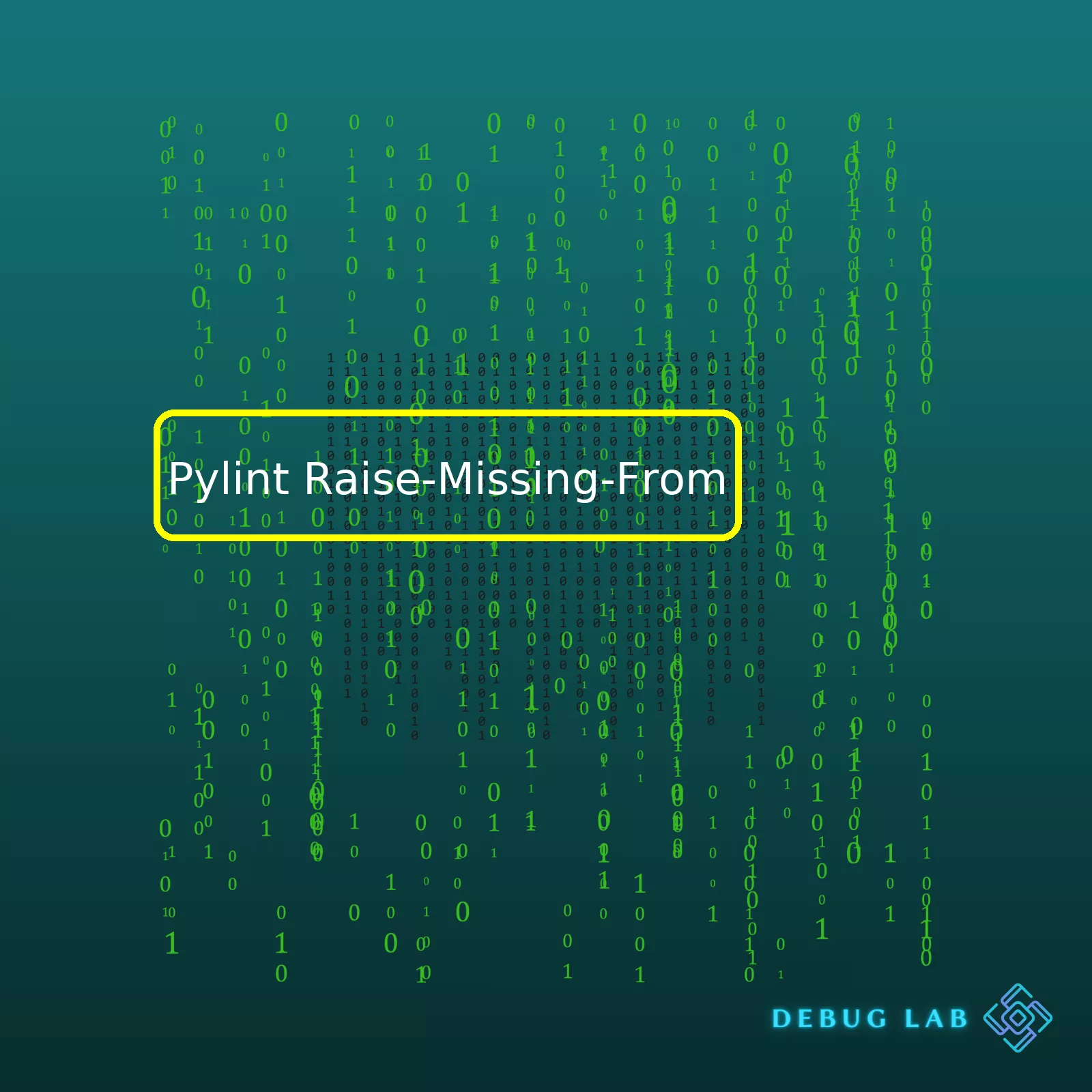
html
Pylint | Raise-Missing-From | Description |
---|---|---|
Pylint | raise-missing-from | This error occurs when there is a ‘raise’ statement in an except block without specifying a source from which the error was detected. |
html
Pylint | Raise-Missing-From | Description |
---|---|---|
Pylint | raise-missing-from | This error occurs when there is a ‘raise’ statement in an except block without specifying a source from which the error was detected. |
The
raise-missing-from
warning in Pylint occurs when a ‘raise’ statement is used in the ‘except’ block, and no source of the original error is specified with a ‘from’. Python 3 introduced a new way of raising exceptions: the ‘from’ keyword allows you to attach the original traceback information to your custom exception handling, providing more context on what may have caused it.
