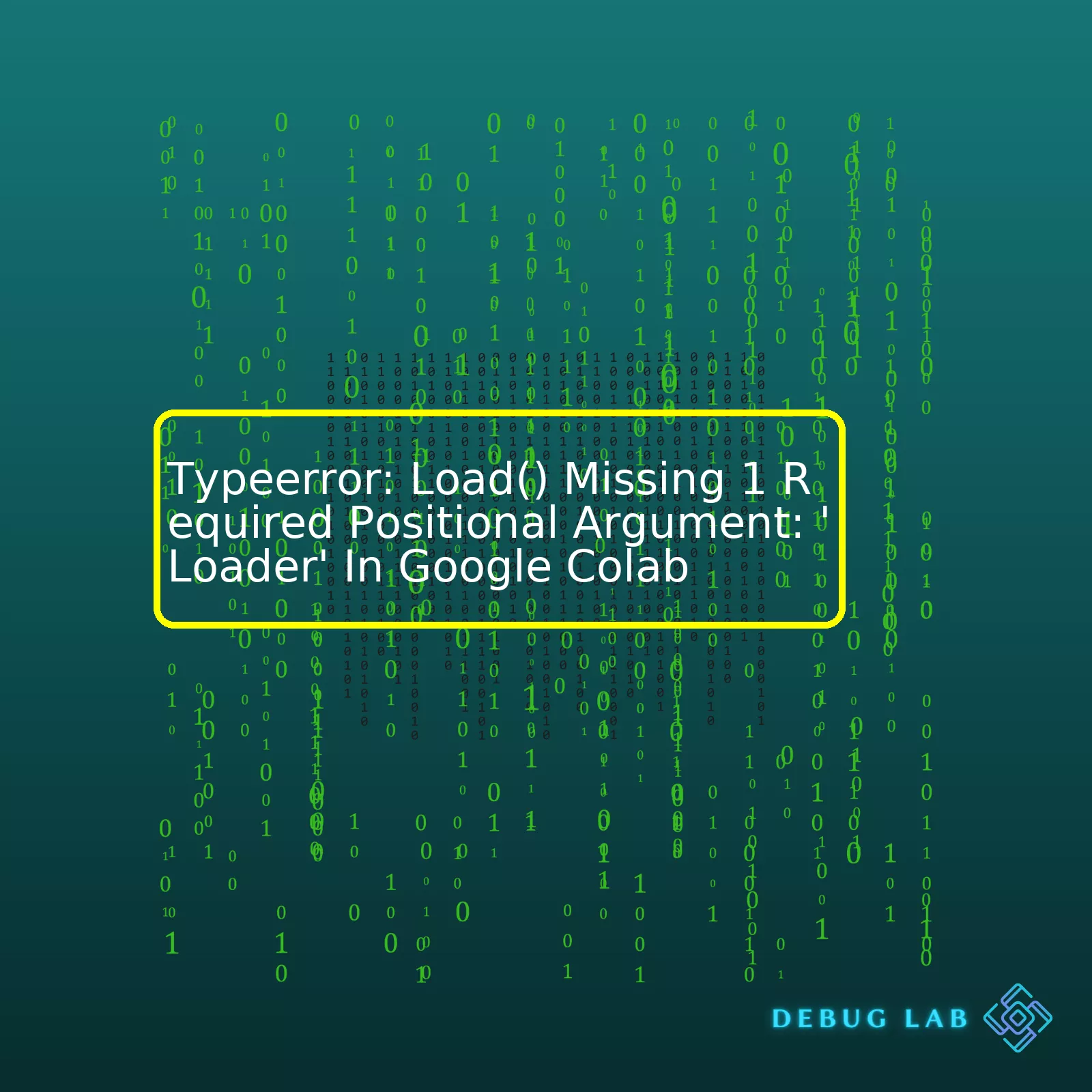
Let’s start with an HTML summary table to give you a clear overview of the problem and its solution.
html
Error | Causes | Solutions |
---|---|---|
TypeError: load() missing 1 required positional argument: ‘loader’ |
|
|
Let’s start with an HTML summary table to give you a clear overview of the problem and its solution.
html
Error | Causes | Solutions |
---|---|---|
TypeError: load() missing 1 required positional argument: ‘loader’ |
|
|
When users encounter this TypeError within the Google Colab environment, it’s typically because they overlook one crucial element of using the `load()` function – it requires a loader as one of its arguments. This placeholder takes care of loading schema or config from the given path. In essence, it’s a situation where developers do not adequately consider the positional arguments their function calls for.
