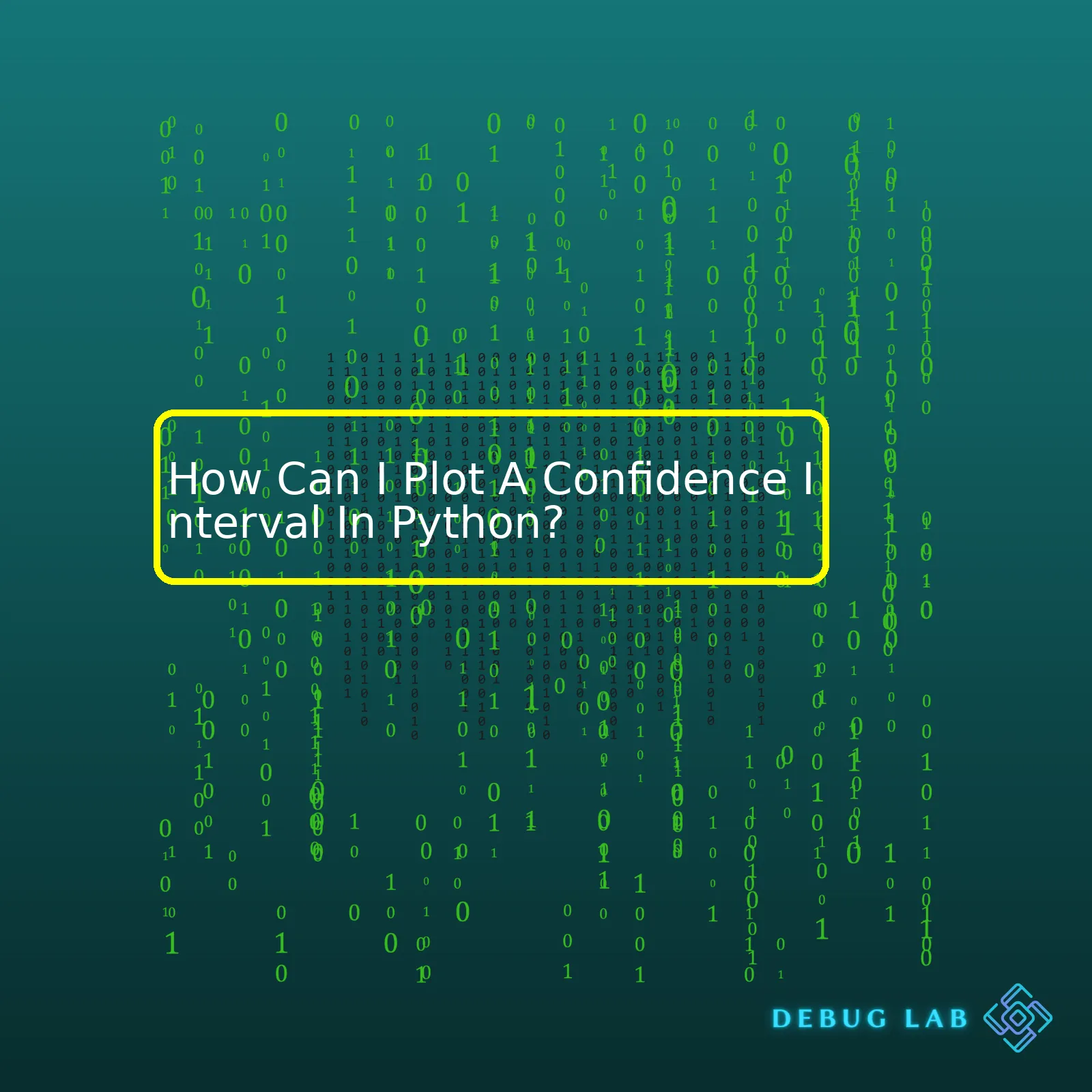
Matplotlib
,
Pandas
and
Seaborn
. The Seaborn library, primarily, offers a function named
sns.kdeplot()
that allows the creation of Kernel Density Estimation (KDE) plots, which are beneficial for illustrating probability densities. Here’s a simple example on how to generate a confidence interval in Python:
import pandas as pd import seaborn as sns import matplotlib.pyplot as plt df = pd.read_csv('your_data.csv') # Creating a KDE plot sns.kdeplot(df['Your_column'], shade=True) plt.title("Confidence Interval") plt.xlabel("X-axis label") plt.ylabel("Density") plt.show()
The following section details each step in the process by breaking it down.
