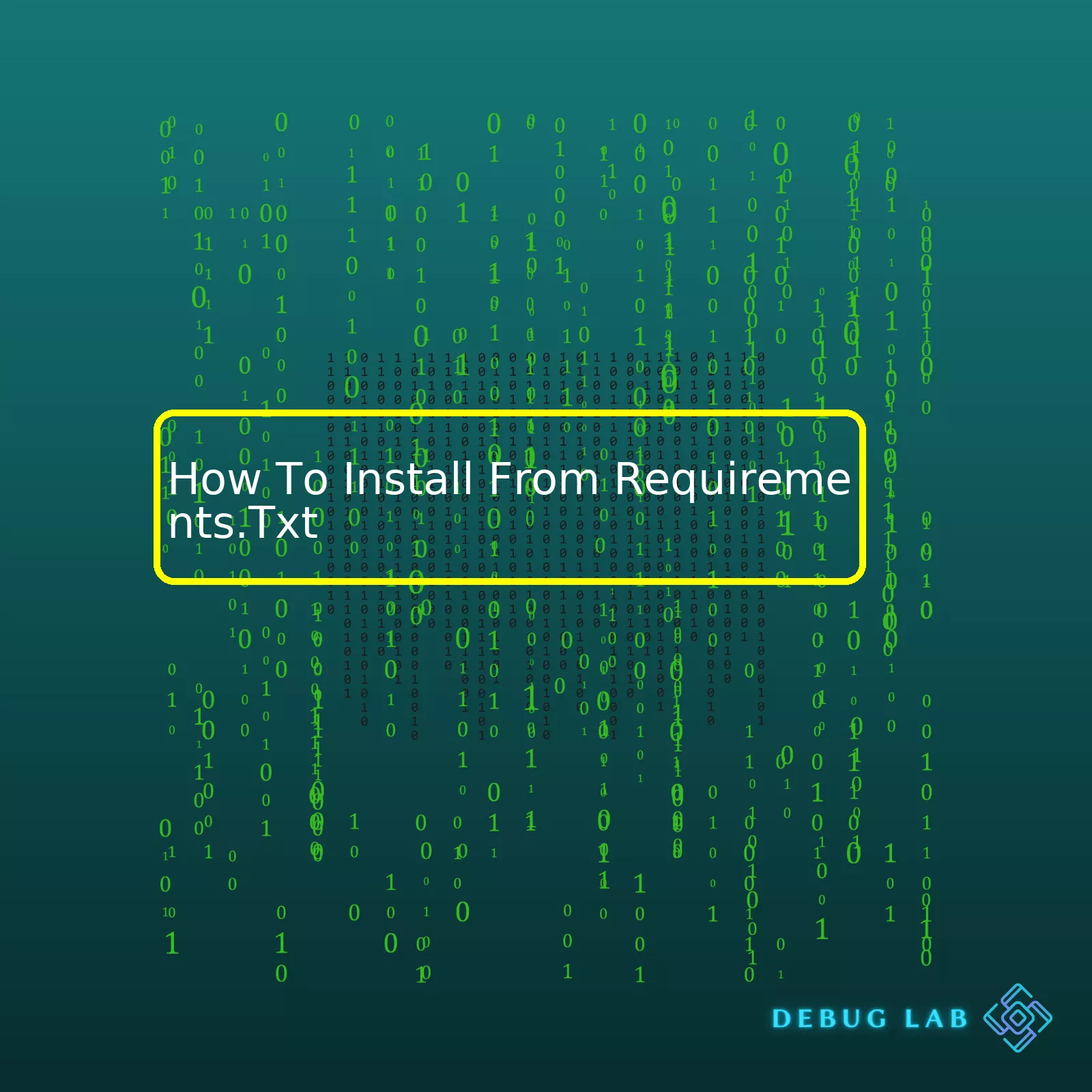
Here’s the summary table:
Steps | Description |
---|---|
Create a Virtual Environment | You should create an isolated environment for your project to avoid conflicts between package dependencies. |
Navigate to your project directory | You must be in the project directory where the requirements.txt is located before executing commands. |
Install Packages from requirements.txt | Run the command
pip install -r requirements.txt to install all the packages listed in the file. |
Now, let’s dive into a more detailed overview of this process:
Creating a virtual environment is crucial as it provides an isolated space for your Python project. This encapsulation prevents interference from other projects on your system and potential conflicts with module versions. You can do this by using the built-in
venv
module in Python3. Execute the command
python3 -m venv /path/to/new/virtual/environment
to create the said environment.