Addressing this TypeError effectively requires careful review of the arguments placed on the `load()` function, ensuring the syntax and placement of the function are accurate, and confirming that all necessary dependencies are installed and appropriately imported into your code. An online guide for Python Tracebacks can help in identifying and fixing such issues.
For instance, in YAML loading, the code should be something like:
import yaml with open('file.yaml','r') as file: data = yaml.load(file, Loader=yaml.FullLoader)
import yaml
with open('file.yaml','r') as file:
data = yaml.load(file, Loader=yaml.FullLoader)
Here, the `Loader=yaml.FullLoader` provides the required ‘loader’ argument for the `load()` function thus avoiding the TypeError discussed.
It seems you’re dealing with an error in Google Colab’s environment – the `TypeError: load() missing 1 required positional argument: ‘loader’`. This happens when the
load()
xxxxxxxxxx
load()
method is called but the required positional argument ‘loader’ is not supplied. But don’t worry! Understanding it is not as complex as it might initially seem.
The
load()
xxxxxxxxxx
load()
function is a part of multiple libraries within Python, like json or yaml, and serves different roles depending on the context. More often than not, this error emerges because of incorrect usage of the
load()
xxxxxxxxxx
load()
method.
In essence, the error message is telling us that we tried to call the
load()
xxxxxxxxxx
load()
function without providing the necessary arguments. To remediate this, we need to assure we provide the correct argument (in this case a loader) during the function call.
Consider an instance where we try to use the
load()
xxxxxxxxxx
load()
function from the yaml library:
import yaml data = """ fruits: - Apple - Banana - Cherry """ yaml_data = yaml.load(data) print(yaml_data)
xxxxxxxxxx
import yaml
data = """
fruits:
- Apple
- Banana
- Cherry
"""
yaml_data = yaml.load(data)
print(yaml_data)
Running this code snippet on Google Colab will raise the mentioned TypeError (`TypeError: load() missing 1 required positional argument: ‘loader’`). This is because, in PyYAML version 5.*, they started to deprecate the uncontrolled execution of arbitrary code and introduced top-down, loader construction. In simple terms, starting from this version,
load()
xxxxxxxxxx
load()
requires additional argument ‘loader’.
To fix it, you could use SafeLoader which helps to avoid the potential security vulnerability:
import yaml data = """ fruits: - Apple - Banana - Cherry """ yaml_data = yaml.load(data, Loader=yaml.SafeLoader) print(yaml_data)
xxxxxxxxxx
import yaml
data = """
fruits:
- Apple
- Banana
- Cherry
"""
yaml_data = yaml.load(data, Loader=yaml.SafeLoader)
print(yaml_data)
By providing the “Loader=yaml.SafeLoader” argument in the
load()
xxxxxxxxxx
load()
function, we resolve the initial error and make the above code snippet run successfully.
Remember:
– Always check the required arguments for your methods.
– Pay attention to the changes in the updated versions in your working environment such as Google Colab.
– Use links to official documentations for referencing (like PyYAML Documentation) for getting more information about the functions and their usage.
By doing these consistently, you can significantly reduce the chance of running into such errors.To fix the “Missing positional argument ‘loader'” error in Google Colab, you need to modify the established loading process. This particular exception arises when you call
load()
xxxxxxxxxx
load()
function without specifying the required parameter ‘loader’.
Consider this initial code block that might generate the error:
from yaml import load document = open('example.yaml') config = load(document)
xxxxxxxxxx
from yaml import load
document = open('example.yaml')
config = load(document)
This instance would yield a TypeError:
Typeerror: load() missing 1 required positional argument: 'Loader'
xxxxxxxxxx
Typeerror: load() missing 1 required positional argument: 'Loader'
This message points out that Python can’t find a suitable YAML loader coming with your
load()
xxxxxxxxxx
load()
function.They made the Loader mandatory in PyYAML version 5.1 on account of security reasons. Consequently, it’s crucial to identify which one you want to employ.