Action Step | Usage Purpose |
---|---|
Importing required libraries | Pandas, Seaborn, and Matplolib are indispensable libraries for plotting data in Python. Pandas help you to manage your data, while Seaborn and Matplotlib are used for creating plots. |
Read CSV File | We read our data from a CSV file into a DataFrame using the pd.read_csv() function provided by pandas. |
Create a KDE Plot | We use sns.kdeplot() from the seaborn library to generate a KDE plot. |
Add Title and Labels | We add a title to the plot and labels to the X and Y axes using functions from matplotlib library. |
Show Plot | We call plt.show() to display our plot. If not called, the plot would be created in the memory but not displayed on the screen. |
In a nutshell, the step-by-step process above aids in generating a Kernel Density Estimation (KDE) plot, thereby drawing out the underlying probability density of a continuous variable. The KDE plot includes a shaded region, which implies that the observation lies within its bounds with a certain level of confidence – typically 95%. This is accessed via the seaborn function
sns.kdeplot()
. Besides computing and displaying visualizations such as KDE plots, seaborn performs statistical aggregation and error estimation more readily when compared to similar Python libraries.
It’s worth noting that the seaborn library also boasts an integrated
kdeplot()
function, enabling fluid distribution visualization overlaying any univariate scatterplot. While we leverage matplotlib functionalities, such as adding concise descriptions to the graph’s respective axes for reference, seaborn remains essential due to its expediency in plotting data distributions in Python.
For more advanced and robust statistical libraries that cater to robust statistical computations, consider referencing [SciPy](https://www.scipy.org/), as it provides enriched control over the underlying mathematics involved. Please refer to [seaborn’s official documentation](https://seaborn.pydata.org/generated/seaborn.kdeplot.html) and [matplotlib pyplot](https://matplotlib.org/stable/tutorials/introductory/pyplot.html) for further understanding.Confidence interval gives us a range of values which is likely to contain an unknown population parameter. Knowing this interval might be very useful in statistics when you try to make some decisions, thus knowing how to compute it might be essential for you.
Implementing it to Python? Yes, that’s also possible and pretty easy! Let me present you with the necessary steps.
First of all, we will use the
scipy
,
numpy
and
matplotlib
libraries. So you should have these pre-installed on your system to proceed. And don’t forget to import them first!
import scipy.stats as stats import numpy as np import matplotlib.pyplot as plt
Alright, let’s say we have a list of data points (or samples). We can generate these using
numpy
.
data = np.random.normal(loc=0, scale=1, size=100)
Now, we can calculate a confidence interval with the
scipy.stats.t.interval()
function. Here, the parameters are alpha (the confidence level), df (degrees of freedom, equal to the sample size minus one), loc (sample mean) and scale (standard error). The standard error can be computed with
scipy.stats.sem()
.
confidence_interval = stats.t.interval(alpha=0.95, df=len(data)-1, loc=np.mean(data), scale=stats.sem(data))
The output of the above expression is a tuple representing our desired confidence interval.
To visualize this interval, we can plot the histogram of our data, alongside two vertical lines indicating the lower and upper bounds of our confidence interval.
plt.hist(data, bins=20, density=True) plt.axvline(x=confidence_interval[0], color='red') plt.axvline(x=confidence_interval[1], color='red') plt.show()
What we will see on the screen would be a nice chart with marked confidence interval boundaries. Ta da! Now we know the boundaries of our expectation.
Usually, confidence intervals are accompanied by a point estimate. For instance, if you are dealing with the mean, t-distribution is typically used. You simply display a range of values around your mean value indicating where you believe the real (population) mean exists. However, note that this does not mean that 95% (if we consider a confidence interval of 95%) of your samples are contained within the confidence interval. Rather, it means in hypothetical sampling, 95% of your calculated intervals over time would hold the population mean value.