Raising an error without preserving its context can make debugging harder, as the original error location and stack trace get lost. It’s a best practice to use the ‘from’ keyword when re-raising caught exceptions.
Using the ‘from’ construct helps propagate the original traceback, making the exception chain clear for anyone reading your logs or debugging your code. A traceback that includes the original source of the error leads developers straight to the problematic area.
When using Pylint to analyze your code, consider enabling the
raise-missing-from
warning to ensure you are not accidentally swallowing valuable traceback information in your exception handling.
A typical fix would look like the following:
try: some_error_raising_operation() except SomeError as e: raise AnotherError("Explanation") from e
You can find more information about this warning on Pylint’s official documentation page found here.Understanding the purpose of Pylint’s
Raise-Missing-From
check requires a grasp of Python’s exception handling system and the “raise from” construct, as well as familiarity with the Pylint static code analysis tool.
Pylint is a tool that analyzes Python code to identify programming errors, enforce a coding standard, and look for code smells. The Pylint
Raise-Missing-From
check is particularly concerned with the proper use of the “raise from” construct in Python.
To comprehend why it’s important, let’s delve into Python’s exception handling system. Exception handling is accomplished using the
try-except
blocks. If the code within the
try
-block causes an exception, the code in the
except
block gets executed. But what happens when you have an exception handling routine itself throws another exception? These are chained exceptions, and they can make deciphering the root cause of an issue rather difficult.
With Python 3’s “raise from” syntax, we have better transparency over these chained exceptions. Here’s how it works:
try: attempt_something() except Exception as e: raise NewException("An error occurred.") from e
In this instance, if the call to
attempt_something()
throws an exception, Python will raise the
NewException
as before, but it will also link to the original exception. It implies that when you print the stack trace, you would observe both exceptions, which provides greater context and makes debugging easier.
This leads us back to Pylint’s
Raise-Missing-From
check. This check validates that when you’re re-raising an exception within an
except
block, you are using the “raise from” syntax to preserve the original exception. If you forget or opt not to use the “raise from” syntax, you’ll get a Pylint
Raise-Missing-From
warning. This is especially useful while working with large codebases or collaborative projects where clear, debuggable error messages are vital.
Remember, readability counts in coding – and knowing the underlying cause of an exception can save hours of work and contribute significantly to your code’s maintainability.
Refer to the official Pylint documentation about controlling messages for more information on managing warnings, including the
Raise-Missing-From
check.Certainly. To efficiently collate errors and understand how context and exception chaining can be used with Pylint’s `raise-missing-from` error, we need to dissect each element and see how they fit together in the world of Python coding.
Python 3 introduced `raise … from`, a syntax that allows an exception to be raised while preserving original traceback information. This is known as PEP 3134: Exception Chaining and Embedded Tracebacks. Having tracebacks attached to each `Exception` object helps debug as it provides the full context, which means every single operation that was performed prior to the exception being thrown.
Consider this example:
def functionA(): return 42 / 0 try: functionA() except ZeroDivisionError as e: raise ValueError("Unexpected error") from e
When an exception occurs in `functionA()`, including a division by zero error, another exception, `ValueError`, is raised using `raise … from`. However, the context where ZeroDivisionError occurred isn’t lost, it becomes part of the new exception’s traceback.
Pylint jumps into the picture here. Pylint is a Python static analysis tool, meaning it reviews your code line by line for potential errors without running it. It helps maintain high standards of coding by highlighting syntax errors, redundancy, or violations of coding standards. One of Pylint’s key features is chock-full of checkers which pinpoint areas in your code that could be improved.