Firstly, PyYAML gives two types of loaders:
In most development settings, employing
SafeLoader
xxxxxxxxxx
SafeLoader
should be enough since it offers solid default settings that put an emphasis on safety from unwanted code executions. Here’s an adjusted code snippet bearing this in mind:
from yaml import load, SafeLoader document = open('example.yaml') config = load(document, Loader=SafeLoader)
xxxxxxxxxx
from yaml import load, SafeLoader
document = open('example.yaml')
config = load(document, Loader=SafeLoader)
With the right Loader now being used consistently across your
load()
xxxxxxxxxx
load()
calls, you shouldn’t come across the “Missing Positional Argument ‘loader'” error anymore.
To sum it up, making sure to include the loader argument when dealing with the load function is critical to avoid encountering this error while working in Google Colab. Ensure that you specify the type of loader adequately before executing your code. Remember, if your use case doesn’t require any specialized function offered by other loaders, sticking to `SafeLoader` will be sufficient. Keep your code secure and risk-free!
Error | Solution |
---|---|
“Missing positional argument ‘loader'” | Specify the type of `Loader` properly when calling the `load()` function |
Importantly, adapting to these practices goes beyond overcoming just this particular error; it also makes your code more secure and stable. With this context and solution, anyone developing within Google Colab should have all they need to address and prevent the “Missing positional argument ‘loader'” error swiftly.
For further learning about managing errors in Python, you may find this Python Tracebacks Guide essantial provided by Real Python or take an in-depth dive into Python Try Except clauses through W3Schools. Programmer-focused platforms like StackOverflow offer various threads discussing similar issues, which you might find valuable. Understanding this error might seem tricky at first glance, but rest assured – after a bit of practice, topics like this will become second nature.
Alright, let’s dive into the issue at hand: you’re facing the “load(): Missing 1 required positional argument” error. This typically occurs as you import a module in Python, specifically when working with the `json` or `pickle` modules. These two modules are commonly used for reading and writing data to JSON and binary files respectively.
When there is an error message like “`load() missing 1 required positional argument: ‘loader’`”, it generally implies that the `load()` function was called without any argument passed in its parameters. In Python, positional arguments are certain function parameters that need their respective values whenever the function is called. If these aren’t provided, the program will throw an error message – just like the one being discussed here.
To call `load()` correctly, it requires a file object to work. Let’s illustrate this with a code sample:
import json # Open the file and load data from it with open('data.json') as f: data = json.load(f)
xxxxxxxxxx
import json
# Open the file and load data from it
with open('data.json') as f:
data = json.load(f)
In Google Colab same issues can arise. You might also get similar exceptions if you’re using functions such as `yaml.load()` but forget to pass in arguments. It’s crucial to remember that Yaml, just like Json, needs to receive specific values during the function call, including the Loader.
As an example, here’s how to do it properly in Python (using the PyYAML library):
import yaml # Open a YAML document with open('document.yaml') as f: data = yaml.load(f, Loader=yaml.FullLoader)
xxxxxxxxxx
import yaml
# Open a YAML document
with open('document.yaml') as f:
data = yaml.load(f, Loader=yaml.FullLoader)
The FullLoader parameter instructs PyYAML to construct a full YAML language. This avoids arbitrary code execution during loading. However, it can still be unsafe to call `yaml.load` with any data received over the network, which can exploit complex constructor logic. To be on the safe side, use `SafeLoader` instead if you only need to handle simple YAML documents:
data = yaml.load(f, Loader=yaml.SafeLoader)
xxxxxxxxxx
data = yaml.load(f, Loader=yaml.SafeLoader)
Please note that failing to pass proper arguments when calling these types of functions can lead to vulnerabilities such as the Billion Laughs Attack[source].