For more sophisticated cases like non-normal distributions or workings with proportions or variances, corresponding adjustments would need to be made, but the main idea remains the same: gather sample(s), calculate statistic(s), compute confidence interval(s), and make your inference. Confidence intervals are such powerful tools you might use in your analysis. Having learned about their implementation python, I hope they will be handy in your future coding projects.
References:
– “Scipy stats.t.interval() function” | SciPy Docs | Available at https://docs.scipy.org/doc/scipy/reference/generated/scipy.stats.t.html
– “Confidence Intervals” | StatYale | Available at http://www.stat.yale.edu/Courses/1997-98/101/confint.htmOf course! Python prides itself on being a language that fosters rapid development, partly due to its useful standard library and an extensive selection of third-party packages. When it comes to plotting confidence intervals, there are several python libraries essential for accomplishing this task.
Matplotlib:
import matplotlib.pyplot as plt
Matplotlib can be considered the grandfather of python’s visualization libraries. Excellent for producing static graphs and has an established ecosystem around it. It provides a low-level interface with lots of freedom at the cost of having to write more code.
Seaborn:
import seaborn as sns
Seaborn is built over Matplotlib and makes building more attractive plots easier. Simply use seaborn’s
sns.barplot()
, which allows you to calculate and plot a simple empirical (observed) confidence interval:
sns.barplot(x="categorical_var", y="numerical_var", data=df) plt.show()
The error bars in barplot by default show a confidence interval based around the mean.
Pandas:
import pandas as pd
Pandas helps with data manipulation and analysis. It introduces dataframes (and series), which come very handy for handling and processing structured data conveniently. You can leverage Pandas alongside Matplotlib to plot confidence intervals with ease:
df.plot(y="numerical_variable", kind='line') plt.fill_between( df.index, df['lower_bound'], df['upper_bound'], color='#539ecd', alpha=0.5 ) plt.show()
Here, ‘lower_bound’ and ‘upper_bound’ are calculated columns containing lower and upper bounds of the confidence interval.
StatsModels:
import statsmodels.api as sm
For statistical modeling, StatsModels offers many statistical tests, including functions to calculate and plot confidence intervals. Here’s an example of using statsmodels to estimate the linear regression model and then overlaying the fit plus confidence interval on top of the scatter plot:
model_fit = sm.OLS(y, sm.add_constant(X)).fit() prediction_interval = model_fit.get_prediction().summary_frame(alpha=0.10) fig, ax = plt.subplots() sns.scatterplot(x=X, y=y, ax=ax) sns.lineplot(x=X, y=prediction_interval['mean'], color='red', ax=ax) ax.fill_between(X, prediction_interval['obs_ci_lower'], prediction_interval['obs_ci_upper'], color='red', alpha=0.3) plt.show()
In this code snippet,
get_prediction().summary_frame(alpha=0.10)
returns a dataframe that contains the predicted values along with two-sided 90% prediction intervals (change
alpha=0.10
to get different intervals).
Each of these tools has a slightly different application and syntax, but when you know where to start, they are incredibly powerful. To plot a confidence interval in Python, you’ll probably want to import one or more of these libraries. Remember, practice makes perfect, so don’t be afraid to test these tools out on your datasets. Happy coding!There’s a fascinating relationship between Statistics and Python, especially when unraveling the intricate concepts such as Confidence Interval. The link might seem abstract but trust me, it’s real – and I’m here to make it enticing.
A Confidence Interval is basically a measure of the reliability of your data. Sure, anyone can collect a batch of numbers, but do you know how trustworthy they are? That’s where Confidence Interval comes into play.
Imagine pitching a new business idea. You need supporting data and fine if you have that already. But the question remains; how sure are you about the accuracy of that data? Confidence Interval answers that by providing a range of values that’s likely to contain an unknown population parameter.
The higher your confidence level (e.g., 99%), the larger your interval will be, implying greater confidence in capturing that elusive parameter. On the other hand, smaller intervals reflect lower confidence levels, revealing how vulnerable we could sometimes be to uncertainty.
But what does all this statistical jargon have to do with Python?