One of these checkers is `raise-missing-from`, which encourages **exception chaining** for better code readability and debugging.
If you’re re-raising exceptions, Pylint encourages you to include the `from` keyword, hence the name `raise-missing-from`. If you inadvertently miss out on using `from` keyword when raising an exception, Pylint will throw a warning: `raise-missing-from`.
Given the following snippet of code as an example:
try: 1 / 0 except ZeroDivisionError: raise ValueError("You tried to divide by zero!")
In this case, running Pylint would result in the `raise-missing-from` warning because the original `ZeroDivisionError` exception has been raised to a `ValueError` without preserving the context through the use of `from`. The correct approach would be:
try: 1 / 0 except ZeroDivisionError as exc: raise ValueError("You tried to divide by zero!") from exc
By utilizing `from`, you link the original exception (`ZeroDivisionError`) to the new one (`ValueError`). This preserves the original context and makes it easier to debug the code using the traceback. Juxtaposing the precedence of the issue that led to the runtime error becomes much simpler, and thus, collating errors efficiently is in reach with this pythonic practice.Pylint, a python static code analysis tool, is incredibly helpful for identifying potential issues in your Python scripts. More often than not, these warnings point out places where you can improve your code, adhere to standards, or prevent bugs from popping up down the line. Among these warnings, Pylint can often throw “raise-missing-from” issues which typically highlight instances where an exception is being raised after another exception. It recommends that these exceptions should use a “from” clause to facilitate exception chaining, making it easier to identify and debug any problems.
To resolve these issues and correct the ‘pylint raise-missing-from’, let’s first take a look at what Pylint is suggesting when it raises this warning.
What the ‘raise-missing-from’ Message Means:
Working with exceptions in Python, there may be instances where you catch a specific exception but then raise a new, different exception. For instance:
try: 1/0 except ZeroDivisionError: raise ValueError("Some value error occurred!")
In the example above, a `ZeroDivisionError` is caught and then immediately a `ValueError` is raised. However, this does nothing to preserve the original traceback of the `ZeroDivisionError`. This traceback can include vital information useful for debugging and understanding what actually went wrong.
How to Correct ‘raise-missing-from’ Issues:
To correct this ‘pylint raise-missing-from’ issue, use the `raise…from` syntax available in Python:
try: 1/0 except ZeroDivisionError as e: raise ValueError("Some value error occurred!") from e
Now the new exception is linked to the original one, forming an exception chain. This enables traceback to provide details about what led to the original error as well.
Writing code like this adheres to the PEP-3134 standard PEP-3134, which allows us to get inline information about the original exception that was raised prior to our explicitly thrown exception. This provides more context and makes debugging easier.
Finally, keep in mind that Pylint checks try to enforce good coding practices. In this case the ‘raise missing from’ message tells you that, whenever possible, you should preserve the original traceback of your errors. Thus, next time you see that Pylint error, all it takes is a little rearranging to clear up the issue!Prior to discussing `raise … from` in Python and specifically looking at `pylint raise-missing-from`, let’s start off with a brief coverage of what error handling mechanisms are. In the context of Python programming, error handling mechanisms refer to ways in which the program itself identifies, reacts to, and potentially remedies issues (or exceptions) that arise while the code is being executed.
Python provides several mechanisms for exception handling, but according to the Zen of Python –– readability counts. Hence, it’s essential to make error reporting as clear as possible. This is where the `raise … from` statement comes into play.
This statement is used when an exception in Python occurs within the except block. To clarify the cause of these new exceptions and to trace them back to the original ones, we use `raise new_exception from original_exception`.
Without `raise …from`, your traceback can be messy and misleading because the end user might not know the root cause of the exception. But with `raise … from`, traceback displays the direct cause of exception.
Here’s a simple example:
try: result = some_potentially_failing_function() except Exception as e: raise RuntimeError("An error occurred") from e
In this example, if `some_potentially_failing_function()` encounters an exception `e`, a `RuntimeError` is thrown, associating it with the original cause `e`.