Once you’ve created the environment, navigate to your project directory using the change directory command
cd /path/to/your/project
. It’s necessary to execute all subsequent pip commands in the project’s root directory to ensure everything gets installed correctly.
Lastly, the command
pip install -r requirements.txt
installs the listed packages in bulk, where ‘requirements.txt’ is a file in your project directory listing the Python dependencies needed for your project to run.
The key takeaway here is that the requirements.txt file is a great tool when working with Python applications. Following these steps will help you significantly reduce setup time for most Python projects while also creating consistent workbench conditions. For further reading, you can refer to the official guide provided by Python Packaging User Guide.
The
requirements.txt
is a powerful tool in the Python ecosystem that’s typically placed in the root directory of a Python project. This plain text file is notably easy to read and only lists the dependencies, i.e., the other Python packages that your software needs to run successfully. It frequently includes version numbers so you can ensure compatibility across different systems.
To install these dependencies from the file, your primary focus would be to run pip install with the
-r
option. This will let Python use the pip package installer to install all needed libraries and their specific versions outlined in
requirements.txt
.
Here’s how this process looks like:
pip install -r requirements.txt
With this simple command executed in the command line or terminal, pip will start processing the listed packages. It should be said, however, that the entire process can only run smoothly if your system has already installed Python and the Python package installer (pip).
In the contents of the
requirements.txt
file, you’ll usually find lines enumerating necessary modules and modules’ versions in syntax resembling this:
pandas==1.2.4 numpy==1.21.1 matplotlib==3.4.2
Each line in the
requirements.txt
file corresponds to a particular package and its specific version number right after the ‘==’ symbol. In the example provided, the pandas library required is version 1.2.4, numpy is version 1.21.1, and matplotlib is version 3.4.2.
Versioning is key in ensuring consistency because modules can often update, and new versions might not be compatible with your code. Therefore, listing the precise versions guarantees that everyone working on or using your project has the same software environment.
This practice keeps all users and contributors on the same page regardless of Python updates or newer versions of dependencies. The uniformity achieved through a
requirements.txt
file minimizes chances of unforeseen errors due to inconsistencies among various system environments.
Overall, running
pip install -r requirements.txt
significantly simplifies the distribution and installation of Python software as it automates dependency installation.
Learn more about
requirements.txt
and more details on its usage here on the official pip documentation.
For additional reading regarding how Python packages work, explore RealPython’s comprehensive guide: RealPython Tutorials: Packages.To install packages from a requirements.txt file, you need Python’s package manager pip. This allows developers to specify the exact versions of the Python libraries their project depends on for execution and prevents version conflicts.
The basic syntax of requirements.txt is straightforward:
library==version
Each line represents a library (or module) and its precise version that the Python program will depend on.
For example:
flake8==3.8.4 requests==2.24.0 numpy==1.19.2 pandas==1.1.3
In this case, we have flake8 version 3.8.4, requests version 2.24.0, numpy version 1.19.2, and pandas version 1.1.3 as our dependencies.
To install these with pip, one may simply execute the command:
pip install -r requirements.txt
It means pip is instructed to install (-r), reading from the requirements.txt file. Pip then iterates over each line in requirements.txt, installing the specified module and exact version.