Well, Python happens to be one of the most sophisticated programming languages capable of plotting Confidence Intervals swiftly. So no more Sleepless nights over tedious calculations and fear of errors.
Outlining the process of plotting a Confidence Interval in Python, the steps can be summarized as:
- Importing the necessary libraries
- Creating/Loading the Data Set
- Calculating the mean and standard error
- Determining and plotting the Confidence Interval
Below is an illustration, using hypothetical data as our example:
import numpy as np import scipy.stats import matplotlib.pyplot as plt # Creating a random dataset np.random.seed(0) data = np.random.normal(0, 1, 1000) # Calculating mean and Standard Error mean = np.mean(data) std_err = scipy.stats.sem(data) # Computing Confidence Interval confidence = 0.95 interval = std_err * scipy.stats.t.ppf((1 + confidence) / 2, len(data) - 1) # Plotting the Confidence Interval plt.figure() plt.plot(data) plt.axhline(y=mean, color='k', linestyle='–') plt.fill_between(range(0,1000), (mean-interval), (mean+interval), color='b', alpha= .1) plt.title('95% Confidence Interval') plt.show()
In this example, we imported the appropriate libraries (NumPy, SciPy, and Matplotlib), created a random dataset using NumPy, calculated the mean and standard error, and eventually computed the Confidence Interval.
Lastly, we plotted the data, marking our mean with a horizontal line and shading the area within our Confidence Interval.
Ultimately, Confidence Intervals enlighten us on the reliability of our data in a statistically sound way. And Python simplifies that process, helping us visualize this fundamental concept clearly.
Haven’t I just made Statistics relevant in day-to-day coding? Now, confidence isn’t just something we possess but a tangible metric we can calculate and plot in Python.Sure, plotting a confidence interval in Python can be done easily by leveraging the power of the Matplotlib library and other statistical modules like SciPy. You’ll generally follow several steps, which I’ll break down before we put it all together:
– Retrieve or generate your data: Your dataset will determine the starting point.
– Calculate the mean: This gives you a “center” for your data.
– Compute the standard deviation: Standard deviation measures the variance of your data.
– Determine the confidence interval: Typically, we use a 95% confidence interval.
Now let’s walk through an example where we create a confidence interval plot using Matplotlib and SciPy:
Firstly, import necessary libraries:
import numpy as np from scipy import stats import matplotlib.pyplot as plt
Create some assumptions for our demo:
np.random.seed(10) data = np.random.normal(0, 1, 1000) # Creating a normal distribution with mean=0 and standard deviation=1 mean = np.mean(data) standard_deviation = np.std(data) confidence_interval = stats.norm.interval(0.95, loc=mean, scale=standard_deviation) # default is 95%
Now that we have calculated our confidence interval, it’s time to plot it. In this scenario, we will be displaying a histogram with our confidence interval:
plt.figure(figsize=(9,7)) plt.hist(data, bins=30, edgecolor='black', alpha=0.5) plt.axvline(x=mean, color='red', linestyle='--', label=f'Mean: {round(mean, 2)}') plt.axvline(x=confidence_interval[0], color='green', linestyle='--', label=f'Lower: {round(confidence_interval[0], 2)}') plt.axvline(x=confidence_interval[1], color='blue', linestyle='--', label=f'Upper: {round(confidence_interval[1], 2)}') plt.legend() plt.show()
In this completed script, you’ve plotted your data using plt.hist(), a function that generates a histogram. The pyplot module’s axvline() function adds a vertical line across the axes – in this case, representing the mean, lower bound, and upper bound of your confidence interval.
The resulting visualization clearly demonstrates your data’s average value (indicated by the red dotted line), along with the range in which 95% of all values fall (between the green and blue dotted lines).When it comes to plotting confidence intervals in Python, it’s likely that you will find various statistical libraries such as SciPy or Statsmodels. However, these libraries can often seem overwhelming and may not always be necessary for simple tasks like calculating confidence intervals and presenting them graphically.
In this case, creating a personalised function might be the better option.
First, let’s start by defining what a confidence interval is: Confidence interval gives us a range of values which is likely to contain an unknown population parameter. This range is derived from a given dataset and with a particular level of confidence (often noted by alpha).
