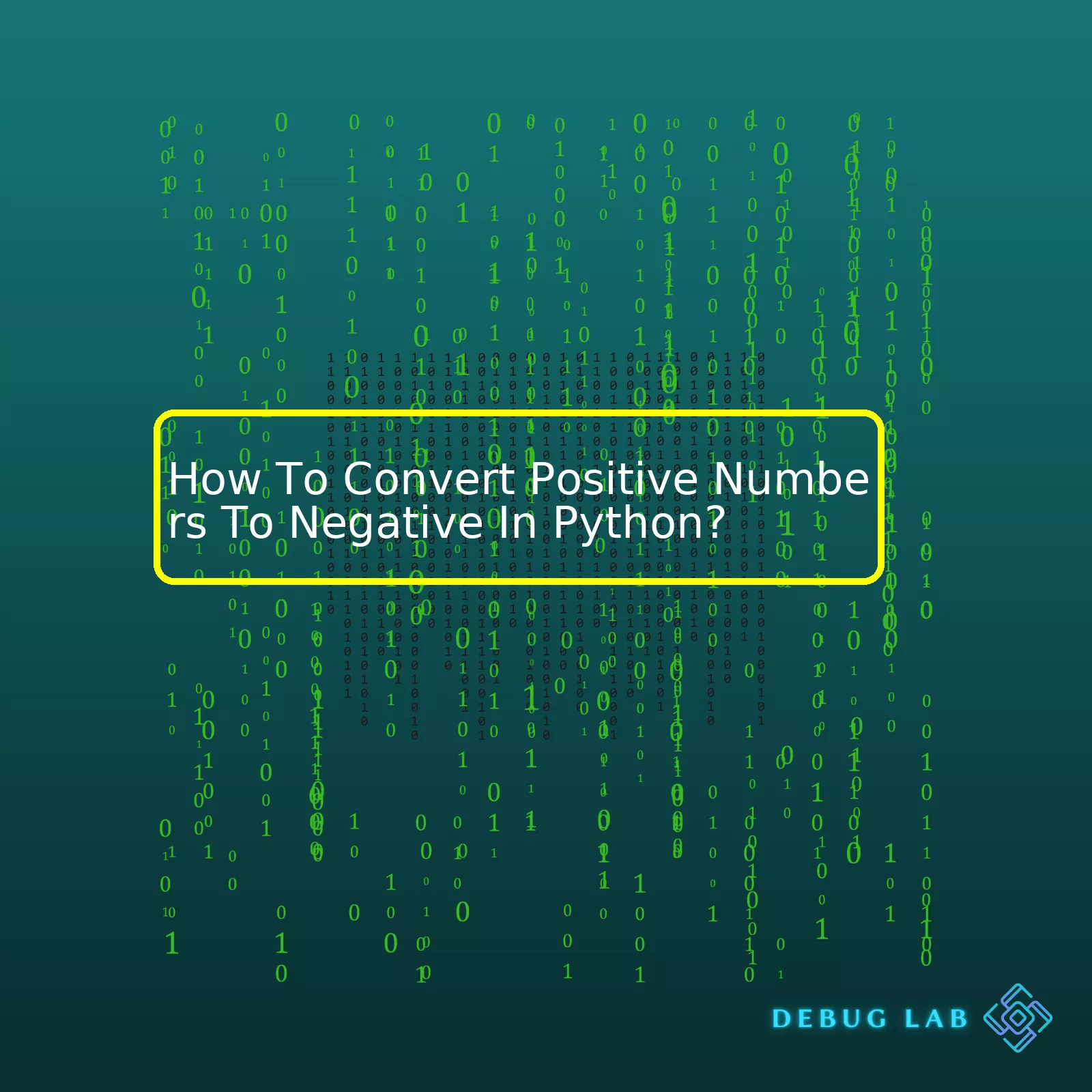
Method | Description |
---|---|
Multiplication by -1 | Multiply the given number by -1. |
Subtraction from 0 | Subtract the given number from 0. |
Built-in int function | Use the int function with a “-” sign before the number as a string. |
Now let’s dive deeper and discuss each of these methods in Python for converting positive numbers into negative.