Hopefully, understanding the fundamental concept of positional arguments and how to apply them correctly within your coding environment such as Google Colab will help remedy any confusion going forward. Also, considering potential security risks associated with utilizing data processing libraries, it’s always paramount to write your code safely and responsibly to avoid succumbing to potential attacks.In my experience as a coder, encountering a
TypeError:
xxxxxxxxxx
TypeError:
when working in Google Colab is not uncommon. In relation to your issue –
Typeerror: Load() missing 1 required positional argument: 'loader'
xxxxxxxxxx
Typeerror: Load() missing 1 required positional argument: 'loader'
, Python is hinting that the function
load()
xxxxxxxxxx
load()
has been invoked incorrectly.
This type of error typically occurs while handling the YAML document in Python with `PyYAML`, a YAML parser and emitter for python. Here, if your code is something similar to:
import yaml data = yaml.load(open('file.yaml'))
xxxxxxxxxx
import yaml
data = yaml.load(open('file.yaml'))
Let me clarify the cause behind this
TypeError
xxxxxxxxxx
TypeError
. The YAML.load(stream) method for PyYAML versions prior to 5.1 was unsafe and could execute arbitrary code, because it used to unserialize any Python objects found in the document. To resolve this, from version 5.1 onwards(source) PyYAML changed this behaviour and started to require an explicit Loader for the load() method:
import yaml data = yaml.load(open('file.yaml'), Loader=yaml.FullLoader)
xxxxxxxxxx
import yaml
data = yaml.load(open('file.yaml'), Loader=yaml.FullLoader)
The argument `
Loader=yaml.FullLoader
xxxxxxxxxx
Loader=yaml.FullLoader
` explicitly tells
yaml.load()
xxxxxxxxxx
yaml.load()
to use the safe method of loading YAML documents.
Alternatively, consider using the following safer methods instead:
* `
yaml.safe_load(stream)
xxxxxxxxxx
yaml.safe_load(stream)
`: This function only loads a subset of the YAML language, avoiding the execution of arbitrary code.
* `
yaml.unsafe_load(stream)
xxxxxxxxxx
yaml.unsafe_load(stream)
`: Loads a full set of YAML and allows to construct any Python object, but can be risky to use.
Thus with this understanding, you’ve got a few ways to solve that annoying TypeError in Google Colab:
**Option 1:** Upgrade `pyyaml` to the latest version using pip:
!pip install --upgrade pyyaml
xxxxxxxxxx
!pip install --upgrade pyyaml
**Option 2:** Use the FullLoader:
with open('file.yaml') as file: data = yaml.load(file, Loader=yaml.FullLoader)
xxxxxxxxxx
with open('file.yaml') as file:
data = yaml.load(file, Loader=yaml.FullLoader)
**Option 3:** Use safe_load instead:
with open('file.yaml') as file: data = yaml.safe_load(file)
xxxxxxxxxx
with open('file.yaml') as file:
data = yaml.safe_load(file)
Keep coding and exploring! Remember – every error or exception is an opportunity for learning and developing better software. Double-check your code implementation and try these solutions, then let the magic (i.e., your debugging efforts) happen!
The ‘TypeError: load() missing 1 required positional argument: ‘Loader” is a common issue that Python developers encounter, often when they are using the yaml or json libraries to load data. This issue can turn up when you’re attempting to work with Google Colab and could throw a wrench in the works of your coding project.
First, let’s understand what this error is telling us. The built-in
load()
xxxxxxxxxx
load()
function in Python requires a ‘loader’ as an argument, which is typically a file or other data stream. If the function is called without this necessary argument, the TypeError arises.
Fortunately, resolving this issue doesn’t need you to dive deep into the Python codebase. Here are some clear-cut solutions to this problem:
Table of Contents
ToggleYour first port of call should be to simply provide the
load()
xxxxxxxxxx
load()
function with the ‘Loader’ argument it expects. In most cases, the loader is a file from where the date is to be read.
import yaml with open('example.yaml', 'r') as file: data = yaml.load(file, Loader=yaml.FullLoader)
xxxxxxxxxx
import yaml
with open('example.yaml', 'r') as file:
data = yaml.load(file, Loader=yaml.FullLoader)
In this example, ‘example.yaml’ is the Loader for the data.