Now let’s consider some additional but important elements of syntax observed in a comprehensive requirements.txt:
Comparative versioning:
Instead of specifying an exact version using
==
, you can specify a minimum (
>=
) or maximum (
<=
) version. Example: Django>=3.0.5
Excluding certain versions:
Use
!=
to establish versions not to use. Example: flask!=0.12.2,!=0.12.3
Specifying a range of versions:
You can state a satisfactory range of versions using comma (equal to AND). Example: numpy>=1.15,<2.0
We must note that pip runs the
setup.py
file if present to identify metadata about the software being installed. For very complex projects, the developer might thus opt to provide this file attached.
Remember that producing a well-organized requirements.txt helps in maintaining your code. It ensures robustness across various environments and expedites seamless teamwork amongst developer communities.
You can also generate a requirements.txt from the current environment by running:
pip freeze > requirements.txt
It outputs all currently installed libraries, permitting others to duplicate your working environment perfectly.
Source: pip documentation.To install Python dependencies from a 'requirements.txt' file, we use pip, the Python package installer. But first things first, ensure that you have properly set up your system. You might wonder "Why do I need to set up the environment? Isn't it just about running a command?" Well, not quite.
Environment setup is crucial because it ensures that dependencies of your project are isolated from each other, meaning that different projects can work with different versions of packages without conflicts. Let's discuss how to set up our system before proceeding to installing dependencies from 'requirements.txt'.
Set Up Virtual Environment
Firstly, it's important to install virtualenv, which is a tool to create isolated Python environments. If you already have pip (Python Package Index), just run this command:
pip install virtualenv
Next, you want to create a new virtual environment. Navigate into the directory where you want the environment to live and run:
virtualenv venv
This will create a new folder named 'venv' in your current directory. This 'venv' directory should not be sent to the server with your code, so add it to .gitignore.
To activate this environment, use this command on Linux/macOS:
source venv/bin/activate
And on Windows:
venv\Scripts\activate
Once your virtual environment is activated, your terminal will now show the name of your activated environment on the side of the line where you type commands.
Installation From Requirements.Txt
Now that you're inside your virtual environment, all the packages you install will be specific to the project you're working on - an amazing way to keep your projects tidy!
It's time to install those dependencies. You can do this easily with the following command:
pip install -r requirements.txt
What this does is tell pip to install (-r) the packages listed in the provided .txt file. This .txt file (in our case, requirements.txt) contains a list of items to be installed using pip.
It's important to note that the 'requirements.txt' is usually present in the root of the project. It lists all the python packages that your application depends on. These could include 'Django' for web applications or 'numpy' for data processing, among others.
You can find a real-world example at Django's GitHub Repository.Link
Here's a sample 'requirements.txt':
Django==3.0.5 djangorestframework==3.11.0 pytz==2020.1 sqlparse==0.3.1
Each line specifies a package and its version number, which is important to ensure system stability.
So, if you have made sure that your environment is properly set up and isolated and the 'requirements.txt' is well-prepared, dependency installation becomes a smooth and manageable process.At times, when dealing with Python projects, you'll notice a file named
requirements.txt
. This is a plain text file where developers store the names of Python package dependencies so that other developers can install all required packages at once instead of individually. Let's look at how to do it.
What You'll Need
Not much is required: just Python installed on your system (which includes pip - a Python package manager) and the
requirements.txt
file in question.
Locating the Requirements.Txt File
The very first step when installing from a
requirements.txt
file is locating it within your project directory. For instance:
/home/user/myproject/requirements.txt
Keep note of the path as we'll use this shortly.
How to Install From Requirements.Txt
To execute commands, you'll need to navigate to your command-line interface or terminal. Here's the code you must enter:
pip install -r /path/to/requirements.txt
Just replace
/path/to/requirements.txt
with your actual location. This tells pip to refer (-r) to your requirements.txt file and install all packages stated within it.
What happens here is pretty straightforward – pip downloads each package mentioned in your
requirements.txt
, looks for the latest version compatible with your Python environment, and installs them.
If there are specific versions of a package that your project needs, they typically are included in the
requirements.txt
file such as below:
Django==3.0.7 djangorestframework==3.11.0 PyJWT==1.7.1
In such cases, pip will only install specified versions of these packages irrespective of whether newer versions are available.