• Multiplication by -1: This is quite straightforward; we just need to multiply the given number by -1. For instance, if we have a variable `x = 10`, to make it negative, we should simply multiply it with -1, like this:
x = x * -1
So now `x` will be -10.
• Subtraction from 0: Another simple method to convert a positive number to a negative number is through subtracting it from 0. If we have a variable `x = 20`, we can make it negative by subtracting it from 0, like so:
xxxxxxxxxx
x = 0 - x
So now `x` will be -20.
• Built-in int function: The last method involves the built-in int function in python. We convert the number into a string (by enclosing it within quotes), then place a “-” sign before it, and finally, parse it back into an integer using the int function. Suppose we have a variable `x = 30`, we can make it negative thusly:
xxxxxxxxxx
x = int('-' + str(x))
And consequently, `x` will be -30.
Although all three methods work perfectly, the first two are more recommended because they don’t require converting variables to other data types and back, hence saves on processing time and resources.(source).Python is not an exception when it comes to utilizing the arithmetic concept of negative numbers. It fully supports the inclusion and manipulation of negative integers in written scripts. Indeed, Python provides multiple methods for converting positive numbers into negative, presenting a degree of flexibility that could be highly relevant to numerous use cases or applications.
A simple option is flipping the sign of a positive number by using the negation operator
xxxxxxxxxx
-
. There’s no need to import any additional Python modules. If we have a variable
xxxxxxxxxx
a = 5
, we can just do:
xxxxxxxxxx
a = 5
negative_a = -a # This will now be -5
This allows you to instantly flip the value of
xxxxxxxxxx
a
from positive to negative. This same negation operator also works for variables containing negative numbers, effectively turning them back into positive.
Another useful approach involves the built-in Python function
xxxxxxxxxx
int()
. We employ the following code structure:
xxxxxxxxxx
a = 5
negative_a = -int(a) # This results in -5
Apply the
xxxxxxxxxx
abs()
function if you want to ensure the conversion of only absolutely positive values into their respective negative form. The
xxxxxxxxxx
abs()
function turns any number into its absolute value:
xxxxxxxxxx
a = -5
absolute_a = abs(a) # This results in 5
negative_a = -abs(absolute_a) # This results in -5
In a nutshell, Python retails the universal arithmetic applicability of negative numbers and extends supportive features to convert positive numbers into negative ones. By toggling the
xxxxxxxxxx
-
operator before the numeric variable or employing the built-in
xxxxxxxxxx
int()
function complemented with simpler arithmetics, users can achieve this objective swiftly and without obstacles. Moreover, developers can use the
xxxxxxxxxx
abs()
function to convert a number to absolute before proceeding to make it negative.
References:
The art of flipping figures from positive to negative and vice versa is a cinch in Python due to the unary operator. The unary operator, represented by a “-“, can be used for converting positive numbers into their negative cohorts.
So efficiently, what we always do is prefix the unary operator ‘-‘ before a positive number or an expression that results in a positive figure, transforming it instantaneously into a negative one.
Let’s reason through a simple illustrative example:
xxxxxxxxxx
positive_number = 25
negative_number = -positive_number
print(negative_number)
In the instance given above, if you were to run this program, the output would be -25. Here, I have prefixed the unary operator before a variable holding a positive number to swivel it into a negative number.
This principle efficaciously works even when we’re dealing with variables holding numeric values. Take note that the unary operator doesn’t modify the original variable but creates a new negated value derived from the initial data.
The secret lies not only in rudimentary conversion but also extends to more complex numerical expressions. For instance:
xxxxxxxxxx
x = 5
y = 10
negative_result = -(x + y)
print(negative_result)
Running the code snippet given above results in an output of -15. This scenario depicted here yields the sum of x and y variables, but thanks to our handy unary operator, the final result is displayed as a negative number.
An important point to chew over is that the unary operator can flip both ways – positive to negative as well as negative to positive.
For example:
xxxxxxxxxx
negative_number = -30
positive_number = -negative_number
print(positive_number)
Running the script above returns output 30. It turns around the negative number back into its positive compatriot.