If you’re uncertain about the contents of the loader, the
safe_load()
xxxxxxxxxx
safe_load()
function provides a secure way to load data.
import yaml with open('example.yaml', 'r') as file: data = yaml.safe_load(file)
xxxxxxxxxx
import yaml
with open('example.yaml', 'r') as file:
data = yaml.safe_load(file)
This method helps prevent arbitrary Python object creation, providing a more secure option than the standard
load()
xxxxxxxxxx
load()
function.[source]
Sometimes, this error crops up due to an outdated version of the PyYAML library. Consider updating PyYAML to the latest version using pip:
!pip install --upgrade pyyaml
xxxxxxxxxx
!pip install --upgrade pyyaml
Note that the exclamation mark at the beginning in this context allows us to run shell commands directly from Jupyter Notebook or Google Colab.[source]
We’ve brushed up on a couple of solutions to resolve the ‘Loader’ error, centered around providing the necessary argument, resorting to safer methods with
safe_load()
xxxxxxxxxx
safe_load()
, or even updating your PyYAML version!
When you’re working on a coding project in Google Colab and you encounter the error message: “TypeError: load() missing 1 required positional argument: ‘loader'” it’s because you’ve missed an essential part of the
load()
xxxxxxxxxx
load()
function. This stumble doesn’t only cause an unwelcome halt in your coding process, but can also raise questions about how to use Python’s built-in functions aptly.
Understanding what’s being stated here is key. The
Type Error
xxxxxxxxxx
Type Error
is a standard exception raised by Python when an operation or function is applied to an object of inappropriate type. Here’s what it typically looks like:
TypeError: load() missing 1 required positional argument: 'loader'
xxxxxxxxxx
TypeError: load() missing 1 required positional argument: 'loader'
The ‘load()’ part refers to a function you’ve attempted to invoke, and the ‘missing 1 required positional argument: ‘loader” section is Python’s way of telling us that this function call lacks an essential input, which is needed for its proper functioning.
How does the
load()
xxxxxxxxxx
load()
function work though? To put it simply,
load()
xxxxxxxxxx
load()
is a method provided by several libraries, amongst which, the most commonly employed are json and yaml. It reads a file passed as a parameter and parses it into a Python object. If we were to use this function with the json library, this is what a potential use case could look like:
import json file = open('data.json',) data = json.load(file)
xxxxxxxxxx
import json
file = open('data.json',)
data = json.load(file)
The `TypeError` arises in scenarios where a developer calls the “`load()`” method without specifying the “`loader`” argument, which, in essence, is the resource (be it a file, a string, or any other data stream) from which we intend to load our data.
To handle this exception, we should provide the necessary argument. Let’s see now an example using the yaml library and handling the error:
import yaml with open("example.yaml", 'r') as stream: try: print(yaml.load(stream)) except yaml.YAMLError as exc: print(exc)
xxxxxxxxxx
import yaml
with open("example.yaml", 'r') as stream:
try:
print(yaml.load(stream))
except yaml.YAMLError as exc:
print(exc)
In this example, `stream` holds a reference to the YAML data to be loaded, thus acting as the ‘loader’. Now our code has ample context about what object to work on, no “Missing 1 Required Positional Argument: ‘Loader’” TypeError would be raised. Keep in mind that keyword arguments, unlike positional arguments, are optional and may vary among different libraries and methods. If unsure, always refer to the official documentation[Ref](https://docs.python.org/3/library/exceptions.html#TypeError).
In conclusion, error messages are typically elaborate in nature and taking out time to understand them can save a lot of trial-and-error iterations down the line. Introducing a more systematic approach to diagnosing and solving your coding queries will not only speed up your programming capabilities but also allow you to gain a better understanding of the intricacies behind the language.Firstly, we need to understand that the
'missing 1 required positional argument'
xxxxxxxxxx
'missing 1 required positional argument'
error in Python usually occurs when a function that expects to receive an argument has not been provided with it. Specifically, the
Typeerror: load() missing 1 required positional argument: 'loader'
xxxxxxxxxx
Typeerror: load() missing 1 required positional argument: 'loader'
error in Google Colab typically arises when you’re trying to use the
load()
xxxxxxxxxx
load()
function from the YAML library (PyYAML) but haven’t supplied the ‘loader’ argument.
