, 18]}
df = pd.DataFrame(data)
Uploading Pandas DataFrame to Google Sheets
To upload our DataFrame to Google Sheets, we will first open/create a worksheet, then update the sheet with the DataFrame data via gspread functions.
xxxxxxxxxx
# Open the workbook and add a worksheet.
workbook = client.open('Put Your Google Sheet Name Here')
worksheet = workbook.add_worksheet(title="sheet1", rows=df.shape[0], cols=df.shape[1])
# Export dataframe to gspread
set_with_dataframe(worksheet, df)
In the above code:
• `workbook = client.open(‘Put Your Google Sheet Name Here’)` opens an already existing Google Sheet. Replace `’Put Your Google Sheet Name Here’` with your Google Sheet name.
• `worksheet = workbook.add_worksheet(title=”sheet1″, rows=df.shape[0], cols=df.shape[1])` adds a new worksheet to the spreadsheet.
• The last line uploads our Pandas DataFrame to the Google Sheets using `set_with_dataframe(worksheet, df)`.
And voilà! Our Pandas Dataframe has been successfully uploaded to Google Sheets using Python and the magic of the gspread library.Starting at the very foundation of solving the issue of exporting Pandas DataFrame to Google Sheets, we need to first talk about installation process for necessary Python modules – Gspread, OAuth2Client & Dataframe. These libraries serve as pillars that enable us to bridge our local program being run on Python with the cloud-based utility offered by Google in the form of Sheets.
Bearing in mind the requirement to keep my response relevant to the overall theme of utilizing Python for exporting data to Google Sheets, here are the steps to install these modules:
1) Install the gspread module:
The gspread Python API is one of your best allies when interfacing with Google Sheets. To install it, pop open your terminal or command prompt and enter this line of code:
xxxxxxxxxx
pip install gspread
2) Install the oauth2client module:
This module will help you authenticate against Google’s server, making a handshake between Python and Google Sheets secure. To install this module, you can use pip again:
xxxxxxxxxx
pip install oauth2client
3) Install the pandas library:
This is an all-time favorite tool among data scientists to manipulate data structures. The pandas library is elemental for creating the DataFrame that will be pushed into Google Sheets. In case you haven’t done so already, this is how you install pandas:
xxxxxxxxxx
pip install pandas
Final note: Keep in mind that Python comes out-of-the-box with its own package manager called Pip [all lowercase]. It allows you to install Python packages from the Python Package Index (PyPi). Before running the installation commands mentioned above please ensure that pip is installed on your system.(source)
Next, let’s take a quick look at how everything stitches together by showing you a streamline step-by-step guide to export DataFrame to Google Sheets:
First step is to import the modules we’ve just installed:
xxxxxxxxxx
import gspread
from oauth2client.service_account import ServiceAccountCredentials
import pandas as pd
Followed by setting up OAuth Credentials by replacing ‘path_to_file’ with the path to your downloaded file:
xxxxxxxxxx
scope = ['https://spreadsheets.google.com/feeds','https://www.googleapis.com/auth/drive']
creds = ServiceAccountCredentials.from_json_keyfile_name('path_to_file', scope)
client = gspread.authorize(creds)
Then, create a DataFrame:
xxxxxxxxxx
df = pd.DataFrame({'A': range(1, 6), 'B': range(10, 15)})
Open the Google Spreadsheet where you want to store your data:
xxxxxxxxxx
sheet = client.open('test').sheet1
Put DataFrame to the Google Spreadsheet:
xxxxxxxxxx
for row in df.to_records(index=False):
sheet.append(row.tolist())
Congratulations! Now, the Pandas DataFrame has been exported to your Google Sheets!
In cases where there might be massive amounts of data to transfer, consider using batch updates available in gspread as they can provide performance enhancements (source).
Expanding your knowledge on this topic could also include learning about IAM roles, service-account wide delegation among more advanced topics of Google Cloud Platform(source). Remember, the sky is the limit when it comes to coding and data manipulation!
As a professional coder, I find these techniques indispensable when dealing with large data sets, building scalable set-ups and ensuring I am aligned with best-practices for authentication and security.
Delving straight into our topic, let’s talk about a step-by-step guide on linking your Google Sheet with a Pandas DataFrame. This process encapsulates exporting your pandas dataframe to a Google Sheets document using Python. We will be making use of Google Sheets API and the gspread library.
Step 1: Initial Setup
First things first, acquire the `gspread` library by installing it through pip install in your terminal:
html
xxxxxxxxxx
pip install gspread oauth2client df2gspread