Finally, don’t forget about the opportunities provided by built-in Python functions like abs(). The function abs() returns the absolute value of a number which is always non-negative. Following the abs() call with a unary operator makes the outcome negative.
xxxxxxxxxx
number = abs(32)
new_negative_number = -number
print(new_negative_number)
That’s the simplicity and elegance of Python at work! It helps turn the complex world of coding into a playground where you, the coder, are in charge.For converting positive numbers to negative in Python, the
xxxxxxxxxx
-
(negative) operator and built-in
xxxxxxxxxx
abs()
function can be utilized expertly. Emphasizing on their importance,
xxxxxxxxxx
-
is a unary operator while
xxxxxxxxxx
abs()
helps by returning absolute values, ensuring conversion stability regardless of initially inputted positive or negative numbers.
Let’s first focus on how to use the
xxxxxxxxxx
-
operator. It’s remarkably straightforward — just prefix the number with this symbol:
xxxxxxxxxx
number = 10
negative_number = -number
print(negative_number)
Executing this code would yield an output:
xxxxxxxxxx
-10
By simply attaching the minus operator before a variable containing a positive value, Python intuitively converts it into its negative counterpart.
Now, let’s explore Python’s built-in
xxxxxxxxxx
abs()
, which stands for ‘absolute’. Useful across varying scenarios, it ensures no glitches arise due to pre-existing negative value inputs.
For instance,
xxxxxxxxxx
number = -10
positive_number = abs(number)
negative_number = -positive_number
print(negative_number)
Here, the output would also be
xxxxxxxxxx
-10
. The initial negative number was smoothly converted into its absolute (or positive) version via the
xxxxxxxxxx
abs()
function. Later, we employed the negative operator to change this positive back to a negative number.
Using the
xxxxxxxxxx
abs()
function provides an extra layer of assurance because it unequivocally turns any given number into a positive one, even if it was negative initially.
To consolidate understanding, here’s a reference table summarizing aforementioned points:
Method | Code Example | Output |
---|---|---|
The Negative Operator |
xxxxxxxxxx 1 1 1 number = 10; print(-number) |
-10 |
Python Built-In abs() Function |
xxxxxxxxxx 1 1 1 number = -10; print(-abs(number)) |
-10 |
It’s vital to appreciate that proficiency in leveraging such simple functionality can significantly impact a coder’s efficiency. For further reading regarding the
xxxxxxxxxx
abs()
function and operator
xxxxxxxxxx
-
, refer to Python’s official documentationHere. This will help you uncover additional details and upgrade your Python skills graphically.
While handling large-scale data processing, databases, or mathematical operations, nuances like these often make Python the preferred language due to its power-packed simplicity and flexibility. Harnessing the best practices, like the ones discussed above, Python coders can ace most programming tasks, pushing project boundaries innovatively.Sure, let’s delve right into how to conduct number inversion in Python, with our focus specifically being on converting positive numbers to negative. This means if we start out with a positive number, say 4, we want our program to transform it into -4.
First things first, Python is well equipped to help us do this and there are several different approaches we can take.
1. Using the Unary Minus Operator:
Think about how you’d write a negative number on paper. You’d write a – sign followed by the number. The same principle applies in Python. The unary minus operator (-) negates the value of an int or float number.
Here’s how you use the unary minus operator:
xxxxxxxxxx
def convert_num_to_neg(num):
return -num
print(convert_num_to_neg(10))
In the above script, I’ve defined a method called “convert_num_to_neg” which accepts one parameter – “num”. Inside the function, we execute the operation “-num”, which simply inverts the sign of the input number, making it negative.
The print statement just tests our function with a positive input, 10. Consequently, when we run it, it should output -10.
2. Using the Multiplication Operator:
We can change a positive number to negative in Python by multiplying it by -1. If you multiply any number by -1, the result is the negative form of that number.
Let’s see how:
xxxxxxxxxx
def convert_num_to_neg(num):
return num * -1
print(convert_num_to_neg(20))
Just like before, we define a function which accepts a number as an input. But this time, instead of using the unary minus to invert the number, we’re multiplying it by -1. And remember, anything multiplied by -1 always results to that thing but in negative form.