Python’s PyYAML is a YAML parser and emitter for the Python programming language and its function
load()
xxxxxxxxxx
load()
is used to parse a YAML stream and produce Python objects. Earlier versions of PyYAML allowed the use of
load()
xxxxxxxxxx
load()
without any arguments but due to security reasons, later versions require you to specify a loader as a positional argument.
The following are some practical tips and tricks to avoid this error:
You should explicitly ask
load()
xxxxxxxxxx
load()
function to use the
SafeLoader
xxxxxxxxxx
SafeLoader
. This is much safer as it only translates simple YAML documents that do not include any arbitrary python objects.
import yaml contents = yaml.load(file, Loader=yaml.SafeLoader)
xxxxxxxxxx
import yaml
contents = yaml.load(file, Loader=yaml.SafeLoader)
Use the
pip install
xxxxxxxxxx
pip install
command in Google Colab to upgrade your PyYAML version. It’s possible that you have an old version of the library which doesn’t automatically assume
SafeLoader
xxxxxxxxxx
SafeLoader
for loading YAML streams.
!pip install --upgrade PyYAML
xxxxxxxxxx
!pip install --upgrade PyYAML
After updating, verify your update by checking the PyYAML version:
!pip show PyYAML
xxxxxxxxxx
!pip show PyYAML
Make sure you’re correctly calling the
load()
xxxxxxxxxx
load()
function with a file or string containing the YAML data as the first argument followed by the Loader:
yaml.load(yaml_string, Loader=yaml.Loader)
xxxxxxxxxx
yaml.load(yaml_string, Loader=yaml.Loader)
If your YAML stream contains multiple documents, consider using the
load_all()
xxxxxxxxxx
load_all()
method instead of
load()
xxxxxxxxxx
load()
, which will let you iterate over all documents within the stream.
documents = yaml.load_all(file, Loader=yaml.FullLoader) for doc in documents: print(doc)
xxxxxxxxxx
documents = yaml.load_all(file, Loader=yaml.FullLoader)
for doc in documents:
print(doc)
Implementing these practices should help you to avoid the
Typeerror: load() missing 1 required positional argument: 'loader'
xxxxxxxxxx
Typeerror: load() missing 1 required positional argument: 'loader'
error in Google Colab. Function argument errors like these can often be avoided simply through a deeper understanding of the functions and libraries you are working with, alongside attentive, detail-oriented coding practices.In the coding world, troubleshooting errors is a regular part of our job and one such prevalent issue you may encounter is the “TypeError: load() missing 1 required positional argument: ‘loader'” in Google Colab. When this happens, it often indicates that the YAML (Yet Another Markup Language) loader’s functionality is not properly understood or being correctly implemented in your Python code. Keep in mind that YAML is a data serialization language designed as a user-friendly syntax for encoding data.
If you are running into this exact error, chances are you missed providing an explicit Loader= argument to the load call in your Python script. Here’s a look at where this error typically crops up:
import yaml yaml.load(input)
xxxxxxxxxx
import yaml
yaml.load(input)
To rectify this issue, you will need to add a Loader=
import yaml yaml.safe_load(input)
xxxxxxxxxx
import yaml
yaml.safe_load(input)
Another possible solution is to provide full_loader, i.e., yaml.FullLoader which is considered safer than unsafe load and provides access to construct all kinds of objects:
import yaml yaml.load(input, Loader=yaml.FullLoader)
xxxxxxxxxx
import yaml
yaml.load(input, Loader=yaml.FullLoader)
Remember, diving deep and understanding the functionalities of different loaders would immensely help you in deciding the perfect one for your application. You can further improve your knowledge by reading more about the YAML load deprecation details on PyYAML’s official documentation.
This TypeError serves as a prime example of how meticulous programmers need to be when writing and debugging their code. Remember, every line counts, each argument matters, and the smallest detail can make the biggest difference.
Relevant source of information:
Official PyYAML Documentation