Now, `pylint`, a tool that checks if a module satisfies a coding standard, introduced a new check `raise-missing-from` starting pylint version 2.6.0. When enabled, pylint will emit a warning if an active exception is available and you’re raising a new one without using `raise … from`. It’s designed to push developers to enhance their traceback messages by including the underlying causes of the errors they encounter in their program.
Hence, using `raise…from` and enabling `pylint raise-missing-from` can make your code more robust and greatly help debugging process by giving more details about the root cause of exception.
Resources:
– Python documentation – Errors and Exceptions
– Pylint What’s New 2.6.0The “raise from” concept in Python is sometimes referred to as exception chaining, and is instrumental in managing errors in a Python application in an optimal way. This capability allows exceptions to be linked together in a chain, assisting in capturing detailed error analysis especially in complex applications.
However, Pylint, a popular linting tool for Python, has a specific check for “raise-missing-from”. When turned on, Pylint will flag cases where a raise statement doesn’t explicitly use the “from” keyword to associate it with another exception.
Consider the below code snippets:
First scenario where there is no exception chaining:
try: do_something_risky() except RiskyError: logger.error("Risky operation failed!") raise MyCustomError("Something bad happened.")
In the above code, when “RiskyError” occurs, we log an error message and then raise a “MyCustomError”.
But what are we losing here?
We’re actually discarding potentially valuable information about the underlying cause of the error — specifically the traceback connected with “RiskyError”. For instance, if `do_something_risky()` failed because of a specific condition within that method, we have lost that critical information by raising `MyCustomError()` without referencing the original `RiskyError`.
This can be solved more efficiently using exception chaining, i.e., using “raise…from”. Let’s look at the updated code:
try: do_something_risky() except RiskyError as err: logger.error("Risky operation failed!") raise MyCustomError("Something bad happened.") from err
The use of “from err” in the raise statement creates a link between “MyCustomError” and the original “RiskyError”, and preserves the traceback of “RiskyError”. As such, when you debug “MyCustomError”, you will also be able to trace back the original exception that triggered it – providing comprehensive error report for you to sift through.
To put it into perspective, consider it yourself to be akin a detective, the exception chaining is your personal investigation report – detailing every little event leading up to the issue at hand.
All this being said, it is still important to realize that not all situations require exception chaining. For instance, if you’re certain that an error occurring in a given location would provide no useful context, or if your custom exception is designed to fully encapsulate any underlying error, then exception chaining might offer no benefit. Employing it appropriately and wisely is key to cleaner, more efficient coding. With that in mind, Pylint’s “raise-missing-from” check can be a very helpful tool in maintaining high quality, easily-debuggable Python code.There are several Python best practices one can employ to avoid common mistakes associated with the ‘raise’ statement, specifically in relation to the Pylint warning ‘raise-missing-from’.
1. Understand raise-missing-from: It’s important to understand what the ‘raise-missing-from’ warning is trying to communicate. This warning is raised by Pylint when it notices that ‘raise exception’ has been used without the ‘from’ keyword. The ‘from’ keyword helps to maintain a clear chain of exceptions which can give more context on where and why the first exception was raised. For instance,
def example_func(): try: 1/0 except ZeroDivisionError as e: raise ValueError("Cannot divide by zero") from e
In the above code snippet, if the division operation fails, a `ZeroDivisionError` will be raised. This exception is then captured and a `ValueError` is raised, annotated with the original exception (`ZeroDivisionError`). This allows for better debugging of the code.
2. Use the ‘from’ Keyword Properly : The next step is to ensure you’re connecting the original and new exceptions by using the ‘from’ keyword. This serves two purposes. First, it allows easy tracking of the exception trail when debugging, and it also silences the ‘raise-missing-from’ warning generated by Pylint.
def foo(): try: some_function_that_might_error() except Exception as exc: raise NewException("There was an issue.") from exc
3. Allow for Detailed Exception Messages: Where possible, make sure your exceptions have detailed messages that clarify the context in which the issue occurred. This benefits your future self or another developer when they’re troubleshooting the issue.
def foo(): try: perform_division() except ZeroDivisionError as e: raise ValueError("The denominator of a division operation was zero.") from e
4. Avoid Silencing Exceptions: One common mistake with using the ‘raise’ statement is silencing the preceding exceptions. By raising a new exception intentionally, the traceback of the original one can get lost. Always use the ‘from’ keyword to keep track of the full sequence of events leading to the final exception.