That’s the power of Python’s versatility, providing multiple methods for accomplishing the same task.
3. Strategic Use of abs() Function:
The built-in abs() function returns the absolute value of a number. In other words, it gets rid of the sign of the number, whether it was originally positive or negative. cleverly combining the abs() function with the unary minus operator or the multiplication operator can achieve our goal.
Lets see how:
xxxxxxxxxx
def convert_num_to_neg(num):
return -abs(num)
print(convert_num_to_neg(30))
Even if the initial number was somehow negative already, applying abs() to it would make it positive, and then the unary – re-negativizes it!
Applying these techniques will enable you to easily convert any positive number to negative in Python.
+ References:
+ [Unary Operators in Python](https://www.geeksforgeeks.org/unary-operators-in-python/)
+ [Python Number abs() Method](https://www.tutorialspoint.com/python/number_abs.htm)In python programming, we often encounter situations that require the conversion of positive numbers to negative. This process generally revolve around two methods: using the built-in subtraction operator and the multiplication operator. However, these approaches still require you to explicitly transform each number. For a more efficient solution, which is especially useful when dealing with large amounts of data or complex computations, creating a function dedicated to this task can be greatly beneficial.
Let’s explore how to create an effective function that does exactly that – converts positive numbers into their negative counterpart:
The function can be created using the Python `def` keyword:
xxxxxxxxxx
def convert_to_negative(number):
return -abs(number)
This `convert_to_negative` function works by first computing the absolute value of the provided argument `number` using the `abs()` function. Since the absolute value of a number is always positive, prefixing it with a “-” sign negates it, effectively converting any passed positive number into a negative.
You can use this function to convert a positive number to its negative equivalent as follows:
xxxxxxxxxx
neg_number = convert_to_negative(10)
print(neg_number) # Output: -10
The mechanism behind this function provides you with two significant advantages:
* **Modularity**: Functions can be easily imported and reused across different modules and projects, saving coding time and effort.
* **Scalability**: You can pass in numbers directly, variables containing numbers, or even results from other computations. This makes the function highly scalable and suitable for complex programs where numbers could be coming in from various sources.
Notably, if you need to convert an array of numbers to negatives, consider leveraging Python’s list comprehension feature. It runs a lot faster than traditional for-loop iterations; hence, it becomes highly resource-efficient when dealing with large datasets. Here’s an example of how to integrate list comprehension with our function ‘convert_to_negative’:
xxxxxxxxxx
positive_numbers = [1, 2, 3, 4, 5]
negative_numbers = [convert_to_negative(num) for num in positive_numbers]
print(negative_numbers) # Output: [-1, -2, -3, -4, -5]
By employing the aforementioned strategies, we can build effective functions for automatic positive to negative conversion, thereby promoting code optimization and improved program efficiency when operating on Python.
To further equip your toolset on Python development, it’s highly recommended to explore official Python documentation on function definitions.
Moreover, practical sources like Real Python’s Function Tutorials are helpful portals to understand usage scenarios better and pick up best practices from expert community. Simultaneously, referencing open source projects and Python enthusiasts’ Github repositories can help you discover other innovative ways to enhance function creation and utilization.In our quest to understand the advanced Python concepts – Map and Lambda functions, the use-case we will be tackling today is converting positive numbers to negatives. Prior to diving into the details of how these two concepts intertwine in Python, it’s crucial to have a cursory understanding of what Map and Lambda functions are.
Lambda functions, also known as anonymous functions, are small, single-line functions declared with the keyword
xxxxxxxxxx
lambda
. These ‘disposable’ functions mainly find use when you need a non-reusable function for a short period of time.
On the other hand, the
xxxxxxxxxx
map()
function works by applying a specified function to every item in an iterable (like a list or tuple), eventually returning a list of the results.
Now, let’s illustrate their usage by converting a list of positive numbers into negative.
The solution involves creating a lambda function that changes a number to its negative value, then maps this function to each item in our list.
## EXAMPLE
my_list = [1, 2, 3, 4, 5]
negatives = list(map(lambda x: -x, my_list))
print(negatives) # outputs: [-1, -2, -3, -4, -5]