Error Handling While Installation
You might encounter a few errors during installation of which version conflict is quite common. If your environment has a different version of a package than what’s specified in
requirements.txt
, pip will throw an error. To fix this issue, you can upgrade or downgrade your existing package to the required version, or use different Python virtual environments for different projects.
Hopefully, the entire process has been broken down into simple steps that anyone can follow to understand how to install dependencies from a
requirements.txt
file. This standard practice keeps Python projects organised, standardizes the development environment across multiple setups and reduces dependency conflicts. A complete guide to pip and requirements.txt can be found at the official pip documentation.
Troubleshooting common issues during installation from requirements.txt falls in line with the proper comprehension of how to install dependencies from a requirements.txt file in your Python project. As we continue further, you'll witness how identifying and solving problems while installing these libraries can be cleared using some quick tricks.
Perhaps the most straightforward way to install dependencies for your Python project is by using the pip install command along with the path to your requirements.txt file which contains the list of libraries needed.
pip install -r /path/to/requirements.txt
Here's a closer look at some common errors and their respective solutions:
Error 1: Version of Python
It might happen sometimes that despite having the correct list in your requirements.txt file, libraries may not install properly due to an incompatible version of Python. In such a case, updating or degrading Python may solve this issue. An analytical approach here would include checking the versions of Python compatible with each library that fails to install, and subsequently updating or downgrading Python accordingly.
Error 2: Virtual environment conflict
The Python packages installed globally on your local system could conflict with those mentioned in your requirements.txt file. Often, this is because different projects require different versions of the same package. A virtual environment can isolate dependencies required by your project only, preventing such conflicts. A solution to this would involve creating a new virtual environment and attempting the installation procedure within it.
Here's how you create a virtual environment called 'venv' and then activate it:
For Unix or MacOS:
python3 -m venv /path/to/new/virtual/environment source env/bin/activate
And for Windows:
py -m venv \path\to\new\virtual\environment .\env\Scripts\activate
Error 3: Syntax error in requirements.txt
If there is a syntax error in the requirements.txt file, installation will fail. The requirements.txt file should be written in the format
Error 4: Dependency conflict
Sometimes, two libraries you're trying to install from the requirements.txt file could depend on different versions of a third library. This dependency conflict causes an installation failure. To resolve this, investigate what's causing the clash and look into potentially replacing one of the clashing libraries or finding a version of the conflicting library that works well for all parties involved.
For programming-related queries, StackOverflowStackOverflow serves as a fantastic forum, where there's a high possibility of someone having faced a similar issue. Plenty of open-source Python projects on GitHub also provide decent guidance on troubleshooting issues. Do use their valuable search feature before diving deep into debugging problematic installs.
Overall, addressing problems associated with the installation process from requirements.txt involves careful analysis of the type of error, the Python version, syntax correctness, dependency clashes and managing library versions effectively. Doing so effectively ensures an easier and smoother project setup.Of course! There are numerous reasons why it's essential to periodically update and edit your
requirements.txt
file for your Python project.
The
requirements.txt
file in a Python application is a text file that lists all the modules needed by the project. These include both custom-built libraries and third-party ones uploaded on PyPI (Python Package Index). This file is vital to ensure repeatability and consistency across different environments and installations, whether you're sharing the code with fellow developers or deploying it in a production environment.
Updating Your Requirements File:
From time to time, developers improve these packages, adding new features, fixing bugs, and enhancing performance. Failing to update dependencies can lead to outdated functionalities, slower operations, and potential security vulnerabilities. Therefore, updating the
requirements.txt
file from time to time is an important task.
To update a package mentioned in your
requirements.txt
, use the following command:
pip install --upgrade PackageName
After upgrading a package, ensure that this change is reflected in your
requirements.txt
. You can freeze the current state of your packages using:
pip freeze > requirements.txt
This will write the updated list of packages along withtheir versions into your
requirements.txt
file.
Editing Your Requirements File:
If you have added or removed a dependency during the development cycle, it becomes necessary to edit your
requirements.txt
file accordingly. This ensures that others replicating your project setup will also include or exclude this package. You can manually add or remove the packages from the file, but a better approach is to let pip handle this process.