Following examples would expound on how to create a Python function to calculate confidence intervals and another Python function to plot said intervals. We’ll use data manipulation library pandas, mathematical functions from numpy, and plotting features from matplotlib library:
import pandas as pd import numpy as np import matplotlib.pyplot as plt # custom function to calculate confidence intervals def compute_confidence_interval(data, confidence=0.95): n = len(data) mean = np.mean(data) se = scipy.stats.sem(data) margin_of_error = se * scipy.stats.t.ppf((1 + confidence) / 2., n-1) return mean - margin_of_error, mean, mean + margin_of_error # custom function to plot confidence intervals def plot_confidence_interval(data, confidence_intervals): plt.figure(figsize=(9,5)) plt.hist(data, bins=20, color='grey', alpha=0.5, label="Data") plt.axvline(confidence_intervals[0], color='red', linestyle='--', label="Lower bound") plt.axvline(confidence_intervals[1], color='blue', label="Mean") plt.axvline(confidence_intervals[2], color='red', linestyle='--', label="Upper bound") plt.legend() plt.show()
The “compute_confidence_interval” function takes two arguments: the ‘data’, in form of a list or a pandas series containing your sample observations, and the ‘confidence’ indicating the degree of confidence required for the interval. It uses functions ‘sem()’ and ‘ppf()’ from scipy library to compute standard error and percent point function which then gets used to calculate margin of error. The function returns lower bound, mean, and upper bound of the confidence interval.
Our other function ‘plot_confidence_interval’ simply creates a histogram using matplotlib.pyplot based on the input data set and marks out the lower bound, mean, and upper bound on this graphical representation.
Ensure though to check numpy’s official documentation before using its functions so that you understand their operation completely. Also, familiarize yourself with matplotlib’s functionalities by referring to the official documentation. This way not only that you are certain the function works correctly but also get to revise over some basic statistics concepts.Indeed, plotting a confidence interval in Python can be achieved easily using libraries such as Seaborn. Confidence Interval is a type of estimation computed from the statistics of observed data. It gives an interval within which a parameter is expected to lie with a certain level of confidence.
Seaborn, as a statistical visualization library, holds the ability to visualize confidence intervals efficiently. Let’s dive into understanding how you can plot a confidence interval chart in Python using seaborn:
Firstly, ensure installing the seaborn and matplotlib libraries in Python. If they’re not installed, use these pip commands:
pip install seaborn pip install matplotlib
Now that our tools are ready let’s utilize them for creating a confidence interval plot:
import seaborn as sns import matplotlib.pyplot as plt # Assuming "data" is your DataFrame and "time" & "value" are columns in it sns.lineplot(x="time", y="value", data=data) plt.show()
This script imports Seaborn and Matplotlib, then plots a line plot with a shaded area representing the confidence interval (by default, Seaborn considers it as 95%). This shaded area depicts the range where we expect the true value to fall into with a given level of confidence.
A more detailed version of this for multiple categories would involve color-coding each category differently. For example:
# Assuming "category" is another column indicating different categories in your data sns.lineplot(x="time", y="value", hue="category", data=data) plt.show()
In the above code, ‘hue’ breaks down the line plots by the specified column (in this case, ‘category’), giving different colors to lines representing different categories.
It’s crucial to note that when plotting a confidence interval of a data distribution with seaborn, underlying statistical errors may occur if your data has some outliers or doesn’t follow Normal Distribution. Handling such cases necessitate advanced techniques and usage of other libraries like scipy for defining functions that measure statistical uncertainty.
The Seaborn library provides vast built-in capabilities to deal with the complexity of statistical data visualizations allowing interpretable and attractive statistical graphics. So, confidence intervals aren’t just limited to line plots, you can incorporate them into bar plots, point plots, and more by simply passing your dataframe to corresponding Seaborn methods.
The code snippets shared are fundamental examples to plot confidence intervals with Seaborn. Of course, based on your specific requirements, adjustments will be necessary to adapt the data and desired confidence levels. Regardless, this foundation should give you a head start.Interactive visualization of confidence intervals in Python is an excellent tool to interpret and showcase your data more effectively. A highly useful library for accomplishing this task is the Bokeh library.