By following these steps, typical mistakes associated with the ‘raise’ statement and the resulting ‘raise-missing-from’ warnings can be prevented. Not only will this lead to higher-quality code that conforms to PEP 8 and other formatting standards, but it will also improve the debuggability of applications, making development and maintenance simpler and more efficient.
Note: Pylint’s documentation is a great resource to gain deeper insights into types of warnings and how to resolve them.
Python exception chaining is a mechanism that allows you to link exceptions so that they carry forward essential traceback information from the original error, maintaining critical context. When using Pylint, a tool that checks for errors in Python code, “raise-missing-from” is a useful feature that helps ensure such linking of tracebacks happens correctly. This checker will warn you if a
raise
statement might benefit from explicitly including a
from
clause to enhance debugging.
Table of Contents
ToggleIn Python, when an error occurs in the try block, the exception is caught in the except block. If an exception is raised in the except block without mentioning the details of the first error, it leads to loss of valuable debugging information. To avoid this, Python 3 introduced explicit exception chaining using the
raise...from
structure.
The usage goes like:
try: # some operation that may fail except Exception as e: raise RuntimeError("an error happened") from e
In this example, if an error arises, a
RuntimeError
is raised and chained with the original exception, retaining its traceback.
Pylint’s “raise-missing-from” comes into play right here. It wields linters’ ability to encourage best practices by warning programmers when their
raise
statements could benefit from explicit exception chaining. In other words, this warns you whenever there is a missed opportunity for better debugging context.
A typical implementation where Pylint would alert is:
try: # some operation that may fail except Exception: raise RuntimeError("an error happened")
The above code lacks the essential ‘from’ keyword which is suggested while dealing with exception handling in Python. Pylint’s “raise-missing-from” rule would flag this as a potential issue in the code quality report, nudging the programmer to modify the implementation consistent with the best practices.
This feature essentially promotes the use of Python Exception Chaining providing coders with superior debugging information. By following the warnings and making changes accordingly, the programmer enhances the readability, traceability, and quality of the codebase.
For more enriching details on Python Exception Handling and Pylint’s raise-missing-from feature, check the official Python documentation here and Pylint documentation here respectively.
From a coding perspective,
Pylint Raise-Missing-From
plays a vital role in Python programming. When you catch and re-raise an exception inside a
try/except
block without the
from
keyword, we miss out on integral traceback information that can prove to be useful for debugging purposes. Pylint, a static code analysis tool, helps us highlight this through the ‘raise-missing-from’ warning.
It’s crucial to understand why accounting for this could greatly improve our debugging efforts:
Pylint Raise-Missing-From
ensures proper linkage of causal relationships among exceptions. By using the keyword
from
to connect the original error with new one, we maintain clarity and enable efficient problem diagnosis.
raise NEW_EXCEPTION from ORIGINAL_EXCEPTION
delivers full context about the error chain, providing us with more comprehensive logging messages. This promotes proactive issue detection and solution implementation.
Here’s how you may typically see this applied in a Python code snippet:
try: # Some operation that may raise 'OriginalException' except OriginalException as e: # Catch the original exception raise NewException("There was a problem running the operation") from e # Re-raise a new exception and associate it with the original exception
Notice how
from e
attaches the original exception (e) to the new one (NewException).
Remember, better code readability results in more effective code maintenance. Leveraging Pylint features such as these allows coders to advance their programming practices, ensuring they are at par with established coding standards.
You can read more about Pylint’s other great features on their official website. Referencing the Python Exception Handling doc here is recommended for more details on Python exceptions and best practices.
To sum up, not only does
Pylint Raise-Missing-From
signal a red flag when we omit relevant traceback data; it also strengthens our ability to track, troubleshoot and resolve issues in our code efficiently and effectively.