When you add a new package for your project, immediately freeze your environment:
pip freeze > requirements.txt
Same way, if you remove a dependency, after uninstalling it run the above command again to update
requirements.txt
.
Installing from Your Requirements File:
For developers cloning your repository or when setting up your project in a new environment, they can easily install all required packages mentioned in
requirements.txt
file using the following command:
pip install -r requirements.txt
This ease of replicating the exact environment, irrespective of operating systems, makes the
requirements.txt
file an essential component of any Python project.
Remember, creating, editing and managing this
requirements.txt
file properly reflects a developer's ability to create reusable, robust, and reliable software. That's the essence of good programming practices!Sure, one important aspect of working with Python projects is managing dependencies. In Python development, a common tool for this practice is the `requirements.txt` file.
Installing from requirements.txt:
After having your Python environment set up, you can easily install the dependencies of your project inscribed in the `requirements.txt` file. The command to execute this procedure in your terminal or command prompt is:
pip install -r requirements.txt
The pip user guide gives more detailed instructions and options.
This command tells pip (Python's package installer) to look at `requirements.txt`, and for every library listed, it does a lookup and installation.
Best Practices for Using requirements.txt:
Knowing how to use `requirements.txt` effectively is important:
• Simplicity and readability count: Just like your Python code, your requirements file should be easy to read. Use comments that start with the hash mark (#) to explain why you’re including or pinning a certain package.
• Keep precise track of packages: For each Python library you use in your project, ensure its addition to the list in `requirements.txt`.
• Pin your packages: Pinning your packages means specifying their version. This is a good practice to prevent unwanted updates that might break your project compatibility. Example:
django==3.0.5
• Use multiple requirement files if necessary: Splitting the list into multiple files such as `requirements_dev.txt` for development specific libraries or `requirements_test.txt` for testing can maintain clear distinction among use-cases.
You can refer to "A Beginner’s Guide to pip" published by Real Python for further insights.
Consider the following example where I've pinned the versions of two packages to illustrate the format of a basic `requirements.txt`:
Django==3.0.5 djangorestframework==3.11.0
These are some popular and sensible practices around the `requirements.txt` mechanism. Understanding these practices will definitely give you an edge when dealing with Python projects.Let's wind up by highlighting the fundamental steps to install from requirements.txt. Essentially, this is a procedural technique that primarily depends on the Python package manager called pip. Essentially, pip does an exceptional job in smoothly automating the process.
Firstly, you need to ensure that both Python and Pip are installed and well set-up. Subsequently, navigate to the directory containing the requirements.txt file using your system command line tool. Run the command
pip install -r requirements.txt
. This implies Pip to install the versions of each library as indicated in the text file.
Take note:
* If any of the required packages fail to install, a clear error message will be presented signifying this so that you can handle it appropriately.
* However, if all goes well, all Python libraries listed in your requirements.txt file should be successfully installed and ready for usage.
But do remember that the particular syntax and commands might vary across different operating systems or cloud/fog environments. In case of an error, recheck the mentioned Python and Pip versions in your text file, and compare against your setup, then rectify if necessary.
Probability of getting errors while installing can also lessen hinting that you periodically keep updating the Python and Pip to their latest versions. To couple with this, write cleaner and executable codes which reduce the complexity and number of dependencies being used.
If a third-party package needed for your project isn't available in the default Python Package Index (PyPI), utilize the PyPI repository. And cleverly manage such external dependencies using Pipenv or custom solution.
Here's a brief code snippet outlining the installation from requirements.txt:
# Assuming the terminal command line is pointing to your project directory pip install -r requirements.txt
In instances whereby there's a need to create the requirements.txt file, you simply need to run
pip freeze > requirements.txt
.
Overall, understanding how to use requirements.txt in Python development makes workflow more efficient and saves a lot of time when setting up new environments or collaborating with other developers. It ensures that multiple parties are working within the same software context thus minimizing the "it works on my machine" syndrome.