To utilize Bokeh for plotting a Confidence Interval, first, it is paramount to understand what a Confidence Interval (CI) is. A CI is a type of estimate computed from the statistics of the observed data. It provides an interval estimate of a population parameter and not a complete range of possible values.
There are several ways to visualize confidence intervals, but one common method is using error bars. Error bars can provide a visual representation of how much estimates of data could vary.
Here is how you could use Bokeh to generate interactive plots:
In Python, you could achieve this as:
# Install bokeh library !pip install bokeh from bokeh.plotting import figure, show, output_file from bokeh.models import ColumnDataSource, Band from bokeh.io import output_notebook output_notebook() # Sample Data x = [1, 2, 3, 4] y = [2.5, 3.5, 2, 4] lower = [1.5, 2.7, 1, 3.1] upper = [3.5, 4.3, 3, 4.9] source = ColumnDataSource(data=dict(x=x, y=y, lower=lower, upper=upper)) p = figure(x_range=(0, 5), y_range=(0, 5), title='Confidence intervals') band = Band(base='x', lower='lower', upper='upper', source=source, level='underlay', fill_alpha=0.5, line_width=1, line_color='black') p.add_layout(band) p.circle('x', 'y', source=source, size=10) output_file('confidence_intervals.html') show(p)
The above code achieves the following tasks:
– The Bokeh libraries are imported and set up for operation within the notebook environment.
– We define sample data including measurement points (x,y) and associated lower and upper bounds for the confidence interval.
– A ColumnDataSource is created that will contain our data. This class is a mapping of column names to sequences of data used as a common source for many glyphs.
– A band container is defined, with its base set as x-values, and lower and upper parameters set as the lower and upper limits for the confidence interval.
– Finally, we add the band layout to our plot and display the plot.
The resulting plot will illustrate the mean estimate and coverage area based on the confidence interval’s lower and upper boundaries. What makes this chart highly beneficial is its interactivity, allowing further analysis of data points by simply hovering over them or panning across the plot.
By using such visualization techniques, we can easily depict where our data lies, including the uncertainty of our predictions. Bokeh serves as a vital tool enabling this feature in Python; it’s convenient, powerful, and flexible in designing custom visualizations suited to individual project requirements.
For detailed understanding of each component, refer to Bokeh Documentation. With plenty of control over the final design, Bokeh undoubtedly empowers your data stories with visually appealing graphics.While graphical visualization of data analysis is often an easier way for us to understand complex trends and patterns, it can be tricky to illustrate certain statistics, like confidence intervals. However, Python, with its potent libraries such as Matplotlib, Seaborn, and SciPy, offers powerful functionalities that ease this process.
When plotting a confidence interval in Python, using **Matplotlib** is quite conventional. You first need to install it through running the command
pip install matplotlib
. Optionality of incorporating error bars by defining `yerr` parameter in the function
plt.errorbar()
eases defining upper and lower limits of a confidence interval.
import matplotlib.pyplot as plt # provided sample mean (x) and confidence interval (y, yerr) plt.errorbar(x, y, yerr=yerr) plt.show()
Elsewhere, using **Seaborn**, which is essentially a high-level interface for Matplotlib, you install via command
pip install seaborn
. The function
sns.barplot()
from Seaborn is particularly handy as it automatically calculates and plots confidence intervals.
import seaborn as sns sns.barplot(x='category', y='values', ci='sd', data=your_data_frame)
In instances where you need explicit control on how your confidence interval is calculated, then **SciPy** is the go-to library, specifically the function
scipy.stats.norm.interval()
.
from scipy import stats confidence_interval = stats.norm.interval(0.95, loc=sample_mean, scale=sigma/sqrt(n))
Remember, however, confidence intervals make sense when your sample size is reasonably large. Predictably, there are multiple ways to calculate and plot confidence intervals but mastery of these methods helps you discern which suits your use case best. So take time, have fun while at it!
For additional understanding leverage material from Python’s official documentation, or utilize popular code sharing platforms like GitHub and Stack Overflow where global developers share their Python code snippets reliably illustrating varied data analytical visualizations including plotting confidence intervals.